-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
element. - // The code flag tells whether this block surrounds - // highlighted code. This will be false when surrounding - // line numbers. - Start(code bool, styleAttr string) string - - // End is called to write the endelement. - End(code bool) string -} - -type preWrapper struct { - start func(code bool, styleAttr string) string - end func(code bool) string -} - -func (p preWrapper) Start(code bool, styleAttr string) string { - return p.start(code, styleAttr) -} - -func (p preWrapper) End(code bool) string { - return p.end(code) -} - -var ( - nopPreWrapper = preWrapper{ - start: func(code bool, styleAttr string) string { return "" }, - end: func(code bool) string { return "" }, - } - defaultPreWrapper = preWrapper{ - start: func(code bool, styleAttr string) string { - return fmt.Sprintf(`
`, styleAttr) - }, - end: func(code bool) string { - return "" - }, - } -) - -// Formatter that generates HTML. -type Formatter struct { - standalone bool - prefix string - Classes bool // Exported field to detect when classes are being used - allClasses bool - preWrapper PreWrapper - tabWidth int - lineNumbers bool - lineNumbersInTable bool - linkableLineNumbers bool - lineNumbersIDPrefix string - highlightRanges highlightRanges - baseLineNumber int -} - -type highlightRanges [][2]int - -func (h highlightRanges) Len() int { return len(h) } -func (h highlightRanges) Swap(i, j int) { h[i], h[j] = h[j], h[i] } -func (h highlightRanges) Less(i, j int) bool { return h[i][0] < h[j][0] } - -func (f *Formatter) Format(w io.Writer, style *chroma.Style, iterator chroma.Iterator) (err error) { - return f.writeHTML(w, style, iterator.Tokens()) -} - -// We deliberately don't use html/template here because it is two orders of magnitude slower (benchmarked). -// -// OTOH we need to be super careful about correct escaping... -func (f *Formatter) writeHTML(w io.Writer, style *chroma.Style, tokens []chroma.Token) (err error) { // nolint: gocyclo - css := f.styleToCSS(style) - if !f.Classes { - for t, style := range css { - css[t] = compressStyle(style) - } - } - if f.standalone { - fmt.Fprint(w, "\n") - if f.Classes { - fmt.Fprint(w, "") - } - fmt.Fprintf(w, "\n", f.styleAttr(css, chroma.Background)) - } - - wrapInTable := f.lineNumbers && f.lineNumbersInTable - - lines := chroma.SplitTokensIntoLines(tokens) - lineDigits := len(fmt.Sprintf("%d", f.baseLineNumber+len(lines)-1)) - highlightIndex := 0 - - if wrapInTable { - // List line numbers in its own
\n", f.styleAttr(css, chroma.LineTableTD)) - fmt.Fprintf(w, f.preWrapper.Start(false, f.styleAttr(css, chroma.Background))) - for index := range lines { - line := f.baseLineNumber + index - highlight, next := f.shouldHighlight(highlightIndex, line) - if next { - highlightIndex++ - } - if highlight { - fmt.Fprintf(w, "", f.styleAttr(css, chroma.LineHighlight)) - } - - fmt.Fprintf(w, "%s\n", f.styleAttr(css, chroma.LineNumbersTable), f.lineIDAttribute(line), f.lineTitleWithLinkIfNeeded(lineDigits, line)) - - if highlight { - fmt.Fprintf(w, "") - } - } - fmt.Fprint(w, f.preWrapper.End(false)) - fmt.Fprint(w, " | \n") - fmt.Fprintf(w, "\n", f.styleAttr(css, chroma.LineTableTD, "width:100%")) - } - - fmt.Fprintf(w, f.preWrapper.Start(true, f.styleAttr(css, chroma.Background))) - - highlightIndex = 0 - for index, tokens := range lines { - // 1-based line number. - line := f.baseLineNumber + index - highlight, next := f.shouldHighlight(highlightIndex, line) - if next { - highlightIndex++ - } - if highlight { - fmt.Fprintf(w, "", f.styleAttr(css, chroma.LineHighlight)) - } - - if f.lineNumbers && !wrapInTable { - fmt.Fprintf(w, "%s", f.styleAttr(css, chroma.LineNumbers), f.lineIDAttribute(line), f.lineTitleWithLinkIfNeeded(lineDigits, line)) - } - - for _, token := range tokens { - html := html.EscapeString(token.String()) - attr := f.styleAttr(css, token.Type) - if attr != "" { - html = fmt.Sprintf("%s", attr, html) - } - fmt.Fprint(w, html) - } - if highlight { - fmt.Fprintf(w, "") - } - } - - fmt.Fprintf(w, f.preWrapper.End(true)) - - if wrapInTable { - fmt.Fprint(w, " |
, B, ...
- {
- `(?[CBIUDTKRPAELZVMSXN])(?<+|«)`,
- ByGroups(Keyword, Punctuation),
- Mutators(
- bracketsFinder(rakuPodFormatter),
- Push("pod-formatter"), MutatorFunc(podFormatter),
- ),
- },
- },
- "pod-formatter": {
- // Placeholder rule, will be replaced by podFormatter. DO NOT REMOVE!
- {`>`, Punctuation, Pop(1)},
- Include("pre-pod-formatter"),
- // Placeholder rule, will be replaced by podFormatter. DO NOT REMOVE!
- {`.+?`, StringOther, nil},
- },
- "variable": {
- {variablePattern, NameVariable, Push("name-adverb")},
- {globalVariablePattern, NameVariableGlobal, Push("name-adverb")},
- {`[$@](?:<.*?>)+`, NameVariable, nil},
- {`\$/`, NameVariable, nil},
- {`\$!`, NameVariable, nil},
- {`[$@%]`, NameVariable, nil},
- },
- "single-quote": {
- {`(?>(?!\s*(?:\d+|\.(?:Int|Numeric)|[$@%][\w':-]+|\s+\[))`, Punctuation, Pop(1)},
- Include("ww"),
- },
- "«": {
- {`»(?!\s*(?:\d+|\.(?:Int|Numeric)|[$@%][\w':-]+|\s+\[))`, Punctuation, Pop(1)},
- Include("ww"),
- },
- "ww": {
- Include("single-quote"),
- Include("qq"),
- },
- "qq": {
- Include("qq-variable"),
- // Function with adverb
- {
- `\w[\w:'-]+(?=:['\w-]+` +
- colonPairOpeningBrackets + `.+?` + colonPairClosingBrackets + `\()`,
- NameFunction,
- Push("qq-function", "name-adverb"),
- },
- // Function without adverb
- {`\w[\w:'-]+(?=\((?!"))`, NameFunction, Push("qq-function")},
- Include("closure"),
- Include("escape-hexadecimal"),
- Include("escape-c-name"),
- Include("escape-qq"),
- {`.+?`, StringDouble, nil},
- },
- "qq-function": {
- {`(\([^"]*?\))`, UsingSelf("root"), nil},
- Default(Pop(1)),
- },
- "qq-variable": {
- {
- `(?>|<.*?>|«.*?»)+`, UsingSelf("root"), nil},
- // Method
- {
- `(\.)([^(\s]+)(\([^"]*?\))`,
- ByGroups(Operator, NameFunction, UsingSelf("root")),
- nil,
- },
- Default(Pop(1)),
- },
- "Q": {
- Include("escape-qq"),
- {`.+?`, String, nil},
- },
- "Q-closure": {
- Include("escape-qq"),
- Include("closure"),
- {`.+?`, String, nil},
- },
- "Q-variable": {
- Include("escape-qq"),
- Include("qq-variable"),
- {`.+?`, String, nil},
- },
- "closure": {
- {`(? -1 {
- idx = utf8.RuneCountInString(text[:idx])
- idx += pos
- }
-
- return idx
-}
-
-// Tells if an array of string contains a string
-func contains(s []string, e string) bool {
- for _, value := range s {
- if value == e {
- return true
- }
- }
- return false
-}
-
-type RakuFormatterRules struct {
- pop, formatter *CompiledRule
-}
-
-// Pop from the pod_formatter_stack and reformat the pod code
-func podFormatterPopper(state *LexerState) error {
- stack, ok := state.Get("pod_formatter_stack").([]RakuFormatterRules)
-
- if ok && len(stack) > 0 {
- // Pop from stack
- stack = stack[:len(stack)-1]
- state.Set("pod_formatter_stack", stack)
- // Call podFormatter to use the last formatter rules
- err := podFormatter(state)
- if err != nil {
- panic(err)
- }
- }
-
- return nil
-}
-
-// Use the rules from pod_formatter_stack to format the pod code
-func podFormatter(state *LexerState) error {
- stack, ok := state.Get("pod_formatter_stack").([]RakuFormatterRules)
- if ok && len(stack) > 0 {
- rules := stack[len(stack)-1]
- state.Rules["pod-formatter"][0] = rules.pop
- state.Rules["pod-formatter"][len(state.Rules["pod-formatter"])-1] = rules.formatter
- }
-
- return nil
-}
-
-type RulePosition int
-
-const (
- topRule RulePosition = iota + 1000
- bottomRule
-)
-
-type RuleMakingConfig struct {
- delimiter []rune
- pattern string
- tokenType TokenType
- mutator Mutator
- rulePosition RulePosition
- state *LexerState
- stateName string
- pushToStack bool
- numberOfDelimiterChars int
-}
-
-// Makes compiled rules and returns them, If rule position is given, rules are added to the state
-// If pushToStack is true, state name will be added to the state stack
-func makeRuleAndPushMaybe(config RuleMakingConfig) *CompiledRule {
- var rePattern string
- if len(config.delimiter) > 0 {
- delimiter := strings.Repeat(string(config.delimiter), config.numberOfDelimiterChars)
- rePattern = regexp2.Escape(delimiter)
- } else {
- rePattern = config.pattern
- }
- regex := regexp2.MustCompile(rePattern, regexp2.None)
-
- cRule := &CompiledRule{
- Rule: Rule{rePattern, config.tokenType, config.mutator},
- Regexp: regex,
- }
- state := config.state
- stateName := config.stateName
- switch config.rulePosition {
- case topRule:
- state.Rules[stateName] =
- append([]*CompiledRule{cRule}, state.Rules[stateName][1:]...)
- case bottomRule:
- state.Rules[stateName] =
- append(state.Rules[stateName][:len(state.Rules[stateName])-1], cRule)
- }
-
- // Push state name to stack if asked
- if config.pushToStack {
- state.Stack = append(state.Stack, config.stateName)
- }
-
- return cRule
-}
-
-// Used when the regex knows its own delimiter and uses `UsingSelf("regex")`,
-// it only puts a placeholder rule at the top of "regex" state
-func makeRegexPoppingRule(state *LexerState) error {
- makeRuleAndPushMaybe(RuleMakingConfig{
- pattern: `^$`,
- rulePosition: topRule,
- state: state,
- stateName: "regex",
- })
-
- return nil
-}
-
-// Emitter for colon pairs, changes token state based on key and brackets
-func colonPair(tokenClass TokenType) Emitter {
- return EmitterFunc(func(groups []string, state *LexerState) Iterator {
- iterators := []Iterator{}
- tokens := []Token{
- {Punctuation, state.NamedGroups[`colon`]},
- {Punctuation, state.NamedGroups[`opening_delimiters`]},
- {Punctuation, state.NamedGroups[`closing_delimiters`]},
- }
-
- // Append colon
- iterators = append(iterators, Literator(tokens[0]))
-
- if tokenClass == NameAttribute {
- iterators = append(iterators, Literator(Token{NameAttribute, state.NamedGroups[`key`]}))
- } else {
- var keyTokenState string
- keyre := regexp.MustCompile(`^\d+$`)
- if keyre.MatchString(state.NamedGroups[`key`]) {
- keyTokenState = "common"
- } else {
- keyTokenState = "Q"
- }
-
- // Use token state to Tokenise key
- if keyTokenState != "" {
- iterator, err := state.Lexer.Tokenise(
- &TokeniseOptions{
- State: keyTokenState,
- Nested: true,
- }, state.NamedGroups[`key`])
-
- if err != nil {
- panic(err)
- } else {
- // Append key
- iterators = append(iterators, iterator)
- }
- }
- }
-
- // Append punctuation
- iterators = append(iterators, Literator(tokens[1]))
-
- var valueTokenState string
-
- switch state.NamedGroups[`opening_delimiters`] {
- case "(", "{", "[":
- valueTokenState = "root"
- case "<<", "«":
- valueTokenState = "ww"
- case "<":
- valueTokenState = "Q"
- }
-
- // Use token state to Tokenise value
- if valueTokenState != "" {
- iterator, err := state.Lexer.Tokenise(
- &TokeniseOptions{
- State: valueTokenState,
- Nested: true,
- }, state.NamedGroups[`value`])
-
- if err != nil {
- panic(err)
- } else {
- // Append value
- iterators = append(iterators, iterator)
- }
- }
- // Append last punctuation
- iterators = append(iterators, Literator(tokens[2]))
-
- return Concaterator(iterators...)
- })
-}
-
-// Emitter for quoting constructs, changes token state based on quote name and adverbs
-func quote(groups []string, state *LexerState) Iterator {
- keyword := state.NamedGroups[`keyword`]
- adverbsStr := state.NamedGroups[`adverbs`]
- iterators := []Iterator{}
- tokens := []Token{
- {Keyword, keyword},
- {StringAffix, adverbsStr},
- {Text, state.NamedGroups[`ws`]},
- {Punctuation, state.NamedGroups[`opening_delimiters`]},
- {Punctuation, state.NamedGroups[`closing_delimiters`]},
- }
-
- // Append all tokens before dealing with the main string
- iterators = append(iterators, Literator(tokens[:4]...))
-
- var tokenStates []string
-
- // Set tokenStates based on adverbs
- adverbs := strings.Split(adverbsStr, ":")
- for _, adverb := range adverbs {
- switch adverb {
- case "c", "closure":
- tokenStates = append(tokenStates, "Q-closure")
- case "qq":
- tokenStates = append(tokenStates, "qq")
- case "ww":
- tokenStates = append(tokenStates, "ww")
- case "s", "scalar", "a", "array", "h", "hash", "f", "function":
- tokenStates = append(tokenStates, "Q-variable")
- }
- }
-
- var tokenState string
-
- switch {
- case keyword == "qq" || contains(tokenStates, "qq"):
- tokenState = "qq"
- case adverbsStr == "ww" || contains(tokenStates, "ww"):
- tokenState = "ww"
- case contains(tokenStates, "Q-closure") && contains(tokenStates, "Q-variable"):
- tokenState = "qq"
- case contains(tokenStates, "Q-closure"):
- tokenState = "Q-closure"
- case contains(tokenStates, "Q-variable"):
- tokenState = "Q-variable"
- default:
- tokenState = "Q"
- }
-
- iterator, err := state.Lexer.Tokenise(
- &TokeniseOptions{
- State: tokenState,
- Nested: true,
- }, state.NamedGroups[`value`])
-
- if err != nil {
- panic(err)
- } else {
- iterators = append(iterators, iterator)
- }
-
- // Append the last punctuation
- iterators = append(iterators, Literator(tokens[4]))
-
- return Concaterator(iterators...)
-}
-
-// Emitter for pod config, tokenises the properties with "colon-pair-attribute" state
-func podConfig(groups []string, state *LexerState) Iterator {
- // Tokenise pod config
- iterator, err := state.Lexer.Tokenise(
- &TokeniseOptions{
- State: "colon-pair-attribute",
- Nested: true,
- }, groups[0])
-
- if err != nil {
- panic(err)
- } else {
- return iterator
- }
-}
-
-// Emitter for pod code, tokenises the code based on the lang specified
-func podCode(groups []string, state *LexerState) Iterator {
- iterators := []Iterator{}
- tokens := []Token{
- {Comment, state.NamedGroups[`ws`]},
- {Keyword, state.NamedGroups[`keyword`]},
- {Keyword, state.NamedGroups[`ws2`]},
- {Keyword, state.NamedGroups[`name`]},
- {StringDoc, state.NamedGroups[`value`]},
- {Comment, state.NamedGroups[`ws3`]},
- {Keyword, state.NamedGroups[`end_keyword`]},
- {Keyword, state.NamedGroups[`ws4`]},
- {Keyword, state.NamedGroups[`name`]},
- }
-
- // Append all tokens before dealing with the pod config
- iterators = append(iterators, Literator(tokens[:4]...))
-
- // Tokenise pod config
- iterators = append(iterators, podConfig([]string{state.NamedGroups[`config`]}, state))
-
- langMatch := regexp.MustCompile(`:lang\W+(\w+)`).FindStringSubmatch(state.NamedGroups[`config`])
- var lang string
- if len(langMatch) > 1 {
- lang = langMatch[1]
- }
-
- // Tokenise code based on lang property
- sublexer := internal.Get(lang)
- if sublexer != nil {
- iterator, err := sublexer.Tokenise(nil, state.NamedGroups[`value`])
-
- if err != nil {
- panic(err)
- } else {
- iterators = append(iterators, iterator)
- }
- } else {
- iterators = append(iterators, Literator(tokens[4]))
- }
-
- // Append the rest of the tokens
- iterators = append(iterators, Literator(tokens[5:]...))
-
- return Concaterator(iterators...)
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/r/reasonml.go b/vendor/github.com/alecthomas/chroma/lexers/r/reasonml.go
deleted file mode 100644
index 0ade569..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/r/reasonml.go
+++ /dev/null
@@ -1,71 +0,0 @@
-package r
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-// Reasonml lexer.
-var Reasonml = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "ReasonML",
- Aliases: []string{"reason", "reasonml"},
- Filenames: []string{"*.re", "*.rei"},
- MimeTypes: []string{"text/x-reasonml"},
- },
- reasonmlRules,
-))
-
-func reasonmlRules() Rules {
- return Rules{
- "escape-sequence": {
- {`\\[\\"\'ntbr]`, LiteralStringEscape, nil},
- {`\\[0-9]{3}`, LiteralStringEscape, nil},
- {`\\x[0-9a-fA-F]{2}`, LiteralStringEscape, nil},
- },
- "root": {
- {`\s+`, Text, nil},
- {`false|true|\(\)|\[\]`, NameBuiltinPseudo, nil},
- {`\b([A-Z][\w\']*)(?=\s*\.)`, NameNamespace, Push("dotted")},
- {`\b([A-Z][\w\']*)`, NameClass, nil},
- {`//.*?\n`, CommentSingle, nil},
- {`\/\*(?![\/])`, CommentMultiline, Push("comment")},
- {`\b(as|assert|begin|class|constraint|do|done|downto|else|end|exception|external|false|for|fun|esfun|function|functor|if|in|include|inherit|initializer|lazy|let|switch|module|pub|mutable|new|nonrec|object|of|open|pri|rec|sig|struct|then|to|true|try|type|val|virtual|when|while|with)\b`, Keyword, nil},
- {"(~|\\}|\\|]|\\||\\|\\||\\{<|\\{|`|_|]|\\[\\||\\[>|\\[<|\\[|\\?\\?|\\?|>\\}|>]|>|=|<-|<|;;|;|:>|:=|::|:|\\.\\.\\.|\\.\\.|\\.|=>|-\\.|-|,|\\+|\\*|\\)|\\(|&&|&|#|!=)", OperatorWord, nil},
- {`([=<>@^|&+\*/$%-]|[!?~])?[!$%&*+\./:<=>?@^|~-]`, Operator, nil},
- {`\b(and|asr|land|lor|lsl|lsr|lxor|mod|or)\b`, OperatorWord, nil},
- {`\b(unit|int|float|bool|string|char|list|array)\b`, KeywordType, nil},
- {`[^\W\d][\w']*`, Name, nil},
- {`-?\d[\d_]*(.[\d_]*)?([eE][+\-]?\d[\d_]*)`, LiteralNumberFloat, nil},
- {`0[xX][\da-fA-F][\da-fA-F_]*`, LiteralNumberHex, nil},
- {`0[oO][0-7][0-7_]*`, LiteralNumberOct, nil},
- {`0[bB][01][01_]*`, LiteralNumberBin, nil},
- {`\d[\d_]*`, LiteralNumberInteger, nil},
- {`'(?:(\\[\\\"'ntbr ])|(\\[0-9]{3})|(\\x[0-9a-fA-F]{2}))'`, LiteralStringChar, nil},
- {`'.'`, LiteralStringChar, nil},
- {`'`, Keyword, nil},
- {`"`, LiteralStringDouble, Push("string")},
- {`[~?][a-z][\w\']*:`, NameVariable, nil},
- },
- "comment": {
- {`[^\/*]+`, CommentMultiline, nil},
- {`\/\*`, CommentMultiline, Push()},
- {`\*\/`, CommentMultiline, Pop(1)},
- {`[\*]`, CommentMultiline, nil},
- },
- "string": {
- {`[^\\"]+`, LiteralStringDouble, nil},
- Include("escape-sequence"),
- {`\\\n`, LiteralStringDouble, nil},
- {`"`, LiteralStringDouble, Pop(1)},
- },
- "dotted": {
- {`\s+`, Text, nil},
- {`\.`, Punctuation, nil},
- {`[A-Z][\w\']*(?=\s*\.)`, NameNamespace, nil},
- {`[A-Z][\w\']*`, NameClass, Pop(1)},
- {`[a-z_][\w\']*`, Name, Pop(1)},
- Default(Pop(1)),
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/r/regedit.go b/vendor/github.com/alecthomas/chroma/lexers/r/regedit.go
deleted file mode 100644
index 6177234..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/r/regedit.go
+++ /dev/null
@@ -1,36 +0,0 @@
-package r
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-// Reg lexer.
-var Reg = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "reg",
- Aliases: []string{"registry"},
- Filenames: []string{"*.reg"},
- MimeTypes: []string{"text/x-windows-registry"},
- },
- regRules,
-))
-
-func regRules() Rules {
- return Rules{
- "root": {
- {`Windows Registry Editor.*`, Text, nil},
- {`\s+`, Text, nil},
- {`[;#].*`, CommentSingle, nil},
- {`(\[)(-?)(HKEY_[A-Z_]+)(.*?\])$`, ByGroups(Keyword, Operator, NameBuiltin, Keyword), nil},
- {`("(?:\\"|\\\\|[^"])+")([ \t]*)(=)([ \t]*)`, ByGroups(NameAttribute, Text, Operator, Text), Push("value")},
- {`(.*?)([ \t]*)(=)([ \t]*)`, ByGroups(NameAttribute, Text, Operator, Text), Push("value")},
- },
- "value": {
- {`-`, Operator, Pop(1)},
- {`(dword|hex(?:\([0-9a-fA-F]\))?)(:)([0-9a-fA-F,]+)`, ByGroups(NameVariable, Punctuation, LiteralNumber), Pop(1)},
- {`.+`, LiteralString, Pop(1)},
- Default(Pop(1)),
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/r/rexx.go b/vendor/github.com/alecthomas/chroma/lexers/r/rexx.go
deleted file mode 100644
index 38ca768..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/r/rexx.go
+++ /dev/null
@@ -1,63 +0,0 @@
-package r
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-// Rexx lexer.
-var Rexx = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "Rexx",
- Aliases: []string{"rexx", "arexx"},
- Filenames: []string{"*.rexx", "*.rex", "*.rx", "*.arexx"},
- MimeTypes: []string{"text/x-rexx"},
- NotMultiline: true,
- CaseInsensitive: true,
- },
- rexxRules,
-))
-
-func rexxRules() Rules {
- return Rules{
- "root": {
- {`\s`, TextWhitespace, nil},
- {`/\*`, CommentMultiline, Push("comment")},
- {`"`, LiteralString, Push("string_double")},
- {`'`, LiteralString, Push("string_single")},
- {`[0-9]+(\.[0-9]+)?(e[+-]?[0-9])?`, LiteralNumber, nil},
- {`([a-z_]\w*)(\s*)(:)(\s*)(procedure)\b`, ByGroups(NameFunction, TextWhitespace, Operator, TextWhitespace, KeywordDeclaration), nil},
- {`([a-z_]\w*)(\s*)(:)`, ByGroups(NameLabel, TextWhitespace, Operator), nil},
- Include("function"),
- Include("keyword"),
- Include("operator"),
- {`[a-z_]\w*`, Text, nil},
- },
- "function": {
- {Words(``, `(\s*)(\()`, `abbrev`, `abs`, `address`, `arg`, `b2x`, `bitand`, `bitor`, `bitxor`, `c2d`, `c2x`, `center`, `charin`, `charout`, `chars`, `compare`, `condition`, `copies`, `d2c`, `d2x`, `datatype`, `date`, `delstr`, `delword`, `digits`, `errortext`, `form`, `format`, `fuzz`, `insert`, `lastpos`, `left`, `length`, `linein`, `lineout`, `lines`, `max`, `min`, `overlay`, `pos`, `queued`, `random`, `reverse`, `right`, `sign`, `sourceline`, `space`, `stream`, `strip`, `substr`, `subword`, `symbol`, `time`, `trace`, `translate`, `trunc`, `value`, `verify`, `word`, `wordindex`, `wordlength`, `wordpos`, `words`, `x2b`, `x2c`, `x2d`, `xrange`), ByGroups(NameBuiltin, TextWhitespace, Operator), nil},
- },
- "keyword": {
- {`(address|arg|by|call|do|drop|else|end|exit|for|forever|if|interpret|iterate|leave|nop|numeric|off|on|options|parse|pull|push|queue|return|say|select|signal|to|then|trace|until|while)\b`, KeywordReserved, nil},
- },
- "operator": {
- {`(-|//|/|\(|\)|\*\*|\*|\\<<|\\<|\\==|\\=|\\>>|\\>|\\|\|\||\||&&|&|%|\+|<<=|<<|<=|<>|<|==|=|><|>=|>>=|>>|>|¬<<|¬<|¬==|¬=|¬>>|¬>|¬|\.|,)`, Operator, nil},
- },
- "string_double": {
- {`[^"\n]+`, LiteralString, nil},
- {`""`, LiteralString, nil},
- {`"`, LiteralString, Pop(1)},
- {`\n`, Text, Pop(1)},
- },
- "string_single": {
- {`[^\'\n]`, LiteralString, nil},
- {`\'\'`, LiteralString, nil},
- {`\'`, LiteralString, Pop(1)},
- {`\n`, Text, Pop(1)},
- },
- "comment": {
- {`[^*]+`, CommentMultiline, nil},
- {`\*/`, CommentMultiline, Pop(1)},
- {`\*`, CommentMultiline, nil},
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/r/rst.go b/vendor/github.com/alecthomas/chroma/lexers/r/rst.go
deleted file mode 100644
index 2a87479..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/r/rst.go
+++ /dev/null
@@ -1,90 +0,0 @@
-package r
-
-import (
- "strings"
-
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-// Restructuredtext lexer.
-var Restructuredtext = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "reStructuredText",
- Aliases: []string{"rst", "rest", "restructuredtext"},
- Filenames: []string{"*.rst", "*.rest"},
- MimeTypes: []string{"text/x-rst", "text/prs.fallenstein.rst"},
- },
- restructuredtextRules,
-))
-
-func restructuredtextRules() Rules {
- return Rules{
- "root": {
- {"^(=+|-+|`+|:+|\\.+|\\'+|\"+|~+|\\^+|_+|\\*+|\\++|#+)([ \\t]*\\n)(.+)(\\n)(\\1)(\\n)", ByGroups(GenericHeading, Text, GenericHeading, Text, GenericHeading, Text), nil},
- {"^(\\S.*)(\\n)(={3,}|-{3,}|`{3,}|:{3,}|\\.{3,}|\\'{3,}|\"{3,}|~{3,}|\\^{3,}|_{3,}|\\*{3,}|\\+{3,}|#{3,})(\\n)", ByGroups(GenericHeading, Text, GenericHeading, Text), nil},
- {`^(\s*)([-*+])( .+\n(?:\1 .+\n)*)`, ByGroups(Text, LiteralNumber, UsingSelf("inline")), nil},
- {`^(\s*)([0-9#ivxlcmIVXLCM]+\.)( .+\n(?:\1 .+\n)*)`, ByGroups(Text, LiteralNumber, UsingSelf("inline")), nil},
- {`^(\s*)(\(?[0-9#ivxlcmIVXLCM]+\))( .+\n(?:\1 .+\n)*)`, ByGroups(Text, LiteralNumber, UsingSelf("inline")), nil},
- {`^(\s*)([A-Z]+\.)( .+\n(?:\1 .+\n)+)`, ByGroups(Text, LiteralNumber, UsingSelf("inline")), nil},
- {`^(\s*)(\(?[A-Za-z]+\))( .+\n(?:\1 .+\n)+)`, ByGroups(Text, LiteralNumber, UsingSelf("inline")), nil},
- {`^(\s*)(\|)( .+\n(?:\| .+\n)*)`, ByGroups(Text, Operator, UsingSelf("inline")), nil},
- {`^( *\.\.)(\s*)((?:source)?code(?:-block)?)(::)([ \t]*)([^\n]+)(\n[ \t]*\n)([ \t]+)(.*)(\n)((?:(?:\8.*|)\n)+)`, EmitterFunc(rstCodeBlock), nil},
- {`^( *\.\.)(\s*)([\w:-]+?)(::)(?:([ \t]*)(.*))`, ByGroups(Punctuation, Text, OperatorWord, Punctuation, Text, UsingSelf("inline")), nil},
- {`^( *\.\.)(\s*)(_(?:[^:\\]|\\.)+:)(.*?)$`, ByGroups(Punctuation, Text, NameTag, UsingSelf("inline")), nil},
- {`^( *\.\.)(\s*)(\[.+\])(.*?)$`, ByGroups(Punctuation, Text, NameTag, UsingSelf("inline")), nil},
- {`^( *\.\.)(\s*)(\|.+\|)(\s*)([\w:-]+?)(::)(?:([ \t]*)(.*))`, ByGroups(Punctuation, Text, NameTag, Text, OperatorWord, Punctuation, Text, UsingSelf("inline")), nil},
- {`^ *\.\..*(\n( +.*\n|\n)+)?`, CommentPreproc, nil},
- {`^( *)(:[a-zA-Z-]+:)(\s*)$`, ByGroups(Text, NameClass, Text), nil},
- {`^( *)(:.*?:)([ \t]+)(.*?)$`, ByGroups(Text, NameClass, Text, NameFunction), nil},
- {`^(\S.*(?)(`__?)", ByGroups(LiteralString, LiteralStringInterpol, LiteralString), nil},
- {"`.+?`__?", LiteralString, nil},
- {"(`.+?`)(:[a-zA-Z0-9:-]+?:)?", ByGroups(NameVariable, NameAttribute), nil},
- {"(:[a-zA-Z0-9:-]+?:)(`.+?`)", ByGroups(NameAttribute, NameVariable), nil},
- {`\*\*.+?\*\*`, GenericStrong, nil},
- {`\*.+?\*`, GenericEmph, nil},
- {`\[.*?\]_`, LiteralString, nil},
- {`<.+?>`, NameTag, nil},
- {"[^\\\\\\n\\[*`:]+", Text, nil},
- {`.`, Text, nil},
- },
- "literal": {
- {"[^`]+", LiteralString, nil},
- {"``((?=$)|(?=[-/:.,; \\n\\x00\\\u2010\\\u2011\\\u2012\\\u2013\\\u2014\\\u00a0\\'\\\"\\)\\]\\}\\>\\\u2019\\\u201d\\\u00bb\\!\\?]))", LiteralString, Pop(1)},
- {"`", LiteralString, nil},
- },
- }
-}
-
-func rstCodeBlock(groups []string, state *LexerState) Iterator {
- iterators := []Iterator{}
- tokens := []Token{
- {Punctuation, groups[1]},
- {Text, groups[2]},
- {OperatorWord, groups[3]},
- {Punctuation, groups[4]},
- {Text, groups[5]},
- {Keyword, groups[6]},
- {Text, groups[7]},
- }
- code := strings.Join(groups[8:], "")
- lexer := internal.Get(groups[6])
- if lexer == nil {
- tokens = append(tokens, Token{String, code})
- iterators = append(iterators, Literator(tokens...))
- } else {
- sub, err := lexer.Tokenise(nil, code)
- if err != nil {
- panic(err)
- }
- iterators = append(iterators, Literator(tokens...), sub)
- }
- return Concaterator(iterators...)
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/r/ruby.go b/vendor/github.com/alecthomas/chroma/lexers/r/ruby.go
deleted file mode 100644
index 296cae9..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/r/ruby.go
+++ /dev/null
@@ -1,254 +0,0 @@
-package r
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-// Ruby lexer.
-var Ruby = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "Ruby",
- Aliases: []string{"rb", "ruby", "duby"},
- Filenames: []string{"*.rb", "*.rbw", "Rakefile", "*.rake", "*.gemspec", "*.rbx", "*.duby", "Gemfile"},
- MimeTypes: []string{"text/x-ruby", "application/x-ruby"},
- DotAll: true,
- },
- rubyRules,
-))
-
-func rubyRules() Rules {
- return Rules{
- "root": {
- {`\A#!.+?$`, CommentHashbang, nil},
- {`#.*?$`, CommentSingle, nil},
- {`=begin\s.*?\n=end.*?$`, CommentMultiline, nil},
- {Words(``, `\b`, `BEGIN`, `END`, `alias`, `begin`, `break`, `case`, `defined?`, `do`, `else`, `elsif`, `end`, `ensure`, `for`, `if`, `in`, `next`, `redo`, `rescue`, `raise`, `retry`, `return`, `super`, `then`, `undef`, `unless`, `until`, `when`, `while`, `yield`), Keyword, nil},
- {`(module)(\s+)([a-zA-Z_]\w*(?:::[a-zA-Z_]\w*)*)`, ByGroups(Keyword, Text, NameNamespace), nil},
- {`(def)(\s+)`, ByGroups(Keyword, Text), Push("funcname")},
- {"def(?=[*%&^`~+-/\\[<>=])", Keyword, Push("funcname")},
- {`(class)(\s+)`, ByGroups(Keyword, Text), Push("classname")},
- {Words(``, `\b`, `initialize`, `new`, `loop`, `include`, `extend`, `raise`, `attr_reader`, `attr_writer`, `attr_accessor`, `attr`, `catch`, `throw`, `private`, `module_function`, `public`, `protected`, `true`, `false`, `nil`), KeywordPseudo, nil},
- {`(not|and|or)\b`, OperatorWord, nil},
- {Words(``, `\?`, `autoload`, `block_given`, `const_defined`, `eql`, `equal`, `frozen`, `include`, `instance_of`, `is_a`, `iterator`, `kind_of`, `method_defined`, `nil`, `private_method_defined`, `protected_method_defined`, `public_method_defined`, `respond_to`, `tainted`), NameBuiltin, nil},
- {`(chomp|chop|exit|gsub|sub)!`, NameBuiltin, nil},
- {Words(`(?~!:])|(?<=(?:\s|;)when\s)|(?<=(?:\s|;)or\s)|(?<=(?:\s|;)and\s)|(?<=\.index\s)|(?<=\.scan\s)|(?<=\.sub\s)|(?<=\.sub!\s)|(?<=\.gsub\s)|(?<=\.gsub!\s)|(?<=\.match\s)|(?<=(?:\s|;)if\s)|(?<=(?:\s|;)elsif\s)|(?<=^when\s)|(?<=^index\s)|(?<=^scan\s)|(?<=^sub\s)|(?<=^gsub\s)|(?<=^sub!\s)|(?<=^gsub!\s)|(?<=^match\s)|(?<=^if\s)|(?<=^elsif\s))(\s*)(/)`, ByGroups(Text, LiteralStringRegex), Push("multiline-regex")},
- {`(?<=\(|,|\[)/`, LiteralStringRegex, Push("multiline-regex")},
- {`(\s+)(/)(?![\s=])`, ByGroups(Text, LiteralStringRegex), Push("multiline-regex")},
- {`(0_?[0-7]+(?:_[0-7]+)*)(\s*)([/?])?`, ByGroups(LiteralNumberOct, Text, Operator), nil},
- {`(0x[0-9A-Fa-f]+(?:_[0-9A-Fa-f]+)*)(\s*)([/?])?`, ByGroups(LiteralNumberHex, Text, Operator), nil},
- {`(0b[01]+(?:_[01]+)*)(\s*)([/?])?`, ByGroups(LiteralNumberBin, Text, Operator), nil},
- {`([\d]+(?:[_e]\d+)*)(\s*)([/?])?`, ByGroups(LiteralNumberInteger, Text, Operator), nil},
- {`@@[a-zA-Z_]\w*`, NameVariableClass, nil},
- {`@[a-zA-Z_]\w*`, NameVariableInstance, nil},
- {`\$\w+`, NameVariableGlobal, nil},
- {"\\$[!@&`\\'+~=/\\\\,;.<>_*$?:\"^-]", NameVariableGlobal, nil},
- {`\$-[0adFiIlpvw]`, NameVariableGlobal, nil},
- {`::`, Operator, nil},
- Include("strings"),
- {`\?(\\[MC]-)*(\\([\\abefnrstv#"\']|x[a-fA-F0-9]{1,2}|[0-7]{1,3})|\S)(?!\w)`, LiteralStringChar, nil},
- {`[A-Z]\w+`, NameConstant, nil},
- {Words(`(\.|::)`, ``, `*`, `**`, `-`, `+`, `-@`, `+@`, `/`, `%`, `&`, `|`, `^`, "`", `~`, `[]`, `[]=`, `<<`, `>>`, `<`, `<>`, `<=>`, `>`, `>=`, `==`, `===`), ByGroups(Operator, NameOperator), nil},
- {"(\\.|::)([a-zA-Z_]\\w*[!?]?|[*%&^`~+\\-/\\[<>=])", ByGroups(Operator, Name), nil},
- {`[a-zA-Z_]\w*[!?]?`, Name, nil},
- {`(\[|\]|\*\*|<|>>?|>=|<=|<=>|=~|={3}|!~|&&?|\|\||\.{1,3})`, Operator, nil},
- {`[-+/*%=<>&!^|~]=?`, Operator, nil},
- {`[(){};,/?:\\]`, Punctuation, nil},
- {`\s+`, Text, nil},
- },
- "funcname": {
- {`\(`, Punctuation, Push("defexpr")},
- {"(?:([a-zA-Z_]\\w*)(\\.))?([a-zA-Z_]\\w*[!?]?|\\*\\*?|[-+]@?|[/%&|^`~]|\\[\\]=?|<<|>>|<=?>|>=?|===?)", ByGroups(NameClass, Operator, NameFunction), Pop(1)},
- Default(Pop(1)),
- },
- "classname": {
- {`\(`, Punctuation, Push("defexpr")},
- {`<<`, Operator, Pop(1)},
- {`[A-Z_]\w*`, NameClass, Pop(1)},
- Default(Pop(1)),
- },
- "defexpr": {
- {`(\))(\.|::)?`, ByGroups(Punctuation, Operator), Pop(1)},
- {`\(`, Operator, Push()},
- Include("root"),
- },
- "in-intp": {
- {`\{`, LiteralStringInterpol, Push()},
- {`\}`, LiteralStringInterpol, Pop(1)},
- Include("root"),
- },
- "string-intp": {
- {`#\{`, LiteralStringInterpol, Push("in-intp")},
- {`#@@?[a-zA-Z_]\w*`, LiteralStringInterpol, nil},
- {`#\$[a-zA-Z_]\w*`, LiteralStringInterpol, nil},
- },
- "string-intp-escaped": {
- Include("string-intp"),
- {`\\([\\abefnrstv#"\']|x[a-fA-F0-9]{1,2}|[0-7]{1,3})`, LiteralStringEscape, nil},
- },
- "interpolated-regex": {
- Include("string-intp"),
- {`[\\#]`, LiteralStringRegex, nil},
- {`[^\\#]+`, LiteralStringRegex, nil},
- },
- "interpolated-string": {
- Include("string-intp"),
- {`[\\#]`, LiteralStringOther, nil},
- {`[^\\#]+`, LiteralStringOther, nil},
- },
- "multiline-regex": {
- Include("string-intp"),
- {`\\\\`, LiteralStringRegex, nil},
- {`\\/`, LiteralStringRegex, nil},
- {`[\\#]`, LiteralStringRegex, nil},
- {`[^\\/#]+`, LiteralStringRegex, nil},
- {`/[mixounse]*`, LiteralStringRegex, Pop(1)},
- },
- "end-part": {
- {`.+`, CommentPreproc, Pop(1)},
- },
- "strings": {
- {`\:@{0,2}[a-zA-Z_]\w*[!?]?`, LiteralStringSymbol, nil},
- {Words(`\:@{0,2}`, ``, `*`, `**`, `-`, `+`, `-@`, `+@`, `/`, `%`, `&`, `|`, `^`, "`", `~`, `[]`, `[]=`, `<<`, `>>`, `<`, `<>`, `<=>`, `>`, `>=`, `==`, `===`), LiteralStringSymbol, nil},
- {`:'(\\\\|\\'|[^'])*'`, LiteralStringSymbol, nil},
- {`'(\\\\|\\'|[^'])*'`, LiteralStringSingle, nil},
- {`:"`, LiteralStringSymbol, Push("simple-sym")},
- {`([a-zA-Z_]\w*)(:)(?!:)`, ByGroups(LiteralStringSymbol, Punctuation), nil},
- {`"`, LiteralStringDouble, Push("simple-string")},
- {"(?&!^|~,(])(\s*)(%([\t ])(?:(?:\\\3|(?!\3).)*)\3)`, ByGroups(Text, LiteralStringOther, None), nil},
- {`^(\s*)(%([\t ])(?:(?:\\\3|(?!\3).)*)\3)`, ByGroups(Text, LiteralStringOther, None), nil},
- {`(%([^a-zA-Z0-9\s]))((?:\\\2|(?!\2).)*)(\2)`, String, nil},
- },
- "simple-string": {
- Include("string-intp-escaped"),
- {`[^\\"#]+`, LiteralStringDouble, nil},
- {`[\\#]`, LiteralStringDouble, nil},
- {`"`, LiteralStringDouble, Pop(1)},
- },
- "simple-sym": {
- Include("string-intp-escaped"),
- {`[^\\"#]+`, LiteralStringSymbol, nil},
- {`[\\#]`, LiteralStringSymbol, nil},
- {`"`, LiteralStringSymbol, Pop(1)},
- },
- "simple-backtick": {
- Include("string-intp-escaped"),
- {"[^\\\\`#]+", LiteralStringBacktick, nil},
- {`[\\#]`, LiteralStringBacktick, nil},
- {"`", LiteralStringBacktick, Pop(1)},
- },
- "cb-intp-string": {
- {`\\[\\{}]`, LiteralStringOther, nil},
- {`\{`, LiteralStringOther, Push()},
- {`\}`, LiteralStringOther, Pop(1)},
- Include("string-intp-escaped"),
- {`[\\#{}]`, LiteralStringOther, nil},
- {`[^\\#{}]+`, LiteralStringOther, nil},
- },
- "cb-string": {
- {`\\[\\{}]`, LiteralStringOther, nil},
- {`\{`, LiteralStringOther, Push()},
- {`\}`, LiteralStringOther, Pop(1)},
- {`[\\#{}]`, LiteralStringOther, nil},
- {`[^\\#{}]+`, LiteralStringOther, nil},
- },
- "cb-regex": {
- {`\\[\\{}]`, LiteralStringRegex, nil},
- {`\{`, LiteralStringRegex, Push()},
- {`\}[mixounse]*`, LiteralStringRegex, Pop(1)},
- Include("string-intp"),
- {`[\\#{}]`, LiteralStringRegex, nil},
- {`[^\\#{}]+`, LiteralStringRegex, nil},
- },
- "sb-intp-string": {
- {`\\[\\\[\]]`, LiteralStringOther, nil},
- {`\[`, LiteralStringOther, Push()},
- {`\]`, LiteralStringOther, Pop(1)},
- Include("string-intp-escaped"),
- {`[\\#\[\]]`, LiteralStringOther, nil},
- {`[^\\#\[\]]+`, LiteralStringOther, nil},
- },
- "sb-string": {
- {`\\[\\\[\]]`, LiteralStringOther, nil},
- {`\[`, LiteralStringOther, Push()},
- {`\]`, LiteralStringOther, Pop(1)},
- {`[\\#\[\]]`, LiteralStringOther, nil},
- {`[^\\#\[\]]+`, LiteralStringOther, nil},
- },
- "sb-regex": {
- {`\\[\\\[\]]`, LiteralStringRegex, nil},
- {`\[`, LiteralStringRegex, Push()},
- {`\][mixounse]*`, LiteralStringRegex, Pop(1)},
- Include("string-intp"),
- {`[\\#\[\]]`, LiteralStringRegex, nil},
- {`[^\\#\[\]]+`, LiteralStringRegex, nil},
- },
- "pa-intp-string": {
- {`\\[\\()]`, LiteralStringOther, nil},
- {`\(`, LiteralStringOther, Push()},
- {`\)`, LiteralStringOther, Pop(1)},
- Include("string-intp-escaped"),
- {`[\\#()]`, LiteralStringOther, nil},
- {`[^\\#()]+`, LiteralStringOther, nil},
- },
- "pa-string": {
- {`\\[\\()]`, LiteralStringOther, nil},
- {`\(`, LiteralStringOther, Push()},
- {`\)`, LiteralStringOther, Pop(1)},
- {`[\\#()]`, LiteralStringOther, nil},
- {`[^\\#()]+`, LiteralStringOther, nil},
- },
- "pa-regex": {
- {`\\[\\()]`, LiteralStringRegex, nil},
- {`\(`, LiteralStringRegex, Push()},
- {`\)[mixounse]*`, LiteralStringRegex, Pop(1)},
- Include("string-intp"),
- {`[\\#()]`, LiteralStringRegex, nil},
- {`[^\\#()]+`, LiteralStringRegex, nil},
- },
- "ab-intp-string": {
- {`\\[\\<>]`, LiteralStringOther, nil},
- {`<`, LiteralStringOther, Push()},
- {`>`, LiteralStringOther, Pop(1)},
- Include("string-intp-escaped"),
- {`[\\#<>]`, LiteralStringOther, nil},
- {`[^\\#<>]+`, LiteralStringOther, nil},
- },
- "ab-string": {
- {`\\[\\<>]`, LiteralStringOther, nil},
- {`<`, LiteralStringOther, Push()},
- {`>`, LiteralStringOther, Pop(1)},
- {`[\\#<>]`, LiteralStringOther, nil},
- {`[^\\#<>]+`, LiteralStringOther, nil},
- },
- "ab-regex": {
- {`\\[\\<>]`, LiteralStringRegex, nil},
- {`<`, LiteralStringRegex, Push()},
- {`>[mixounse]*`, LiteralStringRegex, Pop(1)},
- Include("string-intp"),
- {`[\\#<>]`, LiteralStringRegex, nil},
- {`[^\\#<>]+`, LiteralStringRegex, nil},
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/r/rust.go b/vendor/github.com/alecthomas/chroma/lexers/r/rust.go
deleted file mode 100644
index 25d42e5..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/r/rust.go
+++ /dev/null
@@ -1,139 +0,0 @@
-package r
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-// Rust lexer.
-var Rust = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "Rust",
- Aliases: []string{"rust"},
- Filenames: []string{"*.rs", "*.rs.in"},
- MimeTypes: []string{"text/rust"},
- EnsureNL: true,
- },
- rustRules,
-))
-
-func rustRules() Rules {
- return Rules{
- "root": {
- {`#![^[\r\n].*$`, CommentPreproc, nil},
- Default(Push("base")),
- },
- "base": {
- {`\n`, TextWhitespace, nil},
- {`\s+`, TextWhitespace, nil},
- {`//!.*?\n`, LiteralStringDoc, nil},
- {`///(\n|[^/].*?\n)`, LiteralStringDoc, nil},
- {`//(.*?)\n`, CommentSingle, nil},
- {`/\*\*(\n|[^/*])`, LiteralStringDoc, Push("doccomment")},
- {`/\*!`, LiteralStringDoc, Push("doccomment")},
- {`/\*`, CommentMultiline, Push("comment")},
- {`r#*"(?:\\.|[^\\;])*"#*`, LiteralString, nil},
- {`"(?:\\.|[^\\"])*"`, LiteralString, nil},
- {`\$([a-zA-Z_]\w*|\(,?|\),?|,?)`, CommentPreproc, nil},
- {Words(``, `\b`, `as`, `async`, `await`, `const`, `crate`, `else`, `extern`, `for`, `if`, `impl`, `in`, `loop`, `match`, `move`, `mut`, `pub`, `ref`, `return`, `static`, `super`, `trait`, `unsafe`, `use`, `where`, `while`), Keyword, nil},
- {Words(``, `\b`, `abstract`, `become`, `box`, `do`, `final`, `macro`, `override`, `priv`, `try`, `typeof`, `unsized`, `virtual`, `yield`), KeywordReserved, nil},
- {`(true|false)\b`, KeywordConstant, nil},
- {`mod\b`, Keyword, Push("modname")},
- {`let\b`, KeywordDeclaration, nil},
- {`fn\b`, Keyword, Push("funcname")},
- {`(struct|enum|type|union)\b`, Keyword, Push("typename")},
- {`(default)(\s+)(type|fn)\b`, ByGroups(Keyword, Text, Keyword), nil},
- {Words(``, `\b`, `u8`, `u16`, `u32`, `u64`, `u128`, `i8`, `i16`, `i32`, `i64`, `i128`, `usize`, `isize`, `f32`, `f64`, `str`, `bool`), KeywordType, nil},
- {`self\b`, NameBuiltinPseudo, nil},
- {Words(``, `\b`, `Copy`, `Send`, `Sized`, `Sync`, `Drop`, `Fn`, `FnMut`, `FnOnce`, `Box`, `ToOwned`, `Clone`, `PartialEq`, `PartialOrd`, `Eq`, `Ord`, `AsRef`, `AsMut`, `Into`, `From`, `Default`, `Iterator`, `Extend`, `IntoIterator`, `DoubleEndedIterator`, `ExactSizeIterator`, `Option`, `Some`, `None`, `Result`, `Ok`, `Err`, `SliceConcatExt`, `String`, `ToString`, `Vec`), NameBuiltin, nil},
- {`::\b`, Text, nil},
- {`(?::|->)`, Text, Push("typename")},
- {`(break|continue)(\s*)(\'[A-Za-z_]\w*)?`, ByGroups(Keyword, TextWhitespace, NameLabel), nil},
- {`'(\\['"\\nrt]|\\x[0-7][0-9a-fA-F]|\\0|\\u\{[0-9a-fA-F]{1,6}\}|.)'`, LiteralStringChar, nil},
- {`b'(\\['"\\nrt]|\\x[0-9a-fA-F]{2}|\\0|\\u\{[0-9a-fA-F]{1,6}\}|.)'`, LiteralStringChar, nil},
- {`0b[01_]+`, LiteralNumberBin, Push("number_lit")},
- {`0o[0-7_]+`, LiteralNumberOct, Push("number_lit")},
- {`0[xX][0-9a-fA-F_]+`, LiteralNumberHex, Push("number_lit")},
- {`[0-9][0-9_]*(\.[0-9_]+[eE][+\-]?[0-9_]+|\.[0-9_]*(?!\.)|[eE][+\-]?[0-9_]+)`, LiteralNumberFloat, Push("number_lit")},
- {`[0-9][0-9_]*`, LiteralNumberInteger, Push("number_lit")},
- {`b"`, LiteralString, Push("bytestring")},
- {`b?r(#*)".*?"\1`, LiteralString, nil},
- {`'static`, NameBuiltin, nil},
- {`'[a-zA-Z_]\w*`, NameAttribute, nil},
- {`[{}()\[\],.;]`, Punctuation, nil},
- {`[+\-*/%&|<>^!~@=:?]`, Operator, nil},
- {`(r#)?[a-zA-Z_]\w*`, Name, nil},
- {`#!?\[`, CommentPreproc, Push("attribute[")},
- {`([A-Za-z_]\w*)(!)(\s*)([A-Za-z_]\w*)?(\s*)(\{)`, ByGroups(CommentPreproc, Punctuation, TextWhitespace, Name, TextWhitespace, Punctuation), Push("macro{")},
- {`([A-Za-z_]\w*)(!)(\s*)([A-Za-z_]\w*)?(\()`, ByGroups(CommentPreproc, Punctuation, TextWhitespace, Name, Punctuation), Push("macro(")},
- },
- "comment": {
- {`[^*/]+`, CommentMultiline, nil},
- {`/\*`, CommentMultiline, Push()},
- {`\*/`, CommentMultiline, Pop(1)},
- {`[*/]`, CommentMultiline, nil},
- },
- "doccomment": {
- {`[^*/]+`, LiteralStringDoc, nil},
- {`/\*`, LiteralStringDoc, Push()},
- {`\*/`, LiteralStringDoc, Pop(1)},
- {`[*/]`, LiteralStringDoc, nil},
- },
- "modname": {
- {`\s+`, Text, nil},
- {`[a-zA-Z_]\w*`, NameNamespace, Pop(1)},
- Default(Pop(1)),
- },
- "funcname": {
- {`\s+`, Text, nil},
- {`[a-zA-Z_]\w*`, NameFunction, Pop(1)},
- Default(Pop(1)),
- },
- "typename": {
- {`\s+`, Text, nil},
- {`&`, KeywordPseudo, nil},
- {Words(``, `\b`, `Copy`, `Send`, `Sized`, `Sync`, `Drop`, `Fn`, `FnMut`, `FnOnce`, `Box`, `ToOwned`, `Clone`, `PartialEq`, `PartialOrd`, `Eq`, `Ord`, `AsRef`, `AsMut`, `Into`, `From`, `Default`, `Iterator`, `Extend`, `IntoIterator`, `DoubleEndedIterator`, `ExactSizeIterator`, `Option`, `Some`, `None`, `Result`, `Ok`, `Err`, `SliceConcatExt`, `String`, `ToString`, `Vec`), NameBuiltin, nil},
- {Words(``, `\b`, `u8`, `u16`, `u32`, `u64`, `i8`, `i16`, `i32`, `i64`, `usize`, `isize`, `f32`, `f64`, `str`, `bool`), KeywordType, nil},
- {`[a-zA-Z_]\w*`, NameClass, Pop(1)},
- Default(Pop(1)),
- },
- "number_lit": {
- {`[ui](8|16|32|64|size)`, Keyword, Pop(1)},
- {`f(32|64)`, Keyword, Pop(1)},
- Default(Pop(1)),
- },
- "string": {
- {`"`, LiteralString, Pop(1)},
- {`\\['"\\nrt]|\\x[0-7][0-9a-fA-F]|\\0|\\u\{[0-9a-fA-F]{1,6}\}`, LiteralStringEscape, nil},
- {`[^\\"]+`, LiteralString, nil},
- {`\\`, LiteralString, nil},
- },
- "bytestring": {
- {`\\x[89a-fA-F][0-9a-fA-F]`, LiteralStringEscape, nil},
- Include("string"),
- },
- "macro{": {
- {`\{`, Operator, Push()},
- {`\}`, Operator, Pop(1)},
- },
- "macro(": {
- {`\(`, Operator, Push()},
- {`\)`, Operator, Pop(1)},
- },
- "attribute_common": {
- {`"`, LiteralString, Push("string")},
- {`\[`, CommentPreproc, Push("attribute[")},
- {`\(`, CommentPreproc, Push("attribute(")},
- },
- "attribute[": {
- Include("attribute_common"),
- {`\];?`, CommentPreproc, Pop(1)},
- {`[^"\]]+`, CommentPreproc, nil},
- },
- "attribute(": {
- Include("attribute_common"),
- {`\);?`, CommentPreproc, Pop(1)},
- {`[^")]+`, CommentPreproc, nil},
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/s/sas.go b/vendor/github.com/alecthomas/chroma/lexers/s/sas.go
deleted file mode 100644
index 016a91b..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/s/sas.go
+++ /dev/null
@@ -1,98 +0,0 @@
-package s
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-// Sas lexer.
-var Sas = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "SAS",
- Aliases: []string{"sas"},
- Filenames: []string{"*.SAS", "*.sas"},
- MimeTypes: []string{"text/x-sas", "text/sas", "application/x-sas"},
- CaseInsensitive: true,
- },
- sasRules,
-))
-
-func sasRules() Rules {
- return Rules{
- "root": {
- Include("comments"),
- Include("proc-data"),
- Include("cards-datalines"),
- Include("logs"),
- Include("general"),
- {`.`, Text, nil},
- {`\\\n`, Text, nil},
- {`\n`, Text, nil},
- },
- "comments": {
- {`^\s*\*.*?;`, Comment, nil},
- {`/\*.*?\*/`, Comment, nil},
- {`^\s*\*(.|\n)*?;`, CommentMultiline, nil},
- {`/[*](.|\n)*?[*]/`, CommentMultiline, nil},
- },
- "proc-data": {
- {`(^|;)\s*(proc \w+|data|run|quit)[\s;]`, KeywordReserved, nil},
- },
- "cards-datalines": {
- {`^\s*(datalines|cards)\s*;\s*$`, Keyword, Push("data")},
- },
- "data": {
- {`(.|\n)*^\s*;\s*$`, Other, Pop(1)},
- },
- "logs": {
- {`\n?^\s*%?put `, Keyword, Push("log-messages")},
- },
- "log-messages": {
- {`NOTE(:|-).*`, Generic, Pop(1)},
- {`WARNING(:|-).*`, GenericEmph, Pop(1)},
- {`ERROR(:|-).*`, GenericError, Pop(1)},
- Include("general"),
- },
- "general": {
- Include("keywords"),
- Include("vars-strings"),
- Include("special"),
- Include("numbers"),
- },
- "keywords": {
- {Words(`\b`, `\b`, `abort`, `array`, `attrib`, `by`, `call`, `cards`, `cards4`, `catname`, `continue`, `datalines`, `datalines4`, `delete`, `delim`, `delimiter`, `display`, `dm`, `drop`, `endsas`, `error`, `file`, `filename`, `footnote`, `format`, `goto`, `in`, `infile`, `informat`, `input`, `keep`, `label`, `leave`, `length`, `libname`, `link`, `list`, `lostcard`, `merge`, `missing`, `modify`, `options`, `output`, `out`, `page`, `put`, `redirect`, `remove`, `rename`, `replace`, `retain`, `return`, `select`, `set`, `skip`, `startsas`, `stop`, `title`, `update`, `waitsas`, `where`, `window`, `x`, `systask`), Keyword, nil},
- {Words(`\b`, `\b`, `add`, `and`, `alter`, `as`, `cascade`, `check`, `create`, `delete`, `describe`, `distinct`, `drop`, `foreign`, `from`, `group`, `having`, `index`, `insert`, `into`, `in`, `key`, `like`, `message`, `modify`, `msgtype`, `not`, `null`, `on`, `or`, `order`, `primary`, `references`, `reset`, `restrict`, `select`, `set`, `table`, `unique`, `update`, `validate`, `view`, `where`), Keyword, nil},
- {Words(`\b`, `\b`, `do`, `if`, `then`, `else`, `end`, `until`, `while`), Keyword, nil},
- {Words(`%`, `\b`, `bquote`, `nrbquote`, `cmpres`, `qcmpres`, `compstor`, `datatyp`, `display`, `do`, `else`, `end`, `eval`, `global`, `goto`, `if`, `index`, `input`, `keydef`, `label`, `left`, `length`, `let`, `local`, `lowcase`, `macro`, `mend`, `nrquote`, `nrstr`, `put`, `qleft`, `qlowcase`, `qscan`, `qsubstr`, `qsysfunc`, `qtrim`, `quote`, `qupcase`, `scan`, `str`, `substr`, `superq`, `syscall`, `sysevalf`, `sysexec`, `sysfunc`, `sysget`, `syslput`, `sysprod`, `sysrc`, `sysrput`, `then`, `to`, `trim`, `unquote`, `until`, `upcase`, `verify`, `while`, `window`), NameBuiltin, nil},
- {Words(`\b`, `\(`, `abs`, `addr`, `airy`, `arcos`, `arsin`, `atan`, `attrc`, `attrn`, `band`, `betainv`, `blshift`, `bnot`, `bor`, `brshift`, `bxor`, `byte`, `cdf`, `ceil`, `cexist`, `cinv`, `close`, `cnonct`, `collate`, `compbl`, `compound`, `compress`, `cos`, `cosh`, `css`, `curobs`, `cv`, `daccdb`, `daccdbsl`, `daccsl`, `daccsyd`, `dacctab`, `dairy`, `date`, `datejul`, `datepart`, `datetime`, `day`, `dclose`, `depdb`, `depdbsl`, `depsl`, `depsyd`, `deptab`, `dequote`, `dhms`, `dif`, `digamma`, `dim`, `dinfo`, `dnum`, `dopen`, `doptname`, `doptnum`, `dread`, `dropnote`, `dsname`, `erf`, `erfc`, `exist`, `exp`, `fappend`, `fclose`, `fcol`, `fdelete`, `fetch`, `fetchobs`, `fexist`, `fget`, `fileexist`, `filename`, `fileref`, `finfo`, `finv`, `fipname`, `fipnamel`, `fipstate`, `floor`, `fnonct`, `fnote`, `fopen`, `foptname`, `foptnum`, `fpoint`, `fpos`, `fput`, `fread`, `frewind`, `frlen`, `fsep`, `fuzz`, `fwrite`, `gaminv`, `gamma`, `getoption`, `getvarc`, `getvarn`, `hbound`, `hms`, `hosthelp`, `hour`, `ibessel`, `index`, `indexc`, `indexw`, `input`, `inputc`, `inputn`, `int`, `intck`, `intnx`, `intrr`, `irr`, `jbessel`, `juldate`, `kurtosis`, `lag`, `lbound`, `left`, `length`, `lgamma`, `libname`, `libref`, `log`, `log10`, `log2`, `logpdf`, `logpmf`, `logsdf`, `lowcase`, `max`, `mdy`, `mean`, `min`, `minute`, `mod`, `month`, `mopen`, `mort`, `n`, `netpv`, `nmiss`, `normal`, `note`, `npv`, `open`, `ordinal`, `pathname`, `pdf`, `peek`, `peekc`, `pmf`, `point`, `poisson`, `poke`, `probbeta`, `probbnml`, `probchi`, `probf`, `probgam`, `probhypr`, `probit`, `probnegb`, `probnorm`, `probt`, `put`, `putc`, `putn`, `qtr`, `quote`, `ranbin`, `rancau`, `ranexp`, `rangam`, `range`, `rank`, `rannor`, `ranpoi`, `rantbl`, `rantri`, `ranuni`, `repeat`, `resolve`, `reverse`, `rewind`, `right`, `round`, `saving`, `scan`, `sdf`, `second`, `sign`, `sin`, `sinh`, `skewness`, `soundex`, `spedis`, `sqrt`, `std`, `stderr`, `stfips`, `stname`, `stnamel`, `substr`, `sum`, `symget`, `sysget`, `sysmsg`, `sysprod`, `sysrc`, `system`, `tan`, `tanh`, `time`, `timepart`, `tinv`, `tnonct`, `today`, `translate`, `tranwrd`, `trigamma`, `trim`, `trimn`, `trunc`, `uniform`, `upcase`, `uss`, `var`, `varfmt`, `varinfmt`, `varlabel`, `varlen`, `varname`, `varnum`, `varray`, `varrayx`, `vartype`, `verify`, `vformat`, `vformatd`, `vformatdx`, `vformatn`, `vformatnx`, `vformatw`, `vformatwx`, `vformatx`, `vinarray`, `vinarrayx`, `vinformat`, `vinformatd`, `vinformatdx`, `vinformatn`, `vinformatnx`, `vinformatw`, `vinformatwx`, `vinformatx`, `vlabel`, `vlabelx`, `vlength`, `vlengthx`, `vname`, `vnamex`, `vtype`, `vtypex`, `weekday`, `year`, `yyq`, `zipfips`, `zipname`, `zipnamel`, `zipstate`), NameBuiltin, nil},
- },
- "vars-strings": {
- {`&[a-z_]\w{0,31}\.?`, NameVariable, nil},
- {`%[a-z_]\w{0,31}`, NameFunction, nil},
- {`\'`, LiteralString, Push("string_squote")},
- {`"`, LiteralString, Push("string_dquote")},
- },
- "string_squote": {
- {`'`, LiteralString, Pop(1)},
- {`\\\\|\\"|\\\n`, LiteralStringEscape, nil},
- {`[^$\'\\]+`, LiteralString, nil},
- {`[$\'\\]`, LiteralString, nil},
- },
- "string_dquote": {
- {`"`, LiteralString, Pop(1)},
- {`\\\\|\\"|\\\n`, LiteralStringEscape, nil},
- {`&`, NameVariable, Push("validvar")},
- {`[^$&"\\]+`, LiteralString, nil},
- {`[$"\\]`, LiteralString, nil},
- },
- "validvar": {
- {`[a-z_]\w{0,31}\.?`, NameVariable, Pop(1)},
- },
- "numbers": {
- {`\b[+-]?([0-9]+(\.[0-9]+)?|\.[0-9]+|\.)(E[+-]?[0-9]+)?i?\b`, LiteralNumber, nil},
- },
- "special": {
- {`(null|missing|_all_|_automatic_|_character_|_n_|_infile_|_name_|_null_|_numeric_|_user_|_webout_)`, KeywordConstant, nil},
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/s/sass.go b/vendor/github.com/alecthomas/chroma/lexers/s/sass.go
deleted file mode 100644
index 1b73377..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/s/sass.go
+++ /dev/null
@@ -1,148 +0,0 @@
-package s
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-// Sass lexer.
-var Sass = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "Sass",
- Aliases: []string{"sass"},
- Filenames: []string{"*.sass"},
- MimeTypes: []string{"text/x-sass"},
- CaseInsensitive: true,
- },
- sassRules,
-))
-
-func sassRules() Rules {
- return Rules{
- // "root": {
- // },
- "root": {
- {`[ \t]*\n`, Text, nil},
- // { `[ \t]*`, ?? ??, nil },
- // { `//[^\n]*`, ?? .callback at 0x106936048> ??, Push("root") },
- // { `/\*[^\n]*`, ?? .callback at 0x1069360d0> ??, Push("root") },
- {`@import`, Keyword, Push("import")},
- {`@for`, Keyword, Push("for")},
- {`@(debug|warn|if|while)`, Keyword, Push("value")},
- {`(@mixin)( [\w-]+)`, ByGroups(Keyword, NameFunction), Push("value")},
- {`(@include)( [\w-]+)`, ByGroups(Keyword, NameDecorator), Push("value")},
- {`@extend`, Keyword, Push("selector")},
- {`@[\w-]+`, Keyword, Push("selector")},
- {`=[\w-]+`, NameFunction, Push("value")},
- {`\+[\w-]+`, NameDecorator, Push("value")},
- {`([!$][\w-]\w*)([ \t]*(?:(?:\|\|)?=|:))`, ByGroups(NameVariable, Operator), Push("value")},
- {`:`, NameAttribute, Push("old-style-attr")},
- {`(?=.+?[=:]([^a-z]|$))`, NameAttribute, Push("new-style-attr")},
- Default(Push("selector")),
- },
- "single-comment": {
- {`.+`, CommentSingle, nil},
- {`\n`, Text, Push("root")},
- },
- "multi-comment": {
- {`.+`, CommentMultiline, nil},
- {`\n`, Text, Push("root")},
- },
- "import": {
- {`[ \t]+`, Text, nil},
- {`\S+`, LiteralString, nil},
- {`\n`, Text, Push("root")},
- },
- "old-style-attr": {
- {`[^\s:="\[]+`, NameAttribute, nil},
- {`#\{`, LiteralStringInterpol, Push("interpolation")},
- {`[ \t]*=`, Operator, Push("value")},
- Default(Push("value")),
- },
- "new-style-attr": {
- {`[^\s:="\[]+`, NameAttribute, nil},
- {`#\{`, LiteralStringInterpol, Push("interpolation")},
- {`[ \t]*[=:]`, Operator, Push("value")},
- },
- "inline-comment": {
- {`(\\#|#(?=[^\n{])|\*(?=[^\n/])|[^\n#*])+`, CommentMultiline, nil},
- {`#\{`, LiteralStringInterpol, Push("interpolation")},
- {`\*/`, Comment, Pop(1)},
- },
- "value": {
- {`[ \t]+`, Text, nil},
- {`[!$][\w-]+`, NameVariable, nil},
- {`url\(`, LiteralStringOther, Push("string-url")},
- {`[a-z_-][\w-]*(?=\()`, NameFunction, nil},
- {Words(``, `\b`, `align-content`, `align-items`, `align-self`, `alignment-baseline`, `all`, `animation`, `animation-delay`, `animation-direction`, `animation-duration`, `animation-fill-mode`, `animation-iteration-count`, `animation-name`, `animation-play-state`, `animation-timing-function`, `appearance`, `azimuth`, `backface-visibility`, `background`, `background-attachment`, `background-blend-mode`, `background-clip`, `background-color`, `background-image`, `background-origin`, `background-position`, `background-repeat`, `background-size`, `baseline-shift`, `bookmark-label`, `bookmark-level`, `bookmark-state`, `border`, `border-bottom`, `border-bottom-color`, `border-bottom-left-radius`, `border-bottom-right-radius`, `border-bottom-style`, `border-bottom-width`, `border-boundary`, `border-collapse`, `border-color`, `border-image`, `border-image-outset`, `border-image-repeat`, `border-image-slice`, `border-image-source`, `border-image-width`, `border-left`, `border-left-color`, `border-left-style`, `border-left-width`, `border-radius`, `border-right`, `border-right-color`, `border-right-style`, `border-right-width`, `border-spacing`, `border-style`, `border-top`, `border-top-color`, `border-top-left-radius`, `border-top-right-radius`, `border-top-style`, `border-top-width`, `border-width`, `bottom`, `box-decoration-break`, `box-shadow`, `box-sizing`, `box-snap`, `box-suppress`, `break-after`, `break-before`, `break-inside`, `caption-side`, `caret`, `caret-animation`, `caret-color`, `caret-shape`, `chains`, `clear`, `clip`, `clip-path`, `clip-rule`, `color`, `color-interpolation-filters`, `column-count`, `column-fill`, `column-gap`, `column-rule`, `column-rule-color`, `column-rule-style`, `column-rule-width`, `column-span`, `column-width`, `columns`, `content`, `counter-increment`, `counter-reset`, `counter-set`, `crop`, `cue`, `cue-after`, `cue-before`, `cursor`, `direction`, `display`, `dominant-baseline`, `elevation`, `empty-cells`, `filter`, `flex`, `flex-basis`, `flex-direction`, `flex-flow`, `flex-grow`, `flex-shrink`, `flex-wrap`, `float`, `float-defer`, `float-offset`, `float-reference`, `flood-color`, `flood-opacity`, `flow`, `flow-from`, `flow-into`, `font`, `font-family`, `font-feature-settings`, `font-kerning`, `font-language-override`, `font-size`, `font-size-adjust`, `font-stretch`, `font-style`, `font-synthesis`, `font-variant`, `font-variant-alternates`, `font-variant-caps`, `font-variant-east-asian`, `font-variant-ligatures`, `font-variant-numeric`, `font-variant-position`, `font-weight`, `footnote-display`, `footnote-policy`, `glyph-orientation-vertical`, `grid`, `grid-area`, `grid-auto-columns`, `grid-auto-flow`, `grid-auto-rows`, `grid-column`, `grid-column-end`, `grid-column-gap`, `grid-column-start`, `grid-gap`, `grid-row`, `grid-row-end`, `grid-row-gap`, `grid-row-start`, `grid-template`, `grid-template-areas`, `grid-template-columns`, `grid-template-rows`, `hanging-punctuation`, `height`, `hyphenate-character`, `hyphenate-limit-chars`, `hyphenate-limit-last`, `hyphenate-limit-lines`, `hyphenate-limit-zone`, `hyphens`, `image-orientation`, `image-resolution`, `initial-letter`, `initial-letter-align`, `initial-letter-wrap`, `isolation`, `justify-content`, `justify-items`, `justify-self`, `left`, `letter-spacing`, `lighting-color`, `line-break`, `line-grid`, `line-height`, `line-snap`, `list-style`, `list-style-image`, `list-style-position`, `list-style-type`, `margin`, `margin-bottom`, `margin-left`, `margin-right`, `margin-top`, `marker-side`, `marquee-direction`, `marquee-loop`, `marquee-speed`, `marquee-style`, `mask`, `mask-border`, `mask-border-mode`, `mask-border-outset`, `mask-border-repeat`, `mask-border-slice`, `mask-border-source`, `mask-border-width`, `mask-clip`, `mask-composite`, `mask-image`, `mask-mode`, `mask-origin`, `mask-position`, `mask-repeat`, `mask-size`, `mask-type`, `max-height`, `max-lines`, `max-width`, `min-height`, `min-width`, `mix-blend-mode`, `motion`, `motion-offset`, `motion-path`, `motion-rotation`, `move-to`, `nav-down`, `nav-left`, `nav-right`, `nav-up`, `object-fit`, `object-position`, `offset-after`, `offset-before`, `offset-end`, `offset-start`, `opacity`, `order`, `orphans`, `outline`, `outline-color`, `outline-offset`, `outline-style`, `outline-width`, `overflow`, `overflow-style`, `overflow-wrap`, `overflow-x`, `overflow-y`, `padding`, `padding-bottom`, `padding-left`, `padding-right`, `padding-top`, `page`, `page-break-after`, `page-break-before`, `page-break-inside`, `page-policy`, `pause`, `pause-after`, `pause-before`, `perspective`, `perspective-origin`, `pitch`, `pitch-range`, `play-during`, `polar-angle`, `polar-distance`, `position`, `presentation-level`, `quotes`, `region-fragment`, `resize`, `rest`, `rest-after`, `rest-before`, `richness`, `right`, `rotation`, `rotation-point`, `ruby-align`, `ruby-merge`, `ruby-position`, `running`, `scroll-snap-coordinate`, `scroll-snap-destination`, `scroll-snap-points-x`, `scroll-snap-points-y`, `scroll-snap-type`, `shape-image-threshold`, `shape-inside`, `shape-margin`, `shape-outside`, `size`, `speak`, `speak-as`, `speak-header`, `speak-numeral`, `speak-punctuation`, `speech-rate`, `stress`, `string-set`, `tab-size`, `table-layout`, `text-align`, `text-align-last`, `text-combine-upright`, `text-decoration`, `text-decoration-color`, `text-decoration-line`, `text-decoration-skip`, `text-decoration-style`, `text-emphasis`, `text-emphasis-color`, `text-emphasis-position`, `text-emphasis-style`, `text-indent`, `text-justify`, `text-orientation`, `text-overflow`, `text-shadow`, `text-space-collapse`, `text-space-trim`, `text-spacing`, `text-transform`, `text-underline-position`, `text-wrap`, `top`, `transform`, `transform-origin`, `transform-style`, `transition`, `transition-delay`, `transition-duration`, `transition-property`, `transition-timing-function`, `unicode-bidi`, `user-select`, `vertical-align`, `visibility`, `voice-balance`, `voice-duration`, `voice-family`, `voice-pitch`, `voice-range`, `voice-rate`, `voice-stress`, `voice-volume`, `volume`, `white-space`, `widows`, `width`, `will-change`, `word-break`, `word-spacing`, `word-wrap`, `wrap-after`, `wrap-before`, `wrap-flow`, `wrap-inside`, `wrap-through`, `writing-mode`, `z-index`, `above`, `absolute`, `always`, `armenian`, `aural`, `auto`, `avoid`, `baseline`, `behind`, `below`, `bidi-override`, `blink`, `block`, `bold`, `bolder`, `both`, `capitalize`, `center-left`, `center-right`, `center`, `circle`, `cjk-ideographic`, `close-quote`, `collapse`, `condensed`, `continuous`, `crop`, `crosshair`, `cross`, `cursive`, `dashed`, `decimal-leading-zero`, `decimal`, `default`, `digits`, `disc`, `dotted`, `double`, `e-resize`, `embed`, `extra-condensed`, `extra-expanded`, `expanded`, `fantasy`, `far-left`, `far-right`, `faster`, `fast`, `fixed`, `georgian`, `groove`, `hebrew`, `help`, `hidden`, `hide`, `higher`, `high`, `hiragana-iroha`, `hiragana`, `icon`, `inherit`, `inline-table`, `inline`, `inset`, `inside`, `invert`, `italic`, `justify`, `katakana-iroha`, `katakana`, `landscape`, `larger`, `large`, `left-side`, `leftwards`, `level`, `lighter`, `line-through`, `list-item`, `loud`, `lower-alpha`, `lower-greek`, `lower-roman`, `lowercase`, `ltr`, `lower`, `low`, `medium`, `message-box`, `middle`, `mix`, `monospace`, `n-resize`, `narrower`, `ne-resize`, `no-close-quote`, `no-open-quote`, `no-repeat`, `none`, `normal`, `nowrap`, `nw-resize`, `oblique`, `once`, `open-quote`, `outset`, `outside`, `overline`, `pointer`, `portrait`, `px`, `relative`, `repeat-x`, `repeat-y`, `repeat`, `rgb`, `ridge`, `right-side`, `rightwards`, `s-resize`, `sans-serif`, `scroll`, `se-resize`, `semi-condensed`, `semi-expanded`, `separate`, `serif`, `show`, `silent`, `slow`, `slower`, `small-caps`, `small-caption`, `smaller`, `soft`, `solid`, `spell-out`, `square`, `static`, `status-bar`, `super`, `sw-resize`, `table-caption`, `table-cell`, `table-column`, `table-column-group`, `table-footer-group`, `table-header-group`, `table-row`, `table-row-group`, `text`, `text-bottom`, `text-top`, `thick`, `thin`, `transparent`, `ultra-condensed`, `ultra-expanded`, `underline`, `upper-alpha`, `upper-latin`, `upper-roman`, `uppercase`, `url`, `visible`, `w-resize`, `wait`, `wider`, `x-fast`, `x-high`, `x-large`, `x-loud`, `x-low`, `x-small`, `x-soft`, `xx-large`, `xx-small`, `yes`), NameConstant, nil},
- {Words(``, `\b`, `aliceblue`, `antiquewhite`, `aqua`, `aquamarine`, `azure`, `beige`, `bisque`, `black`, `blanchedalmond`, `blue`, `blueviolet`, `brown`, `burlywood`, `cadetblue`, `chartreuse`, `chocolate`, `coral`, `cornflowerblue`, `cornsilk`, `crimson`, `cyan`, `darkblue`, `darkcyan`, `darkgoldenrod`, `darkgray`, `darkgreen`, `darkgrey`, `darkkhaki`, `darkmagenta`, `darkolivegreen`, `darkorange`, `darkorchid`, `darkred`, `darksalmon`, `darkseagreen`, `darkslateblue`, `darkslategray`, `darkslategrey`, `darkturquoise`, `darkviolet`, `deeppink`, `deepskyblue`, `dimgray`, `dimgrey`, `dodgerblue`, `firebrick`, `floralwhite`, `forestgreen`, `fuchsia`, `gainsboro`, `ghostwhite`, `gold`, `goldenrod`, `gray`, `green`, `greenyellow`, `grey`, `honeydew`, `hotpink`, `indianred`, `indigo`, `ivory`, `khaki`, `lavender`, `lavenderblush`, `lawngreen`, `lemonchiffon`, `lightblue`, `lightcoral`, `lightcyan`, `lightgoldenrodyellow`, `lightgray`, `lightgreen`, `lightgrey`, `lightpink`, `lightsalmon`, `lightseagreen`, `lightskyblue`, `lightslategray`, `lightslategrey`, `lightsteelblue`, `lightyellow`, `lime`, `limegreen`, `linen`, `magenta`, `maroon`, `mediumaquamarine`, `mediumblue`, `mediumorchid`, `mediumpurple`, `mediumseagreen`, `mediumslateblue`, `mediumspringgreen`, `mediumturquoise`, `mediumvioletred`, `midnightblue`, `mintcream`, `mistyrose`, `moccasin`, `navajowhite`, `navy`, `oldlace`, `olive`, `olivedrab`, `orange`, `orangered`, `orchid`, `palegoldenrod`, `palegreen`, `paleturquoise`, `palevioletred`, `papayawhip`, `peachpuff`, `peru`, `pink`, `plum`, `powderblue`, `purple`, `rebeccapurple`, `red`, `rosybrown`, `royalblue`, `saddlebrown`, `salmon`, `sandybrown`, `seagreen`, `seashell`, `sienna`, `silver`, `skyblue`, `slateblue`, `slategray`, `slategrey`, `snow`, `springgreen`, `steelblue`, `tan`, `teal`, `thistle`, `tomato`, `turquoise`, `violet`, `wheat`, `white`, `whitesmoke`, `yellow`, `yellowgreen`, `transparent`), NameEntity, nil},
- {Words(``, `\b`, `black`, `silver`, `gray`, `white`, `maroon`, `red`, `purple`, `fuchsia`, `green`, `lime`, `olive`, `yellow`, `navy`, `blue`, `teal`, `aqua`), NameBuiltin, nil},
- {`\!(important|default)`, NameException, nil},
- {`(true|false)`, NamePseudo, nil},
- {`(and|or|not)`, OperatorWord, nil},
- {`/\*`, CommentMultiline, Push("inline-comment")},
- {`//[^\n]*`, CommentSingle, nil},
- {`\#[a-z0-9]{1,6}`, LiteralNumberHex, nil},
- {`(-?\d+)(\%|[a-z]+)?`, ByGroups(LiteralNumberInteger, KeywordType), nil},
- {`(-?\d*\.\d+)(\%|[a-z]+)?`, ByGroups(LiteralNumberFloat, KeywordType), nil},
- {`#\{`, LiteralStringInterpol, Push("interpolation")},
- {`[~^*!&%<>|+=@:,./?-]+`, Operator, nil},
- {`[\[\]()]+`, Punctuation, nil},
- {`"`, LiteralStringDouble, Push("string-double")},
- {`'`, LiteralStringSingle, Push("string-single")},
- {`[a-z_-][\w-]*`, Name, nil},
- {`\n`, Text, Push("root")},
- },
- "interpolation": {
- {`\}`, LiteralStringInterpol, Pop(1)},
- Include("value"),
- },
- "selector": {
- {`[ \t]+`, Text, nil},
- {`\:`, NameDecorator, Push("pseudo-class")},
- {`\.`, NameClass, Push("class")},
- {`\#`, NameNamespace, Push("id")},
- {`[\w-]+`, NameTag, nil},
- {`#\{`, LiteralStringInterpol, Push("interpolation")},
- {`&`, Keyword, nil},
- {`[~^*!&\[\]()<>|+=@:;,./?-]`, Operator, nil},
- {`"`, LiteralStringDouble, Push("string-double")},
- {`'`, LiteralStringSingle, Push("string-single")},
- {`\n`, Text, Push("root")},
- },
- "string-double": {
- {`(\\.|#(?=[^\n{])|[^\n"#])+`, LiteralStringDouble, nil},
- {`#\{`, LiteralStringInterpol, Push("interpolation")},
- {`"`, LiteralStringDouble, Pop(1)},
- },
- "string-single": {
- {`(\\.|#(?=[^\n{])|[^\n'#])+`, LiteralStringSingle, nil},
- {`#\{`, LiteralStringInterpol, Push("interpolation")},
- {`'`, LiteralStringSingle, Pop(1)},
- },
- "string-url": {
- {`(\\#|#(?=[^\n{])|[^\n#)])+`, LiteralStringOther, nil},
- {`#\{`, LiteralStringInterpol, Push("interpolation")},
- {`\)`, LiteralStringOther, Pop(1)},
- },
- "pseudo-class": {
- {`[\w-]+`, NameDecorator, nil},
- {`#\{`, LiteralStringInterpol, Push("interpolation")},
- Default(Pop(1)),
- },
- "class": {
- {`[\w-]+`, NameClass, nil},
- {`#\{`, LiteralStringInterpol, Push("interpolation")},
- Default(Pop(1)),
- },
- "id": {
- {`[\w-]+`, NameNamespace, nil},
- {`#\{`, LiteralStringInterpol, Push("interpolation")},
- Default(Pop(1)),
- },
- "for": {
- {`(from|to|through)`, OperatorWord, nil},
- Include("value"),
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/s/scala.go b/vendor/github.com/alecthomas/chroma/lexers/s/scala.go
deleted file mode 100644
index 2885d01..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/s/scala.go
+++ /dev/null
@@ -1,116 +0,0 @@
-package s
-
-import (
- "fmt"
-
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-// Scala lexer.
-var Scala = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "Scala",
- Aliases: []string{"scala"},
- Filenames: []string{"*.scala"},
- MimeTypes: []string{"text/x-scala"},
- DotAll: true,
- },
- scalaRules,
-))
-
-func scalaRules() Rules {
- var (
- scalaOp = "[-~\\^\\*!%&\\\\<>\\|+=:/?@\xa6-\xa7\xa9\xac\xae\xb0-\xb1\xb6\xd7\xf7\u03f6\u0482\u0606-\u0608\u060e-\u060f\u06e9\u06fd-\u06fe\u07f6\u09fa\u0b70\u0bf3-\u0bf8\u0bfa\u0c7f\u0cf1-\u0cf2\u0d79\u0f01-\u0f03\u0f13-\u0f17\u0f1a-\u0f1f\u0f34\u0f36\u0f38\u0fbe-\u0fc5\u0fc7-\u0fcf\u109e-\u109f\u1360\u1390-\u1399\u1940\u19e0-\u19ff\u1b61-\u1b6a\u1b74-\u1b7c\u2044\u2052\u207a-\u207c\u208a-\u208c\u2100-\u2101\u2103-\u2106\u2108-\u2109\u2114\u2116-\u2118\u211e-\u2123\u2125\u2127\u2129\u212e\u213a-\u213b\u2140-\u2144\u214a-\u214d\u214f\u2190-\u2328\u232b-\u244a\u249c-\u24e9\u2500-\u2767\u2794-\u27c4\u27c7-\u27e5\u27f0-\u2982\u2999-\u29d7\u29dc-\u29fb\u29fe-\u2b54\u2ce5-\u2cea\u2e80-\u2ffb\u3004\u3012-\u3013\u3020\u3036-\u3037\u303e-\u303f\u3190-\u3191\u3196-\u319f\u31c0-\u31e3\u3200-\u321e\u322a-\u3250\u3260-\u327f\u328a-\u32b0\u32c0-\u33ff\u4dc0-\u4dff\ua490-\ua4c6\ua828-\ua82b\ufb29\ufdfd\ufe62\ufe64-\ufe66\uff0b\uff1c-\uff1e\uff5c\uff5e\uffe2\uffe4\uffe8-\uffee\ufffc-\ufffd]+"
- scalaUpper = "[A-Z\\$_\xc0-\xd6\xd8-\xde\u0100\u0102\u0104\u0106\u0108\u010a\u010c\u010e\u0110\u0112\u0114\u0116\u0118\u011a\u011c\u011e\u0120\u0122\u0124\u0126\u0128\u012a\u012c\u012e\u0130\u0132\u0134\u0136\u0139\u013b\u013d\u013f\u0141\u0143\u0145\u0147\u014a\u014c\u014e\u0150\u0152\u0154\u0156\u0158\u015a\u015c\u015e\u0160\u0162\u0164\u0166\u0168\u016a\u016c\u016e\u0170\u0172\u0174\u0176\u0178-\u0179\u017b\u017d\u0181-\u0182\u0184\u0186-\u0187\u0189-\u018b\u018e-\u0191\u0193-\u0194\u0196-\u0198\u019c-\u019d\u019f-\u01a0\u01a2\u01a4\u01a6-\u01a7\u01a9\u01ac\u01ae-\u01af\u01b1-\u01b3\u01b5\u01b7-\u01b8\u01bc\u01c4\u01c7\u01ca\u01cd\u01cf\u01d1\u01d3\u01d5\u01d7\u01d9\u01db\u01de\u01e0\u01e2\u01e4\u01e6\u01e8\u01ea\u01ec\u01ee\u01f1\u01f4\u01f6-\u01f8\u01fa\u01fc\u01fe\u0200\u0202\u0204\u0206\u0208\u020a\u020c\u020e\u0210\u0212\u0214\u0216\u0218\u021a\u021c\u021e\u0220\u0222\u0224\u0226\u0228\u022a\u022c\u022e\u0230\u0232\u023a-\u023b\u023d-\u023e\u0241\u0243-\u0246\u0248\u024a\u024c\u024e\u0370\u0372\u0376\u0386\u0388-\u038f\u0391-\u03ab\u03cf\u03d2-\u03d4\u03d8\u03da\u03dc\u03de\u03e0\u03e2\u03e4\u03e6\u03e8\u03ea\u03ec\u03ee\u03f4\u03f7\u03f9-\u03fa\u03fd-\u042f\u0460\u0462\u0464\u0466\u0468\u046a\u046c\u046e\u0470\u0472\u0474\u0476\u0478\u047a\u047c\u047e\u0480\u048a\u048c\u048e\u0490\u0492\u0494\u0496\u0498\u049a\u049c\u049e\u04a0\u04a2\u04a4\u04a6\u04a8\u04aa\u04ac\u04ae\u04b0\u04b2\u04b4\u04b6\u04b8\u04ba\u04bc\u04be\u04c0-\u04c1\u04c3\u04c5\u04c7\u04c9\u04cb\u04cd\u04d0\u04d2\u04d4\u04d6\u04d8\u04da\u04dc\u04de\u04e0\u04e2\u04e4\u04e6\u04e8\u04ea\u04ec\u04ee\u04f0\u04f2\u04f4\u04f6\u04f8\u04fa\u04fc\u04fe\u0500\u0502\u0504\u0506\u0508\u050a\u050c\u050e\u0510\u0512\u0514\u0516\u0518\u051a\u051c\u051e\u0520\u0522\u0531-\u0556\u10a0-\u10c5\u1e00\u1e02\u1e04\u1e06\u1e08\u1e0a\u1e0c\u1e0e\u1e10\u1e12\u1e14\u1e16\u1e18\u1e1a\u1e1c\u1e1e\u1e20\u1e22\u1e24\u1e26\u1e28\u1e2a\u1e2c\u1e2e\u1e30\u1e32\u1e34\u1e36\u1e38\u1e3a\u1e3c\u1e3e\u1e40\u1e42\u1e44\u1e46\u1e48\u1e4a\u1e4c\u1e4e\u1e50\u1e52\u1e54\u1e56\u1e58\u1e5a\u1e5c\u1e5e\u1e60\u1e62\u1e64\u1e66\u1e68\u1e6a\u1e6c\u1e6e\u1e70\u1e72\u1e74\u1e76\u1e78\u1e7a\u1e7c\u1e7e\u1e80\u1e82\u1e84\u1e86\u1e88\u1e8a\u1e8c\u1e8e\u1e90\u1e92\u1e94\u1e9e\u1ea0\u1ea2\u1ea4\u1ea6\u1ea8\u1eaa\u1eac\u1eae\u1eb0\u1eb2\u1eb4\u1eb6\u1eb8\u1eba\u1ebc\u1ebe\u1ec0\u1ec2\u1ec4\u1ec6\u1ec8\u1eca\u1ecc\u1ece\u1ed0\u1ed2\u1ed4\u1ed6\u1ed8\u1eda\u1edc\u1ede\u1ee0\u1ee2\u1ee4\u1ee6\u1ee8\u1eea\u1eec\u1eee\u1ef0\u1ef2\u1ef4\u1ef6\u1ef8\u1efa\u1efc\u1efe\u1f08-\u1f0f\u1f18-\u1f1d\u1f28-\u1f2f\u1f38-\u1f3f\u1f48-\u1f4d\u1f59-\u1f5f\u1f68-\u1f6f\u1fb8-\u1fbb\u1fc8-\u1fcb\u1fd8-\u1fdb\u1fe8-\u1fec\u1ff8-\u1ffb\u2102\u2107\u210b-\u210d\u2110-\u2112\u2115\u2119-\u211d\u2124\u2126\u2128\u212a-\u212d\u2130-\u2133\u213e-\u213f\u2145\u2183\u2c00-\u2c2e\u2c60\u2c62-\u2c64\u2c67\u2c69\u2c6b\u2c6d-\u2c6f\u2c72\u2c75\u2c80\u2c82\u2c84\u2c86\u2c88\u2c8a\u2c8c\u2c8e\u2c90\u2c92\u2c94\u2c96\u2c98\u2c9a\u2c9c\u2c9e\u2ca0\u2ca2\u2ca4\u2ca6\u2ca8\u2caa\u2cac\u2cae\u2cb0\u2cb2\u2cb4\u2cb6\u2cb8\u2cba\u2cbc\u2cbe\u2cc0\u2cc2\u2cc4\u2cc6\u2cc8\u2cca\u2ccc\u2cce\u2cd0\u2cd2\u2cd4\u2cd6\u2cd8\u2cda\u2cdc\u2cde\u2ce0\u2ce2\ua640\ua642\ua644\ua646\ua648\ua64a\ua64c\ua64e\ua650\ua652\ua654\ua656\ua658\ua65a\ua65c\ua65e\ua662\ua664\ua666\ua668\ua66a\ua66c\ua680\ua682\ua684\ua686\ua688\ua68a\ua68c\ua68e\ua690\ua692\ua694\ua696\ua722\ua724\ua726\ua728\ua72a\ua72c\ua72e\ua732\ua734\ua736\ua738\ua73a\ua73c\ua73e\ua740\ua742\ua744\ua746\ua748\ua74a\ua74c\ua74e\ua750\ua752\ua754\ua756\ua758\ua75a\ua75c\ua75e\ua760\ua762\ua764\ua766\ua768\ua76a\ua76c\ua76e\ua779\ua77b\ua77d-\ua77e\ua780\ua782\ua784\ua786\ua78b\uff21-\uff3a]"
- scalaLetter = `[a-zA-Z\\$_ªµºÀ-ÖØ-öø-ʯͰ-ͳͶ-ͷͻ-ͽΆΈ-ϵϷ-ҁҊ-Ֆա-ևא-ײء-ؿف-يٮ-ٯٱ-ۓەۮ-ۯۺ-ۼۿܐܒ-ܯݍ-ޥޱߊ-ߪऄ-हऽॐक़-ॡॲ-ॿঅ-হঽৎড়-ৡৰ-ৱਅ-ਹਖ਼-ਫ਼ੲ-ੴઅ-હઽૐ-ૡଅ-ହଽଡ଼-ୡୱஃ-ஹௐఅ-ఽౘ-ౡಅ-ಹಽೞ-ೡഅ-ഽൠ-ൡൺ-ൿඅ-ෆก-ะา-ำเ-ๅກ-ະາ-ຳຽ-ໄໜ-ༀཀ-ཬྈ-ྋက-ဪဿၐ-ၕၚ-ၝၡၥ-ၦၮ-ၰၵ-ႁႎႠ-ჺᄀ-ፚᎀ-ᎏᎠ-ᙬᙯ-ᙶᚁ-ᚚᚠ-ᛪᛮ-ᜑᜠ-ᜱᝀ-ᝑᝠ-ᝰក-ឳៜᠠ-ᡂᡄ-ᢨᢪ-ᤜᥐ-ᦩᧁ-ᧇᨀ-ᨖᬅ-ᬳᭅ-ᭋᮃ-ᮠᮮ-ᮯᰀ-ᰣᱍ-ᱏᱚ-ᱷᴀ-ᴫᵢ-ᵷᵹ-ᶚḀ-ᾼιῂ-ῌῐ-Ίῠ-Ῥῲ-ῼⁱⁿℂℇℊ-ℓℕℙ-ℝℤΩℨK-ℭℯ-ℹℼ-ℿⅅ-ⅉⅎⅠ-ↈⰀ-ⱼⲀ-ⳤⴀ-ⵥⶀ-ⷞ〆-〇〡-〩〸-〺〼ぁ-ゖゟァ-ヺヿ-ㆎㆠ-ㆷㇰ-ㇿ㐀-䶵一-ꀔꀖ-ꒌꔀ-ꘋꘐ-ꘟꘪ-ꙮꚀ-ꚗꜢ-ꝯꝱ-ꞇꞋ-ꠁꠃ-ꠅꠇ-ꠊꠌ-ꠢꡀ-ꡳꢂ-ꢳꤊ-ꤥꤰ-ꥆꨀ-ꨨꩀ-ꩂꩄ-ꩋ가-힣豈-יִײַ-ﬨשׁ-ﴽﵐ-ﷻﹰ-ﻼA-Za-zヲ-ッア-ンᅠ-ᅵ]`
- scalaIDRest = fmt.Sprintf(`%s(?:%s|[0-9])*(?:(?<=_)%s)?`, scalaLetter, scalaLetter, scalaOp)
- )
-
- return Rules{
- "root": {
- {`(class|trait|object)(\s+)`, ByGroups(Keyword, Text), Push("class")},
- {`[^\S\n]+`, Text, nil},
- {`//.*?\n`, CommentSingle, nil},
- {`/\*`, CommentMultiline, Push("comment")},
- {`@` + scalaIDRest, NameDecorator, nil},
- {`(abstract|ca(?:se|tch)|d(?:ef|o)|e(?:lse|xtends)|f(?:inal(?:ly)?|or(?:Some)?)|i(?:f|mplicit)|lazy|match|new|override|pr(?:ivate|otected)|re(?:quires|turn)|s(?:ealed|uper)|t(?:h(?:is|row)|ry)|va[lr]|w(?:hile|ith)|yield)\b|(<[%:-]|=>|>:|[#=@_⇒←])(\b|(?=\s)|$)`, Keyword, nil},
- {`:(?!` + scalaOp + `%s)`, Keyword, Push("type")},
- {fmt.Sprintf("%s%s\\b", scalaUpper, scalaIDRest), NameClass, nil},
- {`(true|false|null)\b`, KeywordConstant, nil},
- {`(import|package)(\s+)`, ByGroups(Keyword, Text), Push("import")},
- {`(type)(\s+)`, ByGroups(Keyword, Text), Push("type")},
- {`""".*?"""(?!")`, LiteralString, nil},
- {`"(\\\\|\\"|[^"])*"`, LiteralString, nil},
- {`'\\.'|'[^\\]'|'\\u[0-9a-fA-F]{4}'`, LiteralStringChar, nil},
- {"'" + scalaIDRest, TextSymbol, nil},
- {`[fs]"""`, LiteralString, Push("interptriplestring")},
- {`[fs]"`, LiteralString, Push("interpstring")},
- {`raw"(\\\\|\\"|[^"])*"`, LiteralString, nil},
- {scalaIDRest, Name, nil},
- {"`[^`]+`", Name, nil},
- {`\[`, Operator, Push("typeparam")},
- {`[(){};,.#]`, Operator, nil},
- {scalaOp, Operator, nil},
- {`([0-9][0-9]*\.[0-9]*|\.[0-9]+)([eE][+-]?[0-9]+)?[fFdD]?`, LiteralNumberFloat, nil},
- {`0x[0-9a-fA-F]+`, LiteralNumberHex, nil},
- {`[0-9]+L?`, LiteralNumberInteger, nil},
- {`\n`, Text, nil},
- },
- "class": {
- {fmt.Sprintf("(%s|%s|`[^`]+`)(\\s*)(\\[)", scalaIDRest, scalaOp), ByGroups(NameClass, Text, Operator), Push("typeparam")},
- {`\s+`, Text, nil},
- {`\{`, Operator, Pop(1)},
- {`\(`, Operator, Pop(1)},
- {`//.*?\n`, CommentSingle, Pop(1)},
- {fmt.Sprintf("%s|%s|`[^`]+`", scalaIDRest, scalaOp), NameClass, Pop(1)},
- },
- "type": {
- {`\s+`, Text, nil},
- {`<[%:]|>:|[#_]|forSome|type`, Keyword, nil},
- {`([,);}]|=>|=|⇒)(\s*)`, ByGroups(Operator, Text), Pop(1)},
- {`[({]`, Operator, Push()},
- {fmt.Sprintf("((?:%s|%s|`[^`]+`)(?:\\.(?:%s|%s|`[^`]+`))*)(\\s*)(\\[)", scalaIDRest, scalaOp, scalaIDRest, scalaOp), ByGroups(KeywordType, Text, Operator), Push("#pop", "typeparam")},
- {fmt.Sprintf("((?:%s|%s|`[^`]+`)(?:\\.(?:%s|%s|`[^`]+`))*)(\\s*)$", scalaIDRest, scalaOp, scalaIDRest, scalaOp), ByGroups(KeywordType, Text), Pop(1)},
- {`//.*?\n`, CommentSingle, Pop(1)},
- {fmt.Sprintf("\\.|%s|%s|`[^`]+`", scalaIDRest, scalaOp), KeywordType, nil},
- },
- "typeparam": {
- {`[\s,]+`, Text, nil},
- {`<[%:]|=>|>:|[#_⇒]|forSome|type`, Keyword, nil},
- {`([\])}])`, Operator, Pop(1)},
- {`[(\[{]`, Operator, Push()},
- {fmt.Sprintf("\\.|%s|%s|`[^`]+`", scalaIDRest, scalaOp), KeywordType, nil},
- },
- "comment": {
- {`[^/*]+`, CommentMultiline, nil},
- {`/\*`, CommentMultiline, Push()},
- {`\*/`, CommentMultiline, Pop(1)},
- {`[*/]`, CommentMultiline, nil},
- },
- "import": {
- {fmt.Sprintf("(%s|\\.)+", scalaIDRest), NameNamespace, Pop(1)},
- },
- "interpstringcommon": {
- {`[^"$\\]+`, LiteralString, nil},
- {`\$\$`, LiteralString, nil},
- {`\$` + scalaLetter + `(?:` + scalaLetter + `|\d)*`, LiteralStringInterpol, nil},
- {`\$\{`, LiteralStringInterpol, Push("interpbrace")},
- {`\\.`, LiteralString, nil},
- },
- "interptriplestring": {
- {`"""(?!")`, LiteralString, Pop(1)},
- {`"`, LiteralString, nil},
- Include("interpstringcommon"),
- },
- "interpstring": {
- {`"`, LiteralString, Pop(1)},
- Include("interpstringcommon"),
- },
- "interpbrace": {
- {`\}`, LiteralStringInterpol, Pop(1)},
- {`\{`, LiteralStringInterpol, Push()},
- Include("root"),
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/s/scheme.go b/vendor/github.com/alecthomas/chroma/lexers/s/scheme.go
deleted file mode 100644
index d2f177e..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/s/scheme.go
+++ /dev/null
@@ -1,57 +0,0 @@
-package s
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-// nolint
-
-// Scheme lexer.
-var SchemeLang = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "Scheme",
- Aliases: []string{"scheme", "scm"},
- Filenames: []string{"*.scm", "*.ss"},
- MimeTypes: []string{"text/x-scheme", "application/x-scheme"},
- },
- schemeLangRules,
-))
-
-func schemeLangRules() Rules {
- return Rules{
- "root": {
- {`;.*$`, CommentSingle, nil},
- {`#\|`, CommentMultiline, Push("multiline-comment")},
- {`#;\s*\(`, Comment, Push("commented-form")},
- {`#!r6rs`, Comment, nil},
- {`\s+`, Text, nil},
- {`-?\d+\.\d+`, LiteralNumberFloat, nil},
- {`-?\d+`, LiteralNumberInteger, nil},
- {`"(\\\\|\\"|[^"])*"`, LiteralString, nil},
- {`'[\w!$%&*+,/:<=>?@^~|-]+`, LiteralStringSymbol, nil},
- {`#\\([()/'\"._!§$%& ?=+-]|[a-zA-Z0-9]+)`, LiteralStringChar, nil},
- {`(#t|#f)`, NameConstant, nil},
- {"('|#|`|,@|,|\\.)", Operator, nil},
- {`(lambda |define |if |else |cond |and |or |case |let |let\* |letrec |begin |do |delay |set\! |\=\> |quote |quasiquote |unquote |unquote\-splicing |define\-syntax |let\-syntax |letrec\-syntax |syntax\-rules )`, Keyword, nil},
- {`(?<='\()[\w!$%&*+,/:<=>?@^~|-]+`, NameVariable, nil},
- {`(?<=#\()[\w!$%&*+,/:<=>?@^~|-]+`, NameVariable, nil},
- {`(?<=\()(\* |\+ |\- |\/ |\< |\<\= |\= |\> |\>\= |abs |acos |angle |append |apply |asin |assoc |assq |assv |atan |boolean\? |caaaar |caaadr |caaar |caadar |caaddr |caadr |caar |cadaar |cadadr |cadar |caddar |cadddr |caddr |cadr |call\-with\-current\-continuation |call\-with\-input\-file |call\-with\-output\-file |call\-with\-values |call\/cc |car |cdaaar |cdaadr |cdaar |cdadar |cdaddr |cdadr |cdar |cddaar |cddadr |cddar |cdddar |cddddr |cdddr |cddr |cdr |ceiling |char\-\>integer |char\-alphabetic\? |char\-ci\<\=\? |char\-ci\<\? |char\-ci\=\? |char\-ci\>\=\? |char\-ci\>\? |char\-downcase |char\-lower\-case\? |char\-numeric\? |char\-ready\? |char\-upcase |char\-upper\-case\? |char\-whitespace\? |char\<\=\? |char\<\? |char\=\? |char\>\=\? |char\>\? |char\? |close\-input\-port |close\-output\-port |complex\? |cons |cos |current\-input\-port |current\-output\-port |denominator |display |dynamic\-wind |eof\-object\? |eq\? |equal\? |eqv\? |eval |even\? |exact\-\>inexact |exact\? |exp |expt |floor |for\-each |force |gcd |imag\-part |inexact\-\>exact |inexact\? |input\-port\? |integer\-\>char |integer\? |interaction\-environment |lcm |length |list |list\-\>string |list\-\>vector |list\-ref |list\-tail |list\? |load |log |magnitude |make\-polar |make\-rectangular |make\-string |make\-vector |map |max |member |memq |memv |min |modulo |negative\? |newline |not |null\-environment |null\? |number\-\>string |number\? |numerator |odd\? |open\-input\-file |open\-output\-file |output\-port\? |pair\? |peek\-char |port\? |positive\? |procedure\? |quotient |rational\? |rationalize |read |read\-char |real\-part |real\? |remainder |reverse |round |scheme\-report\-environment |set\-car\! |set\-cdr\! |sin |sqrt |string |string\-\>list |string\-\>number |string\-\>symbol |string\-append |string\-ci\<\=\? |string\-ci\<\? |string\-ci\=\? |string\-ci\>\=\? |string\-ci\>\? |string\-copy |string\-fill\! |string\-length |string\-ref |string\-set\! |string\<\=\? |string\<\? |string\=\? |string\>\=\? |string\>\? |string\? |substring |symbol\-\>string |symbol\? |tan |transcript\-off |transcript\-on |truncate |values |vector |vector\-\>list |vector\-fill\! |vector\-length |vector\-ref |vector\-set\! |vector\? |with\-input\-from\-file |with\-output\-to\-file |write |write\-char |zero\? )`, NameBuiltin, nil},
- {`(?<=\()[\w!$%&*+,/:<=>?@^~|-]+`, NameFunction, nil},
- {`[\w!$%&*+,/:<=>?@^~|-]+`, NameVariable, nil},
- {`(\(|\))`, Punctuation, nil},
- {`(\[|\])`, Punctuation, nil},
- },
- "multiline-comment": {
- {`#\|`, CommentMultiline, Push()},
- {`\|#`, CommentMultiline, Pop(1)},
- {`[^|#]+`, CommentMultiline, nil},
- {`[|#]`, CommentMultiline, nil},
- },
- "commented-form": {
- {`\(`, Comment, Push()},
- {`\)`, Comment, Pop(1)},
- {`[^()]+`, Comment, nil},
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/s/scilab.go b/vendor/github.com/alecthomas/chroma/lexers/s/scilab.go
deleted file mode 100644
index f816492..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/s/scilab.go
+++ /dev/null
@@ -1,48 +0,0 @@
-package s
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-// Scilab lexer.
-var Scilab = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "Scilab",
- Aliases: []string{"scilab"},
- Filenames: []string{"*.sci", "*.sce", "*.tst"},
- MimeTypes: []string{"text/scilab"},
- },
- scilabRules,
-))
-
-func scilabRules() Rules {
- return Rules{
- "root": {
- {`//.*?$`, CommentSingle, nil},
- {`^\s*function`, Keyword, Push("deffunc")},
- {Words(``, `\b`, `__FILE__`, `__LINE__`, `break`, `case`, `catch`, `classdef`, `continue`, `do`, `else`, `elseif`, `end`, `end_try_catch`, `end_unwind_protect`, `endclassdef`, `endevents`, `endfor`, `endfunction`, `endif`, `endmethods`, `endproperties`, `endswitch`, `endwhile`, `events`, `for`, `function`, `get`, `global`, `if`, `methods`, `otherwise`, `persistent`, `properties`, `return`, `set`, `static`, `switch`, `try`, `until`, `unwind_protect`, `unwind_protect_cleanup`, `while`), Keyword, nil},
- {Words(``, `\b`, `!!_invoke_`, `%H5Object_e`, `%H5Object_fieldnames`, `%H5Object_p`, `%XMLAttr_6`, `%XMLAttr_e`, `%XMLAttr_i_XMLElem`, `%XMLAttr_length`, `%XMLAttr_p`, `%XMLAttr_size`, `%XMLDoc_6`, `%XMLDoc_e`, `%XMLDoc_i_XMLList`, `%XMLDoc_p`, `%XMLElem_6`, `%XMLElem_e`, `%XMLElem_i_XMLDoc`, `%XMLElem_i_XMLElem`, `%XMLElem_i_XMLList`, `%XMLElem_p`, `%XMLList_6`, `%XMLList_e`, `%XMLList_i_XMLElem`, `%XMLList_i_XMLList`, `%XMLList_length`, `%XMLList_p`, `%XMLList_size`, `%XMLNs_6`, `%XMLNs_e`, `%XMLNs_i_XMLElem`, `%XMLNs_p`, `%XMLSet_6`, `%XMLSet_e`, `%XMLSet_length`, `%XMLSet_p`, `%XMLSet_size`, `%XMLValid_p`, `%_EClass_6`, `%_EClass_e`, `%_EClass_p`, `%_EObj_0`, `%_EObj_1__EObj`, `%_EObj_1_b`, `%_EObj_1_c`, `%_EObj_1_i`, `%_EObj_1_s`, `%_EObj_2__EObj`, `%_EObj_2_b`, `%_EObj_2_c`, `%_EObj_2_i`, `%_EObj_2_s`, `%_EObj_3__EObj`, `%_EObj_3_b`, `%_EObj_3_c`, `%_EObj_3_i`, `%_EObj_3_s`, `%_EObj_4__EObj`, `%_EObj_4_b`, `%_EObj_4_c`, `%_EObj_4_i`, `%_EObj_4_s`, `%_EObj_5`, `%_EObj_6`, `%_EObj_a__EObj`, `%_EObj_a_b`, `%_EObj_a_c`, `%_EObj_a_i`, `%_EObj_a_s`, `%_EObj_d__EObj`, `%_EObj_d_b`, `%_EObj_d_c`, `%_EObj_d_i`, `%_EObj_d_s`, `%_EObj_disp`, `%_EObj_e`, `%_EObj_g__EObj`, `%_EObj_g_b`, `%_EObj_g_c`, `%_EObj_g_i`, `%_EObj_g_s`, `%_EObj_h__EObj`, `%_EObj_h_b`, `%_EObj_h_c`, `%_EObj_h_i`, `%_EObj_h_s`, `%_EObj_i__EObj`, `%_EObj_j__EObj`, `%_EObj_j_b`, `%_EObj_j_c`, `%_EObj_j_i`, `%_EObj_j_s`, `%_EObj_k__EObj`, `%_EObj_k_b`, `%_EObj_k_c`, `%_EObj_k_i`, `%_EObj_k_s`, `%_EObj_l__EObj`, `%_EObj_l_b`, `%_EObj_l_c`, `%_EObj_l_i`, `%_EObj_l_s`, `%_EObj_m__EObj`, `%_EObj_m_b`, `%_EObj_m_c`, `%_EObj_m_i`, `%_EObj_m_s`, `%_EObj_n__EObj`, `%_EObj_n_b`, `%_EObj_n_c`, `%_EObj_n_i`, `%_EObj_n_s`, `%_EObj_o__EObj`, `%_EObj_o_b`, `%_EObj_o_c`, `%_EObj_o_i`, `%_EObj_o_s`, `%_EObj_p`, `%_EObj_p__EObj`, `%_EObj_p_b`, `%_EObj_p_c`, `%_EObj_p_i`, `%_EObj_p_s`, `%_EObj_q__EObj`, `%_EObj_q_b`, `%_EObj_q_c`, `%_EObj_q_i`, `%_EObj_q_s`, `%_EObj_r__EObj`, `%_EObj_r_b`, `%_EObj_r_c`, `%_EObj_r_i`, `%_EObj_r_s`, `%_EObj_s__EObj`, `%_EObj_s_b`, `%_EObj_s_c`, `%_EObj_s_i`, `%_EObj_s_s`, `%_EObj_t`, `%_EObj_x__EObj`, `%_EObj_x_b`, `%_EObj_x_c`, `%_EObj_x_i`, `%_EObj_x_s`, `%_EObj_y__EObj`, `%_EObj_y_b`, `%_EObj_y_c`, `%_EObj_y_i`, `%_EObj_y_s`, `%_EObj_z__EObj`, `%_EObj_z_b`, `%_EObj_z_c`, `%_EObj_z_i`, `%_EObj_z_s`, `%_eigs`, `%_load`, `%b_1__EObj`, `%b_2__EObj`, `%b_3__EObj`, `%b_4__EObj`, `%b_a__EObj`, `%b_d__EObj`, `%b_g__EObj`, `%b_h__EObj`, `%b_i_XMLList`, `%b_i__EObj`, `%b_j__EObj`, `%b_k__EObj`, `%b_l__EObj`, `%b_m__EObj`, `%b_n__EObj`, `%b_o__EObj`, `%b_p__EObj`, `%b_q__EObj`, `%b_r__EObj`, `%b_s__EObj`, `%b_x__EObj`, `%b_y__EObj`, `%b_z__EObj`, `%c_1__EObj`, `%c_2__EObj`, `%c_3__EObj`, `%c_4__EObj`, `%c_a__EObj`, `%c_d__EObj`, `%c_g__EObj`, `%c_h__EObj`, `%c_i_XMLAttr`, `%c_i_XMLDoc`, `%c_i_XMLElem`, `%c_i_XMLList`, `%c_i__EObj`, `%c_j__EObj`, `%c_k__EObj`, `%c_l__EObj`, `%c_m__EObj`, `%c_n__EObj`, `%c_o__EObj`, `%c_p__EObj`, `%c_q__EObj`, `%c_r__EObj`, `%c_s__EObj`, `%c_x__EObj`, `%c_y__EObj`, `%c_z__EObj`, `%ce_i_XMLList`, `%fptr_i_XMLList`, `%h_i_XMLList`, `%hm_i_XMLList`, `%i_1__EObj`, `%i_2__EObj`, `%i_3__EObj`, `%i_4__EObj`, `%i_a__EObj`, `%i_abs`, `%i_cumprod`, `%i_cumsum`, `%i_d__EObj`, `%i_diag`, `%i_g__EObj`, `%i_h__EObj`, `%i_i_XMLList`, `%i_i__EObj`, `%i_j__EObj`, `%i_k__EObj`, `%i_l__EObj`, `%i_m__EObj`, `%i_matrix`, `%i_max`, `%i_maxi`, `%i_min`, `%i_mini`, `%i_mput`, `%i_n__EObj`, `%i_o__EObj`, `%i_p`, `%i_p__EObj`, `%i_prod`, `%i_q__EObj`, `%i_r__EObj`, `%i_s__EObj`, `%i_sum`, `%i_tril`, `%i_triu`, `%i_x__EObj`, `%i_y__EObj`, `%i_z__EObj`, `%ip_i_XMLList`, `%l_i_XMLList`, `%l_i__EObj`, `%lss_i_XMLList`, `%mc_i_XMLList`, `%msp_full`, `%msp_i_XMLList`, `%msp_spget`, `%p_i_XMLList`, `%ptr_i_XMLList`, `%r_i_XMLList`, `%s_1__EObj`, `%s_2__EObj`, `%s_3__EObj`, `%s_4__EObj`, `%s_a__EObj`, `%s_d__EObj`, `%s_g__EObj`, `%s_h__EObj`, `%s_i_XMLList`, `%s_i__EObj`, `%s_j__EObj`, `%s_k__EObj`, `%s_l__EObj`, `%s_m__EObj`, `%s_n__EObj`, `%s_o__EObj`, `%s_p__EObj`, `%s_q__EObj`, `%s_r__EObj`, `%s_s__EObj`, `%s_x__EObj`, `%s_y__EObj`, `%s_z__EObj`, `%sp_i_XMLList`, `%spb_i_XMLList`, `%st_i_XMLList`, `Calendar`, `ClipBoard`, `Matplot`, `Matplot1`, `PlaySound`, `TCL_DeleteInterp`, `TCL_DoOneEvent`, `TCL_EvalFile`, `TCL_EvalStr`, `TCL_ExistArray`, `TCL_ExistInterp`, `TCL_ExistVar`, `TCL_GetVar`, `TCL_GetVersion`, `TCL_SetVar`, `TCL_UnsetVar`, `TCL_UpVar`, `_`, `_code2str`, `_d`, `_str2code`, `about`, `abs`, `acos`, `addModulePreferences`, `addcolor`, `addf`, `addhistory`, `addinter`, `addlocalizationdomain`, `amell`, `and`, `argn`, `arl2_ius`, `ascii`, `asin`, `atan`, `backslash`, `balanc`, `banner`, `base2dec`, `basename`, `bdiag`, `beep`, `besselh`, `besseli`, `besselj`, `besselk`, `bessely`, `beta`, `bezout`, `bfinit`, `blkfc1i`, `blkslvi`, `bool2s`, `browsehistory`, `browsevar`, `bsplin3val`, `buildDoc`, `buildouttb`, `bvode`, `c_link`, `call`, `callblk`, `captions`, `cd`, `cdfbet`, `cdfbin`, `cdfchi`, `cdfchn`, `cdff`, `cdffnc`, `cdfgam`, `cdfnbn`, `cdfnor`, `cdfpoi`, `cdft`, `ceil`, `champ`, `champ1`, `chdir`, `chol`, `clc`, `clean`, `clear`, `clearfun`, `clearglobal`, `closeEditor`, `closeEditvar`, `closeXcos`, `code2str`, `coeff`, `color`, `comp`, `completion`, `conj`, `contour2di`, `contr`, `conv2`, `convstr`, `copy`, `copyfile`, `corr`, `cos`, `coserror`, `createdir`, `cshep2d`, `csvDefault`, `csvIsnum`, `csvRead`, `csvStringToDouble`, `csvTextScan`, `csvWrite`, `ctree2`, `ctree3`, `ctree4`, `cumprod`, `cumsum`, `curblock`, `curblockc`, `daskr`, `dasrt`, `dassl`, `data2sig`, `datatipCreate`, `datatipManagerMode`, `datatipMove`, `datatipRemove`, `datatipSetDisplay`, `datatipSetInterp`, `datatipSetOrientation`, `datatipSetStyle`, `datatipToggle`, `dawson`, `dct`, `debug`, `dec2base`, `deff`, `definedfields`, `degree`, `delbpt`, `delete`, `deletefile`, `delip`, `delmenu`, `det`, `dgettext`, `dhinf`, `diag`, `diary`, `diffobjs`, `disp`, `dispbpt`, `displayhistory`, `disposefftwlibrary`, `dlgamma`, `dnaupd`, `dneupd`, `double`, `drawaxis`, `drawlater`, `drawnow`, `driver`, `dsaupd`, `dsearch`, `dseupd`, `dst`, `duplicate`, `editvar`, `emptystr`, `end_scicosim`, `ereduc`, `erf`, `erfc`, `erfcx`, `erfi`, `errcatch`, `errclear`, `error`, `eval_cshep2d`, `exec`, `execstr`, `exists`, `exit`, `exp`, `expm`, `exportUI`, `export_to_hdf5`, `eye`, `fadj2sp`, `fec`, `feval`, `fft`, `fftw`, `fftw_flags`, `fftw_forget_wisdom`, `fftwlibraryisloaded`, `figure`, `file`, `filebrowser`, `fileext`, `fileinfo`, `fileparts`, `filesep`, `find`, `findBD`, `findfiles`, `fire_closing_finished`, `floor`, `format`, `fort`, `fprintfMat`, `freq`, `frexp`, `fromc`, `fromjava`, `fscanfMat`, `fsolve`, `fstair`, `full`, `fullpath`, `funcprot`, `funptr`, `gamma`, `gammaln`, `geom3d`, `get`, `getURL`, `get_absolute_file_path`, `get_fftw_wisdom`, `getblocklabel`, `getcallbackobject`, `getdate`, `getdebuginfo`, `getdefaultlanguage`, `getdrives`, `getdynlibext`, `getenv`, `getfield`, `gethistory`, `gethistoryfile`, `getinstalledlookandfeels`, `getio`, `getlanguage`, `getlongpathname`, `getlookandfeel`, `getmd5`, `getmemory`, `getmodules`, `getos`, `getpid`, `getrelativefilename`, `getscicosvars`, `getscilabmode`, `getshortpathname`, `gettext`, `getvariablesonstack`, `getversion`, `glist`, `global`, `glue`, `grand`, `graphicfunction`, `grayplot`, `grep`, `gsort`, `gstacksize`, `h5attr`, `h5close`, `h5cp`, `h5dataset`, `h5dump`, `h5exists`, `h5flush`, `h5get`, `h5group`, `h5isArray`, `h5isAttr`, `h5isCompound`, `h5isFile`, `h5isGroup`, `h5isList`, `h5isRef`, `h5isSet`, `h5isSpace`, `h5isType`, `h5isVlen`, `h5label`, `h5ln`, `h5ls`, `h5mount`, `h5mv`, `h5open`, `h5read`, `h5readattr`, `h5rm`, `h5umount`, `h5write`, `h5writeattr`, `havewindow`, `helpbrowser`, `hess`, `hinf`, `historymanager`, `historysize`, `host`, `htmlDump`, `htmlRead`, `htmlReadStr`, `htmlWrite`, `iconvert`, `ieee`, `ilib_verbose`, `imag`, `impl`, `import_from_hdf5`, `imult`, `inpnvi`, `int`, `int16`, `int2d`, `int32`, `int3d`, `int8`, `interp`, `interp2d`, `interp3d`, `intg`, `intppty`, `inttype`, `inv`, `invoke_lu`, `is_handle_valid`, `is_hdf5_file`, `isalphanum`, `isascii`, `isdef`, `isdigit`, `isdir`, `isequal`, `isequalbitwise`, `iserror`, `isfile`, `isglobal`, `isletter`, `isnum`, `isreal`, `iswaitingforinput`, `jallowClassReloading`, `jarray`, `jautoTranspose`, `jautoUnwrap`, `javaclasspath`, `javalibrarypath`, `jcast`, `jcompile`, `jconvMatrixMethod`, `jcreatejar`, `jdeff`, `jdisableTrace`, `jenableTrace`, `jexists`, `jgetclassname`, `jgetfield`, `jgetfields`, `jgetinfo`, `jgetmethods`, `jimport`, `jinvoke`, `jinvoke_db`, `jnewInstance`, `jremove`, `jsetfield`, `junwrap`, `junwraprem`, `jwrap`, `jwrapinfloat`, `kron`, `lasterror`, `ldiv`, `ldivf`, `legendre`, `length`, `lib`, `librarieslist`, `libraryinfo`, `light`, `linear_interpn`, `lines`, `link`, `linmeq`, `list`, `listvar_in_hdf5`, `load`, `loadGui`, `loadScicos`, `loadXcos`, `loadfftwlibrary`, `loadhistory`, `log`, `log1p`, `lsq`, `lsq_splin`, `lsqrsolve`, `lsslist`, `lstcat`, `lstsize`, `ltitr`, `lu`, `ludel`, `lufact`, `luget`, `lusolve`, `macr2lst`, `macr2tree`, `matfile_close`, `matfile_listvar`, `matfile_open`, `matfile_varreadnext`, `matfile_varwrite`, `matrix`, `max`, `maxfiles`, `mclearerr`, `mclose`, `meof`, `merror`, `messagebox`, `mfprintf`, `mfscanf`, `mget`, `mgeti`, `mgetl`, `mgetstr`, `min`, `mlist`, `mode`, `model2blk`, `mopen`, `move`, `movefile`, `mprintf`, `mput`, `mputl`, `mputstr`, `mscanf`, `mseek`, `msprintf`, `msscanf`, `mtell`, `mtlb_mode`, `mtlb_sparse`, `mucomp`, `mulf`, `name2rgb`, `nearfloat`, `newaxes`, `newest`, `newfun`, `nnz`, `norm`, `notify`, `number_properties`, `ode`, `odedc`, `ones`, `openged`, `opentk`, `optim`, `or`, `ordmmd`, `parallel_concurrency`, `parallel_run`, `param3d`, `param3d1`, `part`, `pathconvert`, `pathsep`, `phase_simulation`, `plot2d`, `plot2d1`, `plot2d2`, `plot2d3`, `plot2d4`, `plot3d`, `plot3d1`, `plotbrowser`, `pointer_xproperty`, `poly`, `ppol`, `pppdiv`, `predef`, `preferences`, `print`, `printf`, `printfigure`, `printsetupbox`, `prod`, `progressionbar`, `prompt`, `pwd`, `qld`, `qp_solve`, `qr`, `raise_window`, `rand`, `rankqr`, `rat`, `rcond`, `rdivf`, `read`, `read4b`, `read_csv`, `readb`, `readgateway`, `readmps`, `real`, `realtime`, `realtimeinit`, `regexp`, `relocate_handle`, `remez`, `removeModulePreferences`, `removedir`, `removelinehistory`, `res_with_prec`, `resethistory`, `residu`, `resume`, `return`, `ricc`, `rlist`, `roots`, `rotate_axes`, `round`, `rpem`, `rtitr`, `rubberbox`, `save`, `saveGui`, `saveafterncommands`, `saveconsecutivecommands`, `savehistory`, `schur`, `sci_haltscicos`, `sci_tree2`, `sci_tree3`, `sci_tree4`, `sciargs`, `scicos_debug`, `scicos_debug_count`, `scicos_time`, `scicosim`, `scinotes`, `sctree`, `semidef`, `set`, `set_blockerror`, `set_fftw_wisdom`, `set_xproperty`, `setbpt`, `setdefaultlanguage`, `setenv`, `setfield`, `sethistoryfile`, `setlanguage`, `setlookandfeel`, `setmenu`, `sfact`, `sfinit`, `show_window`, `sident`, `sig2data`, `sign`, `simp`, `simp_mode`, `sin`, `size`, `slash`, `sleep`, `sorder`, `sparse`, `spchol`, `spcompack`, `spec`, `spget`, `splin`, `splin2d`, `splin3d`, `splitURL`, `spones`, `sprintf`, `sqrt`, `stacksize`, `str2code`, `strcat`, `strchr`, `strcmp`, `strcspn`, `strindex`, `string`, `stringbox`, `stripblanks`, `strncpy`, `strrchr`, `strrev`, `strsplit`, `strspn`, `strstr`, `strsubst`, `strtod`, `strtok`, `subf`, `sum`, `svd`, `swap_handles`, `symfcti`, `syredi`, `system_getproperty`, `system_setproperty`, `ta2lpd`, `tan`, `taucs_chdel`, `taucs_chfact`, `taucs_chget`, `taucs_chinfo`, `taucs_chsolve`, `tempname`, `testmatrix`, `timer`, `tlist`, `tohome`, `tokens`, `toolbar`, `toprint`, `tr_zer`, `tril`, `triu`, `type`, `typename`, `uiDisplayTree`, `uicontextmenu`, `uicontrol`, `uigetcolor`, `uigetdir`, `uigetfile`, `uigetfont`, `uimenu`, `uint16`, `uint32`, `uint8`, `uipopup`, `uiputfile`, `uiwait`, `ulink`, `umf_ludel`, `umf_lufact`, `umf_luget`, `umf_luinfo`, `umf_lusolve`, `umfpack`, `unglue`, `unix`, `unsetmenu`, `unzoom`, `updatebrowsevar`, `usecanvas`, `useeditor`, `user`, `var2vec`, `varn`, `vec2var`, `waitbar`, `warnBlockByUID`, `warning`, `what`, `where`, `whereis`, `who`, `winsid`, `with_module`, `writb`, `write`, `write4b`, `write_csv`, `x_choose`, `x_choose_modeless`, `x_dialog`, `x_mdialog`, `xarc`, `xarcs`, `xarrows`, `xchange`, `xchoicesi`, `xclick`, `xcos`, `xcosAddToolsMenu`, `xcosConfigureXmlFile`, `xcosDiagramToScilab`, `xcosPalCategoryAdd`, `xcosPalDelete`, `xcosPalDisable`, `xcosPalEnable`, `xcosPalGenerateIcon`, `xcosPalGet`, `xcosPalLoad`, `xcosPalMove`, `xcosSimulationStarted`, `xcosUpdateBlock`, `xdel`, `xend`, `xfarc`, `xfarcs`, `xfpoly`, `xfpolys`, `xfrect`, `xget`, `xgetmouse`, `xgraduate`, `xgrid`, `xinit`, `xlfont`, `xls_open`, `xls_read`, `xmlAddNs`, `xmlAppend`, `xmlAsNumber`, `xmlAsText`, `xmlDTD`, `xmlDelete`, `xmlDocument`, `xmlDump`, `xmlElement`, `xmlFormat`, `xmlGetNsByHref`, `xmlGetNsByPrefix`, `xmlGetOpenDocs`, `xmlIsValidObject`, `xmlName`, `xmlNs`, `xmlRead`, `xmlReadStr`, `xmlRelaxNG`, `xmlRemove`, `xmlSchema`, `xmlSetAttributes`, `xmlValidate`, `xmlWrite`, `xmlXPath`, `xname`, `xpause`, `xpoly`, `xpolys`, `xrect`, `xrects`, `xs2bmp`, `xs2emf`, `xs2eps`, `xs2gif`, `xs2jpg`, `xs2pdf`, `xs2png`, `xs2ppm`, `xs2ps`, `xs2svg`, `xsegs`, `xset`, `xstring`, `xstringb`, `xtitle`, `zeros`, `znaupd`, `zneupd`, `zoom_rect`, `abort`, `apropos`, `break`, `case`, `catch`, `continue`, `do`, `else`, `elseif`, `end`, `endfunction`, `for`, `function`, `help`, `if`, `pause`, `quit`, `select`, `then`, `try`, `while`, `!_deff_wrapper`, `%0_i_st`, `%3d_i_h`, `%Block_xcosUpdateBlock`, `%TNELDER_p`, `%TNELDER_string`, `%TNMPLOT_p`, `%TNMPLOT_string`, `%TOPTIM_p`, `%TOPTIM_string`, `%TSIMPLEX_p`, `%TSIMPLEX_string`, `%_EVoid_p`, `%_gsort`, `%_listvarinfile`, `%_rlist`, `%_save`, `%_sodload`, `%_strsplit`, `%_unwrap`, `%ar_p`, `%asn`, `%b_a_b`, `%b_a_s`, `%b_c_s`, `%b_c_spb`, `%b_cumprod`, `%b_cumsum`, `%b_d_s`, `%b_diag`, `%b_e`, `%b_f_s`, `%b_f_spb`, `%b_g_s`, `%b_g_spb`, `%b_grand`, `%b_h_s`, `%b_h_spb`, `%b_i_b`, `%b_i_ce`, `%b_i_h`, `%b_i_hm`, `%b_i_s`, `%b_i_sp`, `%b_i_spb`, `%b_i_st`, `%b_iconvert`, `%b_l_b`, `%b_l_s`, `%b_m_b`, `%b_m_s`, `%b_matrix`, `%b_n_hm`, `%b_o_hm`, `%b_p_s`, `%b_prod`, `%b_r_b`, `%b_r_s`, `%b_s_b`, `%b_s_s`, `%b_string`, `%b_sum`, `%b_tril`, `%b_triu`, `%b_x_b`, `%b_x_s`, `%bicg`, `%bicgstab`, `%c_a_c`, `%c_b_c`, `%c_b_s`, `%c_diag`, `%c_dsearch`, `%c_e`, `%c_eye`, `%c_f_s`, `%c_grand`, `%c_i_c`, `%c_i_ce`, `%c_i_h`, `%c_i_hm`, `%c_i_lss`, `%c_i_r`, `%c_i_s`, `%c_i_st`, `%c_matrix`, `%c_n_l`, `%c_n_st`, `%c_o_l`, `%c_o_st`, `%c_ones`, `%c_rand`, `%c_tril`, `%c_triu`, `%cblock_c_cblock`, `%cblock_c_s`, `%cblock_e`, `%cblock_f_cblock`, `%cblock_p`, `%cblock_size`, `%ce_6`, `%ce_c_ce`, `%ce_e`, `%ce_f_ce`, `%ce_i_ce`, `%ce_i_s`, `%ce_i_st`, `%ce_matrix`, `%ce_p`, `%ce_size`, `%ce_string`, `%ce_t`, `%cgs`, `%champdat_i_h`, `%choose`, `%diagram_xcos`, `%dir_p`, `%fptr_i_st`, `%grand_perm`, `%grayplot_i_h`, `%h_i_st`, `%hmS_k_hmS_generic`, `%hm_1_hm`, `%hm_1_s`, `%hm_2_hm`, `%hm_2_s`, `%hm_3_hm`, `%hm_3_s`, `%hm_4_hm`, `%hm_4_s`, `%hm_5`, `%hm_a_hm`, `%hm_a_r`, `%hm_a_s`, `%hm_abs`, `%hm_and`, `%hm_bool2s`, `%hm_c_hm`, `%hm_ceil`, `%hm_conj`, `%hm_cos`, `%hm_cumprod`, `%hm_cumsum`, `%hm_d_hm`, `%hm_d_s`, `%hm_degree`, `%hm_dsearch`, `%hm_e`, `%hm_exp`, `%hm_eye`, `%hm_f_hm`, `%hm_find`, `%hm_floor`, `%hm_g_hm`, `%hm_grand`, `%hm_gsort`, `%hm_h_hm`, `%hm_i_b`, `%hm_i_ce`, `%hm_i_h`, `%hm_i_hm`, `%hm_i_i`, `%hm_i_p`, `%hm_i_r`, `%hm_i_s`, `%hm_i_st`, `%hm_iconvert`, `%hm_imag`, `%hm_int`, `%hm_isnan`, `%hm_isreal`, `%hm_j_hm`, `%hm_j_s`, `%hm_k_hm`, `%hm_k_s`, `%hm_log`, `%hm_m_p`, `%hm_m_r`, `%hm_m_s`, `%hm_matrix`, `%hm_max`, `%hm_mean`, `%hm_median`, `%hm_min`, `%hm_n_b`, `%hm_n_c`, `%hm_n_hm`, `%hm_n_i`, `%hm_n_p`, `%hm_n_s`, `%hm_o_b`, `%hm_o_c`, `%hm_o_hm`, `%hm_o_i`, `%hm_o_p`, `%hm_o_s`, `%hm_ones`, `%hm_or`, `%hm_p`, `%hm_prod`, `%hm_q_hm`, `%hm_r_s`, `%hm_rand`, `%hm_real`, `%hm_round`, `%hm_s`, `%hm_s_hm`, `%hm_s_r`, `%hm_s_s`, `%hm_sign`, `%hm_sin`, `%hm_size`, `%hm_sqrt`, `%hm_stdev`, `%hm_string`, `%hm_sum`, `%hm_x_hm`, `%hm_x_p`, `%hm_x_s`, `%hm_zeros`, `%i_1_s`, `%i_2_s`, `%i_3_s`, `%i_4_s`, `%i_Matplot`, `%i_a_i`, `%i_a_s`, `%i_and`, `%i_ascii`, `%i_b_s`, `%i_bezout`, `%i_champ`, `%i_champ1`, `%i_contour`, `%i_contour2d`, `%i_d_i`, `%i_d_s`, `%i_dsearch`, `%i_e`, `%i_fft`, `%i_g_i`, `%i_gcd`, `%i_grand`, `%i_h_i`, `%i_i_ce`, `%i_i_h`, `%i_i_hm`, `%i_i_i`, `%i_i_s`, `%i_i_st`, `%i_j_i`, `%i_j_s`, `%i_l_s`, `%i_lcm`, `%i_length`, `%i_m_i`, `%i_m_s`, `%i_mfprintf`, `%i_mprintf`, `%i_msprintf`, `%i_n_s`, `%i_o_s`, `%i_or`, `%i_p_i`, `%i_p_s`, `%i_plot2d`, `%i_plot2d1`, `%i_plot2d2`, `%i_q_s`, `%i_r_i`, `%i_r_s`, `%i_round`, `%i_s_i`, `%i_s_s`, `%i_sign`, `%i_string`, `%i_x_i`, `%i_x_s`, `%ip_a_s`, `%ip_i_st`, `%ip_m_s`, `%ip_n_ip`, `%ip_o_ip`, `%ip_p`, `%ip_part`, `%ip_s_s`, `%ip_string`, `%k`, `%l_i_h`, `%l_i_s`, `%l_i_st`, `%l_isequal`, `%l_n_c`, `%l_n_l`, `%l_n_m`, `%l_n_p`, `%l_n_s`, `%l_n_st`, `%l_o_c`, `%l_o_l`, `%l_o_m`, `%l_o_p`, `%l_o_s`, `%l_o_st`, `%lss_a_lss`, `%lss_a_p`, `%lss_a_r`, `%lss_a_s`, `%lss_c_lss`, `%lss_c_p`, `%lss_c_r`, `%lss_c_s`, `%lss_e`, `%lss_eye`, `%lss_f_lss`, `%lss_f_p`, `%lss_f_r`, `%lss_f_s`, `%lss_i_ce`, `%lss_i_lss`, `%lss_i_p`, `%lss_i_r`, `%lss_i_s`, `%lss_i_st`, `%lss_inv`, `%lss_l_lss`, `%lss_l_p`, `%lss_l_r`, `%lss_l_s`, `%lss_m_lss`, `%lss_m_p`, `%lss_m_r`, `%lss_m_s`, `%lss_n_lss`, `%lss_n_p`, `%lss_n_r`, `%lss_n_s`, `%lss_norm`, `%lss_o_lss`, `%lss_o_p`, `%lss_o_r`, `%lss_o_s`, `%lss_ones`, `%lss_r_lss`, `%lss_r_p`, `%lss_r_r`, `%lss_r_s`, `%lss_rand`, `%lss_s`, `%lss_s_lss`, `%lss_s_p`, `%lss_s_r`, `%lss_s_s`, `%lss_size`, `%lss_t`, `%lss_v_lss`, `%lss_v_p`, `%lss_v_r`, `%lss_v_s`, `%lt_i_s`, `%m_n_l`, `%m_o_l`, `%mc_i_h`, `%mc_i_s`, `%mc_i_st`, `%mc_n_st`, `%mc_o_st`, `%mc_string`, `%mps_p`, `%mps_string`, `%msp_a_s`, `%msp_abs`, `%msp_e`, `%msp_find`, `%msp_i_s`, `%msp_i_st`, `%msp_length`, `%msp_m_s`, `%msp_maxi`, `%msp_n_msp`, `%msp_nnz`, `%msp_o_msp`, `%msp_p`, `%msp_sparse`, `%msp_spones`, `%msp_t`, `%p_a_lss`, `%p_a_r`, `%p_c_lss`, `%p_c_r`, `%p_cumprod`, `%p_cumsum`, `%p_d_p`, `%p_d_r`, `%p_d_s`, `%p_det`, `%p_e`, `%p_f_lss`, `%p_f_r`, `%p_grand`, `%p_i_ce`, `%p_i_h`, `%p_i_hm`, `%p_i_lss`, `%p_i_p`, `%p_i_r`, `%p_i_s`, `%p_i_st`, `%p_inv`, `%p_j_s`, `%p_k_p`, `%p_k_r`, `%p_k_s`, `%p_l_lss`, `%p_l_p`, `%p_l_r`, `%p_l_s`, `%p_m_hm`, `%p_m_lss`, `%p_m_r`, `%p_matrix`, `%p_n_l`, `%p_n_lss`, `%p_n_r`, `%p_o_l`, `%p_o_lss`, `%p_o_r`, `%p_o_sp`, `%p_p_s`, `%p_part`, `%p_prod`, `%p_q_p`, `%p_q_r`, `%p_q_s`, `%p_r_lss`, `%p_r_p`, `%p_r_r`, `%p_r_s`, `%p_s_lss`, `%p_s_r`, `%p_simp`, `%p_string`, `%p_sum`, `%p_v_lss`, `%p_v_p`, `%p_v_r`, `%p_v_s`, `%p_x_hm`, `%p_x_r`, `%p_y_p`, `%p_y_r`, `%p_y_s`, `%p_z_p`, `%p_z_r`, `%p_z_s`, `%pcg`, `%plist_p`, `%plist_string`, `%r_0`, `%r_a_hm`, `%r_a_lss`, `%r_a_p`, `%r_a_r`, `%r_a_s`, `%r_c_lss`, `%r_c_p`, `%r_c_r`, `%r_c_s`, `%r_clean`, `%r_cumprod`, `%r_cumsum`, `%r_d_p`, `%r_d_r`, `%r_d_s`, `%r_det`, `%r_diag`, `%r_e`, `%r_eye`, `%r_f_lss`, `%r_f_p`, `%r_f_r`, `%r_f_s`, `%r_i_ce`, `%r_i_hm`, `%r_i_lss`, `%r_i_p`, `%r_i_r`, `%r_i_s`, `%r_i_st`, `%r_inv`, `%r_j_s`, `%r_k_p`, `%r_k_r`, `%r_k_s`, `%r_l_lss`, `%r_l_p`, `%r_l_r`, `%r_l_s`, `%r_m_hm`, `%r_m_lss`, `%r_m_p`, `%r_m_r`, `%r_m_s`, `%r_matrix`, `%r_n_lss`, `%r_n_p`, `%r_n_r`, `%r_n_s`, `%r_norm`, `%r_o_lss`, `%r_o_p`, `%r_o_r`, `%r_o_s`, `%r_ones`, `%r_p`, `%r_p_s`, `%r_prod`, `%r_q_p`, `%r_q_r`, `%r_q_s`, `%r_r_lss`, `%r_r_p`, `%r_r_r`, `%r_r_s`, `%r_rand`, `%r_s`, `%r_s_hm`, `%r_s_lss`, `%r_s_p`, `%r_s_r`, `%r_s_s`, `%r_simp`, `%r_size`, `%r_string`, `%r_sum`, `%r_t`, `%r_tril`, `%r_triu`, `%r_v_lss`, `%r_v_p`, `%r_v_r`, `%r_v_s`, `%r_varn`, `%r_x_p`, `%r_x_r`, `%r_x_s`, `%r_y_p`, `%r_y_r`, `%r_y_s`, `%r_z_p`, `%r_z_r`, `%r_z_s`, `%s_1_hm`, `%s_1_i`, `%s_2_hm`, `%s_2_i`, `%s_3_hm`, `%s_3_i`, `%s_4_hm`, `%s_4_i`, `%s_5`, `%s_a_b`, `%s_a_hm`, `%s_a_i`, `%s_a_ip`, `%s_a_lss`, `%s_a_msp`, `%s_a_r`, `%s_a_sp`, `%s_and`, `%s_b_i`, `%s_b_s`, `%s_bezout`, `%s_c_b`, `%s_c_cblock`, `%s_c_lss`, `%s_c_r`, `%s_c_sp`, `%s_d_b`, `%s_d_i`, `%s_d_p`, `%s_d_r`, `%s_d_sp`, `%s_e`, `%s_f_b`, `%s_f_cblock`, `%s_f_lss`, `%s_f_r`, `%s_f_sp`, `%s_g_b`, `%s_g_s`, `%s_gcd`, `%s_grand`, `%s_h_b`, `%s_h_s`, `%s_i_b`, `%s_i_c`, `%s_i_ce`, `%s_i_h`, `%s_i_hm`, `%s_i_i`, `%s_i_lss`, `%s_i_p`, `%s_i_r`, `%s_i_s`, `%s_i_sp`, `%s_i_spb`, `%s_i_st`, `%s_j_i`, `%s_k_hm`, `%s_k_p`, `%s_k_r`, `%s_k_sp`, `%s_l_b`, `%s_l_hm`, `%s_l_i`, `%s_l_lss`, `%s_l_p`, `%s_l_r`, `%s_l_s`, `%s_l_sp`, `%s_lcm`, `%s_m_b`, `%s_m_hm`, `%s_m_i`, `%s_m_ip`, `%s_m_lss`, `%s_m_msp`, `%s_m_r`, `%s_matrix`, `%s_n_hm`, `%s_n_i`, `%s_n_l`, `%s_n_lss`, `%s_n_r`, `%s_n_st`, `%s_o_hm`, `%s_o_i`, `%s_o_l`, `%s_o_lss`, `%s_o_r`, `%s_o_st`, `%s_or`, `%s_p_b`, `%s_p_i`, `%s_pow`, `%s_q_hm`, `%s_q_i`, `%s_q_p`, `%s_q_r`, `%s_q_sp`, `%s_r_b`, `%s_r_i`, `%s_r_lss`, `%s_r_p`, `%s_r_r`, `%s_r_s`, `%s_r_sp`, `%s_s_b`, `%s_s_hm`, `%s_s_i`, `%s_s_ip`, `%s_s_lss`, `%s_s_r`, `%s_s_sp`, `%s_simp`, `%s_v_lss`, `%s_v_p`, `%s_v_r`, `%s_v_s`, `%s_x_b`, `%s_x_hm`, `%s_x_i`, `%s_x_r`, `%s_y_p`, `%s_y_r`, `%s_y_sp`, `%s_z_p`, `%s_z_r`, `%s_z_sp`, `%sn`, `%sp_a_s`, `%sp_a_sp`, `%sp_and`, `%sp_c_s`, `%sp_ceil`, `%sp_conj`, `%sp_cos`, `%sp_cumprod`, `%sp_cumsum`, `%sp_d_s`, `%sp_d_sp`, `%sp_det`, `%sp_diag`, `%sp_e`, `%sp_exp`, `%sp_f_s`, `%sp_floor`, `%sp_grand`, `%sp_gsort`, `%sp_i_ce`, `%sp_i_h`, `%sp_i_s`, `%sp_i_sp`, `%sp_i_st`, `%sp_int`, `%sp_inv`, `%sp_k_s`, `%sp_k_sp`, `%sp_l_s`, `%sp_l_sp`, `%sp_length`, `%sp_max`, `%sp_min`, `%sp_norm`, `%sp_or`, `%sp_p_s`, `%sp_prod`, `%sp_q_s`, `%sp_q_sp`, `%sp_r_s`, `%sp_r_sp`, `%sp_round`, `%sp_s_s`, `%sp_s_sp`, `%sp_sin`, `%sp_sqrt`, `%sp_string`, `%sp_sum`, `%sp_tril`, `%sp_triu`, `%sp_y_s`, `%sp_y_sp`, `%sp_z_s`, `%sp_z_sp`, `%spb_and`, `%spb_c_b`, `%spb_cumprod`, `%spb_cumsum`, `%spb_diag`, `%spb_e`, `%spb_f_b`, `%spb_g_b`, `%spb_g_spb`, `%spb_h_b`, `%spb_h_spb`, `%spb_i_b`, `%spb_i_ce`, `%spb_i_h`, `%spb_i_st`, `%spb_or`, `%spb_prod`, `%spb_sum`, `%spb_tril`, `%spb_triu`, `%st_6`, `%st_c_st`, `%st_e`, `%st_f_st`, `%st_i_b`, `%st_i_c`, `%st_i_fptr`, `%st_i_h`, `%st_i_i`, `%st_i_ip`, `%st_i_lss`, `%st_i_msp`, `%st_i_p`, `%st_i_r`, `%st_i_s`, `%st_i_sp`, `%st_i_spb`, `%st_i_st`, `%st_matrix`, `%st_n_c`, `%st_n_l`, `%st_n_mc`, `%st_n_p`, `%st_n_s`, `%st_o_c`, `%st_o_l`, `%st_o_mc`, `%st_o_p`, `%st_o_s`, `%st_o_tl`, `%st_p`, `%st_size`, `%st_string`, `%st_t`, `%ticks_i_h`, `%xls_e`, `%xls_p`, `%xlssheet_e`, `%xlssheet_p`, `%xlssheet_size`, `%xlssheet_string`, `DominationRank`, `G_make`, `IsAScalar`, `NDcost`, `OS_Version`, `PlotSparse`, `ReadHBSparse`, `TCL_CreateSlave`, `abcd`, `abinv`, `accept_func_default`, `accept_func_vfsa`, `acf`, `acosd`, `acosh`, `acoshm`, `acosm`, `acot`, `acotd`, `acoth`, `acsc`, `acscd`, `acsch`, `add_demo`, `add_help_chapter`, `add_module_help_chapter`, `add_param`, `add_profiling`, `adj2sp`, `aff2ab`, `ana_style`, `analpf`, `analyze`, `aplat`, `arhnk`, `arl2`, `arma2p`, `arma2ss`, `armac`, `armax`, `armax1`, `arobasestring2strings`, `arsimul`, `ascii2string`, `asciimat`, `asec`, `asecd`, `asech`, `asind`, `asinh`, `asinhm`, `asinm`, `assert_checkalmostequal`, `assert_checkequal`, `assert_checkerror`, `assert_checkfalse`, `assert_checkfilesequal`, `assert_checktrue`, `assert_comparecomplex`, `assert_computedigits`, `assert_cond2reltol`, `assert_cond2reqdigits`, `assert_generror`, `atand`, `atanh`, `atanhm`, `atanm`, `atomsAutoload`, `atomsAutoloadAdd`, `atomsAutoloadDel`, `atomsAutoloadList`, `atomsCategoryList`, `atomsCheckModule`, `atomsDepTreeShow`, `atomsGetConfig`, `atomsGetInstalled`, `atomsGetInstalledPath`, `atomsGetLoaded`, `atomsGetLoadedPath`, `atomsInstall`, `atomsIsInstalled`, `atomsIsLoaded`, `atomsList`, `atomsLoad`, `atomsQuit`, `atomsRemove`, `atomsRepositoryAdd`, `atomsRepositoryDel`, `atomsRepositoryList`, `atomsRestoreConfig`, `atomsSaveConfig`, `atomsSearch`, `atomsSetConfig`, `atomsShow`, `atomsSystemInit`, `atomsSystemUpdate`, `atomsTest`, `atomsUpdate`, `atomsVersion`, `augment`, `auread`, `auwrite`, `balreal`, `bench_run`, `bilin`, `bilt`, `bin2dec`, `binomial`, `bitand`, `bitcmp`, `bitget`, `bitor`, `bitset`, `bitxor`, `black`, `blanks`, `bloc2exp`, `bloc2ss`, `block_parameter_error`, `bode`, `bode_asymp`, `bstap`, `buttmag`, `bvodeS`, `bytecode`, `bytecodewalk`, `cainv`, `calendar`, `calerf`, `calfrq`, `canon`, `casc`, `cat`, `cat_code`, `cb_m2sci_gui`, `ccontrg`, `cell`, `cell2mat`, `cellstr`, `center`, `cepstrum`, `cfspec`, `char`, `chart`, `cheb1mag`, `cheb2mag`, `check_gateways`, `check_modules_xml`, `check_versions`, `chepol`, `chfact`, `chsolve`, `classmarkov`, `clean_help`, `clock`, `cls2dls`, `cmb_lin`, `cmndred`, `cmoment`, `coding_ga_binary`, `coding_ga_identity`, `coff`, `coffg`, `colcomp`, `colcompr`, `colinout`, `colregul`, `companion`, `complex`, `compute_initial_temp`, `cond`, `cond2sp`, `condestsp`, `configure_msifort`, `configure_msvc`, `conjgrad`, `cont_frm`, `cont_mat`, `contrss`, `conv`, `convert_to_float`, `convertindex`, `convol`, `convol2d`, `copfac`, `correl`, `cosd`, `cosh`, `coshm`, `cosm`, `cotd`, `cotg`, `coth`, `cothm`, `cov`, `covar`, `createXConfiguration`, `createfun`, `createstruct`, `cross`, `crossover_ga_binary`, `crossover_ga_default`, `csc`, `cscd`, `csch`, `csgn`, `csim`, `cspect`, `ctr_gram`, `czt`, `dae`, `daeoptions`, `damp`, `datafit`, `date`, `datenum`, `datevec`, `dbphi`, `dcf`, `ddp`, `dec2bin`, `dec2hex`, `dec2oct`, `del_help_chapter`, `del_module_help_chapter`, `demo_begin`, `demo_choose`, `demo_compiler`, `demo_end`, `demo_file_choice`, `demo_folder_choice`, `demo_function_choice`, `demo_gui`, `demo_run`, `demo_viewCode`, `denom`, `derivat`, `derivative`, `des2ss`, `des2tf`, `detectmsifort64tools`, `detectmsvc64tools`, `determ`, `detr`, `detrend`, `devtools_run_builder`, `dhnorm`, `diff`, `diophant`, `dir`, `dirname`, `dispfiles`, `dllinfo`, `dscr`, `dsimul`, `dt_ility`, `dtsi`, `edit`, `edit_error`, `editor`, `eigenmarkov`, `eigs`, `ell1mag`, `enlarge_shape`, `entropy`, `eomday`, `epred`, `eqfir`, `eqiir`, `equil`, `equil1`, `erfinv`, `etime`, `eval`, `evans`, `evstr`, `example_run`, `expression2code`, `extract_help_examples`, `factor`, `factorial`, `factors`, `faurre`, `ffilt`, `fft2`, `fftshift`, `fieldnames`, `filt_sinc`, `filter`, `findABCD`, `findAC`, `findBDK`, `findR`, `find_freq`, `find_links`, `find_scicos_version`, `findm`, `findmsifortcompiler`, `findmsvccompiler`, `findx0BD`, `firstnonsingleton`, `fix`, `fixedpointgcd`, `flipdim`, `flts`, `fminsearch`, `formatBlackTip`, `formatBodeMagTip`, `formatBodePhaseTip`, `formatGainplotTip`, `formatHallModuleTip`, `formatHallPhaseTip`, `formatNicholsGainTip`, `formatNicholsPhaseTip`, `formatNyquistTip`, `formatPhaseplotTip`, `formatSgridDampingTip`, `formatSgridFreqTip`, `formatZgridDampingTip`, `formatZgridFreqTip`, `format_txt`, `fourplan`, `frep2tf`, `freson`, `frfit`, `frmag`, `fseek_origin`, `fsfirlin`, `fspec`, `fspecg`, `fstabst`, `ftest`, `ftuneq`, `fullfile`, `fullrf`, `fullrfk`, `fun2string`, `g_margin`, `gainplot`, `gamitg`, `gcare`, `gcd`, `gencompilationflags_unix`, `generateBlockImage`, `generateBlockImages`, `generic_i_ce`, `generic_i_h`, `generic_i_hm`, `generic_i_s`, `generic_i_st`, `genlib`, `genmarkov`, `geomean`, `getDiagramVersion`, `getModelicaPath`, `getPreferencesValue`, `get_file_path`, `get_function_path`, `get_param`, `get_profile`, `get_scicos_version`, `getd`, `getscilabkeywords`, `getshell`, `gettklib`, `gfare`, `gfrancis`, `givens`, `glever`, `gmres`, `group`, `gschur`, `gspec`, `gtild`, `h2norm`, `h_cl`, `h_inf`, `h_inf_st`, `h_norm`, `hallchart`, `halt`, `hank`, `hankelsv`, `harmean`, `haveacompiler`, `head_comments`, `help_from_sci`, `help_skeleton`, `hermit`, `hex2dec`, `hilb`, `hilbert`, `histc`, `horner`, `householder`, `hrmt`, `htrianr`, `hypermat`, `idct`, `idst`, `ifft`, `ifftshift`, `iir`, `iirgroup`, `iirlp`, `iirmod`, `ilib_build`, `ilib_build_jar`, `ilib_compile`, `ilib_for_link`, `ilib_gen_Make`, `ilib_gen_Make_unix`, `ilib_gen_cleaner`, `ilib_gen_gateway`, `ilib_gen_loader`, `ilib_include_flag`, `ilib_mex_build`, `im_inv`, `importScicosDiagram`, `importScicosPal`, `importXcosDiagram`, `imrep2ss`, `ind2sub`, `inistate`, `init_ga_default`, `init_param`, `initial_scicos_tables`, `input`, `instruction2code`, `intc`, `intdec`, `integrate`, `interp1`, `interpln`, `intersect`, `intl`, `intsplin`, `inttrap`, `inv_coeff`, `invr`, `invrs`, `invsyslin`, `iqr`, `isLeapYear`, `is_absolute_path`, `is_param`, `iscell`, `iscellstr`, `iscolumn`, `isempty`, `isfield`, `isinf`, `ismatrix`, `isnan`, `isrow`, `isscalar`, `issparse`, `issquare`, `isstruct`, `isvector`, `jmat`, `justify`, `kalm`, `karmarkar`, `kernel`, `kpure`, `krac2`, `kroneck`, `lattn`, `lattp`, `launchtest`, `lcf`, `lcm`, `lcmdiag`, `leastsq`, `leqe`, `leqr`, `lev`, `levin`, `lex_sort`, `lft`, `lin`, `lin2mu`, `lincos`, `lindquist`, `linf`, `linfn`, `linsolve`, `linspace`, `list2vec`, `list_param`, `listfiles`, `listfunctions`, `listvarinfile`, `lmisolver`, `lmitool`, `loadXcosLibs`, `loadmatfile`, `loadwave`, `log10`, `log2`, `logm`, `logspace`, `lqe`, `lqg`, `lqg2stan`, `lqg_ltr`, `lqr`, `ls`, `lyap`, `m2sci_gui`, `m_circle`, `macglov`, `macrovar`, `mad`, `makecell`, `manedit`, `mapsound`, `markp2ss`, `matfile2sci`, `mdelete`, `mean`, `meanf`, `median`, `members`, `mese`, `meshgrid`, `mfft`, `mfile2sci`, `minreal`, `minss`, `mkdir`, `modulo`, `moment`, `mrfit`, `msd`, `mstr2sci`, `mtlb`, `mtlb_0`, `mtlb_a`, `mtlb_all`, `mtlb_any`, `mtlb_axes`, `mtlb_axis`, `mtlb_beta`, `mtlb_box`, `mtlb_choices`, `mtlb_close`, `mtlb_colordef`, `mtlb_cond`, `mtlb_cov`, `mtlb_cumprod`, `mtlb_cumsum`, `mtlb_dec2hex`, `mtlb_delete`, `mtlb_diag`, `mtlb_diff`, `mtlb_dir`, `mtlb_double`, `mtlb_e`, `mtlb_echo`, `mtlb_error`, `mtlb_eval`, `mtlb_exist`, `mtlb_eye`, `mtlb_false`, `mtlb_fft`, `mtlb_fftshift`, `mtlb_filter`, `mtlb_find`, `mtlb_findstr`, `mtlb_fliplr`, `mtlb_fopen`, `mtlb_format`, `mtlb_fprintf`, `mtlb_fread`, `mtlb_fscanf`, `mtlb_full`, `mtlb_fwrite`, `mtlb_get`, `mtlb_grid`, `mtlb_hold`, `mtlb_i`, `mtlb_ifft`, `mtlb_image`, `mtlb_imp`, `mtlb_int16`, `mtlb_int32`, `mtlb_int8`, `mtlb_is`, `mtlb_isa`, `mtlb_isfield`, `mtlb_isletter`, `mtlb_isspace`, `mtlb_l`, `mtlb_legendre`, `mtlb_linspace`, `mtlb_logic`, `mtlb_logical`, `mtlb_loglog`, `mtlb_lower`, `mtlb_max`, `mtlb_mean`, `mtlb_median`, `mtlb_mesh`, `mtlb_meshdom`, `mtlb_min`, `mtlb_more`, `mtlb_num2str`, `mtlb_ones`, `mtlb_pcolor`, `mtlb_plot`, `mtlb_prod`, `mtlb_qr`, `mtlb_qz`, `mtlb_rand`, `mtlb_randn`, `mtlb_rcond`, `mtlb_realmax`, `mtlb_realmin`, `mtlb_s`, `mtlb_semilogx`, `mtlb_semilogy`, `mtlb_setstr`, `mtlb_size`, `mtlb_sort`, `mtlb_sortrows`, `mtlb_sprintf`, `mtlb_sscanf`, `mtlb_std`, `mtlb_strcmp`, `mtlb_strcmpi`, `mtlb_strfind`, `mtlb_strrep`, `mtlb_subplot`, `mtlb_sum`, `mtlb_t`, `mtlb_toeplitz`, `mtlb_tril`, `mtlb_triu`, `mtlb_true`, `mtlb_type`, `mtlb_uint16`, `mtlb_uint32`, `mtlb_uint8`, `mtlb_upper`, `mtlb_var`, `mtlb_zeros`, `mu2lin`, `mutation_ga_binary`, `mutation_ga_default`, `mvcorrel`, `mvvacov`, `nancumsum`, `nand2mean`, `nanmax`, `nanmean`, `nanmeanf`, `nanmedian`, `nanmin`, `nanreglin`, `nanstdev`, `nansum`, `narsimul`, `ndgrid`, `ndims`, `nehari`, `neigh_func_csa`, `neigh_func_default`, `neigh_func_fsa`, `neigh_func_vfsa`, `neldermead_cget`, `neldermead_configure`, `neldermead_costf`, `neldermead_defaultoutput`, `neldermead_destroy`, `neldermead_function`, `neldermead_get`, `neldermead_log`, `neldermead_new`, `neldermead_restart`, `neldermead_search`, `neldermead_updatesimp`, `nextpow2`, `nfreq`, `nicholschart`, `nlev`, `nmplot_cget`, `nmplot_configure`, `nmplot_contour`, `nmplot_destroy`, `nmplot_function`, `nmplot_get`, `nmplot_historyplot`, `nmplot_log`, `nmplot_new`, `nmplot_outputcmd`, `nmplot_restart`, `nmplot_search`, `nmplot_simplexhistory`, `noisegen`, `nonreg_test_run`, `now`, `nthroot`, `null`, `num2cell`, `numderivative`, `numdiff`, `numer`, `nyquist`, `nyquistfrequencybounds`, `obs_gram`, `obscont`, `observer`, `obsv_mat`, `obsvss`, `oct2dec`, `odeoptions`, `optim_ga`, `optim_moga`, `optim_nsga`, `optim_nsga2`, `optim_sa`, `optimbase_cget`, `optimbase_checkbounds`, `optimbase_checkcostfun`, `optimbase_checkx0`, `optimbase_configure`, `optimbase_destroy`, `optimbase_function`, `optimbase_get`, `optimbase_hasbounds`, `optimbase_hasconstraints`, `optimbase_hasnlcons`, `optimbase_histget`, `optimbase_histset`, `optimbase_incriter`, `optimbase_isfeasible`, `optimbase_isinbounds`, `optimbase_isinnonlincons`, `optimbase_log`, `optimbase_logshutdown`, `optimbase_logstartup`, `optimbase_new`, `optimbase_outputcmd`, `optimbase_outstruct`, `optimbase_proj2bnds`, `optimbase_set`, `optimbase_stoplog`, `optimbase_terminate`, `optimget`, `optimplotfunccount`, `optimplotfval`, `optimplotx`, `optimset`, `optimsimplex_center`, `optimsimplex_check`, `optimsimplex_compsomefv`, `optimsimplex_computefv`, `optimsimplex_deltafv`, `optimsimplex_deltafvmax`, `optimsimplex_destroy`, `optimsimplex_dirmat`, `optimsimplex_fvmean`, `optimsimplex_fvstdev`, `optimsimplex_fvvariance`, `optimsimplex_getall`, `optimsimplex_getallfv`, `optimsimplex_getallx`, `optimsimplex_getfv`, `optimsimplex_getn`, `optimsimplex_getnbve`, `optimsimplex_getve`, `optimsimplex_getx`, `optimsimplex_gradientfv`, `optimsimplex_log`, `optimsimplex_new`, `optimsimplex_reflect`, `optimsimplex_setall`, `optimsimplex_setallfv`, `optimsimplex_setallx`, `optimsimplex_setfv`, `optimsimplex_setn`, `optimsimplex_setnbve`, `optimsimplex_setve`, `optimsimplex_setx`, `optimsimplex_shrink`, `optimsimplex_size`, `optimsimplex_sort`, `optimsimplex_xbar`, `orth`, `output_ga_default`, `output_moga_default`, `output_nsga2_default`, `output_nsga_default`, `p_margin`, `pack`, `pareto_filter`, `parrot`, `pbig`, `pca`, `pcg`, `pdiv`, `pen2ea`, `pencan`, `pencost`, `penlaur`, `perctl`, `perl`, `perms`, `permute`, `pertrans`, `pfactors`, `pfss`, `phasemag`, `phaseplot`, `phc`, `pinv`, `playsnd`, `plotprofile`, `plzr`, `pmodulo`, `pol2des`, `pol2str`, `polar`, `polfact`, `prbs_a`, `prettyprint`, `primes`, `princomp`, `profile`, `proj`, `projsl`, `projspec`, `psmall`, `pspect`, `qmr`, `qpsolve`, `quart`, `quaskro`, `rafiter`, `randpencil`, `range`, `rank`, `readxls`, `recompilefunction`, `recons`, `reglin`, `regress`, `remezb`, `remove_param`, `remove_profiling`, `repfreq`, `replace_Ix_by_Fx`, `repmat`, `reset_profiling`, `resize_matrix`, `returntoscilab`, `rhs2code`, `ric_desc`, `riccati`, `rmdir`, `routh_t`, `rowcomp`, `rowcompr`, `rowinout`, `rowregul`, `rowshuff`, `rref`, `sample`, `samplef`, `samwr`, `savematfile`, `savewave`, `scanf`, `sci2exp`, `sciGUI_init`, `sci_sparse`, `scicos_getvalue`, `scicos_simulate`, `scicos_workspace_init`, `scisptdemo`, `scitest`, `sdiff`, `sec`, `secd`, `sech`, `selection_ga_elitist`, `selection_ga_random`, `sensi`, `setPreferencesValue`, `set_param`, `setdiff`, `sgrid`, `show_margins`, `show_pca`, `showprofile`, `signm`, `sinc`, `sincd`, `sind`, `sinh`, `sinhm`, `sinm`, `sm2des`, `sm2ss`, `smga`, `smooth`, `solve`, `sound`, `soundsec`, `sp2adj`, `spaninter`, `spanplus`, `spantwo`, `specfact`, `speye`, `sprand`, `spzeros`, `sqroot`, `sqrtm`, `squarewave`, `squeeze`, `srfaur`, `srkf`, `ss2des`, `ss2ss`, `ss2tf`, `sskf`, `ssprint`, `ssrand`, `st_deviation`, `st_i_generic`, `st_ility`, `stabil`, `statgain`, `stdev`, `stdevf`, `steadycos`, `strange`, `strcmpi`, `struct`, `sub2ind`, `sva`, `svplot`, `sylm`, `sylv`, `sysconv`, `sysdiag`, `sysfact`, `syslin`, `syssize`, `system`, `systmat`, `tabul`, `tand`, `tanh`, `tanhm`, `tanm`, `tbx_build_blocks`, `tbx_build_cleaner`, `tbx_build_gateway`, `tbx_build_gateway_clean`, `tbx_build_gateway_loader`, `tbx_build_help`, `tbx_build_help_loader`, `tbx_build_loader`, `tbx_build_localization`, `tbx_build_macros`, `tbx_build_pal_loader`, `tbx_build_src`, `tbx_builder`, `tbx_builder_gateway`, `tbx_builder_gateway_lang`, `tbx_builder_help`, `tbx_builder_help_lang`, `tbx_builder_macros`, `tbx_builder_src`, `tbx_builder_src_lang`, `tbx_generate_pofile`, `temp_law_csa`, `temp_law_default`, `temp_law_fsa`, `temp_law_huang`, `temp_law_vfsa`, `test_clean`, `test_on_columns`, `test_run`, `test_run_level`, `testexamples`, `tf2des`, `tf2ss`, `thrownan`, `tic`, `time_id`, `toc`, `toeplitz`, `tokenpos`, `toolboxes`, `trace`, `trans`, `translatepaths`, `tree2code`, `trfmod`, `trianfml`, `trimmean`, `trisolve`, `trzeros`, `typeof`, `ui_observer`, `union`, `unique`, `unit_test_run`, `unix_g`, `unix_s`, `unix_w`, `unix_x`, `unobs`, `unpack`, `unwrap`, `variance`, `variancef`, `vec2list`, `vectorfind`, `ver`, `warnobsolete`, `wavread`, `wavwrite`, `wcenter`, `weekday`, `wfir`, `wfir_gui`, `whereami`, `who_user`, `whos`, `wiener`, `wigner`, `window`, `winlist`, `with_javasci`, `with_macros_source`, `with_modelica_compiler`, `with_tk`, `xcorr`, `xcosBlockEval`, `xcosBlockInterface`, `xcosCodeGeneration`, `xcosConfigureModelica`, `xcosPal`, `xcosPalAdd`, `xcosPalAddBlock`, `xcosPalExport`, `xcosPalGenerateAllIcons`, `xcosShowBlockWarning`, `xcosValidateBlockSet`, `xcosValidateCompareBlock`, `xcos_compile`, `xcos_debug_gui`, `xcos_run`, `xcos_simulate`, `xcov`, `xmltochm`, `xmltoformat`, `xmltohtml`, `xmltojar`, `xmltopdf`, `xmltops`, `xmltoweb`, `yulewalk`, `zeropen`, `zgrid`, `zpbutt`, `zpch1`, `zpch2`, `zpell`), NameBuiltin, nil},
- {Words(``, `\b`, `$`, `%F`, `%T`, `%e`, `%eps`, `%f`, `%fftw`, `%gui`, `%i`, `%inf`, `%io`, `%modalWarning`, `%nan`, `%pi`, `%s`, `%t`, `%tk`, `%toolboxes`, `%toolboxes_dir`, `%z`, `PWD`, `SCI`, `SCIHOME`, `TMPDIR`, `arnoldilib`, `assertlib`, `atomslib`, `cacsdlib`, `compatibility_functilib`, `corelib`, `data_structureslib`, `demo_toolslib`, `development_toolslib`, `differential_equationlib`, `dynamic_linklib`, `elementary_functionslib`, `enull`, `evoid`, `external_objectslib`, `fd`, `fileiolib`, `functionslib`, `genetic_algorithmslib`, `helptoolslib`, `home`, `integerlib`, `interpolationlib`, `iolib`, `jnull`, `jvoid`, `linear_algebralib`, `m2scilib`, `matiolib`, `modules_managerlib`, `neldermeadlib`, `optimbaselib`, `optimizationlib`, `optimsimplexlib`, `output_streamlib`, `overloadinglib`, `parameterslib`, `polynomialslib`, `preferenceslib`, `randliblib`, `scicos_autolib`, `scicos_utilslib`, `scinoteslib`, `signal_processinglib`, `simulated_annealinglib`, `soundlib`, `sparselib`, `special_functionslib`, `spreadsheetlib`, `statisticslib`, `stringlib`, `tclscilib`, `timelib`, `umfpacklib`, `xcoslib`), NameConstant, nil},
- {`-|==|~=|<|>|<=|>=|&&|&|~|\|\|?`, Operator, nil},
- {`\.\*|\*|\+|\.\^|\.\\|\.\/|\/|\\`, Operator, nil},
- {`[\[\](){}@.,=:;]`, Punctuation, nil},
- {`"[^"]*"`, LiteralString, nil},
- {`(?<=[\w)\].])\'+`, Operator, nil},
- {`(?|+=@:,./?-]+`, Operator, nil},
- {`[\[\]()]+`, Punctuation, nil},
- {`"`, LiteralStringDouble, Push("string-double")},
- {`'`, LiteralStringSingle, Push("string-single")},
- {`[a-z_-][\w-]*`, Name, nil},
- {`\n`, Text, nil},
- {`[;{}]`, Punctuation, Pop(1)},
- },
- "interpolation": {
- {`\}`, LiteralStringInterpol, Pop(1)},
- Include("value"),
- },
- "selector": {
- {`[ \t]+`, Text, nil},
- {`\:`, NameDecorator, Push("pseudo-class")},
- {`\.`, NameClass, Push("class")},
- {`#\{`, LiteralStringInterpol, Push("interpolation")},
- {`\#`, NameNamespace, Push("id")},
- {`&`, Keyword, nil},
- {`[~^*!&\[\]()<>|+=@:,./?-]`, Operator, nil},
- {`(%)([\w-]+)`, ByGroups(Operator, NameClass), nil},
- {`"`, LiteralStringDouble, Push("string-double")},
- {`'`, LiteralStringSingle, Push("string-single")},
- {`\n`, Text, nil},
- {`[;{}]`, Punctuation, Pop(1)},
- {`[\w-]+`, NameTag, nil},
- },
- "string-double": {
- {`(\\.|#(?=[^\n{])|[^\n"#])+`, LiteralStringDouble, nil},
- {`#\{`, LiteralStringInterpol, Push("interpolation")},
- {`"`, LiteralStringDouble, Pop(1)},
- },
- "string-single": {
- {`(\\.|#(?=[^\n{])|[^\n'#])+`, LiteralStringSingle, nil},
- {`#\{`, LiteralStringInterpol, Push("interpolation")},
- {`'`, LiteralStringSingle, Pop(1)},
- },
- "string-url": {
- {`(\\#|#(?=[^\n{])|[^\n#)])+`, LiteralStringOther, nil},
- {`#\{`, LiteralStringInterpol, Push("interpolation")},
- {`\)`, LiteralStringOther, Pop(1)},
- },
- "pseudo-class": {
- {`[\w-]+`, NameDecorator, nil},
- {`#\{`, LiteralStringInterpol, Push("interpolation")},
- Default(Pop(1)),
- },
- "class": {
- {`[\w-]+`, NameClass, nil},
- {`#\{`, LiteralStringInterpol, Push("interpolation")},
- Default(Pop(1)),
- },
- "id": {
- {`[\w-]+`, NameNamespace, nil},
- {`#\{`, LiteralStringInterpol, Push("interpolation")},
- Default(Pop(1)),
- },
- "for": {
- {`(from|to|through)`, OperatorWord, nil},
- Include("value"),
- },
- "each": {
- {`in`, OperatorWord, nil},
- Include("value"),
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/s/smalltalk.go b/vendor/github.com/alecthomas/chroma/lexers/s/smalltalk.go
deleted file mode 100644
index b4143d0..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/s/smalltalk.go
+++ /dev/null
@@ -1,103 +0,0 @@
-package s
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-// Smalltalk lexer.
-var Smalltalk = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "Smalltalk",
- Aliases: []string{"smalltalk", "squeak", "st"},
- Filenames: []string{"*.st"},
- MimeTypes: []string{"text/x-smalltalk"},
- },
- smalltalkRules,
-))
-
-func smalltalkRules() Rules {
- return Rules{
- "root": {
- {`(<)(\w+:)(.*?)(>)`, ByGroups(Text, Keyword, Text, Text), nil},
- Include("squeak fileout"),
- Include("whitespaces"),
- Include("method definition"),
- {`(\|)([\w\s]*)(\|)`, ByGroups(Operator, NameVariable, Operator), nil},
- Include("objects"),
- {`\^|:=|_`, Operator, nil},
- {`[\]({}.;!]`, Text, nil},
- },
- "method definition": {
- {`([a-zA-Z]+\w*:)(\s*)(\w+)`, ByGroups(NameFunction, Text, NameVariable), nil},
- {`^(\b[a-zA-Z]+\w*\b)(\s*)$`, ByGroups(NameFunction, Text), nil},
- {`^([-+*/\\~<>=|&!?,@%]+)(\s*)(\w+)(\s*)$`, ByGroups(NameFunction, Text, NameVariable, Text), nil},
- },
- "blockvariables": {
- Include("whitespaces"),
- {`(:)(\s*)(\w+)`, ByGroups(Operator, Text, NameVariable), nil},
- {`\|`, Operator, Pop(1)},
- Default(Pop(1)),
- },
- "literals": {
- {`'(''|[^'])*'`, LiteralString, Push("afterobject")},
- {`\$.`, LiteralStringChar, Push("afterobject")},
- {`#\(`, LiteralStringSymbol, Push("parenth")},
- {`\)`, Text, Push("afterobject")},
- {`(\d+r)?-?\d+(\.\d+)?(e-?\d+)?`, LiteralNumber, Push("afterobject")},
- },
- "_parenth_helper": {
- Include("whitespaces"),
- {`(\d+r)?-?\d+(\.\d+)?(e-?\d+)?`, LiteralNumber, nil},
- {`[-+*/\\~<>=|!?,@%\w:]+`, LiteralStringSymbol, nil},
- {`'(''|[^'])*'`, LiteralString, nil},
- {`\$.`, LiteralStringChar, nil},
- {`#*\(`, LiteralStringSymbol, Push("inner_parenth")},
- },
- "parenth": {
- {`\)`, LiteralStringSymbol, Push("root", "afterobject")},
- Include("_parenth_helper"),
- },
- "inner_parenth": {
- {`\)`, LiteralStringSymbol, Pop(1)},
- Include("_parenth_helper"),
- },
- "whitespaces": {
- {`\s+`, Text, nil},
- {`"(""|[^"])*"`, Comment, nil},
- },
- "objects": {
- {`\[`, Text, Push("blockvariables")},
- {`\]`, Text, Push("afterobject")},
- {`\b(self|super|true|false|nil|thisContext)\b`, NameBuiltinPseudo, Push("afterobject")},
- {`\b[A-Z]\w*(?!:)\b`, NameClass, Push("afterobject")},
- {`\b[a-z]\w*(?!:)\b`, NameVariable, Push("afterobject")},
- {`#("(""|[^"])*"|[-+*/\\~<>=|&!?,@%]+|[\w:]+)`, LiteralStringSymbol, Push("afterobject")},
- Include("literals"),
- },
- "afterobject": {
- {`! !$`, Keyword, Pop(1)},
- Include("whitespaces"),
- {`\b(ifTrue:|ifFalse:|whileTrue:|whileFalse:|timesRepeat:)`, NameBuiltin, Pop(1)},
- {`\b(new\b(?!:))`, NameBuiltin, nil},
- {`:=|_`, Operator, Pop(1)},
- {`\b[a-zA-Z]+\w*:`, NameFunction, Pop(1)},
- {`\b[a-zA-Z]+\w*`, NameFunction, nil},
- {`\w+:?|[-+*/\\~<>=|&!?,@%]+`, NameFunction, Pop(1)},
- {`\.`, Punctuation, Pop(1)},
- {`;`, Punctuation, nil},
- {`[\])}]`, Text, nil},
- {`[\[({]`, Text, Pop(1)},
- },
- "squeak fileout": {
- {`^"(""|[^"])*"!`, Keyword, nil},
- {`^'(''|[^'])*'!`, Keyword, nil},
- {`^(!)(\w+)( commentStamp: )(.*?)( prior: .*?!\n)(.*?)(!)`, ByGroups(Keyword, NameClass, Keyword, LiteralString, Keyword, Text, Keyword), nil},
- {`^(!)(\w+(?: class)?)( methodsFor: )('(?:''|[^'])*')(.*?!)`, ByGroups(Keyword, NameClass, Keyword, LiteralString, Keyword), nil},
- {`^(\w+)( subclass: )(#\w+)(\s+instanceVariableNames: )(.*?)(\s+classVariableNames: )(.*?)(\s+poolDictionaries: )(.*?)(\s+category: )(.*?)(!)`, ByGroups(NameClass, Keyword, LiteralStringSymbol, Keyword, LiteralString, Keyword, LiteralString, Keyword, LiteralString, Keyword, LiteralString, Keyword), nil},
- {`^(\w+(?: class)?)(\s+instanceVariableNames: )(.*?)(!)`, ByGroups(NameClass, Keyword, LiteralString, Keyword), nil},
- {`(!\n)(\].*)(! !)$`, ByGroups(Keyword, Text, Keyword), nil},
- {`! !$`, Keyword, nil},
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/s/smarty.go b/vendor/github.com/alecthomas/chroma/lexers/s/smarty.go
deleted file mode 100644
index 566efbb..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/s/smarty.go
+++ /dev/null
@@ -1,44 +0,0 @@
-package s
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- . "github.com/alecthomas/chroma/lexers/circular" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-// Smarty lexer.
-var Smarty = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "Smarty",
- Aliases: []string{"smarty"},
- Filenames: []string{"*.tpl"},
- MimeTypes: []string{"application/x-smarty"},
- DotAll: true,
- },
- smartyRules,
-))
-
-func smartyRules() Rules {
- return Rules{
- "root": {
- {`[^{]+`, Other, nil},
- {`(\{)(\*.*?\*)(\})`, ByGroups(CommentPreproc, Comment, CommentPreproc), nil},
- {`(\{php\})(.*?)(\{/php\})`, ByGroups(CommentPreproc, Using(PHP), CommentPreproc), nil},
- {`(\{)(/?[a-zA-Z_]\w*)(\s*)`, ByGroups(CommentPreproc, NameFunction, Text), Push("smarty")},
- {`\{`, CommentPreproc, Push("smarty")},
- },
- "smarty": {
- {`\s+`, Text, nil},
- {`\{`, CommentPreproc, Push()},
- {`\}`, CommentPreproc, Pop(1)},
- {`#[a-zA-Z_]\w*#`, NameVariable, nil},
- {`\$[a-zA-Z_]\w*(\.\w+)*`, NameVariable, nil},
- {`[~!%^&*()+=|\[\]:;,.<>/?@-]`, Operator, nil},
- {`(true|false|null)\b`, KeywordConstant, nil},
- {`[0-9](\.[0-9]*)?(eE[+-][0-9])?[flFLdD]?|0[xX][0-9a-fA-F]+[Ll]?`, LiteralNumber, nil},
- {`"(\\\\|\\"|[^"])*"`, LiteralStringDouble, nil},
- {`'(\\\\|\\'|[^'])*'`, LiteralStringSingle, nil},
- {`[a-zA-Z_]\w*`, NameAttribute, nil},
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/s/sml.go b/vendor/github.com/alecthomas/chroma/lexers/s/sml.go
deleted file mode 100644
index c795ea8..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/s/sml.go
+++ /dev/null
@@ -1,204 +0,0 @@
-package s
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-// Standard ML lexer.
-var StandardML = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "Standard ML",
- Aliases: []string{"sml"},
- Filenames: []string{"*.sml", "*.sig", "*.fun"},
- MimeTypes: []string{"text/x-standardml", "application/x-standardml"},
- },
- standardMLRules,
-))
-
-func standardMLRules() Rules {
- return Rules{
- "whitespace": {
- {`\s+`, Text, nil},
- {`\(\*`, CommentMultiline, Push("comment")},
- },
- "delimiters": {
- {`\(|\[|\{`, Punctuation, Push("main")},
- {`\)|\]|\}`, Punctuation, Pop(1)},
- {`\b(let|if|local)\b(?!\')`, KeywordReserved, Push("main", "main")},
- {`\b(struct|sig|while)\b(?!\')`, KeywordReserved, Push("main")},
- {`\b(do|else|end|in|then)\b(?!\')`, KeywordReserved, Pop(1)},
- },
- "core": {
- {`(_|\}|\{|\)|;|,|\[|\(|\]|\.\.\.)`, Punctuation, nil},
- {`#"`, LiteralStringChar, Push("char")},
- {`"`, LiteralStringDouble, Push("string")},
- {`~?0x[0-9a-fA-F]+`, LiteralNumberHex, nil},
- {`0wx[0-9a-fA-F]+`, LiteralNumberHex, nil},
- {`0w\d+`, LiteralNumberInteger, nil},
- {`~?\d+\.\d+[eE]~?\d+`, LiteralNumberFloat, nil},
- {`~?\d+\.\d+`, LiteralNumberFloat, nil},
- {`~?\d+[eE]~?\d+`, LiteralNumberFloat, nil},
- {`~?\d+`, LiteralNumberInteger, nil},
- {`#\s*[1-9][0-9]*`, NameLabel, nil},
- {`#\s*([a-zA-Z][\w']*)`, NameLabel, nil},
- {"#\\s+([!%&$#+\\-/:<=>?@\\\\~`^|*]+)", NameLabel, nil},
- {`\b(datatype|abstype)\b(?!\')`, KeywordReserved, Push("dname")},
- {`(?=\b(exception)\b(?!\'))`, Text, Push("ename")},
- {`\b(functor|include|open|signature|structure)\b(?!\')`, KeywordReserved, Push("sname")},
- {`\b(type|eqtype)\b(?!\')`, KeywordReserved, Push("tname")},
- {`\'[\w\']*`, NameDecorator, nil},
- {`([a-zA-Z][\w']*)(\.)`, NameNamespace, Push("dotted")},
- {`\b(abstype|and|andalso|as|case|datatype|do|else|end|exception|fn|fun|handle|if|in|infix|infixr|let|local|nonfix|of|op|open|orelse|raise|rec|then|type|val|with|withtype|while|eqtype|functor|include|sharing|sig|signature|struct|structure|where)\b`, KeywordReserved, nil},
- {`([a-zA-Z][\w']*)`, Name, nil},
- {`\b(:|\|,=|=>|->|#|:>)\b`, KeywordReserved, nil},
- {"([!%&$#+\\-/:<=>?@\\\\~`^|*]+)", Name, nil},
- },
- "dotted": {
- {`([a-zA-Z][\w']*)(\.)`, NameNamespace, nil},
- // ignoring reserved words
- {`([a-zA-Z][\w']*)`, Name, Pop(1)},
- // ignoring reserved words
- {"([!%&$#+\\-/:<=>?@\\\\~`^|*]+)", Name, Pop(1)},
- {`\s+`, Error, nil},
- {`\S+`, Error, nil},
- },
- "root": {
- Default(Push("main")),
- },
- "main": {
- Include("whitespace"),
- {`\b(val|and)\b(?!\')`, KeywordReserved, Push("vname")},
- {`\b(fun)\b(?!\')`, KeywordReserved, Push("#pop", "main-fun", "fname")},
- Include("delimiters"),
- Include("core"),
- {`\S+`, Error, nil},
- },
- "main-fun": {
- Include("whitespace"),
- {`\s`, Text, nil},
- {`\(\*`, CommentMultiline, Push("comment")},
- {`\b(fun|and)\b(?!\')`, KeywordReserved, Push("fname")},
- {`\b(val)\b(?!\')`, KeywordReserved, Push("#pop", "main", "vname")},
- {`\|`, Punctuation, Push("fname")},
- {`\b(case|handle)\b(?!\')`, KeywordReserved, Push("#pop", "main")},
- Include("delimiters"),
- Include("core"),
- {`\S+`, Error, nil},
- },
- "char": {
- {`[^"\\]`, LiteralStringChar, nil},
- {`\\[\\"abtnvfr]`, LiteralStringEscape, nil},
- {`\\\^[\x40-\x5e]`, LiteralStringEscape, nil},
- {`\\[0-9]{3}`, LiteralStringEscape, nil},
- {`\\u[0-9a-fA-F]{4}`, LiteralStringEscape, nil},
- {`\\\s+\\`, LiteralStringInterpol, nil},
- {`"`, LiteralStringChar, Pop(1)},
- },
- "string": {
- {`[^"\\]`, LiteralStringDouble, nil},
- {`\\[\\"abtnvfr]`, LiteralStringEscape, nil},
- {`\\\^[\x40-\x5e]`, LiteralStringEscape, nil},
- {`\\[0-9]{3}`, LiteralStringEscape, nil},
- {`\\u[0-9a-fA-F]{4}`, LiteralStringEscape, nil},
- {`\\\s+\\`, LiteralStringInterpol, nil},
- {`"`, LiteralStringDouble, Pop(1)},
- },
- "breakout": {
- {`(?=\b(where|do|handle|if|sig|op|while|case|as|else|signature|andalso|struct|infixr|functor|in|structure|then|local|rec|end|fun|of|orelse|val|include|fn|with|exception|let|and|infix|sharing|datatype|type|abstype|withtype|eqtype|nonfix|raise|open)\b(?!\'))`, Text, Pop(1)},
- },
- "sname": {
- Include("whitespace"),
- Include("breakout"),
- {`([a-zA-Z][\w']*)`, NameNamespace, nil},
- Default(Pop(1)),
- },
- "fname": {
- Include("whitespace"),
- {`\'[\w\']*`, NameDecorator, nil},
- {`\(`, Punctuation, Push("tyvarseq")},
- {`([a-zA-Z][\w']*)`, NameFunction, Pop(1)},
- {"([!%&$#+\\-/:<=>?@\\\\~`^|*]+)", NameFunction, Pop(1)},
- Default(Pop(1)),
- },
- "vname": {
- Include("whitespace"),
- {`\'[\w\']*`, NameDecorator, nil},
- {`\(`, Punctuation, Push("tyvarseq")},
- {"([a-zA-Z][\\w']*)(\\s*)(=(?![!%&$#+\\-/:<=>?@\\\\~`^|*]+))", ByGroups(NameVariable, Text, Punctuation), Pop(1)},
- {"([!%&$#+\\-/:<=>?@\\\\~`^|*]+)(\\s*)(=(?![!%&$#+\\-/:<=>?@\\\\~`^|*]+))", ByGroups(NameVariable, Text, Punctuation), Pop(1)},
- {`([a-zA-Z][\w']*)`, NameVariable, Pop(1)},
- {"([!%&$#+\\-/:<=>?@\\\\~`^|*]+)", NameVariable, Pop(1)},
- Default(Pop(1)),
- },
- "tname": {
- Include("whitespace"),
- Include("breakout"),
- {`\'[\w\']*`, NameDecorator, nil},
- {`\(`, Punctuation, Push("tyvarseq")},
- {"=(?![!%&$#+\\-/:<=>?@\\\\~`^|*]+)", Punctuation, Push("#pop", "typbind")},
- {`([a-zA-Z][\w']*)`, KeywordType, nil},
- {"([!%&$#+\\-/:<=>?@\\\\~`^|*]+)", KeywordType, nil},
- {`\S+`, Error, Pop(1)},
- },
- "typbind": {
- Include("whitespace"),
- {`\b(and)\b(?!\')`, KeywordReserved, Push("#pop", "tname")},
- Include("breakout"),
- Include("core"),
- {`\S+`, Error, Pop(1)},
- },
- "dname": {
- Include("whitespace"),
- Include("breakout"),
- {`\'[\w\']*`, NameDecorator, nil},
- {`\(`, Punctuation, Push("tyvarseq")},
- {`(=)(\s*)(datatype)`, ByGroups(Punctuation, Text, KeywordReserved), Pop(1)},
- {"=(?![!%&$#+\\-/:<=>?@\\\\~`^|*]+)", Punctuation, Push("#pop", "datbind", "datcon")},
- {`([a-zA-Z][\w']*)`, KeywordType, nil},
- {"([!%&$#+\\-/:<=>?@\\\\~`^|*]+)", KeywordType, nil},
- {`\S+`, Error, Pop(1)},
- },
- "datbind": {
- Include("whitespace"),
- {`\b(and)\b(?!\')`, KeywordReserved, Push("#pop", "dname")},
- {`\b(withtype)\b(?!\')`, KeywordReserved, Push("#pop", "tname")},
- {`\b(of)\b(?!\')`, KeywordReserved, nil},
- {`(\|)(\s*)([a-zA-Z][\w']*)`, ByGroups(Punctuation, Text, NameClass), nil},
- {"(\\|)(\\s+)([!%&$#+\\-/:<=>?@\\\\~`^|*]+)", ByGroups(Punctuation, Text, NameClass), nil},
- Include("breakout"),
- Include("core"),
- {`\S+`, Error, nil},
- },
- "ename": {
- Include("whitespace"),
- {`(exception|and)\b(\s+)([a-zA-Z][\w']*)`, ByGroups(KeywordReserved, Text, NameClass), nil},
- {"(exception|and)\\b(\\s*)([!%&$#+\\-/:<=>?@\\\\~`^|*]+)", ByGroups(KeywordReserved, Text, NameClass), nil},
- {`\b(of)\b(?!\')`, KeywordReserved, nil},
- Include("breakout"),
- Include("core"),
- {`\S+`, Error, nil},
- },
- "datcon": {
- Include("whitespace"),
- {`([a-zA-Z][\w']*)`, NameClass, Pop(1)},
- {"([!%&$#+\\-/:<=>?@\\\\~`^|*]+)", NameClass, Pop(1)},
- {`\S+`, Error, Pop(1)},
- },
- "tyvarseq": {
- {`\s`, Text, nil},
- {`\(\*`, CommentMultiline, Push("comment")},
- {`\'[\w\']*`, NameDecorator, nil},
- {`[a-zA-Z][\w']*`, Name, nil},
- {`,`, Punctuation, nil},
- {`\)`, Punctuation, Pop(1)},
- {"[!%&$#+\\-/:<=>?@\\\\~`^|*]+", Name, nil},
- },
- "comment": {
- {`[^(*)]`, CommentMultiline, nil},
- {`\(\*`, CommentMultiline, Push()},
- {`\*\)`, CommentMultiline, Pop(1)},
- {`[(*)]`, CommentMultiline, nil},
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/s/snobol.go b/vendor/github.com/alecthomas/chroma/lexers/s/snobol.go
deleted file mode 100644
index 4529034..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/s/snobol.go
+++ /dev/null
@@ -1,52 +0,0 @@
-package s
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-// Snobol lexer.
-var Snobol = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "Snobol",
- Aliases: []string{"snobol"},
- Filenames: []string{"*.snobol"},
- MimeTypes: []string{"text/x-snobol"},
- },
- snobolRules,
-))
-
-func snobolRules() Rules {
- return Rules{
- "root": {
- {`\*.*\n`, Comment, nil},
- {`[+.] `, Punctuation, Push("statement")},
- {`-.*\n`, Comment, nil},
- {`END\s*\n`, NameLabel, Push("heredoc")},
- {`[A-Za-z$][\w$]*`, NameLabel, Push("statement")},
- {`\s+`, Text, Push("statement")},
- },
- "statement": {
- {`\s*\n`, Text, Pop(1)},
- {`\s+`, Text, nil},
- {`(?<=[^\w.])(LT|LE|EQ|NE|GE|GT|INTEGER|IDENT|DIFFER|LGT|SIZE|REPLACE|TRIM|DUPL|REMDR|DATE|TIME|EVAL|APPLY|OPSYN|LOAD|UNLOAD|LEN|SPAN|BREAK|ANY|NOTANY|TAB|RTAB|REM|POS|RPOS|FAIL|FENCE|ABORT|ARB|ARBNO|BAL|SUCCEED|INPUT|OUTPUT|TERMINAL)(?=[^\w.])`, NameBuiltin, nil},
- {`[A-Za-z][\w.]*`, Name, nil},
- {`\*\*|[?$.!%*/#+\-@|&\\=]`, Operator, nil},
- {`"[^"]*"`, LiteralString, nil},
- {`'[^']*'`, LiteralString, nil},
- {`[0-9]+(?=[^.EeDd])`, LiteralNumberInteger, nil},
- {`[0-9]+(\.[0-9]*)?([EDed][-+]?[0-9]+)?`, LiteralNumberFloat, nil},
- {`:`, Punctuation, Push("goto")},
- {`[()<>,;]`, Punctuation, nil},
- },
- "goto": {
- {`\s*\n`, Text, Pop(2)},
- {`\s+`, Text, nil},
- {`F|S`, Keyword, nil},
- {`(\()([A-Za-z][\w.]*)(\))`, ByGroups(Punctuation, NameLabel, Punctuation), nil},
- },
- "heredoc": {
- {`.*\n`, LiteralStringHeredoc, nil},
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/s/solidity.go b/vendor/github.com/alecthomas/chroma/lexers/s/solidity.go
deleted file mode 100644
index 62a3a40..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/s/solidity.go
+++ /dev/null
@@ -1,118 +0,0 @@
-package s
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-// Solidity lexer.
-var Solidity = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "Solidity",
- Aliases: []string{"sol", "solidity"},
- Filenames: []string{"*.sol"},
- MimeTypes: []string{},
- DotAll: true,
- },
- solidityRules,
-))
-
-func solidityRules() Rules {
- return Rules{
- "assembly": {
- Include("comments"),
- Include("numbers"),
- Include("strings"),
- Include("whitespace"),
- {`\{`, Punctuation, Push()},
- {`\}`, Punctuation, Pop(1)},
- {`[(),]`, Punctuation, nil},
- {`:=|=:`, Operator, nil},
- {`(let)(\s*)(\w*\b)`, ByGroups(OperatorWord, Text, NameVariable), nil},
- {`(\w*\b)(\:[^=])`, ByGroups(NameLabel, Punctuation), nil},
- {`(stop|add|mul|sub|div|sdiv|mod|smod|addmod|mulmod|exp|signextend|lt|gt|slt|sgt|eq|iszero|and|or|xor|not|byte|keccak256|sha3|address|balance|origin|caller|callvalue|calldataload|calldatasize|calldatacopy|codesize|codecopy|gasprice|extcodesize|extcodecopy|blockhash|coinbase|timestamp|number|difficulty|gaslimit|pop|mload|mstore|mstore8|sload|sstore|for|switch|jump|jumpi|pc|msize|gas|jumpdest|push1|push2|push32|dup1|dup2|dup16|swap1|swap2|swap16|log0|log1|log4|create|call|callcode|return|delegatecall|suicide|returndatasize|returndatacopy|staticcall|revert|invalid)\b`, NameFunction, nil},
- {`[a-zA-Z_]\w*`, Name, nil},
- },
- "comments": {
- {`//([\w\W]*?\n)`, CommentSingle, nil},
- {`/[*][\w\W]*?[*]/`, CommentMultiline, nil},
- {`/[*][\w\W]*`, CommentMultiline, nil},
- },
- "keywords-other": {
- {Words(``, `\b`, `for`, `in`, `while`, `do`, `break`, `return`, `returns`, `continue`, `if`, `else`, `try`, `catch`, `throw`, `_`, `new`, `delete`, `is`, `as`, `from`, `memory`, `storage`), Keyword, nil},
- {`assembly\b`, Keyword, Push("assembly")},
- {`(contract|interface|enum|event|struct)(\s+)([a-zA-Z_]\w*)`, ByGroups(KeywordDeclaration, Text, NameClass), nil},
- {`(function|modifier)(\s+)([a-zA-Z_]\w*)`, ByGroups(KeywordDeclaration, Text, NameFunction), nil},
- {Words(``, `\b`, `contract`, `interface`, `enum`, `event`, `constructor`, `function`, `library`, `mapping`, `modifier`, `struct`, `var`), KeywordDeclaration, nil},
- {Words(``, `\b`, `abstract`, `external`, `internal`, `private`, `public`), Keyword, nil},
- {Words(``, `\b`, `anonymous`, `constant`, `immutable`, `indexed`, `override`, `payable`, `pure`, `view`, `virtual`), Keyword, nil},
- {`(import|using)\b`, KeywordNamespace, nil},
- {`pragma (solidity|experimental)\b`, Keyword, nil},
- {Words(``, `\b`, `after`, `alias`, `apply`, `auto`, `case`, `copyof`, `default`, `define`, `final`, `implements`, `inline`, `let`, `macro`, `match`, `mutable`, `null`, `of`, `partial`, `promise`, `reference`, `relocatable`, `sealed`, `sizeof`, `static`, `supports`, `switch`, `typedef`, `typeof`, `unchecked`), KeywordReserved, nil},
- {`(true|false)\b`, KeywordConstant, nil},
- {`(wei|finney|szabo|ether)\b`, KeywordConstant, nil},
- {`(seconds|minutes|hours|days|weeks|years)\b`, KeywordConstant, nil},
- },
- "keywords-types": {
- {Words(``, `\b`, `address`, `bool`, `byte`, `bytes`, `int`, `fixed`, `string`, `ufixed`, `uint`), KeywordType, nil},
- {Words(``, `\b`, `int8`, `int16`, `int24`, `int32`, `int40`, `int48`, `int56`, `int64`, `int72`, `int80`, `int88`, `int96`, `int104`, `int112`, `int120`, `int128`, `int136`, `int144`, `int152`, `int160`, `int168`, `int176`, `int184`, `int192`, `int200`, `int208`, `int216`, `int224`, `int232`, `int240`, `int248`, `int256`), KeywordType, nil},
- {Words(``, `\b`, `uint8`, `uint16`, `uint24`, `uint32`, `uint40`, `uint48`, `uint56`, `uint64`, `uint72`, `uint80`, `uint88`, `uint96`, `uint104`, `uint112`, `uint120`, `uint128`, `uint136`, `uint144`, `uint152`, `uint160`, `uint168`, `uint176`, `uint184`, `uint192`, `uint200`, `uint208`, `uint216`, `uint224`, `uint232`, `uint240`, `uint248`, `uint256`), KeywordType, nil},
- {Words(``, `\b`, `bytes1`, `bytes2`, `bytes3`, `bytes4`, `bytes5`, `bytes6`, `bytes7`, `bytes8`, `bytes9`, `bytes10`, `bytes11`, `bytes12`, `bytes13`, `bytes14`, `bytes15`, `bytes16`, `bytes17`, `bytes18`, `bytes19`, `bytes20`, `bytes21`, `bytes22`, `bytes23`, `bytes24`, `bytes25`, `bytes26`, `bytes27`, `bytes28`, `bytes29`, `bytes30`, `bytes31`, `bytes32`), KeywordType, nil},
- {Words(``, `\b`, `fixed8x0`, `fixed8x1`, `fixed8x2`, `fixed8x3`, `fixed8x4`, `fixed8x5`, `fixed8x6`, `fixed8x7`, `fixed8x8`, `fixed8x9`, `fixed8x10`, `fixed8x11`, `fixed8x12`, `fixed8x13`, `fixed8x14`, `fixed8x15`, `fixed8x16`, `fixed8x17`, `fixed8x18`, `fixed8x19`, `fixed8x20`, `fixed8x21`, `fixed8x22`, `fixed8x23`, `fixed8x24`, `fixed8x25`, `fixed8x26`, `fixed8x27`, `fixed8x28`, `fixed8x29`, `fixed8x30`, `fixed8x31`, `fixed8x32`, `fixed8x33`, `fixed8x34`, `fixed8x35`, `fixed8x36`, `fixed8x37`, `fixed8x38`, `fixed8x39`, `fixed8x40`, `fixed8x41`, `fixed8x42`, `fixed8x43`, `fixed8x44`, `fixed8x45`, `fixed8x46`, `fixed8x47`, `fixed8x48`, `fixed8x49`, `fixed8x50`, `fixed8x51`, `fixed8x52`, `fixed8x53`, `fixed8x54`, `fixed8x55`, `fixed8x56`, `fixed8x57`, `fixed8x58`, `fixed8x59`, `fixed8x60`, `fixed8x61`, `fixed8x62`, `fixed8x63`, `fixed8x64`, `fixed8x65`, `fixed8x66`, `fixed8x67`, `fixed8x68`, `fixed8x69`, `fixed8x70`, `fixed8x71`, `fixed8x72`, `fixed8x73`, `fixed8x74`, `fixed8x75`, `fixed8x76`, `fixed8x77`, `fixed8x78`, `fixed8x79`, `fixed8x80`, `fixed16x0`, `fixed16x1`, `fixed16x2`, `fixed16x3`, `fixed16x4`, `fixed16x5`, `fixed16x6`, `fixed16x7`, `fixed16x8`, `fixed16x9`, `fixed16x10`, `fixed16x11`, `fixed16x12`, `fixed16x13`, `fixed16x14`, `fixed16x15`, `fixed16x16`, `fixed16x17`, `fixed16x18`, `fixed16x19`, `fixed16x20`, `fixed16x21`, `fixed16x22`, `fixed16x23`, `fixed16x24`, `fixed16x25`, `fixed16x26`, `fixed16x27`, `fixed16x28`, `fixed16x29`, `fixed16x30`, `fixed16x31`, `fixed16x32`, `fixed16x33`, `fixed16x34`, `fixed16x35`, `fixed16x36`, `fixed16x37`, `fixed16x38`, `fixed16x39`, `fixed16x40`, `fixed16x41`, `fixed16x42`, `fixed16x43`, `fixed16x44`, `fixed16x45`, `fixed16x46`, `fixed16x47`, `fixed16x48`, `fixed16x49`, `fixed16x50`, `fixed16x51`, `fixed16x52`, `fixed16x53`, `fixed16x54`, `fixed16x55`, `fixed16x56`, `fixed16x57`, `fixed16x58`, `fixed16x59`, `fixed16x60`, `fixed16x61`, `fixed16x62`, `fixed16x63`, `fixed16x64`, `fixed16x65`, `fixed16x66`, `fixed16x67`, `fixed16x68`, `fixed16x69`, `fixed16x70`, `fixed16x71`, `fixed16x72`, `fixed16x73`, `fixed16x74`, `fixed16x75`, `fixed16x76`, `fixed16x77`, `fixed16x78`, `fixed16x79`, `fixed16x80`, `fixed24x0`, `fixed24x1`, `fixed24x2`, `fixed24x3`, `fixed24x4`, `fixed24x5`, `fixed24x6`, `fixed24x7`, `fixed24x8`, `fixed24x9`, `fixed24x10`, `fixed24x11`, `fixed24x12`, `fixed24x13`, `fixed24x14`, `fixed24x15`, `fixed24x16`, `fixed24x17`, `fixed24x18`, `fixed24x19`, `fixed24x20`, `fixed24x21`, `fixed24x22`, `fixed24x23`, `fixed24x24`, `fixed24x25`, `fixed24x26`, `fixed24x27`, `fixed24x28`, `fixed24x29`, `fixed24x30`, `fixed24x31`, `fixed24x32`, `fixed24x33`, `fixed24x34`, `fixed24x35`, `fixed24x36`, `fixed24x37`, `fixed24x38`, `fixed24x39`, `fixed24x40`, `fixed24x41`, `fixed24x42`, `fixed24x43`, `fixed24x44`, `fixed24x45`, `fixed24x46`, `fixed24x47`, `fixed24x48`, `fixed24x49`, `fixed24x50`, `fixed24x51`, `fixed24x52`, `fixed24x53`, `fixed24x54`, `fixed24x55`, `fixed24x56`, `fixed24x57`, `fixed24x58`, `fixed24x59`, `fixed24x60`, `fixed24x61`, `fixed24x62`, `fixed24x63`, `fixed24x64`, `fixed24x65`, `fixed24x66`, `fixed24x67`, `fixed24x68`, `fixed24x69`, `fixed24x70`, `fixed24x71`, `fixed24x72`, `fixed24x73`, `fixed24x74`, `fixed24x75`, `fixed24x76`, `fixed24x77`, `fixed24x78`, `fixed24x79`, `fixed24x80`, `fixed32x0`, `fixed32x1`, `fixed32x2`, `fixed32x3`, `fixed32x4`, `fixed32x5`, `fixed32x6`, `fixed32x7`, `fixed32x8`, `fixed32x9`, `fixed32x10`, `fixed32x11`, `fixed32x12`, `fixed32x13`, `fixed32x14`, `fixed32x15`, `fixed32x16`, `fixed32x17`, `fixed32x18`, `fixed32x19`, `fixed32x20`, `fixed32x21`, `fixed32x22`, `fixed32x23`, `fixed32x24`, `fixed32x25`, `fixed32x26`, `fixed32x27`, `fixed32x28`, `fixed32x29`, `fixed32x30`, `fixed32x31`, `fixed32x32`, `fixed32x33`, `fixed32x34`, `fixed32x35`, `fixed32x36`, `fixed32x37`, `fixed32x38`, `fixed32x39`, `fixed32x40`, `fixed32x41`, `fixed32x42`, `fixed32x43`, `fixed32x44`, `fixed32x45`, `fixed32x46`, `fixed32x47`, `fixed32x48`, `fixed32x49`, `fixed32x50`, `fixed32x51`, `fixed32x52`, `fixed32x53`, `fixed32x54`, `fixed32x55`, `fixed32x56`, `fixed32x57`, `fixed32x58`, `fixed32x59`, `fixed32x60`, `fixed32x61`, `fixed32x62`, `fixed32x63`, `fixed32x64`, `fixed32x65`, `fixed32x66`, `fixed32x67`, `fixed32x68`, `fixed32x69`, `fixed32x70`, `fixed32x71`, `fixed32x72`, `fixed32x73`, `fixed32x74`, `fixed32x75`, `fixed32x76`, `fixed32x77`, `fixed32x78`, `fixed32x79`, `fixed32x80`, `fixed40x0`, `fixed40x1`, `fixed40x2`, `fixed40x3`, `fixed40x4`, `fixed40x5`, `fixed40x6`, `fixed40x7`, `fixed40x8`, `fixed40x9`, `fixed40x10`, `fixed40x11`, `fixed40x12`, `fixed40x13`, `fixed40x14`, `fixed40x15`, `fixed40x16`, `fixed40x17`, `fixed40x18`, `fixed40x19`, `fixed40x20`, `fixed40x21`, `fixed40x22`, `fixed40x23`, `fixed40x24`, `fixed40x25`, `fixed40x26`, `fixed40x27`, `fixed40x28`, `fixed40x29`, `fixed40x30`, `fixed40x31`, `fixed40x32`, `fixed40x33`, `fixed40x34`, `fixed40x35`, `fixed40x36`, `fixed40x37`, `fixed40x38`, `fixed40x39`, `fixed40x40`, `fixed40x41`, `fixed40x42`, `fixed40x43`, `fixed40x44`, `fixed40x45`, `fixed40x46`, `fixed40x47`, `fixed40x48`, `fixed40x49`, `fixed40x50`, `fixed40x51`, `fixed40x52`, `fixed40x53`, `fixed40x54`, `fixed40x55`, `fixed40x56`, `fixed40x57`, `fixed40x58`, `fixed40x59`, `fixed40x60`, `fixed40x61`, `fixed40x62`, `fixed40x63`, `fixed40x64`, `fixed40x65`, `fixed40x66`, `fixed40x67`, `fixed40x68`, `fixed40x69`, `fixed40x70`, `fixed40x71`, `fixed40x72`, `fixed40x73`, `fixed40x74`, `fixed40x75`, `fixed40x76`, `fixed40x77`, `fixed40x78`, `fixed40x79`, `fixed40x80`, `fixed48x0`, `fixed48x1`, `fixed48x2`, `fixed48x3`, `fixed48x4`, `fixed48x5`, `fixed48x6`, `fixed48x7`, `fixed48x8`, `fixed48x9`, `fixed48x10`, `fixed48x11`, `fixed48x12`, `fixed48x13`, `fixed48x14`, `fixed48x15`, `fixed48x16`, `fixed48x17`, `fixed48x18`, `fixed48x19`, `fixed48x20`, `fixed48x21`, `fixed48x22`, `fixed48x23`, `fixed48x24`, `fixed48x25`, `fixed48x26`, `fixed48x27`, `fixed48x28`, `fixed48x29`, `fixed48x30`, `fixed48x31`, `fixed48x32`, `fixed48x33`, `fixed48x34`, `fixed48x35`, `fixed48x36`, `fixed48x37`, `fixed48x38`, `fixed48x39`, `fixed48x40`, `fixed48x41`, `fixed48x42`, `fixed48x43`, `fixed48x44`, `fixed48x45`, `fixed48x46`, `fixed48x47`, `fixed48x48`, `fixed48x49`, `fixed48x50`, `fixed48x51`, `fixed48x52`, `fixed48x53`, `fixed48x54`, `fixed48x55`, `fixed48x56`, `fixed48x57`, `fixed48x58`, `fixed48x59`, `fixed48x60`, `fixed48x61`, `fixed48x62`, `fixed48x63`, `fixed48x64`, `fixed48x65`, `fixed48x66`, `fixed48x67`, `fixed48x68`, `fixed48x69`, `fixed48x70`, `fixed48x71`, `fixed48x72`, `fixed48x73`, `fixed48x74`, `fixed48x75`, `fixed48x76`, `fixed48x77`, `fixed48x78`, `fixed48x79`, `fixed48x80`, `fixed56x0`, `fixed56x1`, `fixed56x2`, `fixed56x3`, `fixed56x4`, `fixed56x5`, `fixed56x6`, `fixed56x7`, `fixed56x8`, `fixed56x9`, `fixed56x10`, `fixed56x11`, `fixed56x12`, `fixed56x13`, `fixed56x14`, `fixed56x15`, `fixed56x16`, `fixed56x17`, `fixed56x18`, `fixed56x19`, `fixed56x20`, `fixed56x21`, `fixed56x22`, `fixed56x23`, `fixed56x24`, `fixed56x25`, `fixed56x26`, `fixed56x27`, `fixed56x28`, `fixed56x29`, `fixed56x30`, `fixed56x31`, `fixed56x32`, `fixed56x33`, `fixed56x34`, `fixed56x35`, `fixed56x36`, `fixed56x37`, `fixed56x38`, `fixed56x39`, `fixed56x40`, `fixed56x41`, `fixed56x42`, `fixed56x43`, `fixed56x44`, `fixed56x45`, `fixed56x46`, `fixed56x47`, `fixed56x48`, `fixed56x49`, `fixed56x50`, `fixed56x51`, `fixed56x52`, `fixed56x53`, `fixed56x54`, `fixed56x55`, `fixed56x56`, `fixed56x57`, `fixed56x58`, `fixed56x59`, `fixed56x60`, `fixed56x61`, `fixed56x62`, `fixed56x63`, `fixed56x64`, `fixed56x65`, `fixed56x66`, `fixed56x67`, `fixed56x68`, `fixed56x69`, `fixed56x70`, `fixed56x71`, `fixed56x72`, `fixed56x73`, `fixed56x74`, `fixed56x75`, `fixed56x76`, `fixed56x77`, `fixed56x78`, `fixed56x79`, `fixed56x80`, `fixed64x0`, `fixed64x1`, `fixed64x2`, `fixed64x3`, `fixed64x4`, `fixed64x5`, `fixed64x6`, `fixed64x7`, `fixed64x8`, `fixed64x9`, `fixed64x10`, `fixed64x11`, `fixed64x12`, `fixed64x13`, `fixed64x14`, `fixed64x15`, `fixed64x16`, `fixed64x17`, `fixed64x18`, `fixed64x19`, `fixed64x20`, `fixed64x21`, `fixed64x22`, `fixed64x23`, `fixed64x24`, `fixed64x25`, `fixed64x26`, `fixed64x27`, `fixed64x28`, `fixed64x29`, `fixed64x30`, `fixed64x31`, `fixed64x32`, `fixed64x33`, `fixed64x34`, `fixed64x35`, `fixed64x36`, `fixed64x37`, `fixed64x38`, `fixed64x39`, `fixed64x40`, `fixed64x41`, `fixed64x42`, `fixed64x43`, `fixed64x44`, `fixed64x45`, `fixed64x46`, `fixed64x47`, `fixed64x48`, `fixed64x49`, `fixed64x50`, `fixed64x51`, `fixed64x52`, `fixed64x53`, `fixed64x54`, `fixed64x55`, `fixed64x56`, `fixed64x57`, `fixed64x58`, `fixed64x59`, `fixed64x60`, `fixed64x61`, `fixed64x62`, `fixed64x63`, `fixed64x64`, `fixed64x65`, `fixed64x66`, `fixed64x67`, `fixed64x68`, `fixed64x69`, `fixed64x70`, `fixed64x71`, `fixed64x72`, `fixed64x73`, `fixed64x74`, `fixed64x75`, `fixed64x76`, `fixed64x77`, `fixed64x78`, `fixed64x79`, `fixed64x80`, `fixed72x0`, `fixed72x1`, `fixed72x2`, `fixed72x3`, `fixed72x4`, `fixed72x5`, `fixed72x6`, `fixed72x7`, `fixed72x8`, `fixed72x9`, `fixed72x10`, `fixed72x11`, `fixed72x12`, `fixed72x13`, `fixed72x14`, `fixed72x15`, `fixed72x16`, `fixed72x17`, `fixed72x18`, `fixed72x19`, `fixed72x20`, `fixed72x21`, `fixed72x22`, `fixed72x23`, `fixed72x24`, `fixed72x25`, `fixed72x26`, `fixed72x27`, `fixed72x28`, `fixed72x29`, `fixed72x30`, `fixed72x31`, `fixed72x32`, `fixed72x33`, `fixed72x34`, `fixed72x35`, `fixed72x36`, `fixed72x37`, `fixed72x38`, `fixed72x39`, `fixed72x40`, `fixed72x41`, `fixed72x42`, `fixed72x43`, `fixed72x44`, `fixed72x45`, `fixed72x46`, `fixed72x47`, `fixed72x48`, `fixed72x49`, `fixed72x50`, `fixed72x51`, `fixed72x52`, `fixed72x53`, `fixed72x54`, `fixed72x55`, `fixed72x56`, `fixed72x57`, `fixed72x58`, `fixed72x59`, `fixed72x60`, `fixed72x61`, `fixed72x62`, `fixed72x63`, `fixed72x64`, `fixed72x65`, `fixed72x66`, `fixed72x67`, `fixed72x68`, `fixed72x69`, `fixed72x70`, `fixed72x71`, `fixed72x72`, `fixed72x73`, `fixed72x74`, `fixed72x75`, `fixed72x76`, `fixed72x77`, `fixed72x78`, `fixed72x79`, `fixed72x80`, `fixed80x0`, `fixed80x1`, `fixed80x2`, `fixed80x3`, `fixed80x4`, `fixed80x5`, `fixed80x6`, `fixed80x7`, `fixed80x8`, `fixed80x9`, `fixed80x10`, `fixed80x11`, `fixed80x12`, `fixed80x13`, `fixed80x14`, `fixed80x15`, `fixed80x16`, `fixed80x17`, `fixed80x18`, `fixed80x19`, `fixed80x20`, `fixed80x21`, `fixed80x22`, `fixed80x23`, `fixed80x24`, `fixed80x25`, `fixed80x26`, `fixed80x27`, `fixed80x28`, `fixed80x29`, `fixed80x30`, `fixed80x31`, `fixed80x32`, `fixed80x33`, `fixed80x34`, `fixed80x35`, `fixed80x36`, `fixed80x37`, `fixed80x38`, `fixed80x39`, `fixed80x40`, `fixed80x41`, `fixed80x42`, `fixed80x43`, `fixed80x44`, `fixed80x45`, `fixed80x46`, `fixed80x47`, `fixed80x48`, `fixed80x49`, `fixed80x50`, `fixed80x51`, `fixed80x52`, `fixed80x53`, `fixed80x54`, `fixed80x55`, `fixed80x56`, `fixed80x57`, `fixed80x58`, `fixed80x59`, `fixed80x60`, `fixed80x61`, `fixed80x62`, `fixed80x63`, `fixed80x64`, `fixed80x65`, `fixed80x66`, `fixed80x67`, `fixed80x68`, `fixed80x69`, `fixed80x70`, `fixed80x71`, `fixed80x72`, `fixed80x73`, `fixed80x74`, `fixed80x75`, `fixed80x76`, `fixed80x77`, `fixed80x78`, `fixed80x79`, `fixed80x80`, `fixed88x0`, `fixed88x1`, `fixed88x2`, `fixed88x3`, `fixed88x4`, `fixed88x5`, `fixed88x6`, `fixed88x7`, `fixed88x8`, `fixed88x9`, `fixed88x10`, `fixed88x11`, `fixed88x12`, `fixed88x13`, `fixed88x14`, `fixed88x15`, `fixed88x16`, `fixed88x17`, `fixed88x18`, `fixed88x19`, `fixed88x20`, `fixed88x21`, `fixed88x22`, `fixed88x23`, `fixed88x24`, `fixed88x25`, `fixed88x26`, `fixed88x27`, `fixed88x28`, `fixed88x29`, `fixed88x30`, `fixed88x31`, `fixed88x32`, `fixed88x33`, `fixed88x34`, `fixed88x35`, `fixed88x36`, `fixed88x37`, `fixed88x38`, `fixed88x39`, `fixed88x40`, `fixed88x41`, `fixed88x42`, `fixed88x43`, `fixed88x44`, `fixed88x45`, `fixed88x46`, `fixed88x47`, `fixed88x48`, `fixed88x49`, `fixed88x50`, `fixed88x51`, `fixed88x52`, `fixed88x53`, `fixed88x54`, `fixed88x55`, `fixed88x56`, `fixed88x57`, `fixed88x58`, `fixed88x59`, `fixed88x60`, `fixed88x61`, `fixed88x62`, `fixed88x63`, `fixed88x64`, `fixed88x65`, `fixed88x66`, `fixed88x67`, `fixed88x68`, `fixed88x69`, `fixed88x70`, `fixed88x71`, `fixed88x72`, `fixed88x73`, `fixed88x74`, `fixed88x75`, `fixed88x76`, `fixed88x77`, `fixed88x78`, `fixed88x79`, `fixed88x80`, `fixed96x0`, `fixed96x1`, `fixed96x2`, `fixed96x3`, `fixed96x4`, `fixed96x5`, `fixed96x6`, `fixed96x7`, `fixed96x8`, `fixed96x9`, `fixed96x10`, `fixed96x11`, `fixed96x12`, `fixed96x13`, `fixed96x14`, `fixed96x15`, `fixed96x16`, `fixed96x17`, `fixed96x18`, `fixed96x19`, `fixed96x20`, `fixed96x21`, `fixed96x22`, `fixed96x23`, `fixed96x24`, `fixed96x25`, `fixed96x26`, `fixed96x27`, `fixed96x28`, `fixed96x29`, `fixed96x30`, `fixed96x31`, `fixed96x32`, `fixed96x33`, `fixed96x34`, `fixed96x35`, `fixed96x36`, `fixed96x37`, `fixed96x38`, `fixed96x39`, `fixed96x40`, `fixed96x41`, `fixed96x42`, `fixed96x43`, `fixed96x44`, `fixed96x45`, `fixed96x46`, `fixed96x47`, `fixed96x48`, `fixed96x49`, `fixed96x50`, `fixed96x51`, `fixed96x52`, `fixed96x53`, `fixed96x54`, `fixed96x55`, `fixed96x56`, `fixed96x57`, `fixed96x58`, `fixed96x59`, `fixed96x60`, `fixed96x61`, `fixed96x62`, `fixed96x63`, `fixed96x64`, `fixed96x65`, `fixed96x66`, `fixed96x67`, `fixed96x68`, `fixed96x69`, `fixed96x70`, `fixed96x71`, `fixed96x72`, `fixed96x73`, `fixed96x74`, `fixed96x75`, `fixed96x76`, `fixed96x77`, `fixed96x78`, `fixed96x79`, `fixed96x80`, `fixed104x0`, `fixed104x1`, `fixed104x2`, `fixed104x3`, `fixed104x4`, `fixed104x5`, `fixed104x6`, `fixed104x7`, `fixed104x8`, `fixed104x9`, `fixed104x10`, `fixed104x11`, `fixed104x12`, `fixed104x13`, `fixed104x14`, `fixed104x15`, `fixed104x16`, `fixed104x17`, `fixed104x18`, `fixed104x19`, `fixed104x20`, `fixed104x21`, `fixed104x22`, `fixed104x23`, `fixed104x24`, `fixed104x25`, `fixed104x26`, `fixed104x27`, `fixed104x28`, `fixed104x29`, `fixed104x30`, `fixed104x31`, `fixed104x32`, `fixed104x33`, `fixed104x34`, `fixed104x35`, `fixed104x36`, `fixed104x37`, `fixed104x38`, `fixed104x39`, `fixed104x40`, `fixed104x41`, `fixed104x42`, `fixed104x43`, `fixed104x44`, `fixed104x45`, `fixed104x46`, `fixed104x47`, `fixed104x48`, `fixed104x49`, `fixed104x50`, `fixed104x51`, `fixed104x52`, `fixed104x53`, `fixed104x54`, `fixed104x55`, `fixed104x56`, `fixed104x57`, `fixed104x58`, `fixed104x59`, `fixed104x60`, `fixed104x61`, `fixed104x62`, `fixed104x63`, `fixed104x64`, `fixed104x65`, `fixed104x66`, `fixed104x67`, `fixed104x68`, `fixed104x69`, `fixed104x70`, `fixed104x71`, `fixed104x72`, `fixed104x73`, `fixed104x74`, `fixed104x75`, `fixed104x76`, `fixed104x77`, `fixed104x78`, `fixed104x79`, `fixed104x80`, `fixed112x0`, `fixed112x1`, `fixed112x2`, `fixed112x3`, `fixed112x4`, `fixed112x5`, `fixed112x6`, `fixed112x7`, `fixed112x8`, `fixed112x9`, `fixed112x10`, `fixed112x11`, `fixed112x12`, `fixed112x13`, `fixed112x14`, `fixed112x15`, `fixed112x16`, `fixed112x17`, `fixed112x18`, `fixed112x19`, `fixed112x20`, `fixed112x21`, `fixed112x22`, `fixed112x23`, `fixed112x24`, `fixed112x25`, `fixed112x26`, `fixed112x27`, `fixed112x28`, `fixed112x29`, `fixed112x30`, `fixed112x31`, `fixed112x32`, `fixed112x33`, `fixed112x34`, `fixed112x35`, `fixed112x36`, `fixed112x37`, `fixed112x38`, `fixed112x39`, `fixed112x40`, `fixed112x41`, `fixed112x42`, `fixed112x43`, `fixed112x44`, `fixed112x45`, `fixed112x46`, `fixed112x47`, `fixed112x48`, `fixed112x49`, `fixed112x50`, `fixed112x51`, `fixed112x52`, `fixed112x53`, `fixed112x54`, `fixed112x55`, `fixed112x56`, `fixed112x57`, `fixed112x58`, `fixed112x59`, `fixed112x60`, `fixed112x61`, `fixed112x62`, `fixed112x63`, `fixed112x64`, `fixed112x65`, `fixed112x66`, `fixed112x67`, `fixed112x68`, `fixed112x69`, `fixed112x70`, `fixed112x71`, `fixed112x72`, `fixed112x73`, `fixed112x74`, `fixed112x75`, `fixed112x76`, `fixed112x77`, `fixed112x78`, `fixed112x79`, `fixed112x80`, `fixed120x0`, `fixed120x1`, `fixed120x2`, `fixed120x3`, `fixed120x4`, `fixed120x5`, `fixed120x6`, `fixed120x7`, `fixed120x8`, `fixed120x9`, `fixed120x10`, `fixed120x11`, `fixed120x12`, `fixed120x13`, `fixed120x14`, `fixed120x15`, `fixed120x16`, `fixed120x17`, `fixed120x18`, `fixed120x19`, `fixed120x20`, `fixed120x21`, `fixed120x22`, `fixed120x23`, `fixed120x24`, `fixed120x25`, `fixed120x26`, `fixed120x27`, `fixed120x28`, `fixed120x29`, `fixed120x30`, `fixed120x31`, `fixed120x32`, `fixed120x33`, `fixed120x34`, `fixed120x35`, `fixed120x36`, `fixed120x37`, `fixed120x38`, `fixed120x39`, `fixed120x40`, `fixed120x41`, `fixed120x42`, `fixed120x43`, `fixed120x44`, `fixed120x45`, `fixed120x46`, `fixed120x47`, `fixed120x48`, `fixed120x49`, `fixed120x50`, `fixed120x51`, `fixed120x52`, `fixed120x53`, `fixed120x54`, `fixed120x55`, `fixed120x56`, `fixed120x57`, `fixed120x58`, `fixed120x59`, `fixed120x60`, `fixed120x61`, `fixed120x62`, `fixed120x63`, `fixed120x64`, `fixed120x65`, `fixed120x66`, `fixed120x67`, `fixed120x68`, `fixed120x69`, `fixed120x70`, `fixed120x71`, `fixed120x72`, `fixed120x73`, `fixed120x74`, `fixed120x75`, `fixed120x76`, `fixed120x77`, `fixed120x78`, `fixed120x79`, `fixed120x80`, `fixed128x0`, `fixed128x1`, `fixed128x2`, `fixed128x3`, `fixed128x4`, `fixed128x5`, `fixed128x6`, `fixed128x7`, `fixed128x8`, `fixed128x9`, `fixed128x10`, `fixed128x11`, `fixed128x12`, `fixed128x13`, `fixed128x14`, `fixed128x15`, `fixed128x16`, `fixed128x17`, `fixed128x18`, `fixed128x19`, `fixed128x20`, `fixed128x21`, `fixed128x22`, `fixed128x23`, `fixed128x24`, `fixed128x25`, `fixed128x26`, `fixed128x27`, `fixed128x28`, `fixed128x29`, `fixed128x30`, `fixed128x31`, `fixed128x32`, `fixed128x33`, `fixed128x34`, `fixed128x35`, `fixed128x36`, `fixed128x37`, `fixed128x38`, `fixed128x39`, `fixed128x40`, `fixed128x41`, `fixed128x42`, `fixed128x43`, `fixed128x44`, `fixed128x45`, `fixed128x46`, `fixed128x47`, `fixed128x48`, `fixed128x49`, `fixed128x50`, `fixed128x51`, `fixed128x52`, `fixed128x53`, `fixed128x54`, `fixed128x55`, `fixed128x56`, `fixed128x57`, `fixed128x58`, `fixed128x59`, `fixed128x60`, `fixed128x61`, `fixed128x62`, `fixed128x63`, `fixed128x64`, `fixed128x65`, `fixed128x66`, `fixed128x67`, `fixed128x68`, `fixed128x69`, `fixed128x70`, `fixed128x71`, `fixed128x72`, `fixed128x73`, `fixed128x74`, `fixed128x75`, `fixed128x76`, `fixed128x77`, `fixed128x78`, `fixed128x79`, `fixed128x80`, `fixed136x0`, `fixed136x1`, `fixed136x2`, `fixed136x3`, `fixed136x4`, `fixed136x5`, `fixed136x6`, `fixed136x7`, `fixed136x8`, `fixed136x9`, `fixed136x10`, `fixed136x11`, `fixed136x12`, `fixed136x13`, `fixed136x14`, `fixed136x15`, `fixed136x16`, `fixed136x17`, `fixed136x18`, `fixed136x19`, `fixed136x20`, `fixed136x21`, `fixed136x22`, `fixed136x23`, `fixed136x24`, `fixed136x25`, `fixed136x26`, `fixed136x27`, `fixed136x28`, `fixed136x29`, `fixed136x30`, `fixed136x31`, `fixed136x32`, `fixed136x33`, `fixed136x34`, `fixed136x35`, `fixed136x36`, `fixed136x37`, `fixed136x38`, `fixed136x39`, `fixed136x40`, `fixed136x41`, `fixed136x42`, `fixed136x43`, `fixed136x44`, `fixed136x45`, `fixed136x46`, `fixed136x47`, `fixed136x48`, `fixed136x49`, `fixed136x50`, `fixed136x51`, `fixed136x52`, `fixed136x53`, `fixed136x54`, `fixed136x55`, `fixed136x56`, `fixed136x57`, `fixed136x58`, `fixed136x59`, `fixed136x60`, `fixed136x61`, `fixed136x62`, `fixed136x63`, `fixed136x64`, `fixed136x65`, `fixed136x66`, `fixed136x67`, `fixed136x68`, `fixed136x69`, `fixed136x70`, `fixed136x71`, `fixed136x72`, `fixed136x73`, `fixed136x74`, `fixed136x75`, `fixed136x76`, `fixed136x77`, `fixed136x78`, `fixed136x79`, `fixed136x80`, `fixed144x0`, `fixed144x1`, `fixed144x2`, `fixed144x3`, `fixed144x4`, `fixed144x5`, `fixed144x6`, `fixed144x7`, `fixed144x8`, `fixed144x9`, `fixed144x10`, `fixed144x11`, `fixed144x12`, `fixed144x13`, `fixed144x14`, `fixed144x15`, `fixed144x16`, `fixed144x17`, `fixed144x18`, `fixed144x19`, `fixed144x20`, `fixed144x21`, `fixed144x22`, `fixed144x23`, `fixed144x24`, `fixed144x25`, `fixed144x26`, `fixed144x27`, `fixed144x28`, `fixed144x29`, `fixed144x30`, `fixed144x31`, `fixed144x32`, `fixed144x33`, `fixed144x34`, `fixed144x35`, `fixed144x36`, `fixed144x37`, `fixed144x38`, `fixed144x39`, `fixed144x40`, `fixed144x41`, `fixed144x42`, `fixed144x43`, `fixed144x44`, `fixed144x45`, `fixed144x46`, `fixed144x47`, `fixed144x48`, `fixed144x49`, `fixed144x50`, `fixed144x51`, `fixed144x52`, `fixed144x53`, `fixed144x54`, `fixed144x55`, `fixed144x56`, `fixed144x57`, `fixed144x58`, `fixed144x59`, `fixed144x60`, `fixed144x61`, `fixed144x62`, `fixed144x63`, `fixed144x64`, `fixed144x65`, `fixed144x66`, `fixed144x67`, `fixed144x68`, `fixed144x69`, `fixed144x70`, `fixed144x71`, `fixed144x72`, `fixed144x73`, `fixed144x74`, `fixed144x75`, `fixed144x76`, `fixed144x77`, `fixed144x78`, `fixed144x79`, `fixed144x80`, `fixed152x0`, `fixed152x1`, `fixed152x2`, `fixed152x3`, `fixed152x4`, `fixed152x5`, `fixed152x6`, `fixed152x7`, `fixed152x8`, `fixed152x9`, `fixed152x10`, `fixed152x11`, `fixed152x12`, `fixed152x13`, `fixed152x14`, `fixed152x15`, `fixed152x16`, `fixed152x17`, `fixed152x18`, `fixed152x19`, `fixed152x20`, `fixed152x21`, `fixed152x22`, `fixed152x23`, `fixed152x24`, `fixed152x25`, `fixed152x26`, `fixed152x27`, `fixed152x28`, `fixed152x29`, `fixed152x30`, `fixed152x31`, `fixed152x32`, `fixed152x33`, `fixed152x34`, `fixed152x35`, `fixed152x36`, `fixed152x37`, `fixed152x38`, `fixed152x39`, `fixed152x40`, `fixed152x41`, `fixed152x42`, `fixed152x43`, `fixed152x44`, `fixed152x45`, `fixed152x46`, `fixed152x47`, `fixed152x48`, `fixed152x49`, `fixed152x50`, `fixed152x51`, `fixed152x52`, `fixed152x53`, `fixed152x54`, `fixed152x55`, `fixed152x56`, `fixed152x57`, `fixed152x58`, `fixed152x59`, `fixed152x60`, `fixed152x61`, `fixed152x62`, `fixed152x63`, `fixed152x64`, `fixed152x65`, `fixed152x66`, `fixed152x67`, `fixed152x68`, `fixed152x69`, `fixed152x70`, `fixed152x71`, `fixed152x72`, `fixed152x73`, `fixed152x74`, `fixed152x75`, `fixed152x76`, `fixed152x77`, `fixed152x78`, `fixed152x79`, `fixed152x80`, `fixed160x0`, `fixed160x1`, `fixed160x2`, `fixed160x3`, `fixed160x4`, `fixed160x5`, `fixed160x6`, `fixed160x7`, `fixed160x8`, `fixed160x9`, `fixed160x10`, `fixed160x11`, `fixed160x12`, `fixed160x13`, `fixed160x14`, `fixed160x15`, `fixed160x16`, `fixed160x17`, `fixed160x18`, `fixed160x19`, `fixed160x20`, `fixed160x21`, `fixed160x22`, `fixed160x23`, `fixed160x24`, `fixed160x25`, `fixed160x26`, `fixed160x27`, `fixed160x28`, `fixed160x29`, `fixed160x30`, `fixed160x31`, `fixed160x32`, `fixed160x33`, `fixed160x34`, `fixed160x35`, `fixed160x36`, `fixed160x37`, `fixed160x38`, `fixed160x39`, `fixed160x40`, `fixed160x41`, `fixed160x42`, `fixed160x43`, `fixed160x44`, `fixed160x45`, `fixed160x46`, `fixed160x47`, `fixed160x48`, `fixed160x49`, `fixed160x50`, `fixed160x51`, `fixed160x52`, `fixed160x53`, `fixed160x54`, `fixed160x55`, `fixed160x56`, `fixed160x57`, `fixed160x58`, `fixed160x59`, `fixed160x60`, `fixed160x61`, `fixed160x62`, `fixed160x63`, `fixed160x64`, `fixed160x65`, `fixed160x66`, `fixed160x67`, `fixed160x68`, `fixed160x69`, `fixed160x70`, `fixed160x71`, `fixed160x72`, `fixed160x73`, `fixed160x74`, `fixed160x75`, `fixed160x76`, `fixed160x77`, `fixed160x78`, `fixed160x79`, `fixed160x80`, `fixed168x0`, `fixed168x1`, `fixed168x2`, `fixed168x3`, `fixed168x4`, `fixed168x5`, `fixed168x6`, `fixed168x7`, `fixed168x8`, `fixed168x9`, `fixed168x10`, `fixed168x11`, `fixed168x12`, `fixed168x13`, `fixed168x14`, `fixed168x15`, `fixed168x16`, `fixed168x17`, `fixed168x18`, `fixed168x19`, `fixed168x20`, `fixed168x21`, `fixed168x22`, `fixed168x23`, `fixed168x24`, `fixed168x25`, `fixed168x26`, `fixed168x27`, `fixed168x28`, `fixed168x29`, `fixed168x30`, `fixed168x31`, `fixed168x32`, `fixed168x33`, `fixed168x34`, `fixed168x35`, `fixed168x36`, `fixed168x37`, `fixed168x38`, `fixed168x39`, `fixed168x40`, `fixed168x41`, `fixed168x42`, `fixed168x43`, `fixed168x44`, `fixed168x45`, `fixed168x46`, `fixed168x47`, `fixed168x48`, `fixed168x49`, `fixed168x50`, `fixed168x51`, `fixed168x52`, `fixed168x53`, `fixed168x54`, `fixed168x55`, `fixed168x56`, `fixed168x57`, `fixed168x58`, `fixed168x59`, `fixed168x60`, `fixed168x61`, `fixed168x62`, `fixed168x63`, `fixed168x64`, `fixed168x65`, `fixed168x66`, `fixed168x67`, `fixed168x68`, `fixed168x69`, `fixed168x70`, `fixed168x71`, `fixed168x72`, `fixed168x73`, `fixed168x74`, `fixed168x75`, `fixed168x76`, `fixed168x77`, `fixed168x78`, `fixed168x79`, `fixed168x80`, `fixed176x0`, `fixed176x1`, `fixed176x2`, `fixed176x3`, `fixed176x4`, `fixed176x5`, `fixed176x6`, `fixed176x7`, `fixed176x8`, `fixed176x9`, `fixed176x10`, `fixed176x11`, `fixed176x12`, `fixed176x13`, `fixed176x14`, `fixed176x15`, `fixed176x16`, `fixed176x17`, `fixed176x18`, `fixed176x19`, `fixed176x20`, `fixed176x21`, `fixed176x22`, `fixed176x23`, `fixed176x24`, `fixed176x25`, `fixed176x26`, `fixed176x27`, `fixed176x28`, `fixed176x29`, `fixed176x30`, `fixed176x31`, `fixed176x32`, `fixed176x33`, `fixed176x34`, `fixed176x35`, `fixed176x36`, `fixed176x37`, `fixed176x38`, `fixed176x39`, `fixed176x40`, `fixed176x41`, `fixed176x42`, `fixed176x43`, `fixed176x44`, `fixed176x45`, `fixed176x46`, `fixed176x47`, `fixed176x48`, `fixed176x49`, `fixed176x50`, `fixed176x51`, `fixed176x52`, `fixed176x53`, `fixed176x54`, `fixed176x55`, `fixed176x56`, `fixed176x57`, `fixed176x58`, `fixed176x59`, `fixed176x60`, `fixed176x61`, `fixed176x62`, `fixed176x63`, `fixed176x64`, `fixed176x65`, `fixed176x66`, `fixed176x67`, `fixed176x68`, `fixed176x69`, `fixed176x70`, `fixed176x71`, `fixed176x72`, `fixed176x73`, `fixed176x74`, `fixed176x75`, `fixed176x76`, `fixed176x77`, `fixed176x78`, `fixed176x79`, `fixed176x80`, `fixed184x0`, `fixed184x1`, `fixed184x2`, `fixed184x3`, `fixed184x4`, `fixed184x5`, `fixed184x6`, `fixed184x7`, `fixed184x8`, `fixed184x9`, `fixed184x10`, `fixed184x11`, `fixed184x12`, `fixed184x13`, `fixed184x14`, `fixed184x15`, `fixed184x16`, `fixed184x17`, `fixed184x18`, `fixed184x19`, `fixed184x20`, `fixed184x21`, `fixed184x22`, `fixed184x23`, `fixed184x24`, `fixed184x25`, `fixed184x26`, `fixed184x27`, `fixed184x28`, `fixed184x29`, `fixed184x30`, `fixed184x31`, `fixed184x32`, `fixed184x33`, `fixed184x34`, `fixed184x35`, `fixed184x36`, `fixed184x37`, `fixed184x38`, `fixed184x39`, `fixed184x40`, `fixed184x41`, `fixed184x42`, `fixed184x43`, `fixed184x44`, `fixed184x45`, `fixed184x46`, `fixed184x47`, `fixed184x48`, `fixed184x49`, `fixed184x50`, `fixed184x51`, `fixed184x52`, `fixed184x53`, `fixed184x54`, `fixed184x55`, `fixed184x56`, `fixed184x57`, `fixed184x58`, `fixed184x59`, `fixed184x60`, `fixed184x61`, `fixed184x62`, `fixed184x63`, `fixed184x64`, `fixed184x65`, `fixed184x66`, `fixed184x67`, `fixed184x68`, `fixed184x69`, `fixed184x70`, `fixed184x71`, `fixed184x72`, `fixed192x0`, `fixed192x1`, `fixed192x2`, `fixed192x3`, `fixed192x4`, `fixed192x5`, `fixed192x6`, `fixed192x7`, `fixed192x8`, `fixed192x9`, `fixed192x10`, `fixed192x11`, `fixed192x12`, `fixed192x13`, `fixed192x14`, `fixed192x15`, `fixed192x16`, `fixed192x17`, `fixed192x18`, `fixed192x19`, `fixed192x20`, `fixed192x21`, `fixed192x22`, `fixed192x23`, `fixed192x24`, `fixed192x25`, `fixed192x26`, `fixed192x27`, `fixed192x28`, `fixed192x29`, `fixed192x30`, `fixed192x31`, `fixed192x32`, `fixed192x33`, `fixed192x34`, `fixed192x35`, `fixed192x36`, `fixed192x37`, `fixed192x38`, `fixed192x39`, `fixed192x40`, `fixed192x41`, `fixed192x42`, `fixed192x43`, `fixed192x44`, `fixed192x45`, `fixed192x46`, `fixed192x47`, `fixed192x48`, `fixed192x49`, `fixed192x50`, `fixed192x51`, `fixed192x52`, `fixed192x53`, `fixed192x54`, `fixed192x55`, `fixed192x56`, `fixed192x57`, `fixed192x58`, `fixed192x59`, `fixed192x60`, `fixed192x61`, `fixed192x62`, `fixed192x63`, `fixed192x64`, `fixed200x0`, `fixed200x1`, `fixed200x2`, `fixed200x3`, `fixed200x4`, `fixed200x5`, `fixed200x6`, `fixed200x7`, `fixed200x8`, `fixed200x9`, `fixed200x10`, `fixed200x11`, `fixed200x12`, `fixed200x13`, `fixed200x14`, `fixed200x15`, `fixed200x16`, `fixed200x17`, `fixed200x18`, `fixed200x19`, `fixed200x20`, `fixed200x21`, `fixed200x22`, `fixed200x23`, `fixed200x24`, `fixed200x25`, `fixed200x26`, `fixed200x27`, `fixed200x28`, `fixed200x29`, `fixed200x30`, `fixed200x31`, `fixed200x32`, `fixed200x33`, `fixed200x34`, `fixed200x35`, `fixed200x36`, `fixed200x37`, `fixed200x38`, `fixed200x39`, `fixed200x40`, `fixed200x41`, `fixed200x42`, `fixed200x43`, `fixed200x44`, `fixed200x45`, `fixed200x46`, `fixed200x47`, `fixed200x48`, `fixed200x49`, `fixed200x50`, `fixed200x51`, `fixed200x52`, `fixed200x53`, `fixed200x54`, `fixed200x55`, `fixed200x56`, `fixed208x0`, `fixed208x1`, `fixed208x2`, `fixed208x3`, `fixed208x4`, `fixed208x5`, `fixed208x6`, `fixed208x7`, `fixed208x8`, `fixed208x9`, `fixed208x10`, `fixed208x11`, `fixed208x12`, `fixed208x13`, `fixed208x14`, `fixed208x15`, `fixed208x16`, `fixed208x17`, `fixed208x18`, `fixed208x19`, `fixed208x20`, `fixed208x21`, `fixed208x22`, `fixed208x23`, `fixed208x24`, `fixed208x25`, `fixed208x26`, `fixed208x27`, `fixed208x28`, `fixed208x29`, `fixed208x30`, `fixed208x31`, `fixed208x32`, `fixed208x33`, `fixed208x34`, `fixed208x35`, `fixed208x36`, `fixed208x37`, `fixed208x38`, `fixed208x39`, `fixed208x40`, `fixed208x41`, `fixed208x42`, `fixed208x43`, `fixed208x44`, `fixed208x45`, `fixed208x46`, `fixed208x47`, `fixed208x48`, `fixed216x0`, `fixed216x1`, `fixed216x2`, `fixed216x3`, `fixed216x4`, `fixed216x5`, `fixed216x6`, `fixed216x7`, `fixed216x8`, `fixed216x9`, `fixed216x10`, `fixed216x11`, `fixed216x12`, `fixed216x13`, `fixed216x14`, `fixed216x15`, `fixed216x16`, `fixed216x17`, `fixed216x18`, `fixed216x19`, `fixed216x20`, `fixed216x21`, `fixed216x22`, `fixed216x23`, `fixed216x24`, `fixed216x25`, `fixed216x26`, `fixed216x27`, `fixed216x28`, `fixed216x29`, `fixed216x30`, `fixed216x31`, `fixed216x32`, `fixed216x33`, `fixed216x34`, `fixed216x35`, `fixed216x36`, `fixed216x37`, `fixed216x38`, `fixed216x39`, `fixed216x40`, `fixed224x0`, `fixed224x1`, `fixed224x2`, `fixed224x3`, `fixed224x4`, `fixed224x5`, `fixed224x6`, `fixed224x7`, `fixed224x8`, `fixed224x9`, `fixed224x10`, `fixed224x11`, `fixed224x12`, `fixed224x13`, `fixed224x14`, `fixed224x15`, `fixed224x16`, `fixed224x17`, `fixed224x18`, `fixed224x19`, `fixed224x20`, `fixed224x21`, `fixed224x22`, `fixed224x23`, `fixed224x24`, `fixed224x25`, `fixed224x26`, `fixed224x27`, `fixed224x28`, `fixed224x29`, `fixed224x30`, `fixed224x31`, `fixed224x32`, `fixed232x0`, `fixed232x1`, `fixed232x2`, `fixed232x3`, `fixed232x4`, `fixed232x5`, `fixed232x6`, `fixed232x7`, `fixed232x8`, `fixed232x9`, `fixed232x10`, `fixed232x11`, `fixed232x12`, `fixed232x13`, `fixed232x14`, `fixed232x15`, `fixed232x16`, `fixed232x17`, `fixed232x18`, `fixed232x19`, `fixed232x20`, `fixed232x21`, `fixed232x22`, `fixed232x23`, `fixed232x24`, `fixed240x0`, `fixed240x1`, `fixed240x2`, `fixed240x3`, `fixed240x4`, `fixed240x5`, `fixed240x6`, `fixed240x7`, `fixed240x8`, `fixed240x9`, `fixed240x10`, `fixed240x11`, `fixed240x12`, `fixed240x13`, `fixed240x14`, `fixed240x15`, `fixed240x16`, `fixed248x0`, `fixed248x1`, `fixed248x2`, `fixed248x3`, `fixed248x4`, `fixed248x5`, `fixed248x6`, `fixed248x7`, `fixed248x8`, `fixed256x0`), KeywordType, nil},
- {Words(``, `\b`, `ufixed8x0`, `ufixed8x1`, `ufixed8x2`, `ufixed8x3`, `ufixed8x4`, `ufixed8x5`, `ufixed8x6`, `ufixed8x7`, `ufixed8x8`, `ufixed8x9`, `ufixed8x10`, `ufixed8x11`, `ufixed8x12`, `ufixed8x13`, `ufixed8x14`, `ufixed8x15`, `ufixed8x16`, `ufixed8x17`, `ufixed8x18`, `ufixed8x19`, `ufixed8x20`, `ufixed8x21`, `ufixed8x22`, `ufixed8x23`, `ufixed8x24`, `ufixed8x25`, `ufixed8x26`, `ufixed8x27`, `ufixed8x28`, `ufixed8x29`, `ufixed8x30`, `ufixed8x31`, `ufixed8x32`, `ufixed8x33`, `ufixed8x34`, `ufixed8x35`, `ufixed8x36`, `ufixed8x37`, `ufixed8x38`, `ufixed8x39`, `ufixed8x40`, `ufixed8x41`, `ufixed8x42`, `ufixed8x43`, `ufixed8x44`, `ufixed8x45`, `ufixed8x46`, `ufixed8x47`, `ufixed8x48`, `ufixed8x49`, `ufixed8x50`, `ufixed8x51`, `ufixed8x52`, `ufixed8x53`, `ufixed8x54`, `ufixed8x55`, `ufixed8x56`, `ufixed8x57`, `ufixed8x58`, `ufixed8x59`, `ufixed8x60`, `ufixed8x61`, `ufixed8x62`, `ufixed8x63`, `ufixed8x64`, `ufixed8x65`, `ufixed8x66`, `ufixed8x67`, `ufixed8x68`, `ufixed8x69`, `ufixed8x70`, `ufixed8x71`, `ufixed8x72`, `ufixed8x73`, `ufixed8x74`, `ufixed8x75`, `ufixed8x76`, `ufixed8x77`, `ufixed8x78`, `ufixed8x79`, `ufixed8x80`, `ufixed16x0`, `ufixed16x1`, `ufixed16x2`, `ufixed16x3`, `ufixed16x4`, `ufixed16x5`, `ufixed16x6`, `ufixed16x7`, `ufixed16x8`, `ufixed16x9`, `ufixed16x10`, `ufixed16x11`, `ufixed16x12`, `ufixed16x13`, `ufixed16x14`, `ufixed16x15`, `ufixed16x16`, `ufixed16x17`, `ufixed16x18`, `ufixed16x19`, `ufixed16x20`, `ufixed16x21`, `ufixed16x22`, `ufixed16x23`, `ufixed16x24`, `ufixed16x25`, `ufixed16x26`, `ufixed16x27`, `ufixed16x28`, `ufixed16x29`, `ufixed16x30`, `ufixed16x31`, `ufixed16x32`, `ufixed16x33`, `ufixed16x34`, `ufixed16x35`, `ufixed16x36`, `ufixed16x37`, `ufixed16x38`, `ufixed16x39`, `ufixed16x40`, `ufixed16x41`, `ufixed16x42`, `ufixed16x43`, `ufixed16x44`, `ufixed16x45`, `ufixed16x46`, `ufixed16x47`, `ufixed16x48`, `ufixed16x49`, `ufixed16x50`, `ufixed16x51`, `ufixed16x52`, `ufixed16x53`, `ufixed16x54`, `ufixed16x55`, `ufixed16x56`, `ufixed16x57`, `ufixed16x58`, `ufixed16x59`, `ufixed16x60`, `ufixed16x61`, `ufixed16x62`, `ufixed16x63`, `ufixed16x64`, `ufixed16x65`, `ufixed16x66`, `ufixed16x67`, `ufixed16x68`, `ufixed16x69`, `ufixed16x70`, `ufixed16x71`, `ufixed16x72`, `ufixed16x73`, `ufixed16x74`, `ufixed16x75`, `ufixed16x76`, `ufixed16x77`, `ufixed16x78`, `ufixed16x79`, `ufixed16x80`, `ufixed24x0`, `ufixed24x1`, `ufixed24x2`, `ufixed24x3`, `ufixed24x4`, `ufixed24x5`, `ufixed24x6`, `ufixed24x7`, `ufixed24x8`, `ufixed24x9`, `ufixed24x10`, `ufixed24x11`, `ufixed24x12`, `ufixed24x13`, `ufixed24x14`, `ufixed24x15`, `ufixed24x16`, `ufixed24x17`, `ufixed24x18`, `ufixed24x19`, `ufixed24x20`, `ufixed24x21`, `ufixed24x22`, `ufixed24x23`, `ufixed24x24`, `ufixed24x25`, `ufixed24x26`, `ufixed24x27`, `ufixed24x28`, `ufixed24x29`, `ufixed24x30`, `ufixed24x31`, `ufixed24x32`, `ufixed24x33`, `ufixed24x34`, `ufixed24x35`, `ufixed24x36`, `ufixed24x37`, `ufixed24x38`, `ufixed24x39`, `ufixed24x40`, `ufixed24x41`, `ufixed24x42`, `ufixed24x43`, `ufixed24x44`, `ufixed24x45`, `ufixed24x46`, `ufixed24x47`, `ufixed24x48`, `ufixed24x49`, `ufixed24x50`, `ufixed24x51`, `ufixed24x52`, `ufixed24x53`, `ufixed24x54`, `ufixed24x55`, `ufixed24x56`, `ufixed24x57`, `ufixed24x58`, `ufixed24x59`, `ufixed24x60`, `ufixed24x61`, `ufixed24x62`, `ufixed24x63`, `ufixed24x64`, `ufixed24x65`, `ufixed24x66`, `ufixed24x67`, `ufixed24x68`, `ufixed24x69`, `ufixed24x70`, `ufixed24x71`, `ufixed24x72`, `ufixed24x73`, `ufixed24x74`, `ufixed24x75`, `ufixed24x76`, `ufixed24x77`, `ufixed24x78`, `ufixed24x79`, `ufixed24x80`, `ufixed32x0`, `ufixed32x1`, `ufixed32x2`, `ufixed32x3`, `ufixed32x4`, `ufixed32x5`, `ufixed32x6`, `ufixed32x7`, `ufixed32x8`, `ufixed32x9`, `ufixed32x10`, `ufixed32x11`, `ufixed32x12`, `ufixed32x13`, `ufixed32x14`, `ufixed32x15`, `ufixed32x16`, `ufixed32x17`, `ufixed32x18`, `ufixed32x19`, `ufixed32x20`, `ufixed32x21`, `ufixed32x22`, `ufixed32x23`, `ufixed32x24`, `ufixed32x25`, `ufixed32x26`, `ufixed32x27`, `ufixed32x28`, `ufixed32x29`, `ufixed32x30`, `ufixed32x31`, `ufixed32x32`, `ufixed32x33`, `ufixed32x34`, `ufixed32x35`, `ufixed32x36`, `ufixed32x37`, `ufixed32x38`, `ufixed32x39`, `ufixed32x40`, `ufixed32x41`, `ufixed32x42`, `ufixed32x43`, `ufixed32x44`, `ufixed32x45`, `ufixed32x46`, `ufixed32x47`, `ufixed32x48`, `ufixed32x49`, `ufixed32x50`, `ufixed32x51`, `ufixed32x52`, `ufixed32x53`, `ufixed32x54`, `ufixed32x55`, `ufixed32x56`, `ufixed32x57`, `ufixed32x58`, `ufixed32x59`, `ufixed32x60`, `ufixed32x61`, `ufixed32x62`, `ufixed32x63`, `ufixed32x64`, `ufixed32x65`, `ufixed32x66`, `ufixed32x67`, `ufixed32x68`, `ufixed32x69`, `ufixed32x70`, `ufixed32x71`, `ufixed32x72`, `ufixed32x73`, `ufixed32x74`, `ufixed32x75`, `ufixed32x76`, `ufixed32x77`, `ufixed32x78`, `ufixed32x79`, `ufixed32x80`, `ufixed40x0`, `ufixed40x1`, `ufixed40x2`, `ufixed40x3`, `ufixed40x4`, `ufixed40x5`, `ufixed40x6`, `ufixed40x7`, `ufixed40x8`, `ufixed40x9`, `ufixed40x10`, `ufixed40x11`, `ufixed40x12`, `ufixed40x13`, `ufixed40x14`, `ufixed40x15`, `ufixed40x16`, `ufixed40x17`, `ufixed40x18`, `ufixed40x19`, `ufixed40x20`, `ufixed40x21`, `ufixed40x22`, `ufixed40x23`, `ufixed40x24`, `ufixed40x25`, `ufixed40x26`, `ufixed40x27`, `ufixed40x28`, `ufixed40x29`, `ufixed40x30`, `ufixed40x31`, `ufixed40x32`, `ufixed40x33`, `ufixed40x34`, `ufixed40x35`, `ufixed40x36`, `ufixed40x37`, `ufixed40x38`, `ufixed40x39`, `ufixed40x40`, `ufixed40x41`, `ufixed40x42`, `ufixed40x43`, `ufixed40x44`, `ufixed40x45`, `ufixed40x46`, `ufixed40x47`, `ufixed40x48`, `ufixed40x49`, `ufixed40x50`, `ufixed40x51`, `ufixed40x52`, `ufixed40x53`, `ufixed40x54`, `ufixed40x55`, `ufixed40x56`, `ufixed40x57`, `ufixed40x58`, `ufixed40x59`, `ufixed40x60`, `ufixed40x61`, `ufixed40x62`, `ufixed40x63`, `ufixed40x64`, `ufixed40x65`, `ufixed40x66`, `ufixed40x67`, `ufixed40x68`, `ufixed40x69`, `ufixed40x70`, `ufixed40x71`, `ufixed40x72`, `ufixed40x73`, `ufixed40x74`, `ufixed40x75`, `ufixed40x76`, `ufixed40x77`, `ufixed40x78`, `ufixed40x79`, `ufixed40x80`, `ufixed48x0`, `ufixed48x1`, `ufixed48x2`, `ufixed48x3`, `ufixed48x4`, `ufixed48x5`, `ufixed48x6`, `ufixed48x7`, `ufixed48x8`, `ufixed48x9`, `ufixed48x10`, `ufixed48x11`, `ufixed48x12`, `ufixed48x13`, `ufixed48x14`, `ufixed48x15`, `ufixed48x16`, `ufixed48x17`, `ufixed48x18`, `ufixed48x19`, `ufixed48x20`, `ufixed48x21`, `ufixed48x22`, `ufixed48x23`, `ufixed48x24`, `ufixed48x25`, `ufixed48x26`, `ufixed48x27`, `ufixed48x28`, `ufixed48x29`, `ufixed48x30`, `ufixed48x31`, `ufixed48x32`, `ufixed48x33`, `ufixed48x34`, `ufixed48x35`, `ufixed48x36`, `ufixed48x37`, `ufixed48x38`, `ufixed48x39`, `ufixed48x40`, `ufixed48x41`, `ufixed48x42`, `ufixed48x43`, `ufixed48x44`, `ufixed48x45`, `ufixed48x46`, `ufixed48x47`, `ufixed48x48`, `ufixed48x49`, `ufixed48x50`, `ufixed48x51`, `ufixed48x52`, `ufixed48x53`, `ufixed48x54`, `ufixed48x55`, `ufixed48x56`, `ufixed48x57`, `ufixed48x58`, `ufixed48x59`, `ufixed48x60`, `ufixed48x61`, `ufixed48x62`, `ufixed48x63`, `ufixed48x64`, `ufixed48x65`, `ufixed48x66`, `ufixed48x67`, `ufixed48x68`, `ufixed48x69`, `ufixed48x70`, `ufixed48x71`, `ufixed48x72`, `ufixed48x73`, `ufixed48x74`, `ufixed48x75`, `ufixed48x76`, `ufixed48x77`, `ufixed48x78`, `ufixed48x79`, `ufixed48x80`, `ufixed56x0`, `ufixed56x1`, `ufixed56x2`, `ufixed56x3`, `ufixed56x4`, `ufixed56x5`, `ufixed56x6`, `ufixed56x7`, `ufixed56x8`, `ufixed56x9`, `ufixed56x10`, `ufixed56x11`, `ufixed56x12`, `ufixed56x13`, `ufixed56x14`, `ufixed56x15`, `ufixed56x16`, `ufixed56x17`, `ufixed56x18`, `ufixed56x19`, `ufixed56x20`, `ufixed56x21`, `ufixed56x22`, `ufixed56x23`, `ufixed56x24`, `ufixed56x25`, `ufixed56x26`, `ufixed56x27`, `ufixed56x28`, `ufixed56x29`, `ufixed56x30`, `ufixed56x31`, `ufixed56x32`, `ufixed56x33`, `ufixed56x34`, `ufixed56x35`, `ufixed56x36`, `ufixed56x37`, `ufixed56x38`, `ufixed56x39`, `ufixed56x40`, `ufixed56x41`, `ufixed56x42`, `ufixed56x43`, `ufixed56x44`, `ufixed56x45`, `ufixed56x46`, `ufixed56x47`, `ufixed56x48`, `ufixed56x49`, `ufixed56x50`, `ufixed56x51`, `ufixed56x52`, `ufixed56x53`, `ufixed56x54`, `ufixed56x55`, `ufixed56x56`, `ufixed56x57`, `ufixed56x58`, `ufixed56x59`, `ufixed56x60`, `ufixed56x61`, `ufixed56x62`, `ufixed56x63`, `ufixed56x64`, `ufixed56x65`, `ufixed56x66`, `ufixed56x67`, `ufixed56x68`, `ufixed56x69`, `ufixed56x70`, `ufixed56x71`, `ufixed56x72`, `ufixed56x73`, `ufixed56x74`, `ufixed56x75`, `ufixed56x76`, `ufixed56x77`, `ufixed56x78`, `ufixed56x79`, `ufixed56x80`, `ufixed64x0`, `ufixed64x1`, `ufixed64x2`, `ufixed64x3`, `ufixed64x4`, `ufixed64x5`, `ufixed64x6`, `ufixed64x7`, `ufixed64x8`, `ufixed64x9`, `ufixed64x10`, `ufixed64x11`, `ufixed64x12`, `ufixed64x13`, `ufixed64x14`, `ufixed64x15`, `ufixed64x16`, `ufixed64x17`, `ufixed64x18`, `ufixed64x19`, `ufixed64x20`, `ufixed64x21`, `ufixed64x22`, `ufixed64x23`, `ufixed64x24`, `ufixed64x25`, `ufixed64x26`, `ufixed64x27`, `ufixed64x28`, `ufixed64x29`, `ufixed64x30`, `ufixed64x31`, `ufixed64x32`, `ufixed64x33`, `ufixed64x34`, `ufixed64x35`, `ufixed64x36`, `ufixed64x37`, `ufixed64x38`, `ufixed64x39`, `ufixed64x40`, `ufixed64x41`, `ufixed64x42`, `ufixed64x43`, `ufixed64x44`, `ufixed64x45`, `ufixed64x46`, `ufixed64x47`, `ufixed64x48`, `ufixed64x49`, `ufixed64x50`, `ufixed64x51`, `ufixed64x52`, `ufixed64x53`, `ufixed64x54`, `ufixed64x55`, `ufixed64x56`, `ufixed64x57`, `ufixed64x58`, `ufixed64x59`, `ufixed64x60`, `ufixed64x61`, `ufixed64x62`, `ufixed64x63`, `ufixed64x64`, `ufixed64x65`, `ufixed64x66`, `ufixed64x67`, `ufixed64x68`, `ufixed64x69`, `ufixed64x70`, `ufixed64x71`, `ufixed64x72`, `ufixed64x73`, `ufixed64x74`, `ufixed64x75`, `ufixed64x76`, `ufixed64x77`, `ufixed64x78`, `ufixed64x79`, `ufixed64x80`, `ufixed72x0`, `ufixed72x1`, `ufixed72x2`, `ufixed72x3`, `ufixed72x4`, `ufixed72x5`, `ufixed72x6`, `ufixed72x7`, `ufixed72x8`, `ufixed72x9`, `ufixed72x10`, `ufixed72x11`, `ufixed72x12`, `ufixed72x13`, `ufixed72x14`, `ufixed72x15`, `ufixed72x16`, `ufixed72x17`, `ufixed72x18`, `ufixed72x19`, `ufixed72x20`, `ufixed72x21`, `ufixed72x22`, `ufixed72x23`, `ufixed72x24`, `ufixed72x25`, `ufixed72x26`, `ufixed72x27`, `ufixed72x28`, `ufixed72x29`, `ufixed72x30`, `ufixed72x31`, `ufixed72x32`, `ufixed72x33`, `ufixed72x34`, `ufixed72x35`, `ufixed72x36`, `ufixed72x37`, `ufixed72x38`, `ufixed72x39`, `ufixed72x40`, `ufixed72x41`, `ufixed72x42`, `ufixed72x43`, `ufixed72x44`, `ufixed72x45`, `ufixed72x46`, `ufixed72x47`, `ufixed72x48`, `ufixed72x49`, `ufixed72x50`, `ufixed72x51`, `ufixed72x52`, `ufixed72x53`, `ufixed72x54`, `ufixed72x55`, `ufixed72x56`, `ufixed72x57`, `ufixed72x58`, `ufixed72x59`, `ufixed72x60`, `ufixed72x61`, `ufixed72x62`, `ufixed72x63`, `ufixed72x64`, `ufixed72x65`, `ufixed72x66`, `ufixed72x67`, `ufixed72x68`, `ufixed72x69`, `ufixed72x70`, `ufixed72x71`, `ufixed72x72`, `ufixed72x73`, `ufixed72x74`, `ufixed72x75`, `ufixed72x76`, `ufixed72x77`, `ufixed72x78`, `ufixed72x79`, `ufixed72x80`, `ufixed80x0`, `ufixed80x1`, `ufixed80x2`, `ufixed80x3`, `ufixed80x4`, `ufixed80x5`, `ufixed80x6`, `ufixed80x7`, `ufixed80x8`, `ufixed80x9`, `ufixed80x10`, `ufixed80x11`, `ufixed80x12`, `ufixed80x13`, `ufixed80x14`, `ufixed80x15`, `ufixed80x16`, `ufixed80x17`, `ufixed80x18`, `ufixed80x19`, `ufixed80x20`, `ufixed80x21`, `ufixed80x22`, `ufixed80x23`, `ufixed80x24`, `ufixed80x25`, `ufixed80x26`, `ufixed80x27`, `ufixed80x28`, `ufixed80x29`, `ufixed80x30`, `ufixed80x31`, `ufixed80x32`, `ufixed80x33`, `ufixed80x34`, `ufixed80x35`, `ufixed80x36`, `ufixed80x37`, `ufixed80x38`, `ufixed80x39`, `ufixed80x40`, `ufixed80x41`, `ufixed80x42`, `ufixed80x43`, `ufixed80x44`, `ufixed80x45`, `ufixed80x46`, `ufixed80x47`, `ufixed80x48`, `ufixed80x49`, `ufixed80x50`, `ufixed80x51`, `ufixed80x52`, `ufixed80x53`, `ufixed80x54`, `ufixed80x55`, `ufixed80x56`, `ufixed80x57`, `ufixed80x58`, `ufixed80x59`, `ufixed80x60`, `ufixed80x61`, `ufixed80x62`, `ufixed80x63`, `ufixed80x64`, `ufixed80x65`, `ufixed80x66`, `ufixed80x67`, `ufixed80x68`, `ufixed80x69`, `ufixed80x70`, `ufixed80x71`, `ufixed80x72`, `ufixed80x73`, `ufixed80x74`, `ufixed80x75`, `ufixed80x76`, `ufixed80x77`, `ufixed80x78`, `ufixed80x79`, `ufixed80x80`, `ufixed88x0`, `ufixed88x1`, `ufixed88x2`, `ufixed88x3`, `ufixed88x4`, `ufixed88x5`, `ufixed88x6`, `ufixed88x7`, `ufixed88x8`, `ufixed88x9`, `ufixed88x10`, `ufixed88x11`, `ufixed88x12`, `ufixed88x13`, `ufixed88x14`, `ufixed88x15`, `ufixed88x16`, `ufixed88x17`, `ufixed88x18`, `ufixed88x19`, `ufixed88x20`, `ufixed88x21`, `ufixed88x22`, `ufixed88x23`, `ufixed88x24`, `ufixed88x25`, `ufixed88x26`, `ufixed88x27`, `ufixed88x28`, `ufixed88x29`, `ufixed88x30`, `ufixed88x31`, `ufixed88x32`, `ufixed88x33`, `ufixed88x34`, `ufixed88x35`, `ufixed88x36`, `ufixed88x37`, `ufixed88x38`, `ufixed88x39`, `ufixed88x40`, `ufixed88x41`, `ufixed88x42`, `ufixed88x43`, `ufixed88x44`, `ufixed88x45`, `ufixed88x46`, `ufixed88x47`, `ufixed88x48`, `ufixed88x49`, `ufixed88x50`, `ufixed88x51`, `ufixed88x52`, `ufixed88x53`, `ufixed88x54`, `ufixed88x55`, `ufixed88x56`, `ufixed88x57`, `ufixed88x58`, `ufixed88x59`, `ufixed88x60`, `ufixed88x61`, `ufixed88x62`, `ufixed88x63`, `ufixed88x64`, `ufixed88x65`, `ufixed88x66`, `ufixed88x67`, `ufixed88x68`, `ufixed88x69`, `ufixed88x70`, `ufixed88x71`, `ufixed88x72`, `ufixed88x73`, `ufixed88x74`, `ufixed88x75`, `ufixed88x76`, `ufixed88x77`, `ufixed88x78`, `ufixed88x79`, `ufixed88x80`, `ufixed96x0`, `ufixed96x1`, `ufixed96x2`, `ufixed96x3`, `ufixed96x4`, `ufixed96x5`, `ufixed96x6`, `ufixed96x7`, `ufixed96x8`, `ufixed96x9`, `ufixed96x10`, `ufixed96x11`, `ufixed96x12`, `ufixed96x13`, `ufixed96x14`, `ufixed96x15`, `ufixed96x16`, `ufixed96x17`, `ufixed96x18`, `ufixed96x19`, `ufixed96x20`, `ufixed96x21`, `ufixed96x22`, `ufixed96x23`, `ufixed96x24`, `ufixed96x25`, `ufixed96x26`, `ufixed96x27`, `ufixed96x28`, `ufixed96x29`, `ufixed96x30`, `ufixed96x31`, `ufixed96x32`, `ufixed96x33`, `ufixed96x34`, `ufixed96x35`, `ufixed96x36`, `ufixed96x37`, `ufixed96x38`, `ufixed96x39`, `ufixed96x40`, `ufixed96x41`, `ufixed96x42`, `ufixed96x43`, `ufixed96x44`, `ufixed96x45`, `ufixed96x46`, `ufixed96x47`, `ufixed96x48`, `ufixed96x49`, `ufixed96x50`, `ufixed96x51`, `ufixed96x52`, `ufixed96x53`, `ufixed96x54`, `ufixed96x55`, `ufixed96x56`, `ufixed96x57`, `ufixed96x58`, `ufixed96x59`, `ufixed96x60`, `ufixed96x61`, `ufixed96x62`, `ufixed96x63`, `ufixed96x64`, `ufixed96x65`, `ufixed96x66`, `ufixed96x67`, `ufixed96x68`, `ufixed96x69`, `ufixed96x70`, `ufixed96x71`, `ufixed96x72`, `ufixed96x73`, `ufixed96x74`, `ufixed96x75`, `ufixed96x76`, `ufixed96x77`, `ufixed96x78`, `ufixed96x79`, `ufixed96x80`, `ufixed104x0`, `ufixed104x1`, `ufixed104x2`, `ufixed104x3`, `ufixed104x4`, `ufixed104x5`, `ufixed104x6`, `ufixed104x7`, `ufixed104x8`, `ufixed104x9`, `ufixed104x10`, `ufixed104x11`, `ufixed104x12`, `ufixed104x13`, `ufixed104x14`, `ufixed104x15`, `ufixed104x16`, `ufixed104x17`, `ufixed104x18`, `ufixed104x19`, `ufixed104x20`, `ufixed104x21`, `ufixed104x22`, `ufixed104x23`, `ufixed104x24`, `ufixed104x25`, `ufixed104x26`, `ufixed104x27`, `ufixed104x28`, `ufixed104x29`, `ufixed104x30`, `ufixed104x31`, `ufixed104x32`, `ufixed104x33`, `ufixed104x34`, `ufixed104x35`, `ufixed104x36`, `ufixed104x37`, `ufixed104x38`, `ufixed104x39`, `ufixed104x40`, `ufixed104x41`, `ufixed104x42`, `ufixed104x43`, `ufixed104x44`, `ufixed104x45`, `ufixed104x46`, `ufixed104x47`, `ufixed104x48`, `ufixed104x49`, `ufixed104x50`, `ufixed104x51`, `ufixed104x52`, `ufixed104x53`, `ufixed104x54`, `ufixed104x55`, `ufixed104x56`, `ufixed104x57`, `ufixed104x58`, `ufixed104x59`, `ufixed104x60`, `ufixed104x61`, `ufixed104x62`, `ufixed104x63`, `ufixed104x64`, `ufixed104x65`, `ufixed104x66`, `ufixed104x67`, `ufixed104x68`, `ufixed104x69`, `ufixed104x70`, `ufixed104x71`, `ufixed104x72`, `ufixed104x73`, `ufixed104x74`, `ufixed104x75`, `ufixed104x76`, `ufixed104x77`, `ufixed104x78`, `ufixed104x79`, `ufixed104x80`, `ufixed112x0`, `ufixed112x1`, `ufixed112x2`, `ufixed112x3`, `ufixed112x4`, `ufixed112x5`, `ufixed112x6`, `ufixed112x7`, `ufixed112x8`, `ufixed112x9`, `ufixed112x10`, `ufixed112x11`, `ufixed112x12`, `ufixed112x13`, `ufixed112x14`, `ufixed112x15`, `ufixed112x16`, `ufixed112x17`, `ufixed112x18`, `ufixed112x19`, `ufixed112x20`, `ufixed112x21`, `ufixed112x22`, `ufixed112x23`, `ufixed112x24`, `ufixed112x25`, `ufixed112x26`, `ufixed112x27`, `ufixed112x28`, `ufixed112x29`, `ufixed112x30`, `ufixed112x31`, `ufixed112x32`, `ufixed112x33`, `ufixed112x34`, `ufixed112x35`, `ufixed112x36`, `ufixed112x37`, `ufixed112x38`, `ufixed112x39`, `ufixed112x40`, `ufixed112x41`, `ufixed112x42`, `ufixed112x43`, `ufixed112x44`, `ufixed112x45`, `ufixed112x46`, `ufixed112x47`, `ufixed112x48`, `ufixed112x49`, `ufixed112x50`, `ufixed112x51`, `ufixed112x52`, `ufixed112x53`, `ufixed112x54`, `ufixed112x55`, `ufixed112x56`, `ufixed112x57`, `ufixed112x58`, `ufixed112x59`, `ufixed112x60`, `ufixed112x61`, `ufixed112x62`, `ufixed112x63`, `ufixed112x64`, `ufixed112x65`, `ufixed112x66`, `ufixed112x67`, `ufixed112x68`, `ufixed112x69`, `ufixed112x70`, `ufixed112x71`, `ufixed112x72`, `ufixed112x73`, `ufixed112x74`, `ufixed112x75`, `ufixed112x76`, `ufixed112x77`, `ufixed112x78`, `ufixed112x79`, `ufixed112x80`, `ufixed120x0`, `ufixed120x1`, `ufixed120x2`, `ufixed120x3`, `ufixed120x4`, `ufixed120x5`, `ufixed120x6`, `ufixed120x7`, `ufixed120x8`, `ufixed120x9`, `ufixed120x10`, `ufixed120x11`, `ufixed120x12`, `ufixed120x13`, `ufixed120x14`, `ufixed120x15`, `ufixed120x16`, `ufixed120x17`, `ufixed120x18`, `ufixed120x19`, `ufixed120x20`, `ufixed120x21`, `ufixed120x22`, `ufixed120x23`, `ufixed120x24`, `ufixed120x25`, `ufixed120x26`, `ufixed120x27`, `ufixed120x28`, `ufixed120x29`, `ufixed120x30`, `ufixed120x31`, `ufixed120x32`, `ufixed120x33`, `ufixed120x34`, `ufixed120x35`, `ufixed120x36`, `ufixed120x37`, `ufixed120x38`, `ufixed120x39`, `ufixed120x40`, `ufixed120x41`, `ufixed120x42`, `ufixed120x43`, `ufixed120x44`, `ufixed120x45`, `ufixed120x46`, `ufixed120x47`, `ufixed120x48`, `ufixed120x49`, `ufixed120x50`, `ufixed120x51`, `ufixed120x52`, `ufixed120x53`, `ufixed120x54`, `ufixed120x55`, `ufixed120x56`, `ufixed120x57`, `ufixed120x58`, `ufixed120x59`, `ufixed120x60`, `ufixed120x61`, `ufixed120x62`, `ufixed120x63`, `ufixed120x64`, `ufixed120x65`, `ufixed120x66`, `ufixed120x67`, `ufixed120x68`, `ufixed120x69`, `ufixed120x70`, `ufixed120x71`, `ufixed120x72`, `ufixed120x73`, `ufixed120x74`, `ufixed120x75`, `ufixed120x76`, `ufixed120x77`, `ufixed120x78`, `ufixed120x79`, `ufixed120x80`, `ufixed128x0`, `ufixed128x1`, `ufixed128x2`, `ufixed128x3`, `ufixed128x4`, `ufixed128x5`, `ufixed128x6`, `ufixed128x7`, `ufixed128x8`, `ufixed128x9`, `ufixed128x10`, `ufixed128x11`, `ufixed128x12`, `ufixed128x13`, `ufixed128x14`, `ufixed128x15`, `ufixed128x16`, `ufixed128x17`, `ufixed128x18`, `ufixed128x19`, `ufixed128x20`, `ufixed128x21`, `ufixed128x22`, `ufixed128x23`, `ufixed128x24`, `ufixed128x25`, `ufixed128x26`, `ufixed128x27`, `ufixed128x28`, `ufixed128x29`, `ufixed128x30`, `ufixed128x31`, `ufixed128x32`, `ufixed128x33`, `ufixed128x34`, `ufixed128x35`, `ufixed128x36`, `ufixed128x37`, `ufixed128x38`, `ufixed128x39`, `ufixed128x40`, `ufixed128x41`, `ufixed128x42`, `ufixed128x43`, `ufixed128x44`, `ufixed128x45`, `ufixed128x46`, `ufixed128x47`, `ufixed128x48`, `ufixed128x49`, `ufixed128x50`, `ufixed128x51`, `ufixed128x52`, `ufixed128x53`, `ufixed128x54`, `ufixed128x55`, `ufixed128x56`, `ufixed128x57`, `ufixed128x58`, `ufixed128x59`, `ufixed128x60`, `ufixed128x61`, `ufixed128x62`, `ufixed128x63`, `ufixed128x64`, `ufixed128x65`, `ufixed128x66`, `ufixed128x67`, `ufixed128x68`, `ufixed128x69`, `ufixed128x70`, `ufixed128x71`, `ufixed128x72`, `ufixed128x73`, `ufixed128x74`, `ufixed128x75`, `ufixed128x76`, `ufixed128x77`, `ufixed128x78`, `ufixed128x79`, `ufixed128x80`, `ufixed136x0`, `ufixed136x1`, `ufixed136x2`, `ufixed136x3`, `ufixed136x4`, `ufixed136x5`, `ufixed136x6`, `ufixed136x7`, `ufixed136x8`, `ufixed136x9`, `ufixed136x10`, `ufixed136x11`, `ufixed136x12`, `ufixed136x13`, `ufixed136x14`, `ufixed136x15`, `ufixed136x16`, `ufixed136x17`, `ufixed136x18`, `ufixed136x19`, `ufixed136x20`, `ufixed136x21`, `ufixed136x22`, `ufixed136x23`, `ufixed136x24`, `ufixed136x25`, `ufixed136x26`, `ufixed136x27`, `ufixed136x28`, `ufixed136x29`, `ufixed136x30`, `ufixed136x31`, `ufixed136x32`, `ufixed136x33`, `ufixed136x34`, `ufixed136x35`, `ufixed136x36`, `ufixed136x37`, `ufixed136x38`, `ufixed136x39`, `ufixed136x40`, `ufixed136x41`, `ufixed136x42`, `ufixed136x43`, `ufixed136x44`, `ufixed136x45`, `ufixed136x46`, `ufixed136x47`, `ufixed136x48`, `ufixed136x49`, `ufixed136x50`, `ufixed136x51`, `ufixed136x52`, `ufixed136x53`, `ufixed136x54`, `ufixed136x55`, `ufixed136x56`, `ufixed136x57`, `ufixed136x58`, `ufixed136x59`, `ufixed136x60`, `ufixed136x61`, `ufixed136x62`, `ufixed136x63`, `ufixed136x64`, `ufixed136x65`, `ufixed136x66`, `ufixed136x67`, `ufixed136x68`, `ufixed136x69`, `ufixed136x70`, `ufixed136x71`, `ufixed136x72`, `ufixed136x73`, `ufixed136x74`, `ufixed136x75`, `ufixed136x76`, `ufixed136x77`, `ufixed136x78`, `ufixed136x79`, `ufixed136x80`, `ufixed144x0`, `ufixed144x1`, `ufixed144x2`, `ufixed144x3`, `ufixed144x4`, `ufixed144x5`, `ufixed144x6`, `ufixed144x7`, `ufixed144x8`, `ufixed144x9`, `ufixed144x10`, `ufixed144x11`, `ufixed144x12`, `ufixed144x13`, `ufixed144x14`, `ufixed144x15`, `ufixed144x16`, `ufixed144x17`, `ufixed144x18`, `ufixed144x19`, `ufixed144x20`, `ufixed144x21`, `ufixed144x22`, `ufixed144x23`, `ufixed144x24`, `ufixed144x25`, `ufixed144x26`, `ufixed144x27`, `ufixed144x28`, `ufixed144x29`, `ufixed144x30`, `ufixed144x31`, `ufixed144x32`, `ufixed144x33`, `ufixed144x34`, `ufixed144x35`, `ufixed144x36`, `ufixed144x37`, `ufixed144x38`, `ufixed144x39`, `ufixed144x40`, `ufixed144x41`, `ufixed144x42`, `ufixed144x43`, `ufixed144x44`, `ufixed144x45`, `ufixed144x46`, `ufixed144x47`, `ufixed144x48`, `ufixed144x49`, `ufixed144x50`, `ufixed144x51`, `ufixed144x52`, `ufixed144x53`, `ufixed144x54`, `ufixed144x55`, `ufixed144x56`, `ufixed144x57`, `ufixed144x58`, `ufixed144x59`, `ufixed144x60`, `ufixed144x61`, `ufixed144x62`, `ufixed144x63`, `ufixed144x64`, `ufixed144x65`, `ufixed144x66`, `ufixed144x67`, `ufixed144x68`, `ufixed144x69`, `ufixed144x70`, `ufixed144x71`, `ufixed144x72`, `ufixed144x73`, `ufixed144x74`, `ufixed144x75`, `ufixed144x76`, `ufixed144x77`, `ufixed144x78`, `ufixed144x79`, `ufixed144x80`, `ufixed152x0`, `ufixed152x1`, `ufixed152x2`, `ufixed152x3`, `ufixed152x4`, `ufixed152x5`, `ufixed152x6`, `ufixed152x7`, `ufixed152x8`, `ufixed152x9`, `ufixed152x10`, `ufixed152x11`, `ufixed152x12`, `ufixed152x13`, `ufixed152x14`, `ufixed152x15`, `ufixed152x16`, `ufixed152x17`, `ufixed152x18`, `ufixed152x19`, `ufixed152x20`, `ufixed152x21`, `ufixed152x22`, `ufixed152x23`, `ufixed152x24`, `ufixed152x25`, `ufixed152x26`, `ufixed152x27`, `ufixed152x28`, `ufixed152x29`, `ufixed152x30`, `ufixed152x31`, `ufixed152x32`, `ufixed152x33`, `ufixed152x34`, `ufixed152x35`, `ufixed152x36`, `ufixed152x37`, `ufixed152x38`, `ufixed152x39`, `ufixed152x40`, `ufixed152x41`, `ufixed152x42`, `ufixed152x43`, `ufixed152x44`, `ufixed152x45`, `ufixed152x46`, `ufixed152x47`, `ufixed152x48`, `ufixed152x49`, `ufixed152x50`, `ufixed152x51`, `ufixed152x52`, `ufixed152x53`, `ufixed152x54`, `ufixed152x55`, `ufixed152x56`, `ufixed152x57`, `ufixed152x58`, `ufixed152x59`, `ufixed152x60`, `ufixed152x61`, `ufixed152x62`, `ufixed152x63`, `ufixed152x64`, `ufixed152x65`, `ufixed152x66`, `ufixed152x67`, `ufixed152x68`, `ufixed152x69`, `ufixed152x70`, `ufixed152x71`, `ufixed152x72`, `ufixed152x73`, `ufixed152x74`, `ufixed152x75`, `ufixed152x76`, `ufixed152x77`, `ufixed152x78`, `ufixed152x79`, `ufixed152x80`, `ufixed160x0`, `ufixed160x1`, `ufixed160x2`, `ufixed160x3`, `ufixed160x4`, `ufixed160x5`, `ufixed160x6`, `ufixed160x7`, `ufixed160x8`, `ufixed160x9`, `ufixed160x10`, `ufixed160x11`, `ufixed160x12`, `ufixed160x13`, `ufixed160x14`, `ufixed160x15`, `ufixed160x16`, `ufixed160x17`, `ufixed160x18`, `ufixed160x19`, `ufixed160x20`, `ufixed160x21`, `ufixed160x22`, `ufixed160x23`, `ufixed160x24`, `ufixed160x25`, `ufixed160x26`, `ufixed160x27`, `ufixed160x28`, `ufixed160x29`, `ufixed160x30`, `ufixed160x31`, `ufixed160x32`, `ufixed160x33`, `ufixed160x34`, `ufixed160x35`, `ufixed160x36`, `ufixed160x37`, `ufixed160x38`, `ufixed160x39`, `ufixed160x40`, `ufixed160x41`, `ufixed160x42`, `ufixed160x43`, `ufixed160x44`, `ufixed160x45`, `ufixed160x46`, `ufixed160x47`, `ufixed160x48`, `ufixed160x49`, `ufixed160x50`, `ufixed160x51`, `ufixed160x52`, `ufixed160x53`, `ufixed160x54`, `ufixed160x55`, `ufixed160x56`, `ufixed160x57`, `ufixed160x58`, `ufixed160x59`, `ufixed160x60`, `ufixed160x61`, `ufixed160x62`, `ufixed160x63`, `ufixed160x64`, `ufixed160x65`, `ufixed160x66`, `ufixed160x67`, `ufixed160x68`, `ufixed160x69`, `ufixed160x70`, `ufixed160x71`, `ufixed160x72`, `ufixed160x73`, `ufixed160x74`, `ufixed160x75`, `ufixed160x76`, `ufixed160x77`, `ufixed160x78`, `ufixed160x79`, `ufixed160x80`, `ufixed168x0`, `ufixed168x1`, `ufixed168x2`, `ufixed168x3`, `ufixed168x4`, `ufixed168x5`, `ufixed168x6`, `ufixed168x7`, `ufixed168x8`, `ufixed168x9`, `ufixed168x10`, `ufixed168x11`, `ufixed168x12`, `ufixed168x13`, `ufixed168x14`, `ufixed168x15`, `ufixed168x16`, `ufixed168x17`, `ufixed168x18`, `ufixed168x19`, `ufixed168x20`, `ufixed168x21`, `ufixed168x22`, `ufixed168x23`, `ufixed168x24`, `ufixed168x25`, `ufixed168x26`, `ufixed168x27`, `ufixed168x28`, `ufixed168x29`, `ufixed168x30`, `ufixed168x31`, `ufixed168x32`, `ufixed168x33`, `ufixed168x34`, `ufixed168x35`, `ufixed168x36`, `ufixed168x37`, `ufixed168x38`, `ufixed168x39`, `ufixed168x40`, `ufixed168x41`, `ufixed168x42`, `ufixed168x43`, `ufixed168x44`, `ufixed168x45`, `ufixed168x46`, `ufixed168x47`, `ufixed168x48`, `ufixed168x49`, `ufixed168x50`, `ufixed168x51`, `ufixed168x52`, `ufixed168x53`, `ufixed168x54`, `ufixed168x55`, `ufixed168x56`, `ufixed168x57`, `ufixed168x58`, `ufixed168x59`, `ufixed168x60`, `ufixed168x61`, `ufixed168x62`, `ufixed168x63`, `ufixed168x64`, `ufixed168x65`, `ufixed168x66`, `ufixed168x67`, `ufixed168x68`, `ufixed168x69`, `ufixed168x70`, `ufixed168x71`, `ufixed168x72`, `ufixed168x73`, `ufixed168x74`, `ufixed168x75`, `ufixed168x76`, `ufixed168x77`, `ufixed168x78`, `ufixed168x79`, `ufixed168x80`, `ufixed176x0`, `ufixed176x1`, `ufixed176x2`, `ufixed176x3`, `ufixed176x4`, `ufixed176x5`, `ufixed176x6`, `ufixed176x7`, `ufixed176x8`, `ufixed176x9`, `ufixed176x10`, `ufixed176x11`, `ufixed176x12`, `ufixed176x13`, `ufixed176x14`, `ufixed176x15`, `ufixed176x16`, `ufixed176x17`, `ufixed176x18`, `ufixed176x19`, `ufixed176x20`, `ufixed176x21`, `ufixed176x22`, `ufixed176x23`, `ufixed176x24`, `ufixed176x25`, `ufixed176x26`, `ufixed176x27`, `ufixed176x28`, `ufixed176x29`, `ufixed176x30`, `ufixed176x31`, `ufixed176x32`, `ufixed176x33`, `ufixed176x34`, `ufixed176x35`, `ufixed176x36`, `ufixed176x37`, `ufixed176x38`, `ufixed176x39`, `ufixed176x40`, `ufixed176x41`, `ufixed176x42`, `ufixed176x43`, `ufixed176x44`, `ufixed176x45`, `ufixed176x46`, `ufixed176x47`, `ufixed176x48`, `ufixed176x49`, `ufixed176x50`, `ufixed176x51`, `ufixed176x52`, `ufixed176x53`, `ufixed176x54`, `ufixed176x55`, `ufixed176x56`, `ufixed176x57`, `ufixed176x58`, `ufixed176x59`, `ufixed176x60`, `ufixed176x61`, `ufixed176x62`, `ufixed176x63`, `ufixed176x64`, `ufixed176x65`, `ufixed176x66`, `ufixed176x67`, `ufixed176x68`, `ufixed176x69`, `ufixed176x70`, `ufixed176x71`, `ufixed176x72`, `ufixed176x73`, `ufixed176x74`, `ufixed176x75`, `ufixed176x76`, `ufixed176x77`, `ufixed176x78`, `ufixed176x79`, `ufixed176x80`, `ufixed184x0`, `ufixed184x1`, `ufixed184x2`, `ufixed184x3`, `ufixed184x4`, `ufixed184x5`, `ufixed184x6`, `ufixed184x7`, `ufixed184x8`, `ufixed184x9`, `ufixed184x10`, `ufixed184x11`, `ufixed184x12`, `ufixed184x13`, `ufixed184x14`, `ufixed184x15`, `ufixed184x16`, `ufixed184x17`, `ufixed184x18`, `ufixed184x19`, `ufixed184x20`, `ufixed184x21`, `ufixed184x22`, `ufixed184x23`, `ufixed184x24`, `ufixed184x25`, `ufixed184x26`, `ufixed184x27`, `ufixed184x28`, `ufixed184x29`, `ufixed184x30`, `ufixed184x31`, `ufixed184x32`, `ufixed184x33`, `ufixed184x34`, `ufixed184x35`, `ufixed184x36`, `ufixed184x37`, `ufixed184x38`, `ufixed184x39`, `ufixed184x40`, `ufixed184x41`, `ufixed184x42`, `ufixed184x43`, `ufixed184x44`, `ufixed184x45`, `ufixed184x46`, `ufixed184x47`, `ufixed184x48`, `ufixed184x49`, `ufixed184x50`, `ufixed184x51`, `ufixed184x52`, `ufixed184x53`, `ufixed184x54`, `ufixed184x55`, `ufixed184x56`, `ufixed184x57`, `ufixed184x58`, `ufixed184x59`, `ufixed184x60`, `ufixed184x61`, `ufixed184x62`, `ufixed184x63`, `ufixed184x64`, `ufixed184x65`, `ufixed184x66`, `ufixed184x67`, `ufixed184x68`, `ufixed184x69`, `ufixed184x70`, `ufixed184x71`, `ufixed184x72`, `ufixed192x0`, `ufixed192x1`, `ufixed192x2`, `ufixed192x3`, `ufixed192x4`, `ufixed192x5`, `ufixed192x6`, `ufixed192x7`, `ufixed192x8`, `ufixed192x9`, `ufixed192x10`, `ufixed192x11`, `ufixed192x12`, `ufixed192x13`, `ufixed192x14`, `ufixed192x15`, `ufixed192x16`, `ufixed192x17`, `ufixed192x18`, `ufixed192x19`, `ufixed192x20`, `ufixed192x21`, `ufixed192x22`, `ufixed192x23`, `ufixed192x24`, `ufixed192x25`, `ufixed192x26`, `ufixed192x27`, `ufixed192x28`, `ufixed192x29`, `ufixed192x30`, `ufixed192x31`, `ufixed192x32`, `ufixed192x33`, `ufixed192x34`, `ufixed192x35`, `ufixed192x36`, `ufixed192x37`, `ufixed192x38`, `ufixed192x39`, `ufixed192x40`, `ufixed192x41`, `ufixed192x42`, `ufixed192x43`, `ufixed192x44`, `ufixed192x45`, `ufixed192x46`, `ufixed192x47`, `ufixed192x48`, `ufixed192x49`, `ufixed192x50`, `ufixed192x51`, `ufixed192x52`, `ufixed192x53`, `ufixed192x54`, `ufixed192x55`, `ufixed192x56`, `ufixed192x57`, `ufixed192x58`, `ufixed192x59`, `ufixed192x60`, `ufixed192x61`, `ufixed192x62`, `ufixed192x63`, `ufixed192x64`, `ufixed200x0`, `ufixed200x1`, `ufixed200x2`, `ufixed200x3`, `ufixed200x4`, `ufixed200x5`, `ufixed200x6`, `ufixed200x7`, `ufixed200x8`, `ufixed200x9`, `ufixed200x10`, `ufixed200x11`, `ufixed200x12`, `ufixed200x13`, `ufixed200x14`, `ufixed200x15`, `ufixed200x16`, `ufixed200x17`, `ufixed200x18`, `ufixed200x19`, `ufixed200x20`, `ufixed200x21`, `ufixed200x22`, `ufixed200x23`, `ufixed200x24`, `ufixed200x25`, `ufixed200x26`, `ufixed200x27`, `ufixed200x28`, `ufixed200x29`, `ufixed200x30`, `ufixed200x31`, `ufixed200x32`, `ufixed200x33`, `ufixed200x34`, `ufixed200x35`, `ufixed200x36`, `ufixed200x37`, `ufixed200x38`, `ufixed200x39`, `ufixed200x40`, `ufixed200x41`, `ufixed200x42`, `ufixed200x43`, `ufixed200x44`, `ufixed200x45`, `ufixed200x46`, `ufixed200x47`, `ufixed200x48`, `ufixed200x49`, `ufixed200x50`, `ufixed200x51`, `ufixed200x52`, `ufixed200x53`, `ufixed200x54`, `ufixed200x55`, `ufixed200x56`, `ufixed208x0`, `ufixed208x1`, `ufixed208x2`, `ufixed208x3`, `ufixed208x4`, `ufixed208x5`, `ufixed208x6`, `ufixed208x7`, `ufixed208x8`, `ufixed208x9`, `ufixed208x10`, `ufixed208x11`, `ufixed208x12`, `ufixed208x13`, `ufixed208x14`, `ufixed208x15`, `ufixed208x16`, `ufixed208x17`, `ufixed208x18`, `ufixed208x19`, `ufixed208x20`, `ufixed208x21`, `ufixed208x22`, `ufixed208x23`, `ufixed208x24`, `ufixed208x25`, `ufixed208x26`, `ufixed208x27`, `ufixed208x28`, `ufixed208x29`, `ufixed208x30`, `ufixed208x31`, `ufixed208x32`, `ufixed208x33`, `ufixed208x34`, `ufixed208x35`, `ufixed208x36`, `ufixed208x37`, `ufixed208x38`, `ufixed208x39`, `ufixed208x40`, `ufixed208x41`, `ufixed208x42`, `ufixed208x43`, `ufixed208x44`, `ufixed208x45`, `ufixed208x46`, `ufixed208x47`, `ufixed208x48`, `ufixed216x0`, `ufixed216x1`, `ufixed216x2`, `ufixed216x3`, `ufixed216x4`, `ufixed216x5`, `ufixed216x6`, `ufixed216x7`, `ufixed216x8`, `ufixed216x9`, `ufixed216x10`, `ufixed216x11`, `ufixed216x12`, `ufixed216x13`, `ufixed216x14`, `ufixed216x15`, `ufixed216x16`, `ufixed216x17`, `ufixed216x18`, `ufixed216x19`, `ufixed216x20`, `ufixed216x21`, `ufixed216x22`, `ufixed216x23`, `ufixed216x24`, `ufixed216x25`, `ufixed216x26`, `ufixed216x27`, `ufixed216x28`, `ufixed216x29`, `ufixed216x30`, `ufixed216x31`, `ufixed216x32`, `ufixed216x33`, `ufixed216x34`, `ufixed216x35`, `ufixed216x36`, `ufixed216x37`, `ufixed216x38`, `ufixed216x39`, `ufixed216x40`, `ufixed224x0`, `ufixed224x1`, `ufixed224x2`, `ufixed224x3`, `ufixed224x4`, `ufixed224x5`, `ufixed224x6`, `ufixed224x7`, `ufixed224x8`, `ufixed224x9`, `ufixed224x10`, `ufixed224x11`, `ufixed224x12`, `ufixed224x13`, `ufixed224x14`, `ufixed224x15`, `ufixed224x16`, `ufixed224x17`, `ufixed224x18`, `ufixed224x19`, `ufixed224x20`, `ufixed224x21`, `ufixed224x22`, `ufixed224x23`, `ufixed224x24`, `ufixed224x25`, `ufixed224x26`, `ufixed224x27`, `ufixed224x28`, `ufixed224x29`, `ufixed224x30`, `ufixed224x31`, `ufixed224x32`, `ufixed232x0`, `ufixed232x1`, `ufixed232x2`, `ufixed232x3`, `ufixed232x4`, `ufixed232x5`, `ufixed232x6`, `ufixed232x7`, `ufixed232x8`, `ufixed232x9`, `ufixed232x10`, `ufixed232x11`, `ufixed232x12`, `ufixed232x13`, `ufixed232x14`, `ufixed232x15`, `ufixed232x16`, `ufixed232x17`, `ufixed232x18`, `ufixed232x19`, `ufixed232x20`, `ufixed232x21`, `ufixed232x22`, `ufixed232x23`, `ufixed232x24`, `ufixed240x0`, `ufixed240x1`, `ufixed240x2`, `ufixed240x3`, `ufixed240x4`, `ufixed240x5`, `ufixed240x6`, `ufixed240x7`, `ufixed240x8`, `ufixed240x9`, `ufixed240x10`, `ufixed240x11`, `ufixed240x12`, `ufixed240x13`, `ufixed240x14`, `ufixed240x15`, `ufixed240x16`, `ufixed248x0`, `ufixed248x1`, `ufixed248x2`, `ufixed248x3`, `ufixed248x4`, `ufixed248x5`, `ufixed248x6`, `ufixed248x7`, `ufixed248x8`, `ufixed256x0`), KeywordType, nil},
- },
- "numbers": {
- {`0[xX][0-9a-fA-F]+`, LiteralNumberHex, nil},
- {`[0-9]+`, LiteralNumberInteger, nil},
- },
- "string-parse-common": {
- {`\\(u[0-9a-fA-F]{4}|x..|[^x])`, LiteralStringEscape, nil},
- {`[^\\"\'\n]+`, LiteralString, nil},
- {`\\\n`, LiteralString, nil},
- {`\\`, LiteralString, nil},
- },
- "string-parse-double": {
- {`"`, LiteralString, Pop(1)},
- {`'`, LiteralString, nil},
- },
- "string-parse-single": {
- {`'`, LiteralString, Pop(1)},
- {`"`, LiteralString, nil},
- },
- "strings": {
- {`hex'[0-9a-fA-F]+'`, LiteralString, nil},
- {`hex"[0-9a-fA-F]+"`, LiteralString, nil},
- {`"`, LiteralString, Combined("string-parse-common", "string-parse-double")},
- {`'`, LiteralString, Combined("string-parse-common", "string-parse-single")},
- },
- "whitespace": {
- {`\s+`, Text, nil},
- },
- "root": {
- Include("comments"),
- Include("keywords-types"),
- Include("keywords-other"),
- Include("numbers"),
- Include("strings"),
- Include("whitespace"),
- {`\+\+|--|\*\*|\?|:|~|&&|\|\||=>|==?|!=?|(<<|>>>?|[-<>+*%&|^/])=?`, Operator, nil},
- {`[{(\[;,]`, Punctuation, nil},
- {`[})\].]`, Punctuation, nil},
- {`(abi|block|msg|tx)\b`, NameBuiltin, nil},
- {`(?!abi\.)(decode|encode|encodePacked|encodeWithSelector|encodeWithSignature|encodeWithSelector)\b`, NameBuiltin, nil},
- {`(?!block\.)(chainid|coinbase|difficulty|gaslimit|number|timestamp)\b`, NameBuiltin, nil},
- {`(?!msg\.)(data|gas|sender|value)\b`, NameBuiltin, nil},
- {`(?!tx\.)(gasprice|origin)\b`, NameBuiltin, nil},
- {`(type)(\()([a-zA-Z_]\w*)(\))`, ByGroups(NameBuiltin, Punctuation, NameClass, Punctuation), nil},
- {`(?!type\([a-zA-Z_]\w*\)\.)(creationCode|interfaceId|max|min|name|runtimeCode)\b`, NameBuiltin, nil},
- {`(now|this|super|gasleft)\b`, NameBuiltin, nil},
- {`(selfdestruct|suicide)\b`, NameBuiltin, nil},
- {`(?!0x[0-9a-fA-F]+\.)(balance|code|codehash|send|transfer)\b`, NameBuiltin, nil},
- {`(assert|revert|require)\b`, NameBuiltin, nil},
- {`(call|callcode|delegatecall)\b`, NameBuiltin, nil},
- {`selector\b`, NameBuiltin, nil},
- {`(addmod|blockhash|ecrecover|keccak256|mulmod|ripemd160|sha256|sha3)\b`, NameBuiltin, nil},
- {`[a-zA-Z_]\w*`, Name, nil},
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/s/sparql.go b/vendor/github.com/alecthomas/chroma/lexers/s/sparql.go
deleted file mode 100644
index eef73d9..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/s/sparql.go
+++ /dev/null
@@ -1,73 +0,0 @@
-package s
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-// Sparql lexer.
-var Sparql = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "SPARQL",
- Aliases: []string{"sparql"},
- Filenames: []string{"*.rq", "*.sparql"},
- MimeTypes: []string{"application/sparql-query"},
- },
- sparqlRules,
-))
-
-func sparqlRules() Rules {
- return Rules{
- "root": {
- {`\s+`, Text, nil},
- {`((?i)select|construct|describe|ask|where|filter|group\s+by|minus|distinct|reduced|from\s+named|from|order\s+by|desc|asc|limit|offset|bindings|load|clear|drop|create|add|move|copy|insert\s+data|delete\s+data|delete\s+where|delete|insert|using\s+named|using|graph|default|named|all|optional|service|silent|bind|union|not\s+in|in|as|having|to|prefix|base)\b`, Keyword, nil},
- {`(a)\b`, Keyword, nil},
- {"(<(?:[^<>\"{}|^`\\\\\\x00-\\x20])*>)", NameLabel, nil},
- {`(_:[0-9a-zA-ZÀ-ÖØ-öø-˿Ͱ-ͽͿ--⁰-Ⰰ-、-豈-﷏ﷰ-�_](?:[a-zA-ZÀ-ÖØ-öø-˿Ͱ-ͽͿ--⁰-Ⰰ-、-豈-﷏ﷰ-�_\-0-9·̀-ͯ‿-⁀.]*[a-zA-ZÀ-ÖØ-öø-˿Ͱ-ͽͿ--⁰-Ⰰ-、-豈-﷏ﷰ-�_\-0-9·̀-ͯ‿-⁀])?)`, NameLabel, nil},
- {`[?$][0-9a-zA-ZÀ-ÖØ-öø-˿Ͱ-ͽͿ--⁰-Ⰰ-、-豈-﷏ﷰ-�_][a-zA-ZÀ-ÖØ-öø-˿Ͱ-ͽͿ--⁰-Ⰰ-、-豈-﷏ﷰ-�_0-9·̀-ͯ‿-⁀]*`, NameVariable, nil},
- {`([a-zA-ZÀ-ÖØ-öø-˿Ͱ-ͽͿ--⁰-Ⰰ-、-豈-﷏ﷰ-�](?:[a-zA-ZÀ-ÖØ-öø-˿Ͱ-ͽͿ--⁰-Ⰰ-、-豈-﷏ﷰ-�_\-0-9·̀-ͯ‿-⁀.]*[a-zA-ZÀ-ÖØ-öø-˿Ͱ-ͽͿ--⁰-Ⰰ-、-豈-﷏ﷰ-�_\-0-9·̀-ͯ‿-⁀])?)?(\:)((?:[a-zA-ZÀ-ÖØ-öø-˿Ͱ-ͽͿ--⁰-Ⰰ-、-豈-﷏ﷰ-�_:0-9]|(?:%[0-9A-Fa-f][0-9A-Fa-f])|(?:\\[ _~.\-!$&"()*+,;=/?#@%]))(?:(?:[a-zA-ZÀ-ÖØ-öø-˿Ͱ-ͽͿ--⁰-Ⰰ-、-豈-﷏ﷰ-�_\-0-9·̀-ͯ‿-⁀.:]|(?:%[0-9A-Fa-f][0-9A-Fa-f])|(?:\\[ _~.\-!$&"()*+,;=/?#@%]))*(?:[a-zA-ZÀ-ÖØ-öø-˿Ͱ-ͽͿ--⁰-Ⰰ-、-豈-﷏ﷰ-�_\-0-9·̀-ͯ‿-⁀:]|(?:%[0-9A-Fa-f][0-9A-Fa-f])|(?:\\[ _~.\-!$&"()*+,;=/?#@%])))?)?`, ByGroups(NameNamespace, Punctuation, NameTag), nil},
- {`((?i)str|lang|langmatches|datatype|bound|iri|uri|bnode|rand|abs|ceil|floor|round|concat|strlen|ucase|lcase|encode_for_uri|contains|strstarts|strends|strbefore|strafter|year|month|day|hours|minutes|seconds|timezone|tz|now|md5|sha1|sha256|sha384|sha512|coalesce|if|strlang|strdt|sameterm|isiri|isuri|isblank|isliteral|isnumeric|regex|substr|replace|exists|not\s+exists|count|sum|min|max|avg|sample|group_concat|separator)\b`, NameFunction, nil},
- {`(true|false)`, KeywordConstant, nil},
- {`[+\-]?(\d+\.\d*[eE][+-]?\d+|\.?\d+[eE][+-]?\d+)`, LiteralNumberFloat, nil},
- {`[+\-]?(\d+\.\d*|\.\d+)`, LiteralNumberFloat, nil},
- {`[+\-]?\d+`, LiteralNumberInteger, nil},
- {`(\|\||&&|=|\*|\-|\+|/|!=|<=|>=|!|<|>)`, Operator, nil},
- {`[(){}.;,:^\[\]]`, Punctuation, nil},
- {`#[^\n]*`, Comment, nil},
- {`"""`, LiteralString, Push("triple-double-quoted-string")},
- {`"`, LiteralString, Push("single-double-quoted-string")},
- {`'''`, LiteralString, Push("triple-single-quoted-string")},
- {`'`, LiteralString, Push("single-single-quoted-string")},
- },
- "triple-double-quoted-string": {
- {`"""`, LiteralString, Push("end-of-string")},
- {`[^\\]+`, LiteralString, nil},
- {`\\`, LiteralString, Push("string-escape")},
- },
- "single-double-quoted-string": {
- {`"`, LiteralString, Push("end-of-string")},
- {`[^"\\\n]+`, LiteralString, nil},
- {`\\`, LiteralString, Push("string-escape")},
- },
- "triple-single-quoted-string": {
- {`'''`, LiteralString, Push("end-of-string")},
- {`[^\\]+`, LiteralString, nil},
- {`\\`, LiteralStringEscape, Push("string-escape")},
- },
- "single-single-quoted-string": {
- {`'`, LiteralString, Push("end-of-string")},
- {`[^'\\\n]+`, LiteralString, nil},
- {`\\`, LiteralString, Push("string-escape")},
- },
- "string-escape": {
- {`u[0-9A-Fa-f]{4}`, LiteralStringEscape, Pop(1)},
- {`U[0-9A-Fa-f]{8}`, LiteralStringEscape, Pop(1)},
- {`.`, LiteralStringEscape, Pop(1)},
- },
- "end-of-string": {
- {`(@)([a-zA-Z]+(?:-[a-zA-Z0-9]+)*)`, ByGroups(Operator, NameFunction), Pop(2)},
- {`\^\^`, Operator, Pop(2)},
- Default(Pop(2)),
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/s/sql.go b/vendor/github.com/alecthomas/chroma/lexers/s/sql.go
deleted file mode 100644
index fb8c8ed..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/s/sql.go
+++ /dev/null
@@ -1,53 +0,0 @@
-package s
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-// SQL lexer.
-var SQL = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "SQL",
- Aliases: []string{"sql"},
- Filenames: []string{"*.sql"},
- MimeTypes: []string{"text/x-sql"},
- NotMultiline: true,
- CaseInsensitive: true,
- },
- sqlRules,
-))
-
-func sqlRules() Rules {
- return Rules{
- "root": {
- {`\s+`, TextWhitespace, nil},
- {`--.*\n?`, CommentSingle, nil},
- {`/\*`, CommentMultiline, Push("multiline-comments")},
- {`'`, LiteralStringSingle, Push("string")},
- {`"`, LiteralStringDouble, Push("double-string")},
- {Words(``, `\b`, `ABORT`, `ABS`, `ABSOLUTE`, `ACCESS`, `ADA`, `ADD`, `ADMIN`, `AFTER`, `AGGREGATE`, `ALIAS`, `ALL`, `ALLOCATE`, `ALTER`, `ANALYSE`, `ANALYZE`, `AND`, `ANY`, `ARE`, `AS`, `ASC`, `ASENSITIVE`, `ASSERTION`, `ASSIGNMENT`, `ASYMMETRIC`, `AT`, `ATOMIC`, `AUTHORIZATION`, `AVG`, `BACKWARD`, `BEFORE`, `BEGIN`, `BETWEEN`, `BITVAR`, `BIT_LENGTH`, `BOTH`, `BREADTH`, `BY`, `C`, `CACHE`, `CALL`, `CALLED`, `CARDINALITY`, `CASCADE`, `CASCADED`, `CASE`, `CAST`, `CATALOG`, `CATALOG_NAME`, `CHAIN`, `CHARACTERISTICS`, `CHARACTER_LENGTH`, `CHARACTER_SET_CATALOG`, `CHARACTER_SET_NAME`, `CHARACTER_SET_SCHEMA`, `CHAR_LENGTH`, `CHECK`, `CHECKED`, `CHECKPOINT`, `CLASS`, `CLASS_ORIGIN`, `CLOB`, `CLOSE`, `CLUSTER`, `COALSECE`, `COBOL`, `COLLATE`, `COLLATION`, `COLLATION_CATALOG`, `COLLATION_NAME`, `COLLATION_SCHEMA`, `COLUMN`, `COLUMN_NAME`, `COMMAND_FUNCTION`, `COMMAND_FUNCTION_CODE`, `COMMENT`, `COMMIT`, `COMMITTED`, `COMPLETION`, `CONDITION_NUMBER`, `CONNECT`, `CONNECTION`, `CONNECTION_NAME`, `CONSTRAINT`, `CONSTRAINTS`, `CONSTRAINT_CATALOG`, `CONSTRAINT_NAME`, `CONSTRAINT_SCHEMA`, `CONSTRUCTOR`, `CONTAINS`, `CONTINUE`, `CONVERSION`, `CONVERT`, `COPY`, `CORRESPONTING`, `COUNT`, `CREATE`, `CREATEDB`, `CREATEUSER`, `CROSS`, `CUBE`, `CURRENT`, `CURRENT_DATE`, `CURRENT_PATH`, `CURRENT_ROLE`, `CURRENT_TIME`, `CURRENT_TIMESTAMP`, `CURRENT_USER`, `CURSOR`, `CURSOR_NAME`, `CYCLE`, `DATA`, `DATABASE`, `DATETIME_INTERVAL_CODE`, `DATETIME_INTERVAL_PRECISION`, `DAY`, `DEALLOCATE`, `DECLARE`, `DEFAULT`, `DEFAULTS`, `DEFERRABLE`, `DEFERRED`, `DEFINED`, `DEFINER`, `DELETE`, `DELIMITER`, `DELIMITERS`, `DEREF`, `DESC`, `DESCRIBE`, `DESCRIPTOR`, `DESTROY`, `DESTRUCTOR`, `DETERMINISTIC`, `DIAGNOSTICS`, `DICTIONARY`, `DISCONNECT`, `DISPATCH`, `DISTINCT`, `DO`, `DOMAIN`, `DROP`, `DYNAMIC`, `DYNAMIC_FUNCTION`, `DYNAMIC_FUNCTION_CODE`, `EACH`, `ELSE`, `ELSIF`, `ENCODING`, `ENCRYPTED`, `END`, `END-EXEC`, `EQUALS`, `ESCAPE`, `EVERY`, `EXCEPTION`, `EXCEPT`, `EXCLUDING`, `EXCLUSIVE`, `EXEC`, `EXECUTE`, `EXISTING`, `EXISTS`, `EXPLAIN`, `EXTERNAL`, `EXTRACT`, `FALSE`, `FETCH`, `FINAL`, `FIRST`, `FOR`, `FORCE`, `FOREIGN`, `FORTRAN`, `FORWARD`, `FOUND`, `FREE`, `FREEZE`, `FROM`, `FULL`, `FUNCTION`, `G`, `GENERAL`, `GENERATED`, `GET`, `GLOBAL`, `GO`, `GOTO`, `GRANT`, `GRANTED`, `GROUP`, `GROUPING`, `HANDLER`, `HAVING`, `HIERARCHY`, `HOLD`, `HOST`, `IDENTITY`, `IF`, `IGNORE`, `ILIKE`, `IMMEDIATE`, `IMMUTABLE`, `IMPLEMENTATION`, `IMPLICIT`, `IN`, `INCLUDING`, `INCREMENT`, `INDEX`, `INDITCATOR`, `INFIX`, `INHERITS`, `INITIALIZE`, `INITIALLY`, `INNER`, `INOUT`, `INPUT`, `INSENSITIVE`, `INSERT`, `INSTANTIABLE`, `INSTEAD`, `INTERSECT`, `INTO`, `INVOKER`, `IS`, `ISNULL`, `ISOLATION`, `ITERATE`, `JOIN`, `KEY`, `KEY_MEMBER`, `KEY_TYPE`, `LANCOMPILER`, `LANGUAGE`, `LARGE`, `LAST`, `LATERAL`, `LEADING`, `LEFT`, `LENGTH`, `LESS`, `LEVEL`, `LIKE`, `LIMIT`, `LISTEN`, `LOAD`, `LOCAL`, `LOCALTIME`, `LOCALTIMESTAMP`, `LOCATION`, `LOCATOR`, `LOCK`, `LOWER`, `MAP`, `MATCH`, `MAX`, `MAXVALUE`, `MESSAGE_LENGTH`, `MESSAGE_OCTET_LENGTH`, `MESSAGE_TEXT`, `METHOD`, `MIN`, `MINUTE`, `MINVALUE`, `MOD`, `MODE`, `MODIFIES`, `MODIFY`, `MONTH`, `MORE`, `MOVE`, `MUMPS`, `NAMES`, `NATIONAL`, `NATURAL`, `NCHAR`, `NCLOB`, `NEW`, `NEXT`, `NO`, `NOCREATEDB`, `NOCREATEUSER`, `NONE`, `NOT`, `NOTHING`, `NOTIFY`, `NOTNULL`, `NULL`, `NULLABLE`, `NULLIF`, `OBJECT`, `OCTET_LENGTH`, `OF`, `OFF`, `OFFSET`, `OIDS`, `OLD`, `ON`, `ONLY`, `OPEN`, `OPERATION`, `OPERATOR`, `OPTION`, `OPTIONS`, `OR`, `ORDER`, `ORDINALITY`, `OUT`, `OUTER`, `OUTPUT`, `OVERLAPS`, `OVERLAY`, `OVERRIDING`, `OWNER`, `PAD`, `PARAMETER`, `PARAMETERS`, `PARAMETER_MODE`, `PARAMATER_NAME`, `PARAMATER_ORDINAL_POSITION`, `PARAMETER_SPECIFIC_CATALOG`, `PARAMETER_SPECIFIC_NAME`, `PARAMATER_SPECIFIC_SCHEMA`, `PARTIAL`, `PASCAL`, `PENDANT`, `PLACING`, `PLI`, `POSITION`, `POSTFIX`, `PRECISION`, `PREFIX`, `PREORDER`, `PREPARE`, `PRESERVE`, `PRIMARY`, `PRIOR`, `PRIVILEGES`, `PROCEDURAL`, `PROCEDURE`, `PUBLIC`, `READ`, `READS`, `RECHECK`, `RECURSIVE`, `REF`, `REFERENCES`, `REFERENCING`, `REINDEX`, `RELATIVE`, `RENAME`, `REPEATABLE`, `REPLACE`, `RESET`, `RESTART`, `RESTRICT`, `RESULT`, `RETURN`, `RETURNED_LENGTH`, `RETURNED_OCTET_LENGTH`, `RETURNED_SQLSTATE`, `RETURNS`, `REVOKE`, `RIGHT`, `ROLE`, `ROLLBACK`, `ROLLUP`, `ROUTINE`, `ROUTINE_CATALOG`, `ROUTINE_NAME`, `ROUTINE_SCHEMA`, `ROW`, `ROWS`, `ROW_COUNT`, `RULE`, `SAVE_POINT`, `SCALE`, `SCHEMA`, `SCHEMA_NAME`, `SCOPE`, `SCROLL`, `SEARCH`, `SECOND`, `SECURITY`, `SELECT`, `SELF`, `SENSITIVE`, `SERIALIZABLE`, `SERVER_NAME`, `SESSION`, `SESSION_USER`, `SET`, `SETOF`, `SETS`, `SHARE`, `SHOW`, `SIMILAR`, `SIMPLE`, `SIZE`, `SOME`, `SOURCE`, `SPACE`, `SPECIFIC`, `SPECIFICTYPE`, `SPECIFIC_NAME`, `SQL`, `SQLCODE`, `SQLERROR`, `SQLEXCEPTION`, `SQLSTATE`, `SQLWARNINIG`, `STABLE`, `START`, `STATE`, `STATEMENT`, `STATIC`, `STATISTICS`, `STDIN`, `STDOUT`, `STORAGE`, `STRICT`, `STRUCTURE`, `STYPE`, `SUBCLASS_ORIGIN`, `SUBLIST`, `SUBSTRING`, `SUM`, `SYMMETRIC`, `SYSID`, `SYSTEM`, `SYSTEM_USER`, `TABLE`, `TABLE_NAME`, ` TEMP`, `TEMPLATE`, `TEMPORARY`, `TERMINATE`, `THAN`, `THEN`, `TIMESTAMP`, `TIMEZONE_HOUR`, `TIMEZONE_MINUTE`, `TO`, `TOAST`, `TRAILING`, `TRANSACTION`, `TRANSACTIONS_COMMITTED`, `TRANSACTIONS_ROLLED_BACK`, `TRANSACTION_ACTIVE`, `TRANSFORM`, `TRANSFORMS`, `TRANSLATE`, `TRANSLATION`, `TREAT`, `TRIGGER`, `TRIGGER_CATALOG`, `TRIGGER_NAME`, `TRIGGER_SCHEMA`, `TRIM`, `TRUE`, `TRUNCATE`, `TRUSTED`, `TYPE`, `UNCOMMITTED`, `UNDER`, `UNENCRYPTED`, `UNION`, `UNIQUE`, `UNKNOWN`, `UNLISTEN`, `UNNAMED`, `UNNEST`, `UNTIL`, `UPDATE`, `UPPER`, `USAGE`, `USER`, `USER_DEFINED_TYPE_CATALOG`, `USER_DEFINED_TYPE_NAME`, `USER_DEFINED_TYPE_SCHEMA`, `USING`, `VACUUM`, `VALID`, `VALIDATOR`, `VALUES`, `VARIABLE`, `VERBOSE`, `VERSION`, `VIEW`, `VOLATILE`, `WHEN`, `WHENEVER`, `WHERE`, `WITH`, `WITHOUT`, `WORK`, `WRITE`, `YEAR`, `ZONE`), Keyword, nil},
- {Words(``, `\b`, `ARRAY`, `BIGINT`, `BINARY`, `BIT`, `BLOB`, `BOOLEAN`, `CHAR`, `CHARACTER`, `DATE`, `DEC`, `DECIMAL`, `FLOAT`, `INT`, `INTEGER`, `INTERVAL`, `NUMBER`, `NUMERIC`, `REAL`, `SERIAL`, `SMALLINT`, `VARCHAR`, `VARYING`, `INT8`, `SERIAL8`, `TEXT`), NameBuiltin, nil},
- {"[+*/<>=~!@#%^&|`?-]", Operator, nil},
- {`[0-9]+`, LiteralNumberInteger, nil},
- {`[a-z_][\w$]*`, Name, nil},
- {`[;:()\[\],.]`, Punctuation, nil},
- },
- "multiline-comments": {
- {`/\*`, CommentMultiline, Push("multiline-comments")},
- {`\*/`, CommentMultiline, Pop(1)},
- {`[^/*]+`, CommentMultiline, nil},
- {`[/*]`, CommentMultiline, nil},
- },
- "string": {
- {`[^']+`, LiteralStringSingle, nil},
- {`''`, LiteralStringSingle, nil},
- {`'`, LiteralStringSingle, Pop(1)},
- },
- "double-string": {
- {`[^"]+`, LiteralStringDouble, nil},
- {`""`, LiteralStringDouble, nil},
- {`"`, LiteralStringDouble, Pop(1)},
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/s/squid.go b/vendor/github.com/alecthomas/chroma/lexers/s/squid.go
deleted file mode 100644
index b4da3da..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/s/squid.go
+++ /dev/null
@@ -1,42 +0,0 @@
-package s
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-// Squidconf lexer.
-var Squidconf = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "SquidConf",
- Aliases: []string{"squidconf", "squid.conf", "squid"},
- Filenames: []string{"squid.conf"},
- MimeTypes: []string{"text/x-squidconf"},
- NotMultiline: true,
- CaseInsensitive: true,
- },
- squidconfRules,
-))
-
-func squidconfRules() Rules {
- return Rules{
- "root": {
- {`\s+`, TextWhitespace, nil},
- {`#`, Comment, Push("comment")},
- {Words(`\b`, `\b`, `access_log`, `acl`, `always_direct`, `announce_host`, `announce_period`, `announce_port`, `announce_to`, `anonymize_headers`, `append_domain`, `as_whois_server`, `auth_param_basic`, `authenticate_children`, `authenticate_program`, `authenticate_ttl`, `broken_posts`, `buffered_logs`, `cache_access_log`, `cache_announce`, `cache_dir`, `cache_dns_program`, `cache_effective_group`, `cache_effective_user`, `cache_host`, `cache_host_acl`, `cache_host_domain`, `cache_log`, `cache_mem`, `cache_mem_high`, `cache_mem_low`, `cache_mgr`, `cachemgr_passwd`, `cache_peer`, `cache_peer_access`, `cahce_replacement_policy`, `cache_stoplist`, `cache_stoplist_pattern`, `cache_store_log`, `cache_swap`, `cache_swap_high`, `cache_swap_log`, `cache_swap_low`, `client_db`, `client_lifetime`, `client_netmask`, `connect_timeout`, `coredump_dir`, `dead_peer_timeout`, `debug_options`, `delay_access`, `delay_class`, `delay_initial_bucket_level`, `delay_parameters`, `delay_pools`, `deny_info`, `dns_children`, `dns_defnames`, `dns_nameservers`, `dns_testnames`, `emulate_httpd_log`, `err_html_text`, `fake_user_agent`, `firewall_ip`, `forwarded_for`, `forward_snmpd_port`, `fqdncache_size`, `ftpget_options`, `ftpget_program`, `ftp_list_width`, `ftp_passive`, `ftp_user`, `half_closed_clients`, `header_access`, `header_replace`, `hierarchy_stoplist`, `high_response_time_warning`, `high_page_fault_warning`, `hosts_file`, `htcp_port`, `http_access`, `http_anonymizer`, `httpd_accel`, `httpd_accel_host`, `httpd_accel_port`, `httpd_accel_uses_host_header`, `httpd_accel_with_proxy`, `http_port`, `http_reply_access`, `icp_access`, `icp_hit_stale`, `icp_port`, `icp_query_timeout`, `ident_lookup`, `ident_lookup_access`, `ident_timeout`, `incoming_http_average`, `incoming_icp_average`, `inside_firewall`, `ipcache_high`, `ipcache_low`, `ipcache_size`, `local_domain`, `local_ip`, `logfile_rotate`, `log_fqdn`, `log_icp_queries`, `log_mime_hdrs`, `maximum_object_size`, `maximum_single_addr_tries`, `mcast_groups`, `mcast_icp_query_timeout`, `mcast_miss_addr`, `mcast_miss_encode_key`, `mcast_miss_port`, `memory_pools`, `memory_pools_limit`, `memory_replacement_policy`, `mime_table`, `min_http_poll_cnt`, `min_icp_poll_cnt`, `minimum_direct_hops`, `minimum_object_size`, `minimum_retry_timeout`, `miss_access`, `negative_dns_ttl`, `negative_ttl`, `neighbor_timeout`, `neighbor_type_domain`, `netdb_high`, `netdb_low`, `netdb_ping_period`, `netdb_ping_rate`, `never_direct`, `no_cache`, `passthrough_proxy`, `pconn_timeout`, `pid_filename`, `pinger_program`, `positive_dns_ttl`, `prefer_direct`, `proxy_auth`, `proxy_auth_realm`, `query_icmp`, `quick_abort`, `quick_abort_max`, `quick_abort_min`, `quick_abort_pct`, `range_offset_limit`, `read_timeout`, `redirect_children`, `redirect_program`, `redirect_rewrites_host_header`, `reference_age`, `refresh_pattern`, `reload_into_ims`, `request_body_max_size`, `request_size`, `request_timeout`, `shutdown_lifetime`, `single_parent_bypass`, `siteselect_timeout`, `snmp_access`, `snmp_incoming_address`, `snmp_port`, `source_ping`, `ssl_proxy`, `store_avg_object_size`, `store_objects_per_bucket`, `strip_query_terms`, `swap_level1_dirs`, `swap_level2_dirs`, `tcp_incoming_address`, `tcp_outgoing_address`, `tcp_recv_bufsize`, `test_reachability`, `udp_hit_obj`, `udp_hit_obj_size`, `udp_incoming_address`, `udp_outgoing_address`, `unique_hostname`, `unlinkd_program`, `uri_whitespace`, `useragent_log`, `visible_hostname`, `wais_relay`, `wais_relay_host`, `wais_relay_port`), Keyword, nil},
- {Words(`\b`, `\b`, `proxy-only`, `weight`, `ttl`, `no-query`, `default`, `round-robin`, `multicast-responder`, `on`, `off`, `all`, `deny`, `allow`, `via`, `parent`, `no-digest`, `heap`, `lru`, `realm`, `children`, `q1`, `q2`, `credentialsttl`, `none`, `disable`, `offline_toggle`, `diskd`), NameConstant, nil},
- {Words(`\b`, `\b`, `shutdown`, `info`, `parameter`, `server_list`, `client_list`, `squid.conf`), LiteralString, nil},
- {Words(`stats/`, `\b`, `objects`, `vm_objects`, `utilization`, `ipcache`, `fqdncache`, `dns`, `redirector`, `io`, `reply_headers`, `filedescriptors`, `netdb`), LiteralString, nil},
- {Words(`log/`, `=`, `status`, `enable`, `disable`, `clear`), LiteralString, nil},
- {Words(`\b`, `\b`, `url_regex`, `urlpath_regex`, `referer_regex`, `port`, `proto`, `req_mime_type`, `rep_mime_type`, `method`, `browser`, `user`, `src`, `dst`, `time`, `dstdomain`, `ident`, `snmp_community`), Keyword, nil},
- {`(?:(?:(?:[3-9]\d?|2(?:5[0-5]|[0-4]?\d)?|1\d{0,2}|0x0*[0-9a-f]{1,2}|0+[1-3]?[0-7]{0,2})(?:\.(?:[3-9]\d?|2(?:5[0-5]|[0-4]?\d)?|1\d{0,2}|0x0*[0-9a-f]{1,2}|0+[1-3]?[0-7]{0,2})){3})|(?!.*::.*::)(?:(?!:)|:(?=:))(?:[0-9a-f]{0,4}(?:(?<=::)|(?\=|\<|\>|\=\=|\!\=|\&\&|\|\||\=|\:\=|\?\=|\+\=|\-\=|\*\=|\/\=|\%\=|\?|\:)`, Operator, nil},
- {`\b(and|if unless|in|is|is a|is defined|is not|isnt|or|not)\b`, OperatorWord, nil},
- {`(\#[a-f0-9]{3,6})`, LiteralNumberHex, nil},
- {`\n`, Text, nil},
- },
- "inline-comment": {
- {`\*/`, Comment, Pop(1)},
- },
- "atrule": {
- {`\{`, Punctuation, Push("atcontent")},
- {`$`, Punctuation, Pop(1)},
- Include("root"),
- },
- "atcontent": {
- Include("root"),
- {`\}`, Punctuation, Pop(2)},
- },
- "function-start": {
- {`\)`, Punctuation, Pop(1)},
- Include("root"),
- },
- "values": {
- {`\s+`, Text, nil},
- {`(\#[a-f0-9]{3,6})`, LiteralNumberHex, nil},
- {Words(`\b`, `\b`, `absolute`, `alias`, `all`, `all-petite-caps`, `all-scroll`, `all-small-caps`, `allow-end`, `alpha`, `alternate`, `alternate-reverse`, `always`, `armenian`, `auto`, `avoid`, `avoid-column`, `avoid-page`, `backwards`, `balance`, `baseline`, `below`, `blink`, `block`, `bold`, `bolder`, `border-box`, `both`, `bottom`, `box-decoration`, `break-word`, `capitalize`, `cell`, `center`, `circle`, `clip`, `clone`, `close-quote`, `col-resize`, `collapse`, `color`, `color-burn`, `color-dodge`, `column`, `column-reverse`, `compact`, `condensed`, `contain`, `container`, `content-box`, `context-menu`, `copy`, `cover`, `crisp-edges`, `crosshair`, `currentColor`, `cursive`, `darken`, `dashed`, `decimal`, `decimal-leading-zero`, `default`, `descendants`, `difference`, `digits`, `disc`, `distribute`, `dot`, `dotted`, `double`, `double-circle`, `e-resize`, `each-line`, `ease`, `ease-in`, `ease-in-out`, `ease-out`, `edges`, `ellipsis`, `end`, `ew-resize`, `exclusion`, `expanded`, `extra-condensed`, `extra-expanded`, `fantasy`, `fill`, `fill-box`, `filled`, `first`, `fixed`, `flat`, `flex`, `flex-end`, `flex-start`, `flip`, `force-end`, `forwards`, `from-image`, `full-width`, `geometricPrecision`, `georgian`, `groove`, `hanging`, `hard-light`, `help`, `hidden`, `hide`, `horizontal`, `hue`, `icon`, `infinite`, `inherit`, `initial`, `ink`, `inline`, `inline-block`, `inline-flex`, `inline-table`, `inset`, `inside`, `inter-word`, `invert`, `isolate`, `italic`, `justify`, `large`, `larger`, `last`, `left`, `lighten`, `lighter`, `line-through`, `linear`, `list-item`, `local`, `loose`, `lower-alpha`, `lower-greek`, `lower-latin`, `lower-roman`, `lowercase`, `ltr`, `luminance`, `luminosity`, `mandatory`, `manipulation`, `manual`, `margin-box`, `match-parent`, `medium`, `mixed`, `monospace`, `move`, `multiply`, `n-resize`, `ne-resize`, `nesw-resize`, `no-close-quote`, `no-drop`, `no-open-quote`, `no-repeat`, `none`, `normal`, `not-allowed`, `nowrap`, `ns-resize`, `nw-resize`, `nwse-resize`, `objects`, `oblique`, `off`, `on`, `open`, `open-quote`, `optimizeLegibility`, `optimizeSpeed`, `outset`, `outside`, `over`, `overlay`, `overline`, `padding-box`, `page`, `pan-down`, `pan-left`, `pan-right`, `pan-up`, `pan-x`, `pan-y`, `paused`, `petite-caps`, `pixelated`, `pointer`, `preserve-3d`, `progress`, `proximity`, `relative`, `repeat`, `repeat no-repeat`, `repeat-x`, `repeat-y`, `reverse`, `ridge`, `right`, `round`, `row`, `row-resize`, `row-reverse`, `rtl`, `ruby`, `ruby-base`, `ruby-base-container`, `ruby-text`, `ruby-text-container`, `run-in`, `running`, `s-resize`, `sans-serif`, `saturation`, `scale-down`, `screen`, `scroll`, `se-resize`, `semi-condensed`, `semi-expanded`, `separate`, `serif`, `sesame`, `show`, `sideways`, `sideways-left`, `sideways-right`, `slice`, `small`, `small-caps`, `smaller`, `smooth`, `snap`, `soft-light`, `solid`, `space`, `space-around`, `space-between`, `spaces`, `square`, `start`, `static`, `step-end`, `step-start`, `sticky`, `stretch`, `strict`, `stroke-box`, `style`, `sw-resize`, `table`, `table-caption`, `table-cell`, `table-column`, `table-column-group`, `table-footer-group`, `table-header-group`, `table-row`, `table-row-group`, `text`, `thick`, `thin`, `titling-caps`, `to`, `top`, `triangle`, `ultra-condensed`, `ultra-expanded`, `under`, `underline`, `unicase`, `unset`, `upper-alpha`, `upper-latin`, `upper-roman`, `uppercase`, `upright`, `use-glyph-orientation`, `vertical`, `vertical-text`, `view-box`, `visible`, `w-resize`, `wait`, `wavy`, `weight`, `weight style`, `wrap`, `wrap-reverse`, `x-large`, `x-small`, `xx-large`, `xx-small`, `zoom-in`, `zoom-out`), KeywordConstant, nil},
- {`\;?`, Punctuation, Pop(1)},
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/s/svelte.go b/vendor/github.com/alecthomas/chroma/lexers/s/svelte.go
deleted file mode 100644
index 1f91a21..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/s/svelte.go
+++ /dev/null
@@ -1,73 +0,0 @@
-package s
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/h"
- "github.com/alecthomas/chroma/lexers/internal"
- "github.com/alecthomas/chroma/lexers/t"
-)
-
-// Svelte lexer.
-var Svelte = internal.Register(DelegatingLexer(h.HTML, MustNewLazyLexer(
- &Config{
- Name: "Svelte",
- Aliases: []string{"svelte"},
- Filenames: []string{"*.svelte"},
- MimeTypes: []string{"application/x-svelte"},
- DotAll: true,
- },
- svelteRules,
-)))
-
-func svelteRules() Rules {
- return Rules{
- "root": {
- // Let HTML handle the comments, including comments containing script and style tags
- {``, Other, Pop(1)},
- {`.+?`, Other, nil},
- },
- "templates": {
- {`}`, Punctuation, Pop(1)},
- // Let TypeScript handle strings and the curly braces inside them
- {`(?|[<&?](?=\\w)|(?<=\\w)[>!?]", Punctuation, nil},
- {`[/=\-+!*%<>&|^?~]+`, Operator, nil},
- {`[a-zA-Z_]\w*`, Name, nil},
- },
- "keywords": {
- {Words(``, `\b`, `as`, `break`, `case`, `catch`, `continue`, `default`, `defer`, `do`, `else`, `fallthrough`, `for`, `guard`, `if`, `in`, `is`, `repeat`, `return`, `#selector`, `switch`, `throw`, `try`, `where`, `while`), Keyword, nil},
- {`@availability\([^)]+\)`, KeywordReserved, nil},
- {Words(``, `\b`, `associativity`, `convenience`, `dynamic`, `didSet`, `final`, `get`, `indirect`, `infix`, `inout`, `lazy`, `left`, `mutating`, `none`, `nonmutating`, `optional`, `override`, `postfix`, `precedence`, `prefix`, `Protocol`, `required`, `rethrows`, `right`, `set`, `throws`, `Type`, `unowned`, `weak`, `willSet`, `@availability`, `@autoclosure`, `@noreturn`, `@NSApplicationMain`, `@NSCopying`, `@NSManaged`, `@objc`, `@UIApplicationMain`, `@IBAction`, `@IBDesignable`, `@IBInspectable`, `@IBOutlet`), KeywordReserved, nil},
- {`(as|dynamicType|false|is|nil|self|Self|super|true|__COLUMN__|__FILE__|__FUNCTION__|__LINE__|_|#(?:file|line|column|function))\b`, KeywordConstant, nil},
- {`import\b`, KeywordDeclaration, Push("module")},
- {`(class|enum|extension|struct|protocol)(\s+)([a-zA-Z_]\w*)`, ByGroups(KeywordDeclaration, Text, NameClass), nil},
- {`(func)(\s+)([a-zA-Z_]\w*)`, ByGroups(KeywordDeclaration, Text, NameFunction), nil},
- {`(var|let)(\s+)([a-zA-Z_]\w*)`, ByGroups(KeywordDeclaration, Text, NameVariable), nil},
- {Words(``, `\b`, `class`, `deinit`, `enum`, `extension`, `func`, `import`, `init`, `internal`, `let`, `operator`, `private`, `protocol`, `public`, `static`, `struct`, `subscript`, `typealias`, `var`), KeywordDeclaration, nil},
- },
- "comment": {
- {`:param: [a-zA-Z_]\w*|:returns?:|(FIXME|MARK|TODO):`, CommentSpecial, nil},
- },
- "comment-single": {
- {`\n`, Text, Pop(1)},
- Include("comment"),
- {`[^\n]`, CommentSingle, nil},
- },
- "comment-multi": {
- Include("comment"),
- {`[^*/]`, CommentMultiline, nil},
- {`/\*`, CommentMultiline, Push()},
- {`\*/`, CommentMultiline, Pop(1)},
- {`[*/]`, CommentMultiline, nil},
- },
- "module": {
- {`\n`, Text, Pop(1)},
- {`[a-zA-Z_]\w*`, NameClass, nil},
- Include("root"),
- },
- "preproc": {
- {`\n`, Text, Pop(1)},
- Include("keywords"),
- {`[A-Za-z]\w*`, CommentPreproc, nil},
- Include("root"),
- },
- "string": {
- {`\\\(`, LiteralStringInterpol, Push("string-intp")},
- {`"`, LiteralString, Pop(1)},
- {`\\['"\\nrt]|\\x[0-9a-fA-F]{2}|\\[0-7]{1,3}|\\u[0-9a-fA-F]{4}|\\U[0-9a-fA-F]{8}`, LiteralStringEscape, nil},
- {`[^\\"]+`, LiteralString, nil},
- {`\\`, LiteralString, nil},
- },
- "string-intp": {
- {`\(`, LiteralStringInterpol, Push()},
- {`\)`, LiteralStringInterpol, Pop(1)},
- Include("root"),
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/s/systemd.go b/vendor/github.com/alecthomas/chroma/lexers/s/systemd.go
deleted file mode 100644
index 0da9782..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/s/systemd.go
+++ /dev/null
@@ -1,33 +0,0 @@
-package s
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-var SYSTEMD = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "SYSTEMD",
- Aliases: []string{"systemd"},
- // Suspects: man systemd.index | grep -E 'systemd\..*configuration'
- Filenames: []string{"*.automount", "*.device", "*.dnssd", "*.link", "*.mount", "*.netdev", "*.network", "*.path", "*.scope", "*.service", "*.slice", "*.socket", "*.swap", "*.target", "*.timer"},
- MimeTypes: []string{"text/plain"},
- },
- systemdRules,
-))
-
-func systemdRules() Rules {
- return Rules{
- "root": {
- {`\s+`, Text, nil},
- {`[;#].*`, Comment, nil},
- {`\[.*?\]$`, Keyword, nil},
- {`(.*?)(=)(.*)(\\\n)`, ByGroups(NameAttribute, Operator, LiteralString, Text), Push("continuation")},
- {`(.*?)(=)(.*)`, ByGroups(NameAttribute, Operator, LiteralString), nil},
- },
- "continuation": {
- {`(.*?)(\\\n)`, ByGroups(LiteralString, Text), nil},
- {`(.*)`, LiteralString, Pop(1)},
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/s/systemverilog.go b/vendor/github.com/alecthomas/chroma/lexers/s/systemverilog.go
deleted file mode 100644
index a9a77f5..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/s/systemverilog.go
+++ /dev/null
@@ -1,77 +0,0 @@
-package s
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-// Systemverilog lexer.
-var Systemverilog = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "systemverilog",
- Aliases: []string{"systemverilog", "sv"},
- Filenames: []string{"*.sv", "*.svh"},
- MimeTypes: []string{"text/x-systemverilog"},
- EnsureNL: true,
- },
- systemvarilogRules,
-))
-
-func systemvarilogRules() Rules {
- return Rules{
- "root": {
- {"^\\s*`define", CommentPreproc, Push("macro")},
- {`^(\s*)(package)(\s+)`, ByGroups(Text, KeywordNamespace, Text), nil},
- {`^(\s*)(import)(\s+)("DPI(?:-C)?")(\s+)`, ByGroups(Text, KeywordNamespace, Text, LiteralString, Text), nil},
- {`^(\s*)(import)(\s+)`, ByGroups(Text, KeywordNamespace, Text), Push("import")},
- {`\n`, Text, nil},
- {`\s+`, Text, nil},
- {`\\\n`, Text, nil},
- {`/(\\\n)?/(\n|(.|\n)*?[^\\]\n)`, CommentSingle, nil},
- {`/(\\\n)?[*](.|\n)*?[*](\\\n)?/`, CommentMultiline, nil},
- {`[{}#@]`, Punctuation, nil},
- {`L?"`, LiteralString, Push("string")},
- {`L?'(\\.|\\[0-7]{1,3}|\\x[a-fA-F0-9]{1,2}|[^\\\'\n])'`, LiteralStringChar, nil},
- {`(\d+\.\d*|\.\d+|\d+)[eE][+-]?\d+[lL]?`, LiteralNumberFloat, nil},
- {`(\d+\.\d*|\.\d+|\d+[fF])[fF]?`, LiteralNumberFloat, nil},
- {`([0-9]+)|(\'h)[0-9a-fA-F]+`, LiteralNumberHex, nil},
- {`([0-9]+)|(\'b)[01]+`, LiteralNumberBin, nil},
- {`([0-9]+)|(\'d)[0-9]+`, LiteralNumberInteger, nil},
- {`([0-9]+)|(\'o)[0-7]+`, LiteralNumberOct, nil},
- {`\'[01xz]`, LiteralNumber, nil},
- {`\d+[Ll]?`, LiteralNumberInteger, nil},
- {`\*/`, Error, nil},
- {`[~!%^&*+=|?:<>/-]`, Operator, nil},
- {`[()\[\],.;\']`, Punctuation, nil},
- {"`[a-zA-Z_]\\w*", NameConstant, nil},
- {Words(``, `\b`, `accept_on`, `alias`, `always`, `always_comb`, `always_ff`, `always_latch`, `and`, `assert`, `assign`, `assume`, `automatic`, `before`, `begin`, `bind`, `bins`, `binsof`, `bit`, `break`, `buf`, `bufif0`, `bufif1`, `byte`, `case`, `casex`, `casez`, `cell`, `chandle`, `checker`, `class`, `clocking`, `cmos`, `config`, `const`, `constraint`, `context`, `continue`, `cover`, `covergroup`, `coverpoint`, `cross`, `deassign`, `default`, `defparam`, `design`, `disable`, `dist`, `do`, `edge`, `else`, `end`, `endcase`, `endchecker`, `endclass`, `endclocking`, `endconfig`, `endfunction`, `endgenerate`, `endgroup`, `endinterface`, `endmodule`, `endpackage`, `endprimitive`, `endprogram`, `endproperty`, `endsequence`, `endspecify`, `endtable`, `endtask`, `enum`, `event`, `eventually`, `expect`, `export`, `extends`, `extern`, `final`, `first_match`, `for`, `force`, `foreach`, `forever`, `fork`, `forkjoin`, `function`, `generate`, `genvar`, `global`, `highz0`, `highz1`, `if`, `iff`, `ifnone`, `ignore_bins`, `illegal_bins`, `implies`, `import`, `incdir`, `include`, `initial`, `inout`, `input`, `inside`, `instance`, `int`, `integer`, `interface`, `intersect`, `join`, `join_any`, `join_none`, `large`, `let`, `liblist`, `library`, `local`, `localparam`, `logic`, `longint`, `macromodule`, `matches`, `medium`, `modport`, `module`, `nand`, `negedge`, `new`, `nexttime`, `nmos`, `nor`, `noshowcancelled`, `not`, `notif0`, `notif1`, `null`, `or`, `output`, `package`, `packed`, `parameter`, `pmos`, `posedge`, `primitive`, `priority`, `program`, `property`, `protected`, `pull0`, `pull1`, `pulldown`, `pullup`, `pulsestyle_ondetect`, `pulsestyle_onevent`, `pure`, `rand`, `randc`, `randcase`, `randsequence`, `rcmos`, `real`, `realtime`, `ref`, `reg`, `reject_on`, `release`, `repeat`, `restrict`, `return`, `rnmos`, `rpmos`, `rtran`, `rtranif0`, `rtranif1`, `s_always`, `s_eventually`, `s_nexttime`, `s_until`, `s_until_with`, `scalared`, `sequence`, `shortint`, `shortreal`, `showcancelled`, `signed`, `small`, `solve`, `specify`, `specparam`, `static`, `string`, `strong`, `strong0`, `strong1`, `struct`, `super`, `supply0`, `supply1`, `sync_accept_on`, `sync_reject_on`, `table`, `tagged`, `task`, `this`, `throughout`, `time`, `timeprecision`, `timeunit`, `tran`, `tranif0`, `tranif1`, `tri`, `tri0`, `tri1`, `triand`, `trior`, `trireg`, `type`, `typedef`, `union`, `unique`, `unique0`, `unsigned`, `until`, `until_with`, `untyped`, `use`, `uwire`, `var`, `vectored`, `virtual`, `void`, `wait`, `wait_order`, `wand`, `weak`, `weak0`, `weak1`, `while`, `wildcard`, `wire`, `with`, `within`, `wor`, `xnor`, `xor`), Keyword, nil},
- {Words(``, `\b`, "`__FILE__", "`__LINE__", "`begin_keywords", "`celldefine", "`default_nettype", "`define", "`else", "`elsif", "`end_keywords", "`endcelldefine", "`endif", "`ifdef", "`ifndef", "`include", "`line", "`nounconnected_drive", "`pragma", "`resetall", "`timescale", "`unconnected_drive", "`undef", "`undefineall"), CommentPreproc, nil},
- {Words(``, `\b`, `$display`, `$displayb`, `$displayh`, `$displayo`, `$dumpall`, `$dumpfile`, `$dumpflush`, `$dumplimit`, `$dumpoff`, `$dumpon`, `$dumpports`, `$dumpportsall`, `$dumpportsflush`, `$dumpportslimit`, `$dumpportsoff`, `$dumpportson`, `$dumpvars`, `$fclose`, `$fdisplay`, `$fdisplayb`, `$fdisplayh`, `$fdisplayo`, `$feof`, `$ferror`, `$fflush`, `$fgetc`, `$fgets`, `$finish`, `$fmonitor`, `$fmonitorb`, `$fmonitorh`, `$fmonitoro`, `$fopen`, `$fread`, `$fscanf`, `$fseek`, `$fstrobe`, `$fstrobeb`, `$fstrobeh`, `$fstrobeo`, `$ftell`, `$fwrite`, `$fwriteb`, `$fwriteh`, `$fwriteo`, `$monitor`, `$monitorb`, `$monitorh`, `$monitoro`, `$monitoroff`, `$monitoron`, `$plusargs`, `$random`, `$readmemb`, `$readmemh`, `$rewind`, `$sformat`, `$sformatf`, `$sscanf`, `$strobe`, `$strobeb`, `$strobeh`, `$strobeo`, `$swrite`, `$swriteb`, `$swriteh`, `$swriteo`, `$test`, `$ungetc`, `$value$plusargs`, `$write`, `$writeb`, `$writeh`, `$writememb`, `$writememh`, `$writeo`), NameBuiltin, nil},
- {`(class)(\s+)`, ByGroups(Keyword, Text), Push("classname")},
- {Words(``, `\b`, `byte`, `shortint`, `int`, `longint`, `integer`, `time`, `bit`, `logic`, `reg`, `supply0`, `supply1`, `tri`, `triand`, `trior`, `tri0`, `tri1`, `trireg`, `uwire`, `wire`, `wand`, `woshortreal`, `real`, `realtime`), KeywordType, nil},
- {`[a-zA-Z_]\w*:(?!:)`, NameLabel, nil},
- {`\$?[a-zA-Z_]\w*`, Name, nil},
- },
- "classname": {
- {`[a-zA-Z_]\w*`, NameClass, Pop(1)},
- },
- "string": {
- {`"`, LiteralString, Pop(1)},
- {`\\([\\abfnrtv"\']|x[a-fA-F0-9]{2,4}|[0-7]{1,3})`, LiteralStringEscape, nil},
- {`[^\\"\n]+`, LiteralString, nil},
- {`\\\n`, LiteralString, nil},
- {`\\`, LiteralString, nil},
- },
- "macro": {
- {`[^/\n]+`, CommentPreproc, nil},
- {`/[*](.|\n)*?[*]/`, CommentMultiline, nil},
- {`//.*?\n`, CommentSingle, Pop(1)},
- {`/`, CommentPreproc, nil},
- {`(?<=\\)\n`, CommentPreproc, nil},
- {`\n`, CommentPreproc, Pop(1)},
- },
- "import": {
- {`[\w:]+\*?`, NameNamespace, Pop(1)},
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/t/tablegen.go b/vendor/github.com/alecthomas/chroma/lexers/t/tablegen.go
deleted file mode 100644
index ca05ed3..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/t/tablegen.go
+++ /dev/null
@@ -1,46 +0,0 @@
-package t
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-// TableGen lexer.
-var Tablegen = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "TableGen",
- Aliases: []string{"tablegen"},
- Filenames: []string{"*.td"},
- MimeTypes: []string{"text/x-tablegen"},
- },
- tablegenRules,
-))
-
-func tablegenRules() Rules {
- return Rules{
- "root": {
- Include("macro"),
- Include("whitespace"),
- {`c?"[^"]*?"`, LiteralString, nil},
- Include("keyword"),
- {`\$[_a-zA-Z][_\w]*`, NameVariable, nil},
- {`\d*[_a-zA-Z][_\w]*`, NameVariable, nil},
- {`\[\{[\w\W]*?\}\]`, LiteralString, nil},
- {`[+-]?\d+|0x[\da-fA-F]+|0b[01]+`, LiteralNumber, nil},
- {`[=<>{}\[\]()*.,!:;]`, Punctuation, nil},
- },
- "macro": {
- {`(#include\s+)("[^"]*")`, ByGroups(CommentPreproc, LiteralString), nil},
- {`^\s*#(ifdef|ifndef)\s+[_\w][_\w\d]*`, CommentPreproc, nil},
- {`^\s*#define\s+[_\w][_\w\d]*`, CommentPreproc, nil},
- {`^\s*#endif`, CommentPreproc, nil},
- },
- "whitespace": {
- {`(\n|\s)+`, Text, nil},
- {`//.*?\n`, Comment, nil},
- },
- "keyword": {
- {Words(``, `\b`, `bit`, `bits`, `class`, `code`, `dag`, `def`, `defm`, `field`, `foreach`, `in`, `int`, `let`, `list`, `multiclass`, `string`), Keyword, nil},
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/t/tasm.go b/vendor/github.com/alecthomas/chroma/lexers/t/tasm.go
deleted file mode 100644
index 0164a73..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/t/tasm.go
+++ /dev/null
@@ -1,65 +0,0 @@
-package t
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-// Tasm lexer.
-var Tasm = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "TASM",
- Aliases: []string{"tasm"},
- Filenames: []string{"*.asm", "*.ASM", "*.tasm"},
- MimeTypes: []string{"text/x-tasm"},
- CaseInsensitive: true,
- },
- tasmRules,
-))
-
-func tasmRules() Rules {
- return Rules{
- "root": {
- {`^\s*%`, CommentPreproc, Push("preproc")},
- Include("whitespace"),
- {`[@a-z$._?][\w$.?#@~]*:`, NameLabel, nil},
- {`BITS|USE16|USE32|SECTION|SEGMENT|ABSOLUTE|EXTERN|GLOBAL|ORG|ALIGN|STRUC|ENDSTRUC|ENDS|COMMON|CPU|GROUP|UPPERCASE|INCLUDE|EXPORT|LIBRARY|MODULE|PROC|ENDP|USES|ARG|DATASEG|UDATASEG|END|IDEAL|P386|MODEL|ASSUME|CODESEG|SIZE`, Keyword, Push("instruction-args")},
- {`([@a-z$._?][\w$.?#@~]*)(\s+)(db|dd|dw|T[A-Z][a-z]+)`, ByGroups(NameConstant, KeywordDeclaration, KeywordDeclaration), Push("instruction-args")},
- {`(?:res|d)[bwdqt]|times`, KeywordDeclaration, Push("instruction-args")},
- {`[@a-z$._?][\w$.?#@~]*`, NameFunction, Push("instruction-args")},
- {`[\r\n]+`, Text, nil},
- },
- "instruction-args": {
- {"\"(\\\\\"|[^\"\\n])*\"|'(\\\\'|[^'\\n])*'|`(\\\\`|[^`\\n])*`", LiteralString, nil},
- {`(?:0x[0-9a-f]+|$0[0-9a-f]*|[0-9]+[0-9a-f]*h)`, LiteralNumberHex, nil},
- {`[0-7]+q`, LiteralNumberOct, nil},
- {`[01]+b`, LiteralNumberBin, nil},
- {`[0-9]+\.e?[0-9]+`, LiteralNumberFloat, nil},
- {`[0-9]+`, LiteralNumberInteger, nil},
- Include("punctuation"),
- {`r[0-9][0-5]?[bwd]|[a-d][lh]|[er]?[a-d]x|[er]?[sb]p|[er]?[sd]i|[c-gs]s|st[0-7]|mm[0-7]|cr[0-4]|dr[0-367]|tr[3-7]`, NameBuiltin, nil},
- {`[@a-z$._?][\w$.?#@~]*`, NameVariable, nil},
- {`(\\\s*)(;.*)([\r\n])`, ByGroups(Text, CommentSingle, Text), nil},
- {`[\r\n]+`, Text, Pop(1)},
- Include("whitespace"),
- },
- "preproc": {
- {`[^;\n]+`, CommentPreproc, nil},
- {`;.*?\n`, CommentSingle, Pop(1)},
- {`\n`, CommentPreproc, Pop(1)},
- },
- "whitespace": {
- {`[\n\r]`, Text, nil},
- {`\\[\n\r]`, Text, nil},
- {`[ \t]+`, Text, nil},
- {`;.*`, CommentSingle, nil},
- },
- "punctuation": {
- {`[,():\[\]]+`, Punctuation, nil},
- {`[&|^<>+*=/%~-]+`, Operator, nil},
- {`[$]+`, KeywordConstant, nil},
- {`seg|wrt|strict`, OperatorWord, nil},
- {`byte|[dq]?word`, KeywordType, nil},
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/t/tcl.go b/vendor/github.com/alecthomas/chroma/lexers/t/tcl.go
deleted file mode 100644
index 5f7030a..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/t/tcl.go
+++ /dev/null
@@ -1,120 +0,0 @@
-package t
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-// Tcl lexer.
-var Tcl = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "Tcl",
- Aliases: []string{"tcl"},
- Filenames: []string{"*.tcl", "*.rvt"},
- MimeTypes: []string{"text/x-tcl", "text/x-script.tcl", "application/x-tcl"},
- },
- tclRules,
-))
-
-func tclRules() Rules {
- return Rules{
- "root": {
- Include("command"),
- Include("basic"),
- Include("data"),
- {`\}`, Keyword, nil},
- },
- "command": {
- {Words(`\b`, `\b`, `after`, `apply`, `array`, `break`, `catch`, `continue`, `elseif`, `else`, `error`, `eval`, `expr`, `for`, `foreach`, `global`, `if`, `namespace`, `proc`, `rename`, `return`, `set`, `switch`, `then`, `trace`, `unset`, `update`, `uplevel`, `upvar`, `variable`, `vwait`, `while`), Keyword, Push("params")},
- {Words(`\b`, `\b`, `append`, `bgerror`, `binary`, `cd`, `chan`, `clock`, `close`, `concat`, `dde`, `dict`, `encoding`, `eof`, `exec`, `exit`, `fblocked`, `fconfigure`, `fcopy`, `file`, `fileevent`, `flush`, `format`, `gets`, `glob`, `history`, `http`, `incr`, `info`, `interp`, `join`, `lappend`, `lassign`, `lindex`, `linsert`, `list`, `llength`, `load`, `loadTk`, `lrange`, `lrepeat`, `lreplace`, `lreverse`, `lsearch`, `lset`, `lsort`, `mathfunc`, `mathop`, `memory`, `msgcat`, `open`, `package`, `pid`, `pkg::create`, `pkg_mkIndex`, `platform`, `platform::shell`, `puts`, `pwd`, `re_syntax`, `read`, `refchan`, `regexp`, `registry`, `regsub`, `scan`, `seek`, `socket`, `source`, `split`, `string`, `subst`, `tell`, `time`, `tm`, `unknown`, `unload`), NameBuiltin, Push("params")},
- {`([\w.-]+)`, NameVariable, Push("params")},
- {`#`, Comment, Push("comment")},
- },
- "command-in-brace": {
- {Words(`\b`, `\b`, `after`, `apply`, `array`, `break`, `catch`, `continue`, `elseif`, `else`, `error`, `eval`, `expr`, `for`, `foreach`, `global`, `if`, `namespace`, `proc`, `rename`, `return`, `set`, `switch`, `then`, `trace`, `unset`, `update`, `uplevel`, `upvar`, `variable`, `vwait`, `while`), Keyword, Push("params-in-brace")},
- {Words(`\b`, `\b`, `append`, `bgerror`, `binary`, `cd`, `chan`, `clock`, `close`, `concat`, `dde`, `dict`, `encoding`, `eof`, `exec`, `exit`, `fblocked`, `fconfigure`, `fcopy`, `file`, `fileevent`, `flush`, `format`, `gets`, `glob`, `history`, `http`, `incr`, `info`, `interp`, `join`, `lappend`, `lassign`, `lindex`, `linsert`, `list`, `llength`, `load`, `loadTk`, `lrange`, `lrepeat`, `lreplace`, `lreverse`, `lsearch`, `lset`, `lsort`, `mathfunc`, `mathop`, `memory`, `msgcat`, `open`, `package`, `pid`, `pkg::create`, `pkg_mkIndex`, `platform`, `platform::shell`, `puts`, `pwd`, `re_syntax`, `read`, `refchan`, `regexp`, `registry`, `regsub`, `scan`, `seek`, `socket`, `source`, `split`, `string`, `subst`, `tell`, `time`, `tm`, `unknown`, `unload`), NameBuiltin, Push("params-in-brace")},
- {`([\w.-]+)`, NameVariable, Push("params-in-brace")},
- {`#`, Comment, Push("comment")},
- },
- "command-in-bracket": {
- {Words(`\b`, `\b`, `after`, `apply`, `array`, `break`, `catch`, `continue`, `elseif`, `else`, `error`, `eval`, `expr`, `for`, `foreach`, `global`, `if`, `namespace`, `proc`, `rename`, `return`, `set`, `switch`, `then`, `trace`, `unset`, `update`, `uplevel`, `upvar`, `variable`, `vwait`, `while`), Keyword, Push("params-in-bracket")},
- {Words(`\b`, `\b`, `append`, `bgerror`, `binary`, `cd`, `chan`, `clock`, `close`, `concat`, `dde`, `dict`, `encoding`, `eof`, `exec`, `exit`, `fblocked`, `fconfigure`, `fcopy`, `file`, `fileevent`, `flush`, `format`, `gets`, `glob`, `history`, `http`, `incr`, `info`, `interp`, `join`, `lappend`, `lassign`, `lindex`, `linsert`, `list`, `llength`, `load`, `loadTk`, `lrange`, `lrepeat`, `lreplace`, `lreverse`, `lsearch`, `lset`, `lsort`, `mathfunc`, `mathop`, `memory`, `msgcat`, `open`, `package`, `pid`, `pkg::create`, `pkg_mkIndex`, `platform`, `platform::shell`, `puts`, `pwd`, `re_syntax`, `read`, `refchan`, `regexp`, `registry`, `regsub`, `scan`, `seek`, `socket`, `source`, `split`, `string`, `subst`, `tell`, `time`, `tm`, `unknown`, `unload`), NameBuiltin, Push("params-in-bracket")},
- {`([\w.-]+)`, NameVariable, Push("params-in-bracket")},
- {`#`, Comment, Push("comment")},
- },
- "command-in-paren": {
- {Words(`\b`, `\b`, `after`, `apply`, `array`, `break`, `catch`, `continue`, `elseif`, `else`, `error`, `eval`, `expr`, `for`, `foreach`, `global`, `if`, `namespace`, `proc`, `rename`, `return`, `set`, `switch`, `then`, `trace`, `unset`, `update`, `uplevel`, `upvar`, `variable`, `vwait`, `while`), Keyword, Push("params-in-paren")},
- {Words(`\b`, `\b`, `append`, `bgerror`, `binary`, `cd`, `chan`, `clock`, `close`, `concat`, `dde`, `dict`, `encoding`, `eof`, `exec`, `exit`, `fblocked`, `fconfigure`, `fcopy`, `file`, `fileevent`, `flush`, `format`, `gets`, `glob`, `history`, `http`, `incr`, `info`, `interp`, `join`, `lappend`, `lassign`, `lindex`, `linsert`, `list`, `llength`, `load`, `loadTk`, `lrange`, `lrepeat`, `lreplace`, `lreverse`, `lsearch`, `lset`, `lsort`, `mathfunc`, `mathop`, `memory`, `msgcat`, `open`, `package`, `pid`, `pkg::create`, `pkg_mkIndex`, `platform`, `platform::shell`, `puts`, `pwd`, `re_syntax`, `read`, `refchan`, `regexp`, `registry`, `regsub`, `scan`, `seek`, `socket`, `source`, `split`, `string`, `subst`, `tell`, `time`, `tm`, `unknown`, `unload`), NameBuiltin, Push("params-in-paren")},
- {`([\w.-]+)`, NameVariable, Push("params-in-paren")},
- {`#`, Comment, Push("comment")},
- },
- "basic": {
- {`\(`, Keyword, Push("paren")},
- {`\[`, Keyword, Push("bracket")},
- {`\{`, Keyword, Push("brace")},
- {`"`, LiteralStringDouble, Push("string")},
- {`(eq|ne|in|ni)\b`, OperatorWord, nil},
- {`!=|==|<<|>>|<=|>=|&&|\|\||\*\*|[-+~!*/%<>&^|?:]`, Operator, nil},
- },
- "data": {
- {`\s+`, Text, nil},
- {`0x[a-fA-F0-9]+`, LiteralNumberHex, nil},
- {`0[0-7]+`, LiteralNumberOct, nil},
- {`\d+\.\d+`, LiteralNumberFloat, nil},
- {`\d+`, LiteralNumberInteger, nil},
- {`\$([\w.:-]+)`, NameVariable, nil},
- {`([\w.:-]+)`, Text, nil},
- },
- "params": {
- {`;`, Keyword, Pop(1)},
- {`\n`, Text, Pop(1)},
- {`(else|elseif|then)\b`, Keyword, nil},
- Include("basic"),
- Include("data"),
- },
- "params-in-brace": {
- {`\}`, Keyword, Push("#pop", "#pop")},
- Include("params"),
- },
- "params-in-paren": {
- {`\)`, Keyword, Push("#pop", "#pop")},
- Include("params"),
- },
- "params-in-bracket": {
- {`\]`, Keyword, Push("#pop", "#pop")},
- Include("params"),
- },
- "string": {
- {`\[`, LiteralStringDouble, Push("string-square")},
- {`(?s)(\\\\|\\[0-7]+|\\.|[^"\\])`, LiteralStringDouble, nil},
- {`"`, LiteralStringDouble, Pop(1)},
- },
- "string-square": {
- {`\[`, LiteralStringDouble, Push("string-square")},
- {`(?s)(\\\\|\\[0-7]+|\\.|\\\n|[^\]\\])`, LiteralStringDouble, nil},
- {`\]`, LiteralStringDouble, Pop(1)},
- },
- "brace": {
- {`\}`, Keyword, Pop(1)},
- Include("command-in-brace"),
- Include("basic"),
- Include("data"),
- },
- "paren": {
- {`\)`, Keyword, Pop(1)},
- Include("command-in-paren"),
- Include("basic"),
- Include("data"),
- },
- "bracket": {
- {`\]`, Keyword, Pop(1)},
- Include("command-in-bracket"),
- Include("basic"),
- Include("data"),
- },
- "comment": {
- {`.*[^\\]\n`, Comment, Pop(1)},
- {`.*\\\n`, Comment, nil},
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/t/tcsh.go b/vendor/github.com/alecthomas/chroma/lexers/t/tcsh.go
deleted file mode 100644
index fec0cb2..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/t/tcsh.go
+++ /dev/null
@@ -1,63 +0,0 @@
-package t
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-// Tcsh lexer.
-var Tcsh = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "Tcsh",
- Aliases: []string{"tcsh", "csh"},
- Filenames: []string{"*.tcsh", "*.csh"},
- MimeTypes: []string{"application/x-csh"},
- },
- tcshRules,
-))
-
-func tcshRules() Rules {
- return Rules{
- "root": {
- Include("basic"),
- {`\$\(`, Keyword, Push("paren")},
- {`\$\{#?`, Keyword, Push("curly")},
- {"`", LiteralStringBacktick, Push("backticks")},
- Include("data"),
- },
- "basic": {
- {`\b(if|endif|else|while|then|foreach|case|default|continue|goto|breaksw|end|switch|endsw)\s*\b`, Keyword, nil},
- {`\b(alias|alloc|bg|bindkey|break|builtins|bye|caller|cd|chdir|complete|dirs|echo|echotc|eval|exec|exit|fg|filetest|getxvers|glob|getspath|hashstat|history|hup|inlib|jobs|kill|limit|log|login|logout|ls-F|migrate|newgrp|nice|nohup|notify|onintr|popd|printenv|pushd|rehash|repeat|rootnode|popd|pushd|set|shift|sched|setenv|setpath|settc|setty|setxvers|shift|source|stop|suspend|source|suspend|telltc|time|umask|unalias|uncomplete|unhash|universe|unlimit|unset|unsetenv|ver|wait|warp|watchlog|where|which)\s*\b`, NameBuiltin, nil},
- {`#.*`, Comment, nil},
- {`\\[\w\W]`, LiteralStringEscape, nil},
- {`(\b\w+)(\s*)(=)`, ByGroups(NameVariable, Text, Operator), nil},
- {`[\[\]{}()=]+`, Operator, nil},
- {`<<\s*(\'?)\\?(\w+)[\w\W]+?\2`, LiteralString, nil},
- {`;`, Punctuation, nil},
- },
- "data": {
- {`(?s)"(\\\\|\\[0-7]+|\\.|[^"\\])*"`, LiteralStringDouble, nil},
- {`(?s)'(\\\\|\\[0-7]+|\\.|[^'\\])*'`, LiteralStringSingle, nil},
- {`\s+`, Text, nil},
- {"[^=\\s\\[\\]{}()$\"\\'`\\\\;#]+", Text, nil},
- {`\d+(?= |\Z)`, LiteralNumber, nil},
- {`\$#?(\w+|.)`, NameVariable, nil},
- },
- "curly": {
- {`\}`, Keyword, Pop(1)},
- {`:-`, Keyword, nil},
- {`\w+`, NameVariable, nil},
- {"[^}:\"\\'`$]+", Punctuation, nil},
- {`:`, Punctuation, nil},
- Include("root"),
- },
- "paren": {
- {`\)`, Keyword, Pop(1)},
- Include("root"),
- },
- "backticks": {
- {"`", LiteralStringBacktick, Pop(1)},
- Include("root"),
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/t/termcap.go b/vendor/github.com/alecthomas/chroma/lexers/t/termcap.go
deleted file mode 100644
index 8db20b5..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/t/termcap.go
+++ /dev/null
@@ -1,46 +0,0 @@
-package t
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-// Termcap lexer.
-var Termcap = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "Termcap",
- Aliases: []string{"termcap"},
- Filenames: []string{"termcap", "termcap.src"},
- MimeTypes: []string{},
- },
- termcapRules,
-))
-
-func termcapRules() Rules {
- return Rules{
- "root": {
- {`^#.*$`, Comment, nil},
- {`^[^\s#:|]+`, NameTag, Push("names")},
- },
- "names": {
- {`\n`, Text, Pop(1)},
- {`:`, Punctuation, Push("defs")},
- {`\|`, Punctuation, nil},
- {`[^:|]+`, NameAttribute, nil},
- },
- "defs": {
- {`\\\n[ \t]*`, Text, nil},
- {`\n[ \t]*`, Text, Pop(2)},
- {`(#)([0-9]+)`, ByGroups(Operator, LiteralNumber), nil},
- {`=`, Operator, Push("data")},
- {`:`, Punctuation, nil},
- {`[^\s:=#]+`, NameClass, nil},
- },
- "data": {
- {`\\072`, Literal, nil},
- {`:`, Punctuation, Pop(1)},
- {`[^:\\]+`, Literal, nil},
- {`.`, Literal, nil},
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/t/terminfo.go b/vendor/github.com/alecthomas/chroma/lexers/t/terminfo.go
deleted file mode 100644
index b3f2735..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/t/terminfo.go
+++ /dev/null
@@ -1,46 +0,0 @@
-package t
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-// Terminfo lexer.
-var Terminfo = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "Terminfo",
- Aliases: []string{"terminfo"},
- Filenames: []string{"terminfo", "terminfo.src"},
- MimeTypes: []string{},
- },
- terminfoRules,
-))
-
-func terminfoRules() Rules {
- return Rules{
- "root": {
- {`^#.*$`, Comment, nil},
- {`^[^\s#,|]+`, NameTag, Push("names")},
- },
- "names": {
- {`\n`, Text, Pop(1)},
- {`(,)([ \t]*)`, ByGroups(Punctuation, Text), Push("defs")},
- {`\|`, Punctuation, nil},
- {`[^,|]+`, NameAttribute, nil},
- },
- "defs": {
- {`\n[ \t]+`, Text, nil},
- {`\n`, Text, Pop(2)},
- {`(#)([0-9]+)`, ByGroups(Operator, LiteralNumber), nil},
- {`=`, Operator, Push("data")},
- {`(,)([ \t]*)`, ByGroups(Punctuation, Text), nil},
- {`[^\s,=#]+`, NameClass, nil},
- },
- "data": {
- {`\\[,\\]`, Literal, nil},
- {`(,)([ \t]*)`, ByGroups(Punctuation, Text), Pop(1)},
- {`[^\\,]+`, Literal, nil},
- {`.`, Literal, nil},
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/t/terraform.go b/vendor/github.com/alecthomas/chroma/lexers/t/terraform.go
deleted file mode 100644
index c9c0905..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/t/terraform.go
+++ /dev/null
@@ -1,64 +0,0 @@
-package t
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-// Terraform lexer.
-var Terraform = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "Terraform",
- Aliases: []string{"terraform", "tf"},
- Filenames: []string{"*.tf"},
- MimeTypes: []string{"application/x-tf", "application/x-terraform"},
- },
- terraformRules,
-))
-
-func terraformRules() Rules {
- return Rules{
- "root": {
- {`[\[\](),.{}]`, Punctuation, nil},
- {`-?[0-9]+`, LiteralNumber, nil},
- {`=>`, Punctuation, nil},
- {Words(``, `\b`, `true`, `false`), KeywordConstant, nil},
- {`/(?s)\*(((?!\*/).)*)\*/`, CommentMultiline, nil},
- {`\s*(#|//).*\n`, CommentSingle, nil},
- {`([a-zA-Z]\w*)(\s*)(=(?!>))`, ByGroups(NameAttribute, Text, Text), nil},
- {Words(`^\s*`, `\b`, `variable`, `data`, `resource`, `provider`, `provisioner`, `module`, `output`), KeywordReserved, nil},
- {Words(``, `\b`, `for`, `in`), Keyword, nil},
- {Words(``, ``, `count`, `data`, `var`, `module`, `each`), NameBuiltin, nil},
- {Words(``, `\b`, `abs`, `ceil`, `floor`, `log`, `max`, `min`, `parseint`, `pow`, `signum`), NameBuiltin, nil},
- {Words(``, `\b`, `chomp`, `format`, `formatlist`, `indent`, `join`, `lower`, `regex`, `regexall`, `replace`, `split`, `strrev`, `substr`, `title`, `trim`, `trimprefix`, `trimsuffix`, `trimspace`, `upper`), NameBuiltin, nil},
- {Words(`[^.]`, `\b`, `chunklist`, `coalesce`, `coalescelist`, `compact`, `concat`, `contains`, `distinct`, `element`, `flatten`, `index`, `keys`, `length`, `list`, `lookup`, `map`, `matchkeys`, `merge`, `range`, `reverse`, `setintersection`, `setproduct`, `setsubtract`, `setunion`, `slice`, `sort`, `transpose`, `values`, `zipmap`), NameBuiltin, nil},
- {Words(`[^.]`, `\b`, `base64decode`, `base64encode`, `base64gzip`, `csvdecode`, `jsondecode`, `jsonencode`, `urlencode`, `yamldecode`, `yamlencode`), NameBuiltin, nil},
- {Words(``, `\b`, `abspath`, `dirname`, `pathexpand`, `basename`, `file`, `fileexists`, `fileset`, `filebase64`, `templatefile`), NameBuiltin, nil},
- {Words(``, `\b`, `formatdate`, `timeadd`, `timestamp`), NameBuiltin, nil},
- {Words(``, `\b`, `base64sha256`, `base64sha512`, `bcrypt`, `filebase64sha256`, `filebase64sha512`, `filemd5`, `filesha1`, `filesha256`, `filesha512`, `md5`, `rsadecrypt`, `sha1`, `sha256`, `sha512`, `uuid`, `uuidv5`), NameBuiltin, nil},
- {Words(``, `\b`, `cidrhost`, `cidrnetmask`, `cidrsubnet`), NameBuiltin, nil},
- {Words(``, `\b`, `can`, `tobool`, `tolist`, `tomap`, `tonumber`, `toset`, `tostring`, `try`), NameBuiltin, nil},
- {`=(?!>)|\+|-|\*|\/|:|!|%|>|<(?!<)|>=|<=|==|!=|&&|\||\?`, Operator, nil},
- {`\n|\s+|\\\n`, Text, nil},
- {`[a-zA-Z]\w*`, NameOther, nil},
- {`"`, LiteralStringDouble, Push("string")},
- {`(?s)(<<-?)(\w+)(\n\s*(?:(?!\2).)*\s*\n\s*)(\2)`, ByGroups(Operator, Operator, String, Operator), nil},
- },
- "declaration": {
- {`(\s*)("(?:\\\\|\\"|[^"])*")(\s*)`, ByGroups(Text, NameVariable, Text), nil},
- {`\{`, Punctuation, Pop(1)},
- },
- "string": {
- {`"`, LiteralStringDouble, Pop(1)},
- {`\\\\`, LiteralStringDouble, nil},
- {`\\\\"`, LiteralStringDouble, nil},
- {`\$\{`, LiteralStringInterpol, Push("interp-inside")},
- {`\$`, LiteralStringDouble, nil},
- {`[^"\\\\$]+`, LiteralStringDouble, nil},
- },
- "interp-inside": {
- {`\}`, LiteralStringInterpol, Pop(1)},
- Include("root"),
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/t/tex.go b/vendor/github.com/alecthomas/chroma/lexers/t/tex.go
deleted file mode 100644
index f9413b3..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/t/tex.go
+++ /dev/null
@@ -1,60 +0,0 @@
-package t
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-// Tex lexer.
-var TeX = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "TeX",
- Aliases: []string{"tex", "latex"},
- Filenames: []string{"*.tex", "*.aux", "*.toc"},
- MimeTypes: []string{"text/x-tex", "text/x-latex"},
- },
- texRules,
-))
-
-func texRules() Rules {
- return Rules{
- "general": {
- {`%.*?\n`, Comment, nil},
- {`[{}]`, NameBuiltin, nil},
- {`[&_^]`, NameBuiltin, nil},
- },
- "root": {
- {`\\\[`, LiteralStringBacktick, Push("displaymath")},
- {`\\\(`, LiteralString, Push("inlinemath")},
- {`\$\$`, LiteralStringBacktick, Push("displaymath")},
- {`\$`, LiteralString, Push("inlinemath")},
- {`\\([a-zA-Z]+|.)`, Keyword, Push("command")},
- {`\\$`, Keyword, nil},
- Include("general"),
- {`[^\\$%&_^{}]+`, Text, nil},
- },
- "math": {
- {`\\([a-zA-Z]+|.)`, NameVariable, nil},
- Include("general"),
- {`[0-9]+`, LiteralNumber, nil},
- {`[-=!+*/()\[\]]`, Operator, nil},
- {`[^=!+*/()\[\]\\$%&_^{}0-9-]+`, NameBuiltin, nil},
- },
- "inlinemath": {
- {`\\\)`, LiteralString, Pop(1)},
- {`\$`, LiteralString, Pop(1)},
- Include("math"),
- },
- "displaymath": {
- {`\\\]`, LiteralString, Pop(1)},
- {`\$\$`, LiteralString, Pop(1)},
- {`\$`, NameBuiltin, nil},
- Include("math"),
- },
- "command": {
- {`\[.*?\]`, NameAttribute, nil},
- {`\*`, Keyword, nil},
- Default(Pop(1)),
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/t/thrift.go b/vendor/github.com/alecthomas/chroma/lexers/t/thrift.go
deleted file mode 100644
index 61669d7..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/t/thrift.go
+++ /dev/null
@@ -1,77 +0,0 @@
-package t
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-// Thrift lexer.
-var Thrift = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "Thrift",
- Aliases: []string{"thrift"},
- Filenames: []string{"*.thrift"},
- MimeTypes: []string{"application/x-thrift"},
- },
- thriftRules,
-))
-
-func thriftRules() Rules {
- return Rules{
- "root": {
- Include("whitespace"),
- Include("comments"),
- {`"`, LiteralStringDouble, Combined("stringescape", "dqs")},
- {`\'`, LiteralStringSingle, Combined("stringescape", "sqs")},
- {`(namespace)(\s+)`, ByGroups(KeywordNamespace, TextWhitespace), Push("namespace")},
- {`(enum|union|struct|service|exception)(\s+)`, ByGroups(KeywordDeclaration, TextWhitespace), Push("class")},
- {`((?:(?:[^\W\d]|\$)[\w.\[\]$<>]*\s+)+?)((?:[^\W\d]|\$)[\w$]*)(\s*)(\()`, ByGroups(UsingSelf("root"), NameFunction, Text, Operator), nil},
- Include("keywords"),
- Include("numbers"),
- {`[&=]`, Operator, nil},
- {`[:;,{}()<>\[\]]`, Punctuation, nil},
- {`[a-zA-Z_](\.\w|\w)*`, Name, nil},
- },
- "whitespace": {
- {`\n`, TextWhitespace, nil},
- {`\s+`, TextWhitespace, nil},
- },
- "comments": {
- {`#.*$`, Comment, nil},
- {`//.*?\n`, Comment, nil},
- {`/\*[\w\W]*?\*/`, CommentMultiline, nil},
- },
- "stringescape": {
- {`\\([\\nrt"\'])`, LiteralStringEscape, nil},
- },
- "dqs": {
- {`"`, LiteralStringDouble, Pop(1)},
- {`[^\\"\n]+`, LiteralStringDouble, nil},
- },
- "sqs": {
- {`'`, LiteralStringSingle, Pop(1)},
- {`[^\\\'\n]+`, LiteralStringSingle, nil},
- },
- "namespace": {
- {`[a-z*](\.\w|\w)*`, NameNamespace, Pop(1)},
- Default(Pop(1)),
- },
- "class": {
- {`[a-zA-Z_]\w*`, NameClass, Pop(1)},
- Default(Pop(1)),
- },
- "keywords": {
- {`(async|oneway|extends|throws|required|optional)\b`, Keyword, nil},
- {`(true|false)\b`, KeywordConstant, nil},
- {`(const|typedef)\b`, KeywordDeclaration, nil},
- {Words(``, `\b`, `cpp_namespace`, `cpp_include`, `cpp_type`, `java_package`, `cocoa_prefix`, `csharp_namespace`, `delphi_namespace`, `php_namespace`, `py_module`, `perl_package`, `ruby_namespace`, `smalltalk_category`, `smalltalk_prefix`, `xsd_all`, `xsd_optional`, `xsd_nillable`, `xsd_namespace`, `xsd_attrs`, `include`), KeywordNamespace, nil},
- {Words(``, `\b`, `void`, `bool`, `byte`, `i16`, `i32`, `i64`, `double`, `string`, `binary`, `map`, `list`, `set`, `slist`, `senum`), KeywordType, nil},
- {Words(`\b`, `\b`, `BEGIN`, `END`, `__CLASS__`, `__DIR__`, `__FILE__`, `__FUNCTION__`, `__LINE__`, `__METHOD__`, `__NAMESPACE__`, `abstract`, `alias`, `and`, `args`, `as`, `assert`, `begin`, `break`, `case`, `catch`, `class`, `clone`, `continue`, `declare`, `def`, `default`, `del`, `delete`, `do`, `dynamic`, `elif`, `else`, `elseif`, `elsif`, `end`, `enddeclare`, `endfor`, `endforeach`, `endif`, `endswitch`, `endwhile`, `ensure`, `except`, `exec`, `finally`, `float`, `for`, `foreach`, `function`, `global`, `goto`, `if`, `implements`, `import`, `in`, `inline`, `instanceof`, `interface`, `is`, `lambda`, `module`, `native`, `new`, `next`, `nil`, `not`, `or`, `pass`, `public`, `print`, `private`, `protected`, `raise`, `redo`, `rescue`, `retry`, `register`, `return`, `self`, `sizeof`, `static`, `super`, `switch`, `synchronized`, `then`, `this`, `throw`, `transient`, `try`, `undef`, `unless`, `unsigned`, `until`, `use`, `var`, `virtual`, `volatile`, `when`, `while`, `with`, `xor`, `yield`), KeywordReserved, nil},
- },
- "numbers": {
- {`[+-]?(\d+\.\d+([eE][+-]?\d+)?|\.?\d+[eE][+-]?\d+)`, LiteralNumberFloat, nil},
- {`[+-]?0x[0-9A-Fa-f]+`, LiteralNumberHex, nil},
- {`[+-]?[0-9]+`, LiteralNumberInteger, nil},
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/t/toml.go b/vendor/github.com/alecthomas/chroma/lexers/t/toml.go
deleted file mode 100644
index 18a44c5..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/t/toml.go
+++ /dev/null
@@ -1,33 +0,0 @@
-package t
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-var TOML = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "TOML",
- Aliases: []string{"toml"},
- Filenames: []string{"*.toml"},
- MimeTypes: []string{"text/x-toml"},
- },
- tomlRules,
-))
-
-func tomlRules() Rules {
- return Rules{
- "root": {
- {`\s+`, Text, nil},
- {`#.*`, Comment, nil},
- {Words(``, `\b`, `true`, `false`), KeywordConstant, nil},
- {`\d\d\d\d-\d{2}-\d{2}T\d{2}:\d{2}:\d{2}(\.\d\+)?(Z|[+-]\d{2}:\d{2})`, LiteralDate, nil},
- {`[+-]?[0-9](_?\d)*\.\d+`, LiteralNumberFloat, nil},
- {`[+-]?[0-9](_?\d)*`, LiteralNumberInteger, nil},
- {`"(\\\\|\\"|[^"])*"`, StringDouble, nil},
- {`'(\\\\|\\'|[^'])*'`, StringSingle, nil},
- {`[.,=\[\]{}]`, Punctuation, nil},
- {`[A-Za-z0-9_-]+`, NameOther, nil},
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/t/tradingview.go b/vendor/github.com/alecthomas/chroma/lexers/t/tradingview.go
deleted file mode 100644
index bf303c6..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/t/tradingview.go
+++ /dev/null
@@ -1,44 +0,0 @@
-package t
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-// TradingView lexer
-var TradingView = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "TradingView",
- Aliases: []string{"tradingview", "tv"},
- Filenames: []string{"*.tv"},
- MimeTypes: []string{"text/x-tradingview"},
- DotAll: true,
- EnsureNL: true,
- },
- tradingViewRules,
-))
-
-func tradingViewRules() Rules {
- return Rules{
- "root": {
- {`[^\S\n]+|\n|[()]`, Text, nil},
- {`(//.*?)(\n)`, ByGroups(CommentSingle, Text), nil},
- {`>=|<=|==|!=|>|<|\?|-|\+|\*|\/|%|\[|\]`, Operator, nil},
- {`[:,.]`, Punctuation, nil},
- {`=`, KeywordPseudo, nil},
- {`"(\\\\|\\"|[^"\n])*["\n]`, LiteralString, nil},
- {`'\\.'|'[^\\]'`, LiteralString, nil},
- {`[0-9](\.[0-9]*)?([eE][+-][0-9]+)?`, LiteralNumber, nil},
- {`#[a-fA-F0-9]{8}|#[a-fA-F0-9]{6}|#[a-fA-F0-9]{3}`, LiteralStringOther, nil},
- {`(abs|acos|alertcondition|alma|asin|atan|atr|avg|barcolor|barssince|bgcolor|cci|ceil|change|cog|color\.new|correlation|cos|crossover|crossunder|cum|dev|ema|exp|falling|fill|fixnan|floor|heikinashi|highest|highestbars|hline|iff|kagi|label\.(delete|get_text|get_x|get_y|new|set_color|set_size|set_style|set_text|set_textcolor|set_x|set_xloc|set_xy|set_y|set_yloc)|line\.(new|delete|get_x1|get_x2|get_y1|get_y2|set_color|set_width|set_style|set_extend|set_xy1|set_xy2|set_x1|set_x2|set_y1|set_y2|set_xloc)|linebreak|linreg|log|log10|lowest|lowestbars|macd|max|max_bars_back|min|mom|nz|percentile_(linear_interpolation|nearest_rank)|percentrank|pivothigh|pivotlow|plot|plotarrow|plotbar|plotcandle|plotchar|plotshape|pointfigure|pow|renko|rising|rma|roc|round|rsi|sar|security|sign|sin|sma|sqrt|stdev|stoch|study|sum|swma|tan|timestamp|tostring|tsi|valuewhen|variance|vwma|wma|strategy\.(cancel|cancel_all|close|close_all|entry|exit|order|risk\.(allow_entry_in|max_cons_loss_days|max_drawdown|max_intraday_filled_orders|max_intraday_loss|max_position_size)))\b`, NameFunction, nil},
- {`\b(bool|color|cross|dayofmonth|dayofweek|float|hour|input|int|label|line|minute|month|na|offset|second|strategy|string|tickerid|time|tr|vwap|weekofyear|year)(\()`, ByGroups(NameFunction, Text), nil}, // functions that can also be variable
- {`(accdist|adjustment\.(dividends|none|splits)|aqua|area|areabr|bar_index|black|blue|bool|circles|close|columns|currency\.(AUD|CAD|CHF|EUR|GBP|HKD|JPY|NOK|NONE|NZD|RUB|SEK|SGD|TRY|USD|ZAR)|color\.(aqua|black|blue|fuchsia|gray|green|lime|maroon|navy|olive|orange|purple|red|silver|teal|white|yellow)|dashed|dotted|dayofweek\.(monday|tuesday|wednesday|thursday|friday|saturday|sunday)|extend\.(both|left|right|none)|float|format\.(inherit|price|volume)|friday|fuchsia|gray|green|high|histogram|hl2|hlc3|hline\.style_(dotted|solid|dashed)|input\.(bool|float|integer|resolution|session|source|string|symbol)|integer|interval|isdaily|isdwm|isintraday|ismonthly|isweekly|label\.style_(arrowdown|arrowup|circle|cross|diamond|flag|labeldown|labelup|none|square|triangledown|triangleup|xcross)|lime|line\.style_(dashed|dotted|solid|arrow_both|arrow_left|arrow_right)|linebr|location\.(abovebar|absolute|belowbar|bottom|top)|low|maroon|monday|n|navy|ohlc4|olive|open|orange|period|plot\.style_(area|areabr|circles|columns|cross|histogram|line|linebr|stepline)|purple|red|resolution|saturday|scale\.(left|none|right)|session|session\.(extended|regular)|silver|size\.(auto|huge|large|normal|small|tiny)|solid|source|stepline|string|sunday|symbol|syminfo\.(mintick|pointvalue|prefix|root|session|ticker|tickerid|timezone)|teal|thursday|ticker|timeframe\.(isdaily|isdwm|isintraday|ismonthly|isweekly|multiplier|period)|timenow|tuesday|volume|wednesday|white|yellow|strategy\.(cash|closedtrades|commission\.(cash_per_contract|cash_per_order|percent)|direction\.(all|long|short)|equity|eventrades|fixed|grossloss|grossprofit|initial_capital|long|losstrades|max_contracts_held_(all|long|short)|max_drawdown|netprofit|oca\.(cancel|none|reduce)|openprofit|opentrades|percent_of_equity|position_avg_price|position_entry_name|position_size|short|wintrades)|shape\.(arrowdown|arrowup|circle|cross|diamond|flag|labeldown|labelup|square|triangledown|triangleup|xcross)|barstate\.is(first|history|last|new|realtime)|barmerge\.(gaps_on|gaps_off|lookahead_on|lookahead_off)|xloc\.bar_(index|time)|yloc\.(abovebar|belowbar|price))\b`, NameVariable, nil},
- {`(cross|dayofmonth|dayofweek|hour|minute|month|na|second|tickerid|time|tr|vwap|weekofyear|year)(\b[^\(])`, ByGroups(NameVariable, Text), nil}, // variables that can also be function
- {`(int|float|bool|color|string|label|line)(\b[^\(=.])`, ByGroups(KeywordType, Text), nil}, // types that can also be a function
- {`(var)\b`, KeywordType, nil},
- {`(true|false)\b`, KeywordConstant, nil},
- {`(and|or|not|if|else|for|to)\b`, OperatorWord, nil},
- {`@?[_a-zA-Z]\w*`, Text, nil},
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/t/transactsql.go b/vendor/github.com/alecthomas/chroma/lexers/t/transactsql.go
deleted file mode 100644
index e5beb06..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/t/transactsql.go
+++ /dev/null
@@ -1,64 +0,0 @@
-package t
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-// TransactSQL lexer.
-var TransactSQL = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "Transact-SQL",
- Aliases: []string{"tsql", "t-sql"},
- MimeTypes: []string{"text/x-tsql"},
- NotMultiline: true,
- CaseInsensitive: true,
- },
- transactSQLRules,
-))
-
-func transactSQLRules() Rules {
- return Rules{
- "root": {
- {`\s+`, TextWhitespace, nil},
- {`--(?m).*?$\n?`, CommentSingle, nil},
- {`/\*`, CommentMultiline, Push("multiline-comments")},
- {`'`, LiteralStringSingle, Push("string")},
- {`"`, LiteralStringName, Push("quoted-ident")},
- {Words(``, ``, `!<`, `!=`, `!>`, `<`, `<=`, `<>`, `=`, `>`, `>=`, `+`, `+=`, `-`, `-=`, `*`, `*=`, `/`, `/=`, `%`, `%=`, `&`, `&=`, `|`, `|=`, `^`, `^=`, `~`, `::`), Operator, nil},
- {Words(``, `\b`, `all`, `and`, `any`, `between`, `except`, `exists`, `in`, `intersect`, `like`, `not`, `or`, `some`, `union`), OperatorWord, nil},
- {Words(``, `\b`, `bigint`, `binary`, `bit`, `char`, `cursor`, `date`, `datetime`, `datetime2`, `datetimeoffset`, `decimal`, `float`, `hierarchyid`, `image`, `int`, `money`, `nchar`, `ntext`, `numeric`, `nvarchar`, `real`, `smalldatetime`, `smallint`, `smallmoney`, `sql_variant`, `table`, `text`, `time`, `timestamp`, `tinyint`, `uniqueidentifier`, `varbinary`, `varchar`, `xml`), NameClass, nil},
- {Words(``, `\b`, `$partition`, `abs`, `acos`, `app_name`, `applock_mode`, `applock_test`, `ascii`, `asin`, `assemblyproperty`, `atan`, `atn2`, `avg`, `binary_checksum`, `cast`, `ceiling`, `certencoded`, `certprivatekey`, `char`, `charindex`, `checksum`, `checksum_agg`, `choose`, `col_length`, `col_name`, `columnproperty`, `compress`, `concat`, `connectionproperty`, `context_info`, `convert`, `cos`, `cot`, `count`, `count_big`, `current_request_id`, `current_timestamp`, `current_transaction_id`, `current_user`, `cursor_status`, `database_principal_id`, `databasepropertyex`, `dateadd`, `datediff`, `datediff_big`, `datefromparts`, `datename`, `datepart`, `datetime2fromparts`, `datetimefromparts`, `datetimeoffsetfromparts`, `day`, `db_id`, `db_name`, `decompress`, `degrees`, `dense_rank`, `difference`, `eomonth`, `error_line`, `error_message`, `error_number`, `error_procedure`, `error_severity`, `error_state`, `exp`, `file_id`, `file_idex`, `file_name`, `filegroup_id`, `filegroup_name`, `filegroupproperty`, `fileproperty`, `floor`, `format`, `formatmessage`, `fulltextcatalogproperty`, `fulltextserviceproperty`, `get_filestream_transaction_context`, `getansinull`, `getdate`, `getutcdate`, `grouping`, `grouping_id`, `has_perms_by_name`, `host_id`, `host_name`, `iif`, `index_col`, `indexkey_property`, `indexproperty`, `is_member`, `is_rolemember`, `is_srvrolemember`, `isdate`, `isjson`, `isnull`, `isnumeric`, `json_modify`, `json_query`, `json_value`, `left`, `len`, `log`, `log10`, `lower`, `ltrim`, `max`, `min`, `min_active_rowversion`, `month`, `nchar`, `newid`, `newsequentialid`, `ntile`, `object_definition`, `object_id`, `object_name`, `object_schema_name`, `objectproperty`, `objectpropertyex`, `opendatasource`, `openjson`, `openquery`, `openrowset`, `openxml`, `original_db_name`, `original_login`, `parse`, `parsename`, `patindex`, `permissions`, `pi`, `power`, `pwdcompare`, `pwdencrypt`, `quotename`, `radians`, `rand`, `rank`, `replace`, `replicate`, `reverse`, `right`, `round`, `row_number`, `rowcount_big`, `rtrim`, `schema_id`, `schema_name`, `scope_identity`, `serverproperty`, `session_context`, `session_user`, `sign`, `sin`, `smalldatetimefromparts`, `soundex`, `sp_helplanguage`, `space`, `sqrt`, `square`, `stats_date`, `stdev`, `stdevp`, `str`, `string_escape`, `string_split`, `stuff`, `substring`, `sum`, `suser_id`, `suser_name`, `suser_sid`, `suser_sname`, `switchoffset`, `sysdatetime`, `sysdatetimeoffset`, `system_user`, `sysutcdatetime`, `tan`, `textptr`, `textvalid`, `timefromparts`, `todatetimeoffset`, `try_cast`, `try_convert`, `try_parse`, `type_id`, `type_name`, `typeproperty`, `unicode`, `upper`, `user_id`, `user_name`, `var`, `varp`, `xact_state`, `year`), NameFunction, nil},
- {`(goto)(\s+)(\w+\b)`, ByGroups(Keyword, TextWhitespace, NameLabel), nil},
- {Words(``, `\b`, `absolute`, `action`, `ada`, `add`, `admin`, `after`, `aggregate`, `alias`, `all`, `allocate`, `alter`, `and`, `any`, `are`, `array`, `as`, `asc`, `asensitive`, `assertion`, `asymmetric`, `at`, `atomic`, `authorization`, `avg`, `backup`, `before`, `begin`, `between`, `binary`, `bit`, `bit_length`, `blob`, `boolean`, `both`, `breadth`, `break`, `browse`, `bulk`, `by`, `call`, `called`, `cardinality`, `cascade`, `cascaded`, `case`, `cast`, `catalog`, `catch`, `char`, `char_length`, `character`, `character_length`, `check`, `checkpoint`, `class`, `clob`, `close`, `clustered`, `coalesce`, `collate`, `collation`, `collect`, `column`, `commit`, `completion`, `compute`, `condition`, `connect`, `connection`, `constraint`, `constraints`, `constructor`, `contains`, `containstable`, `continue`, `convert`, `corr`, `corresponding`, `count`, `covar_pop`, `covar_samp`, `create`, `cross`, `cube`, `cume_dist`, `current`, `current_catalog`, `current_date`, `current_default_transform_group`, `current_path`, `current_role`, `current_schema`, `current_time`, `current_timestamp`, `current_transform_group_for_type`, `current_user`, `cursor`, `cycle`, `data`, `database`, `date`, `day`, `dbcc`, `deallocate`, `dec`, `decimal`, `declare`, `default`, `deferrable`, `deferred`, `delete`, `deny`, `depth`, `deref`, `desc`, `describe`, `descriptor`, `destroy`, `destructor`, `deterministic`, `diagnostics`, `dictionary`, `disconnect`, `disk`, `distinct`, `distributed`, `domain`, `double`, `drop`, `dump`, `dynamic`, `each`, `element`, `else`, `end`, `end-exec`, `equals`, `errlvl`, `escape`, `every`, `except`, `exception`, `exec`, `execute`, `exists`, `exit`, `external`, `extract`, `false`, `fetch`, `file`, `fillfactor`, `filter`, `first`, `float`, `for`, `foreign`, `fortran`, `found`, `free`, `freetext`, `freetexttable`, `from`, `full`, `fulltexttable`, `function`, `fusion`, `general`, `get`, `global`, `go`, `goto`, `grant`, `group`, `grouping`, `having`, `hold`, `holdlock`, `host`, `hour`, `identity`, `identity_insert`, `identitycol`, `if`, `ignore`, `immediate`, `in`, `include`, `index`, `indicator`, `initialize`, `initially`, `inner`, `inout`, `input`, `insensitive`, `insert`, `int`, `integer`, `intersect`, `intersection`, `interval`, `into`, `is`, `isolation`, `iterate`, `join`, `key`, `kill`, `language`, `large`, `last`, `lateral`, `leading`, `left`, `less`, `level`, `like`, `like_regex`, `limit`, `lineno`, `ln`, `load`, `local`, `localtime`, `localtimestamp`, `locator`, `lower`, `map`, `match`, `max`, `member`, `merge`, `method`, `min`, `minute`, `mod`, `modifies`, `modify`, `module`, `month`, `multiset`, `names`, `national`, `natural`, `nchar`, `nclob`, `new`, `next`, `no`, `nocheck`, `nonclustered`, `none`, `normalize`, `not`, `null`, `nullif`, `numeric`, `object`, `occurrences_regex`, `octet_length`, `of`, `off`, `offsets`, `old`, `on`, `only`, `open`, `opendatasource`, `openquery`, `openrowset`, `openxml`, `operation`, `option`, `or`, `order`, `ordinality`, `out`, `outer`, `output`, `over`, `overlaps`, `overlay`, `pad`, `parameter`, `parameters`, `partial`, `partition`, `pascal`, `path`, `percent`, `percent_rank`, `percentile_cont`, `percentile_disc`, `pivot`, `plan`, `position`, `position_regex`, `postfix`, `precision`, `prefix`, `preorder`, `prepare`, `preserve`, `primary`, `print`, `prior`, `privileges`, `proc`, `procedure`, `public`, `raiserror`, `range`, `read`, `reads`, `readtext`, `real`, `reconfigure`, `recursive`, `ref`, `references`, `referencing`, `regr_avgx`, `regr_avgy`, `regr_count`, `regr_intercept`, `regr_r2`, `regr_slope`, `regr_sxx`, `regr_sxy`, `regr_syy`, `relative`, `release`, `replication`, `restore`, `restrict`, `result`, `return`, `returns`, `revert`, `revoke`, `right`, `role`, `rollback`, `rollup`, `routine`, `row`, `rowcount`, `rowguidcol`, `rows`, `rule`, `save`, `savepoint`, `schema`, `scope`, `scroll`, `search`, `second`, `section`, `securityaudit`, `select`, `semantickeyphrasetable`, `semanticsimilaritydetailstable`, `semanticsimilaritytable`, `sensitive`, `sequence`, `session`, `session_user`, `set`, `sets`, `setuser`, `shutdown`, `similar`, `size`, `smallint`, `some`, `space`, `specific`, `specifictype`, `sql`, `sqlca`, `sqlcode`, `sqlerror`, `sqlexception`, `sqlstate`, `sqlwarning`, `start`, `state`, `statement`, `static`, `statistics`, `stddev_pop`, `stddev_samp`, `structure`, `submultiset`, `substring`, `substring_regex`, `sum`, `symmetric`, `system`, `system_user`, `table`, `tablesample`, `temporary`, `terminate`, `textsize`, `than`, `then`, `throw`, `time`, `timestamp`, `timezone_hour`, `timezone_minute`, `to`, `top`, `trailing`, `tran`, `transaction`, `translate`, `translate_regex`, `translation`, `treat`, `trigger`, `trim`, `true`, `truncate`, `try`, `try_convert`, `tsequal`, `uescape`, `under`, `union`, `unique`, `unknown`, `unnest`, `unpivot`, `update`, `updatetext`, `upper`, `usage`, `use`, `user`, `using`, `value`, `values`, `var_pop`, `var_samp`, `varchar`, `variable`, `varying`, `view`, `waitfor`, `when`, `whenever`, `where`, `while`, `width_bucket`, `window`, `with`, `within`, `without`, `work`, `write`, `writetext`, `xmlagg`, `xmlattributes`, `xmlbinary`, `xmlcast`, `xmlcomment`, `xmlconcat`, `xmldocument`, `xmlelement`, `xmlexists`, `xmlforest`, `xmliterate`, `xmlnamespaces`, `xmlparse`, `xmlpi`, `xmlquery`, `xmlserialize`, `xmltable`, `xmltext`, `xmlvalidate`, `year`, `zone`), Keyword, nil},
- {`(\[)([^]]+)(\])`, ByGroups(Operator, Name, Operator), nil},
- {`0x[0-9a-f]+`, LiteralNumberHex, nil},
- {`[0-9]+\.[0-9]*(e[+-]?[0-9]+)?`, LiteralNumberFloat, nil},
- {`\.[0-9]+(e[+-]?[0-9]+)?`, LiteralNumberFloat, nil},
- {`[0-9]+e[+-]?[0-9]+`, LiteralNumberFloat, nil},
- {`[0-9]+`, LiteralNumberInteger, nil},
- {`[;(),.]`, Punctuation, nil},
- {`@@\w+`, NameBuiltin, nil},
- {`@\w+`, NameVariable, nil},
- {`(\w+)(:)`, ByGroups(NameLabel, Punctuation), nil},
- {`#?#?\w+`, Name, nil},
- {`\?`, NameVariableMagic, nil},
- },
- "multiline-comments": {
- {`/\*`, CommentMultiline, Push("multiline-comments")},
- {`\*/`, CommentMultiline, Pop(1)},
- {`[^/*]+`, CommentMultiline, nil},
- {`[/*]`, CommentMultiline, nil},
- },
- "string": {
- {`[^']+`, LiteralStringSingle, nil},
- {`''`, LiteralStringSingle, nil},
- {`'`, LiteralStringSingle, Pop(1)},
- },
- "quoted-ident": {
- {`[^"]+`, LiteralStringName, nil},
- {`""`, LiteralStringName, nil},
- {`"`, LiteralStringName, Pop(1)},
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/t/turing.go b/vendor/github.com/alecthomas/chroma/lexers/t/turing.go
deleted file mode 100644
index e34a6c1..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/t/turing.go
+++ /dev/null
@@ -1,47 +0,0 @@
-package t
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-// Turing lexer.
-var Turing = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "Turing",
- Aliases: []string{"turing"},
- Filenames: []string{"*.turing", "*.tu"},
- MimeTypes: []string{"text/x-turing"},
- },
- turingRules,
-))
-
-func turingRules() Rules {
- return Rules{
- "root": {
- {`\n`, Text, nil},
- {`\s+`, Text, nil},
- {`\\\n`, Text, nil},
- {`%(.*?)\n`, CommentSingle, nil},
- {`/(\\\n)?[*](.|\n)*?[*](\\\n)?/`, CommentMultiline, nil},
- {`(var|fcn|function|proc|procedure|process|class|end|record|type|begin|case|loop|for|const|union|monitor|module|handler)\b`, KeywordDeclaration, nil},
- {`(all|asm|assert|bind|bits|body|break|by|cheat|checked|close|condition|decreasing|def|deferred|else|elsif|exit|export|external|flexible|fork|forward|free|get|if|implement|import|include|inherit|init|invariant|label|new|objectclass|of|opaque|open|packed|pause|pervasive|post|pre|priority|put|quit|read|register|result|seek|self|set|signal|skip|tag|tell|then|timeout|to|unchecked|unqualified|wait|when|write)\b`, Keyword, nil},
- {`(true|false)\b`, KeywordConstant, nil},
- {Words(``, `\b`, `addressint`, `array`, `boolean`, `char`, `int`, `int1`, `int2`, `int4`, `int8`, `nat`, `nat1`, `nat2`, `nat4`, `nat8`, `pointer`, `real`, `real4`, `real8`, `string`, `enum`), KeywordType, nil},
- {`\d+i`, LiteralNumber, nil},
- {`\d+\.\d*([Ee][-+]\d+)?i`, LiteralNumber, nil},
- {`\.\d+([Ee][-+]\d+)?i`, LiteralNumber, nil},
- {`\d+[Ee][-+]\d+i`, LiteralNumber, nil},
- {`\d+(\.\d+[eE][+\-]?\d+|\.\d*|[eE][+\-]?\d+)`, LiteralNumberFloat, nil},
- {`\.\d+([eE][+\-]?\d+)?`, LiteralNumberFloat, nil},
- {`0[0-7]+`, LiteralNumberOct, nil},
- {`0[xX][0-9a-fA-F]+`, LiteralNumberHex, nil},
- {`(0|[1-9][0-9]*)`, LiteralNumberInteger, nil},
- {`(div|mod|rem|\*\*|=|<|>|>=|<=|not=|not|and|or|xor|=>|in|shl|shr|->|~|~=|~in|&|:=|\.\.|[\^+\-*/])`, Operator, nil},
- {`'(\\['"\\abfnrtv]|\\x[0-9a-fA-F]{2}|\\[0-7]{1,3}|\\u[0-9a-fA-F]{4}|\\U[0-9a-fA-F]{8}|[^\\])'`, LiteralStringChar, nil},
- {`"(\\\\|\\"|[^"])*"`, LiteralString, nil},
- {`[()\[\]{}.,:]`, Punctuation, nil},
- {`[^\W\d]\w*`, NameOther, nil},
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/t/turtle.go b/vendor/github.com/alecthomas/chroma/lexers/t/turtle.go
deleted file mode 100644
index 437f375..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/t/turtle.go
+++ /dev/null
@@ -1,71 +0,0 @@
-package t
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-// Turtle lexer.
-var Turtle = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "Turtle",
- Aliases: []string{"turtle"},
- Filenames: []string{"*.ttl"},
- MimeTypes: []string{"text/turtle", "application/x-turtle"},
- NotMultiline: true,
- CaseInsensitive: true,
- },
- turtleRules,
-))
-
-func turtleRules() Rules {
- return Rules{
- "root": {
- {`\s+`, TextWhitespace, nil},
- {"(@base|BASE)(\\s+)(<[^<>\"{}|^`\\\\\\x00-\\x20]*>)(\\s*)(\\.?)", ByGroups(Keyword, TextWhitespace, NameVariable, TextWhitespace, Punctuation), nil},
- {"(@prefix|PREFIX)(\\s+)((?:[a-z][\\w-]*)?\\:)(\\s+)(<[^<>\"{}|^`\\\\\\x00-\\x20]*>)(\\s*)(\\.?)", ByGroups(Keyword, TextWhitespace, NameNamespace, TextWhitespace, NameVariable, TextWhitespace, Punctuation), nil},
- {`(?<=\s)a(?=\s)`, KeywordType, nil},
- {"(<[^<>\"{}|^`\\\\\\x00-\\x20]*>)", NameVariable, nil},
- {`((?:[a-z][\w-]*)?\:)([a-z][\w-]*)`, ByGroups(NameNamespace, NameTag), nil},
- {`#[^\n]+`, Comment, nil},
- {`\b(true|false)\b`, Literal, nil},
- {`[+\-]?\d*\.\d+`, LiteralNumberFloat, nil},
- {`[+\-]?\d*(:?\.\d+)?E[+\-]?\d+`, LiteralNumberFloat, nil},
- {`[+\-]?\d+`, LiteralNumberInteger, nil},
- {`[\[\](){}.;,:^]`, Punctuation, nil},
- {`"""`, LiteralString, Push("triple-double-quoted-string")},
- {`"`, LiteralString, Push("single-double-quoted-string")},
- {`'''`, LiteralString, Push("triple-single-quoted-string")},
- {`'`, LiteralString, Push("single-single-quoted-string")},
- },
- "triple-double-quoted-string": {
- {`"""`, LiteralString, Push("end-of-string")},
- {`[^\\]+`, LiteralString, nil},
- {`\\`, LiteralString, Push("string-escape")},
- },
- "single-double-quoted-string": {
- {`"`, LiteralString, Push("end-of-string")},
- {`[^"\\\n]+`, LiteralString, nil},
- {`\\`, LiteralString, Push("string-escape")},
- },
- "triple-single-quoted-string": {
- {`'''`, LiteralString, Push("end-of-string")},
- {`[^\\]+`, LiteralString, nil},
- {`\\`, LiteralString, Push("string-escape")},
- },
- "single-single-quoted-string": {
- {`'`, LiteralString, Push("end-of-string")},
- {`[^'\\\n]+`, LiteralString, nil},
- {`\\`, LiteralString, Push("string-escape")},
- },
- "string-escape": {
- {`.`, LiteralString, Pop(1)},
- },
- "end-of-string": {
- {`(@)([a-z]+(:?-[a-z0-9]+)*)`, ByGroups(Operator, GenericEmph, GenericEmph), Pop(2)},
- {"(\\^\\^)(<[^<>\"{}|^`\\\\\\x00-\\x20]*>)", ByGroups(Operator, GenericEmph), Pop(2)},
- {`(\^\^)((?:[a-z][\w-]*)?\:)([a-z][\w-]*)`, ByGroups(Operator, GenericEmph, GenericEmph), Pop(2)},
- Default(Pop(2)),
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/t/twig.go b/vendor/github.com/alecthomas/chroma/lexers/t/twig.go
deleted file mode 100644
index 0e0c1f1..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/t/twig.go
+++ /dev/null
@@ -1,58 +0,0 @@
-package t
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-// Twig lexer.
-var Twig = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "Twig",
- Aliases: []string{"twig"},
- Filenames: []string{},
- MimeTypes: []string{"application/x-twig"},
- DotAll: true,
- },
- twigRules,
-))
-
-func twigRules() Rules {
- return Rules{
- "root": {
- {`[^{]+`, Other, nil},
- {`\{\{`, CommentPreproc, Push("var")},
- {`\{\#.*?\#\}`, Comment, nil},
- {`(\{%)(-?\s*)(raw)(\s*-?)(%\})(.*?)(\{%)(-?\s*)(endraw)(\s*-?)(%\})`, ByGroups(CommentPreproc, Text, Keyword, Text, CommentPreproc, Other, CommentPreproc, Text, Keyword, Text, CommentPreproc), nil},
- {`(\{%)(-?\s*)(verbatim)(\s*-?)(%\})(.*?)(\{%)(-?\s*)(endverbatim)(\s*-?)(%\})`, ByGroups(CommentPreproc, Text, Keyword, Text, CommentPreproc, Other, CommentPreproc, Text, Keyword, Text, CommentPreproc), nil},
- {`(\{%)(-?\s*)(filter)(\s+)((?:[\\_a-z]|[^\x00-\x7f])(?:[\\\w-]|[^\x00-\x7f])*)`, ByGroups(CommentPreproc, Text, Keyword, Text, NameFunction), Push("tag")},
- {`(\{%)(-?\s*)([a-zA-Z_]\w*)`, ByGroups(CommentPreproc, Text, Keyword), Push("tag")},
- {`\{`, Other, nil},
- },
- "varnames": {
- {`(\|)(\s*)((?:[\\_a-z]|[^\x00-\x7f])(?:[\\\w-]|[^\x00-\x7f])*)`, ByGroups(Operator, Text, NameFunction), nil},
- {`(is)(\s+)(not)?(\s*)((?:[\\_a-z]|[^\x00-\x7f])(?:[\\\w-]|[^\x00-\x7f])*)`, ByGroups(Keyword, Text, Keyword, Text, NameFunction), nil},
- {`(?i)(true|false|none|null)\b`, KeywordPseudo, nil},
- {`(in|not|and|b-and|or|b-or|b-xor|isif|elseif|else|importconstant|defined|divisibleby|empty|even|iterable|odd|sameasmatches|starts\s+with|ends\s+with)\b`, Keyword, nil},
- {`(loop|block|parent)\b`, NameBuiltin, nil},
- {`(?:[\\_a-z]|[^\x00-\x7f])(?:[\\\w-]|[^\x00-\x7f])*`, NameVariable, nil},
- {`\.(?:[\\_a-z]|[^\x00-\x7f])(?:[\\\w-]|[^\x00-\x7f])*`, NameVariable, nil},
- {`\.[0-9]+`, LiteralNumber, nil},
- {`:?"(\\\\|\\"|[^"])*"`, LiteralStringDouble, nil},
- {`:?'(\\\\|\\'|[^'])*'`, LiteralStringSingle, nil},
- {`([{}()\[\]+\-*/,:~%]|\.\.|\?|:|\*\*|\/\/|!=|[><=]=?)`, Operator, nil},
- {`[0-9](\.[0-9]*)?(eE[+-][0-9])?[flFLdD]?|0[xX][0-9a-fA-F]+[Ll]?`, LiteralNumber, nil},
- },
- "var": {
- {`\s+`, Text, nil},
- {`(-?)(\}\})`, ByGroups(Text, CommentPreproc), Pop(1)},
- Include("varnames"),
- },
- "tag": {
- {`\s+`, Text, nil},
- {`(-?)(%\})`, ByGroups(Text, CommentPreproc), Pop(1)},
- Include("varnames"),
- {`.`, Punctuation, nil},
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/t/typescript.go b/vendor/github.com/alecthomas/chroma/lexers/t/typescript.go
deleted file mode 100644
index 0625bb0..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/t/typescript.go
+++ /dev/null
@@ -1,101 +0,0 @@
-package t
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-// TypeScript lexer.
-var TypeScript = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "TypeScript",
- Aliases: []string{"ts", "tsx", "typescript"},
- Filenames: []string{"*.ts", "*.tsx"},
- MimeTypes: []string{"text/x-typescript"},
- DotAll: true,
- EnsureNL: true,
- },
- typeScriptRules,
-))
-
-func typeScriptRules() Rules {
- return Rules{
- "commentsandwhitespace": {
- {`\s+`, Text, nil},
- {``, Comment, Pop(1)},
- {`-`, Comment, nil},
- },
- "tag": {
- {`\s+`, Text, nil},
- {`[\w.:-]+\s*=`, NameAttribute, Push("attr")},
- {`/?\s*>`, NameTag, Pop(1)},
- },
- "attr": {
- {`\s+`, Text, nil},
- {`".*?"`, LiteralString, Pop(1)},
- {`'.*?'`, LiteralString, Pop(1)},
- {`[^\s>]+`, LiteralString, Pop(1)},
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/x/xorg.go b/vendor/github.com/alecthomas/chroma/lexers/x/xorg.go
deleted file mode 100644
index 7936a51..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/x/xorg.go
+++ /dev/null
@@ -1,29 +0,0 @@
-package x
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-// Xorg lexer.
-var Xorg = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "Xorg",
- Aliases: []string{"xorg.conf"},
- Filenames: []string{"xorg.conf"},
- MimeTypes: []string{},
- },
- xorgRules,
-))
-
-func xorgRules() Rules {
- return Rules{
- "root": {
- {`\s+`, TextWhitespace, nil},
- {`#.*$`, Comment, nil},
- {`((|Sub)Section)(\s+)("\w+")`, ByGroups(KeywordNamespace, LiteralStringEscape, TextWhitespace, LiteralStringEscape), nil},
- {`(End(|Sub)Section)`, KeywordNamespace, nil},
- {`(\w+)(\s+)([^\n#]+)`, ByGroups(NameKeyword, TextWhitespace, LiteralString), nil},
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/y/yaml.go b/vendor/github.com/alecthomas/chroma/lexers/y/yaml.go
deleted file mode 100644
index 488f760..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/y/yaml.go
+++ /dev/null
@@ -1,58 +0,0 @@
-package y
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-var YAML = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "YAML",
- Aliases: []string{"yaml"},
- Filenames: []string{"*.yaml", "*.yml"},
- MimeTypes: []string{"text/x-yaml"},
- },
- yamlRules,
-))
-
-func yamlRules() Rules {
- return Rules{
- "root": {
- Include("whitespace"),
- {`^---`, NameNamespace, nil},
- {`^\.\.\.`, NameNamespace, nil},
- {`[\n?]?\s*- `, Text, nil},
- {`#.*$`, Comment, nil},
- {`!![^\s]+`, CommentPreproc, nil},
- {`&[^\s]+`, CommentPreproc, nil},
- {`\*[^\s]+`, CommentPreproc, nil},
- {`^%include\s+[^\n\r]+`, CommentPreproc, nil},
- Include("key"),
- Include("value"),
- {`[?:,\[\]]`, Punctuation, nil},
- {`.`, Text, nil},
- },
- "value": {
- {`([>|](?:[+-])?)(\n(^ {1,})(?:.*\n*(?:^\3 *).*)*)`, ByGroups(Punctuation, StringDoc, Whitespace), nil},
- {Words(``, `\b`, "true", "True", "TRUE", "false", "False", "FALSE", "null",
- "y", "Y", "yes", "Yes", "YES", "n", "N", "no", "No", "NO",
- "on", "On", "ON", "off", "Off", "OFF"), KeywordConstant, nil},
- {`"(?:\\.|[^"])*"`, StringDouble, nil},
- {`'(?:\\.|[^'])*'`, StringSingle, nil},
- {`\d\d\d\d-\d\d-\d\d([T ]\d\d:\d\d:\d\d(\.\d+)?(Z|\s+[-+]\d+)?)?`, LiteralDate, nil},
- {`\b[+\-]?(0x[\da-f]+|0o[0-7]+|(\d+\.?\d*|\.?\d+)(e[\+\-]?\d+)?|\.inf|\.nan)\b`, Number, nil},
- {`([^\{\}\[\]\?,\:\!\-\*&\@].*)( )+(#.*)`, ByGroups(Literal, Whitespace, Comment), nil},
- {`[^\{\}\[\]\?,\:\!\-\*&\@].*`, Literal, nil},
- },
- "key": {
- {`"[^"\n].*": `, NameTag, nil},
- {`(-)( )([^"\n{]*)(:)( )`, ByGroups(Punctuation, Whitespace, NameTag, Punctuation, Whitespace), nil},
- {`([^"\n{]*)(:)( )`, ByGroups(NameTag, Punctuation, Whitespace), nil},
- {`([^"\n{]*)(:)(\n)`, ByGroups(NameTag, Punctuation, Whitespace), nil},
- },
- "whitespace": {
- {`\s+`, Whitespace, nil},
- {`\n+`, Whitespace, nil},
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/lexers/y/yang.go b/vendor/github.com/alecthomas/chroma/lexers/y/yang.go
deleted file mode 100644
index 36349eb..0000000
--- a/vendor/github.com/alecthomas/chroma/lexers/y/yang.go
+++ /dev/null
@@ -1,71 +0,0 @@
-package y
-
-import (
- . "github.com/alecthomas/chroma" // nolint
- "github.com/alecthomas/chroma/lexers/internal"
-)
-
-var YANG = internal.Register(MustNewLazyLexer(
- &Config{
- Name: "YANG",
- Aliases: []string{"yang"},
- Filenames: []string{"*.yang"},
- MimeTypes: []string{"application/yang"},
- },
- yangRules,
-))
-
-func yangRules() Rules {
- return Rules{
- "root": {
- {`\s+`, Whitespace, nil},
- {`[\{\}\;]+`, Punctuation, nil},
- {`(?<|^!?/\-*&~:]`, Operator, nil},
- {`[{}()\[\],.;]`, Punctuation, nil},
- },
- "string": {
- {`\\(x[a-fA-F0-9]{2}|u[a-fA-F0-9]{4}|U[a-fA-F0-9]{6}|[nr\\t\'"])`, LiteralStringEscape, nil},
- {`[^\\"\n]+`, LiteralString, nil},
- {`"`, LiteralString, Pop(1)},
- },
- }
-}
diff --git a/vendor/github.com/alecthomas/chroma/mutators.go b/vendor/github.com/alecthomas/chroma/mutators.go
deleted file mode 100644
index bd6d720..0000000
--- a/vendor/github.com/alecthomas/chroma/mutators.go
+++ /dev/null
@@ -1,131 +0,0 @@
-package chroma
-
-import (
- "fmt"
- "strings"
-)
-
-// A Mutator modifies the behaviour of the lexer.
-type Mutator interface {
- // Mutate the lexer state machine as it is processing.
- Mutate(state *LexerState) error
-}
-
-// A LexerMutator is an additional interface that a Mutator can implement
-// to modify the lexer when it is compiled.
-type LexerMutator interface {
- // Rules are the lexer rules, state is the state key for the rule the mutator is associated with.
- MutateLexer(rules CompiledRules, state string, rule int) error
-}
-
-// A MutatorFunc is a Mutator that mutates the lexer state machine as it is processing.
-type MutatorFunc func(state *LexerState) error
-
-func (m MutatorFunc) Mutate(state *LexerState) error { return m(state) } // nolint
-
-// Mutators applies a set of Mutators in order.
-func Mutators(modifiers ...Mutator) MutatorFunc {
- return func(state *LexerState) error {
- for _, modifier := range modifiers {
- if err := modifier.Mutate(state); err != nil {
- return err
- }
- }
- return nil
- }
-}
-
-type includeMutator struct {
- state string
-}
-
-// Include the given state.
-func Include(state string) Rule {
- return Rule{Mutator: &includeMutator{state}}
-}
-
-func (i *includeMutator) Mutate(s *LexerState) error {
- return fmt.Errorf("should never reach here Include(%q)", i.state)
-}
-
-func (i *includeMutator) MutateLexer(rules CompiledRules, state string, rule int) error {
- includedRules, ok := rules[i.state]
- if !ok {
- return fmt.Errorf("invalid include state %q", i.state)
- }
- rules[state] = append(rules[state][:rule], append(includedRules, rules[state][rule+1:]...)...)
- return nil
-}
-
-type combinedMutator struct {
- states []string
-}
-
-// Combined creates a new anonymous state from the given states, and pushes that state.
-func Combined(states ...string) Mutator {
- return &combinedMutator{states}
-}
-
-func (c *combinedMutator) Mutate(s *LexerState) error {
- return fmt.Errorf("should never reach here Combined(%v)", c.states)
-}
-
-func (c *combinedMutator) MutateLexer(rules CompiledRules, state string, rule int) error {
- name := "__combined_" + strings.Join(c.states, "__")
- if _, ok := rules[name]; !ok {
- combined := []*CompiledRule{}
- for _, state := range c.states {
- rules, ok := rules[state]
- if !ok {
- return fmt.Errorf("invalid combine state %q", state)
- }
- combined = append(combined, rules...)
- }
- rules[name] = combined
- }
- rules[state][rule].Mutator = Push(name)
- return nil
-}
-
-// Push states onto the stack.
-func Push(states ...string) MutatorFunc {
- return func(s *LexerState) error {
- if len(states) == 0 {
- s.Stack = append(s.Stack, s.State)
- } else {
- for _, state := range states {
- if state == "#pop" {
- s.Stack = s.Stack[:len(s.Stack)-1]
- } else {
- s.Stack = append(s.Stack, state)
- }
- }
- }
- return nil
- }
-}
-
-// Pop state from the stack when rule matches.
-func Pop(n int) MutatorFunc {
- return func(state *LexerState) error {
- if len(state.Stack) == 0 {
- return fmt.Errorf("nothing to pop")
- }
- state.Stack = state.Stack[:len(state.Stack)-n]
- return nil
- }
-}
-
-// Default returns a Rule that applies a set of Mutators.
-func Default(mutators ...Mutator) Rule {
- return Rule{Mutator: Mutators(mutators...)}
-}
-
-// Stringify returns the raw string for a set of tokens.
-func Stringify(tokens ...Token) string {
- out := []string{}
- for _, t := range tokens {
- out = append(out, t.Value)
- }
- return strings.Join(out, "")
-}
diff --git a/vendor/github.com/alecthomas/chroma/pygments-lexers.txt b/vendor/github.com/alecthomas/chroma/pygments-lexers.txt
deleted file mode 100644
index bf4d9de..0000000
--- a/vendor/github.com/alecthomas/chroma/pygments-lexers.txt
+++ /dev/null
@@ -1,322 +0,0 @@
-Generated with:
-
- g 'class.*RegexLexer' | pawk --strict -F: '"pygments.lexers.%s.%s" % (f[0].split(".")[0], f[2].split()[1].split("(")[0])' > lexers.txt
-
-kotlin:
- invalid unicode escape sequences
- FIXED: Have to disable wide Unicode characters in unistring.py
-
-pygments.lexers.ambient.AmbientTalkLexer
-pygments.lexers.ampl.AmplLexer
-pygments.lexers.actionscript.ActionScriptLexer
-pygments.lexers.actionscript.ActionScript3Lexer
-pygments.lexers.actionscript.MxmlLexer
-pygments.lexers.algebra.GAPLexer
-pygments.lexers.algebra.MathematicaLexer
-pygments.lexers.algebra.MuPADLexer
-pygments.lexers.algebra.BCLexer
-pygments.lexers.apl.APLLexer
-pygments.lexers.bibtex.BibTeXLexer
-pygments.lexers.bibtex.BSTLexer
-pygments.lexers.basic.BlitzMaxLexer
-pygments.lexers.basic.BlitzBasicLexer
-pygments.lexers.basic.MonkeyLexer
-pygments.lexers.basic.CbmBasicV2Lexer
-pygments.lexers.basic.QBasicLexer
-pygments.lexers.automation.AutohotkeyLexer
-pygments.lexers.automation.AutoItLexer
-pygments.lexers.archetype.AtomsLexer
-pygments.lexers.c_like.ClayLexer
-pygments.lexers.c_like.ValaLexer
-pygments.lexers.asm.GasLexer
-pygments.lexers.asm.ObjdumpLexer
-pygments.lexers.asm.HsailLexer
-pygments.lexers.asm.LlvmLexer
-pygments.lexers.asm.NasmLexer
-pygments.lexers.asm.TasmLexer
-pygments.lexers.asm.Ca65Lexer
-pygments.lexers.business.CobolLexer
-pygments.lexers.business.ABAPLexer
-pygments.lexers.business.OpenEdgeLexer
-pygments.lexers.business.GoodDataCLLexer
-pygments.lexers.business.MaqlLexer
-pygments.lexers.capnproto.CapnProtoLexer
-pygments.lexers.chapel.ChapelLexer
-pygments.lexers.clean.CleanLexer
-pygments.lexers.c_cpp.CFamilyLexer
-pygments.lexers.console.VCTreeStatusLexer
-pygments.lexers.console.PyPyLogLexer
-pygments.lexers.csound.CsoundLexer
-pygments.lexers.csound.CsoundDocumentLexer
-pygments.lexers.csound.CsoundDocumentLexer
-pygments.lexers.crystal.CrystalLexer
-pygments.lexers.dalvik.SmaliLexer
-pygments.lexers.css.CssLexer
-pygments.lexers.css.SassLexer
-pygments.lexers.css.ScssLexer
-pygments.lexers.configs.IniLexer
-pygments.lexers.configs.RegeditLexer
-pygments.lexers.configs.PropertiesLexer
-pygments.lexers.configs.KconfigLexer
-pygments.lexers.configs.Cfengine3Lexer
-pygments.lexers.configs.ApacheConfLexer
-pygments.lexers.configs.SquidConfLexer
-pygments.lexers.configs.NginxConfLexer
-pygments.lexers.configs.LighttpdConfLexer
-pygments.lexers.configs.DockerLexer
-pygments.lexers.configs.TerraformLexer
-pygments.lexers.configs.TermcapLexer
-pygments.lexers.configs.TerminfoLexer
-pygments.lexers.configs.PkgConfigLexer
-pygments.lexers.configs.PacmanConfLexer
-pygments.lexers.data.YamlLexer
-pygments.lexers.data.JsonLexer
-pygments.lexers.diff.DiffLexer
-pygments.lexers.diff.DarcsPatchLexer
-pygments.lexers.diff.WDiffLexer
-pygments.lexers.dotnet.CSharpLexer
-pygments.lexers.dotnet.NemerleLexer
-pygments.lexers.dotnet.BooLexer
-pygments.lexers.dotnet.VbNetLexer
-pygments.lexers.dotnet.GenericAspxLexer
-pygments.lexers.dotnet.FSharpLexer
-pygments.lexers.dylan.DylanLexer
-pygments.lexers.dylan.DylanLidLexer
-pygments.lexers.ecl.ECLLexer
-pygments.lexers.eiffel.EiffelLexer
-pygments.lexers.dsls.ProtoBufLexer
-pygments.lexers.dsls.ThriftLexer
-pygments.lexers.dsls.BroLexer
-pygments.lexers.dsls.PuppetLexer
-pygments.lexers.dsls.RslLexer
-pygments.lexers.dsls.MscgenLexer
-pygments.lexers.dsls.VGLLexer
-pygments.lexers.dsls.AlloyLexer
-pygments.lexers.dsls.PanLexer
-pygments.lexers.dsls.CrmshLexer
-pygments.lexers.dsls.FlatlineLexer
-pygments.lexers.dsls.SnowballLexer
-pygments.lexers.elm.ElmLexer
-pygments.lexers.erlang.ErlangLexer
-pygments.lexers.erlang.ElixirLexer
-pygments.lexers.ezhil.EzhilLexer
-pygments.lexers.esoteric.BrainfuckLexer
-pygments.lexers.esoteric.BefungeLexer
-pygments.lexers.esoteric.CAmkESLexer
-pygments.lexers.esoteric.CapDLLexer
-pygments.lexers.esoteric.RedcodeLexer
-pygments.lexers.esoteric.AheuiLexer
-pygments.lexers.factor.FactorLexer
-pygments.lexers.fantom.FantomLexer
-pygments.lexers.felix.FelixLexer
-pygments.lexers.forth.ForthLexer
-pygments.lexers.fortran.FortranLexer
-pygments.lexers.fortran.FortranFixedLexer
-pygments.lexers.go.GoLexer
-pygments.lexers.foxpro.FoxProLexer
-pygments.lexers.graph.CypherLexer
-pygments.lexers.grammar_notation.BnfLexer
-pygments.lexers.grammar_notation.AbnfLexer
-pygments.lexers.grammar_notation.JsgfLexer
-pygments.lexers.graphics.GLShaderLexer
-pygments.lexers.graphics.PostScriptLexer
-pygments.lexers.graphics.AsymptoteLexer
-pygments.lexers.graphics.GnuplotLexer
-pygments.lexers.graphics.PovrayLexer
-pygments.lexers.hexdump.HexdumpLexer
-pygments.lexers.haskell.HaskellLexer
-pygments.lexers.haskell.IdrisLexer
-pygments.lexers.haskell.AgdaLexer
-pygments.lexers.haskell.CryptolLexer
-pygments.lexers.haskell.KokaLexer
-pygments.lexers.haxe.HaxeLexer
-pygments.lexers.haxe.HxmlLexer
-pygments.lexers.hdl.VerilogLexer
-pygments.lexers.hdl.SystemVerilogLexer
-pygments.lexers.hdl.VhdlLexer
-pygments.lexers.idl.IDLLexer
-pygments.lexers.inferno.LimboLexer
-pygments.lexers.igor.IgorLexer
-pygments.lexers.html.HtmlLexer
-pygments.lexers.html.DtdLexer
-pygments.lexers.html.XmlLexer
-pygments.lexers.html.HamlLexer
-pygments.lexers.html.ScamlLexer
-pygments.lexers.html.PugLexer
-pygments.lexers.installers.NSISLexer
-pygments.lexers.installers.RPMSpecLexer
-pygments.lexers.installers.SourcesListLexer
-pygments.lexers.installers.DebianControlLexer
-pygments.lexers.iolang.IoLexer
-pygments.lexers.julia.JuliaLexer
-pygments.lexers.int_fiction.Inform6Lexer
-pygments.lexers.int_fiction.Inform7Lexer
-pygments.lexers.int_fiction.Tads3Lexer
-pygments.lexers.make.BaseMakefileLexer
-pygments.lexers.make.CMakeLexer
-pygments.lexers.javascript.JavascriptLexer
-pygments.lexers.javascript.KalLexer
-pygments.lexers.javascript.LiveScriptLexer
-pygments.lexers.javascript.DartLexer
-pygments.lexers.javascript.TypeScriptLexer
-pygments.lexers.javascript.LassoLexer
-pygments.lexers.javascript.ObjectiveJLexer
-pygments.lexers.javascript.CoffeeScriptLexer
-pygments.lexers.javascript.MaskLexer
-pygments.lexers.javascript.EarlGreyLexer
-pygments.lexers.javascript.JuttleLexer
-pygments.lexers.jvm.JavaLexer
-pygments.lexers.jvm.ScalaLexer
-pygments.lexers.jvm.GosuLexer
-pygments.lexers.jvm.GroovyLexer
-pygments.lexers.jvm.IokeLexer
-pygments.lexers.jvm.ClojureLexer
-pygments.lexers.jvm.TeaLangLexer
-pygments.lexers.jvm.CeylonLexer
-pygments.lexers.jvm.KotlinLexer
-pygments.lexers.jvm.XtendLexer
-pygments.lexers.jvm.PigLexer
-pygments.lexers.jvm.GoloLexer
-pygments.lexers.jvm.JasminLexer
-pygments.lexers.markup.BBCodeLexer
-pygments.lexers.markup.MoinWikiLexer
-pygments.lexers.markup.RstLexer
-pygments.lexers.markup.TexLexer
-pygments.lexers.markup.GroffLexer
-pygments.lexers.markup.MozPreprocHashLexer
-pygments.lexers.markup.MarkdownLexer
-pygments.lexers.ml.SMLLexer
-pygments.lexers.ml.OcamlLexer
-pygments.lexers.ml.OpaLexer
-pygments.lexers.modeling.ModelicaLexer
-pygments.lexers.modeling.BugsLexer
-pygments.lexers.modeling.JagsLexer
-pygments.lexers.modeling.StanLexer
-pygments.lexers.matlab.MatlabLexer
-pygments.lexers.matlab.OctaveLexer
-pygments.lexers.matlab.ScilabLexer
-pygments.lexers.monte.MonteLexer
-pygments.lexers.lisp.SchemeLexer
-pygments.lexers.lisp.CommonLispLexer
-pygments.lexers.lisp.HyLexer
-pygments.lexers.lisp.RacketLexer
-pygments.lexers.lisp.NewLispLexer
-pygments.lexers.lisp.EmacsLispLexer
-pygments.lexers.lisp.ShenLexer
-pygments.lexers.lisp.XtlangLexer
-pygments.lexers.modula2.Modula2Lexer
-pygments.lexers.ncl.NCLLexer
-pygments.lexers.nim.NimLexer
-pygments.lexers.nit.NitLexer
-pygments.lexers.nix.NixLexer
-pygments.lexers.oberon.ComponentPascalLexer
-pygments.lexers.ooc.OocLexer
-pygments.lexers.objective.SwiftLexer
-pygments.lexers.parasail.ParaSailLexer
-pygments.lexers.pawn.SourcePawnLexer
-pygments.lexers.pawn.PawnLexer
-pygments.lexers.pascal.AdaLexer
-pygments.lexers.parsers.RagelLexer
-pygments.lexers.parsers.RagelEmbeddedLexer
-pygments.lexers.parsers.AntlrLexer
-pygments.lexers.parsers.TreetopBaseLexer
-pygments.lexers.parsers.EbnfLexer
-pygments.lexers.php.ZephirLexer
-pygments.lexers.php.PhpLexer
-pygments.lexers.perl.PerlLexer
-pygments.lexers.perl.Perl6Lexer
-pygments.lexers.praat.PraatLexer
-pygments.lexers.prolog.PrologLexer
-pygments.lexers.prolog.LogtalkLexer
-pygments.lexers.qvt.QVToLexer
-pygments.lexers.rdf.SparqlLexer
-pygments.lexers.rdf.TurtleLexer
-pygments.lexers.python.PythonLexer
-pygments.lexers.python.Python3Lexer
-pygments.lexers.python.PythonTracebackLexer
-pygments.lexers.python.Python3TracebackLexer
-pygments.lexers.python.CythonLexer
-pygments.lexers.python.DgLexer
-pygments.lexers.rebol.RebolLexer
-pygments.lexers.rebol.RedLexer
-pygments.lexers.resource.ResourceLexer
-pygments.lexers.rnc.RNCCompactLexer
-pygments.lexers.roboconf.RoboconfGraphLexer
-pygments.lexers.roboconf.RoboconfInstancesLexer
-pygments.lexers.rust.RustLexer
-pygments.lexers.ruby.RubyLexer
-pygments.lexers.ruby.FancyLexer
-pygments.lexers.sas.SASLexer
-pygments.lexers.smalltalk.SmalltalkLexer
-pygments.lexers.smalltalk.NewspeakLexer
-pygments.lexers.smv.NuSMVLexer
-pygments.lexers.shell.BashLexer
-pygments.lexers.shell.BatchLexer
-pygments.lexers.shell.TcshLexer
-pygments.lexers.shell.PowerShellLexer
-pygments.lexers.shell.FishShellLexer
-pygments.lexers.snobol.SnobolLexer
-pygments.lexers.scripting.LuaLexer
-pygments.lexers.scripting.ChaiscriptLexer
-pygments.lexers.scripting.LSLLexer
-pygments.lexers.scripting.AppleScriptLexer
-pygments.lexers.scripting.RexxLexer
-pygments.lexers.scripting.MOOCodeLexer
-pygments.lexers.scripting.HybrisLexer
-pygments.lexers.scripting.EasytrieveLexer
-pygments.lexers.scripting.JclLexer
-pygments.lexers.supercollider.SuperColliderLexer
-pygments.lexers.stata.StataLexer
-pygments.lexers.tcl.TclLexer
-pygments.lexers.sql.PostgresLexer
-pygments.lexers.sql.PlPgsqlLexer
-pygments.lexers.sql.PsqlRegexLexer
-pygments.lexers.sql.SqlLexer
-pygments.lexers.sql.TransactSqlLexer
-pygments.lexers.sql.MySqlLexer
-pygments.lexers.sql.RqlLexer
-pygments.lexers.testing.GherkinLexer
-pygments.lexers.testing.TAPLexer
-pygments.lexers.textedit.AwkLexer
-pygments.lexers.textedit.VimLexer
-pygments.lexers.textfmts.IrcLogsLexer
-pygments.lexers.textfmts.GettextLexer
-pygments.lexers.textfmts.HttpLexer
-pygments.lexers.textfmts.TodotxtLexer
-pygments.lexers.trafficscript.RtsLexer
-pygments.lexers.theorem.CoqLexer
-pygments.lexers.theorem.IsabelleLexer
-pygments.lexers.theorem.LeanLexer
-pygments.lexers.templates.SmartyLexer
-pygments.lexers.templates.VelocityLexer
-pygments.lexers.templates.DjangoLexer
-pygments.lexers.templates.MyghtyLexer
-pygments.lexers.templates.MasonLexer
-pygments.lexers.templates.MakoLexer
-pygments.lexers.templates.CheetahLexer
-pygments.lexers.templates.GenshiTextLexer
-pygments.lexers.templates.GenshiMarkupLexer
-pygments.lexers.templates.JspRootLexer
-pygments.lexers.templates.EvoqueLexer
-pygments.lexers.templates.ColdfusionLexer
-pygments.lexers.templates.ColdfusionMarkupLexer
-pygments.lexers.templates.TeaTemplateRootLexer
-pygments.lexers.templates.HandlebarsLexer
-pygments.lexers.templates.LiquidLexer
-pygments.lexers.templates.TwigLexer
-pygments.lexers.templates.Angular2Lexer
-pygments.lexers.urbi.UrbiscriptLexer
-pygments.lexers.typoscript.TypoScriptCssDataLexer
-pygments.lexers.typoscript.TypoScriptHtmlDataLexer
-pygments.lexers.typoscript.TypoScriptLexer
-pygments.lexers.varnish.VCLLexer
-pygments.lexers.verification.BoogieLexer
-pygments.lexers.verification.SilverLexer
-pygments.lexers.x10.X10Lexer
-pygments.lexers.whiley.WhileyLexer
-pygments.lexers.xorg.XorgLexer
-pygments.lexers.webmisc.DuelLexer
-pygments.lexers.webmisc.XQueryLexer
-pygments.lexers.webmisc.QmlLexer
-pygments.lexers.webmisc.CirruLexer
-pygments.lexers.webmisc.SlimLexer
diff --git a/vendor/github.com/alecthomas/chroma/quick/quick.go b/vendor/github.com/alecthomas/chroma/quick/quick.go
deleted file mode 100644
index 1ed3db7..0000000
--- a/vendor/github.com/alecthomas/chroma/quick/quick.go
+++ /dev/null
@@ -1,44 +0,0 @@
-// Package quick provides simple, no-configuration source code highlighting.
-package quick
-
-import (
- "io"
-
- "github.com/alecthomas/chroma"
- "github.com/alecthomas/chroma/formatters"
- "github.com/alecthomas/chroma/lexers"
- "github.com/alecthomas/chroma/styles"
-)
-
-// Highlight some text.
-//
-// Lexer, formatter and style may be empty, in which case a best-effort is made.
-func Highlight(w io.Writer, source, lexer, formatter, style string) error {
- // Determine lexer.
- l := lexers.Get(lexer)
- if l == nil {
- l = lexers.Analyse(source)
- }
- if l == nil {
- l = lexers.Fallback
- }
- l = chroma.Coalesce(l)
-
- // Determine formatter.
- f := formatters.Get(formatter)
- if f == nil {
- f = formatters.Fallback
- }
-
- // Determine style.
- s := styles.Get(style)
- if s == nil {
- s = styles.Fallback
- }
-
- it, err := l.Tokenise(nil, source)
- if err != nil {
- return err
- }
- return f.Format(w, s, it)
-}
diff --git a/vendor/github.com/alecthomas/chroma/regexp.go b/vendor/github.com/alecthomas/chroma/regexp.go
deleted file mode 100644
index 73f8031..0000000
--- a/vendor/github.com/alecthomas/chroma/regexp.go
+++ /dev/null
@@ -1,565 +0,0 @@
-package chroma
-
-import (
- "fmt"
- "os"
- "regexp"
- "sort"
- "strings"
- "sync"
- "time"
- "unicode/utf8"
-
- "github.com/dlclark/regexp2"
-)
-
-// A Rule is the fundamental matching unit of the Regex lexer state machine.
-type Rule struct {
- Pattern string
- Type Emitter
- Mutator Mutator
-}
-
-// An Emitter takes group matches and returns tokens.
-type Emitter interface {
- // Emit tokens for the given regex groups.
- Emit(groups []string, state *LexerState) Iterator
-}
-
-// EmitterFunc is a function that is an Emitter.
-type EmitterFunc func(groups []string, state *LexerState) Iterator
-
-// Emit tokens for groups.
-func (e EmitterFunc) Emit(groups []string, state *LexerState) Iterator {
- return e(groups, state)
-}
-
-// ByGroups emits a token for each matching group in the rule's regex.
-func ByGroups(emitters ...Emitter) Emitter {
- return EmitterFunc(func(groups []string, state *LexerState) Iterator {
- iterators := make([]Iterator, 0, len(groups)-1)
- if len(emitters) != len(groups)-1 {
- iterators = append(iterators, Error.Emit(groups, state))
- // panic(errors.Errorf("number of groups %q does not match number of emitters %v", groups, emitters))
- } else {
- for i, group := range groups[1:] {
- if emitters[i] != nil {
- iterators = append(iterators, emitters[i].Emit([]string{group}, state))
- }
- }
- }
- return Concaterator(iterators...)
- })
-}
-
-// ByGroupNames emits a token for each named matching group in the rule's regex.
-func ByGroupNames(emitters map[string]Emitter) Emitter {
- return EmitterFunc(func(groups []string, state *LexerState) Iterator {
- iterators := make([]Iterator, 0, len(state.NamedGroups)-1)
- if len(state.NamedGroups)-1 == 0 {
- if emitter, ok := emitters[`0`]; ok {
- iterators = append(iterators, emitter.Emit(groups, state))
- } else {
- iterators = append(iterators, Error.Emit(groups, state))
- }
- } else {
- ruleRegex := state.Rules[state.State][state.Rule].Regexp
- for i := 1; i < len(state.NamedGroups); i++ {
- groupName := ruleRegex.GroupNameFromNumber(i)
- group := state.NamedGroups[groupName]
- if emitter, ok := emitters[groupName]; ok {
- if emitter != nil {
- iterators = append(iterators, emitter.Emit([]string{group}, state))
- }
- } else {
- iterators = append(iterators, Error.Emit([]string{group}, state))
- }
- }
- }
- return Concaterator(iterators...)
- })
-}
-
-// UsingByGroup emits tokens for the matched groups in the regex using a
-// "sublexer". Used when lexing code blocks where the name of a sublexer is
-// contained within the block, for example on a Markdown text block or SQL
-// language block.
-//
-// The sublexer will be retrieved using sublexerGetFunc (typically
-// internal.Get), using the captured value from the matched sublexerNameGroup.
-//
-// If sublexerGetFunc returns a non-nil lexer for the captured sublexerNameGroup,
-// then tokens for the matched codeGroup will be emitted using the retrieved
-// lexer. Otherwise, if the sublexer is nil, then tokens will be emitted from
-// the passed emitter.
-//
-// Example:
-//
-// var Markdown = internal.Register(MustNewLexer(
-// &Config{
-// Name: "markdown",
-// Aliases: []string{"md", "mkd"},
-// Filenames: []string{"*.md", "*.mkd", "*.markdown"},
-// MimeTypes: []string{"text/x-markdown"},
-// },
-// Rules{
-// "root": {
-// {"^(```)(\\w+)(\\n)([\\w\\W]*?)(^```$)",
-// UsingByGroup(
-// internal.Get,
-// 2, 4,
-// String, String, String, Text, String,
-// ),
-// nil,
-// },
-// },
-// },
-// ))
-//
-// See the lexers/m/markdown.go for the complete example.
-//
-// Note: panic's if the number emitters does not equal the number of matched
-// groups in the regex.
-func UsingByGroup(sublexerGetFunc func(string) Lexer, sublexerNameGroup, codeGroup int, emitters ...Emitter) Emitter {
- return EmitterFunc(func(groups []string, state *LexerState) Iterator {
- // bounds check
- if len(emitters) != len(groups)-1 {
- panic("UsingByGroup expects number of emitters to be the same as len(groups)-1")
- }
-
- // grab sublexer
- sublexer := sublexerGetFunc(groups[sublexerNameGroup])
-
- // build iterators
- iterators := make([]Iterator, len(groups)-1)
- for i, group := range groups[1:] {
- if i == codeGroup-1 && sublexer != nil {
- var err error
- iterators[i], err = sublexer.Tokenise(nil, groups[codeGroup])
- if err != nil {
- panic(err)
- }
- } else if emitters[i] != nil {
- iterators[i] = emitters[i].Emit([]string{group}, state)
- }
- }
-
- return Concaterator(iterators...)
- })
-}
-
-// Using returns an Emitter that uses a given Lexer for parsing and emitting.
-func Using(lexer Lexer) Emitter {
- return EmitterFunc(func(groups []string, _ *LexerState) Iterator {
- it, err := lexer.Tokenise(&TokeniseOptions{State: "root", Nested: true}, groups[0])
- if err != nil {
- panic(err)
- }
- return it
- })
-}
-
-// UsingSelf is like Using, but uses the current Lexer.
-func UsingSelf(stateName string) Emitter {
- return EmitterFunc(func(groups []string, state *LexerState) Iterator {
- it, err := state.Lexer.Tokenise(&TokeniseOptions{State: stateName, Nested: true}, groups[0])
- if err != nil {
- panic(err)
- }
- return it
- })
-}
-
-// Words creates a regex that matches any of the given literal words.
-func Words(prefix, suffix string, words ...string) string {
- sort.Slice(words, func(i, j int) bool {
- return len(words[j]) < len(words[i])
- })
- for i, word := range words {
- words[i] = regexp.QuoteMeta(word)
- }
- return prefix + `(` + strings.Join(words, `|`) + `)` + suffix
-}
-
-// Tokenise text using lexer, returning tokens as a slice.
-func Tokenise(lexer Lexer, options *TokeniseOptions, text string) ([]Token, error) {
- var out []Token
- it, err := lexer.Tokenise(options, text)
- if err != nil {
- return nil, err
- }
- for t := it(); t != EOF; t = it() {
- out = append(out, t)
- }
- return out, nil
-}
-
-// Rules maps from state to a sequence of Rules.
-type Rules map[string][]Rule
-
-// Rename clones rules then a rule.
-func (r Rules) Rename(oldRule, newRule string) Rules {
- r = r.Clone()
- r[newRule] = r[oldRule]
- delete(r, oldRule)
- return r
-}
-
-// Clone returns a clone of the Rules.
-func (r Rules) Clone() Rules {
- out := map[string][]Rule{}
- for key, rules := range r {
- out[key] = make([]Rule, len(rules))
- copy(out[key], rules)
- }
- return out
-}
-
-// Merge creates a clone of "r" then merges "rules" into the clone.
-func (r Rules) Merge(rules Rules) Rules {
- out := r.Clone()
- for k, v := range rules.Clone() {
- out[k] = v
- }
- return out
-}
-
-// MustNewLazyLexer creates a new Lexer with deferred rules generation or panics.
-func MustNewLazyLexer(config *Config, rulesFunc func() Rules) *RegexLexer {
- lexer, err := NewLazyLexer(config, rulesFunc)
- if err != nil {
- panic(err)
- }
- return lexer
-}
-
-// NewLazyLexer creates a new regex-based Lexer with deferred rules generation.
-func NewLazyLexer(config *Config, rulesFunc func() Rules) (*RegexLexer, error) {
- if config == nil {
- config = &Config{}
- }
- return &RegexLexer{
- config: config,
- compilerFunc: rulesFunc,
- }, nil
-}
-
-// MustNewLexer creates a new Lexer or panics.
-//
-// Deprecated: Use MustNewLazyLexer instead.
-func MustNewLexer(config *Config, rules Rules) *RegexLexer { // nolint: forbidigo
- lexer, err := NewLexer(config, rules) // nolint: forbidigo
- if err != nil {
- panic(err)
- }
- return lexer
-}
-
-// NewLexer creates a new regex-based Lexer.
-//
-// "rules" is a state machine transitition map. Each key is a state. Values are sets of rules
-// that match input, optionally modify lexer state, and output tokens.
-//
-// Deprecated: Use NewLazyLexer instead.
-func NewLexer(config *Config, rules Rules) (*RegexLexer, error) { // nolint: forbidigo
- return NewLazyLexer(config, func() Rules { return rules })
-}
-
-// Trace enables debug tracing.
-func (r *RegexLexer) Trace(trace bool) *RegexLexer {
- r.trace = trace
- return r
-}
-
-// A CompiledRule is a Rule with a pre-compiled regex.
-//
-// Note that regular expressions are lazily compiled on first use of the lexer.
-type CompiledRule struct {
- Rule
- Regexp *regexp2.Regexp
- flags string
-}
-
-// CompiledRules is a map of rule name to sequence of compiled rules in that rule.
-type CompiledRules map[string][]*CompiledRule
-
-// LexerState contains the state for a single lex.
-type LexerState struct {
- Lexer *RegexLexer
- Text []rune
- Pos int
- Rules CompiledRules
- Stack []string
- State string
- Rule int
- // Group matches.
- Groups []string
- // Named Group matches.
- NamedGroups map[string]string
- // Custum context for mutators.
- MutatorContext map[interface{}]interface{}
- iteratorStack []Iterator
- options *TokeniseOptions
- newlineAdded bool
-}
-
-// Set mutator context.
-func (l *LexerState) Set(key interface{}, value interface{}) {
- l.MutatorContext[key] = value
-}
-
-// Get mutator context.
-func (l *LexerState) Get(key interface{}) interface{} {
- return l.MutatorContext[key]
-}
-
-// Iterator returns the next Token from the lexer.
-func (l *LexerState) Iterator() Token { // nolint: gocognit
- end := len(l.Text)
- if l.newlineAdded {
- end--
- }
- for l.Pos < end && len(l.Stack) > 0 {
- // Exhaust the iterator stack, if any.
- for len(l.iteratorStack) > 0 {
- n := len(l.iteratorStack) - 1
- t := l.iteratorStack[n]()
- if t == EOF {
- l.iteratorStack = l.iteratorStack[:n]
- continue
- }
- return t
- }
-
- l.State = l.Stack[len(l.Stack)-1]
- if l.Lexer.trace {
- fmt.Fprintf(os.Stderr, "%s: pos=%d, text=%q\n", l.State, l.Pos, string(l.Text[l.Pos:]))
- }
- selectedRule, ok := l.Rules[l.State]
- if !ok {
- panic("unknown state " + l.State)
- }
- ruleIndex, rule, groups, namedGroups := matchRules(l.Text, l.Pos, selectedRule)
- // No match.
- if groups == nil {
- // From Pygments :\
- //
- // If the RegexLexer encounters a newline that is flagged as an error token, the stack is
- // emptied and the lexer continues scanning in the 'root' state. This can help producing
- // error-tolerant highlighting for erroneous input, e.g. when a single-line string is not
- // closed.
- if l.Text[l.Pos] == '\n' && l.State != l.options.State {
- l.Stack = []string{l.options.State}
- continue
- }
- l.Pos++
- return Token{Error, string(l.Text[l.Pos-1 : l.Pos])}
- }
- l.Rule = ruleIndex
- l.Groups = groups
- l.NamedGroups = namedGroups
- l.Pos += utf8.RuneCountInString(groups[0])
- if rule.Mutator != nil {
- if err := rule.Mutator.Mutate(l); err != nil {
- panic(err)
- }
- }
- if rule.Type != nil {
- l.iteratorStack = append(l.iteratorStack, rule.Type.Emit(l.Groups, l))
- }
- }
- // Exhaust the IteratorStack, if any.
- // Duplicate code, but eh.
- for len(l.iteratorStack) > 0 {
- n := len(l.iteratorStack) - 1
- t := l.iteratorStack[n]()
- if t == EOF {
- l.iteratorStack = l.iteratorStack[:n]
- continue
- }
- return t
- }
-
- // If we get to here and we still have text, return it as an error.
- if l.Pos != len(l.Text) && len(l.Stack) == 0 {
- value := string(l.Text[l.Pos:])
- l.Pos = len(l.Text)
- return Token{Type: Error, Value: value}
- }
- return EOF
-}
-
-// RegexLexer is the default lexer implementation used in Chroma.
-type RegexLexer struct {
- config *Config
- analyser func(text string) float32
- trace bool
-
- mu sync.Mutex
- compiled bool
- rules map[string][]*CompiledRule
- compilerFunc func() Rules
- compileOnce sync.Once
-}
-
-// SetAnalyser sets the analyser function used to perform content inspection.
-func (r *RegexLexer) SetAnalyser(analyser func(text string) float32) *RegexLexer {
- r.analyser = analyser
- return r
-}
-
-func (r *RegexLexer) AnalyseText(text string) float32 { // nolint
- if r.analyser != nil {
- return r.analyser(text)
- }
- return 0.0
-}
-
-func (r *RegexLexer) Config() *Config { // nolint
- return r.config
-}
-
-// Regex compilation is deferred until the lexer is used. This is to avoid significant init() time costs.
-func (r *RegexLexer) maybeCompile() (err error) {
- r.mu.Lock()
- defer r.mu.Unlock()
- if r.compiled {
- return nil
- }
- for state, rules := range r.rules {
- for i, rule := range rules {
- if rule.Regexp == nil {
- pattern := "(?:" + rule.Pattern + ")"
- if rule.flags != "" {
- pattern = "(?" + rule.flags + ")" + pattern
- }
- pattern = `\G` + pattern
- rule.Regexp, err = regexp2.Compile(pattern, regexp2.RE2)
- if err != nil {
- return fmt.Errorf("failed to compile rule %s.%d: %s", state, i, err)
- }
- rule.Regexp.MatchTimeout = time.Millisecond * 250
- }
- }
- }
-restart:
- seen := map[LexerMutator]bool{}
- for state := range r.rules {
- for i := 0; i < len(r.rules[state]); i++ {
- rule := r.rules[state][i]
- if compile, ok := rule.Mutator.(LexerMutator); ok {
- if seen[compile] {
- return fmt.Errorf("saw mutator %T twice; this should not happen", compile)
- }
- seen[compile] = true
- if err := compile.MutateLexer(r.rules, state, i); err != nil {
- return err
- }
- // Process the rules again in case the mutator added/removed rules.
- //
- // This sounds bad, but shouldn't be significant in practice.
- goto restart
- }
- }
- }
- r.compiled = true
- return nil
-}
-
-func (r *RegexLexer) compileRules() error {
- rules := r.compilerFunc()
- if _, ok := rules["root"]; !ok {
- return fmt.Errorf("no \"root\" state")
- }
- compiledRules := map[string][]*CompiledRule{}
- for state, rules := range rules {
- compiledRules[state] = nil
- for _, rule := range rules {
- flags := ""
- if !r.config.NotMultiline {
- flags += "m"
- }
- if r.config.CaseInsensitive {
- flags += "i"
- }
- if r.config.DotAll {
- flags += "s"
- }
- compiledRules[state] = append(compiledRules[state], &CompiledRule{Rule: rule, flags: flags})
- }
- }
-
- r.rules = compiledRules
- return nil
-}
-
-func (r *RegexLexer) Tokenise(options *TokeniseOptions, text string) (Iterator, error) { // nolint
- var err error
- if r.compilerFunc != nil {
- r.compileOnce.Do(func() {
- err = r.compileRules()
- })
- }
- if err != nil {
- return nil, err
- }
- if err := r.maybeCompile(); err != nil {
- return nil, err
- }
- if options == nil {
- options = defaultOptions
- }
- if options.EnsureLF {
- text = ensureLF(text)
- }
- newlineAdded := false
- if !options.Nested && r.config.EnsureNL && !strings.HasSuffix(text, "\n") {
- text += "\n"
- newlineAdded = true
- }
- state := &LexerState{
- newlineAdded: newlineAdded,
- options: options,
- Lexer: r,
- Text: []rune(text),
- Stack: []string{options.State},
- Rules: r.rules,
- MutatorContext: map[interface{}]interface{}{},
- }
- return state.Iterator, nil
-}
-
-func matchRules(text []rune, pos int, rules []*CompiledRule) (int, *CompiledRule, []string, map[string]string) {
- for i, rule := range rules {
- match, err := rule.Regexp.FindRunesMatchStartingAt(text, pos)
- if match != nil && err == nil && match.Index == pos {
- groups := []string{}
- namedGroups := make(map[string]string)
- for _, g := range match.Groups() {
- namedGroups[g.Name] = g.String()
- groups = append(groups, g.String())
- }
- return i, rule, groups, namedGroups
- }
- }
- return 0, &CompiledRule{}, nil, nil
-}
-
-// replace \r and \r\n with \n
-// same as strings.ReplaceAll but more efficient
-func ensureLF(text string) string {
- buf := make([]byte, len(text))
- var j int
- for i := 0; i < len(text); i++ {
- c := text[i]
- if c == '\r' {
- if i < len(text)-1 && text[i+1] == '\n' {
- continue
- }
- c = '\n'
- }
- buf[j] = c
- j++
- }
- return string(buf[:j])
-}
diff --git a/vendor/github.com/alecthomas/chroma/remap.go b/vendor/github.com/alecthomas/chroma/remap.go
deleted file mode 100644
index cfb5c38..0000000
--- a/vendor/github.com/alecthomas/chroma/remap.go
+++ /dev/null
@@ -1,80 +0,0 @@
-package chroma
-
-type remappingLexer struct {
- lexer Lexer
- mapper func(Token) []Token
-}
-
-// RemappingLexer remaps a token to a set of, potentially empty, tokens.
-func RemappingLexer(lexer Lexer, mapper func(Token) []Token) Lexer {
- return &remappingLexer{lexer, mapper}
-}
-
-func (r *remappingLexer) Config() *Config {
- return r.lexer.Config()
-}
-
-func (r *remappingLexer) Tokenise(options *TokeniseOptions, text string) (Iterator, error) {
- it, err := r.lexer.Tokenise(options, text)
- if err != nil {
- return nil, err
- }
- var buffer []Token
- return func() Token {
- for {
- if len(buffer) > 0 {
- t := buffer[0]
- buffer = buffer[1:]
- return t
- }
- t := it()
- if t == EOF {
- return t
- }
- buffer = r.mapper(t)
- }
- }, nil
-}
-
-// TypeMapping defines type maps for the TypeRemappingLexer.
-type TypeMapping []struct {
- From, To TokenType
- Words []string
-}
-
-// TypeRemappingLexer remaps types of tokens coming from a parent Lexer.
-//
-// eg. Map "defvaralias" tokens of type NameVariable to NameFunction:
-//
-// mapping := TypeMapping{
-// {NameVariable, NameFunction, []string{"defvaralias"},
-// }
-// lexer = TypeRemappingLexer(lexer, mapping)
-func TypeRemappingLexer(lexer Lexer, mapping TypeMapping) Lexer {
- // Lookup table for fast remapping.
- lut := map[TokenType]map[string]TokenType{}
- for _, rt := range mapping {
- km, ok := lut[rt.From]
- if !ok {
- km = map[string]TokenType{}
- lut[rt.From] = km
- }
- if len(rt.Words) == 0 {
- km[""] = rt.To
- } else {
- for _, k := range rt.Words {
- km[k] = rt.To
- }
- }
- }
- return RemappingLexer(lexer, func(t Token) []Token {
- if k, ok := lut[t.Type]; ok {
- if tt, ok := k[t.Value]; ok {
- t.Type = tt
- } else if tt, ok := k[""]; ok {
- t.Type = tt
- }
- }
- return []Token{t}
- })
-}
diff --git a/vendor/github.com/alecthomas/chroma/style.go b/vendor/github.com/alecthomas/chroma/style.go
deleted file mode 100644
index 1319fc4..0000000
--- a/vendor/github.com/alecthomas/chroma/style.go
+++ /dev/null
@@ -1,344 +0,0 @@
-package chroma
-
-import (
- "fmt"
- "strings"
-)
-
-// Trilean value for StyleEntry value inheritance.
-type Trilean uint8
-
-// Trilean states.
-const (
- Pass Trilean = iota
- Yes
- No
-)
-
-func (t Trilean) String() string {
- switch t {
- case Yes:
- return "Yes"
- case No:
- return "No"
- default:
- return "Pass"
- }
-}
-
-// Prefix returns s with "no" as a prefix if Trilean is no.
-func (t Trilean) Prefix(s string) string {
- if t == Yes {
- return s
- } else if t == No {
- return "no" + s
- }
- return ""
-}
-
-// A StyleEntry in the Style map.
-type StyleEntry struct {
- // Hex colours.
- Colour Colour
- Background Colour
- Border Colour
-
- Bold Trilean
- Italic Trilean
- Underline Trilean
- NoInherit bool
-}
-
-func (s StyleEntry) String() string {
- out := []string{}
- if s.Bold != Pass {
- out = append(out, s.Bold.Prefix("bold"))
- }
- if s.Italic != Pass {
- out = append(out, s.Italic.Prefix("italic"))
- }
- if s.Underline != Pass {
- out = append(out, s.Underline.Prefix("underline"))
- }
- if s.NoInherit {
- out = append(out, "noinherit")
- }
- if s.Colour.IsSet() {
- out = append(out, s.Colour.String())
- }
- if s.Background.IsSet() {
- out = append(out, "bg:"+s.Background.String())
- }
- if s.Border.IsSet() {
- out = append(out, "border:"+s.Border.String())
- }
- return strings.Join(out, " ")
-}
-
-// Sub subtracts e from s where elements match.
-func (s StyleEntry) Sub(e StyleEntry) StyleEntry {
- out := StyleEntry{}
- if e.Colour != s.Colour {
- out.Colour = s.Colour
- }
- if e.Background != s.Background {
- out.Background = s.Background
- }
- if e.Bold != s.Bold {
- out.Bold = s.Bold
- }
- if e.Italic != s.Italic {
- out.Italic = s.Italic
- }
- if e.Underline != s.Underline {
- out.Underline = s.Underline
- }
- if e.Border != s.Border {
- out.Border = s.Border
- }
- return out
-}
-
-// Inherit styles from ancestors.
-//
-// Ancestors should be provided from oldest to newest.
-func (s StyleEntry) Inherit(ancestors ...StyleEntry) StyleEntry {
- out := s
- for i := len(ancestors) - 1; i >= 0; i-- {
- if out.NoInherit {
- return out
- }
- ancestor := ancestors[i]
- if !out.Colour.IsSet() {
- out.Colour = ancestor.Colour
- }
- if !out.Background.IsSet() {
- out.Background = ancestor.Background
- }
- if !out.Border.IsSet() {
- out.Border = ancestor.Border
- }
- if out.Bold == Pass {
- out.Bold = ancestor.Bold
- }
- if out.Italic == Pass {
- out.Italic = ancestor.Italic
- }
- if out.Underline == Pass {
- out.Underline = ancestor.Underline
- }
- }
- return out
-}
-
-func (s StyleEntry) IsZero() bool {
- return s.Colour == 0 && s.Background == 0 && s.Border == 0 && s.Bold == Pass && s.Italic == Pass &&
- s.Underline == Pass && !s.NoInherit
-}
-
-// A StyleBuilder is a mutable structure for building styles.
-//
-// Once built, a Style is immutable.
-type StyleBuilder struct {
- entries map[TokenType]string
- name string
- parent *Style
-}
-
-func NewStyleBuilder(name string) *StyleBuilder {
- return &StyleBuilder{name: name, entries: map[TokenType]string{}}
-}
-
-func (s *StyleBuilder) AddAll(entries StyleEntries) *StyleBuilder {
- for ttype, entry := range entries {
- s.entries[ttype] = entry
- }
- return s
-}
-
-func (s *StyleBuilder) Get(ttype TokenType) StyleEntry {
- // This is less than ideal, but it's the price for having to check errors on each Add().
- entry, _ := ParseStyleEntry(s.entries[ttype])
- return entry.Inherit(s.parent.Get(ttype))
-}
-
-// Add an entry to the Style map.
-//
-// See http://pygments.org/docs/styles/#style-rules for details.
-func (s *StyleBuilder) Add(ttype TokenType, entry string) *StyleBuilder { // nolint: gocyclo
- s.entries[ttype] = entry
- return s
-}
-
-func (s *StyleBuilder) AddEntry(ttype TokenType, entry StyleEntry) *StyleBuilder {
- s.entries[ttype] = entry.String()
- return s
-}
-
-func (s *StyleBuilder) Build() (*Style, error) {
- style := &Style{
- Name: s.name,
- entries: map[TokenType]StyleEntry{},
- parent: s.parent,
- }
- for ttype, descriptor := range s.entries {
- entry, err := ParseStyleEntry(descriptor)
- if err != nil {
- return nil, fmt.Errorf("invalid entry for %s: %s", ttype, err)
- }
- style.entries[ttype] = entry
- }
- return style, nil
-}
-
-// StyleEntries mapping TokenType to colour definition.
-type StyleEntries map[TokenType]string
-
-// NewStyle creates a new style definition.
-func NewStyle(name string, entries StyleEntries) (*Style, error) {
- return NewStyleBuilder(name).AddAll(entries).Build()
-}
-
-// MustNewStyle creates a new style or panics.
-func MustNewStyle(name string, entries StyleEntries) *Style {
- style, err := NewStyle(name, entries)
- if err != nil {
- panic(err)
- }
- return style
-}
-
-// A Style definition.
-//
-// See http://pygments.org/docs/styles/ for details. Semantics are intended to be identical.
-type Style struct {
- Name string
- entries map[TokenType]StyleEntry
- parent *Style
-}
-
-// Types that are styled.
-func (s *Style) Types() []TokenType {
- dedupe := map[TokenType]bool{}
- for tt := range s.entries {
- dedupe[tt] = true
- }
- if s.parent != nil {
- for _, tt := range s.parent.Types() {
- dedupe[tt] = true
- }
- }
- out := make([]TokenType, 0, len(dedupe))
- for tt := range dedupe {
- out = append(out, tt)
- }
- return out
-}
-
-// Builder creates a mutable builder from this Style.
-//
-// The builder can then be safely modified. This is a cheap operation.
-func (s *Style) Builder() *StyleBuilder {
- return &StyleBuilder{
- name: s.Name,
- entries: map[TokenType]string{},
- parent: s,
- }
-}
-
-// Has checks if an exact style entry match exists for a token type.
-//
-// This is distinct from Get() which will merge parent tokens.
-func (s *Style) Has(ttype TokenType) bool {
- return !s.get(ttype).IsZero() || s.synthesisable(ttype)
-}
-
-// Get a style entry. Will try sub-category or category if an exact match is not found, and
-// finally return the Background.
-func (s *Style) Get(ttype TokenType) StyleEntry {
- return s.get(ttype).Inherit(
- s.get(Background),
- s.get(Text),
- s.get(ttype.Category()),
- s.get(ttype.SubCategory()))
-}
-
-func (s *Style) get(ttype TokenType) StyleEntry {
- out := s.entries[ttype]
- if out.IsZero() && s.parent != nil {
- return s.parent.get(ttype)
- }
- if out.IsZero() && s.synthesisable(ttype) {
- out = s.synthesise(ttype)
- }
- return out
-}
-
-func (s *Style) synthesise(ttype TokenType) StyleEntry {
- bg := s.get(Background)
- text := StyleEntry{Colour: bg.Colour}
- text.Colour = text.Colour.BrightenOrDarken(0.5)
-
- switch ttype {
- // If we don't have a line highlight colour, make one that is 10% brighter/darker than the background.
- case LineHighlight:
- return StyleEntry{Background: bg.Background.BrightenOrDarken(0.1)}
-
- // If we don't have line numbers, use the text colour but 20% brighter/darker
- case LineNumbers, LineNumbersTable:
- return text
-
- default:
- return StyleEntry{}
- }
-}
-
-func (s *Style) synthesisable(ttype TokenType) bool {
- return ttype == LineHighlight || ttype == LineNumbers || ttype == LineNumbersTable
-}
-
-// ParseStyleEntry parses a Pygments style entry.
-func ParseStyleEntry(entry string) (StyleEntry, error) { // nolint: gocyclo
- out := StyleEntry{}
- parts := strings.Fields(entry)
- for _, part := range parts {
- switch {
- case part == "italic":
- out.Italic = Yes
- case part == "noitalic":
- out.Italic = No
- case part == "bold":
- out.Bold = Yes
- case part == "nobold":
- out.Bold = No
- case part == "underline":
- out.Underline = Yes
- case part == "nounderline":
- out.Underline = No
- case part == "inherit":
- out.NoInherit = false
- case part == "noinherit":
- out.NoInherit = true
- case part == "bg:":
- out.Background = 0
- case strings.HasPrefix(part, "bg:#"):
- out.Background = ParseColour(part[3:])
- if !out.Background.IsSet() {
- return StyleEntry{}, fmt.Errorf("invalid background colour %q", part)
- }
- case strings.HasPrefix(part, "border:#"):
- out.Border = ParseColour(part[7:])
- if !out.Border.IsSet() {
- return StyleEntry{}, fmt.Errorf("invalid border colour %q", part)
- }
- case strings.HasPrefix(part, "#"):
- out.Colour = ParseColour(part)
- if !out.Colour.IsSet() {
- return StyleEntry{}, fmt.Errorf("invalid colour %q", part)
- }
- default:
- return StyleEntry{}, fmt.Errorf("unknown style element %q", part)
- }
- }
- return out, nil
-}
diff --git a/vendor/github.com/alecthomas/chroma/styles/abap.go b/vendor/github.com/alecthomas/chroma/styles/abap.go
deleted file mode 100644
index b6d07fb..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/abap.go
+++ /dev/null
@@ -1,18 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// Abap style.
-var Abap = Register(chroma.MustNewStyle("abap", chroma.StyleEntries{
- chroma.Comment: "italic #888",
- chroma.CommentSpecial: "#888",
- chroma.Keyword: "#00f",
- chroma.OperatorWord: "#00f",
- chroma.Name: "#000",
- chroma.LiteralNumber: "#3af",
- chroma.LiteralString: "#5a2",
- chroma.Error: "#F00",
- chroma.Background: " bg:#ffffff",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/algol.go b/vendor/github.com/alecthomas/chroma/styles/algol.go
deleted file mode 100644
index 1e8a7b4..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/algol.go
+++ /dev/null
@@ -1,25 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// Algol style.
-var Algol = Register(chroma.MustNewStyle("algol", chroma.StyleEntries{
- chroma.Comment: "italic #888",
- chroma.CommentPreproc: "bold noitalic #888",
- chroma.CommentSpecial: "bold noitalic #888",
- chroma.Keyword: "underline bold",
- chroma.KeywordDeclaration: "italic",
- chroma.NameBuiltin: "bold italic",
- chroma.NameBuiltinPseudo: "bold italic",
- chroma.NameNamespace: "bold italic #666",
- chroma.NameClass: "bold italic #666",
- chroma.NameFunction: "bold italic #666",
- chroma.NameVariable: "bold italic #666",
- chroma.NameConstant: "bold italic #666",
- chroma.OperatorWord: "bold",
- chroma.LiteralString: "italic #666",
- chroma.Error: "border:#FF0000",
- chroma.Background: " bg:#ffffff",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/algol_nu.go b/vendor/github.com/alecthomas/chroma/styles/algol_nu.go
deleted file mode 100644
index f8c6f17..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/algol_nu.go
+++ /dev/null
@@ -1,25 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// AlgolNu style.
-var AlgolNu = Register(chroma.MustNewStyle("algol_nu", chroma.StyleEntries{
- chroma.Comment: "italic #888",
- chroma.CommentPreproc: "bold noitalic #888",
- chroma.CommentSpecial: "bold noitalic #888",
- chroma.Keyword: "bold",
- chroma.KeywordDeclaration: "italic",
- chroma.NameBuiltin: "bold italic",
- chroma.NameBuiltinPseudo: "bold italic",
- chroma.NameNamespace: "bold italic #666",
- chroma.NameClass: "bold italic #666",
- chroma.NameFunction: "bold italic #666",
- chroma.NameVariable: "bold italic #666",
- chroma.NameConstant: "bold italic #666",
- chroma.OperatorWord: "bold",
- chroma.LiteralString: "italic #666",
- chroma.Error: "border:#FF0000",
- chroma.Background: " bg:#ffffff",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/api.go b/vendor/github.com/alecthomas/chroma/styles/api.go
deleted file mode 100644
index f3ce673..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/api.go
+++ /dev/null
@@ -1,37 +0,0 @@
-package styles
-
-import (
- "sort"
-
- "github.com/alecthomas/chroma"
-)
-
-// Registry of Styles.
-var Registry = map[string]*chroma.Style{}
-
-// Fallback style. Reassign to change the default fallback style.
-var Fallback = SwapOff
-
-// Register a chroma.Style.
-func Register(style *chroma.Style) *chroma.Style {
- Registry[style.Name] = style
- return style
-}
-
-// Names of all available styles.
-func Names() []string {
- out := []string{}
- for name := range Registry {
- out = append(out, name)
- }
- sort.Strings(out)
- return out
-}
-
-// Get named style, or Fallback.
-func Get(name string) *chroma.Style {
- if style, ok := Registry[name]; ok {
- return style
- }
- return Fallback
-}
diff --git a/vendor/github.com/alecthomas/chroma/styles/arduino.go b/vendor/github.com/alecthomas/chroma/styles/arduino.go
deleted file mode 100644
index 9e48fb4..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/arduino.go
+++ /dev/null
@@ -1,25 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// Arduino style.
-var Arduino = Register(chroma.MustNewStyle("arduino", chroma.StyleEntries{
- chroma.Error: "#a61717",
- chroma.Comment: "#95a5a6",
- chroma.CommentPreproc: "#728E00",
- chroma.Keyword: "#728E00",
- chroma.KeywordConstant: "#00979D",
- chroma.KeywordPseudo: "#00979D",
- chroma.KeywordReserved: "#00979D",
- chroma.KeywordType: "#00979D",
- chroma.Operator: "#728E00",
- chroma.Name: "#434f54",
- chroma.NameBuiltin: "#728E00",
- chroma.NameFunction: "#D35400",
- chroma.NameOther: "#728E00",
- chroma.LiteralNumber: "#8A7B52",
- chroma.LiteralString: "#7F8C8D",
- chroma.Background: " bg:#ffffff",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/autumn.go b/vendor/github.com/alecthomas/chroma/styles/autumn.go
deleted file mode 100644
index 3966372..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/autumn.go
+++ /dev/null
@@ -1,43 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// Autumn style.
-var Autumn = Register(chroma.MustNewStyle("autumn", chroma.StyleEntries{
- chroma.TextWhitespace: "#bbbbbb",
- chroma.Comment: "italic #aaaaaa",
- chroma.CommentPreproc: "noitalic #4c8317",
- chroma.CommentSpecial: "italic #0000aa",
- chroma.Keyword: "#0000aa",
- chroma.KeywordType: "#00aaaa",
- chroma.OperatorWord: "#0000aa",
- chroma.NameBuiltin: "#00aaaa",
- chroma.NameFunction: "#00aa00",
- chroma.NameClass: "underline #00aa00",
- chroma.NameNamespace: "underline #00aaaa",
- chroma.NameVariable: "#aa0000",
- chroma.NameConstant: "#aa0000",
- chroma.NameEntity: "bold #800",
- chroma.NameAttribute: "#1e90ff",
- chroma.NameTag: "bold #1e90ff",
- chroma.NameDecorator: "#888888",
- chroma.LiteralString: "#aa5500",
- chroma.LiteralStringSymbol: "#0000aa",
- chroma.LiteralStringRegex: "#009999",
- chroma.LiteralNumber: "#009999",
- chroma.GenericHeading: "bold #000080",
- chroma.GenericSubheading: "bold #800080",
- chroma.GenericDeleted: "#aa0000",
- chroma.GenericInserted: "#00aa00",
- chroma.GenericError: "#aa0000",
- chroma.GenericEmph: "italic",
- chroma.GenericStrong: "bold",
- chroma.GenericPrompt: "#555555",
- chroma.GenericOutput: "#888888",
- chroma.GenericTraceback: "#aa0000",
- chroma.GenericUnderline: "underline",
- chroma.Error: "#F00 bg:#FAA",
- chroma.Background: " bg:#ffffff",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/base16-snazzy.go b/vendor/github.com/alecthomas/chroma/styles/base16-snazzy.go
deleted file mode 100644
index 160c75b..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/base16-snazzy.go
+++ /dev/null
@@ -1,81 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// Base16Snazzy style
-var Base16Snazzy = Register(chroma.MustNewStyle("base16-snazzy", chroma.StyleEntries{
- chroma.Comment: "#78787e",
- chroma.CommentHashbang: "#78787e",
- chroma.CommentMultiline: "#78787e",
- chroma.CommentPreproc: "#78787e",
- chroma.CommentSingle: "#78787e",
- chroma.CommentSpecial: "#78787e",
- chroma.Generic: "#e2e4e5",
- chroma.GenericDeleted: "#ff5c57",
- chroma.GenericEmph: "#e2e4e5 underline",
- chroma.GenericError: "#ff5c57",
- chroma.GenericHeading: "#e2e4e5 bold",
- chroma.GenericInserted: "#e2e4e5 bold",
- chroma.GenericOutput: "#43454f",
- chroma.GenericPrompt: "#e2e4e5",
- chroma.GenericStrong: "#e2e4e5 italic",
- chroma.GenericSubheading: "#e2e4e5 bold",
- chroma.GenericTraceback: "#e2e4e5",
- chroma.GenericUnderline: "underline",
- chroma.Error: "#ff5c57",
- chroma.Keyword: "#ff6ac1",
- chroma.KeywordConstant: "#ff6ac1",
- chroma.KeywordDeclaration: "#ff5c57",
- chroma.KeywordNamespace: "#ff6ac1",
- chroma.KeywordPseudo: "#ff6ac1",
- chroma.KeywordReserved: "#ff6ac1",
- chroma.KeywordType: "#9aedfe",
- chroma.Literal: "#e2e4e5",
- chroma.LiteralDate: "#e2e4e5",
- chroma.Name: "#e2e4e5",
- chroma.NameAttribute: "#57c7ff",
- chroma.NameBuiltin: "#ff5c57",
- chroma.NameBuiltinPseudo: "#e2e4e5",
- chroma.NameClass: "#f3f99d",
- chroma.NameConstant: "#ff9f43",
- chroma.NameDecorator: "#ff9f43",
- chroma.NameEntity: "#e2e4e5",
- chroma.NameException: "#e2e4e5",
- chroma.NameFunction: "#57c7ff",
- chroma.NameLabel: "#ff5c57",
- chroma.NameNamespace: "#e2e4e5",
- chroma.NameOther: "#e2e4e5",
- chroma.NameTag: "#ff6ac1",
- chroma.NameVariable: "#ff5c57",
- chroma.NameVariableClass: "#ff5c57",
- chroma.NameVariableGlobal: "#ff5c57",
- chroma.NameVariableInstance: "#ff5c57",
- chroma.LiteralNumber: "#ff9f43",
- chroma.LiteralNumberBin: "#ff9f43",
- chroma.LiteralNumberFloat: "#ff9f43",
- chroma.LiteralNumberHex: "#ff9f43",
- chroma.LiteralNumberInteger: "#ff9f43",
- chroma.LiteralNumberIntegerLong: "#ff9f43",
- chroma.LiteralNumberOct: "#ff9f43",
- chroma.Operator: "#ff6ac1",
- chroma.OperatorWord: "#ff6ac1",
- chroma.Other: "#e2e4e5",
- chroma.Punctuation: "#e2e4e5",
- chroma.LiteralString: "#5af78e",
- chroma.LiteralStringBacktick: "#5af78e",
- chroma.LiteralStringChar: "#5af78e",
- chroma.LiteralStringDoc: "#5af78e",
- chroma.LiteralStringDouble: "#5af78e",
- chroma.LiteralStringEscape: "#5af78e",
- chroma.LiteralStringHeredoc: "#5af78e",
- chroma.LiteralStringInterpol: "#5af78e",
- chroma.LiteralStringOther: "#5af78e",
- chroma.LiteralStringRegex: "#5af78e",
- chroma.LiteralStringSingle: "#5af78e",
- chroma.LiteralStringSymbol: "#5af78e",
- chroma.Text: "#e2e4e5",
- chroma.TextWhitespace: "#e2e4e5",
- chroma.Background: " bg:#282a36",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/borland.go b/vendor/github.com/alecthomas/chroma/styles/borland.go
deleted file mode 100644
index 9c0fff6..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/borland.go
+++ /dev/null
@@ -1,33 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// Borland style.
-var Borland = Register(chroma.MustNewStyle("borland", chroma.StyleEntries{
- chroma.TextWhitespace: "#bbbbbb",
- chroma.Comment: "italic #008800",
- chroma.CommentPreproc: "noitalic #008080",
- chroma.CommentSpecial: "noitalic bold",
- chroma.LiteralString: "#0000FF",
- chroma.LiteralStringChar: "#800080",
- chroma.LiteralNumber: "#0000FF",
- chroma.Keyword: "bold #000080",
- chroma.OperatorWord: "bold",
- chroma.NameTag: "bold #000080",
- chroma.NameAttribute: "#FF0000",
- chroma.GenericHeading: "#999999",
- chroma.GenericSubheading: "#aaaaaa",
- chroma.GenericDeleted: "bg:#ffdddd #000000",
- chroma.GenericInserted: "bg:#ddffdd #000000",
- chroma.GenericError: "#aa0000",
- chroma.GenericEmph: "italic",
- chroma.GenericStrong: "bold",
- chroma.GenericPrompt: "#555555",
- chroma.GenericOutput: "#888888",
- chroma.GenericTraceback: "#aa0000",
- chroma.GenericUnderline: "underline",
- chroma.Error: "bg:#e3d2d2 #a61717",
- chroma.Background: " bg:#ffffff",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/bw.go b/vendor/github.com/alecthomas/chroma/styles/bw.go
deleted file mode 100644
index 3e800d5..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/bw.go
+++ /dev/null
@@ -1,30 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// BlackWhite style.
-var BlackWhite = Register(chroma.MustNewStyle("bw", chroma.StyleEntries{
- chroma.Comment: "italic",
- chroma.CommentPreproc: "noitalic",
- chroma.Keyword: "bold",
- chroma.KeywordPseudo: "nobold",
- chroma.KeywordType: "nobold",
- chroma.OperatorWord: "bold",
- chroma.NameClass: "bold",
- chroma.NameNamespace: "bold",
- chroma.NameException: "bold",
- chroma.NameEntity: "bold",
- chroma.NameTag: "bold",
- chroma.LiteralString: "italic",
- chroma.LiteralStringInterpol: "bold",
- chroma.LiteralStringEscape: "bold",
- chroma.GenericHeading: "bold",
- chroma.GenericSubheading: "bold",
- chroma.GenericEmph: "italic",
- chroma.GenericStrong: "bold",
- chroma.GenericPrompt: "bold",
- chroma.Error: "border:#FF0000",
- chroma.Background: " bg:#ffffff",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/colorful.go b/vendor/github.com/alecthomas/chroma/styles/colorful.go
deleted file mode 100644
index dc77c5b..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/colorful.go
+++ /dev/null
@@ -1,59 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// Colorful style.
-var Colorful = Register(chroma.MustNewStyle("colorful", chroma.StyleEntries{
- chroma.TextWhitespace: "#bbbbbb",
- chroma.Comment: "#888",
- chroma.CommentPreproc: "#579",
- chroma.CommentSpecial: "bold #cc0000",
- chroma.Keyword: "bold #080",
- chroma.KeywordPseudo: "#038",
- chroma.KeywordType: "#339",
- chroma.Operator: "#333",
- chroma.OperatorWord: "bold #000",
- chroma.NameBuiltin: "#007020",
- chroma.NameFunction: "bold #06B",
- chroma.NameClass: "bold #B06",
- chroma.NameNamespace: "bold #0e84b5",
- chroma.NameException: "bold #F00",
- chroma.NameVariable: "#963",
- chroma.NameVariableInstance: "#33B",
- chroma.NameVariableClass: "#369",
- chroma.NameVariableGlobal: "bold #d70",
- chroma.NameConstant: "bold #036",
- chroma.NameLabel: "bold #970",
- chroma.NameEntity: "bold #800",
- chroma.NameAttribute: "#00C",
- chroma.NameTag: "#070",
- chroma.NameDecorator: "bold #555",
- chroma.LiteralString: "bg:#fff0f0",
- chroma.LiteralStringChar: "#04D bg:",
- chroma.LiteralStringDoc: "#D42 bg:",
- chroma.LiteralStringInterpol: "bg:#eee",
- chroma.LiteralStringEscape: "bold #666",
- chroma.LiteralStringRegex: "bg:#fff0ff #000",
- chroma.LiteralStringSymbol: "#A60 bg:",
- chroma.LiteralStringOther: "#D20",
- chroma.LiteralNumber: "bold #60E",
- chroma.LiteralNumberInteger: "bold #00D",
- chroma.LiteralNumberFloat: "bold #60E",
- chroma.LiteralNumberHex: "bold #058",
- chroma.LiteralNumberOct: "bold #40E",
- chroma.GenericHeading: "bold #000080",
- chroma.GenericSubheading: "bold #800080",
- chroma.GenericDeleted: "#A00000",
- chroma.GenericInserted: "#00A000",
- chroma.GenericError: "#FF0000",
- chroma.GenericEmph: "italic",
- chroma.GenericStrong: "bold",
- chroma.GenericPrompt: "bold #c65d09",
- chroma.GenericOutput: "#888",
- chroma.GenericTraceback: "#04D",
- chroma.GenericUnderline: "underline",
- chroma.Error: "#F00 bg:#FAA",
- chroma.Background: " bg:#ffffff",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/doom-one.go b/vendor/github.com/alecthomas/chroma/styles/doom-one.go
deleted file mode 100644
index 6450455..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/doom-one.go
+++ /dev/null
@@ -1,58 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// Doom One style. Inspired by Atom One and Doom Emacs's Atom One theme
-var DoomOne = Register(chroma.MustNewStyle("doom-one", chroma.StyleEntries{
- chroma.Text: "#b0c4de",
- chroma.Error: "#b0c4de",
- chroma.Comment: "italic #8a93a5",
- chroma.CommentHashbang: "bold",
- chroma.Keyword: "#c678dd",
- chroma.KeywordType: "#ef8383",
- chroma.KeywordConstant: "bold #b756ff",
- chroma.Operator: "#c7bf54",
- chroma.OperatorWord: "bold #b756ff",
- chroma.Punctuation: "#b0c4de",
- chroma.Name: "#c1abea",
- chroma.NameAttribute: "#b3d23c",
- chroma.NameBuiltin: "#ef8383",
- chroma.NameClass: "#76a9f9",
- chroma.NameConstant: "bold #b756ff",
- chroma.NameDecorator: "#e5c07b",
- chroma.NameEntity: "#bda26f",
- chroma.NameException: "bold #fd7474",
- chroma.NameFunction: "#00b1f7",
- chroma.NameProperty: "#cebc3a",
- chroma.NameLabel: "#f5a40d",
- chroma.NameNamespace: "#76a9f9",
- chroma.NameTag: "#e06c75",
- chroma.NameVariable: "#DCAEEA",
- chroma.NameVariableGlobal: "bold #DCAEEA",
- chroma.NameVariableInstance: "#e06c75",
- chroma.Literal: "#98c379",
- chroma.Number: "#d19a66",
- chroma.String: "#98c379",
- chroma.StringDoc: "#7e97c3",
- chroma.StringDouble: "#63c381",
- chroma.StringEscape: "bold #d26464",
- chroma.StringHeredoc: "#98c379",
- chroma.StringInterpol: "#98c379",
- chroma.StringOther: "#70b33f",
- chroma.StringRegex: "#56b6c2",
- chroma.StringSingle: "#98c379",
- chroma.StringSymbol: "#56b6c2",
- chroma.Generic: "#b0c4de",
- chroma.GenericEmph: "italic",
- chroma.GenericHeading: "bold #a2cbff",
- chroma.GenericInserted: "#a6e22e",
- chroma.GenericOutput: "#a6e22e",
- chroma.GenericUnderline: "underline",
- chroma.GenericPrompt: "#a6e22e",
- chroma.GenericStrong: "bold",
- chroma.GenericSubheading: "#a2cbff",
- chroma.GenericTraceback: "#a2cbff",
- chroma.Background: "#b0c4de bg:#282c34",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/doom-one2.go b/vendor/github.com/alecthomas/chroma/styles/doom-one2.go
deleted file mode 100644
index 4654173..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/doom-one2.go
+++ /dev/null
@@ -1,71 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// Doom One 2 style. Inspired by Atom One and Doom Emacs's Atom One theme
-var DoomOne2 = Register(chroma.MustNewStyle("doom-one2", chroma.StyleEntries{
- chroma.Text: "#b0c4de",
- chroma.Error: "#b0c4de",
- chroma.Comment: "italic #8a93a5",
- chroma.CommentHashbang: "bold",
- chroma.Keyword: "#76a9f9",
- chroma.KeywordConstant: "#e5c07b",
- chroma.KeywordType: "#e5c07b",
- chroma.Operator: "#54b1c7",
- chroma.OperatorWord: "bold #b756ff",
- chroma.Punctuation: "#abb2bf",
- chroma.Name: "#aa89ea",
- chroma.NameAttribute: "#cebc3a",
- chroma.NameBuiltin: "#e5c07b",
- chroma.NameClass: "#ca72ff",
- chroma.NameConstant: "bold",
- chroma.NameDecorator: "#e5c07b",
- chroma.NameEntity: "#bda26f",
- chroma.NameException: "bold #fd7474",
- chroma.NameFunction: "#00b1f7",
- chroma.NameProperty: "#cebc3a",
- chroma.NameLabel: "#f5a40d",
- chroma.NameNamespace: "#ca72ff",
- chroma.NameTag: "#76a9f9",
- chroma.NameVariable: "#DCAEEA",
- chroma.NameVariableClass: "#DCAEEA",
- chroma.NameVariableGlobal: "bold #DCAEEA",
- chroma.NameVariableInstance: "#e06c75",
- chroma.NameVariableMagic: "#DCAEEA",
- chroma.Literal: "#98c379",
- chroma.LiteralDate: "#98c379",
- chroma.Number: "#d19a66",
- chroma.NumberBin: "#d19a66",
- chroma.NumberFloat: "#d19a66",
- chroma.NumberHex: "#d19a66",
- chroma.NumberInteger: "#d19a66",
- chroma.NumberIntegerLong: "#d19a66",
- chroma.NumberOct: "#d19a66",
- chroma.String: "#98c379",
- chroma.StringAffix: "#98c379",
- chroma.StringBacktick: "#98c379",
- chroma.StringDelimiter: "#98c379",
- chroma.StringDoc: "#7e97c3",
- chroma.StringDouble: "#63c381",
- chroma.StringEscape: "bold #d26464",
- chroma.StringHeredoc: "#98c379",
- chroma.StringInterpol: "#98c379",
- chroma.StringOther: "#70b33f",
- chroma.StringRegex: "#56b6c2",
- chroma.StringSingle: "#98c379",
- chroma.StringSymbol: "#56b6c2",
- chroma.Generic: "#b0c4de",
- chroma.GenericDeleted: "#b0c4de",
- chroma.GenericEmph: "italic",
- chroma.GenericHeading: "bold #a2cbff",
- chroma.GenericInserted: "#a6e22e",
- chroma.GenericOutput: "#a6e22e",
- chroma.GenericUnderline: "underline",
- chroma.GenericPrompt: "#a6e22e",
- chroma.GenericStrong: "bold",
- chroma.GenericSubheading: "#a2cbff",
- chroma.GenericTraceback: "#a2cbff",
- chroma.Background: "#b0c4de bg:#282c34",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/dracula.go b/vendor/github.com/alecthomas/chroma/styles/dracula.go
deleted file mode 100644
index d1542f2..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/dracula.go
+++ /dev/null
@@ -1,81 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// Dracula Style
-var Dracula = Register(chroma.MustNewStyle("dracula", chroma.StyleEntries{
- chroma.Comment: "#6272a4",
- chroma.CommentHashbang: "#6272a4",
- chroma.CommentMultiline: "#6272a4",
- chroma.CommentPreproc: "#ff79c6",
- chroma.CommentSingle: "#6272a4",
- chroma.CommentSpecial: "#6272a4",
- chroma.Generic: "#f8f8f2",
- chroma.GenericDeleted: "#ff5555",
- chroma.GenericEmph: "#f8f8f2 underline",
- chroma.GenericError: "#f8f8f2",
- chroma.GenericHeading: "#f8f8f2 bold",
- chroma.GenericInserted: "#50fa7b bold",
- chroma.GenericOutput: "#44475a",
- chroma.GenericPrompt: "#f8f8f2",
- chroma.GenericStrong: "#f8f8f2",
- chroma.GenericSubheading: "#f8f8f2 bold",
- chroma.GenericTraceback: "#f8f8f2",
- chroma.GenericUnderline: "underline",
- chroma.Error: "#f8f8f2",
- chroma.Keyword: "#ff79c6",
- chroma.KeywordConstant: "#ff79c6",
- chroma.KeywordDeclaration: "#8be9fd italic",
- chroma.KeywordNamespace: "#ff79c6",
- chroma.KeywordPseudo: "#ff79c6",
- chroma.KeywordReserved: "#ff79c6",
- chroma.KeywordType: "#8be9fd",
- chroma.Literal: "#f8f8f2",
- chroma.LiteralDate: "#f8f8f2",
- chroma.Name: "#f8f8f2",
- chroma.NameAttribute: "#50fa7b",
- chroma.NameBuiltin: "#8be9fd italic",
- chroma.NameBuiltinPseudo: "#f8f8f2",
- chroma.NameClass: "#50fa7b",
- chroma.NameConstant: "#f8f8f2",
- chroma.NameDecorator: "#f8f8f2",
- chroma.NameEntity: "#f8f8f2",
- chroma.NameException: "#f8f8f2",
- chroma.NameFunction: "#50fa7b",
- chroma.NameLabel: "#8be9fd italic",
- chroma.NameNamespace: "#f8f8f2",
- chroma.NameOther: "#f8f8f2",
- chroma.NameTag: "#ff79c6",
- chroma.NameVariable: "#8be9fd italic",
- chroma.NameVariableClass: "#8be9fd italic",
- chroma.NameVariableGlobal: "#8be9fd italic",
- chroma.NameVariableInstance: "#8be9fd italic",
- chroma.LiteralNumber: "#bd93f9",
- chroma.LiteralNumberBin: "#bd93f9",
- chroma.LiteralNumberFloat: "#bd93f9",
- chroma.LiteralNumberHex: "#bd93f9",
- chroma.LiteralNumberInteger: "#bd93f9",
- chroma.LiteralNumberIntegerLong: "#bd93f9",
- chroma.LiteralNumberOct: "#bd93f9",
- chroma.Operator: "#ff79c6",
- chroma.OperatorWord: "#ff79c6",
- chroma.Other: "#f8f8f2",
- chroma.Punctuation: "#f8f8f2",
- chroma.LiteralString: "#f1fa8c",
- chroma.LiteralStringBacktick: "#f1fa8c",
- chroma.LiteralStringChar: "#f1fa8c",
- chroma.LiteralStringDoc: "#f1fa8c",
- chroma.LiteralStringDouble: "#f1fa8c",
- chroma.LiteralStringEscape: "#f1fa8c",
- chroma.LiteralStringHeredoc: "#f1fa8c",
- chroma.LiteralStringInterpol: "#f1fa8c",
- chroma.LiteralStringOther: "#f1fa8c",
- chroma.LiteralStringRegex: "#f1fa8c",
- chroma.LiteralStringSingle: "#f1fa8c",
- chroma.LiteralStringSymbol: "#f1fa8c",
- chroma.Text: "#f8f8f2",
- chroma.TextWhitespace: "#f8f8f2",
- chroma.Background: " bg:#282a36",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/emacs.go b/vendor/github.com/alecthomas/chroma/styles/emacs.go
deleted file mode 100644
index 4835abd..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/emacs.go
+++ /dev/null
@@ -1,51 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// Emacs style.
-var Emacs = Register(chroma.MustNewStyle("emacs", chroma.StyleEntries{
- chroma.TextWhitespace: "#bbbbbb",
- chroma.Comment: "italic #008800",
- chroma.CommentPreproc: "noitalic",
- chroma.CommentSpecial: "noitalic bold",
- chroma.Keyword: "bold #AA22FF",
- chroma.KeywordPseudo: "nobold",
- chroma.KeywordType: "bold #00BB00",
- chroma.Operator: "#666666",
- chroma.OperatorWord: "bold #AA22FF",
- chroma.NameBuiltin: "#AA22FF",
- chroma.NameFunction: "#00A000",
- chroma.NameClass: "#0000FF",
- chroma.NameNamespace: "bold #0000FF",
- chroma.NameException: "bold #D2413A",
- chroma.NameVariable: "#B8860B",
- chroma.NameConstant: "#880000",
- chroma.NameLabel: "#A0A000",
- chroma.NameEntity: "bold #999999",
- chroma.NameAttribute: "#BB4444",
- chroma.NameTag: "bold #008000",
- chroma.NameDecorator: "#AA22FF",
- chroma.LiteralString: "#BB4444",
- chroma.LiteralStringDoc: "italic",
- chroma.LiteralStringInterpol: "bold #BB6688",
- chroma.LiteralStringEscape: "bold #BB6622",
- chroma.LiteralStringRegex: "#BB6688",
- chroma.LiteralStringSymbol: "#B8860B",
- chroma.LiteralStringOther: "#008000",
- chroma.LiteralNumber: "#666666",
- chroma.GenericHeading: "bold #000080",
- chroma.GenericSubheading: "bold #800080",
- chroma.GenericDeleted: "#A00000",
- chroma.GenericInserted: "#00A000",
- chroma.GenericError: "#FF0000",
- chroma.GenericEmph: "italic",
- chroma.GenericStrong: "bold",
- chroma.GenericPrompt: "bold #000080",
- chroma.GenericOutput: "#888",
- chroma.GenericTraceback: "#04D",
- chroma.GenericUnderline: "underline",
- chroma.Error: "border:#FF0000",
- chroma.Background: " bg:#f8f8f8",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/friendly.go b/vendor/github.com/alecthomas/chroma/styles/friendly.go
deleted file mode 100644
index ad02341..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/friendly.go
+++ /dev/null
@@ -1,51 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// Friendly style.
-var Friendly = Register(chroma.MustNewStyle("friendly", chroma.StyleEntries{
- chroma.TextWhitespace: "#bbbbbb",
- chroma.Comment: "italic #60a0b0",
- chroma.CommentPreproc: "noitalic #007020",
- chroma.CommentSpecial: "noitalic bg:#fff0f0",
- chroma.Keyword: "bold #007020",
- chroma.KeywordPseudo: "nobold",
- chroma.KeywordType: "nobold #902000",
- chroma.Operator: "#666666",
- chroma.OperatorWord: "bold #007020",
- chroma.NameBuiltin: "#007020",
- chroma.NameFunction: "#06287e",
- chroma.NameClass: "bold #0e84b5",
- chroma.NameNamespace: "bold #0e84b5",
- chroma.NameException: "#007020",
- chroma.NameVariable: "#bb60d5",
- chroma.NameConstant: "#60add5",
- chroma.NameLabel: "bold #002070",
- chroma.NameEntity: "bold #d55537",
- chroma.NameAttribute: "#4070a0",
- chroma.NameTag: "bold #062873",
- chroma.NameDecorator: "bold #555555",
- chroma.LiteralString: "#4070a0",
- chroma.LiteralStringDoc: "italic",
- chroma.LiteralStringInterpol: "italic #70a0d0",
- chroma.LiteralStringEscape: "bold #4070a0",
- chroma.LiteralStringRegex: "#235388",
- chroma.LiteralStringSymbol: "#517918",
- chroma.LiteralStringOther: "#c65d09",
- chroma.LiteralNumber: "#40a070",
- chroma.GenericHeading: "bold #000080",
- chroma.GenericSubheading: "bold #800080",
- chroma.GenericDeleted: "#A00000",
- chroma.GenericInserted: "#00A000",
- chroma.GenericError: "#FF0000",
- chroma.GenericEmph: "italic",
- chroma.GenericStrong: "bold",
- chroma.GenericPrompt: "bold #c65d09",
- chroma.GenericOutput: "#888",
- chroma.GenericTraceback: "#04D",
- chroma.GenericUnderline: "underline",
- chroma.Error: "border:#FF0000",
- chroma.Background: " bg:#f0f0f0",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/fruity.go b/vendor/github.com/alecthomas/chroma/styles/fruity.go
deleted file mode 100644
index c2577fa..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/fruity.go
+++ /dev/null
@@ -1,26 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// Fruity style.
-var Fruity = Register(chroma.MustNewStyle("fruity", chroma.StyleEntries{
- chroma.TextWhitespace: "#888888",
- chroma.Background: "#ffffff bg:#111111",
- chroma.GenericOutput: "#444444 bg:#222222",
- chroma.Keyword: "#fb660a bold",
- chroma.KeywordPseudo: "nobold",
- chroma.LiteralNumber: "#0086f7 bold",
- chroma.NameTag: "#fb660a bold",
- chroma.NameVariable: "#fb660a",
- chroma.Comment: "#008800 bg:#0f140f italic",
- chroma.NameAttribute: "#ff0086 bold",
- chroma.LiteralString: "#0086d2",
- chroma.NameFunction: "#ff0086 bold",
- chroma.GenericHeading: "#ffffff bold",
- chroma.KeywordType: "#cdcaa9 bold",
- chroma.GenericSubheading: "#ffffff bold",
- chroma.NameConstant: "#0086d2",
- chroma.CommentPreproc: "#ff0007 bold",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/github.go b/vendor/github.com/alecthomas/chroma/styles/github.go
deleted file mode 100644
index 7ef2481..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/github.go
+++ /dev/null
@@ -1,51 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// GitHub style.
-var GitHub = Register(chroma.MustNewStyle("github", chroma.StyleEntries{
- chroma.CommentMultiline: "italic #999988",
- chroma.CommentPreproc: "bold #999999",
- chroma.CommentSingle: "italic #999988",
- chroma.CommentSpecial: "bold italic #999999",
- chroma.Comment: "italic #999988",
- chroma.Error: "bg:#e3d2d2 #a61717",
- chroma.GenericDeleted: "bg:#ffdddd #000000",
- chroma.GenericEmph: "italic #000000",
- chroma.GenericError: "#aa0000",
- chroma.GenericHeading: "#999999",
- chroma.GenericInserted: "bg:#ddffdd #000000",
- chroma.GenericOutput: "#888888",
- chroma.GenericPrompt: "#555555",
- chroma.GenericStrong: "bold",
- chroma.GenericSubheading: "#aaaaaa",
- chroma.GenericTraceback: "#aa0000",
- chroma.GenericUnderline: "underline",
- chroma.KeywordType: "bold #445588",
- chroma.Keyword: "bold #000000",
- chroma.LiteralNumber: "#009999",
- chroma.LiteralStringRegex: "#009926",
- chroma.LiteralStringSymbol: "#990073",
- chroma.LiteralString: "#d14",
- chroma.NameAttribute: "#008080",
- chroma.NameBuiltinPseudo: "#999999",
- chroma.NameBuiltin: "#0086B3",
- chroma.NameClass: "bold #445588",
- chroma.NameConstant: "#008080",
- chroma.NameDecorator: "bold #3c5d5d",
- chroma.NameEntity: "#800080",
- chroma.NameException: "bold #990000",
- chroma.NameFunction: "bold #990000",
- chroma.NameLabel: "bold #990000",
- chroma.NameNamespace: "#555555",
- chroma.NameTag: "#000080",
- chroma.NameVariableClass: "#008080",
- chroma.NameVariableGlobal: "#008080",
- chroma.NameVariableInstance: "#008080",
- chroma.NameVariable: "#008080",
- chroma.Operator: "bold #000000",
- chroma.TextWhitespace: "#bbbbbb",
- chroma.Background: " bg:#ffffff",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/hr_dark.go b/vendor/github.com/alecthomas/chroma/styles/hr_dark.go
deleted file mode 100644
index 10bb64f..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/hr_dark.go
+++ /dev/null
@@ -1,17 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// Theme based on HackerRank Dark Editor theme
-var HrDark = Register(chroma.MustNewStyle("hrdark", chroma.StyleEntries{
- chroma.Comment: "italic #828b96",
- chroma.Keyword: "#ff636f",
- chroma.OperatorWord: "#ff636f",
- chroma.Name: "#58a1dd",
- chroma.Literal: "#a6be9d",
- chroma.Operator: "#ff636f",
- chroma.Background: "#1d2432",
- chroma.Other: "#fff",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/hr_high_contrast.go b/vendor/github.com/alecthomas/chroma/styles/hr_high_contrast.go
deleted file mode 100644
index d198858..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/hr_high_contrast.go
+++ /dev/null
@@ -1,19 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// Theme based on HackerRank High Contrast Editor Theme
-var HrHighContrast = Register(chroma.MustNewStyle("hr_high_contrast", chroma.StyleEntries{
- chroma.Comment: "#5a8349",
- chroma.Keyword: "#467faf",
- chroma.OperatorWord: "#467faf",
- chroma.Name: "#ffffff",
- chroma.LiteralString: "#a87662",
- chroma.LiteralNumber: "#fff",
- chroma.LiteralStringBoolean: "#467faf",
- chroma.Operator: "#e4e400",
- chroma.Background: "#000",
- chroma.Other: "#d5d500",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/igor.go b/vendor/github.com/alecthomas/chroma/styles/igor.go
deleted file mode 100644
index 6a6d4cd..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/igor.go
+++ /dev/null
@@ -1,16 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// Igor style.
-var Igor = Register(chroma.MustNewStyle("igor", chroma.StyleEntries{
- chroma.Comment: "italic #FF0000",
- chroma.Keyword: "#0000FF",
- chroma.NameFunction: "#C34E00",
- chroma.NameDecorator: "#CC00A3",
- chroma.NameClass: "#007575",
- chroma.LiteralString: "#009C00",
- chroma.Background: " bg:#ffffff",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/lovelace.go b/vendor/github.com/alecthomas/chroma/styles/lovelace.go
deleted file mode 100644
index 074cc08..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/lovelace.go
+++ /dev/null
@@ -1,60 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// Lovelace style.
-var Lovelace = Register(chroma.MustNewStyle("lovelace", chroma.StyleEntries{
- chroma.TextWhitespace: "#a89028",
- chroma.Comment: "italic #888888",
- chroma.CommentHashbang: "#287088",
- chroma.CommentMultiline: "#888888",
- chroma.CommentPreproc: "noitalic #289870",
- chroma.Keyword: "#2838b0",
- chroma.KeywordConstant: "italic #444444",
- chroma.KeywordDeclaration: "italic",
- chroma.KeywordType: "italic",
- chroma.Operator: "#666666",
- chroma.OperatorWord: "#a848a8",
- chroma.Punctuation: "#888888",
- chroma.NameAttribute: "#388038",
- chroma.NameBuiltin: "#388038",
- chroma.NameBuiltinPseudo: "italic",
- chroma.NameClass: "#287088",
- chroma.NameConstant: "#b85820",
- chroma.NameDecorator: "#287088",
- chroma.NameEntity: "#709030",
- chroma.NameException: "#908828",
- chroma.NameFunction: "#785840",
- chroma.NameFunctionMagic: "#b85820",
- chroma.NameLabel: "#289870",
- chroma.NameNamespace: "#289870",
- chroma.NameTag: "#2838b0",
- chroma.NameVariable: "#b04040",
- chroma.NameVariableGlobal: "#908828",
- chroma.NameVariableMagic: "#b85820",
- chroma.LiteralString: "#b83838",
- chroma.LiteralStringAffix: "#444444",
- chroma.LiteralStringChar: "#a848a8",
- chroma.LiteralStringDelimiter: "#b85820",
- chroma.LiteralStringDoc: "italic #b85820",
- chroma.LiteralStringEscape: "#709030",
- chroma.LiteralStringInterpol: "underline",
- chroma.LiteralStringOther: "#a848a8",
- chroma.LiteralStringRegex: "#a848a8",
- chroma.LiteralNumber: "#444444",
- chroma.GenericDeleted: "#c02828",
- chroma.GenericEmph: "italic",
- chroma.GenericError: "#c02828",
- chroma.GenericHeading: "#666666",
- chroma.GenericSubheading: "#444444",
- chroma.GenericInserted: "#388038",
- chroma.GenericOutput: "#666666",
- chroma.GenericPrompt: "#444444",
- chroma.GenericStrong: "bold",
- chroma.GenericTraceback: "#2838b0",
- chroma.GenericUnderline: "underline",
- chroma.Error: "bg:#a848a8",
- chroma.Background: " bg:#ffffff",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/manni.go b/vendor/github.com/alecthomas/chroma/styles/manni.go
deleted file mode 100644
index 9942e7d..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/manni.go
+++ /dev/null
@@ -1,51 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// Manni style.
-var Manni = Register(chroma.MustNewStyle("manni", chroma.StyleEntries{
- chroma.TextWhitespace: "#bbbbbb",
- chroma.Comment: "italic #0099FF",
- chroma.CommentPreproc: "noitalic #009999",
- chroma.CommentSpecial: "bold",
- chroma.Keyword: "bold #006699",
- chroma.KeywordPseudo: "nobold",
- chroma.KeywordType: "#007788",
- chroma.Operator: "#555555",
- chroma.OperatorWord: "bold #000000",
- chroma.NameBuiltin: "#336666",
- chroma.NameFunction: "#CC00FF",
- chroma.NameClass: "bold #00AA88",
- chroma.NameNamespace: "bold #00CCFF",
- chroma.NameException: "bold #CC0000",
- chroma.NameVariable: "#003333",
- chroma.NameConstant: "#336600",
- chroma.NameLabel: "#9999FF",
- chroma.NameEntity: "bold #999999",
- chroma.NameAttribute: "#330099",
- chroma.NameTag: "bold #330099",
- chroma.NameDecorator: "#9999FF",
- chroma.LiteralString: "#CC3300",
- chroma.LiteralStringDoc: "italic",
- chroma.LiteralStringInterpol: "#AA0000",
- chroma.LiteralStringEscape: "bold #CC3300",
- chroma.LiteralStringRegex: "#33AAAA",
- chroma.LiteralStringSymbol: "#FFCC33",
- chroma.LiteralStringOther: "#CC3300",
- chroma.LiteralNumber: "#FF6600",
- chroma.GenericHeading: "bold #003300",
- chroma.GenericSubheading: "bold #003300",
- chroma.GenericDeleted: "border:#CC0000 bg:#FFCCCC",
- chroma.GenericInserted: "border:#00CC00 bg:#CCFFCC",
- chroma.GenericError: "#FF0000",
- chroma.GenericEmph: "italic",
- chroma.GenericStrong: "bold",
- chroma.GenericPrompt: "bold #000099",
- chroma.GenericOutput: "#AAAAAA",
- chroma.GenericTraceback: "#99CC66",
- chroma.GenericUnderline: "underline",
- chroma.Error: "bg:#FFAAAA #AA0000",
- chroma.Background: " bg:#f0f3f3",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/monokai.go b/vendor/github.com/alecthomas/chroma/styles/monokai.go
deleted file mode 100644
index 2586795..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/monokai.go
+++ /dev/null
@@ -1,36 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// Monokai style.
-var Monokai = Register(chroma.MustNewStyle("monokai", chroma.StyleEntries{
- chroma.Text: "#f8f8f2",
- chroma.Error: "#960050 bg:#1e0010",
- chroma.Comment: "#75715e",
- chroma.Keyword: "#66d9ef",
- chroma.KeywordNamespace: "#f92672",
- chroma.Operator: "#f92672",
- chroma.Punctuation: "#f8f8f2",
- chroma.Name: "#f8f8f2",
- chroma.NameAttribute: "#a6e22e",
- chroma.NameClass: "#a6e22e",
- chroma.NameConstant: "#66d9ef",
- chroma.NameDecorator: "#a6e22e",
- chroma.NameException: "#a6e22e",
- chroma.NameFunction: "#a6e22e",
- chroma.NameOther: "#a6e22e",
- chroma.NameTag: "#f92672",
- chroma.LiteralNumber: "#ae81ff",
- chroma.Literal: "#ae81ff",
- chroma.LiteralDate: "#e6db74",
- chroma.LiteralString: "#e6db74",
- chroma.LiteralStringEscape: "#ae81ff",
- chroma.GenericDeleted: "#f92672",
- chroma.GenericEmph: "italic",
- chroma.GenericInserted: "#a6e22e",
- chroma.GenericStrong: "bold",
- chroma.GenericSubheading: "#75715e",
- chroma.Background: "bg:#272822",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/monokailight.go b/vendor/github.com/alecthomas/chroma/styles/monokailight.go
deleted file mode 100644
index 61818a6..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/monokailight.go
+++ /dev/null
@@ -1,33 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// MonokaiLight style.
-var MonokaiLight = Register(chroma.MustNewStyle("monokailight", chroma.StyleEntries{
- chroma.Text: "#272822",
- chroma.Error: "#960050 bg:#1e0010",
- chroma.Comment: "#75715e",
- chroma.Keyword: "#00a8c8",
- chroma.KeywordNamespace: "#f92672",
- chroma.Operator: "#f92672",
- chroma.Punctuation: "#111111",
- chroma.Name: "#111111",
- chroma.NameAttribute: "#75af00",
- chroma.NameClass: "#75af00",
- chroma.NameConstant: "#00a8c8",
- chroma.NameDecorator: "#75af00",
- chroma.NameException: "#75af00",
- chroma.NameFunction: "#75af00",
- chroma.NameOther: "#75af00",
- chroma.NameTag: "#f92672",
- chroma.LiteralNumber: "#ae81ff",
- chroma.Literal: "#ae81ff",
- chroma.LiteralDate: "#d88200",
- chroma.LiteralString: "#d88200",
- chroma.LiteralStringEscape: "#8045FF",
- chroma.GenericEmph: "italic",
- chroma.GenericStrong: "bold",
- chroma.Background: " bg:#fafafa",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/murphy.go b/vendor/github.com/alecthomas/chroma/styles/murphy.go
deleted file mode 100644
index 90e83c7..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/murphy.go
+++ /dev/null
@@ -1,59 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// Murphy style.
-var Murphy = Register(chroma.MustNewStyle("murphy", chroma.StyleEntries{
- chroma.TextWhitespace: "#bbbbbb",
- chroma.Comment: "#666 italic",
- chroma.CommentPreproc: "#579 noitalic",
- chroma.CommentSpecial: "#c00 bold",
- chroma.Keyword: "bold #289",
- chroma.KeywordPseudo: "#08f",
- chroma.KeywordType: "#66f",
- chroma.Operator: "#333",
- chroma.OperatorWord: "bold #000",
- chroma.NameBuiltin: "#072",
- chroma.NameFunction: "bold #5ed",
- chroma.NameClass: "bold #e9e",
- chroma.NameNamespace: "bold #0e84b5",
- chroma.NameException: "bold #F00",
- chroma.NameVariable: "#036",
- chroma.NameVariableInstance: "#aaf",
- chroma.NameVariableClass: "#ccf",
- chroma.NameVariableGlobal: "#f84",
- chroma.NameConstant: "bold #5ed",
- chroma.NameLabel: "bold #970",
- chroma.NameEntity: "#800",
- chroma.NameAttribute: "#007",
- chroma.NameTag: "#070",
- chroma.NameDecorator: "bold #555",
- chroma.LiteralString: "bg:#e0e0ff",
- chroma.LiteralStringChar: "#88F bg:",
- chroma.LiteralStringDoc: "#D42 bg:",
- chroma.LiteralStringInterpol: "bg:#eee",
- chroma.LiteralStringEscape: "bold #666",
- chroma.LiteralStringRegex: "bg:#e0e0ff #000",
- chroma.LiteralStringSymbol: "#fc8 bg:",
- chroma.LiteralStringOther: "#f88",
- chroma.LiteralNumber: "bold #60E",
- chroma.LiteralNumberInteger: "bold #66f",
- chroma.LiteralNumberFloat: "bold #60E",
- chroma.LiteralNumberHex: "bold #058",
- chroma.LiteralNumberOct: "bold #40E",
- chroma.GenericHeading: "bold #000080",
- chroma.GenericSubheading: "bold #800080",
- chroma.GenericDeleted: "#A00000",
- chroma.GenericInserted: "#00A000",
- chroma.GenericError: "#FF0000",
- chroma.GenericEmph: "italic",
- chroma.GenericStrong: "bold",
- chroma.GenericPrompt: "bold #c65d09",
- chroma.GenericOutput: "#888",
- chroma.GenericTraceback: "#04D",
- chroma.GenericUnderline: "underline",
- chroma.Error: "#F00 bg:#FAA",
- chroma.Background: " bg:#ffffff",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/native.go b/vendor/github.com/alecthomas/chroma/styles/native.go
deleted file mode 100644
index 9fae09a..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/native.go
+++ /dev/null
@@ -1,42 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// Native style.
-var Native = Register(chroma.MustNewStyle("native", chroma.StyleEntries{
- chroma.Background: "#d0d0d0 bg:#202020",
- chroma.TextWhitespace: "#666666",
- chroma.Comment: "italic #999999",
- chroma.CommentPreproc: "noitalic bold #cd2828",
- chroma.CommentSpecial: "noitalic bold #e50808 bg:#520000",
- chroma.Keyword: "bold #6ab825",
- chroma.KeywordPseudo: "nobold",
- chroma.OperatorWord: "bold #6ab825",
- chroma.LiteralString: "#ed9d13",
- chroma.LiteralStringOther: "#ffa500",
- chroma.LiteralNumber: "#3677a9",
- chroma.NameBuiltin: "#24909d",
- chroma.NameVariable: "#40ffff",
- chroma.NameConstant: "#40ffff",
- chroma.NameClass: "underline #447fcf",
- chroma.NameFunction: "#447fcf",
- chroma.NameNamespace: "underline #447fcf",
- chroma.NameException: "#bbbbbb",
- chroma.NameTag: "bold #6ab825",
- chroma.NameAttribute: "#bbbbbb",
- chroma.NameDecorator: "#ffa500",
- chroma.GenericHeading: "bold #ffffff",
- chroma.GenericSubheading: "underline #ffffff",
- chroma.GenericDeleted: "#d22323",
- chroma.GenericInserted: "#589819",
- chroma.GenericError: "#d22323",
- chroma.GenericEmph: "italic",
- chroma.GenericStrong: "bold",
- chroma.GenericPrompt: "#aaaaaa",
- chroma.GenericOutput: "#cccccc",
- chroma.GenericTraceback: "#d22323",
- chroma.GenericUnderline: "underline",
- chroma.Error: "bg:#e3d2d2 #a61717",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/nord.go b/vendor/github.com/alecthomas/chroma/styles/nord.go
deleted file mode 100644
index 0fcbc5d..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/nord.go
+++ /dev/null
@@ -1,75 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-var (
- // colors and palettes based on https://www.nordtheme.com/docs/colors-and-palettes
- nord0 = "#2e3440"
- nord1 = "#3b4252" // nolint
- nord2 = "#434c5e" // nolint
- nord3 = "#4c566a"
- nord3b = "#616e87"
-
- nord4 = "#d8dee9"
- nord5 = "#e5e9f0" // nolint
- nord6 = "#eceff4"
-
- nord7 = "#8fbcbb"
- nord8 = "#88c0d0"
- nord9 = "#81a1c1"
- nord10 = "#5e81ac"
-
- nord11 = "#bf616a"
- nord12 = "#d08770"
- nord13 = "#ebcb8b"
- nord14 = "#a3be8c"
- nord15 = "#b48ead"
-)
-
-// Nord, an arctic, north-bluish color palette
-var Nord = Register(chroma.MustNewStyle("nord", chroma.StyleEntries{
- chroma.TextWhitespace: nord4,
- chroma.Comment: "italic " + nord3b,
- chroma.CommentPreproc: nord10,
- chroma.Keyword: "bold " + nord9,
- chroma.KeywordPseudo: "nobold " + nord9,
- chroma.KeywordType: "nobold " + nord9,
- chroma.Operator: nord9,
- chroma.OperatorWord: "bold " + nord9,
- chroma.Name: nord4,
- chroma.NameBuiltin: nord9,
- chroma.NameFunction: nord8,
- chroma.NameClass: nord7,
- chroma.NameNamespace: nord7,
- chroma.NameException: nord11,
- chroma.NameVariable: nord4,
- chroma.NameConstant: nord7,
- chroma.NameLabel: nord7,
- chroma.NameEntity: nord12,
- chroma.NameAttribute: nord7,
- chroma.NameTag: nord9,
- chroma.NameDecorator: nord12,
- chroma.Punctuation: nord6,
- chroma.LiteralString: nord14,
- chroma.LiteralStringDoc: nord3b,
- chroma.LiteralStringInterpol: nord14,
- chroma.LiteralStringEscape: nord13,
- chroma.LiteralStringRegex: nord13,
- chroma.LiteralStringSymbol: nord14,
- chroma.LiteralStringOther: nord14,
- chroma.LiteralNumber: nord15,
- chroma.GenericHeading: "bold " + nord8,
- chroma.GenericSubheading: "bold " + nord8,
- chroma.GenericDeleted: nord11,
- chroma.GenericInserted: nord14,
- chroma.GenericError: nord11,
- chroma.GenericEmph: "italic",
- chroma.GenericStrong: "bold",
- chroma.GenericPrompt: "bold " + nord3,
- chroma.GenericOutput: nord4,
- chroma.GenericTraceback: nord11,
- chroma.Error: nord11,
- chroma.Background: nord4 + " bg:" + nord0,
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/paraiso-dark.go b/vendor/github.com/alecthomas/chroma/styles/paraiso-dark.go
deleted file mode 100644
index c8cf473..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/paraiso-dark.go
+++ /dev/null
@@ -1,44 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// ParaisoDark style.
-var ParaisoDark = Register(chroma.MustNewStyle("paraiso-dark", chroma.StyleEntries{
- chroma.Text: "#e7e9db",
- chroma.Error: "#ef6155",
- chroma.Comment: "#776e71",
- chroma.Keyword: "#815ba4",
- chroma.KeywordNamespace: "#5bc4bf",
- chroma.KeywordType: "#fec418",
- chroma.Operator: "#5bc4bf",
- chroma.Punctuation: "#e7e9db",
- chroma.Name: "#e7e9db",
- chroma.NameAttribute: "#06b6ef",
- chroma.NameClass: "#fec418",
- chroma.NameConstant: "#ef6155",
- chroma.NameDecorator: "#5bc4bf",
- chroma.NameException: "#ef6155",
- chroma.NameFunction: "#06b6ef",
- chroma.NameNamespace: "#fec418",
- chroma.NameOther: "#06b6ef",
- chroma.NameTag: "#5bc4bf",
- chroma.NameVariable: "#ef6155",
- chroma.LiteralNumber: "#f99b15",
- chroma.Literal: "#f99b15",
- chroma.LiteralDate: "#48b685",
- chroma.LiteralString: "#48b685",
- chroma.LiteralStringChar: "#e7e9db",
- chroma.LiteralStringDoc: "#776e71",
- chroma.LiteralStringEscape: "#f99b15",
- chroma.LiteralStringInterpol: "#f99b15",
- chroma.GenericDeleted: "#ef6155",
- chroma.GenericEmph: "italic",
- chroma.GenericHeading: "bold #e7e9db",
- chroma.GenericInserted: "#48b685",
- chroma.GenericPrompt: "bold #776e71",
- chroma.GenericStrong: "bold",
- chroma.GenericSubheading: "bold #5bc4bf",
- chroma.Background: "bg:#2f1e2e",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/paraiso-light.go b/vendor/github.com/alecthomas/chroma/styles/paraiso-light.go
deleted file mode 100644
index b514dfa..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/paraiso-light.go
+++ /dev/null
@@ -1,44 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// ParaisoLight style.
-var ParaisoLight = Register(chroma.MustNewStyle("paraiso-light", chroma.StyleEntries{
- chroma.Text: "#2f1e2e",
- chroma.Error: "#ef6155",
- chroma.Comment: "#8d8687",
- chroma.Keyword: "#815ba4",
- chroma.KeywordNamespace: "#5bc4bf",
- chroma.KeywordType: "#fec418",
- chroma.Operator: "#5bc4bf",
- chroma.Punctuation: "#2f1e2e",
- chroma.Name: "#2f1e2e",
- chroma.NameAttribute: "#06b6ef",
- chroma.NameClass: "#fec418",
- chroma.NameConstant: "#ef6155",
- chroma.NameDecorator: "#5bc4bf",
- chroma.NameException: "#ef6155",
- chroma.NameFunction: "#06b6ef",
- chroma.NameNamespace: "#fec418",
- chroma.NameOther: "#06b6ef",
- chroma.NameTag: "#5bc4bf",
- chroma.NameVariable: "#ef6155",
- chroma.LiteralNumber: "#f99b15",
- chroma.Literal: "#f99b15",
- chroma.LiteralDate: "#48b685",
- chroma.LiteralString: "#48b685",
- chroma.LiteralStringChar: "#2f1e2e",
- chroma.LiteralStringDoc: "#8d8687",
- chroma.LiteralStringEscape: "#f99b15",
- chroma.LiteralStringInterpol: "#f99b15",
- chroma.GenericDeleted: "#ef6155",
- chroma.GenericEmph: "italic",
- chroma.GenericHeading: "bold #2f1e2e",
- chroma.GenericInserted: "#48b685",
- chroma.GenericPrompt: "bold #8d8687",
- chroma.GenericStrong: "bold",
- chroma.GenericSubheading: "bold #5bc4bf",
- chroma.Background: "bg:#e7e9db",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/pastie.go b/vendor/github.com/alecthomas/chroma/styles/pastie.go
deleted file mode 100644
index 9a68544..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/pastie.go
+++ /dev/null
@@ -1,52 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// Pastie style.
-var Pastie = Register(chroma.MustNewStyle("pastie", chroma.StyleEntries{
- chroma.TextWhitespace: "#bbbbbb",
- chroma.Comment: "#888888",
- chroma.CommentPreproc: "bold #cc0000",
- chroma.CommentSpecial: "bg:#fff0f0 bold #cc0000",
- chroma.LiteralString: "bg:#fff0f0 #dd2200",
- chroma.LiteralStringRegex: "bg:#fff0ff #008800",
- chroma.LiteralStringOther: "bg:#f0fff0 #22bb22",
- chroma.LiteralStringSymbol: "#aa6600",
- chroma.LiteralStringInterpol: "#3333bb",
- chroma.LiteralStringEscape: "#0044dd",
- chroma.OperatorWord: "#008800",
- chroma.Keyword: "bold #008800",
- chroma.KeywordPseudo: "nobold",
- chroma.KeywordType: "#888888",
- chroma.NameClass: "bold #bb0066",
- chroma.NameException: "bold #bb0066",
- chroma.NameFunction: "bold #0066bb",
- chroma.NameProperty: "bold #336699",
- chroma.NameNamespace: "bold #bb0066",
- chroma.NameBuiltin: "#003388",
- chroma.NameVariable: "#336699",
- chroma.NameVariableClass: "#336699",
- chroma.NameVariableInstance: "#3333bb",
- chroma.NameVariableGlobal: "#dd7700",
- chroma.NameConstant: "bold #003366",
- chroma.NameTag: "bold #bb0066",
- chroma.NameAttribute: "#336699",
- chroma.NameDecorator: "#555555",
- chroma.NameLabel: "italic #336699",
- chroma.LiteralNumber: "bold #0000DD",
- chroma.GenericHeading: "#333",
- chroma.GenericSubheading: "#666",
- chroma.GenericDeleted: "bg:#ffdddd #000000",
- chroma.GenericInserted: "bg:#ddffdd #000000",
- chroma.GenericError: "#aa0000",
- chroma.GenericEmph: "italic",
- chroma.GenericStrong: "bold",
- chroma.GenericPrompt: "#555555",
- chroma.GenericOutput: "#888888",
- chroma.GenericTraceback: "#aa0000",
- chroma.GenericUnderline: "underline",
- chroma.Error: "bg:#e3d2d2 #a61717",
- chroma.Background: " bg:#ffffff",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/perldoc.go b/vendor/github.com/alecthomas/chroma/styles/perldoc.go
deleted file mode 100644
index e1372fd..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/perldoc.go
+++ /dev/null
@@ -1,44 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// Perldoc style.
-var Perldoc = Register(chroma.MustNewStyle("perldoc", chroma.StyleEntries{
- chroma.TextWhitespace: "#bbbbbb",
- chroma.Comment: "#228B22",
- chroma.CommentPreproc: "#1e889b",
- chroma.CommentSpecial: "#8B008B bold",
- chroma.LiteralString: "#CD5555",
- chroma.LiteralStringHeredoc: "#1c7e71 italic",
- chroma.LiteralStringRegex: "#1c7e71",
- chroma.LiteralStringOther: "#cb6c20",
- chroma.LiteralNumber: "#B452CD",
- chroma.OperatorWord: "#8B008B",
- chroma.Keyword: "#8B008B bold",
- chroma.KeywordType: "#00688B",
- chroma.NameClass: "#008b45 bold",
- chroma.NameException: "#008b45 bold",
- chroma.NameFunction: "#008b45",
- chroma.NameNamespace: "#008b45 underline",
- chroma.NameVariable: "#00688B",
- chroma.NameConstant: "#00688B",
- chroma.NameDecorator: "#707a7c",
- chroma.NameTag: "#8B008B bold",
- chroma.NameAttribute: "#658b00",
- chroma.NameBuiltin: "#658b00",
- chroma.GenericHeading: "bold #000080",
- chroma.GenericSubheading: "bold #800080",
- chroma.GenericDeleted: "#aa0000",
- chroma.GenericInserted: "#00aa00",
- chroma.GenericError: "#aa0000",
- chroma.GenericEmph: "italic",
- chroma.GenericStrong: "bold",
- chroma.GenericPrompt: "#555555",
- chroma.GenericOutput: "#888888",
- chroma.GenericTraceback: "#aa0000",
- chroma.GenericUnderline: "underline",
- chroma.Error: "bg:#e3d2d2 #a61717",
- chroma.Background: " bg:#eeeedd",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/pygments.go b/vendor/github.com/alecthomas/chroma/styles/pygments.go
deleted file mode 100644
index 327033b..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/pygments.go
+++ /dev/null
@@ -1,55 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// Pygments default theme.
-var Pygments = Register(chroma.MustNewStyle("pygments", chroma.StyleEntries{
- chroma.Whitespace: "#bbbbbb",
- chroma.Comment: "italic #408080",
- chroma.CommentPreproc: "noitalic #BC7A00",
-
- chroma.Keyword: "bold #008000",
- chroma.KeywordPseudo: "nobold",
- chroma.KeywordType: "nobold #B00040",
-
- chroma.Operator: "#666666",
- chroma.OperatorWord: "bold #AA22FF",
-
- chroma.NameBuiltin: "#008000",
- chroma.NameFunction: "#0000FF",
- chroma.NameClass: "bold #0000FF",
- chroma.NameNamespace: "bold #0000FF",
- chroma.NameException: "bold #D2413A",
- chroma.NameVariable: "#19177C",
- chroma.NameConstant: "#880000",
- chroma.NameLabel: "#A0A000",
- chroma.NameEntity: "bold #999999",
- chroma.NameAttribute: "#7D9029",
- chroma.NameTag: "bold #008000",
- chroma.NameDecorator: "#AA22FF",
-
- chroma.String: "#BA2121",
- chroma.StringDoc: "italic",
- chroma.StringInterpol: "bold #BB6688",
- chroma.StringEscape: "bold #BB6622",
- chroma.StringRegex: "#BB6688",
- chroma.StringSymbol: "#19177C",
- chroma.StringOther: "#008000",
- chroma.Number: "#666666",
-
- chroma.GenericHeading: "bold #000080",
- chroma.GenericSubheading: "bold #800080",
- chroma.GenericDeleted: "#A00000",
- chroma.GenericInserted: "#00A000",
- chroma.GenericError: "#FF0000",
- chroma.GenericEmph: "italic",
- chroma.GenericStrong: "bold",
- chroma.GenericPrompt: "bold #000080",
- chroma.GenericOutput: "#888",
- chroma.GenericTraceback: "#04D",
- chroma.GenericUnderline: "underline",
-
- chroma.Error: "border:#FF0000",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/rainbow_dash.go b/vendor/github.com/alecthomas/chroma/styles/rainbow_dash.go
deleted file mode 100644
index 37d66ca..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/rainbow_dash.go
+++ /dev/null
@@ -1,47 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// RainbowDash style.
-var RainbowDash = Register(chroma.MustNewStyle("rainbow_dash", chroma.StyleEntries{
- chroma.Comment: "italic #0080ff",
- chroma.CommentPreproc: "noitalic",
- chroma.CommentSpecial: "bold",
- chroma.Error: "bg:#cc0000 #ffffff",
- chroma.GenericDeleted: "border:#c5060b bg:#ffcccc",
- chroma.GenericEmph: "italic",
- chroma.GenericError: "#ff0000",
- chroma.GenericHeading: "bold #2c5dcd",
- chroma.GenericInserted: "border:#00cc00 bg:#ccffcc",
- chroma.GenericOutput: "#aaaaaa",
- chroma.GenericPrompt: "bold #2c5dcd",
- chroma.GenericStrong: "bold",
- chroma.GenericSubheading: "bold #2c5dcd",
- chroma.GenericTraceback: "#c5060b",
- chroma.GenericUnderline: "underline",
- chroma.Keyword: "bold #2c5dcd",
- chroma.KeywordPseudo: "nobold",
- chroma.KeywordType: "#5918bb",
- chroma.NameAttribute: "italic #2c5dcd",
- chroma.NameBuiltin: "bold #5918bb",
- chroma.NameClass: "underline",
- chroma.NameConstant: "#318495",
- chroma.NameDecorator: "bold #ff8000",
- chroma.NameEntity: "bold #5918bb",
- chroma.NameException: "bold #5918bb",
- chroma.NameFunction: "bold #ff8000",
- chroma.NameTag: "bold #2c5dcd",
- chroma.LiteralNumber: "bold #5918bb",
- chroma.Operator: "#2c5dcd",
- chroma.OperatorWord: "bold",
- chroma.LiteralString: "#00cc66",
- chroma.LiteralStringDoc: "italic",
- chroma.LiteralStringEscape: "bold #c5060b",
- chroma.LiteralStringOther: "#318495",
- chroma.LiteralStringSymbol: "bold #c5060b",
- chroma.Text: "#4d4d4d",
- chroma.TextWhitespace: "#cbcbcb",
- chroma.Background: " bg:#ffffff",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/rrt.go b/vendor/github.com/alecthomas/chroma/styles/rrt.go
deleted file mode 100644
index 2ccf2ca..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/rrt.go
+++ /dev/null
@@ -1,20 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// Rrt style.
-var Rrt = Register(chroma.MustNewStyle("rrt", chroma.StyleEntries{
- chroma.CommentPreproc: "#e5e5e5",
- chroma.Comment: "#00ff00",
- chroma.KeywordType: "#ee82ee",
- chroma.Keyword: "#ff0000",
- chroma.LiteralNumber: "#ff6600",
- chroma.LiteralStringSymbol: "#ff6600",
- chroma.LiteralString: "#87ceeb",
- chroma.NameFunction: "#ffff00",
- chroma.NameConstant: "#7fffd4",
- chroma.NameVariable: "#eedd82",
- chroma.Background: "#f8f8f2 bg:#000000",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/solarized-dark.go b/vendor/github.com/alecthomas/chroma/styles/solarized-dark.go
deleted file mode 100644
index 2724df2..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/solarized-dark.go
+++ /dev/null
@@ -1,46 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// SolarizedDark style.
-var SolarizedDark = Register(chroma.MustNewStyle("solarized-dark", chroma.StyleEntries{
- chroma.Keyword: "#719e07",
- chroma.KeywordConstant: "#CB4B16",
- chroma.KeywordDeclaration: "#268BD2",
- chroma.KeywordReserved: "#268BD2",
- chroma.KeywordType: "#DC322F",
- chroma.NameAttribute: "#93A1A1",
- chroma.NameBuiltin: "#B58900",
- chroma.NameBuiltinPseudo: "#268BD2",
- chroma.NameClass: "#268BD2",
- chroma.NameConstant: "#CB4B16",
- chroma.NameDecorator: "#268BD2",
- chroma.NameEntity: "#CB4B16",
- chroma.NameException: "#CB4B16",
- chroma.NameFunction: "#268BD2",
- chroma.NameTag: "#268BD2",
- chroma.NameVariable: "#268BD2",
- chroma.LiteralString: "#2AA198",
- chroma.LiteralStringBacktick: "#586E75",
- chroma.LiteralStringChar: "#2AA198",
- chroma.LiteralStringDoc: "#93A1A1",
- chroma.LiteralStringEscape: "#CB4B16",
- chroma.LiteralStringHeredoc: "#93A1A1",
- chroma.LiteralStringRegex: "#DC322F",
- chroma.LiteralNumber: "#2AA198",
- chroma.Operator: "#719e07",
- chroma.Comment: "#586E75",
- chroma.CommentPreproc: "#719e07",
- chroma.CommentSpecial: "#719e07",
- chroma.GenericDeleted: "#DC322F",
- chroma.GenericEmph: "italic",
- chroma.GenericError: "#DC322F bold",
- chroma.GenericHeading: "#CB4B16",
- chroma.GenericInserted: "#719e07",
- chroma.GenericStrong: "bold",
- chroma.GenericSubheading: "#268BD2",
- chroma.Background: "#93A1A1 bg:#002B36",
- chroma.Other: "#CB4B16",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/solarized-dark256.go b/vendor/github.com/alecthomas/chroma/styles/solarized-dark256.go
deleted file mode 100644
index a24ddc1..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/solarized-dark256.go
+++ /dev/null
@@ -1,48 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// SolarizedDark256 style.
-var SolarizedDark256 = Register(chroma.MustNewStyle("solarized-dark256", chroma.StyleEntries{
- chroma.Keyword: "#5f8700",
- chroma.KeywordConstant: "#d75f00",
- chroma.KeywordDeclaration: "#0087ff",
- chroma.KeywordNamespace: "#d75f00",
- chroma.KeywordReserved: "#0087ff",
- chroma.KeywordType: "#af0000",
- chroma.NameAttribute: "#8a8a8a",
- chroma.NameBuiltin: "#0087ff",
- chroma.NameBuiltinPseudo: "#0087ff",
- chroma.NameClass: "#0087ff",
- chroma.NameConstant: "#d75f00",
- chroma.NameDecorator: "#0087ff",
- chroma.NameEntity: "#d75f00",
- chroma.NameException: "#af8700",
- chroma.NameFunction: "#0087ff",
- chroma.NameTag: "#0087ff",
- chroma.NameVariable: "#0087ff",
- chroma.LiteralString: "#00afaf",
- chroma.LiteralStringBacktick: "#4e4e4e",
- chroma.LiteralStringChar: "#00afaf",
- chroma.LiteralStringDoc: "#00afaf",
- chroma.LiteralStringEscape: "#af0000",
- chroma.LiteralStringHeredoc: "#00afaf",
- chroma.LiteralStringRegex: "#af0000",
- chroma.LiteralNumber: "#00afaf",
- chroma.Operator: "#8a8a8a",
- chroma.OperatorWord: "#5f8700",
- chroma.Comment: "#4e4e4e",
- chroma.CommentPreproc: "#5f8700",
- chroma.CommentSpecial: "#5f8700",
- chroma.GenericDeleted: "#af0000",
- chroma.GenericEmph: "italic",
- chroma.GenericError: "#af0000 bold",
- chroma.GenericHeading: "#d75f00",
- chroma.GenericInserted: "#5f8700",
- chroma.GenericStrong: "bold",
- chroma.GenericSubheading: "#0087ff",
- chroma.Background: "#8a8a8a bg:#1c1c1c",
- chroma.Other: "#d75f00",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/solarized-light.go b/vendor/github.com/alecthomas/chroma/styles/solarized-light.go
deleted file mode 100644
index b6d5234..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/solarized-light.go
+++ /dev/null
@@ -1,24 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// SolarizedLight style.
-var SolarizedLight = Register(chroma.MustNewStyle("solarized-light", chroma.StyleEntries{
- chroma.Text: "bg: #eee8d5 #586e75",
- chroma.Keyword: "#859900",
- chroma.KeywordConstant: "bold",
- chroma.KeywordNamespace: "#dc322f bold",
- chroma.KeywordType: "bold",
- chroma.Name: "#268bd2",
- chroma.NameBuiltin: "#cb4b16",
- chroma.NameClass: "#cb4b16",
- chroma.NameTag: "bold",
- chroma.Literal: "#2aa198",
- chroma.LiteralNumber: "bold",
- chroma.OperatorWord: "#859900",
- chroma.Comment: "#93a1a1 italic",
- chroma.Generic: "#d33682",
- chroma.Background: " bg:#eee8d5",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/swapoff.go b/vendor/github.com/alecthomas/chroma/styles/swapoff.go
deleted file mode 100644
index e4daae6..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/swapoff.go
+++ /dev/null
@@ -1,25 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// SwapOff theme.
-var SwapOff = Register(chroma.MustNewStyle("swapoff", chroma.StyleEntries{
- chroma.Background: "#lightgray bg:#black",
- chroma.Number: "bold #ansiyellow",
- chroma.Comment: "#ansiteal",
- chroma.CommentPreproc: "bold #ansigreen",
- chroma.String: "bold #ansiturquoise",
- chroma.Keyword: "bold #ansiwhite",
- chroma.NameKeyword: "bold #ansiwhite",
- chroma.NameBuiltin: "bold #ansiwhite",
- chroma.GenericHeading: "bold",
- chroma.GenericSubheading: "bold",
- chroma.GenericStrong: "bold",
- chroma.GenericUnderline: "underline",
- chroma.NameTag: "bold",
- chroma.NameAttribute: "#ansiteal",
- chroma.Error: "#ansired",
- chroma.LiteralDate: "bold #ansiyellow",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/tango.go b/vendor/github.com/alecthomas/chroma/styles/tango.go
deleted file mode 100644
index ae1afe0..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/tango.go
+++ /dev/null
@@ -1,79 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// Tango style.
-var Tango = Register(chroma.MustNewStyle("tango", chroma.StyleEntries{
- chroma.TextWhitespace: "underline #f8f8f8",
- chroma.Error: "#a40000 border:#ef2929",
- chroma.Other: "#000000",
- chroma.Comment: "italic #8f5902",
- chroma.CommentMultiline: "italic #8f5902",
- chroma.CommentPreproc: "italic #8f5902",
- chroma.CommentSingle: "italic #8f5902",
- chroma.CommentSpecial: "italic #8f5902",
- chroma.Keyword: "bold #204a87",
- chroma.KeywordConstant: "bold #204a87",
- chroma.KeywordDeclaration: "bold #204a87",
- chroma.KeywordNamespace: "bold #204a87",
- chroma.KeywordPseudo: "bold #204a87",
- chroma.KeywordReserved: "bold #204a87",
- chroma.KeywordType: "bold #204a87",
- chroma.Operator: "bold #ce5c00",
- chroma.OperatorWord: "bold #204a87",
- chroma.Punctuation: "bold #000000",
- chroma.Name: "#000000",
- chroma.NameAttribute: "#c4a000",
- chroma.NameBuiltin: "#204a87",
- chroma.NameBuiltinPseudo: "#3465a4",
- chroma.NameClass: "#000000",
- chroma.NameConstant: "#000000",
- chroma.NameDecorator: "bold #5c35cc",
- chroma.NameEntity: "#ce5c00",
- chroma.NameException: "bold #cc0000",
- chroma.NameFunction: "#000000",
- chroma.NameProperty: "#000000",
- chroma.NameLabel: "#f57900",
- chroma.NameNamespace: "#000000",
- chroma.NameOther: "#000000",
- chroma.NameTag: "bold #204a87",
- chroma.NameVariable: "#000000",
- chroma.NameVariableClass: "#000000",
- chroma.NameVariableGlobal: "#000000",
- chroma.NameVariableInstance: "#000000",
- chroma.LiteralNumber: "bold #0000cf",
- chroma.LiteralNumberFloat: "bold #0000cf",
- chroma.LiteralNumberHex: "bold #0000cf",
- chroma.LiteralNumberInteger: "bold #0000cf",
- chroma.LiteralNumberIntegerLong: "bold #0000cf",
- chroma.LiteralNumberOct: "bold #0000cf",
- chroma.Literal: "#000000",
- chroma.LiteralDate: "#000000",
- chroma.LiteralString: "#4e9a06",
- chroma.LiteralStringBacktick: "#4e9a06",
- chroma.LiteralStringChar: "#4e9a06",
- chroma.LiteralStringDoc: "italic #8f5902",
- chroma.LiteralStringDouble: "#4e9a06",
- chroma.LiteralStringEscape: "#4e9a06",
- chroma.LiteralStringHeredoc: "#4e9a06",
- chroma.LiteralStringInterpol: "#4e9a06",
- chroma.LiteralStringOther: "#4e9a06",
- chroma.LiteralStringRegex: "#4e9a06",
- chroma.LiteralStringSingle: "#4e9a06",
- chroma.LiteralStringSymbol: "#4e9a06",
- chroma.Generic: "#000000",
- chroma.GenericDeleted: "#a40000",
- chroma.GenericEmph: "italic #000000",
- chroma.GenericError: "#ef2929",
- chroma.GenericHeading: "bold #000080",
- chroma.GenericInserted: "#00A000",
- chroma.GenericOutput: "italic #000000",
- chroma.GenericPrompt: "#8f5902",
- chroma.GenericStrong: "bold #000000",
- chroma.GenericSubheading: "bold #800080",
- chroma.GenericTraceback: "bold #a40000",
- chroma.GenericUnderline: "underline",
- chroma.Background: " bg:#f8f8f8",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/trac.go b/vendor/github.com/alecthomas/chroma/styles/trac.go
deleted file mode 100644
index 3b09c44..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/trac.go
+++ /dev/null
@@ -1,42 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// Trac style.
-var Trac = Register(chroma.MustNewStyle("trac", chroma.StyleEntries{
- chroma.TextWhitespace: "#bbbbbb",
- chroma.Comment: "italic #999988",
- chroma.CommentPreproc: "bold noitalic #999999",
- chroma.CommentSpecial: "bold #999999",
- chroma.Operator: "bold",
- chroma.LiteralString: "#bb8844",
- chroma.LiteralStringRegex: "#808000",
- chroma.LiteralNumber: "#009999",
- chroma.Keyword: "bold",
- chroma.KeywordType: "#445588",
- chroma.NameBuiltin: "#999999",
- chroma.NameFunction: "bold #990000",
- chroma.NameClass: "bold #445588",
- chroma.NameException: "bold #990000",
- chroma.NameNamespace: "#555555",
- chroma.NameVariable: "#008080",
- chroma.NameConstant: "#008080",
- chroma.NameTag: "#000080",
- chroma.NameAttribute: "#008080",
- chroma.NameEntity: "#800080",
- chroma.GenericHeading: "#999999",
- chroma.GenericSubheading: "#aaaaaa",
- chroma.GenericDeleted: "bg:#ffdddd #000000",
- chroma.GenericInserted: "bg:#ddffdd #000000",
- chroma.GenericError: "#aa0000",
- chroma.GenericEmph: "italic",
- chroma.GenericStrong: "bold",
- chroma.GenericPrompt: "#555555",
- chroma.GenericOutput: "#888888",
- chroma.GenericTraceback: "#aa0000",
- chroma.GenericUnderline: "underline",
- chroma.Error: "bg:#e3d2d2 #a61717",
- chroma.Background: " bg:#ffffff",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/vim.go b/vendor/github.com/alecthomas/chroma/styles/vim.go
deleted file mode 100644
index 6296042..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/vim.go
+++ /dev/null
@@ -1,36 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// Vim style.
-var Vim = Register(chroma.MustNewStyle("vim", chroma.StyleEntries{
- chroma.Background: "#cccccc bg:#000000",
- chroma.Comment: "#000080",
- chroma.CommentSpecial: "bold #cd0000",
- chroma.Keyword: "#cdcd00",
- chroma.KeywordDeclaration: "#00cd00",
- chroma.KeywordNamespace: "#cd00cd",
- chroma.KeywordType: "#00cd00",
- chroma.Operator: "#3399cc",
- chroma.OperatorWord: "#cdcd00",
- chroma.NameClass: "#00cdcd",
- chroma.NameBuiltin: "#cd00cd",
- chroma.NameException: "bold #666699",
- chroma.NameVariable: "#00cdcd",
- chroma.LiteralString: "#cd0000",
- chroma.LiteralNumber: "#cd00cd",
- chroma.GenericHeading: "bold #000080",
- chroma.GenericSubheading: "bold #800080",
- chroma.GenericDeleted: "#cd0000",
- chroma.GenericInserted: "#00cd00",
- chroma.GenericError: "#FF0000",
- chroma.GenericEmph: "italic",
- chroma.GenericStrong: "bold",
- chroma.GenericPrompt: "bold #000080",
- chroma.GenericOutput: "#888",
- chroma.GenericTraceback: "#04D",
- chroma.GenericUnderline: "underline",
- chroma.Error: "border:#FF0000",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/vs.go b/vendor/github.com/alecthomas/chroma/styles/vs.go
deleted file mode 100644
index acbcb91..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/vs.go
+++ /dev/null
@@ -1,23 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// VisualStudio style.
-var VisualStudio = Register(chroma.MustNewStyle("vs", chroma.StyleEntries{
- chroma.Comment: "#008000",
- chroma.CommentPreproc: "#0000ff",
- chroma.Keyword: "#0000ff",
- chroma.OperatorWord: "#0000ff",
- chroma.KeywordType: "#2b91af",
- chroma.NameClass: "#2b91af",
- chroma.LiteralString: "#a31515",
- chroma.GenericHeading: "bold",
- chroma.GenericSubheading: "bold",
- chroma.GenericEmph: "italic",
- chroma.GenericStrong: "bold",
- chroma.GenericPrompt: "bold",
- chroma.Error: "border:#FF0000",
- chroma.Background: " bg:#ffffff",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/vulcan.go b/vendor/github.com/alecthomas/chroma/styles/vulcan.go
deleted file mode 100644
index 82ad1a1..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/vulcan.go
+++ /dev/null
@@ -1,95 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-var (
- // inspired by Doom Emacs's One Doom Theme
- black = "#282C34"
- grey = "#3E4460"
- grey2 = "#43454f"
- white = "#C9C9C9"
- red = "#CF5967"
- yellow = "#ECBE7B"
- green = "#82CC6A"
- cyan = "#56B6C2"
- blue = "#7FBAF5"
- blue2 = "#57C7FF"
- purple = "#BC74C4"
-)
-
-var Vulcan = Register(chroma.MustNewStyle("vulcan", chroma.StyleEntries{
- chroma.Comment: grey,
- chroma.CommentHashbang: grey + " italic",
- chroma.CommentMultiline: grey,
- chroma.CommentPreproc: blue,
- chroma.CommentSingle: grey,
- chroma.CommentSpecial: purple + " italic",
- chroma.Generic: white,
- chroma.GenericDeleted: red,
- chroma.GenericEmph: white + " underline",
- chroma.GenericError: red + " bold",
- chroma.GenericHeading: yellow + " bold",
- chroma.GenericInserted: yellow,
- chroma.GenericOutput: grey2,
- chroma.GenericPrompt: white,
- chroma.GenericStrong: red + " bold",
- chroma.GenericSubheading: red + " italic",
- chroma.GenericTraceback: white,
- chroma.GenericUnderline: "underline",
- chroma.Error: red,
- chroma.Keyword: blue,
- chroma.KeywordConstant: red + " bg:" + grey2,
- chroma.KeywordDeclaration: blue,
- chroma.KeywordNamespace: purple,
- chroma.KeywordPseudo: purple,
- chroma.KeywordReserved: blue,
- chroma.KeywordType: blue2 + " bold",
- chroma.Literal: white,
- chroma.LiteralDate: blue2,
- chroma.Name: white,
- chroma.NameAttribute: purple,
- chroma.NameBuiltin: blue,
- chroma.NameBuiltinPseudo: blue,
- chroma.NameClass: yellow,
- chroma.NameConstant: yellow,
- chroma.NameDecorator: yellow,
- chroma.NameEntity: white,
- chroma.NameException: red,
- chroma.NameFunction: blue2,
- chroma.NameLabel: red,
- chroma.NameNamespace: white,
- chroma.NameOther: white,
- chroma.NameTag: purple,
- chroma.NameVariable: purple + " italic",
- chroma.NameVariableClass: blue2 + " bold",
- chroma.NameVariableGlobal: yellow,
- chroma.NameVariableInstance: blue2,
- chroma.LiteralNumber: cyan,
- chroma.LiteralNumberBin: blue2,
- chroma.LiteralNumberFloat: cyan,
- chroma.LiteralNumberHex: blue2,
- chroma.LiteralNumberInteger: cyan,
- chroma.LiteralNumberIntegerLong: cyan,
- chroma.LiteralNumberOct: blue2,
- chroma.Operator: purple,
- chroma.OperatorWord: purple,
- chroma.Other: white,
- chroma.Punctuation: cyan,
- chroma.LiteralString: green,
- chroma.LiteralStringBacktick: blue2,
- chroma.LiteralStringChar: blue2,
- chroma.LiteralStringDoc: green,
- chroma.LiteralStringDouble: green,
- chroma.LiteralStringEscape: cyan,
- chroma.LiteralStringHeredoc: cyan,
- chroma.LiteralStringInterpol: green,
- chroma.LiteralStringOther: green,
- chroma.LiteralStringRegex: blue2,
- chroma.LiteralStringSingle: green,
- chroma.LiteralStringSymbol: green,
- chroma.Text: white,
- chroma.TextWhitespace: white,
- chroma.Background: " bg: " + black,
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/xcode-dark.go b/vendor/github.com/alecthomas/chroma/styles/xcode-dark.go
deleted file mode 100644
index a16d946..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/xcode-dark.go
+++ /dev/null
@@ -1,62 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-var (
- // Inspired by Apple's Xcode "Default (Dark)" Theme
- background = "#1F1F24"
- plainText = "#FFFFFF"
- comments = "#6C7986"
- strings = "#FC6A5D"
- numbers = "#D0BF69"
- keywords = "#FC5FA3"
- preprocessorStatements = "#FD8F3F"
- typeDeclarations = "#5DD8FF"
- otherDeclarations = "#41A1C0"
- otherFunctionAndMethodNames = "#A167E6"
- otherTypeNames = "#D0A8FF"
-)
-
-// Xcode dark style
-var XcodeDark = Register(chroma.MustNewStyle("xcode-dark", chroma.StyleEntries{
- chroma.Background: plainText + " bg: " + background,
-
- chroma.Comment: comments,
- chroma.CommentMultiline: comments,
- chroma.CommentPreproc: preprocessorStatements,
- chroma.CommentSingle: comments,
- chroma.CommentSpecial: comments + " italic",
-
- chroma.Error: "#960050",
-
- chroma.Keyword: keywords,
- chroma.KeywordConstant: keywords,
- chroma.KeywordDeclaration: keywords,
- chroma.KeywordReserved: keywords,
-
- chroma.LiteralNumber: numbers,
- chroma.LiteralNumberBin: numbers,
- chroma.LiteralNumberFloat: numbers,
- chroma.LiteralNumberHex: numbers,
- chroma.LiteralNumberInteger: numbers,
- chroma.LiteralNumberOct: numbers,
-
- chroma.LiteralString: strings,
- chroma.LiteralStringEscape: strings,
- chroma.LiteralStringInterpol: plainText,
-
- chroma.Name: plainText,
- chroma.NameBuiltin: otherTypeNames,
- chroma.NameBuiltinPseudo: otherFunctionAndMethodNames,
- chroma.NameClass: typeDeclarations,
- chroma.NameFunction: otherDeclarations,
- chroma.NameVariable: otherDeclarations,
-
- chroma.Operator: plainText,
-
- chroma.Punctuation: plainText,
-
- chroma.Text: plainText,
-}))
diff --git a/vendor/github.com/alecthomas/chroma/styles/xcode.go b/vendor/github.com/alecthomas/chroma/styles/xcode.go
deleted file mode 100644
index 115cf71..0000000
--- a/vendor/github.com/alecthomas/chroma/styles/xcode.go
+++ /dev/null
@@ -1,29 +0,0 @@
-package styles
-
-import (
- "github.com/alecthomas/chroma"
-)
-
-// Xcode style.
-var Xcode = Register(chroma.MustNewStyle("xcode", chroma.StyleEntries{
- chroma.Comment: "#177500",
- chroma.CommentPreproc: "#633820",
- chroma.LiteralString: "#C41A16",
- chroma.LiteralStringChar: "#2300CE",
- chroma.Operator: "#000000",
- chroma.Keyword: "#A90D91",
- chroma.Name: "#000000",
- chroma.NameAttribute: "#836C28",
- chroma.NameClass: "#3F6E75",
- chroma.NameFunction: "#000000",
- chroma.NameBuiltin: "#A90D91",
- chroma.NameBuiltinPseudo: "#5B269A",
- chroma.NameVariable: "#000000",
- chroma.NameTag: "#000000",
- chroma.NameDecorator: "#000000",
- chroma.NameLabel: "#000000",
- chroma.Literal: "#1C01CE",
- chroma.LiteralNumber: "#1C01CE",
- chroma.Error: "#000000",
- chroma.Background: " bg:#ffffff",
-}))
diff --git a/vendor/github.com/alecthomas/chroma/table.py b/vendor/github.com/alecthomas/chroma/table.py
deleted file mode 100644
index 7794539..0000000
--- a/vendor/github.com/alecthomas/chroma/table.py
+++ /dev/null
@@ -1,32 +0,0 @@
-#!/usr/bin/env python3
-import re
-from collections import defaultdict
-from subprocess import check_output
-
-README_FILE = "README.md"
-
-
-lines = check_output(["go", "run", "./cmd/chroma/main.go", "--list"]).decode("utf-8").splitlines()
-lines = [line.strip() for line in lines if line.startswith(" ") and not line.startswith(" ")]
-lines = sorted(lines, key=lambda l: l.lower())
-
-table = defaultdict(list)
-
-for line in lines:
- table[line[0].upper()].append(line)
-
-rows = []
-for key, value in table.items():
- rows.append("{} | {}".format(key, ", ".join(value)))
-tbody = "\n".join(rows)
-
-with open(README_FILE, "r") as f:
- content = f.read()
-
-with open(README_FILE, "w") as f:
- marker = re.compile(r"(?P:----: \\| --------\n).*?(?P\n\n)", re.DOTALL)
- replacement = r"\g%s\g" % tbody
- updated_content = marker.sub(replacement, content)
- f.write(updated_content)
-
-print(tbody)
diff --git a/vendor/github.com/alecthomas/chroma/tokentype_string.go b/vendor/github.com/alecthomas/chroma/tokentype_string.go
deleted file mode 100644
index 6fe3867..0000000
--- a/vendor/github.com/alecthomas/chroma/tokentype_string.go
+++ /dev/null
@@ -1,213 +0,0 @@
-// Code generated by "stringer -type TokenType"; DO NOT EDIT.
-
-package chroma
-
-import "strconv"
-
-func _() {
- // An "invalid array index" compiler error signifies that the constant values have changed.
- // Re-run the stringer command to generate them again.
- var x [1]struct{}
- _ = x[Background - -1]
- _ = x[LineNumbers - -2]
- _ = x[LineNumbersTable - -3]
- _ = x[LineHighlight - -4]
- _ = x[LineTable - -5]
- _ = x[LineTableTD - -6]
- _ = x[Error - -7]
- _ = x[Other - -8]
- _ = x[None - -9]
- _ = x[EOFType-0]
- _ = x[Keyword-1000]
- _ = x[KeywordConstant-1001]
- _ = x[KeywordDeclaration-1002]
- _ = x[KeywordNamespace-1003]
- _ = x[KeywordPseudo-1004]
- _ = x[KeywordReserved-1005]
- _ = x[KeywordType-1006]
- _ = x[Name-2000]
- _ = x[NameAttribute-2001]
- _ = x[NameBuiltin-2002]
- _ = x[NameBuiltinPseudo-2003]
- _ = x[NameClass-2004]
- _ = x[NameConstant-2005]
- _ = x[NameDecorator-2006]
- _ = x[NameEntity-2007]
- _ = x[NameException-2008]
- _ = x[NameFunction-2009]
- _ = x[NameFunctionMagic-2010]
- _ = x[NameKeyword-2011]
- _ = x[NameLabel-2012]
- _ = x[NameNamespace-2013]
- _ = x[NameOperator-2014]
- _ = x[NameOther-2015]
- _ = x[NamePseudo-2016]
- _ = x[NameProperty-2017]
- _ = x[NameTag-2018]
- _ = x[NameVariable-2019]
- _ = x[NameVariableAnonymous-2020]
- _ = x[NameVariableClass-2021]
- _ = x[NameVariableGlobal-2022]
- _ = x[NameVariableInstance-2023]
- _ = x[NameVariableMagic-2024]
- _ = x[Literal-3000]
- _ = x[LiteralDate-3001]
- _ = x[LiteralOther-3002]
- _ = x[LiteralString-3100]
- _ = x[LiteralStringAffix-3101]
- _ = x[LiteralStringAtom-3102]
- _ = x[LiteralStringBacktick-3103]
- _ = x[LiteralStringBoolean-3104]
- _ = x[LiteralStringChar-3105]
- _ = x[LiteralStringDelimiter-3106]
- _ = x[LiteralStringDoc-3107]
- _ = x[LiteralStringDouble-3108]
- _ = x[LiteralStringEscape-3109]
- _ = x[LiteralStringHeredoc-3110]
- _ = x[LiteralStringInterpol-3111]
- _ = x[LiteralStringName-3112]
- _ = x[LiteralStringOther-3113]
- _ = x[LiteralStringRegex-3114]
- _ = x[LiteralStringSingle-3115]
- _ = x[LiteralStringSymbol-3116]
- _ = x[LiteralNumber-3200]
- _ = x[LiteralNumberBin-3201]
- _ = x[LiteralNumberFloat-3202]
- _ = x[LiteralNumberHex-3203]
- _ = x[LiteralNumberInteger-3204]
- _ = x[LiteralNumberIntegerLong-3205]
- _ = x[LiteralNumberOct-3206]
- _ = x[Operator-4000]
- _ = x[OperatorWord-4001]
- _ = x[Punctuation-5000]
- _ = x[Comment-6000]
- _ = x[CommentHashbang-6001]
- _ = x[CommentMultiline-6002]
- _ = x[CommentSingle-6003]
- _ = x[CommentSpecial-6004]
- _ = x[CommentPreproc-6100]
- _ = x[CommentPreprocFile-6101]
- _ = x[Generic-7000]
- _ = x[GenericDeleted-7001]
- _ = x[GenericEmph-7002]
- _ = x[GenericError-7003]
- _ = x[GenericHeading-7004]
- _ = x[GenericInserted-7005]
- _ = x[GenericOutput-7006]
- _ = x[GenericPrompt-7007]
- _ = x[GenericStrong-7008]
- _ = x[GenericSubheading-7009]
- _ = x[GenericTraceback-7010]
- _ = x[GenericUnderline-7011]
- _ = x[Text-8000]
- _ = x[TextWhitespace-8001]
- _ = x[TextSymbol-8002]
- _ = x[TextPunctuation-8003]
-}
-
-const _TokenType_name = "NoneOtherErrorLineTableTDLineTableLineHighlightLineNumbersTableLineNumbersBackgroundEOFTypeKeywordKeywordConstantKeywordDeclarationKeywordNamespaceKeywordPseudoKeywordReservedKeywordTypeNameNameAttributeNameBuiltinNameBuiltinPseudoNameClassNameConstantNameDecoratorNameEntityNameExceptionNameFunctionNameFunctionMagicNameKeywordNameLabelNameNamespaceNameOperatorNameOtherNamePseudoNamePropertyNameTagNameVariableNameVariableAnonymousNameVariableClassNameVariableGlobalNameVariableInstanceNameVariableMagicLiteralLiteralDateLiteralOtherLiteralStringLiteralStringAffixLiteralStringAtomLiteralStringBacktickLiteralStringBooleanLiteralStringCharLiteralStringDelimiterLiteralStringDocLiteralStringDoubleLiteralStringEscapeLiteralStringHeredocLiteralStringInterpolLiteralStringNameLiteralStringOtherLiteralStringRegexLiteralStringSingleLiteralStringSymbolLiteralNumberLiteralNumberBinLiteralNumberFloatLiteralNumberHexLiteralNumberIntegerLiteralNumberIntegerLongLiteralNumberOctOperatorOperatorWordPunctuationCommentCommentHashbangCommentMultilineCommentSingleCommentSpecialCommentPreprocCommentPreprocFileGenericGenericDeletedGenericEmphGenericErrorGenericHeadingGenericInsertedGenericOutputGenericPromptGenericStrongGenericSubheadingGenericTracebackGenericUnderlineTextTextWhitespaceTextSymbolTextPunctuation"
-
-var _TokenType_map = map[TokenType]string{
- -9: _TokenType_name[0:4],
- -8: _TokenType_name[4:9],
- -7: _TokenType_name[9:14],
- -6: _TokenType_name[14:25],
- -5: _TokenType_name[25:34],
- -4: _TokenType_name[34:47],
- -3: _TokenType_name[47:63],
- -2: _TokenType_name[63:74],
- -1: _TokenType_name[74:84],
- 0: _TokenType_name[84:91],
- 1000: _TokenType_name[91:98],
- 1001: _TokenType_name[98:113],
- 1002: _TokenType_name[113:131],
- 1003: _TokenType_name[131:147],
- 1004: _TokenType_name[147:160],
- 1005: _TokenType_name[160:175],
- 1006: _TokenType_name[175:186],
- 2000: _TokenType_name[186:190],
- 2001: _TokenType_name[190:203],
- 2002: _TokenType_name[203:214],
- 2003: _TokenType_name[214:231],
- 2004: _TokenType_name[231:240],
- 2005: _TokenType_name[240:252],
- 2006: _TokenType_name[252:265],
- 2007: _TokenType_name[265:275],
- 2008: _TokenType_name[275:288],
- 2009: _TokenType_name[288:300],
- 2010: _TokenType_name[300:317],
- 2011: _TokenType_name[317:328],
- 2012: _TokenType_name[328:337],
- 2013: _TokenType_name[337:350],
- 2014: _TokenType_name[350:362],
- 2015: _TokenType_name[362:371],
- 2016: _TokenType_name[371:381],
- 2017: _TokenType_name[381:393],
- 2018: _TokenType_name[393:400],
- 2019: _TokenType_name[400:412],
- 2020: _TokenType_name[412:433],
- 2021: _TokenType_name[433:450],
- 2022: _TokenType_name[450:468],
- 2023: _TokenType_name[468:488],
- 2024: _TokenType_name[488:505],
- 3000: _TokenType_name[505:512],
- 3001: _TokenType_name[512:523],
- 3002: _TokenType_name[523:535],
- 3100: _TokenType_name[535:548],
- 3101: _TokenType_name[548:566],
- 3102: _TokenType_name[566:583],
- 3103: _TokenType_name[583:604],
- 3104: _TokenType_name[604:624],
- 3105: _TokenType_name[624:641],
- 3106: _TokenType_name[641:663],
- 3107: _TokenType_name[663:679],
- 3108: _TokenType_name[679:698],
- 3109: _TokenType_name[698:717],
- 3110: _TokenType_name[717:737],
- 3111: _TokenType_name[737:758],
- 3112: _TokenType_name[758:775],
- 3113: _TokenType_name[775:793],
- 3114: _TokenType_name[793:811],
- 3115: _TokenType_name[811:830],
- 3116: _TokenType_name[830:849],
- 3200: _TokenType_name[849:862],
- 3201: _TokenType_name[862:878],
- 3202: _TokenType_name[878:896],
- 3203: _TokenType_name[896:912],
- 3204: _TokenType_name[912:932],
- 3205: _TokenType_name[932:956],
- 3206: _TokenType_name[956:972],
- 4000: _TokenType_name[972:980],
- 4001: _TokenType_name[980:992],
- 5000: _TokenType_name[992:1003],
- 6000: _TokenType_name[1003:1010],
- 6001: _TokenType_name[1010:1025],
- 6002: _TokenType_name[1025:1041],
- 6003: _TokenType_name[1041:1054],
- 6004: _TokenType_name[1054:1068],
- 6100: _TokenType_name[1068:1082],
- 6101: _TokenType_name[1082:1100],
- 7000: _TokenType_name[1100:1107],
- 7001: _TokenType_name[1107:1121],
- 7002: _TokenType_name[1121:1132],
- 7003: _TokenType_name[1132:1144],
- 7004: _TokenType_name[1144:1158],
- 7005: _TokenType_name[1158:1173],
- 7006: _TokenType_name[1173:1186],
- 7007: _TokenType_name[1186:1199],
- 7008: _TokenType_name[1199:1212],
- 7009: _TokenType_name[1212:1229],
- 7010: _TokenType_name[1229:1245],
- 7011: _TokenType_name[1245:1261],
- 8000: _TokenType_name[1261:1265],
- 8001: _TokenType_name[1265:1279],
- 8002: _TokenType_name[1279:1289],
- 8003: _TokenType_name[1289:1304],
-}
-
-func (i TokenType) String() string {
- if str, ok := _TokenType_map[i]; ok {
- return str
- }
- return "TokenType(" + strconv.FormatInt(int64(i), 10) + ")"
-}
diff --git a/vendor/github.com/alecthomas/chroma/types.go b/vendor/github.com/alecthomas/chroma/types.go
deleted file mode 100644
index ede945c..0000000
--- a/vendor/github.com/alecthomas/chroma/types.go
+++ /dev/null
@@ -1,347 +0,0 @@
-package chroma
-
-import (
- "encoding/json"
- "fmt"
-)
-
-//go:generate stringer -type TokenType
-
-// TokenType is the type of token to highlight.
-//
-// It is also an Emitter, emitting a single token of itself
-type TokenType int
-
-func (t TokenType) MarshalJSON() ([]byte, error) { return json.Marshal(t.String()) }
-func (t *TokenType) UnmarshalJSON(data []byte) error {
- key := ""
- err := json.Unmarshal(data, &key)
- if err != nil {
- return err
- }
- for tt, text := range _TokenType_map {
- if text == key {
- *t = tt
- return nil
- }
- }
- return fmt.Errorf("unknown TokenType %q", data)
-}
-
-// Set of TokenTypes.
-//
-// Categories of types are grouped in ranges of 1000, while sub-categories are in ranges of 100. For
-// example, the literal category is in the range 3000-3999. The sub-category for literal strings is
-// in the range 3100-3199.
-
-// Meta token types.
-const (
- // Default background style.
- Background TokenType = -1 - iota
- // Line numbers in output.
- LineNumbers
- // Line numbers in output when in table.
- LineNumbersTable
- // Line higlight style.
- LineHighlight
- // Line numbers table wrapper style.
- LineTable
- // Line numbers table TD wrapper style.
- LineTableTD
- // Input that could not be tokenised.
- Error
- // Other is used by the Delegate lexer to indicate which tokens should be handled by the delegate.
- Other
- // No highlighting.
- None
- // Used as an EOF marker / nil token
- EOFType TokenType = 0
-)
-
-// Keywords.
-const (
- Keyword TokenType = 1000 + iota
- KeywordConstant
- KeywordDeclaration
- KeywordNamespace
- KeywordPseudo
- KeywordReserved
- KeywordType
-)
-
-// Names.
-const (
- Name TokenType = 2000 + iota
- NameAttribute
- NameBuiltin
- NameBuiltinPseudo
- NameClass
- NameConstant
- NameDecorator
- NameEntity
- NameException
- NameFunction
- NameFunctionMagic
- NameKeyword
- NameLabel
- NameNamespace
- NameOperator
- NameOther
- NamePseudo
- NameProperty
- NameTag
- NameVariable
- NameVariableAnonymous
- NameVariableClass
- NameVariableGlobal
- NameVariableInstance
- NameVariableMagic
-)
-
-// Literals.
-const (
- Literal TokenType = 3000 + iota
- LiteralDate
- LiteralOther
-)
-
-// Strings.
-const (
- LiteralString TokenType = 3100 + iota
- LiteralStringAffix
- LiteralStringAtom
- LiteralStringBacktick
- LiteralStringBoolean
- LiteralStringChar
- LiteralStringDelimiter
- LiteralStringDoc
- LiteralStringDouble
- LiteralStringEscape
- LiteralStringHeredoc
- LiteralStringInterpol
- LiteralStringName
- LiteralStringOther
- LiteralStringRegex
- LiteralStringSingle
- LiteralStringSymbol
-)
-
-// Literals.
-const (
- LiteralNumber TokenType = 3200 + iota
- LiteralNumberBin
- LiteralNumberFloat
- LiteralNumberHex
- LiteralNumberInteger
- LiteralNumberIntegerLong
- LiteralNumberOct
-)
-
-// Operators.
-const (
- Operator TokenType = 4000 + iota
- OperatorWord
-)
-
-// Punctuation.
-const (
- Punctuation TokenType = 5000 + iota
-)
-
-// Comments.
-const (
- Comment TokenType = 6000 + iota
- CommentHashbang
- CommentMultiline
- CommentSingle
- CommentSpecial
-)
-
-// Preprocessor "comments".
-const (
- CommentPreproc TokenType = 6100 + iota
- CommentPreprocFile
-)
-
-// Generic tokens.
-const (
- Generic TokenType = 7000 + iota
- GenericDeleted
- GenericEmph
- GenericError
- GenericHeading
- GenericInserted
- GenericOutput
- GenericPrompt
- GenericStrong
- GenericSubheading
- GenericTraceback
- GenericUnderline
-)
-
-// Text.
-const (
- Text TokenType = 8000 + iota
- TextWhitespace
- TextSymbol
- TextPunctuation
-)
-
-// Aliases.
-const (
- Whitespace = TextWhitespace
-
- Date = LiteralDate
-
- String = LiteralString
- StringAffix = LiteralStringAffix
- StringBacktick = LiteralStringBacktick
- StringChar = LiteralStringChar
- StringDelimiter = LiteralStringDelimiter
- StringDoc = LiteralStringDoc
- StringDouble = LiteralStringDouble
- StringEscape = LiteralStringEscape
- StringHeredoc = LiteralStringHeredoc
- StringInterpol = LiteralStringInterpol
- StringOther = LiteralStringOther
- StringRegex = LiteralStringRegex
- StringSingle = LiteralStringSingle
- StringSymbol = LiteralStringSymbol
-
- Number = LiteralNumber
- NumberBin = LiteralNumberBin
- NumberFloat = LiteralNumberFloat
- NumberHex = LiteralNumberHex
- NumberInteger = LiteralNumberInteger
- NumberIntegerLong = LiteralNumberIntegerLong
- NumberOct = LiteralNumberOct
-)
-
-var (
- StandardTypes = map[TokenType]string{
- Background: "chroma",
- LineNumbers: "ln",
- LineNumbersTable: "lnt",
- LineHighlight: "hl",
- LineTable: "lntable",
- LineTableTD: "lntd",
- Text: "",
- Whitespace: "w",
- Error: "err",
- Other: "x",
- // I have no idea what this is used for...
- // Escape: "esc",
-
- Keyword: "k",
- KeywordConstant: "kc",
- KeywordDeclaration: "kd",
- KeywordNamespace: "kn",
- KeywordPseudo: "kp",
- KeywordReserved: "kr",
- KeywordType: "kt",
-
- Name: "n",
- NameAttribute: "na",
- NameBuiltin: "nb",
- NameBuiltinPseudo: "bp",
- NameClass: "nc",
- NameConstant: "no",
- NameDecorator: "nd",
- NameEntity: "ni",
- NameException: "ne",
- NameFunction: "nf",
- NameFunctionMagic: "fm",
- NameProperty: "py",
- NameLabel: "nl",
- NameNamespace: "nn",
- NameOther: "nx",
- NameTag: "nt",
- NameVariable: "nv",
- NameVariableClass: "vc",
- NameVariableGlobal: "vg",
- NameVariableInstance: "vi",
- NameVariableMagic: "vm",
-
- Literal: "l",
- LiteralDate: "ld",
-
- String: "s",
- StringAffix: "sa",
- StringBacktick: "sb",
- StringChar: "sc",
- StringDelimiter: "dl",
- StringDoc: "sd",
- StringDouble: "s2",
- StringEscape: "se",
- StringHeredoc: "sh",
- StringInterpol: "si",
- StringOther: "sx",
- StringRegex: "sr",
- StringSingle: "s1",
- StringSymbol: "ss",
-
- Number: "m",
- NumberBin: "mb",
- NumberFloat: "mf",
- NumberHex: "mh",
- NumberInteger: "mi",
- NumberIntegerLong: "il",
- NumberOct: "mo",
-
- Operator: "o",
- OperatorWord: "ow",
-
- Punctuation: "p",
-
- Comment: "c",
- CommentHashbang: "ch",
- CommentMultiline: "cm",
- CommentPreproc: "cp",
- CommentPreprocFile: "cpf",
- CommentSingle: "c1",
- CommentSpecial: "cs",
-
- Generic: "g",
- GenericDeleted: "gd",
- GenericEmph: "ge",
- GenericError: "gr",
- GenericHeading: "gh",
- GenericInserted: "gi",
- GenericOutput: "go",
- GenericPrompt: "gp",
- GenericStrong: "gs",
- GenericSubheading: "gu",
- GenericTraceback: "gt",
- GenericUnderline: "gl",
- }
-)
-
-func (t TokenType) Parent() TokenType {
- if t%100 != 0 {
- return t / 100 * 100
- }
- if t%1000 != 0 {
- return t / 1000 * 1000
- }
- return 0
-}
-
-func (t TokenType) Category() TokenType {
- return t / 1000 * 1000
-}
-
-func (t TokenType) SubCategory() TokenType {
- return t / 100 * 100
-}
-
-func (t TokenType) InCategory(other TokenType) bool {
- return t/1000 == other/1000
-}
-
-func (t TokenType) InSubCategory(other TokenType) bool {
- return t/100 == other/100
-}
-
-func (t TokenType) Emit(groups []string, _ *LexerState) Iterator {
- return Literator(Token{Type: t, Value: groups[0]})
-}
diff --git a/vendor/github.com/araddon/dateparse/.travis.yml b/vendor/github.com/araddon/dateparse/.travis.yml
deleted file mode 100644
index 3b4b177..0000000
--- a/vendor/github.com/araddon/dateparse/.travis.yml
+++ /dev/null
@@ -1,13 +0,0 @@
-language: go
-
-go:
- - 1.13.x
-
-before_install:
- - go get -t -v ./...
-
-script:
- - go test -race -coverprofile=coverage.txt -covermode=atomic
-
-after_success:
- - bash <(curl -s https://codecov.io/bash)
diff --git a/vendor/github.com/araddon/dateparse/LICENSE b/vendor/github.com/araddon/dateparse/LICENSE
deleted file mode 100644
index f675ed3..0000000
--- a/vendor/github.com/araddon/dateparse/LICENSE
+++ /dev/null
@@ -1,21 +0,0 @@
-The MIT License (MIT)
-
-Copyright (c) 2015-2017 Aaron Raddon
-
-Permission is hereby granted, free of charge, to any person obtaining a copy
-of this software and associated documentation files (the "Software"), to deal
-in the Software without restriction, including without limitation the rights
-to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
-copies of the Software, and to permit persons to whom the Software is
-furnished to do so, subject to the following conditions:
-
-The above copyright notice and this permission notice shall be included in
-all copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
-IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
-FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
-AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
-LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
-OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
-THE SOFTWARE.
diff --git a/vendor/github.com/araddon/dateparse/README.md b/vendor/github.com/araddon/dateparse/README.md
deleted file mode 100644
index fe682dd..0000000
--- a/vendor/github.com/araddon/dateparse/README.md
+++ /dev/null
@@ -1,323 +0,0 @@
-Go Date Parser
----------------------------
-
-Parse many date strings without knowing format in advance. Uses a scanner to read bytes and use a state machine to find format. Much faster than shotgun based parse methods. See [bench_test.go](https://github.com/araddon/dateparse/blob/master/bench_test.go) for performance comparison.
-
-
-[](https://codecov.io/gh/araddon/dateparse)
-[](http://godoc.org/github.com/araddon/dateparse)
-[](https://travis-ci.org/araddon/dateparse)
-[](https://goreportcard.com/report/araddon/dateparse)
-
-**MM/DD/YYYY VS DD/MM/YYYY** Right now this uses mm/dd/yyyy WHEN ambiguous if this is not desired behavior, use `ParseStrict` which will fail on ambiguous date strings.
-
-**Timezones** The location your server is configured affects the results! See example or https://play.golang.org/p/IDHRalIyXh and last paragraph here https://golang.org/pkg/time/#Parse.
-
-
-```go
-
-// Normal parse. Equivalent Timezone rules as time.Parse()
-t, err := dateparse.ParseAny("3/1/2014")
-
-// Parse Strict, error on ambigous mm/dd vs dd/mm dates
-t, err := dateparse.ParseStrict("3/1/2014")
-> returns error
-
-// Return a string that represents the layout to parse the given date-time.
-layout, err := dateparse.ParseFormat("May 8, 2009 5:57:51 PM")
-> "Jan 2, 2006 3:04:05 PM"
-
-```
-
-cli tool for testing dateformats
-----------------------------------
-
-[Date Parse CLI](https://github.com/araddon/dateparse/blob/master/dateparse)
-
-
-Extended example
--------------------
-
-https://github.com/araddon/dateparse/blob/master/example/main.go
-
-```go
-package main
-
-import (
- "flag"
- "fmt"
- "time"
-
- "github.com/scylladb/termtables"
- "github.com/araddon/dateparse"
-)
-
-var examples = []string{
- "May 8, 2009 5:57:51 PM",
- "oct 7, 1970",
- "oct 7, '70",
- "oct. 7, 1970",
- "oct. 7, 70",
- "Mon Jan 2 15:04:05 2006",
- "Mon Jan 2 15:04:05 MST 2006",
- "Mon Jan 02 15:04:05 -0700 2006",
- "Monday, 02-Jan-06 15:04:05 MST",
- "Mon, 02 Jan 2006 15:04:05 MST",
- "Tue, 11 Jul 2017 16:28:13 +0200 (CEST)",
- "Mon, 02 Jan 2006 15:04:05 -0700",
- "Mon 30 Sep 2018 09:09:09 PM UTC",
- "Mon Aug 10 15:44:11 UTC+0100 2015",
- "Thu, 4 Jan 2018 17:53:36 +0000",
- "Fri Jul 03 2015 18:04:07 GMT+0100 (GMT Daylight Time)",
- "Sun, 3 Jan 2021 00:12:23 +0800 (GMT+08:00)",
- "September 17, 2012 10:09am",
- "September 17, 2012 at 10:09am PST-08",
- "September 17, 2012, 10:10:09",
- "October 7, 1970",
- "October 7th, 1970",
- "12 Feb 2006, 19:17",
- "12 Feb 2006 19:17",
- "14 May 2019 19:11:40.164",
- "7 oct 70",
- "7 oct 1970",
- "03 February 2013",
- "1 July 2013",
- "2013-Feb-03",
- // dd/Mon/yyy alpha Months
- "06/Jan/2008:15:04:05 -0700",
- "06/Jan/2008 15:04:05 -0700",
- // mm/dd/yy
- "3/31/2014",
- "03/31/2014",
- "08/21/71",
- "8/1/71",
- "4/8/2014 22:05",
- "04/08/2014 22:05",
- "4/8/14 22:05",
- "04/2/2014 03:00:51",
- "8/8/1965 12:00:00 AM",
- "8/8/1965 01:00:01 PM",
- "8/8/1965 01:00 PM",
- "8/8/1965 1:00 PM",
- "8/8/1965 12:00 AM",
- "4/02/2014 03:00:51",
- "03/19/2012 10:11:59",
- "03/19/2012 10:11:59.3186369",
- // yyyy/mm/dd
- "2014/3/31",
- "2014/03/31",
- "2014/4/8 22:05",
- "2014/04/08 22:05",
- "2014/04/2 03:00:51",
- "2014/4/02 03:00:51",
- "2012/03/19 10:11:59",
- "2012/03/19 10:11:59.3186369",
- // yyyy:mm:dd
- "2014:3:31",
- "2014:03:31",
- "2014:4:8 22:05",
- "2014:04:08 22:05",
- "2014:04:2 03:00:51",
- "2014:4:02 03:00:51",
- "2012:03:19 10:11:59",
- "2012:03:19 10:11:59.3186369",
- // Chinese
- "2014年04月08日",
- // yyyy-mm-ddThh
- "2006-01-02T15:04:05+0000",
- "2009-08-12T22:15:09-07:00",
- "2009-08-12T22:15:09",
- "2009-08-12T22:15:09.988",
- "2009-08-12T22:15:09Z",
- "2017-07-19T03:21:51:897+0100",
- "2019-05-29T08:41-04", // no seconds, 2 digit TZ offset
- // yyyy-mm-dd hh:mm:ss
- "2014-04-26 17:24:37.3186369",
- "2012-08-03 18:31:59.257000000",
- "2014-04-26 17:24:37.123",
- "2013-04-01 22:43",
- "2013-04-01 22:43:22",
- "2014-12-16 06:20:00 UTC",
- "2014-12-16 06:20:00 GMT",
- "2014-04-26 05:24:37 PM",
- "2014-04-26 13:13:43 +0800",
- "2014-04-26 13:13:43 +0800 +08",
- "2014-04-26 13:13:44 +09:00",
- "2012-08-03 18:31:59.257000000 +0000 UTC",
- "2015-09-30 18:48:56.35272715 +0000 UTC",
- "2015-02-18 00:12:00 +0000 GMT",
- "2015-02-18 00:12:00 +0000 UTC",
- "2015-02-08 03:02:00 +0300 MSK m=+0.000000001",
- "2015-02-08 03:02:00.001 +0300 MSK m=+0.000000001",
- "2017-07-19 03:21:51+00:00",
- "2014-04-26",
- "2014-04",
- "2014",
- "2014-05-11 08:20:13,787",
- // yyyy-mm-dd-07:00
- "2020-07-20+08:00",
- // mm.dd.yy
- "3.31.2014",
- "03.31.2014",
- "08.21.71",
- "2014.03",
- "2014.03.30",
- // yyyymmdd and similar
- "20140601",
- "20140722105203",
- // yymmdd hh:mm:yy mysql log
- // 080313 05:21:55 mysqld started
- "171113 14:14:20",
- // unix seconds, ms, micro, nano
- "1332151919",
- "1384216367189",
- "1384216367111222",
- "1384216367111222333",
-}
-
-var (
- timezone = ""
-)
-
-func main() {
- flag.StringVar(&timezone, "timezone", "UTC", "Timezone aka `America/Los_Angeles` formatted time-zone")
- flag.Parse()
-
- if timezone != "" {
- // NOTE: This is very, very important to understand
- // time-parsing in go
- loc, err := time.LoadLocation(timezone)
- if err != nil {
- panic(err.Error())
- }
- time.Local = loc
- }
-
- table := termtables.CreateTable()
-
- table.AddHeaders("Input", "Parsed, and Output as %v")
- for _, dateExample := range examples {
- t, err := dateparse.ParseLocal(dateExample)
- if err != nil {
- panic(err.Error())
- }
- table.AddRow(dateExample, fmt.Sprintf("%v", t))
- }
- fmt.Println(table.Render())
-}
-
-/*
-+-------------------------------------------------------+-----------------------------------------+
-| Input | Parsed, and Output as %v |
-+-------------------------------------------------------+-----------------------------------------+
-| May 8, 2009 5:57:51 PM | 2009-05-08 17:57:51 +0000 UTC |
-| oct 7, 1970 | 1970-10-07 00:00:00 +0000 UTC |
-| oct 7, '70 | 1970-10-07 00:00:00 +0000 UTC |
-| oct. 7, 1970 | 1970-10-07 00:00:00 +0000 UTC |
-| oct. 7, 70 | 1970-10-07 00:00:00 +0000 UTC |
-| Mon Jan 2 15:04:05 2006 | 2006-01-02 15:04:05 +0000 UTC |
-| Mon Jan 2 15:04:05 MST 2006 | 2006-01-02 15:04:05 +0000 MST |
-| Mon Jan 02 15:04:05 -0700 2006 | 2006-01-02 15:04:05 -0700 -0700 |
-| Monday, 02-Jan-06 15:04:05 MST | 2006-01-02 15:04:05 +0000 MST |
-| Mon, 02 Jan 2006 15:04:05 MST | 2006-01-02 15:04:05 +0000 MST |
-| Tue, 11 Jul 2017 16:28:13 +0200 (CEST) | 2017-07-11 16:28:13 +0200 +0200 |
-| Mon, 02 Jan 2006 15:04:05 -0700 | 2006-01-02 15:04:05 -0700 -0700 |
-| Mon 30 Sep 2018 09:09:09 PM UTC | 2018-09-30 21:09:09 +0000 UTC |
-| Mon Aug 10 15:44:11 UTC+0100 2015 | 2015-08-10 15:44:11 +0000 UTC |
-| Thu, 4 Jan 2018 17:53:36 +0000 | 2018-01-04 17:53:36 +0000 UTC |
-| Fri Jul 03 2015 18:04:07 GMT+0100 (GMT Daylight Time) | 2015-07-03 18:04:07 +0100 GMT |
-| Sun, 3 Jan 2021 00:12:23 +0800 (GMT+08:00) | 2021-01-03 00:12:23 +0800 +0800 |
-| September 17, 2012 10:09am | 2012-09-17 10:09:00 +0000 UTC |
-| September 17, 2012 at 10:09am PST-08 | 2012-09-17 10:09:00 -0800 PST |
-| September 17, 2012, 10:10:09 | 2012-09-17 10:10:09 +0000 UTC |
-| October 7, 1970 | 1970-10-07 00:00:00 +0000 UTC |
-| October 7th, 1970 | 1970-10-07 00:00:00 +0000 UTC |
-| 12 Feb 2006, 19:17 | 2006-02-12 19:17:00 +0000 UTC |
-| 12 Feb 2006 19:17 | 2006-02-12 19:17:00 +0000 UTC |
-| 14 May 2019 19:11:40.164 | 2019-05-14 19:11:40.164 +0000 UTC |
-| 7 oct 70 | 1970-10-07 00:00:00 +0000 UTC |
-| 7 oct 1970 | 1970-10-07 00:00:00 +0000 UTC |
-| 03 February 2013 | 2013-02-03 00:00:00 +0000 UTC |
-| 1 July 2013 | 2013-07-01 00:00:00 +0000 UTC |
-| 2013-Feb-03 | 2013-02-03 00:00:00 +0000 UTC |
-| 06/Jan/2008:15:04:05 -0700 | 2008-01-06 15:04:05 -0700 -0700 |
-| 06/Jan/2008 15:04:05 -0700 | 2008-01-06 15:04:05 -0700 -0700 |
-| 3/31/2014 | 2014-03-31 00:00:00 +0000 UTC |
-| 03/31/2014 | 2014-03-31 00:00:00 +0000 UTC |
-| 08/21/71 | 1971-08-21 00:00:00 +0000 UTC |
-| 8/1/71 | 1971-08-01 00:00:00 +0000 UTC |
-| 4/8/2014 22:05 | 2014-04-08 22:05:00 +0000 UTC |
-| 04/08/2014 22:05 | 2014-04-08 22:05:00 +0000 UTC |
-| 4/8/14 22:05 | 2014-04-08 22:05:00 +0000 UTC |
-| 04/2/2014 03:00:51 | 2014-04-02 03:00:51 +0000 UTC |
-| 8/8/1965 12:00:00 AM | 1965-08-08 00:00:00 +0000 UTC |
-| 8/8/1965 01:00:01 PM | 1965-08-08 13:00:01 +0000 UTC |
-| 8/8/1965 01:00 PM | 1965-08-08 13:00:00 +0000 UTC |
-| 8/8/1965 1:00 PM | 1965-08-08 13:00:00 +0000 UTC |
-| 8/8/1965 12:00 AM | 1965-08-08 00:00:00 +0000 UTC |
-| 4/02/2014 03:00:51 | 2014-04-02 03:00:51 +0000 UTC |
-| 03/19/2012 10:11:59 | 2012-03-19 10:11:59 +0000 UTC |
-| 03/19/2012 10:11:59.3186369 | 2012-03-19 10:11:59.3186369 +0000 UTC |
-| 2014/3/31 | 2014-03-31 00:00:00 +0000 UTC |
-| 2014/03/31 | 2014-03-31 00:00:00 +0000 UTC |
-| 2014/4/8 22:05 | 2014-04-08 22:05:00 +0000 UTC |
-| 2014/04/08 22:05 | 2014-04-08 22:05:00 +0000 UTC |
-| 2014/04/2 03:00:51 | 2014-04-02 03:00:51 +0000 UTC |
-| 2014/4/02 03:00:51 | 2014-04-02 03:00:51 +0000 UTC |
-| 2012/03/19 10:11:59 | 2012-03-19 10:11:59 +0000 UTC |
-| 2012/03/19 10:11:59.3186369 | 2012-03-19 10:11:59.3186369 +0000 UTC |
-| 2014:3:31 | 2014-03-31 00:00:00 +0000 UTC |
-| 2014:03:31 | 2014-03-31 00:00:00 +0000 UTC |
-| 2014:4:8 22:05 | 2014-04-08 22:05:00 +0000 UTC |
-| 2014:04:08 22:05 | 2014-04-08 22:05:00 +0000 UTC |
-| 2014:04:2 03:00:51 | 2014-04-02 03:00:51 +0000 UTC |
-| 2014:4:02 03:00:51 | 2014-04-02 03:00:51 +0000 UTC |
-| 2012:03:19 10:11:59 | 2012-03-19 10:11:59 +0000 UTC |
-| 2012:03:19 10:11:59.3186369 | 2012-03-19 10:11:59.3186369 +0000 UTC |
-| 2014年04月08日 | 2014-04-08 00:00:00 +0000 UTC |
-| 2006-01-02T15:04:05+0000 | 2006-01-02 15:04:05 +0000 UTC |
-| 2009-08-12T22:15:09-07:00 | 2009-08-12 22:15:09 -0700 -0700 |
-| 2009-08-12T22:15:09 | 2009-08-12 22:15:09 +0000 UTC |
-| 2009-08-12T22:15:09.988 | 2009-08-12 22:15:09.988 +0000 UTC |
-| 2009-08-12T22:15:09Z | 2009-08-12 22:15:09 +0000 UTC |
-| 2017-07-19T03:21:51:897+0100 | 2017-07-19 03:21:51.897 +0100 +0100 |
-| 2019-05-29T08:41-04 | 2019-05-29 08:41:00 -0400 -0400 |
-| 2014-04-26 17:24:37.3186369 | 2014-04-26 17:24:37.3186369 +0000 UTC |
-| 2012-08-03 18:31:59.257000000 | 2012-08-03 18:31:59.257 +0000 UTC |
-| 2014-04-26 17:24:37.123 | 2014-04-26 17:24:37.123 +0000 UTC |
-| 2013-04-01 22:43 | 2013-04-01 22:43:00 +0000 UTC |
-| 2013-04-01 22:43:22 | 2013-04-01 22:43:22 +0000 UTC |
-| 2014-12-16 06:20:00 UTC | 2014-12-16 06:20:00 +0000 UTC |
-| 2014-12-16 06:20:00 GMT | 2014-12-16 06:20:00 +0000 UTC |
-| 2014-04-26 05:24:37 PM | 2014-04-26 17:24:37 +0000 UTC |
-| 2014-04-26 13:13:43 +0800 | 2014-04-26 13:13:43 +0800 +0800 |
-| 2014-04-26 13:13:43 +0800 +08 | 2014-04-26 13:13:43 +0800 +0800 |
-| 2014-04-26 13:13:44 +09:00 | 2014-04-26 13:13:44 +0900 +0900 |
-| 2012-08-03 18:31:59.257000000 +0000 UTC | 2012-08-03 18:31:59.257 +0000 UTC |
-| 2015-09-30 18:48:56.35272715 +0000 UTC | 2015-09-30 18:48:56.35272715 +0000 UTC |
-| 2015-02-18 00:12:00 +0000 GMT | 2015-02-18 00:12:00 +0000 UTC |
-| 2015-02-18 00:12:00 +0000 UTC | 2015-02-18 00:12:00 +0000 UTC |
-| 2015-02-08 03:02:00 +0300 MSK m=+0.000000001 | 2015-02-08 03:02:00 +0300 +0300 |
-| 2015-02-08 03:02:00.001 +0300 MSK m=+0.000000001 | 2015-02-08 03:02:00.001 +0300 +0300 |
-| 2017-07-19 03:21:51+00:00 | 2017-07-19 03:21:51 +0000 UTC |
-| 2014-04-26 | 2014-04-26 00:00:00 +0000 UTC |
-| 2014-04 | 2014-04-01 00:00:00 +0000 UTC |
-| 2014 | 2014-01-01 00:00:00 +0000 UTC |
-| 2014-05-11 08:20:13,787 | 2014-05-11 08:20:13.787 +0000 UTC |
-| 2020-07-20+08:00 | 2020-07-20 00:00:00 +0800 +0800 |
-| 3.31.2014 | 2014-03-31 00:00:00 +0000 UTC |
-| 03.31.2014 | 2014-03-31 00:00:00 +0000 UTC |
-| 08.21.71 | 1971-08-21 00:00:00 +0000 UTC |
-| 2014.03 | 2014-03-01 00:00:00 +0000 UTC |
-| 2014.03.30 | 2014-03-30 00:00:00 +0000 UTC |
-| 20140601 | 2014-06-01 00:00:00 +0000 UTC |
-| 20140722105203 | 2014-07-22 10:52:03 +0000 UTC |
-| 171113 14:14:20 | 2017-11-13 14:14:20 +0000 UTC |
-| 1332151919 | 2012-03-19 10:11:59 +0000 UTC |
-| 1384216367189 | 2013-11-12 00:32:47.189 +0000 UTC |
-| 1384216367111222 | 2013-11-12 00:32:47.111222 +0000 UTC |
-| 1384216367111222333 | 2013-11-12 00:32:47.111222333 +0000 UTC |
-+-------------------------------------------------------+-----------------------------------------+
-*/
-
-```
diff --git a/vendor/github.com/araddon/dateparse/go.mod b/vendor/github.com/araddon/dateparse/go.mod
deleted file mode 100644
index 071cd5e..0000000
--- a/vendor/github.com/araddon/dateparse/go.mod
+++ /dev/null
@@ -1,9 +0,0 @@
-module github.com/araddon/dateparse
-
-go 1.12
-
-require (
- github.com/mattn/go-runewidth v0.0.10 // indirect
- github.com/scylladb/termtables v0.0.0-20191203121021-c4c0b6d42ff4
- github.com/stretchr/testify v1.7.0
-)
diff --git a/vendor/github.com/araddon/dateparse/go.sum b/vendor/github.com/araddon/dateparse/go.sum
deleted file mode 100644
index 40bf744..0000000
--- a/vendor/github.com/araddon/dateparse/go.sum
+++ /dev/null
@@ -1,18 +0,0 @@
-github.com/davecgh/go-spew v1.1.0 h1:ZDRjVQ15GmhC3fiQ8ni8+OwkZQO4DARzQgrnXU1Liz8=
-github.com/davecgh/go-spew v1.1.0/go.mod h1:J7Y8YcW2NihsgmVo/mv3lAwl/skON4iLHjSsI+c5H38=
-github.com/mattn/go-runewidth v0.0.10 h1:CoZ3S2P7pvtP45xOtBw+/mDL2z0RKI576gSkzRRpdGg=
-github.com/mattn/go-runewidth v0.0.10/go.mod h1:RAqKPSqVFrSLVXbA8x7dzmKdmGzieGRCM46jaSJTDAk=
-github.com/pmezard/go-difflib v1.0.0 h1:4DBwDE0NGyQoBHbLQYPwSUPoCMWR5BEzIk/f1lZbAQM=
-github.com/pmezard/go-difflib v1.0.0/go.mod h1:iKH77koFhYxTK1pcRnkKkqfTogsbg7gZNVY4sRDYZ/4=
-github.com/rivo/uniseg v0.1.0 h1:+2KBaVoUmb9XzDsrx/Ct0W/EYOSFf/nWTauy++DprtY=
-github.com/rivo/uniseg v0.1.0/go.mod h1:J6wj4VEh+S6ZtnVlnTBMWIodfgj8LQOQFoIToxlJtxc=
-github.com/scylladb/termtables v0.0.0-20191203121021-c4c0b6d42ff4 h1:8qmTC5ByIXO3GP/IzBkxcZ/99VITvnIETDhdFz/om7A=
-github.com/scylladb/termtables v0.0.0-20191203121021-c4c0b6d42ff4/go.mod h1:C1a7PQSMz9NShzorzCiG2fk9+xuCgLkPeCvMHYR2OWg=
-github.com/stretchr/objx v0.1.0 h1:4G4v2dO3VZwixGIRoQ5Lfboy6nUhCyYzaqnIAPPhYs4=
-github.com/stretchr/objx v0.1.0/go.mod h1:HFkY916IF+rwdDfMAkV7OtwuqBVzrE8GR6GFx+wExME=
-github.com/stretchr/testify v1.7.0 h1:nwc3DEeHmmLAfoZucVR881uASk0Mfjw8xYJ99tb5CcY=
-github.com/stretchr/testify v1.7.0/go.mod h1:6Fq8oRcR53rry900zMqJjRRixrwX3KX962/h/Wwjteg=
-gopkg.in/check.v1 v0.0.0-20161208181325-20d25e280405 h1:yhCVgyC4o1eVCa2tZl7eS0r+SDo693bJlVdllGtEeKM=
-gopkg.in/check.v1 v0.0.0-20161208181325-20d25e280405/go.mod h1:Co6ibVJAznAaIkqp8huTwlJQCZ016jof/cbN4VW5Yz0=
-gopkg.in/yaml.v3 v3.0.0-20200313102051-9f266ea9e77c h1:dUUwHk2QECo/6vqA44rthZ8ie2QXMNeKRTHCNY2nXvo=
-gopkg.in/yaml.v3 v3.0.0-20200313102051-9f266ea9e77c/go.mod h1:K4uyk7z7BCEPqu6E+C64Yfv1cQ7kz7rIZviUmN+EgEM=
diff --git a/vendor/github.com/araddon/dateparse/parseany.go b/vendor/github.com/araddon/dateparse/parseany.go
deleted file mode 100644
index b9668b2..0000000
--- a/vendor/github.com/araddon/dateparse/parseany.go
+++ /dev/null
@@ -1,2189 +0,0 @@
-// Package dateparse parses date-strings without knowing the format
-// in advance, using a fast lex based approach to eliminate shotgun
-// attempts. It leans towards US style dates when there is a conflict.
-package dateparse
-
-import (
- "fmt"
- "strconv"
- "strings"
- "time"
- "unicode"
- "unicode/utf8"
-)
-
-// func init() {
-// gou.SetupLogging("debug")
-// gou.SetColorOutput()
-// }
-
-var days = []string{
- "mon",
- "tue",
- "wed",
- "thu",
- "fri",
- "sat",
- "sun",
- "monday",
- "tuesday",
- "wednesday",
- "thursday",
- "friday",
- "saturday",
- "sunday",
-}
-
-var months = []string{
- "january",
- "february",
- "march",
- "april",
- "may",
- "june",
- "july",
- "august",
- "september",
- "october",
- "november",
- "december",
-}
-
-type dateState uint8
-type timeState uint8
-
-const (
- dateStart dateState = iota // 0
- dateDigit
- dateDigitSt
- dateYearDash
- dateYearDashAlphaDash
- dateYearDashDash
- dateYearDashDashWs // 5
- dateYearDashDashT
- dateYearDashDashOffset
- dateDigitDash
- dateDigitDashAlpha
- dateDigitDashAlphaDash // 10
- dateDigitDot
- dateDigitDotDot
- dateDigitSlash
- dateDigitYearSlash
- dateDigitSlashAlpha // 15
- dateDigitColon
- dateDigitChineseYear
- dateDigitChineseYearWs
- dateDigitWs
- dateDigitWsMoYear // 20
- dateDigitWsMolong
- dateAlpha
- dateAlphaWs
- dateAlphaWsDigit
- dateAlphaWsDigitMore // 25
- dateAlphaWsDigitMoreWs
- dateAlphaWsDigitMoreWsYear
- dateAlphaWsMonth
- dateAlphaWsDigitYearmaybe
- dateAlphaWsMonthMore
- dateAlphaWsMonthSuffix
- dateAlphaWsMore
- dateAlphaWsAtTime
- dateAlphaWsAlpha
- dateAlphaWsAlphaYearmaybe // 35
- dateAlphaPeriodWsDigit
- dateWeekdayComma
- dateWeekdayAbbrevComma
-)
-const (
- // Time state
- timeIgnore timeState = iota // 0
- timeStart
- timeWs
- timeWsAlpha
- timeWsAlphaWs
- timeWsAlphaZoneOffset // 5
- timeWsAlphaZoneOffsetWs
- timeWsAlphaZoneOffsetWsYear
- timeWsAlphaZoneOffsetWsExtra
- timeWsAMPMMaybe
- timeWsAMPM // 10
- timeWsOffset
- timeWsOffsetWs // 12
- timeWsOffsetColonAlpha
- timeWsOffsetColon
- timeWsYear // 15
- timeOffset
- timeOffsetColon
- timeAlpha
- timePeriod
- timePeriodOffset // 20
- timePeriodOffsetColon
- timePeriodOffsetColonWs
- timePeriodWs
- timePeriodWsAlpha
- timePeriodWsOffset // 25
- timePeriodWsOffsetWs
- timePeriodWsOffsetWsAlpha
- timePeriodWsOffsetColon
- timePeriodWsOffsetColonAlpha
- timeZ
- timeZDigit
-)
-
-var (
- // ErrAmbiguousMMDD for date formats such as 04/02/2014 the mm/dd vs dd/mm are
- // ambiguous, so it is an error for strict parse rules.
- ErrAmbiguousMMDD = fmt.Errorf("This date has ambiguous mm/dd vs dd/mm type format")
-)
-
-func unknownErr(datestr string) error {
- return fmt.Errorf("Could not find format for %q", datestr)
-}
-
-// ParseAny parse an unknown date format, detect the layout.
-// Normal parse. Equivalent Timezone rules as time.Parse().
-// NOTE: please see readme on mmdd vs ddmm ambiguous dates.
-func ParseAny(datestr string, opts ...ParserOption) (time.Time, error) {
- p, err := parseTime(datestr, nil, opts...)
- if err != nil {
- return time.Time{}, err
- }
- return p.parse()
-}
-
-// ParseIn with Location, equivalent to time.ParseInLocation() timezone/offset
-// rules. Using location arg, if timezone/offset info exists in the
-// datestring, it uses the given location rules for any zone interpretation.
-// That is, MST means one thing when using America/Denver and something else
-// in other locations.
-func ParseIn(datestr string, loc *time.Location, opts ...ParserOption) (time.Time, error) {
- p, err := parseTime(datestr, loc, opts...)
- if err != nil {
- return time.Time{}, err
- }
- return p.parse()
-}
-
-// ParseLocal Given an unknown date format, detect the layout,
-// using time.Local, parse.
-//
-// Set Location to time.Local. Same as ParseIn Location but lazily uses
-// the global time.Local variable for Location argument.
-//
-// denverLoc, _ := time.LoadLocation("America/Denver")
-// time.Local = denverLoc
-//
-// t, err := dateparse.ParseLocal("3/1/2014")
-//
-// Equivalent to:
-//
-// t, err := dateparse.ParseIn("3/1/2014", denverLoc)
-//
-func ParseLocal(datestr string, opts ...ParserOption) (time.Time, error) {
- p, err := parseTime(datestr, time.Local, opts...)
- if err != nil {
- return time.Time{}, err
- }
- return p.parse()
-}
-
-// MustParse parse a date, and panic if it can't be parsed. Used for testing.
-// Not recommended for most use-cases.
-func MustParse(datestr string, opts ...ParserOption) time.Time {
- p, err := parseTime(datestr, nil, opts...)
- if err != nil {
- panic(err.Error())
- }
- t, err := p.parse()
- if err != nil {
- panic(err.Error())
- }
- return t
-}
-
-// ParseFormat parse's an unknown date-time string and returns a layout
-// string that can parse this (and exact same format) other date-time strings.
-//
-// layout, err := dateparse.ParseFormat("2013-02-01 00:00:00")
-// // layout = "2006-01-02 15:04:05"
-//
-func ParseFormat(datestr string, opts ...ParserOption) (string, error) {
- p, err := parseTime(datestr, nil, opts...)
- if err != nil {
- return "", err
- }
- _, err = p.parse()
- if err != nil {
- return "", err
- }
- return string(p.format), nil
-}
-
-// ParseStrict parse an unknown date format. IF the date is ambigous
-// mm/dd vs dd/mm then return an error. These return errors: 3.3.2014 , 8/8/71 etc
-func ParseStrict(datestr string, opts ...ParserOption) (time.Time, error) {
- p, err := parseTime(datestr, nil, opts...)
- if err != nil {
- return time.Time{}, err
- }
- if p.ambiguousMD {
- return time.Time{}, ErrAmbiguousMMDD
- }
- return p.parse()
-}
-
-func parseTime(datestr string, loc *time.Location, opts ...ParserOption) (p *parser, err error) {
-
- p = newParser(datestr, loc, opts...)
- if p.retryAmbiguousDateWithSwap {
- // month out of range signifies that a day/month swap is the correct solution to an ambiguous date
- // this is because it means that a day is being interpreted as a month and overflowing the valid value for that
- // by retrying in this case, we can fix a common situation with no assumptions
- defer func() {
- if p != nil && p.ambiguousMD {
- // if it errors out with the following error, swap before we
- // get out of this function to reduce scope it needs to be applied on
- _, err := p.parse()
- if err != nil && strings.Contains(err.Error(), "month out of range") {
- // create the option to reverse the preference
- preferMonthFirst := PreferMonthFirst(!p.preferMonthFirst)
- // turn off the retry to avoid endless recursion
- retryAmbiguousDateWithSwap := RetryAmbiguousDateWithSwap(false)
- modifiedOpts := append(opts, preferMonthFirst, retryAmbiguousDateWithSwap)
- p, err = parseTime(datestr, time.Local, modifiedOpts...)
- }
- }
-
- }()
- }
-
- i := 0
-
- // General strategy is to read rune by rune through the date looking for
- // certain hints of what type of date we are dealing with.
- // Hopefully we only need to read about 5 or 6 bytes before
- // we figure it out and then attempt a parse
-iterRunes:
- for ; i < len(datestr); i++ {
- //r := rune(datestr[i])
- r, bytesConsumed := utf8.DecodeRuneInString(datestr[i:])
- if bytesConsumed > 1 {
- i += (bytesConsumed - 1)
- }
-
- // gou.Debugf("i=%d r=%s state=%d %s", i, string(r), p.stateDate, datestr)
- switch p.stateDate {
- case dateStart:
- if unicode.IsDigit(r) {
- p.stateDate = dateDigit
- } else if unicode.IsLetter(r) {
- p.stateDate = dateAlpha
- } else {
- return nil, unknownErr(datestr)
- }
- case dateDigit:
-
- switch r {
- case '-', '\u2212':
- // 2006-01-02
- // 2013-Feb-03
- // 13-Feb-03
- // 29-Jun-2016
- if i == 4 {
- p.stateDate = dateYearDash
- p.yeari = 0
- p.yearlen = i
- p.moi = i + 1
- p.set(0, "2006")
- } else {
- p.stateDate = dateDigitDash
- }
- case '/':
- // 08/May/2005
- // 03/31/2005
- // 2014/02/24
- p.stateDate = dateDigitSlash
- if i == 4 {
- // 2014/02/24 - Year first /
- p.yearlen = i // since it was start of datestr, i=len
- p.moi = i + 1
- p.setYear()
- p.stateDate = dateDigitYearSlash
- } else {
- // Either Ambiguous dd/mm vs mm/dd OR dd/month/yy
- // 08/May/2005
- // 03/31/2005
- // 31/03/2005
- if i+2 < len(p.datestr) && unicode.IsLetter(rune(datestr[i+1])) {
- // 08/May/2005
- p.stateDate = dateDigitSlashAlpha
- p.moi = i + 1
- p.daylen = 2
- p.dayi = 0
- p.setDay()
- continue
- }
- // Ambiguous dd/mm vs mm/dd the bane of date-parsing
- // 03/31/2005
- // 31/03/2005
- p.ambiguousMD = true
- if p.preferMonthFirst {
- if p.molen == 0 {
- // 03/31/2005
- p.molen = i
- p.setMonth()
- p.dayi = i + 1
- }
- } else {
- if p.daylen == 0 {
- p.daylen = i
- p.setDay()
- p.moi = i + 1
- }
- }
-
- }
-
- case ':':
- // 03/31/2005
- // 2014/02/24
- p.stateDate = dateDigitColon
- if i == 4 {
- p.yearlen = i
- p.moi = i + 1
- p.setYear()
- } else {
- p.ambiguousMD = true
- if p.preferMonthFirst {
- if p.molen == 0 {
- p.molen = i
- p.setMonth()
- p.dayi = i + 1
- }
- }
- }
-
- case '.':
- // 3.31.2014
- // 08.21.71
- // 2014.05
- p.stateDate = dateDigitDot
- if i == 4 {
- p.yearlen = i
- p.moi = i + 1
- p.setYear()
- } else {
- p.ambiguousMD = true
- p.moi = 0
- p.molen = i
- p.setMonth()
- p.dayi = i + 1
- }
-
- case ' ':
- // 18 January 2018
- // 8 January 2018
- // 8 jan 2018
- // 02 Jan 2018 23:59
- // 02 Jan 2018 23:59:34
- // 12 Feb 2006, 19:17
- // 12 Feb 2006, 19:17:22
- if i == 6 {
- p.stateDate = dateDigitSt
- } else {
- p.stateDate = dateDigitWs
- p.dayi = 0
- p.daylen = i
- }
- case '年':
- // Chinese Year
- p.stateDate = dateDigitChineseYear
- case ',':
- return nil, unknownErr(datestr)
- default:
- continue
- }
- p.part1Len = i
-
- case dateDigitSt:
- p.set(0, "060102")
- i = i - 1
- p.stateTime = timeStart
- break iterRunes
- case dateYearDash:
- // dateYearDashDashT
- // 2006-01-02T15:04:05Z07:00
- // 2020-08-17T17:00:00:000+0100
- // dateYearDashDashWs
- // 2013-04-01 22:43:22
- // dateYearDashAlphaDash
- // 2013-Feb-03
- switch r {
- case '-':
- p.molen = i - p.moi
- p.dayi = i + 1
- p.stateDate = dateYearDashDash
- p.setMonth()
- default:
- if unicode.IsLetter(r) {
- p.stateDate = dateYearDashAlphaDash
- }
- }
-
- case dateYearDashDash:
- // dateYearDashDashT
- // 2006-01-02T15:04:05Z07:00
- // dateYearDashDashWs
- // 2013-04-01 22:43:22
- // dateYearDashDashOffset
- // 2020-07-20+00:00
- switch r {
- case '+', '-':
- p.offseti = i
- p.daylen = i - p.dayi
- p.stateDate = dateYearDashDashOffset
- p.setDay()
- case ' ':
- p.daylen = i - p.dayi
- p.stateDate = dateYearDashDashWs
- p.stateTime = timeStart
- p.setDay()
- break iterRunes
- case 'T':
- p.daylen = i - p.dayi
- p.stateDate = dateYearDashDashT
- p.stateTime = timeStart
- p.setDay()
- break iterRunes
- }
-
- case dateYearDashDashT:
- // dateYearDashDashT
- // 2006-01-02T15:04:05Z07:00
- // 2020-08-17T17:00:00:000+0100
-
- case dateYearDashDashOffset:
- // 2020-07-20+00:00
- switch r {
- case ':':
- p.set(p.offseti, "-07:00")
- // case ' ':
- // return nil, unknownErr(datestr)
- }
-
- case dateYearDashAlphaDash:
- // 2013-Feb-03
- switch r {
- case '-':
- p.molen = i - p.moi
- p.set(p.moi, "Jan")
- p.dayi = i + 1
- }
- case dateDigitDash:
- // 13-Feb-03
- // 29-Jun-2016
- if unicode.IsLetter(r) {
- p.stateDate = dateDigitDashAlpha
- p.moi = i
- } else {
- return nil, unknownErr(datestr)
- }
- case dateDigitDashAlpha:
- // 13-Feb-03
- // 28-Feb-03
- // 29-Jun-2016
- switch r {
- case '-':
- p.molen = i - p.moi
- p.set(p.moi, "Jan")
- p.yeari = i + 1
- p.stateDate = dateDigitDashAlphaDash
- }
-
- case dateDigitDashAlphaDash:
- // 13-Feb-03 ambiguous
- // 28-Feb-03 ambiguous
- // 29-Jun-2016 dd-month(alpha)-yyyy
- switch r {
- case ' ':
- // we need to find if this was 4 digits, aka year
- // or 2 digits which makes it ambiguous year/day
- length := i - (p.moi + p.molen + 1)
- if length == 4 {
- p.yearlen = 4
- p.set(p.yeari, "2006")
- // We now also know that part1 was the day
- p.dayi = 0
- p.daylen = p.part1Len
- p.setDay()
- } else if length == 2 {
- // We have no idea if this is
- // yy-mon-dd OR dd-mon-yy
- //
- // We are going to ASSUME (bad, bad) that it is dd-mon-yy which is a horible assumption
- p.ambiguousMD = true
- p.yearlen = 2
- p.set(p.yeari, "06")
- // We now also know that part1 was the day
- p.dayi = 0
- p.daylen = p.part1Len
- p.setDay()
- }
- p.stateTime = timeStart
- break iterRunes
- }
-
- case dateDigitYearSlash:
- // 2014/07/10 06:55:38.156283
- // I honestly don't know if this format ever shows up as yyyy/
-
- switch r {
- case ' ', ':':
- p.stateTime = timeStart
- if p.daylen == 0 {
- p.daylen = i - p.dayi
- p.setDay()
- }
- break iterRunes
- case '/':
- if p.molen == 0 {
- p.molen = i - p.moi
- p.setMonth()
- p.dayi = i + 1
- }
- }
-
- case dateDigitSlashAlpha:
- // 06/May/2008
-
- switch r {
- case '/':
- // |
- // 06/May/2008
- if p.molen == 0 {
- p.set(p.moi, "Jan")
- p.yeari = i + 1
- }
- // We aren't breaking because we are going to re-use this case
- // to find where the date starts, and possible time begins
- case ' ', ':':
- p.stateTime = timeStart
- if p.yearlen == 0 {
- p.yearlen = i - p.yeari
- p.setYear()
- }
- break iterRunes
- }
-
- case dateDigitSlash:
- // 03/19/2012 10:11:59
- // 04/2/2014 03:00:37
- // 3/1/2012 10:11:59
- // 4/8/2014 22:05
- // 3/1/2014
- // 10/13/2014
- // 01/02/2006
- // 1/2/06
-
- switch r {
- case '/':
- // This is the 2nd / so now we should know start pts of all of the dd, mm, yy
- if p.preferMonthFirst {
- if p.daylen == 0 {
- p.daylen = i - p.dayi
- p.setDay()
- p.yeari = i + 1
- }
- } else {
- if p.molen == 0 {
- p.molen = i - p.moi
- p.setMonth()
- p.yeari = i + 1
- }
- }
- // Note no break, we are going to pass by and re-enter this dateDigitSlash
- // and look for ending (space) or not (just date)
- case ' ':
- p.stateTime = timeStart
- if p.yearlen == 0 {
- p.yearlen = i - p.yeari
- p.setYear()
- }
- break iterRunes
- }
-
- case dateDigitColon:
- // 2014:07:10 06:55:38.156283
- // 03:19:2012 10:11:59
- // 04:2:2014 03:00:37
- // 3:1:2012 10:11:59
- // 4:8:2014 22:05
- // 3:1:2014
- // 10:13:2014
- // 01:02:2006
- // 1:2:06
-
- switch r {
- case ' ':
- p.stateTime = timeStart
- if p.yearlen == 0 {
- p.yearlen = i - p.yeari
- p.setYear()
- } else if p.daylen == 0 {
- p.daylen = i - p.dayi
- p.setDay()
- }
- break iterRunes
- case ':':
- if p.yearlen > 0 {
- // 2014:07:10 06:55:38.156283
- if p.molen == 0 {
- p.molen = i - p.moi
- p.setMonth()
- p.dayi = i + 1
- }
- } else if p.preferMonthFirst {
- if p.daylen == 0 {
- p.daylen = i - p.dayi
- p.setDay()
- p.yeari = i + 1
- }
- }
- }
-
- case dateDigitWs:
- // 18 January 2018
- // 8 January 2018
- // 8 jan 2018
- // 1 jan 18
- // 02 Jan 2018 23:59
- // 02 Jan 2018 23:59:34
- // 12 Feb 2006, 19:17
- // 12 Feb 2006, 19:17:22
- switch r {
- case ' ':
- p.yeari = i + 1
- //p.yearlen = 4
- p.dayi = 0
- p.daylen = p.part1Len
- p.setDay()
- p.stateTime = timeStart
- if i > p.daylen+len(" Sep") { // November etc
- // If len greather than space + 3 it must be full month
- p.stateDate = dateDigitWsMolong
- } else {
- // If len=3, the might be Feb or May? Ie ambigous abbreviated but
- // we can parse may with either. BUT, that means the
- // format may not be correct?
- // mo := strings.ToLower(datestr[p.daylen+1 : i])
- p.moi = p.daylen + 1
- p.molen = i - p.moi
- p.set(p.moi, "Jan")
- p.stateDate = dateDigitWsMoYear
- }
- }
-
- case dateDigitWsMoYear:
- // 8 jan 2018
- // 02 Jan 2018 23:59
- // 02 Jan 2018 23:59:34
- // 12 Feb 2006, 19:17
- // 12 Feb 2006, 19:17:22
- switch r {
- case ',':
- p.yearlen = i - p.yeari
- p.setYear()
- i++
- break iterRunes
- case ' ':
- p.yearlen = i - p.yeari
- p.setYear()
- break iterRunes
- }
- case dateDigitWsMolong:
- // 18 January 2018
- // 8 January 2018
-
- case dateDigitChineseYear:
- // dateDigitChineseYear
- // 2014年04月08日
- // weekday %Y年%m月%e日 %A %I:%M %p
- // 2013年07月18日 星期四 10:27 上午
- if r == ' ' {
- p.stateDate = dateDigitChineseYearWs
- break
- }
- case dateDigitDot:
- // This is the 2nd period
- // 3.31.2014
- // 08.21.71
- // 2014.05
- // 2018.09.30
- if r == '.' {
- if p.moi == 0 {
- // 3.31.2014
- p.daylen = i - p.dayi
- p.yeari = i + 1
- p.setDay()
- p.stateDate = dateDigitDotDot
- } else {
- // 2018.09.30
- //p.molen = 2
- p.molen = i - p.moi
- p.dayi = i + 1
- p.setMonth()
- p.stateDate = dateDigitDotDot
- }
- }
- case dateDigitDotDot:
- // iterate all the way through
- case dateAlpha:
- // dateAlphaWS
- // Mon Jan _2 15:04:05 2006
- // Mon Jan _2 15:04:05 MST 2006
- // Mon Jan 02 15:04:05 -0700 2006
- // Mon Aug 10 15:44:11 UTC+0100 2015
- // Fri Jul 03 2015 18:04:07 GMT+0100 (GMT Daylight Time)
- // dateAlphaWSDigit
- // May 8, 2009 5:57:51 PM
- // oct 1, 1970
- // dateAlphaWsMonth
- // April 8, 2009
- // dateAlphaWsMore
- // dateAlphaWsAtTime
- // January 02, 2006 at 3:04pm MST-07
- //
- // dateAlphaPeriodWsDigit
- // oct. 1, 1970
- // dateWeekdayComma
- // Monday, 02 Jan 2006 15:04:05 MST
- // Monday, 02-Jan-06 15:04:05 MST
- // Monday, 02 Jan 2006 15:04:05 -0700
- // Monday, 02 Jan 2006 15:04:05 +0100
- // dateWeekdayAbbrevComma
- // Mon, 02 Jan 2006 15:04:05 MST
- // Mon, 02 Jan 2006 15:04:05 -0700
- // Thu, 13 Jul 2017 08:58:40 +0100
- // Tue, 11 Jul 2017 16:28:13 +0200 (CEST)
- // Mon, 02-Jan-06 15:04:05 MST
- switch {
- case r == ' ':
- // X
- // April 8, 2009
- if i > 3 {
- // Check to see if the alpha is name of month? or Day?
- month := strings.ToLower(datestr[0:i])
- if isMonthFull(month) {
- p.fullMonth = month
- // len(" 31, 2018") = 9
- if len(datestr[i:]) < 10 {
- // April 8, 2009
- p.stateDate = dateAlphaWsMonth
- } else {
- p.stateDate = dateAlphaWsMore
- }
- p.dayi = i + 1
- break
- }
-
- } else {
- // This is possibly ambiguous? May will parse as either though.
- // So, it could return in-correct format.
- // dateAlphaWs
- // May 05, 2005, 05:05:05
- // May 05 2005, 05:05:05
- // Jul 05, 2005, 05:05:05
- // May 8 17:57:51 2009
- // May 8 17:57:51 2009
- // skip & return to dateStart
- // Tue 05 May 2020, 05:05:05
- // Mon Jan 2 15:04:05 2006
-
- maybeDay := strings.ToLower(datestr[0:i])
- if isDay(maybeDay) {
- // using skip throws off indices used by other code; saner to restart
- return parseTime(datestr[i+1:], loc)
- }
- p.stateDate = dateAlphaWs
- }
-
- case r == ',':
- // Mon, 02 Jan 2006
-
- if i == 3 {
- p.stateDate = dateWeekdayAbbrevComma
- p.set(0, "Mon")
- } else {
- p.stateDate = dateWeekdayComma
- p.skip = i + 2
- i++
- // TODO: lets just make this "skip" as we don't need
- // the mon, monday, they are all superfelous and not needed
- // just lay down the skip, no need to fill and then skip
- }
- case r == '.':
- // sept. 28, 2017
- // jan. 28, 2017
- p.stateDate = dateAlphaPeriodWsDigit
- if i == 3 {
- p.molen = i
- p.set(0, "Jan")
- } else if i == 4 {
- // gross
- datestr = datestr[0:i-1] + datestr[i:]
- return parseTime(datestr, loc, opts...)
- } else {
- return nil, unknownErr(datestr)
- }
- }
-
- case dateAlphaWs:
- // dateAlphaWsAlpha
- // Mon Jan _2 15:04:05 2006
- // Mon Jan _2 15:04:05 MST 2006
- // Mon Jan 02 15:04:05 -0700 2006
- // Fri Jul 03 2015 18:04:07 GMT+0100 (GMT Daylight Time)
- // Mon Aug 10 15:44:11 UTC+0100 2015
- // dateAlphaWsDigit
- // May 8, 2009 5:57:51 PM
- // May 8 2009 5:57:51 PM
- // May 8 17:57:51 2009
- // May 8 17:57:51 2009
- // May 08 17:57:51 2009
- // oct 1, 1970
- // oct 7, '70
- switch {
- case unicode.IsLetter(r):
- p.set(0, "Mon")
- p.stateDate = dateAlphaWsAlpha
- p.set(i, "Jan")
- case unicode.IsDigit(r):
- p.set(0, "Jan")
- p.stateDate = dateAlphaWsDigit
- p.dayi = i
- }
-
- case dateAlphaWsDigit:
- // May 8, 2009 5:57:51 PM
- // May 8 2009 5:57:51 PM
- // oct 1, 1970
- // oct 7, '70
- // oct. 7, 1970
- // May 8 17:57:51 2009
- // May 8 17:57:51 2009
- // May 08 17:57:51 2009
- if r == ',' {
- p.daylen = i - p.dayi
- p.setDay()
- p.stateDate = dateAlphaWsDigitMore
- } else if r == ' ' {
- p.daylen = i - p.dayi
- p.setDay()
- p.yeari = i + 1
- p.stateDate = dateAlphaWsDigitYearmaybe
- p.stateTime = timeStart
- } else if unicode.IsLetter(r) {
- p.stateDate = dateAlphaWsMonthSuffix
- i--
- }
- case dateAlphaWsDigitYearmaybe:
- // x
- // May 8 2009 5:57:51 PM
- // May 8 17:57:51 2009
- // May 8 17:57:51 2009
- // May 08 17:57:51 2009
- // Jul 03 2015 18:04:07 GMT+0100 (GMT Daylight Time)
- if r == ':' {
- // Guessed wrong; was not a year
- i = i - 3
- p.stateDate = dateAlphaWsDigit
- p.yeari = 0
- break iterRunes
- } else if r == ' ' {
- // must be year format, not 15:04
- p.yearlen = i - p.yeari
- p.setYear()
- break iterRunes
- }
- case dateAlphaWsDigitMore:
- // x
- // May 8, 2009 5:57:51 PM
- // May 05, 2005, 05:05:05
- // May 05 2005, 05:05:05
- // oct 1, 1970
- // oct 7, '70
- if r == ' ' {
- p.yeari = i + 1
- p.stateDate = dateAlphaWsDigitMoreWs
- }
- case dateAlphaWsDigitMoreWs:
- // x
- // May 8, 2009 5:57:51 PM
- // May 05, 2005, 05:05:05
- // oct 1, 1970
- // oct 7, '70
- switch r {
- case '\'':
- p.yeari = i + 1
- case ' ', ',':
- // x
- // May 8, 2009 5:57:51 PM
- // x
- // May 8, 2009, 5:57:51 PM
- p.stateDate = dateAlphaWsDigitMoreWsYear
- p.yearlen = i - p.yeari
- p.setYear()
- p.stateTime = timeStart
- break iterRunes
- }
-
- case dateAlphaWsMonth:
- // April 8, 2009
- // April 8 2009
- switch r {
- case ' ', ',':
- // x
- // June 8, 2009
- // x
- // June 8 2009
- if p.daylen == 0 {
- p.daylen = i - p.dayi
- p.setDay()
- }
- case 's', 'S', 'r', 'R', 't', 'T', 'n', 'N':
- // st, rd, nd, st
- i--
- p.stateDate = dateAlphaWsMonthSuffix
- default:
- if p.daylen > 0 && p.yeari == 0 {
- p.yeari = i
- }
- }
- case dateAlphaWsMonthMore:
- // X
- // January 02, 2006, 15:04:05
- // January 02 2006, 15:04:05
- // January 02, 2006 15:04:05
- // January 02 2006 15:04:05
- switch r {
- case ',':
- p.yearlen = i - p.yeari
- p.setYear()
- p.stateTime = timeStart
- i++
- break iterRunes
- case ' ':
- p.yearlen = i - p.yeari
- p.setYear()
- p.stateTime = timeStart
- break iterRunes
- }
- case dateAlphaWsMonthSuffix:
- // x
- // April 8th, 2009
- // April 8th 2009
- switch r {
- case 't', 'T':
- if p.nextIs(i, 'h') || p.nextIs(i, 'H') {
- if len(datestr) > i+2 {
- return parseTime(fmt.Sprintf("%s%s", p.datestr[0:i], p.datestr[i+2:]), loc, opts...)
- }
- }
- case 'n', 'N':
- if p.nextIs(i, 'd') || p.nextIs(i, 'D') {
- if len(datestr) > i+2 {
- return parseTime(fmt.Sprintf("%s%s", p.datestr[0:i], p.datestr[i+2:]), loc, opts...)
- }
- }
- case 's', 'S':
- if p.nextIs(i, 't') || p.nextIs(i, 'T') {
- if len(datestr) > i+2 {
- return parseTime(fmt.Sprintf("%s%s", p.datestr[0:i], p.datestr[i+2:]), loc, opts...)
- }
- }
- case 'r', 'R':
- if p.nextIs(i, 'd') || p.nextIs(i, 'D') {
- if len(datestr) > i+2 {
- return parseTime(fmt.Sprintf("%s%s", p.datestr[0:i], p.datestr[i+2:]), loc, opts...)
- }
- }
- }
- case dateAlphaWsMore:
- // January 02, 2006, 15:04:05
- // January 02 2006, 15:04:05
- // January 2nd, 2006, 15:04:05
- // January 2nd 2006, 15:04:05
- // September 17, 2012 at 5:00pm UTC-05
- switch {
- case r == ',':
- // x
- // January 02, 2006, 15:04:05
- if p.nextIs(i, ' ') {
- p.daylen = i - p.dayi
- p.setDay()
- p.yeari = i + 2
- p.stateDate = dateAlphaWsMonthMore
- i++
- }
-
- case r == ' ':
- // x
- // January 02 2006, 15:04:05
- p.daylen = i - p.dayi
- p.setDay()
- p.yeari = i + 1
- p.stateDate = dateAlphaWsMonthMore
- case unicode.IsDigit(r):
- // XX
- // January 02, 2006, 15:04:05
- continue
- case unicode.IsLetter(r):
- // X
- // January 2nd, 2006, 15:04:05
- p.daylen = i - p.dayi
- p.setDay()
- p.stateDate = dateAlphaWsMonthSuffix
- i--
- }
-
- case dateAlphaPeriodWsDigit:
- // oct. 7, '70
- switch {
- case r == ' ':
- // continue
- case unicode.IsDigit(r):
- p.stateDate = dateAlphaWsDigit
- p.dayi = i
- default:
- return p, unknownErr(datestr)
- }
- case dateWeekdayComma:
- // Monday, 02 Jan 2006 15:04:05 MST
- // Monday, 02 Jan 2006 15:04:05 -0700
- // Monday, 02 Jan 2006 15:04:05 +0100
- // Monday, 02-Jan-06 15:04:05 MST
- if p.dayi == 0 {
- p.dayi = i
- }
- switch r {
- case ' ', '-':
- if p.moi == 0 {
- p.moi = i + 1
- p.daylen = i - p.dayi
- p.setDay()
- } else if p.yeari == 0 {
- p.yeari = i + 1
- p.molen = i - p.moi
- p.set(p.moi, "Jan")
- } else {
- p.stateTime = timeStart
- break iterRunes
- }
- }
- case dateWeekdayAbbrevComma:
- // Mon, 02 Jan 2006 15:04:05 MST
- // Mon, 02 Jan 2006 15:04:05 -0700
- // Thu, 13 Jul 2017 08:58:40 +0100
- // Thu, 4 Jan 2018 17:53:36 +0000
- // Tue, 11 Jul 2017 16:28:13 +0200 (CEST)
- // Mon, 02-Jan-06 15:04:05 MST
- switch r {
- case ' ', '-':
- if p.dayi == 0 {
- p.dayi = i + 1
- } else if p.moi == 0 {
- p.daylen = i - p.dayi
- p.setDay()
- p.moi = i + 1
- } else if p.yeari == 0 {
- p.molen = i - p.moi
- p.set(p.moi, "Jan")
- p.yeari = i + 1
- } else {
- p.yearlen = i - p.yeari
- p.setYear()
- p.stateTime = timeStart
- break iterRunes
- }
- }
-
- default:
- break iterRunes
- }
- }
- p.coalesceDate(i)
- if p.stateTime == timeStart {
- // increment first one, since the i++ occurs at end of loop
- if i < len(p.datestr) {
- i++
- }
- // ensure we skip any whitespace prefix
- for ; i < len(datestr); i++ {
- r := rune(datestr[i])
- if r != ' ' {
- break
- }
- }
-
- iterTimeRunes:
- for ; i < len(datestr); i++ {
- r := rune(datestr[i])
-
- // gou.Debugf("i=%d r=%s state=%d iterTimeRunes %s %s", i, string(r), p.stateTime, p.ds(), p.ts())
-
- switch p.stateTime {
- case timeStart:
- // 22:43:22
- // 22:43
- // timeComma
- // 08:20:13,787
- // timeWs
- // 05:24:37 PM
- // 06:20:00 UTC
- // 06:20:00 UTC-05
- // 00:12:00 +0000 UTC
- // 22:18:00 +0000 UTC m=+0.000000001
- // 15:04:05 -0700
- // 15:04:05 -07:00
- // 15:04:05 2008
- // timeOffset
- // 03:21:51+00:00
- // 19:55:00+0100
- // timePeriod
- // 17:24:37.3186369
- // 00:07:31.945167
- // 18:31:59.257000000
- // 00:00:00.000
- // timePeriodOffset
- // 19:55:00.799+0100
- // timePeriodOffsetColon
- // 15:04:05.999-07:00
- // timePeriodWs
- // timePeriodWsOffset
- // 00:07:31.945167 +0000
- // 00:00:00.000 +0000
- // timePeriodWsOffsetAlpha
- // 00:07:31.945167 +0000 UTC
- // 22:18:00.001 +0000 UTC m=+0.000000001
- // 00:00:00.000 +0000 UTC
- // timePeriodWsAlpha
- // 06:20:00.000 UTC
- if p.houri == 0 {
- p.houri = i
- }
- switch r {
- case ',':
- // hm, lets just swap out comma for period. for some reason go
- // won't parse it.
- // 2014-05-11 08:20:13,787
- ds := []byte(p.datestr)
- ds[i] = '.'
- return parseTime(string(ds), loc, opts...)
- case '-', '+':
- // 03:21:51+00:00
- p.stateTime = timeOffset
- if p.seci == 0 {
- // 22:18+0530
- p.minlen = i - p.mini
- } else {
- if p.seclen == 0 {
- p.seclen = i - p.seci
- }
- if p.msi > 0 && p.mslen == 0 {
- p.mslen = i - p.msi
- }
- }
- p.offseti = i
- case '.':
- p.stateTime = timePeriod
- p.seclen = i - p.seci
- p.msi = i + 1
- case 'Z':
- p.stateTime = timeZ
- if p.seci == 0 {
- p.minlen = i - p.mini
- } else {
- p.seclen = i - p.seci
- }
- // (Z)ulu time
- p.loc = time.UTC
- case 'a', 'A':
- if p.nextIs(i, 't') || p.nextIs(i, 'T') {
- // x
- // September 17, 2012 at 5:00pm UTC-05
- i++ // skip t
- if p.nextIs(i, ' ') {
- // x
- // September 17, 2012 at 5:00pm UTC-05
- i++ // skip '
- p.houri = 0 // reset hour
- }
- } else {
- switch {
- case r == 'a' && p.nextIs(i, 'm'):
- p.coalesceTime(i)
- p.set(i, "am")
- case r == 'A' && p.nextIs(i, 'M'):
- p.coalesceTime(i)
- p.set(i, "PM")
- }
- }
-
- case 'p', 'P':
- // Could be AM/PM
- switch {
- case r == 'p' && p.nextIs(i, 'm'):
- p.coalesceTime(i)
- p.set(i, "pm")
- case r == 'P' && p.nextIs(i, 'M'):
- p.coalesceTime(i)
- p.set(i, "PM")
- }
- case ' ':
- p.coalesceTime(i)
- p.stateTime = timeWs
- case ':':
- if p.mini == 0 {
- p.mini = i + 1
- p.hourlen = i - p.houri
- } else if p.seci == 0 {
- p.seci = i + 1
- p.minlen = i - p.mini
- } else if p.seci > 0 {
- // 18:31:59:257 ms uses colon, wtf
- p.seclen = i - p.seci
- p.set(p.seci, "05")
- p.msi = i + 1
-
- // gross, gross, gross. manipulating the datestr is horrible.
- // https://github.com/araddon/dateparse/issues/117
- // Could not get the parsing to work using golang time.Parse() without
- // replacing that colon with period.
- p.set(i, ".")
- datestr = datestr[0:i] + "." + datestr[i+1:]
- p.datestr = datestr
- }
- }
- case timeOffset:
- // 19:55:00+0100
- // timeOffsetColon
- // 15:04:05+07:00
- // 15:04:05-07:00
- if r == ':' {
- p.stateTime = timeOffsetColon
- }
- case timeWs:
- // timeWsAlpha
- // 06:20:00 UTC
- // 06:20:00 UTC-05
- // 15:44:11 UTC+0100 2015
- // 18:04:07 GMT+0100 (GMT Daylight Time)
- // 17:57:51 MST 2009
- // timeWsAMPMMaybe
- // 05:24:37 PM
- // timeWsOffset
- // 15:04:05 -0700
- // 00:12:00 +0000 UTC
- // timeWsOffsetColon
- // 15:04:05 -07:00
- // 17:57:51 -0700 2009
- // timeWsOffsetColonAlpha
- // 00:12:00 +00:00 UTC
- // timeWsYear
- // 00:12:00 2008
- // timeZ
- // 15:04:05.99Z
- switch r {
- case 'A', 'P':
- // Could be AM/PM or could be PST or similar
- p.tzi = i
- p.stateTime = timeWsAMPMMaybe
- case '+', '-':
- p.offseti = i
- p.stateTime = timeWsOffset
- default:
- if unicode.IsLetter(r) {
- // 06:20:00 UTC
- // 06:20:00 UTC-05
- // 15:44:11 UTC+0100 2015
- // 17:57:51 MST 2009
- p.tzi = i
- p.stateTime = timeWsAlpha
- } else if unicode.IsDigit(r) {
- // 00:12:00 2008
- p.stateTime = timeWsYear
- p.yeari = i
- }
- }
- case timeWsAlpha:
- // 06:20:00 UTC
- // 06:20:00 UTC-05
- // timeWsAlphaWs
- // 17:57:51 MST 2009
- // timeWsAlphaZoneOffset
- // timeWsAlphaZoneOffsetWs
- // timeWsAlphaZoneOffsetWsExtra
- // 18:04:07 GMT+0100 (GMT Daylight Time)
- // timeWsAlphaZoneOffsetWsYear
- // 15:44:11 UTC+0100 2015
- switch r {
- case '+', '-':
- p.tzlen = i - p.tzi
- if p.tzlen == 4 {
- p.set(p.tzi, " MST")
- } else if p.tzlen == 3 {
- p.set(p.tzi, "MST")
- }
- p.stateTime = timeWsAlphaZoneOffset
- p.offseti = i
- case ' ':
- // 17:57:51 MST 2009
- // 17:57:51 MST
- p.tzlen = i - p.tzi
- if p.tzlen == 4 {
- p.set(p.tzi, " MST")
- } else if p.tzlen == 3 {
- p.set(p.tzi, "MST")
- }
- p.stateTime = timeWsAlphaWs
- p.yeari = i + 1
- }
- case timeWsAlphaWs:
- // 17:57:51 MST 2009
-
- case timeWsAlphaZoneOffset:
- // 06:20:00 UTC-05
- // timeWsAlphaZoneOffset
- // timeWsAlphaZoneOffsetWs
- // timeWsAlphaZoneOffsetWsExtra
- // 18:04:07 GMT+0100 (GMT Daylight Time)
- // timeWsAlphaZoneOffsetWsYear
- // 15:44:11 UTC+0100 2015
- switch r {
- case ' ':
- p.set(p.offseti, "-0700")
- if p.yeari == 0 {
- p.yeari = i + 1
- }
- p.stateTime = timeWsAlphaZoneOffsetWs
- }
- case timeWsAlphaZoneOffsetWs:
- // timeWsAlphaZoneOffsetWs
- // timeWsAlphaZoneOffsetWsExtra
- // 18:04:07 GMT+0100 (GMT Daylight Time)
- // timeWsAlphaZoneOffsetWsYear
- // 15:44:11 UTC+0100 2015
- if unicode.IsDigit(r) {
- p.stateTime = timeWsAlphaZoneOffsetWsYear
- } else {
- p.extra = i - 1
- p.stateTime = timeWsAlphaZoneOffsetWsExtra
- }
- case timeWsAlphaZoneOffsetWsYear:
- // 15:44:11 UTC+0100 2015
- if unicode.IsDigit(r) {
- p.yearlen = i - p.yeari + 1
- if p.yearlen == 4 {
- p.setYear()
- }
- }
- case timeWsAMPMMaybe:
- // timeWsAMPMMaybe
- // timeWsAMPM
- // 05:24:37 PM
- // timeWsAlpha
- // 00:12:00 PST
- // 15:44:11 UTC+0100 2015
- if r == 'M' {
- //return parse("2006-01-02 03:04:05 PM", datestr, loc)
- p.stateTime = timeWsAMPM
- p.set(i-1, "PM")
- if p.hourlen == 2 {
- p.set(p.houri, "03")
- } else if p.hourlen == 1 {
- p.set(p.houri, "3")
- }
- } else {
- p.stateTime = timeWsAlpha
- }
-
- case timeWsOffset:
- // timeWsOffset
- // 15:04:05 -0700
- // timeWsOffsetWsOffset
- // 17:57:51 -0700 -07
- // timeWsOffsetWs
- // 17:57:51 -0700 2009
- // 00:12:00 +0000 UTC
- // timeWsOffsetColon
- // 15:04:05 -07:00
- // timeWsOffsetColonAlpha
- // 00:12:00 +00:00 UTC
- switch r {
- case ':':
- p.stateTime = timeWsOffsetColon
- case ' ':
- p.set(p.offseti, "-0700")
- p.yeari = i + 1
- p.stateTime = timeWsOffsetWs
- }
- case timeWsOffsetWs:
- // 17:57:51 -0700 2009
- // 00:12:00 +0000 UTC
- // 22:18:00.001 +0000 UTC m=+0.000000001
- // w Extra
- // 17:57:51 -0700 -07
- switch r {
- case '=':
- // eff you golang
- if datestr[i-1] == 'm' {
- p.extra = i - 2
- p.trimExtra()
- break
- }
- case '+', '-', '(':
- // This really doesn't seem valid, but for some reason when round-tripping a go date
- // their is an extra +03 printed out. seems like go bug to me, but, parsing anyway.
- // 00:00:00 +0300 +03
- // 00:00:00 +0300 +0300
- p.extra = i - 1
- p.stateTime = timeWsOffset
- p.trimExtra()
- break
- default:
- switch {
- case unicode.IsDigit(r):
- p.yearlen = i - p.yeari + 1
- if p.yearlen == 4 {
- p.setYear()
- }
- case unicode.IsLetter(r):
- // 15:04:05 -0700 MST
- if p.tzi == 0 {
- p.tzi = i
- }
- }
- }
-
- case timeWsOffsetColon:
- // timeWsOffsetColon
- // 15:04:05 -07:00
- // timeWsOffsetColonAlpha
- // 2015-02-18 00:12:00 +00:00 UTC
- if unicode.IsLetter(r) {
- // 2015-02-18 00:12:00 +00:00 UTC
- p.stateTime = timeWsOffsetColonAlpha
- break iterTimeRunes
- }
- case timePeriod:
- // 15:04:05.999999999+07:00
- // 15:04:05.999999999-07:00
- // 15:04:05.999999+07:00
- // 15:04:05.999999-07:00
- // 15:04:05.999+07:00
- // 15:04:05.999-07:00
- // timePeriod
- // 17:24:37.3186369
- // 00:07:31.945167
- // 18:31:59.257000000
- // 00:00:00.000
- // timePeriodOffset
- // 19:55:00.799+0100
- // timePeriodOffsetColon
- // 15:04:05.999-07:00
- // timePeriodWs
- // timePeriodWsOffset
- // 00:07:31.945167 +0000
- // 00:00:00.000 +0000
- // With Extra
- // 00:00:00.000 +0300 +03
- // timePeriodWsOffsetAlpha
- // 00:07:31.945167 +0000 UTC
- // 00:00:00.000 +0000 UTC
- // 22:18:00.001 +0000 UTC m=+0.000000001
- // timePeriodWsAlpha
- // 06:20:00.000 UTC
- switch r {
- case ' ':
- p.mslen = i - p.msi
- p.stateTime = timePeriodWs
- case '+', '-':
- // This really shouldn't happen
- p.mslen = i - p.msi
- p.offseti = i
- p.stateTime = timePeriodOffset
- default:
- if unicode.IsLetter(r) {
- // 06:20:00.000 UTC
- p.mslen = i - p.msi
- p.stateTime = timePeriodWsAlpha
- }
- }
- case timePeriodOffset:
- // timePeriodOffset
- // 19:55:00.799+0100
- // timePeriodOffsetColon
- // 15:04:05.999-07:00
- // 13:31:51.999-07:00 MST
- if r == ':' {
- p.stateTime = timePeriodOffsetColon
- }
- case timePeriodOffsetColon:
- // timePeriodOffset
- // timePeriodOffsetColon
- // 15:04:05.999-07:00
- // 13:31:51.999 -07:00 MST
- switch r {
- case ' ':
- p.set(p.offseti, "-07:00")
- p.stateTime = timePeriodOffsetColonWs
- p.tzi = i + 1
- }
- case timePeriodOffsetColonWs:
- // continue
- case timePeriodWs:
- // timePeriodWs
- // timePeriodWsOffset
- // 00:07:31.945167 +0000
- // 00:00:00.000 +0000
- // timePeriodWsOffsetAlpha
- // 00:07:31.945167 +0000 UTC
- // 00:00:00.000 +0000 UTC
- // timePeriodWsOffsetColon
- // 13:31:51.999 -07:00 MST
- // timePeriodWsAlpha
- // 06:20:00.000 UTC
- if p.offseti == 0 {
- p.offseti = i
- }
- switch r {
- case '+', '-':
- p.mslen = i - p.msi - 1
- p.stateTime = timePeriodWsOffset
- default:
- if unicode.IsLetter(r) {
- // 00:07:31.945167 +0000 UTC
- // 00:00:00.000 +0000 UTC
- p.stateTime = timePeriodWsOffsetWsAlpha
- break iterTimeRunes
- }
- }
-
- case timePeriodWsOffset:
- // timePeriodWs
- // timePeriodWsOffset
- // 00:07:31.945167 +0000
- // 00:00:00.000 +0000
- // With Extra
- // 00:00:00.000 +0300 +03
- // timePeriodWsOffsetAlpha
- // 00:07:31.945167 +0000 UTC
- // 00:00:00.000 +0000 UTC
- // 03:02:00.001 +0300 MSK m=+0.000000001
- // timePeriodWsOffsetColon
- // 13:31:51.999 -07:00 MST
- // timePeriodWsAlpha
- // 06:20:00.000 UTC
- switch r {
- case ':':
- p.stateTime = timePeriodWsOffsetColon
- case ' ':
- p.set(p.offseti, "-0700")
- case '+', '-':
- // This really doesn't seem valid, but for some reason when round-tripping a go date
- // their is an extra +03 printed out. seems like go bug to me, but, parsing anyway.
- // 00:00:00.000 +0300 +03
- // 00:00:00.000 +0300 +0300
- p.extra = i - 1
- p.trimExtra()
- break
- default:
- if unicode.IsLetter(r) {
- // 00:07:31.945167 +0000 UTC
- // 00:00:00.000 +0000 UTC
- // 03:02:00.001 +0300 MSK m=+0.000000001
- p.stateTime = timePeriodWsOffsetWsAlpha
- }
- }
- case timePeriodWsOffsetWsAlpha:
- // 03:02:00.001 +0300 MSK m=+0.000000001
- // eff you golang
- if r == '=' && datestr[i-1] == 'm' {
- p.extra = i - 2
- p.trimExtra()
- break
- }
-
- case timePeriodWsOffsetColon:
- // 13:31:51.999 -07:00 MST
- switch r {
- case ' ':
- p.set(p.offseti, "-07:00")
- default:
- if unicode.IsLetter(r) {
- // 13:31:51.999 -07:00 MST
- p.tzi = i
- p.stateTime = timePeriodWsOffsetColonAlpha
- }
- }
- case timePeriodWsOffsetColonAlpha:
- // continue
- case timeZ:
- // timeZ
- // 15:04:05.99Z
- // With a time-zone at end after Z
- // 2006-01-02T15:04:05.999999999Z07:00
- // 2006-01-02T15:04:05Z07:00
- // RFC3339 = "2006-01-02T15:04:05Z07:00"
- // RFC3339Nano = "2006-01-02T15:04:05.999999999Z07:00"
- if unicode.IsDigit(r) {
- p.stateTime = timeZDigit
- }
-
- }
- }
-
- switch p.stateTime {
- case timeWsAlpha:
- switch len(p.datestr) - p.tzi {
- case 3:
- // 13:31:51.999 +01:00 CET
- p.set(p.tzi, "MST")
- case 4:
- p.set(p.tzi, "MST")
- p.extra = len(p.datestr) - 1
- p.trimExtra()
- }
-
- case timeWsAlphaWs:
- p.yearlen = i - p.yeari
- p.setYear()
- case timeWsYear:
- p.yearlen = i - p.yeari
- p.setYear()
- case timeWsAlphaZoneOffsetWsExtra:
- p.trimExtra()
- case timeWsAlphaZoneOffset:
- // 06:20:00 UTC-05
- if i-p.offseti < 4 {
- p.set(p.offseti, "-07")
- } else {
- p.set(p.offseti, "-0700")
- }
-
- case timePeriod:
- p.mslen = i - p.msi
- case timeOffset:
-
- switch len(p.datestr) - p.offseti {
- case 0, 1, 2, 4:
- return p, fmt.Errorf("TZ offset not recognized %q near %q (must be 2 or 4 digits optional colon)", datestr, string(datestr[p.offseti:]))
- case 3:
- // 19:55:00+01
- p.set(p.offseti, "-07")
- case 5:
- // 19:55:00+0100
- p.set(p.offseti, "-0700")
- }
-
- case timeWsOffset:
- p.set(p.offseti, "-0700")
- case timeWsOffsetWs:
- // 17:57:51 -0700 2009
- // 00:12:00 +0000 UTC
- if p.tzi > 0 {
- switch len(p.datestr) - p.tzi {
- case 3:
- // 13:31:51.999 +01:00 CET
- p.set(p.tzi, "MST")
- case 4:
- // 13:31:51.999 +01:00 CEST
- p.set(p.tzi, "MST ")
- }
-
- }
- case timeWsOffsetColon:
- // 17:57:51 -07:00
- p.set(p.offseti, "-07:00")
- case timeOffsetColon:
- // 15:04:05+07:00
- p.set(p.offseti, "-07:00")
- case timePeriodOffset:
- // 19:55:00.799+0100
- p.set(p.offseti, "-0700")
- case timePeriodOffsetColon:
- p.set(p.offseti, "-07:00")
- case timePeriodWsOffsetColonAlpha:
- p.tzlen = i - p.tzi
- switch p.tzlen {
- case 3:
- p.set(p.tzi, "MST")
- case 4:
- p.set(p.tzi, "MST ")
- }
- case timePeriodWsOffset:
- p.set(p.offseti, "-0700")
- }
- p.coalesceTime(i)
- }
-
- switch p.stateDate {
- case dateDigit:
- // unixy timestamps ish
- // example ct type
- // 1499979655583057426 19 nanoseconds
- // 1499979795437000 16 micro-seconds
- // 20180722105203 14 yyyyMMddhhmmss
- // 1499979795437 13 milliseconds
- // 1332151919 10 seconds
- // 20140601 8 yyyymmdd
- // 2014 4 yyyy
- t := time.Time{}
- if len(datestr) == len("1499979655583057426") { // 19
- // nano-seconds
- if nanoSecs, err := strconv.ParseInt(datestr, 10, 64); err == nil {
- t = time.Unix(0, nanoSecs)
- }
- } else if len(datestr) == len("1499979795437000") { // 16
- // micro-seconds
- if microSecs, err := strconv.ParseInt(datestr, 10, 64); err == nil {
- t = time.Unix(0, microSecs*1000)
- }
- } else if len(datestr) == len("yyyyMMddhhmmss") { // 14
- // yyyyMMddhhmmss
- p.format = []byte("20060102150405")
- return p, nil
- } else if len(datestr) == len("1332151919000") { // 13
- if miliSecs, err := strconv.ParseInt(datestr, 10, 64); err == nil {
- t = time.Unix(0, miliSecs*1000*1000)
- }
- } else if len(datestr) == len("1332151919") { //10
- if secs, err := strconv.ParseInt(datestr, 10, 64); err == nil {
- t = time.Unix(secs, 0)
- }
- } else if len(datestr) == len("20140601") {
- p.format = []byte("20060102")
- return p, nil
- } else if len(datestr) == len("2014") {
- p.format = []byte("2006")
- return p, nil
- } else if len(datestr) < 4 {
- return nil, fmt.Errorf("unrecognized format, too short %v", datestr)
- }
- if !t.IsZero() {
- if loc == nil {
- p.t = &t
- return p, nil
- }
- t = t.In(loc)
- p.t = &t
- return p, nil
- }
- case dateDigitSt:
- // 171113 14:14:20
- return p, nil
-
- case dateYearDash:
- // 2006-01
- return p, nil
-
- case dateYearDashDash:
- // 2006-01-02
- // 2006-1-02
- // 2006-1-2
- // 2006-01-2
- return p, nil
-
- case dateYearDashDashOffset:
- /// 2020-07-20+00:00
- switch len(p.datestr) - p.offseti {
- case 5:
- p.set(p.offseti, "-0700")
- case 6:
- p.set(p.offseti, "-07:00")
- }
- return p, nil
-
- case dateYearDashAlphaDash:
- // 2013-Feb-03
- // 2013-Feb-3
- p.daylen = i - p.dayi
- p.setDay()
- return p, nil
-
- case dateYearDashDashWs:
- // 2013-04-01
- return p, nil
-
- case dateYearDashDashT:
- return p, nil
-
- case dateDigitDashAlphaDash:
- // 13-Feb-03 ambiguous
- // 28-Feb-03 ambiguous
- // 29-Jun-2016
- length := len(datestr) - (p.moi + p.molen + 1)
- if length == 4 {
- p.yearlen = 4
- p.set(p.yeari, "2006")
- // We now also know that part1 was the day
- p.dayi = 0
- p.daylen = p.part1Len
- p.setDay()
- } else if length == 2 {
- // We have no idea if this is
- // yy-mon-dd OR dd-mon-yy
- //
- // We are going to ASSUME (bad, bad) that it is dd-mon-yy which is a horible assumption
- p.ambiguousMD = true
- p.yearlen = 2
- p.set(p.yeari, "06")
- // We now also know that part1 was the day
- p.dayi = 0
- p.daylen = p.part1Len
- p.setDay()
- }
-
- return p, nil
-
- case dateDigitDot:
- // 2014.05
- p.molen = i - p.moi
- p.setMonth()
- return p, nil
-
- case dateDigitDotDot:
- // 03.31.1981
- // 3.31.2014
- // 3.2.1981
- // 3.2.81
- // 08.21.71
- // 2018.09.30
- return p, nil
-
- case dateDigitWsMoYear:
- // 2 Jan 2018
- // 2 Jan 18
- // 2 Jan 2018 23:59
- // 02 Jan 2018 23:59
- // 12 Feb 2006, 19:17
- return p, nil
-
- case dateDigitWsMolong:
- // 18 January 2018
- // 8 January 2018
- if p.daylen == 2 {
- p.format = []byte("02 January 2006")
- return p, nil
- }
- p.format = []byte("2 January 2006")
- return p, nil // parse("2 January 2006", datestr, loc)
-
- case dateAlphaWsMonth:
- p.yearlen = i - p.yeari
- p.setYear()
- return p, nil
-
- case dateAlphaWsMonthMore:
- return p, nil
-
- case dateAlphaWsDigitMoreWs:
- // oct 1, 1970
- p.yearlen = i - p.yeari
- p.setYear()
- return p, nil
-
- case dateAlphaWsDigitMoreWsYear:
- // May 8, 2009 5:57:51 PM
- // Jun 7, 2005, 05:57:51
- return p, nil
-
- case dateAlphaWsAlpha:
- return p, nil
-
- case dateAlphaWsDigit:
- return p, nil
-
- case dateAlphaWsDigitYearmaybe:
- return p, nil
-
- case dateDigitSlash:
- // 3/1/2014
- // 10/13/2014
- // 01/02/2006
- return p, nil
-
- case dateDigitSlashAlpha:
- // 03/Jun/2014
- return p, nil
-
- case dateDigitYearSlash:
- // 2014/10/13
- return p, nil
-
- case dateDigitColon:
- // 3:1:2014
- // 10:13:2014
- // 01:02:2006
- // 2014:10:13
- return p, nil
-
- case dateDigitChineseYear:
- // dateDigitChineseYear
- // 2014年04月08日
- p.format = []byte("2006年01月02日")
- return p, nil
-
- case dateDigitChineseYearWs:
- p.format = []byte("2006年01月02日 15:04:05")
- return p, nil
-
- case dateWeekdayComma:
- // Monday, 02 Jan 2006 15:04:05 -0700
- // Monday, 02 Jan 2006 15:04:05 +0100
- // Monday, 02-Jan-06 15:04:05 MST
- return p, nil
-
- case dateWeekdayAbbrevComma:
- // Mon, 02-Jan-06 15:04:05 MST
- // Mon, 02 Jan 2006 15:04:05 MST
- return p, nil
-
- }
-
- return nil, unknownErr(datestr)
-}
-
-type parser struct {
- loc *time.Location
- preferMonthFirst bool
- retryAmbiguousDateWithSwap bool
- ambiguousMD bool
- stateDate dateState
- stateTime timeState
- format []byte
- datestr string
- fullMonth string
- skip int
- extra int
- part1Len int
- yeari int
- yearlen int
- moi int
- molen int
- dayi int
- daylen int
- houri int
- hourlen int
- mini int
- minlen int
- seci int
- seclen int
- msi int
- mslen int
- offseti int
- offsetlen int
- tzi int
- tzlen int
- t *time.Time
-}
-
-// ParserOption defines a function signature implemented by options
-// Options defined like this accept the parser and operate on the data within
-type ParserOption func(*parser) error
-
-// PreferMonthFirst is an option that allows preferMonthFirst to be changed from its default
-func PreferMonthFirst(preferMonthFirst bool) ParserOption {
- return func(p *parser) error {
- p.preferMonthFirst = preferMonthFirst
- return nil
- }
-}
-
-// RetryAmbiguousDateWithSwap is an option that allows retryAmbiguousDateWithSwap to be changed from its default
-func RetryAmbiguousDateWithSwap(retryAmbiguousDateWithSwap bool) ParserOption {
- return func(p *parser) error {
- p.retryAmbiguousDateWithSwap = retryAmbiguousDateWithSwap
- return nil
- }
-}
-
-func newParser(dateStr string, loc *time.Location, opts ...ParserOption) *parser {
- p := &parser{
- stateDate: dateStart,
- stateTime: timeIgnore,
- datestr: dateStr,
- loc: loc,
- preferMonthFirst: true,
- retryAmbiguousDateWithSwap: false,
- }
- p.format = []byte(dateStr)
-
- // allow the options to mutate the parser fields from their defaults
- for _, option := range opts {
- option(p)
- }
- return p
-}
-
-func (p *parser) nextIs(i int, b byte) bool {
- if len(p.datestr) > i+1 && p.datestr[i+1] == b {
- return true
- }
- return false
-}
-
-func (p *parser) set(start int, val string) {
- if start < 0 {
- return
- }
- if len(p.format) < start+len(val) {
- return
- }
- for i, r := range val {
- p.format[start+i] = byte(r)
- }
-}
-func (p *parser) setMonth() {
- if p.molen == 2 {
- p.set(p.moi, "01")
- } else if p.molen == 1 {
- p.set(p.moi, "1")
- }
-}
-
-func (p *parser) setDay() {
- if p.daylen == 2 {
- p.set(p.dayi, "02")
- } else if p.daylen == 1 {
- p.set(p.dayi, "2")
- }
-}
-func (p *parser) setYear() {
- if p.yearlen == 2 {
- p.set(p.yeari, "06")
- } else if p.yearlen == 4 {
- p.set(p.yeari, "2006")
- }
-}
-func (p *parser) coalesceDate(end int) {
- if p.yeari > 0 {
- if p.yearlen == 0 {
- p.yearlen = end - p.yeari
- }
- p.setYear()
- }
- if p.moi > 0 && p.molen == 0 {
- p.molen = end - p.moi
- p.setMonth()
- }
- if p.dayi > 0 && p.daylen == 0 {
- p.daylen = end - p.dayi
- p.setDay()
- }
-}
-func (p *parser) ts() string {
- return fmt.Sprintf("h:(%d:%d) m:(%d:%d) s:(%d:%d)", p.houri, p.hourlen, p.mini, p.minlen, p.seci, p.seclen)
-}
-func (p *parser) ds() string {
- return fmt.Sprintf("%s d:(%d:%d) m:(%d:%d) y:(%d:%d)", p.datestr, p.dayi, p.daylen, p.moi, p.molen, p.yeari, p.yearlen)
-}
-func (p *parser) coalesceTime(end int) {
- // 03:04:05
- // 15:04:05
- // 3:04:05
- // 3:4:5
- // 15:04:05.00
- if p.houri > 0 {
- if p.hourlen == 2 {
- p.set(p.houri, "15")
- } else if p.hourlen == 1 {
- p.set(p.houri, "3")
- }
- }
- if p.mini > 0 {
- if p.minlen == 0 {
- p.minlen = end - p.mini
- }
- if p.minlen == 2 {
- p.set(p.mini, "04")
- } else {
- p.set(p.mini, "4")
- }
- }
- if p.seci > 0 {
- if p.seclen == 0 {
- p.seclen = end - p.seci
- }
- if p.seclen == 2 {
- p.set(p.seci, "05")
- } else {
- p.set(p.seci, "5")
- }
- }
-
- if p.msi > 0 {
- for i := 0; i < p.mslen; i++ {
- p.format[p.msi+i] = '0'
- }
- }
-}
-func (p *parser) setFullMonth(month string) {
- if p.moi == 0 {
- p.format = []byte(fmt.Sprintf("%s%s", "January", p.format[len(month):]))
- }
-}
-
-func (p *parser) trimExtra() {
- if p.extra > 0 && len(p.format) > p.extra {
- p.format = p.format[0:p.extra]
- p.datestr = p.datestr[0:p.extra]
- }
-}
-
-// func (p *parser) remove(i, length int) {
-// if len(p.format) > i+length {
-// //append(a[:i], a[j:]...)
-// p.format = append(p.format[0:i], p.format[i+length:]...)
-// }
-// if len(p.datestr) > i+length {
-// //append(a[:i], a[j:]...)
-// p.datestr = fmt.Sprintf("%s%s", p.datestr[0:i], p.datestr[i+length:])
-// }
-// }
-
-func (p *parser) parse() (time.Time, error) {
- if p.t != nil {
- return *p.t, nil
- }
- if len(p.fullMonth) > 0 {
- p.setFullMonth(p.fullMonth)
- }
- if p.skip > 0 && len(p.format) > p.skip {
- p.format = p.format[p.skip:]
- p.datestr = p.datestr[p.skip:]
- }
-
- if p.loc == nil {
- // gou.Debugf("parse layout=%q input=%q \ntx, err := time.Parse(%q, %q)", string(p.format), p.datestr, string(p.format), p.datestr)
- return time.Parse(string(p.format), p.datestr)
- }
- //gou.Debugf("parse layout=%q input=%q \ntx, err := time.ParseInLocation(%q, %q, %v)", string(p.format), p.datestr, string(p.format), p.datestr, p.loc)
- return time.ParseInLocation(string(p.format), p.datestr, p.loc)
-}
-func isDay(alpha string) bool {
- for _, day := range days {
- if alpha == day {
- return true
- }
- }
- return false
-}
-func isMonthFull(alpha string) bool {
- for _, month := range months {
- if alpha == month {
- return true
- }
- }
- return false
-}
diff --git a/vendor/github.com/aymerick/douceur/LICENSE b/vendor/github.com/aymerick/douceur/LICENSE
deleted file mode 100644
index 6ce87cd..0000000
--- a/vendor/github.com/aymerick/douceur/LICENSE
+++ /dev/null
@@ -1,22 +0,0 @@
-The MIT License (MIT)
-
-Copyright (c) 2015 Aymerick JEHANNE
-
-Permission is hereby granted, free of charge, to any person obtaining a copy
-of this software and associated documentation files (the "Software"), to deal
-in the Software without restriction, including without limitation the rights
-to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
-copies of the Software, and to permit persons to whom the Software is
-furnished to do so, subject to the following conditions:
-
-The above copyright notice and this permission notice shall be included in all
-copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
-IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
-FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
-AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
-LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
-OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
-SOFTWARE.
-
diff --git a/vendor/github.com/aymerick/douceur/css/declaration.go b/vendor/github.com/aymerick/douceur/css/declaration.go
deleted file mode 100644
index 61d29d3..0000000
--- a/vendor/github.com/aymerick/douceur/css/declaration.go
+++ /dev/null
@@ -1,60 +0,0 @@
-package css
-
-import "fmt"
-
-// Declaration represents a parsed style property
-type Declaration struct {
- Property string
- Value string
- Important bool
-}
-
-// NewDeclaration instanciates a new Declaration
-func NewDeclaration() *Declaration {
- return &Declaration{}
-}
-
-// Returns string representation of the Declaration
-func (decl *Declaration) String() string {
- return decl.StringWithImportant(true)
-}
-
-// StringWithImportant returns string representation with optional !important part
-func (decl *Declaration) StringWithImportant(option bool) string {
- result := fmt.Sprintf("%s: %s", decl.Property, decl.Value)
-
- if option && decl.Important {
- result += " !important"
- }
-
- result += ";"
-
- return result
-}
-
-// Equal returns true if both Declarations are equals
-func (decl *Declaration) Equal(other *Declaration) bool {
- return (decl.Property == other.Property) && (decl.Value == other.Value) && (decl.Important == other.Important)
-}
-
-//
-// DeclarationsByProperty
-//
-
-// DeclarationsByProperty represents sortable style declarations
-type DeclarationsByProperty []*Declaration
-
-// Implements sort.Interface
-func (declarations DeclarationsByProperty) Len() int {
- return len(declarations)
-}
-
-// Implements sort.Interface
-func (declarations DeclarationsByProperty) Swap(i, j int) {
- declarations[i], declarations[j] = declarations[j], declarations[i]
-}
-
-// Implements sort.Interface
-func (declarations DeclarationsByProperty) Less(i, j int) bool {
- return declarations[i].Property < declarations[j].Property
-}
diff --git a/vendor/github.com/aymerick/douceur/css/rule.go b/vendor/github.com/aymerick/douceur/css/rule.go
deleted file mode 100644
index b5a44b5..0000000
--- a/vendor/github.com/aymerick/douceur/css/rule.go
+++ /dev/null
@@ -1,230 +0,0 @@
-package css
-
-import (
- "fmt"
- "strings"
-)
-
-const (
- indentSpace = 2
-)
-
-// RuleKind represents a Rule kind
-type RuleKind int
-
-// Rule kinds
-const (
- QualifiedRule RuleKind = iota
- AtRule
-)
-
-// At Rules than have Rules inside their block instead of Declarations
-var atRulesWithRulesBlock = []string{
- "@document", "@font-feature-values", "@keyframes", "@media", "@supports",
-}
-
-// Rule represents a parsed CSS rule
-type Rule struct {
- Kind RuleKind
-
- // At Rule name (eg: "@media")
- Name string
-
- // Raw prelude
- Prelude string
-
- // Qualified Rule selectors parsed from prelude
- Selectors []string
-
- // Style properties
- Declarations []*Declaration
-
- // At Rule embedded rules
- Rules []*Rule
-
- // Current rule embedding level
- EmbedLevel int
-}
-
-// NewRule instanciates a new Rule
-func NewRule(kind RuleKind) *Rule {
- return &Rule{
- Kind: kind,
- }
-}
-
-// Returns string representation of rule kind
-func (kind RuleKind) String() string {
- switch kind {
- case QualifiedRule:
- return "Qualified Rule"
- case AtRule:
- return "At Rule"
- default:
- return "WAT"
- }
-}
-
-// EmbedsRules returns true if this rule embeds another rules
-func (rule *Rule) EmbedsRules() bool {
- if rule.Kind == AtRule {
- for _, atRuleName := range atRulesWithRulesBlock {
- if rule.Name == atRuleName {
- return true
- }
- }
- }
-
- return false
-}
-
-// Equal returns true if both rules are equals
-func (rule *Rule) Equal(other *Rule) bool {
- if (rule.Kind != other.Kind) ||
- (rule.Prelude != other.Prelude) ||
- (rule.Name != other.Name) {
- return false
- }
-
- if (len(rule.Selectors) != len(other.Selectors)) ||
- (len(rule.Declarations) != len(other.Declarations)) ||
- (len(rule.Rules) != len(other.Rules)) {
- return false
- }
-
- for i, sel := range rule.Selectors {
- if sel != other.Selectors[i] {
- return false
- }
- }
-
- for i, decl := range rule.Declarations {
- if !decl.Equal(other.Declarations[i]) {
- return false
- }
- }
-
- for i, rule := range rule.Rules {
- if !rule.Equal(other.Rules[i]) {
- return false
- }
- }
-
- return true
-}
-
-// Diff returns a string representation of rules differences
-func (rule *Rule) Diff(other *Rule) []string {
- result := []string{}
-
- if rule.Kind != other.Kind {
- result = append(result, fmt.Sprintf("Kind: %s | %s", rule.Kind.String(), other.Kind.String()))
- }
-
- if rule.Prelude != other.Prelude {
- result = append(result, fmt.Sprintf("Prelude: \"%s\" | \"%s\"", rule.Prelude, other.Prelude))
- }
-
- if rule.Name != other.Name {
- result = append(result, fmt.Sprintf("Name: \"%s\" | \"%s\"", rule.Name, other.Name))
- }
-
- if len(rule.Selectors) != len(other.Selectors) {
- result = append(result, fmt.Sprintf("Selectors: %v | %v", strings.Join(rule.Selectors, ", "), strings.Join(other.Selectors, ", ")))
- } else {
- for i, sel := range rule.Selectors {
- if sel != other.Selectors[i] {
- result = append(result, fmt.Sprintf("Selector: \"%s\" | \"%s\"", sel, other.Selectors[i]))
- }
- }
- }
-
- if len(rule.Declarations) != len(other.Declarations) {
- result = append(result, fmt.Sprintf("Declarations Nb: %d | %d", len(rule.Declarations), len(other.Declarations)))
- } else {
- for i, decl := range rule.Declarations {
- if !decl.Equal(other.Declarations[i]) {
- result = append(result, fmt.Sprintf("Declaration: \"%s\" | \"%s\"", decl.String(), other.Declarations[i].String()))
- }
- }
- }
-
- if len(rule.Rules) != len(other.Rules) {
- result = append(result, fmt.Sprintf("Rules Nb: %d | %d", len(rule.Rules), len(other.Rules)))
- } else {
-
- for i, rule := range rule.Rules {
- if !rule.Equal(other.Rules[i]) {
- result = append(result, fmt.Sprintf("Rule: \"%s\" | \"%s\"", rule.String(), other.Rules[i].String()))
- }
- }
- }
-
- return result
-}
-
-// Returns the string representation of a rule
-func (rule *Rule) String() string {
- result := ""
-
- if rule.Kind == QualifiedRule {
- for i, sel := range rule.Selectors {
- if i != 0 {
- result += ", "
- }
- result += sel
- }
- } else {
- // AtRule
- result += fmt.Sprintf("%s", rule.Name)
-
- if rule.Prelude != "" {
- if result != "" {
- result += " "
- }
- result += fmt.Sprintf("%s", rule.Prelude)
- }
- }
-
- if (len(rule.Declarations) == 0) && (len(rule.Rules) == 0) {
- result += ";"
- } else {
- result += " {\n"
-
- if rule.EmbedsRules() {
- for _, subRule := range rule.Rules {
- result += fmt.Sprintf("%s%s\n", rule.indent(), subRule.String())
- }
- } else {
- for _, decl := range rule.Declarations {
- result += fmt.Sprintf("%s%s\n", rule.indent(), decl.String())
- }
- }
-
- result += fmt.Sprintf("%s}", rule.indentEndBlock())
- }
-
- return result
-}
-
-// Returns identation spaces for declarations and rules
-func (rule *Rule) indent() string {
- result := ""
-
- for i := 0; i < ((rule.EmbedLevel + 1) * indentSpace); i++ {
- result += " "
- }
-
- return result
-}
-
-// Returns identation spaces for end of block character
-func (rule *Rule) indentEndBlock() string {
- result := ""
-
- for i := 0; i < (rule.EmbedLevel * indentSpace); i++ {
- result += " "
- }
-
- return result
-}
diff --git a/vendor/github.com/aymerick/douceur/css/stylesheet.go b/vendor/github.com/aymerick/douceur/css/stylesheet.go
deleted file mode 100644
index 6b32c2e..0000000
--- a/vendor/github.com/aymerick/douceur/css/stylesheet.go
+++ /dev/null
@@ -1,25 +0,0 @@
-package css
-
-// Stylesheet represents a parsed stylesheet
-type Stylesheet struct {
- Rules []*Rule
-}
-
-// NewStylesheet instanciate a new Stylesheet
-func NewStylesheet() *Stylesheet {
- return &Stylesheet{}
-}
-
-// Returns string representation of the Stylesheet
-func (sheet *Stylesheet) String() string {
- result := ""
-
- for _, rule := range sheet.Rules {
- if result != "" {
- result += "\n"
- }
- result += rule.String()
- }
-
- return result
-}
diff --git a/vendor/github.com/aymerick/douceur/parser/parser.go b/vendor/github.com/aymerick/douceur/parser/parser.go
deleted file mode 100644
index 6c4917c..0000000
--- a/vendor/github.com/aymerick/douceur/parser/parser.go
+++ /dev/null
@@ -1,409 +0,0 @@
-package parser
-
-import (
- "errors"
- "fmt"
- "regexp"
- "strings"
-
- "github.com/gorilla/css/scanner"
-
- "github.com/aymerick/douceur/css"
-)
-
-const (
- importantSuffixRegexp = `(?i)\s*!important\s*$`
-)
-
-var (
- importantRegexp *regexp.Regexp
-)
-
-// Parser represents a CSS parser
-type Parser struct {
- scan *scanner.Scanner // Tokenizer
-
- // Tokens parsed but not consumed yet
- tokens []*scanner.Token
-
- // Rule embedding level
- embedLevel int
-}
-
-func init() {
- importantRegexp = regexp.MustCompile(importantSuffixRegexp)
-}
-
-// NewParser instanciates a new parser
-func NewParser(txt string) *Parser {
- return &Parser{
- scan: scanner.New(txt),
- }
-}
-
-// Parse parses a whole stylesheet
-func Parse(text string) (*css.Stylesheet, error) {
- result, err := NewParser(text).ParseStylesheet()
- if err != nil {
- return nil, err
- }
-
- return result, nil
-}
-
-// ParseDeclarations parses CSS declarations
-func ParseDeclarations(text string) ([]*css.Declaration, error) {
- result, err := NewParser(text).ParseDeclarations()
- if err != nil {
- return nil, err
- }
-
- return result, nil
-}
-
-// ParseStylesheet parses a stylesheet
-func (parser *Parser) ParseStylesheet() (*css.Stylesheet, error) {
- result := css.NewStylesheet()
-
- // Parse BOM
- if _, err := parser.parseBOM(); err != nil {
- return result, err
- }
-
- // Parse list of rules
- rules, err := parser.ParseRules()
- if err != nil {
- return result, err
- }
-
- result.Rules = rules
-
- return result, nil
-}
-
-// ParseRules parses a list of rules
-func (parser *Parser) ParseRules() ([]*css.Rule, error) {
- result := []*css.Rule{}
-
- inBlock := false
- if parser.tokenChar("{") {
- // parsing a block of rules
- inBlock = true
- parser.embedLevel++
-
- parser.shiftToken()
- }
-
- for parser.tokenParsable() {
- if parser.tokenIgnorable() {
- parser.shiftToken()
- } else if parser.tokenChar("}") {
- if !inBlock {
- errMsg := fmt.Sprintf("Unexpected } character: %s", parser.nextToken().String())
- return result, errors.New(errMsg)
- }
-
- parser.shiftToken()
- parser.embedLevel--
-
- // finished
- break
- } else {
- rule, err := parser.ParseRule()
- if err != nil {
- return result, err
- }
-
- rule.EmbedLevel = parser.embedLevel
- result = append(result, rule)
- }
- }
-
- return result, parser.err()
-}
-
-// ParseRule parses a rule
-func (parser *Parser) ParseRule() (*css.Rule, error) {
- if parser.tokenAtKeyword() {
- return parser.parseAtRule()
- }
-
- return parser.parseQualifiedRule()
-}
-
-// ParseDeclarations parses a list of declarations
-func (parser *Parser) ParseDeclarations() ([]*css.Declaration, error) {
- result := []*css.Declaration{}
-
- if parser.tokenChar("{") {
- parser.shiftToken()
- }
-
- for parser.tokenParsable() {
- if parser.tokenIgnorable() {
- parser.shiftToken()
- } else if parser.tokenChar("}") {
- // end of block
- parser.shiftToken()
- break
- } else {
- declaration, err := parser.ParseDeclaration()
- if err != nil {
- return result, err
- }
-
- result = append(result, declaration)
- }
- }
-
- return result, parser.err()
-}
-
-// ParseDeclaration parses a declaration
-func (parser *Parser) ParseDeclaration() (*css.Declaration, error) {
- result := css.NewDeclaration()
- curValue := ""
-
- for parser.tokenParsable() {
- if parser.tokenChar(":") {
- result.Property = strings.TrimSpace(curValue)
- curValue = ""
-
- parser.shiftToken()
- } else if parser.tokenChar(";") || parser.tokenChar("}") {
- if result.Property == "" {
- errMsg := fmt.Sprintf("Unexpected ; character: %s", parser.nextToken().String())
- return result, errors.New(errMsg)
- }
-
- if importantRegexp.MatchString(curValue) {
- result.Important = true
- curValue = importantRegexp.ReplaceAllString(curValue, "")
- }
-
- result.Value = strings.TrimSpace(curValue)
-
- if parser.tokenChar(";") {
- parser.shiftToken()
- }
-
- // finished
- break
- } else {
- token := parser.shiftToken()
- curValue += token.Value
- }
- }
-
- // log.Printf("[parsed] Declaration: %s", result.String())
-
- return result, parser.err()
-}
-
-// Parse an At Rule
-func (parser *Parser) parseAtRule() (*css.Rule, error) {
- // parse rule name (eg: "@import")
- token := parser.shiftToken()
-
- result := css.NewRule(css.AtRule)
- result.Name = token.Value
-
- for parser.tokenParsable() {
- if parser.tokenChar(";") {
- parser.shiftToken()
-
- // finished
- break
- } else if parser.tokenChar("{") {
- if result.EmbedsRules() {
- // parse rules block
- rules, err := parser.ParseRules()
- if err != nil {
- return result, err
- }
-
- result.Rules = rules
- } else {
- // parse declarations block
- declarations, err := parser.ParseDeclarations()
- if err != nil {
- return result, err
- }
-
- result.Declarations = declarations
- }
-
- // finished
- break
- } else {
- // parse prelude
- prelude, err := parser.parsePrelude()
- if err != nil {
- return result, err
- }
-
- result.Prelude = prelude
- }
- }
-
- // log.Printf("[parsed] Rule: %s", result.String())
-
- return result, parser.err()
-}
-
-// Parse a Qualified Rule
-func (parser *Parser) parseQualifiedRule() (*css.Rule, error) {
- result := css.NewRule(css.QualifiedRule)
-
- for parser.tokenParsable() {
- if parser.tokenChar("{") {
- if result.Prelude == "" {
- errMsg := fmt.Sprintf("Unexpected { character: %s", parser.nextToken().String())
- return result, errors.New(errMsg)
- }
-
- // parse declarations block
- declarations, err := parser.ParseDeclarations()
- if err != nil {
- return result, err
- }
-
- result.Declarations = declarations
-
- // finished
- break
- } else {
- // parse prelude
- prelude, err := parser.parsePrelude()
- if err != nil {
- return result, err
- }
-
- result.Prelude = prelude
- }
- }
-
- result.Selectors = strings.Split(result.Prelude, ",")
- for i, sel := range result.Selectors {
- result.Selectors[i] = strings.TrimSpace(sel)
- }
-
- // log.Printf("[parsed] Rule: %s", result.String())
-
- return result, parser.err()
-}
-
-// Parse Rule prelude
-func (parser *Parser) parsePrelude() (string, error) {
- result := ""
-
- for parser.tokenParsable() && !parser.tokenEndOfPrelude() {
- token := parser.shiftToken()
- result += token.Value
- }
-
- result = strings.TrimSpace(result)
-
- // log.Printf("[parsed] prelude: %s", result)
-
- return result, parser.err()
-}
-
-// Parse BOM
-func (parser *Parser) parseBOM() (bool, error) {
- if parser.nextToken().Type == scanner.TokenBOM {
- parser.shiftToken()
- return true, nil
- }
-
- return false, parser.err()
-}
-
-// Returns next token without removing it from tokens buffer
-func (parser *Parser) nextToken() *scanner.Token {
- if len(parser.tokens) == 0 {
- // fetch next token
- nextToken := parser.scan.Next()
-
- // log.Printf("[token] %s => %v", nextToken.Type.String(), nextToken.Value)
-
- // queue it
- parser.tokens = append(parser.tokens, nextToken)
- }
-
- return parser.tokens[0]
-}
-
-// Returns next token and remove it from the tokens buffer
-func (parser *Parser) shiftToken() *scanner.Token {
- var result *scanner.Token
-
- result, parser.tokens = parser.tokens[0], parser.tokens[1:]
- return result
-}
-
-// Returns tokenizer error, or nil if no error
-func (parser *Parser) err() error {
- if parser.tokenError() {
- token := parser.nextToken()
- return fmt.Errorf("Tokenizer error: %s", token.String())
- }
-
- return nil
-}
-
-// Returns true if next token is Error
-func (parser *Parser) tokenError() bool {
- return parser.nextToken().Type == scanner.TokenError
-}
-
-// Returns true if next token is EOF
-func (parser *Parser) tokenEOF() bool {
- return parser.nextToken().Type == scanner.TokenEOF
-}
-
-// Returns true if next token is a whitespace
-func (parser *Parser) tokenWS() bool {
- return parser.nextToken().Type == scanner.TokenS
-}
-
-// Returns true if next token is a comment
-func (parser *Parser) tokenComment() bool {
- return parser.nextToken().Type == scanner.TokenComment
-}
-
-// Returns true if next token is a CDO or a CDC
-func (parser *Parser) tokenCDOorCDC() bool {
- switch parser.nextToken().Type {
- case scanner.TokenCDO, scanner.TokenCDC:
- return true
- default:
- return false
- }
-}
-
-// Returns true if next token is ignorable
-func (parser *Parser) tokenIgnorable() bool {
- return parser.tokenWS() || parser.tokenComment() || parser.tokenCDOorCDC()
-}
-
-// Returns true if next token is parsable
-func (parser *Parser) tokenParsable() bool {
- return !parser.tokenEOF() && !parser.tokenError()
-}
-
-// Returns true if next token is an At Rule keyword
-func (parser *Parser) tokenAtKeyword() bool {
- return parser.nextToken().Type == scanner.TokenAtKeyword
-}
-
-// Returns true if next token is given character
-func (parser *Parser) tokenChar(value string) bool {
- token := parser.nextToken()
- return (token.Type == scanner.TokenChar) && (token.Value == value)
-}
-
-// Returns true if next token marks the end of a prelude
-func (parser *Parser) tokenEndOfPrelude() bool {
- return parser.tokenChar(";") || parser.tokenChar("{")
-}
diff --git a/vendor/github.com/charmbracelet/glamour/.gitignore b/vendor/github.com/charmbracelet/glamour/.gitignore
deleted file mode 100644
index b3087b0..0000000
--- a/vendor/github.com/charmbracelet/glamour/.gitignore
+++ /dev/null
@@ -1 +0,0 @@
-cmd/
diff --git a/vendor/github.com/charmbracelet/glamour/.golangci.yml b/vendor/github.com/charmbracelet/glamour/.golangci.yml
deleted file mode 100644
index 36f9966..0000000
--- a/vendor/github.com/charmbracelet/glamour/.golangci.yml
+++ /dev/null
@@ -1,26 +0,0 @@
-run:
- tests: false
-
-issues:
- max-issues-per-linter: 0
- max-same-issues: 0
-
-linters:
- enable:
- - bodyclose
- - dupl
- - exportloopref
- - goconst
- - godot
- - godox
- - goimports
- - gomnd
- - goprintffuncname
- - gosec
- - misspell
- - prealloc
- - rowserrcheck
- - sqlclosecheck
- - unconvert
- - unparam
- - whitespace
diff --git a/vendor/github.com/charmbracelet/glamour/LICENSE b/vendor/github.com/charmbracelet/glamour/LICENSE
deleted file mode 100644
index beda40d..0000000
--- a/vendor/github.com/charmbracelet/glamour/LICENSE
+++ /dev/null
@@ -1,21 +0,0 @@
-MIT License
-
-Copyright (c) 2019 Charmbracelet, Inc
-
-Permission is hereby granted, free of charge, to any person obtaining a copy
-of this software and associated documentation files (the "Software"), to deal
-in the Software without restriction, including without limitation the rights
-to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
-copies of the Software, and to permit persons to whom the Software is
-furnished to do so, subject to the following conditions:
-
-The above copyright notice and this permission notice shall be included in all
-copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
-IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
-FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
-AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
-LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
-OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
-SOFTWARE.
diff --git a/vendor/github.com/charmbracelet/glamour/README.md b/vendor/github.com/charmbracelet/glamour/README.md
deleted file mode 100644
index c7c3cec..0000000
--- a/vendor/github.com/charmbracelet/glamour/README.md
+++ /dev/null
@@ -1,89 +0,0 @@
-# Glamour
-
-
-
-Write handsome command-line tools with *Glamour*.
-
-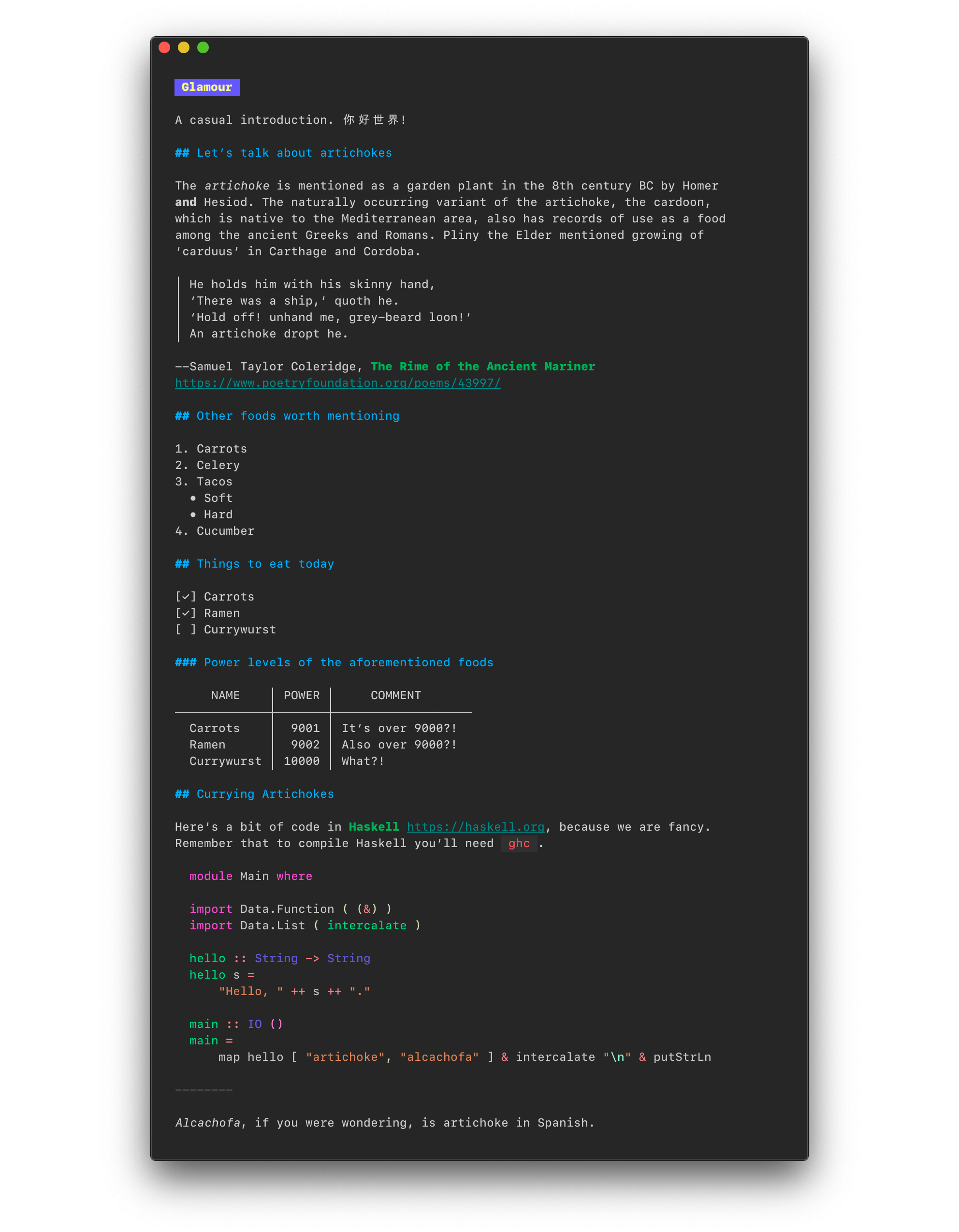
-
-`glamour` lets you render [markdown](https://en.wikipedia.org/wiki/Markdown)
-documents & templates on [ANSI](https://en.wikipedia.org/wiki/ANSI_escape_code)
-compatible terminals. You can create your own stylesheet or simply use one of
-the stylish defaults.
-
-
-## Usage
-
-```go
-import "github.com/charmbracelet/glamour"
-
-in := `# Hello World
-
-This is a simple example of Markdown rendering with Glamour!
-Check out the [other examples](https://github.com/charmbracelet/glamour/tree/master/examples) too.
-
-Bye!
-`
-
-out, err := glamour.Render(in, "dark")
-fmt.Print(out)
-```
-
-
-
-### Custom Renderer
-
-```go
-import "github.com/charmbracelet/glamour"
-
-r, _ := glamour.NewTermRenderer(
- // detect background color and pick either the default dark or light theme
- glamour.WithAutoStyle(),
- // wrap output at specific width
- glamour.WithWordWrap(40),
-)
-
-out, err := r.Render(in)
-fmt.Print(out)
-```
-
-
-## Styles
-
-You can find all available default styles in our [gallery](https://github.com/charmbracelet/glamour/tree/master/styles/gallery).
-Want to create your own style? [Learn how!](https://github.com/charmbracelet/glamour/tree/master/styles)
-
-There are a few options for using a custom style:
-1. Call `glamour.Render(inputText, "desiredStyle")`
-1. Set the `GLAMOUR_STYLE` environment variable to your desired default style or a file location for a style and call `glamour.RenderWithEnvironmentConfig(inputText)`
-1. Set the `GLAMOUR_STYLE` environment variable and pass `glamour.WithEnvironmentConfig()` to your custom renderer
-
-
-## Glamourous Projects
-
-Check out these projects, which use `glamour`:
-- [Glow](https://github.com/charmbracelet/glow), a markdown renderer for
-the command-line.
-- [GitHub CLI](https://github.com/cli/cli), GitHub’s official command line tool.
-- [GLab](https://github.com/profclems/glab), An open source GitLab command line tool.
-
-## License
-
-[MIT](https://github.com/charmbracelet/glamour/raw/master/LICENSE)
-
-
-***
-
-Part of [Charm](https://charm.sh).
-
-
-
-Charm热爱开源 • Charm loves open source
-
diff --git a/vendor/github.com/charmbracelet/glamour/ansi/baseelement.go b/vendor/github.com/charmbracelet/glamour/ansi/baseelement.go
deleted file mode 100644
index c4a388e..0000000
--- a/vendor/github.com/charmbracelet/glamour/ansi/baseelement.go
+++ /dev/null
@@ -1,104 +0,0 @@
-package ansi
-
-import (
- "bytes"
- "io"
- "text/template"
-
- "github.com/muesli/termenv"
-)
-
-// BaseElement renders a styled primitive element.
-type BaseElement struct {
- Token string
- Prefix string
- Suffix string
- Style StylePrimitive
-}
-
-func formatToken(format string, token string) (string, error) {
- var b bytes.Buffer
-
- v := make(map[string]interface{})
- v["text"] = token
-
- tmpl, err := template.New(format).Funcs(TemplateFuncMap).Parse(format)
- if err != nil {
- return "", err
- }
-
- err = tmpl.Execute(&b, v)
- return b.String(), err
-}
-
-func renderText(w io.Writer, p termenv.Profile, rules StylePrimitive, s string) {
- if len(s) == 0 {
- return
- }
-
- out := termenv.String(s)
-
- if rules.Color != nil {
- out = out.Foreground(p.Color(*rules.Color))
- }
- if rules.BackgroundColor != nil {
- out = out.Background(p.Color(*rules.BackgroundColor))
- }
-
- if rules.Underline != nil && *rules.Underline {
- out = out.Underline()
- }
- if rules.Bold != nil && *rules.Bold {
- out = out.Bold()
- }
- if rules.Italic != nil && *rules.Italic {
- out = out.Italic()
- }
- if rules.CrossedOut != nil && *rules.CrossedOut {
- out = out.CrossOut()
- }
- if rules.Overlined != nil && *rules.Overlined {
- out = out.Overline()
- }
- if rules.Inverse != nil && *rules.Inverse {
- out = out.Reverse()
- }
- if rules.Blink != nil && *rules.Blink {
- out = out.Blink()
- }
-
- _, _ = w.Write([]byte(out.String()))
-}
-
-func (e *BaseElement) Render(w io.Writer, ctx RenderContext) error {
- bs := ctx.blockStack
-
- renderText(w, ctx.options.ColorProfile, bs.Current().Style.StylePrimitive, e.Prefix)
- defer func() {
- renderText(w, ctx.options.ColorProfile, bs.Current().Style.StylePrimitive, e.Suffix)
- }()
-
- rules := bs.With(e.Style)
- // render unstyled prefix/suffix
- renderText(w, ctx.options.ColorProfile, bs.Current().Style.StylePrimitive, rules.BlockPrefix)
- defer func() {
- renderText(w, ctx.options.ColorProfile, bs.Current().Style.StylePrimitive, rules.BlockSuffix)
- }()
-
- // render styled prefix/suffix
- renderText(w, ctx.options.ColorProfile, rules, rules.Prefix)
- defer func() {
- renderText(w, ctx.options.ColorProfile, rules, rules.Suffix)
- }()
-
- s := e.Token
- if len(rules.Format) > 0 {
- var err error
- s, err = formatToken(rules.Format, s)
- if err != nil {
- return err
- }
- }
- renderText(w, ctx.options.ColorProfile, rules, s)
- return nil
-}
diff --git a/vendor/github.com/charmbracelet/glamour/ansi/blockelement.go b/vendor/github.com/charmbracelet/glamour/ansi/blockelement.go
deleted file mode 100644
index 893f9e5..0000000
--- a/vendor/github.com/charmbracelet/glamour/ansi/blockelement.go
+++ /dev/null
@@ -1,59 +0,0 @@
-package ansi
-
-import (
- "bytes"
- "io"
-
- "github.com/muesli/reflow/wordwrap"
-)
-
-// BlockElement provides a render buffer for children of a block element.
-// After all children have been rendered into it, it applies indentation and
-// margins around them and writes everything to the parent rendering buffer.
-type BlockElement struct {
- Block *bytes.Buffer
- Style StyleBlock
- Margin bool
- Newline bool
-}
-
-func (e *BlockElement) Render(w io.Writer, ctx RenderContext) error {
- bs := ctx.blockStack
- bs.Push(*e)
-
- renderText(w, ctx.options.ColorProfile, bs.Parent().Style.StylePrimitive, e.Style.BlockPrefix)
- renderText(bs.Current().Block, ctx.options.ColorProfile, bs.Current().Style.StylePrimitive, e.Style.Prefix)
- return nil
-}
-
-func (e *BlockElement) Finish(w io.Writer, ctx RenderContext) error {
- bs := ctx.blockStack
-
- if e.Margin {
- mw := NewMarginWriter(ctx, w, bs.Current().Style)
- _, err := mw.Write(
- wordwrap.Bytes(bs.Current().Block.Bytes(), int(bs.Width(ctx))))
- if err != nil {
- return err
- }
-
- if e.Newline {
- _, err = mw.Write([]byte("\n"))
- if err != nil {
- return err
- }
- }
- } else {
- _, err := bs.Parent().Block.Write(bs.Current().Block.Bytes())
- if err != nil {
- return err
- }
- }
-
- renderText(w, ctx.options.ColorProfile, bs.Current().Style.StylePrimitive, e.Style.Suffix)
- renderText(w, ctx.options.ColorProfile, bs.Parent().Style.StylePrimitive, e.Style.BlockSuffix)
-
- bs.Current().Block.Reset()
- bs.Pop()
- return nil
-}
diff --git a/vendor/github.com/charmbracelet/glamour/ansi/blockstack.go b/vendor/github.com/charmbracelet/glamour/ansi/blockstack.go
deleted file mode 100644
index 96fdb91..0000000
--- a/vendor/github.com/charmbracelet/glamour/ansi/blockstack.go
+++ /dev/null
@@ -1,95 +0,0 @@
-package ansi
-
-import (
- "bytes"
-)
-
-// BlockStack is a stack of block elements, used to calculate the current
-// indentation & margin level during the rendering process.
-type BlockStack []BlockElement
-
-// Len returns the length of the stack.
-func (s *BlockStack) Len() int {
- return len(*s)
-}
-
-// Push appends an item to the stack.
-func (s *BlockStack) Push(e BlockElement) {
- *s = append(*s, e)
-}
-
-// Pop removes the last item on the stack.
-func (s *BlockStack) Pop() {
- stack := *s
- if len(stack) == 0 {
- return
- }
-
- stack = stack[0 : len(stack)-1]
- *s = stack
-}
-
-// Indent returns the current indentation level of all elements in the stack.
-func (s BlockStack) Indent() uint {
- var i uint
-
- for _, v := range s {
- if v.Style.Indent == nil {
- continue
- }
- i += *v.Style.Indent
- }
-
- return i
-}
-
-// Margin returns the current margin level of all elements in the stack.
-func (s BlockStack) Margin() uint {
- var i uint
-
- for _, v := range s {
- if v.Style.Margin == nil {
- continue
- }
- i += *v.Style.Margin
- }
-
- return i
-}
-
-// Width returns the available rendering width
-func (s BlockStack) Width(ctx RenderContext) uint {
- if s.Indent()+s.Margin()*2 > uint(ctx.options.WordWrap) {
- return 0
- }
- return uint(ctx.options.WordWrap) - s.Indent() - s.Margin()*2
-}
-
-// Parent returns the current BlockElement's parent.
-func (s BlockStack) Parent() BlockElement {
- if len(s) == 1 {
- return BlockElement{
- Block: &bytes.Buffer{},
- }
- }
-
- return s[len(s)-2]
-}
-
-// Current returns the current BlockElement.
-func (s BlockStack) Current() BlockElement {
- if len(s) == 0 {
- return BlockElement{
- Block: &bytes.Buffer{},
- }
- }
-
- return s[len(s)-1]
-}
-
-// With returns a StylePrimitive that inherits the current BlockElement's style.
-func (s BlockStack) With(child StylePrimitive) StylePrimitive {
- sb := StyleBlock{}
- sb.StylePrimitive = child
- return cascadeStyle(s.Current().Style, sb, false).StylePrimitive
-}
diff --git a/vendor/github.com/charmbracelet/glamour/ansi/codeblock.go b/vendor/github.com/charmbracelet/glamour/ansi/codeblock.go
deleted file mode 100644
index 3af38df..0000000
--- a/vendor/github.com/charmbracelet/glamour/ansi/codeblock.go
+++ /dev/null
@@ -1,125 +0,0 @@
-package ansi
-
-import (
- "io"
-
- "github.com/alecthomas/chroma"
- "github.com/alecthomas/chroma/quick"
- "github.com/alecthomas/chroma/styles"
- "github.com/muesli/reflow/indent"
-)
-
-// A CodeBlockElement is used to render code blocks.
-type CodeBlockElement struct {
- Code string
- Language string
-}
-
-func chromaStyle(style StylePrimitive) string {
- var s string
-
- if style.Color != nil {
- s = *style.Color
- }
- if style.BackgroundColor != nil {
- if s != "" {
- s += " "
- }
- s += "bg:" + *style.BackgroundColor
- }
- if style.Italic != nil && *style.Italic {
- if s != "" {
- s += " "
- }
- s += "italic"
- }
- if style.Bold != nil && *style.Bold {
- if s != "" {
- s += " "
- }
- s += "bold"
- }
- if style.Underline != nil && *style.Underline {
- if s != "" {
- s += " "
- }
- s += "underline"
- }
-
- return s
-}
-
-func (e *CodeBlockElement) Render(w io.Writer, ctx RenderContext) error {
- bs := ctx.blockStack
-
- var indentation uint
- var margin uint
- rules := ctx.options.Styles.CodeBlock
- if rules.Indent != nil {
- indentation = *rules.Indent
- }
- if rules.Margin != nil {
- margin = *rules.Margin
- }
- theme := rules.Theme
-
- if rules.Chroma != nil && ctx.options.ColorProfile > 1 {
- theme = "charm"
- styles.Register(chroma.MustNewStyle("charm",
- chroma.StyleEntries{
- chroma.Text: chromaStyle(rules.Chroma.Text),
- chroma.Error: chromaStyle(rules.Chroma.Error),
- chroma.Comment: chromaStyle(rules.Chroma.Comment),
- chroma.CommentPreproc: chromaStyle(rules.Chroma.CommentPreproc),
- chroma.Keyword: chromaStyle(rules.Chroma.Keyword),
- chroma.KeywordReserved: chromaStyle(rules.Chroma.KeywordReserved),
- chroma.KeywordNamespace: chromaStyle(rules.Chroma.KeywordNamespace),
- chroma.KeywordType: chromaStyle(rules.Chroma.KeywordType),
- chroma.Operator: chromaStyle(rules.Chroma.Operator),
- chroma.Punctuation: chromaStyle(rules.Chroma.Punctuation),
- chroma.Name: chromaStyle(rules.Chroma.Name),
- chroma.NameBuiltin: chromaStyle(rules.Chroma.NameBuiltin),
- chroma.NameTag: chromaStyle(rules.Chroma.NameTag),
- chroma.NameAttribute: chromaStyle(rules.Chroma.NameAttribute),
- chroma.NameClass: chromaStyle(rules.Chroma.NameClass),
- chroma.NameConstant: chromaStyle(rules.Chroma.NameConstant),
- chroma.NameDecorator: chromaStyle(rules.Chroma.NameDecorator),
- chroma.NameException: chromaStyle(rules.Chroma.NameException),
- chroma.NameFunction: chromaStyle(rules.Chroma.NameFunction),
- chroma.NameOther: chromaStyle(rules.Chroma.NameOther),
- chroma.Literal: chromaStyle(rules.Chroma.Literal),
- chroma.LiteralNumber: chromaStyle(rules.Chroma.LiteralNumber),
- chroma.LiteralDate: chromaStyle(rules.Chroma.LiteralDate),
- chroma.LiteralString: chromaStyle(rules.Chroma.LiteralString),
- chroma.LiteralStringEscape: chromaStyle(rules.Chroma.LiteralStringEscape),
- chroma.GenericDeleted: chromaStyle(rules.Chroma.GenericDeleted),
- chroma.GenericEmph: chromaStyle(rules.Chroma.GenericEmph),
- chroma.GenericInserted: chromaStyle(rules.Chroma.GenericInserted),
- chroma.GenericStrong: chromaStyle(rules.Chroma.GenericStrong),
- chroma.GenericSubheading: chromaStyle(rules.Chroma.GenericSubheading),
- chroma.Background: chromaStyle(rules.Chroma.Background),
- }))
- }
-
- iw := indent.NewWriterPipe(w, indentation+margin, func(wr io.Writer) {
- renderText(w, ctx.options.ColorProfile, bs.Current().Style.StylePrimitive, " ")
- })
-
- if len(theme) > 0 {
- renderText(iw, ctx.options.ColorProfile, bs.Current().Style.StylePrimitive, rules.BlockPrefix)
- err := quick.Highlight(iw, e.Code, e.Language, "terminal256", theme)
- if err != nil {
- return err
- }
- renderText(iw, ctx.options.ColorProfile, bs.Current().Style.StylePrimitive, rules.BlockSuffix)
- return nil
- }
-
- // fallback rendering
- el := &BaseElement{
- Token: e.Code,
- Style: rules.StylePrimitive,
- }
-
- return el.Render(iw, ctx)
-}
diff --git a/vendor/github.com/charmbracelet/glamour/ansi/context.go b/vendor/github.com/charmbracelet/glamour/ansi/context.go
deleted file mode 100644
index ee3c2eb..0000000
--- a/vendor/github.com/charmbracelet/glamour/ansi/context.go
+++ /dev/null
@@ -1,38 +0,0 @@
-package ansi
-
-import (
- "html"
- "strings"
-
- "github.com/microcosm-cc/bluemonday"
-)
-
-// RenderContext holds the current rendering options and state.
-type RenderContext struct {
- options Options
-
- blockStack *BlockStack
- table *TableElement
-
- stripper *bluemonday.Policy
-}
-
-// NewRenderContext returns a new RenderContext.
-func NewRenderContext(options Options) RenderContext {
- return RenderContext{
- options: options,
- blockStack: &BlockStack{},
- table: &TableElement{},
- stripper: bluemonday.StrictPolicy(),
- }
-}
-
-// SanitizeHTML sanitizes HTML content.
-func (ctx RenderContext) SanitizeHTML(s string, trimSpaces bool) string {
- s = ctx.stripper.Sanitize(s)
- if trimSpaces {
- s = strings.TrimSpace(s)
- }
-
- return html.UnescapeString(s)
-}
diff --git a/vendor/github.com/charmbracelet/glamour/ansi/elements.go b/vendor/github.com/charmbracelet/glamour/ansi/elements.go
deleted file mode 100644
index c09ed7f..0000000
--- a/vendor/github.com/charmbracelet/glamour/ansi/elements.go
+++ /dev/null
@@ -1,410 +0,0 @@
-package ansi
-
-import (
- "bytes"
- "fmt"
- "html"
- "io"
- "strings"
-
- east "github.com/yuin/goldmark-emoji/ast"
- "github.com/yuin/goldmark/ast"
- astext "github.com/yuin/goldmark/extension/ast"
-)
-
-// ElementRenderer is called when entering a markdown node.
-type ElementRenderer interface {
- Render(w io.Writer, ctx RenderContext) error
-}
-
-// ElementFinisher is called when leaving a markdown node.
-type ElementFinisher interface {
- Finish(w io.Writer, ctx RenderContext) error
-}
-
-// An Element is used to instruct the renderer how to handle individual markdown
-// nodes.
-type Element struct {
- Entering string
- Exiting string
- Renderer ElementRenderer
- Finisher ElementFinisher
-}
-
-// NewElement returns the appropriate render Element for a given node.
-func (tr *ANSIRenderer) NewElement(node ast.Node, source []byte) Element {
- ctx := tr.context
- // fmt.Print(strings.Repeat(" ", ctx.blockStack.Len()), node.Type(), node.Kind())
- // defer fmt.Println()
-
- switch node.Kind() {
- // Document
- case ast.KindDocument:
- e := &BlockElement{
- Block: &bytes.Buffer{},
- Style: ctx.options.Styles.Document,
- Margin: true,
- }
- return Element{
- Renderer: e,
- Finisher: e,
- }
-
- // Heading
- case ast.KindHeading:
- n := node.(*ast.Heading)
- he := &HeadingElement{
- Level: n.Level,
- First: node.PreviousSibling() == nil,
- }
- return Element{
- Exiting: "",
- Renderer: he,
- Finisher: he,
- }
-
- // Paragraph
- case ast.KindParagraph:
- if node.Parent() != nil && node.Parent().Kind() == ast.KindListItem {
- return Element{}
- }
- return Element{
- Renderer: &ParagraphElement{
- First: node.PreviousSibling() == nil,
- },
- Finisher: &ParagraphElement{},
- }
-
- // Blockquote
- case ast.KindBlockquote:
- e := &BlockElement{
- Block: &bytes.Buffer{},
- Style: cascadeStyle(ctx.blockStack.Current().Style, ctx.options.Styles.BlockQuote, false),
- Margin: true,
- Newline: true,
- }
- return Element{
- Entering: "\n",
- Renderer: e,
- Finisher: e,
- }
-
- // Lists
- case ast.KindList:
- s := ctx.options.Styles.List.StyleBlock
- if s.Indent == nil {
- var i uint
- s.Indent = &i
- }
- n := node.Parent()
- for n != nil {
- if n.Kind() == ast.KindList {
- i := ctx.options.Styles.List.LevelIndent
- s.Indent = &i
- break
- }
- n = n.Parent()
- }
-
- e := &BlockElement{
- Block: &bytes.Buffer{},
- Style: cascadeStyle(ctx.blockStack.Current().Style, s, false),
- Margin: true,
- Newline: true,
- }
- return Element{
- Entering: "\n",
- Renderer: e,
- Finisher: e,
- }
-
- case ast.KindListItem:
- var l uint
- var e uint
- l = 1
- n := node
- for n.PreviousSibling() != nil && (n.PreviousSibling().Kind() == ast.KindListItem) {
- l++
- n = n.PreviousSibling()
- }
- if node.Parent().(*ast.List).IsOrdered() {
- e = l
- }
-
- post := "\n"
- if (node.LastChild() != nil && node.LastChild().Kind() == ast.KindList) ||
- node.NextSibling() == nil {
- post = ""
- }
-
- if node.FirstChild() != nil &&
- node.FirstChild().FirstChild() != nil &&
- node.FirstChild().FirstChild().Kind() == astext.KindTaskCheckBox {
- nc := node.FirstChild().FirstChild().(*astext.TaskCheckBox)
-
- return Element{
- Exiting: post,
- Renderer: &TaskElement{
- Checked: nc.IsChecked,
- },
- }
- }
-
- return Element{
- Exiting: post,
- Renderer: &ItemElement{
- Enumeration: e,
- },
- }
-
- // Text Elements
- case ast.KindText:
- n := node.(*ast.Text)
- s := string(n.Segment.Value(source))
-
- if n.HardLineBreak() || (n.SoftLineBreak()) {
- s += "\n"
- }
- return Element{
- Renderer: &BaseElement{
- Token: html.UnescapeString(s),
- Style: ctx.options.Styles.Text,
- },
- }
-
- case ast.KindEmphasis:
- n := node.(*ast.Emphasis)
- s := string(n.Text(source))
- style := ctx.options.Styles.Emph
- if n.Level > 1 {
- style = ctx.options.Styles.Strong
- }
-
- return Element{
- Renderer: &BaseElement{
- Token: html.UnescapeString(s),
- Style: style,
- },
- }
-
- case astext.KindStrikethrough:
- n := node.(*astext.Strikethrough)
- s := string(n.Text(source))
- style := ctx.options.Styles.Strikethrough
-
- return Element{
- Renderer: &BaseElement{
- Token: html.UnescapeString(s),
- Style: style,
- },
- }
-
- case ast.KindThematicBreak:
- return Element{
- Entering: "",
- Exiting: "",
- Renderer: &BaseElement{
- Style: ctx.options.Styles.HorizontalRule,
- },
- }
-
- // Links
- case ast.KindLink:
- n := node.(*ast.Link)
- return Element{
- Renderer: &LinkElement{
- Text: textFromChildren(node, source),
- BaseURL: ctx.options.BaseURL,
- URL: string(n.Destination),
- },
- }
- case ast.KindAutoLink:
- n := node.(*ast.AutoLink)
- u := string(n.URL(source))
- label := string(n.Label(source))
- if n.AutoLinkType == ast.AutoLinkEmail && !strings.HasPrefix(strings.ToLower(u), "mailto:") {
- u = "mailto:" + u
- }
-
- return Element{
- Renderer: &LinkElement{
- Text: label,
- BaseURL: ctx.options.BaseURL,
- URL: u,
- },
- }
-
- // Images
- case ast.KindImage:
- n := node.(*ast.Image)
- text := string(n.Text(source))
- return Element{
- Renderer: &ImageElement{
- Text: text,
- BaseURL: ctx.options.BaseURL,
- URL: string(n.Destination),
- },
- }
-
- // Code
- case ast.KindFencedCodeBlock:
- n := node.(*ast.FencedCodeBlock)
- l := n.Lines().Len()
- s := ""
- for i := 0; i < l; i++ {
- line := n.Lines().At(i)
- s += string(line.Value(source))
- }
- return Element{
- Entering: "\n",
- Renderer: &CodeBlockElement{
- Code: s,
- Language: string(n.Language(source)),
- },
- }
-
- case ast.KindCodeBlock:
- n := node.(*ast.CodeBlock)
- l := n.Lines().Len()
- s := ""
- for i := 0; i < l; i++ {
- line := n.Lines().At(i)
- s += string(line.Value(source))
- }
- return Element{
- Entering: "\n",
- Renderer: &CodeBlockElement{
- Code: s,
- },
- }
-
- case ast.KindCodeSpan:
- // n := node.(*ast.CodeSpan)
- e := &BlockElement{
- Block: &bytes.Buffer{},
- Style: cascadeStyle(ctx.blockStack.Current().Style, ctx.options.Styles.Code, false),
- }
- return Element{
- Renderer: e,
- Finisher: e,
- }
-
- // Tables
- case astext.KindTable:
- te := &TableElement{}
- return Element{
- Entering: "\n",
- Renderer: te,
- Finisher: te,
- }
-
- case astext.KindTableCell:
- s := ""
- n := node.FirstChild()
- for n != nil {
- s += string(n.Text(source))
- // s += string(n.LinkData.Destination)
- n = n.NextSibling()
- }
-
- return Element{
- Renderer: &TableCellElement{
- Text: s,
- Head: node.Parent().Kind() == astext.KindTableHeader,
- },
- }
-
- case astext.KindTableHeader:
- return Element{
- Finisher: &TableHeadElement{},
- }
- case astext.KindTableRow:
- return Element{
- Finisher: &TableRowElement{},
- }
-
- // HTML Elements
- case ast.KindHTMLBlock:
- n := node.(*ast.HTMLBlock)
- return Element{
- Renderer: &BaseElement{
- Token: ctx.SanitizeHTML(string(n.Text(source)), true) + "\n",
- Style: ctx.options.Styles.HTMLBlock.StylePrimitive,
- },
- }
- case ast.KindRawHTML:
- n := node.(*ast.RawHTML)
- return Element{
- Renderer: &BaseElement{
- Token: ctx.SanitizeHTML(string(n.Text(source)), true),
- Style: ctx.options.Styles.HTMLSpan.StylePrimitive,
- },
- }
-
- // Definition Lists
- case astext.KindDefinitionList:
- e := &BlockElement{
- Block: &bytes.Buffer{},
- Style: cascadeStyle(ctx.blockStack.Current().Style, ctx.options.Styles.DefinitionList, false),
- Margin: true,
- Newline: true,
- }
- return Element{
- Entering: "\n",
- Renderer: e,
- Finisher: e,
- }
-
- case astext.KindDefinitionTerm:
- return Element{
- Renderer: &BaseElement{
- Style: ctx.options.Styles.DefinitionTerm,
- },
- }
-
- case astext.KindDefinitionDescription:
- return Element{
- Renderer: &BaseElement{
- Style: ctx.options.Styles.DefinitionDescription,
- },
- }
-
- // Handled by parents
- case astext.KindTaskCheckBox:
- // handled by KindListItem
- return Element{}
- case ast.KindTextBlock:
- return Element{}
-
- case east.KindEmoji:
- n := node.(*east.Emoji)
- return Element{
- Renderer: &BaseElement{
- Token: string(n.Value.Unicode),
- },
- }
-
- // Unknown case
- default:
- fmt.Println("Warning: unhandled element", node.Kind().String())
- return Element{}
- }
-}
-
-func textFromChildren(node ast.Node, source []byte) string {
- var s string
- for c := node.FirstChild(); c != nil; c = c.NextSibling() {
- if c.Kind() == ast.KindText {
- cn := c.(*ast.Text)
- s += string(cn.Segment.Value(source))
-
- if cn.HardLineBreak() || (cn.SoftLineBreak()) {
- s += "\n"
- }
- } else {
- s += string(c.Text(source))
- }
- }
-
- return s
-}
diff --git a/vendor/github.com/charmbracelet/glamour/ansi/heading.go b/vendor/github.com/charmbracelet/glamour/ansi/heading.go
deleted file mode 100644
index e7ff939..0000000
--- a/vendor/github.com/charmbracelet/glamour/ansi/heading.go
+++ /dev/null
@@ -1,86 +0,0 @@
-package ansi
-
-import (
- "bytes"
- "io"
-
- "github.com/muesli/reflow/indent"
- "github.com/muesli/reflow/wordwrap"
-)
-
-// A HeadingElement is used to render headings.
-type HeadingElement struct {
- Level int
- First bool
-}
-
-func (e *HeadingElement) Render(w io.Writer, ctx RenderContext) error {
- bs := ctx.blockStack
- rules := ctx.options.Styles.Heading
-
- switch e.Level {
- case 1:
- rules = cascadeStyles(true, rules, ctx.options.Styles.H1)
- case 2:
- rules = cascadeStyles(true, rules, ctx.options.Styles.H2)
- case 3:
- rules = cascadeStyles(true, rules, ctx.options.Styles.H3)
- case 4:
- rules = cascadeStyles(true, rules, ctx.options.Styles.H4)
- case 5:
- rules = cascadeStyles(true, rules, ctx.options.Styles.H5)
- case 6:
- rules = cascadeStyles(true, rules, ctx.options.Styles.H6)
- }
-
- if !e.First {
- renderText(w, ctx.options.ColorProfile, bs.Current().Style.StylePrimitive, "\n")
- }
-
- be := BlockElement{
- Block: &bytes.Buffer{},
- Style: cascadeStyle(bs.Current().Style, rules, false),
- }
- bs.Push(be)
-
- renderText(w, ctx.options.ColorProfile, bs.Parent().Style.StylePrimitive, rules.BlockPrefix)
- renderText(bs.Current().Block, ctx.options.ColorProfile, bs.Current().Style.StylePrimitive, rules.Prefix)
- return nil
-}
-
-func (e *HeadingElement) Finish(w io.Writer, ctx RenderContext) error {
- bs := ctx.blockStack
- rules := bs.Current().Style
-
- var indentation uint
- var margin uint
- if rules.Indent != nil {
- indentation = *rules.Indent
- }
- if rules.Margin != nil {
- margin = *rules.Margin
- }
-
- iw := indent.NewWriterPipe(w, indentation+margin, func(wr io.Writer) {
- renderText(w, ctx.options.ColorProfile, bs.Parent().Style.StylePrimitive, " ")
- })
-
- flow := wordwrap.NewWriter(int(bs.Width(ctx) - indentation - margin*2))
- _, err := flow.Write(bs.Current().Block.Bytes())
- if err != nil {
- return err
- }
- flow.Close()
-
- _, err = iw.Write(flow.Bytes())
- if err != nil {
- return err
- }
-
- renderText(w, ctx.options.ColorProfile, bs.Current().Style.StylePrimitive, rules.Suffix)
- renderText(w, ctx.options.ColorProfile, bs.Parent().Style.StylePrimitive, rules.BlockSuffix)
-
- bs.Current().Block.Reset()
- bs.Pop()
- return nil
-}
diff --git a/vendor/github.com/charmbracelet/glamour/ansi/image.go b/vendor/github.com/charmbracelet/glamour/ansi/image.go
deleted file mode 100644
index f5edfaf..0000000
--- a/vendor/github.com/charmbracelet/glamour/ansi/image.go
+++ /dev/null
@@ -1,39 +0,0 @@
-package ansi
-
-import (
- "io"
-)
-
-// An ImageElement is used to render images elements.
-type ImageElement struct {
- Text string
- BaseURL string
- URL string
- Child ElementRenderer // FIXME
-}
-
-func (e *ImageElement) Render(w io.Writer, ctx RenderContext) error {
- if len(e.Text) > 0 {
- el := &BaseElement{
- Token: e.Text,
- Style: ctx.options.Styles.ImageText,
- }
- err := el.Render(w, ctx)
- if err != nil {
- return err
- }
- }
- if len(e.URL) > 0 {
- el := &BaseElement{
- Token: resolveRelativeURL(e.BaseURL, e.URL),
- Prefix: " ",
- Style: ctx.options.Styles.Image,
- }
- err := el.Render(w, ctx)
- if err != nil {
- return err
- }
- }
-
- return nil
-}
diff --git a/vendor/github.com/charmbracelet/glamour/ansi/link.go b/vendor/github.com/charmbracelet/glamour/ansi/link.go
deleted file mode 100644
index 4cb5931..0000000
--- a/vendor/github.com/charmbracelet/glamour/ansi/link.go
+++ /dev/null
@@ -1,78 +0,0 @@
-package ansi
-
-import (
- "io"
- "net/url"
-)
-
-// A LinkElement is used to render hyperlinks.
-type LinkElement struct {
- Text string
- BaseURL string
- URL string
- Child ElementRenderer // FIXME
-}
-
-func (e *LinkElement) Render(w io.Writer, ctx RenderContext) error {
- var textRendered bool
- if len(e.Text) > 0 && e.Text != e.URL {
- textRendered = true
-
- el := &BaseElement{
- Token: e.Text,
- Style: ctx.options.Styles.LinkText,
- }
- err := el.Render(w, ctx)
- if err != nil {
- return err
- }
- }
-
- /*
- if node.LastChild != nil {
- if node.LastChild.Type == bf.Image {
- el := tr.NewElement(node.LastChild)
- err := el.Renderer.Render(w, node.LastChild, tr)
- if err != nil {
- return err
- }
- }
- if len(node.LastChild.Literal) > 0 &&
- string(node.LastChild.Literal) != string(node.LinkData.Destination) {
- textRendered = true
- el := &BaseElement{
- Token: string(node.LastChild.Literal),
- Style: ctx.style[LinkText],
- }
- err := el.Render(w, node.LastChild, tr)
- if err != nil {
- return err
- }
- }
- }
- */
-
- u, err := url.Parse(e.URL)
- if err == nil &&
- "#"+u.Fragment != e.URL { // if the URL only consists of an anchor, ignore it
- pre := " "
- style := ctx.options.Styles.Link
- if !textRendered {
- pre = ""
- style.BlockPrefix = ""
- style.BlockSuffix = ""
- }
-
- el := &BaseElement{
- Token: resolveRelativeURL(e.BaseURL, e.URL),
- Prefix: pre,
- Style: style,
- }
- err := el.Render(w, ctx)
- if err != nil {
- return err
- }
- }
-
- return nil
-}
diff --git a/vendor/github.com/charmbracelet/glamour/ansi/listitem.go b/vendor/github.com/charmbracelet/glamour/ansi/listitem.go
deleted file mode 100644
index a64b10d..0000000
--- a/vendor/github.com/charmbracelet/glamour/ansi/listitem.go
+++ /dev/null
@@ -1,27 +0,0 @@
-package ansi
-
-import (
- "io"
- "strconv"
-)
-
-// An ItemElement is used to render items inside a list.
-type ItemElement struct {
- Enumeration uint
-}
-
-func (e *ItemElement) Render(w io.Writer, ctx RenderContext) error {
- var el *BaseElement
- if e.Enumeration > 0 {
- el = &BaseElement{
- Style: ctx.options.Styles.Enumeration,
- Prefix: strconv.FormatInt(int64(e.Enumeration), 10),
- }
- } else {
- el = &BaseElement{
- Style: ctx.options.Styles.Item,
- }
- }
-
- return el.Render(w, ctx)
-}
diff --git a/vendor/github.com/charmbracelet/glamour/ansi/margin.go b/vendor/github.com/charmbracelet/glamour/ansi/margin.go
deleted file mode 100644
index e039783..0000000
--- a/vendor/github.com/charmbracelet/glamour/ansi/margin.go
+++ /dev/null
@@ -1,52 +0,0 @@
-package ansi
-
-import (
- "io"
-
- "github.com/muesli/reflow/indent"
- "github.com/muesli/reflow/padding"
-)
-
-// MarginWriter is a Writer that applies indentation and padding around
-// whatever you write to it.
-type MarginWriter struct {
- w io.Writer
- pw *padding.Writer
- iw *indent.Writer
-}
-
-// NewMarginWriter returns a new MarginWriter.
-func NewMarginWriter(ctx RenderContext, w io.Writer, rules StyleBlock) *MarginWriter {
- bs := ctx.blockStack
-
- var indentation uint
- var margin uint
- if rules.Indent != nil {
- indentation = *rules.Indent
- }
- if rules.Margin != nil {
- margin = *rules.Margin
- }
-
- pw := padding.NewWriterPipe(w, bs.Width(ctx), func(wr io.Writer) {
- renderText(w, ctx.options.ColorProfile, rules.StylePrimitive, " ")
- })
-
- ic := " "
- if rules.IndentToken != nil {
- ic = *rules.IndentToken
- }
- iw := indent.NewWriterPipe(pw, indentation+margin, func(wr io.Writer) {
- renderText(w, ctx.options.ColorProfile, bs.Parent().Style.StylePrimitive, ic)
- })
-
- return &MarginWriter{
- w: w,
- pw: pw,
- iw: iw,
- }
-}
-
-func (w *MarginWriter) Write(b []byte) (int, error) {
- return w.iw.Write(b)
-}
diff --git a/vendor/github.com/charmbracelet/glamour/ansi/paragraph.go b/vendor/github.com/charmbracelet/glamour/ansi/paragraph.go
deleted file mode 100644
index 71e0725..0000000
--- a/vendor/github.com/charmbracelet/glamour/ansi/paragraph.go
+++ /dev/null
@@ -1,58 +0,0 @@
-package ansi
-
-import (
- "bytes"
- "io"
- "strings"
-
- "github.com/muesli/reflow/wordwrap"
-)
-
-// A ParagraphElement is used to render individual paragraphs.
-type ParagraphElement struct {
- First bool
-}
-
-func (e *ParagraphElement) Render(w io.Writer, ctx RenderContext) error {
- bs := ctx.blockStack
- rules := ctx.options.Styles.Paragraph
-
- if !e.First {
- _, _ = w.Write([]byte("\n"))
- }
- be := BlockElement{
- Block: &bytes.Buffer{},
- Style: cascadeStyle(bs.Current().Style, rules, false),
- }
- bs.Push(be)
-
- renderText(w, ctx.options.ColorProfile, bs.Parent().Style.StylePrimitive, rules.BlockPrefix)
- renderText(bs.Current().Block, ctx.options.ColorProfile, bs.Current().Style.StylePrimitive, rules.Prefix)
- return nil
-}
-
-func (e *ParagraphElement) Finish(w io.Writer, ctx RenderContext) error {
- bs := ctx.blockStack
- rules := bs.Current().Style
-
- mw := NewMarginWriter(ctx, w, rules)
- if len(strings.TrimSpace(bs.Current().Block.String())) > 0 {
- flow := wordwrap.NewWriter(int(bs.Width(ctx)))
- flow.KeepNewlines = false
- _, _ = flow.Write(bs.Current().Block.Bytes())
- flow.Close()
-
- _, err := mw.Write(flow.Bytes())
- if err != nil {
- return err
- }
- _, _ = mw.Write([]byte("\n"))
- }
-
- renderText(w, ctx.options.ColorProfile, bs.Current().Style.StylePrimitive, rules.Suffix)
- renderText(w, ctx.options.ColorProfile, bs.Parent().Style.StylePrimitive, rules.BlockSuffix)
-
- bs.Current().Block.Reset()
- bs.Pop()
- return nil
-}
diff --git a/vendor/github.com/charmbracelet/glamour/ansi/renderer.go b/vendor/github.com/charmbracelet/glamour/ansi/renderer.go
deleted file mode 100644
index ddadbb7..0000000
--- a/vendor/github.com/charmbracelet/glamour/ansi/renderer.go
+++ /dev/null
@@ -1,167 +0,0 @@
-package ansi
-
-import (
- "io"
- "net/url"
- "strings"
-
- "github.com/muesli/termenv"
- east "github.com/yuin/goldmark-emoji/ast"
- "github.com/yuin/goldmark/ast"
- astext "github.com/yuin/goldmark/extension/ast"
- "github.com/yuin/goldmark/renderer"
- "github.com/yuin/goldmark/util"
-)
-
-// Options is used to configure an ANSIRenderer.
-type Options struct {
- BaseURL string
- WordWrap int
- ColorProfile termenv.Profile
- Styles StyleConfig
-}
-
-// ANSIRenderer renders markdown content as ANSI escaped sequences.
-type ANSIRenderer struct {
- context RenderContext
-}
-
-// NewRenderer returns a new ANSIRenderer with style and options set.
-func NewRenderer(options Options) *ANSIRenderer {
- return &ANSIRenderer{
- context: NewRenderContext(options),
- }
-}
-
-// RegisterFuncs implements NodeRenderer.RegisterFuncs.
-func (r *ANSIRenderer) RegisterFuncs(reg renderer.NodeRendererFuncRegisterer) {
- // blocks
- reg.Register(ast.KindDocument, r.renderNode)
- reg.Register(ast.KindHeading, r.renderNode)
- reg.Register(ast.KindBlockquote, r.renderNode)
- reg.Register(ast.KindCodeBlock, r.renderNode)
- reg.Register(ast.KindFencedCodeBlock, r.renderNode)
- reg.Register(ast.KindHTMLBlock, r.renderNode)
- reg.Register(ast.KindList, r.renderNode)
- reg.Register(ast.KindListItem, r.renderNode)
- reg.Register(ast.KindParagraph, r.renderNode)
- reg.Register(ast.KindTextBlock, r.renderNode)
- reg.Register(ast.KindThematicBreak, r.renderNode)
-
- // inlines
- reg.Register(ast.KindAutoLink, r.renderNode)
- reg.Register(ast.KindCodeSpan, r.renderNode)
- reg.Register(ast.KindEmphasis, r.renderNode)
- reg.Register(ast.KindImage, r.renderNode)
- reg.Register(ast.KindLink, r.renderNode)
- reg.Register(ast.KindRawHTML, r.renderNode)
- reg.Register(ast.KindText, r.renderNode)
- reg.Register(ast.KindString, r.renderNode)
-
- // tables
- reg.Register(astext.KindTable, r.renderNode)
- reg.Register(astext.KindTableHeader, r.renderNode)
- reg.Register(astext.KindTableRow, r.renderNode)
- reg.Register(astext.KindTableCell, r.renderNode)
-
- // definitions
- reg.Register(astext.KindDefinitionList, r.renderNode)
- reg.Register(astext.KindDefinitionTerm, r.renderNode)
- reg.Register(astext.KindDefinitionDescription, r.renderNode)
-
- // footnotes
- reg.Register(astext.KindFootnote, r.renderNode)
- reg.Register(astext.KindFootnoteList, r.renderNode)
- reg.Register(astext.KindFootnoteLink, r.renderNode)
- reg.Register(astext.KindFootnoteBacklink, r.renderNode)
-
- // checkboxes
- reg.Register(astext.KindTaskCheckBox, r.renderNode)
-
- // strikethrough
- reg.Register(astext.KindStrikethrough, r.renderNode)
-
- // emoji
- reg.Register(east.KindEmoji, r.renderNode)
-}
-
-func (r *ANSIRenderer) renderNode(w util.BufWriter, source []byte, node ast.Node, entering bool) (ast.WalkStatus, error) {
- // _, _ = w.Write([]byte(node.Type.String()))
- writeTo := io.Writer(w)
- bs := r.context.blockStack
-
- // children get rendered by their parent
- if isChild(node) {
- return ast.WalkContinue, nil
- }
-
- e := r.NewElement(node, source)
- if entering {
- // everything below the Document element gets rendered into a block buffer
- if bs.Len() > 0 {
- writeTo = io.Writer(bs.Current().Block)
- }
-
- _, _ = writeTo.Write([]byte(e.Entering))
- if e.Renderer != nil {
- err := e.Renderer.Render(writeTo, r.context)
- if err != nil {
- return ast.WalkStop, err
- }
- }
- } else {
- // everything below the Document element gets rendered into a block buffer
- if bs.Len() > 0 {
- writeTo = io.Writer(bs.Parent().Block)
- }
-
- // if we're finished rendering the entire document,
- // flush to the real writer
- if node.Type() == ast.TypeDocument {
- writeTo = w
- }
-
- if e.Finisher != nil {
- err := e.Finisher.Finish(writeTo, r.context)
- if err != nil {
- return ast.WalkStop, err
- }
- }
- _, _ = bs.Current().Block.Write([]byte(e.Exiting))
- }
-
- return ast.WalkContinue, nil
-}
-
-func isChild(node ast.Node) bool {
- if node.Parent() != nil && node.Parent().Kind() == ast.KindBlockquote {
- // skip paragraph within blockquote to avoid reflowing text
- return true
- }
- for n := node.Parent(); n != nil; n = n.Parent() {
- // These types are already rendered by their parent
- switch n.Kind() {
- case ast.KindLink, ast.KindImage, ast.KindEmphasis, astext.KindStrikethrough, astext.KindTableCell:
- return true
- }
- }
-
- return false
-}
-
-func resolveRelativeURL(baseURL string, rel string) string {
- u, err := url.Parse(rel)
- if err != nil {
- return rel
- }
- if u.IsAbs() {
- return rel
- }
- u.Path = strings.TrimPrefix(u.Path, "/")
-
- base, err := url.Parse(baseURL)
- if err != nil {
- return rel
- }
- return base.ResolveReference(u).String()
-}
diff --git a/vendor/github.com/charmbracelet/glamour/ansi/style.go b/vendor/github.com/charmbracelet/glamour/ansi/style.go
deleted file mode 100644
index cdff869..0000000
--- a/vendor/github.com/charmbracelet/glamour/ansi/style.go
+++ /dev/null
@@ -1,227 +0,0 @@
-package ansi
-
-// Chroma holds all the chroma settings.
-type Chroma struct {
- Text StylePrimitive `json:"text,omitempty"`
- Error StylePrimitive `json:"error,omitempty"`
- Comment StylePrimitive `json:"comment,omitempty"`
- CommentPreproc StylePrimitive `json:"comment_preproc,omitempty"`
- Keyword StylePrimitive `json:"keyword,omitempty"`
- KeywordReserved StylePrimitive `json:"keyword_reserved,omitempty"`
- KeywordNamespace StylePrimitive `json:"keyword_namespace,omitempty"`
- KeywordType StylePrimitive `json:"keyword_type,omitempty"`
- Operator StylePrimitive `json:"operator,omitempty"`
- Punctuation StylePrimitive `json:"punctuation,omitempty"`
- Name StylePrimitive `json:"name,omitempty"`
- NameBuiltin StylePrimitive `json:"name_builtin,omitempty"`
- NameTag StylePrimitive `json:"name_tag,omitempty"`
- NameAttribute StylePrimitive `json:"name_attribute,omitempty"`
- NameClass StylePrimitive `json:"name_class,omitempty"`
- NameConstant StylePrimitive `json:"name_constant,omitempty"`
- NameDecorator StylePrimitive `json:"name_decorator,omitempty"`
- NameException StylePrimitive `json:"name_exception,omitempty"`
- NameFunction StylePrimitive `json:"name_function,omitempty"`
- NameOther StylePrimitive `json:"name_other,omitempty"`
- Literal StylePrimitive `json:"literal,omitempty"`
- LiteralNumber StylePrimitive `json:"literal_number,omitempty"`
- LiteralDate StylePrimitive `json:"literal_date,omitempty"`
- LiteralString StylePrimitive `json:"literal_string,omitempty"`
- LiteralStringEscape StylePrimitive `json:"literal_string_escape,omitempty"`
- GenericDeleted StylePrimitive `json:"generic_deleted,omitempty"`
- GenericEmph StylePrimitive `json:"generic_emph,omitempty"`
- GenericInserted StylePrimitive `json:"generic_inserted,omitempty"`
- GenericStrong StylePrimitive `json:"generic_strong,omitempty"`
- GenericSubheading StylePrimitive `json:"generic_subheading,omitempty"`
- Background StylePrimitive `json:"background,omitempty"`
-}
-
-// StylePrimitive holds all the basic style settings.
-type StylePrimitive struct {
- BlockPrefix string `json:"block_prefix,omitempty"`
- BlockSuffix string `json:"block_suffix,omitempty"`
- Prefix string `json:"prefix,omitempty"`
- Suffix string `json:"suffix,omitempty"`
- Color *string `json:"color,omitempty"`
- BackgroundColor *string `json:"background_color,omitempty"`
- Underline *bool `json:"underline,omitempty"`
- Bold *bool `json:"bold,omitempty"`
- Italic *bool `json:"italic,omitempty"`
- CrossedOut *bool `json:"crossed_out,omitempty"`
- Faint *bool `json:"faint,omitempty"`
- Conceal *bool `json:"conceal,omitempty"`
- Overlined *bool `json:"overlined,omitempty"`
- Inverse *bool `json:"inverse,omitempty"`
- Blink *bool `json:"blink,omitempty"`
- Format string `json:"format,omitempty"`
-}
-
-// StyleTask holds the style settings for a task item.
-type StyleTask struct {
- StylePrimitive
- Ticked string `json:"ticked,omitempty"`
- Unticked string `json:"unticked,omitempty"`
-}
-
-// StyleBlock holds the basic style settings for block elements.
-type StyleBlock struct {
- StylePrimitive
- Indent *uint `json:"indent,omitempty"`
- IndentToken *string `json:"indent_token,omitempty"`
- Margin *uint `json:"margin,omitempty"`
-}
-
-// StyleCodeBlock holds the style settings for a code block.
-type StyleCodeBlock struct {
- StyleBlock
- Theme string `json:"theme,omitempty"`
- Chroma *Chroma `json:"chroma,omitempty"`
-}
-
-// StyleList holds the style settings for a list.
-type StyleList struct {
- StyleBlock
- LevelIndent uint `json:"level_indent,omitempty"`
-}
-
-// StyleTable holds the style settings for a table.
-type StyleTable struct {
- StyleBlock
- CenterSeparator *string `json:"center_separator,omitempty"`
- ColumnSeparator *string `json:"column_separator,omitempty"`
- RowSeparator *string `json:"row_separator,omitempty"`
-}
-
-// StyleConfig is used to configure the styling behavior of an ANSIRenderer.
-type StyleConfig struct {
- Document StyleBlock `json:"document,omitempty"`
- BlockQuote StyleBlock `json:"block_quote,omitempty"`
- Paragraph StyleBlock `json:"paragraph,omitempty"`
- List StyleList `json:"list,omitempty"`
-
- Heading StyleBlock `json:"heading,omitempty"`
- H1 StyleBlock `json:"h1,omitempty"`
- H2 StyleBlock `json:"h2,omitempty"`
- H3 StyleBlock `json:"h3,omitempty"`
- H4 StyleBlock `json:"h4,omitempty"`
- H5 StyleBlock `json:"h5,omitempty"`
- H6 StyleBlock `json:"h6,omitempty"`
-
- Text StylePrimitive `json:"text,omitempty"`
- Strikethrough StylePrimitive `json:"strikethrough,omitempty"`
- Emph StylePrimitive `json:"emph,omitempty"`
- Strong StylePrimitive `json:"strong,omitempty"`
- HorizontalRule StylePrimitive `json:"hr,omitempty"`
-
- Item StylePrimitive `json:"item,omitempty"`
- Enumeration StylePrimitive `json:"enumeration,omitempty"`
- Task StyleTask `json:"task,omitempty"`
-
- Link StylePrimitive `json:"link,omitempty"`
- LinkText StylePrimitive `json:"link_text,omitempty"`
-
- Image StylePrimitive `json:"image,omitempty"`
- ImageText StylePrimitive `json:"image_text,omitempty"`
-
- Code StyleBlock `json:"code,omitempty"`
- CodeBlock StyleCodeBlock `json:"code_block,omitempty"`
-
- Table StyleTable `json:"table,omitempty"`
-
- DefinitionList StyleBlock `json:"definition_list,omitempty"`
- DefinitionTerm StylePrimitive `json:"definition_term,omitempty"`
- DefinitionDescription StylePrimitive `json:"definition_description,omitempty"`
-
- HTMLBlock StyleBlock `json:"html_block,omitempty"`
- HTMLSpan StyleBlock `json:"html_span,omitempty"`
-}
-
-func cascadeStyles(toBlock bool, s ...StyleBlock) StyleBlock {
- var r StyleBlock
-
- for _, v := range s {
- r = cascadeStyle(r, v, toBlock)
- }
- return r
-}
-
-func cascadeStyle(parent StyleBlock, child StyleBlock, toBlock bool) StyleBlock {
- s := child
-
- s.Color = parent.Color
- s.BackgroundColor = parent.BackgroundColor
- s.Underline = parent.Underline
- s.Bold = parent.Bold
- s.Italic = parent.Italic
- s.CrossedOut = parent.CrossedOut
- s.Faint = parent.Faint
- s.Conceal = parent.Conceal
- s.Overlined = parent.Overlined
- s.Inverse = parent.Inverse
- s.Blink = parent.Blink
-
- if toBlock {
- s.Indent = parent.Indent
- s.Margin = parent.Margin
- s.BlockPrefix = parent.BlockPrefix
- s.BlockSuffix = parent.BlockSuffix
- s.Prefix = parent.Prefix
- s.Suffix = parent.Suffix
- }
-
- if child.Color != nil {
- s.Color = child.Color
- }
- if child.BackgroundColor != nil {
- s.BackgroundColor = child.BackgroundColor
- }
- if child.Indent != nil {
- s.Indent = child.Indent
- }
- if child.Margin != nil {
- s.Margin = child.Margin
- }
- if child.Underline != nil {
- s.Underline = child.Underline
- }
- if child.Bold != nil {
- s.Bold = child.Bold
- }
- if child.Italic != nil {
- s.Italic = child.Italic
- }
- if child.CrossedOut != nil {
- s.CrossedOut = child.CrossedOut
- }
- if child.Faint != nil {
- s.Faint = child.Faint
- }
- if child.Conceal != nil {
- s.Conceal = child.Conceal
- }
- if child.Overlined != nil {
- s.Overlined = child.Overlined
- }
- if child.Inverse != nil {
- s.Inverse = child.Inverse
- }
- if child.Blink != nil {
- s.Blink = child.Blink
- }
- if child.BlockPrefix != "" {
- s.BlockPrefix = child.BlockPrefix
- }
- if child.BlockSuffix != "" {
- s.BlockSuffix = child.BlockSuffix
- }
- if child.Prefix != "" {
- s.Prefix = child.Prefix
- }
- if child.Suffix != "" {
- s.Suffix = child.Suffix
- }
- if child.Format != "" {
- s.Format = child.Format
- }
-
- return s
-}
diff --git a/vendor/github.com/charmbracelet/glamour/ansi/stylewriter.go b/vendor/github.com/charmbracelet/glamour/ansi/stylewriter.go
deleted file mode 100644
index 28c01e0..0000000
--- a/vendor/github.com/charmbracelet/glamour/ansi/stylewriter.go
+++ /dev/null
@@ -1,33 +0,0 @@
-package ansi
-
-import (
- "bytes"
- "io"
-)
-
-// StyleWriter is a Writer that applies styling on whatever you write to it.
-type StyleWriter struct {
- ctx RenderContext
- w io.Writer
- buf bytes.Buffer
- rules StylePrimitive
-}
-
-// NewStyleWriter returns a new StyleWriter.
-func NewStyleWriter(ctx RenderContext, w io.Writer, rules StylePrimitive) *StyleWriter {
- return &StyleWriter{
- ctx: ctx,
- w: w,
- rules: rules,
- }
-}
-
-func (w *StyleWriter) Write(b []byte) (int, error) {
- return w.buf.Write(b)
-}
-
-// Close must be called when you're finished writing to a StyleWriter.
-func (w *StyleWriter) Close() error {
- renderText(w.w, w.ctx.options.ColorProfile, w.rules, w.buf.String())
- return nil
-}
diff --git a/vendor/github.com/charmbracelet/glamour/ansi/table.go b/vendor/github.com/charmbracelet/glamour/ansi/table.go
deleted file mode 100644
index 9432f00..0000000
--- a/vendor/github.com/charmbracelet/glamour/ansi/table.go
+++ /dev/null
@@ -1,108 +0,0 @@
-package ansi
-
-import (
- "io"
-
- "github.com/muesli/reflow/indent"
- "github.com/olekukonko/tablewriter"
-)
-
-// A TableElement is used to render tables.
-type TableElement struct {
- writer *tablewriter.Table
- styleWriter *StyleWriter
- header []string
- cell []string
-}
-
-// A TableRowElement is used to render a single row in a table.
-type TableRowElement struct {
-}
-
-// A TableHeadElement is used to render a table's head element.
-type TableHeadElement struct {
-}
-
-// A TableCellElement is used to render a single cell in a row.
-type TableCellElement struct {
- Text string
- Head bool
-}
-
-func (e *TableElement) Render(w io.Writer, ctx RenderContext) error {
- bs := ctx.blockStack
-
- var indentation uint
- var margin uint
- rules := ctx.options.Styles.Table
- if rules.Indent != nil {
- indentation = *rules.Indent
- }
- if rules.Margin != nil {
- margin = *rules.Margin
- }
-
- iw := indent.NewWriterPipe(w, indentation+margin, func(wr io.Writer) {
- renderText(w, ctx.options.ColorProfile, bs.Current().Style.StylePrimitive, " ")
- })
-
- style := bs.With(rules.StylePrimitive)
- ctx.table.styleWriter = NewStyleWriter(ctx, iw, style)
-
- renderText(w, ctx.options.ColorProfile, bs.Current().Style.StylePrimitive, rules.BlockPrefix)
- renderText(ctx.table.styleWriter, ctx.options.ColorProfile, style, rules.Prefix)
- ctx.table.writer = tablewriter.NewWriter(ctx.table.styleWriter)
- return nil
-}
-
-func (e *TableElement) Finish(w io.Writer, ctx RenderContext) error {
- rules := ctx.options.Styles.Table
-
- ctx.table.writer.SetBorders(tablewriter.Border{Left: false, Top: false, Right: false, Bottom: false})
- if rules.CenterSeparator != nil {
- ctx.table.writer.SetCenterSeparator(*rules.CenterSeparator)
- }
- if rules.ColumnSeparator != nil {
- ctx.table.writer.SetColumnSeparator(*rules.ColumnSeparator)
- }
- if rules.RowSeparator != nil {
- ctx.table.writer.SetRowSeparator(*rules.RowSeparator)
- }
-
- ctx.table.writer.Render()
- ctx.table.writer = nil
-
- renderText(ctx.table.styleWriter, ctx.options.ColorProfile, ctx.blockStack.With(rules.StylePrimitive), rules.Suffix)
- renderText(ctx.table.styleWriter, ctx.options.ColorProfile, ctx.blockStack.Current().Style.StylePrimitive, rules.BlockSuffix)
- return ctx.table.styleWriter.Close()
-}
-
-func (e *TableRowElement) Finish(w io.Writer, ctx RenderContext) error {
- if ctx.table.writer == nil {
- return nil
- }
-
- ctx.table.writer.Append(ctx.table.cell)
- ctx.table.cell = []string{}
- return nil
-}
-
-func (e *TableHeadElement) Finish(w io.Writer, ctx RenderContext) error {
- if ctx.table.writer == nil {
- return nil
- }
-
- ctx.table.writer.SetHeader(ctx.table.header)
- ctx.table.header = []string{}
- return nil
-}
-
-func (e *TableCellElement) Render(w io.Writer, ctx RenderContext) error {
- if e.Head {
- ctx.table.header = append(ctx.table.header, e.Text)
- } else {
- ctx.table.cell = append(ctx.table.cell, e.Text)
- }
-
- return nil
-}
diff --git a/vendor/github.com/charmbracelet/glamour/ansi/task.go b/vendor/github.com/charmbracelet/glamour/ansi/task.go
deleted file mode 100644
index 468c8fa..0000000
--- a/vendor/github.com/charmbracelet/glamour/ansi/task.go
+++ /dev/null
@@ -1,26 +0,0 @@
-package ansi
-
-import (
- "io"
-)
-
-// A TaskElement is used to render tasks inside a todo-list.
-type TaskElement struct {
- Checked bool
-}
-
-func (e *TaskElement) Render(w io.Writer, ctx RenderContext) error {
- var el *BaseElement
-
- pre := ctx.options.Styles.Task.Unticked
- if e.Checked {
- pre = ctx.options.Styles.Task.Ticked
- }
-
- el = &BaseElement{
- Prefix: pre,
- Style: ctx.options.Styles.Task.StylePrimitive,
- }
-
- return el.Render(w, ctx)
-}
diff --git a/vendor/github.com/charmbracelet/glamour/ansi/templatehelper.go b/vendor/github.com/charmbracelet/glamour/ansi/templatehelper.go
deleted file mode 100644
index 2b41820..0000000
--- a/vendor/github.com/charmbracelet/glamour/ansi/templatehelper.go
+++ /dev/null
@@ -1,83 +0,0 @@
-package ansi
-
-import (
- "regexp"
- "strings"
- "text/template"
-)
-
-// TemplateFuncMap contains a few useful template helpers
-var (
- TemplateFuncMap = template.FuncMap{
- "Left": func(values ...interface{}) string {
- s := values[0].(string)
- n := values[1].(int)
- if n > len(s) {
- n = len(s)
- }
-
- return s[:n]
- },
- "Matches": func(values ...interface{}) bool {
- ok, _ := regexp.MatchString(values[1].(string), values[0].(string))
- return ok
- },
- "Mid": func(values ...interface{}) string {
- s := values[0].(string)
- l := values[1].(int)
- if l > len(s) {
- l = len(s)
- }
-
- if len(values) > 2 {
- r := values[2].(int)
- if r > len(s) {
- r = len(s)
- }
- return s[l:r]
- }
- return s[l:]
- },
- "Right": func(values ...interface{}) string {
- s := values[0].(string)
- n := len(s) - values[1].(int)
- if n < 0 {
- n = 0
- }
-
- return s[n:]
- },
- "Last": func(values ...interface{}) string {
- return values[0].([]string)[len(values[0].([]string))-1]
- },
- // strings functions
- "Compare": strings.Compare, // 1.5+ only
- "Contains": strings.Contains,
- "ContainsAny": strings.ContainsAny,
- "Count": strings.Count,
- "EqualFold": strings.EqualFold,
- "HasPrefix": strings.HasPrefix,
- "HasSuffix": strings.HasSuffix,
- "Index": strings.Index,
- "IndexAny": strings.IndexAny,
- "Join": strings.Join,
- "LastIndex": strings.LastIndex,
- "LastIndexAny": strings.LastIndexAny,
- "Repeat": strings.Repeat,
- "Replace": strings.Replace,
- "Split": strings.Split,
- "SplitAfter": strings.SplitAfter,
- "SplitAfterN": strings.SplitAfterN,
- "SplitN": strings.SplitN,
- "Title": strings.Title,
- "ToLower": strings.ToLower,
- "ToTitle": strings.ToTitle,
- "ToUpper": strings.ToUpper,
- "Trim": strings.Trim,
- "TrimLeft": strings.TrimLeft,
- "TrimPrefix": strings.TrimPrefix,
- "TrimRight": strings.TrimRight,
- "TrimSpace": strings.TrimSpace,
- "TrimSuffix": strings.TrimSuffix,
- }
-)
diff --git a/vendor/github.com/charmbracelet/glamour/examples.sh b/vendor/github.com/charmbracelet/glamour/examples.sh
deleted file mode 100644
index 48aecf6..0000000
--- a/vendor/github.com/charmbracelet/glamour/examples.sh
+++ /dev/null
@@ -1,19 +0,0 @@
-#!/bin/bash
-
-set -e
-
-for element in ./styles/examples/*.md; do
- echo "Generating screenshot for element ${element}"
- basename="`basename -s .md ${element}`"
- stylename="${basename}.style"
- filename="${basename}.png"
-
- # take screenshot
- ./termshot -o ./styles/examples/ -f "$filename" glow -s ./styles/examples/${stylename} ${element}
-
- # add border
- convert -bordercolor black -border 16x16 "./styles/examples/$filename" "./styles/examples/$filename"
-
- # optimize filesize
- pngcrush -ow "./styles/examples/$filename"
-done
diff --git a/vendor/github.com/charmbracelet/glamour/gallery.sh b/vendor/github.com/charmbracelet/glamour/gallery.sh
deleted file mode 100644
index d4769f3..0000000
--- a/vendor/github.com/charmbracelet/glamour/gallery.sh
+++ /dev/null
@@ -1,20 +0,0 @@
-#!/bin/bash
-
-for style in ./styles/*.json; do
- echo "Generating screenshot for ${style}"
- filename="`basename -s .json ${style}`.png"
-
- light=""
- if [[ $style == *"light"* ]]; then
- light="-l"
- fi
-
- # take screenshot
- ./termshot ${light} -o ./styles/gallery/ -f "$filename" glow -s ${style}
-
- # add border
- convert -bordercolor black -border 16x16 "./styles/gallery/$filename" "./styles/gallery/$filename"
-
- # optimize filesize
- pngcrush -ow "./styles/gallery/$filename"
-done
diff --git a/vendor/github.com/charmbracelet/glamour/glamour.go b/vendor/github.com/charmbracelet/glamour/glamour.go
deleted file mode 100644
index 7b4a14c..0000000
--- a/vendor/github.com/charmbracelet/glamour/glamour.go
+++ /dev/null
@@ -1,251 +0,0 @@
-package glamour
-
-import (
- "bytes"
- "encoding/json"
- "fmt"
- "io/ioutil"
- "os"
-
- "github.com/muesli/termenv"
- "github.com/yuin/goldmark"
- emoji "github.com/yuin/goldmark-emoji"
- "github.com/yuin/goldmark/extension"
- "github.com/yuin/goldmark/parser"
- "github.com/yuin/goldmark/renderer"
- "github.com/yuin/goldmark/util"
-
- "github.com/charmbracelet/glamour/ansi"
-)
-
-// A TermRendererOption sets an option on a TermRenderer.
-type TermRendererOption func(*TermRenderer) error
-
-// TermRenderer can be used to render markdown content, posing a depth of
-// customization and styles to fit your needs.
-type TermRenderer struct {
- md goldmark.Markdown
- ansiOptions ansi.Options
- buf bytes.Buffer
- renderBuf bytes.Buffer
-}
-
-// Render initializes a new TermRenderer and renders a markdown with a specific
-// style.
-func Render(in string, stylePath string) (string, error) {
- b, err := RenderBytes([]byte(in), stylePath)
- return string(b), err
-}
-
-// RenderWithEnvironmentConfig initializes a new TermRenderer and renders a
-// markdown with a specific style defined by the GLAMOUR_STYLE environment variable.
-func RenderWithEnvironmentConfig(in string) (string, error) {
- b, err := RenderBytes([]byte(in), getEnvironmentStyle())
- return string(b), err
-}
-
-// RenderBytes initializes a new TermRenderer and renders a markdown with a
-// specific style.
-func RenderBytes(in []byte, stylePath string) ([]byte, error) {
- r, err := NewTermRenderer(
- WithStylePath(stylePath),
- )
- if err != nil {
- return nil, err
- }
- return r.RenderBytes(in)
-}
-
-// NewTermRenderer returns a new TermRenderer the given options.
-func NewTermRenderer(options ...TermRendererOption) (*TermRenderer, error) {
- tr := &TermRenderer{
- md: goldmark.New(
- goldmark.WithExtensions(
- extension.GFM,
- extension.DefinitionList,
- ),
- goldmark.WithParserOptions(
- parser.WithAutoHeadingID(),
- ),
- ),
- ansiOptions: ansi.Options{
- WordWrap: 80,
- ColorProfile: termenv.TrueColor,
- },
- }
- for _, o := range options {
- if err := o(tr); err != nil {
- return nil, err
- }
- }
- ar := ansi.NewRenderer(tr.ansiOptions)
- tr.md.SetRenderer(
- renderer.NewRenderer(
- renderer.WithNodeRenderers(
- util.Prioritized(ar, 1000),
- ),
- ),
- )
- return tr, nil
-}
-
-// WithBaseURL sets a TermRenderer's base URL.
-func WithBaseURL(baseURL string) TermRendererOption {
- return func(tr *TermRenderer) error {
- tr.ansiOptions.BaseURL = baseURL
- return nil
- }
-}
-
-// WithColorProfile sets the TermRenderer's color profile
-// (TrueColor / ANSI256 / ANSI).
-func WithColorProfile(profile termenv.Profile) TermRendererOption {
- return func(tr *TermRenderer) error {
- tr.ansiOptions.ColorProfile = profile
- return nil
- }
-}
-
-// WithStandardStyle sets a TermRenderer's styles with a standard (builtin)
-// style.
-func WithStandardStyle(style string) TermRendererOption {
- return func(tr *TermRenderer) error {
- styles, err := getDefaultStyle(style)
- if err != nil {
- return err
- }
- tr.ansiOptions.Styles = *styles
- return nil
- }
-}
-
-// WithAutoStyle sets a TermRenderer's styles with either the standard dark
-// or light style, depending on the terminal's background color at run-time.
-func WithAutoStyle() TermRendererOption {
- return WithStandardStyle("auto")
-}
-
-// WithEnvironmentConfig sets a TermRenderer's styles based on the
-// GLAMOUR_STYLE environment variable.
-func WithEnvironmentConfig() TermRendererOption {
- return WithStylePath(getEnvironmentStyle())
-}
-
-// WithStylePath sets a TermRenderer's style from stylePath. stylePath is first
-// interpreted as a filename. If no such file exists, it is re-interpreted as a
-// standard style.
-func WithStylePath(stylePath string) TermRendererOption {
- return func(tr *TermRenderer) error {
- styles, err := getDefaultStyle(stylePath)
- if err != nil {
- jsonBytes, err := ioutil.ReadFile(stylePath)
- if err != nil {
- return err
- }
-
- return json.Unmarshal(jsonBytes, &tr.ansiOptions.Styles)
- }
- tr.ansiOptions.Styles = *styles
- return nil
- }
-}
-
-// WithStyles sets a TermRenderer's styles.
-func WithStyles(styles ansi.StyleConfig) TermRendererOption {
- return func(tr *TermRenderer) error {
- tr.ansiOptions.Styles = styles
- return nil
- }
-}
-
-// WithStylesFromJSONBytes sets a TermRenderer's styles by parsing styles from
-// jsonBytes.
-func WithStylesFromJSONBytes(jsonBytes []byte) TermRendererOption {
- return func(tr *TermRenderer) error {
- return json.Unmarshal(jsonBytes, &tr.ansiOptions.Styles)
- }
-}
-
-// WithStylesFromJSONFile sets a TermRenderer's styles from a JSON file.
-func WithStylesFromJSONFile(filename string) TermRendererOption {
- return func(tr *TermRenderer) error {
- jsonBytes, err := ioutil.ReadFile(filename)
- if err != nil {
- return err
- }
- return json.Unmarshal(jsonBytes, &tr.ansiOptions.Styles)
- }
-}
-
-// WithWordWrap sets a TermRenderer's word wrap.
-func WithWordWrap(wordWrap int) TermRendererOption {
- return func(tr *TermRenderer) error {
- tr.ansiOptions.WordWrap = wordWrap
- return nil
- }
-}
-
-// WithEmoji sets a TermRenderer's emoji rendering.
-func WithEmoji() TermRendererOption {
- return func(tr *TermRenderer) error {
- emoji.New().Extend(tr.md)
- return nil
- }
-}
-
-func (tr *TermRenderer) Read(b []byte) (int, error) {
- return tr.renderBuf.Read(b)
-}
-
-func (tr *TermRenderer) Write(b []byte) (int, error) {
- return tr.buf.Write(b)
-}
-
-// Close must be called after writing to TermRenderer. You can then retrieve
-// the rendered markdown by calling Read.
-func (tr *TermRenderer) Close() error {
- err := tr.md.Convert(tr.buf.Bytes(), &tr.renderBuf)
- if err != nil {
- return err
- }
-
- tr.buf.Reset()
- return nil
-}
-
-// Render returns the markdown rendered into a string.
-func (tr *TermRenderer) Render(in string) (string, error) {
- b, err := tr.RenderBytes([]byte(in))
- return string(b), err
-}
-
-// RenderBytes returns the markdown rendered into a byte slice.
-func (tr *TermRenderer) RenderBytes(in []byte) ([]byte, error) {
- var buf bytes.Buffer
- err := tr.md.Convert(in, &buf)
- return buf.Bytes(), err
-}
-
-func getEnvironmentStyle() string {
- glamourStyle := os.Getenv("GLAMOUR_STYLE")
- if len(glamourStyle) == 0 {
- glamourStyle = "auto"
- }
-
- return glamourStyle
-}
-
-func getDefaultStyle(style string) (*ansi.StyleConfig, error) {
- if style == "auto" {
- if termenv.HasDarkBackground() {
- return &DarkStyleConfig, nil
- }
- return &LightStyleConfig, nil
- }
-
- styles, ok := DefaultStyles[style]
- if !ok {
- return nil, fmt.Errorf("%s: style not found", style)
- }
- return styles, nil
-}
diff --git a/vendor/github.com/charmbracelet/glamour/go.mod b/vendor/github.com/charmbracelet/glamour/go.mod
deleted file mode 100644
index 4935c04..0000000
--- a/vendor/github.com/charmbracelet/glamour/go.mod
+++ /dev/null
@@ -1,13 +0,0 @@
-module github.com/charmbracelet/glamour
-
-go 1.13
-
-require (
- github.com/alecthomas/chroma v0.8.2
- github.com/microcosm-cc/bluemonday v1.0.6
- github.com/muesli/reflow v0.2.0
- github.com/muesli/termenv v0.8.1
- github.com/olekukonko/tablewriter v0.0.5
- github.com/yuin/goldmark v1.3.3
- github.com/yuin/goldmark-emoji v1.0.1
-)
diff --git a/vendor/github.com/charmbracelet/glamour/go.sum b/vendor/github.com/charmbracelet/glamour/go.sum
deleted file mode 100644
index 6169d59..0000000
--- a/vendor/github.com/charmbracelet/glamour/go.sum
+++ /dev/null
@@ -1,64 +0,0 @@
-github.com/alecthomas/assert v0.0.0-20170929043011-405dbfeb8e38 h1:smF2tmSOzy2Mm+0dGI2AIUHY+w0BUc+4tn40djz7+6U=
-github.com/alecthomas/assert v0.0.0-20170929043011-405dbfeb8e38/go.mod h1:r7bzyVFMNntcxPZXK3/+KdruV1H5KSlyVY0gc+NgInI=
-github.com/alecthomas/chroma v0.8.2 h1:x3zkuE2lUk/RIekyAJ3XRqSCP4zwWDfcw/YJCuCAACg=
-github.com/alecthomas/chroma v0.8.2/go.mod h1:sko8vR34/90zvl5QdcUdvzL3J8NKjAUx9va9jPuFNoM=
-github.com/alecthomas/colour v0.0.0-20160524082231-60882d9e2721 h1:JHZL0hZKJ1VENNfmXvHbgYlbUOvpzYzvy2aZU5gXVeo=
-github.com/alecthomas/colour v0.0.0-20160524082231-60882d9e2721/go.mod h1:QO9JBoKquHd+jz9nshCh40fOfO+JzsoXy8qTHF68zU0=
-github.com/alecthomas/kong v0.2.4/go.mod h1:kQOmtJgV+Lb4aj+I2LEn40cbtawdWJ9Y8QLq+lElKxE=
-github.com/alecthomas/repr v0.0.0-20180818092828-117648cd9897 h1:p9Sln00KOTlrYkxI1zYWl1QLnEqAqEARBEYa8FQnQcY=
-github.com/alecthomas/repr v0.0.0-20180818092828-117648cd9897/go.mod h1:xTS7Pm1pD1mvyM075QCDSRqH6qRLXylzS24ZTpRiSzQ=
-github.com/aymerick/douceur v0.2.0 h1:Mv+mAeH1Q+n9Fr+oyamOlAkUNPWPlA8PPGR0QAaYuPk=
-github.com/aymerick/douceur v0.2.0/go.mod h1:wlT5vV2O3h55X9m7iVYN0TBM0NH/MmbLnd30/FjWUq4=
-github.com/danwakefield/fnmatch v0.0.0-20160403171240-cbb64ac3d964 h1:y5HC9v93H5EPKqaS1UYVg1uYah5Xf51mBfIoWehClUQ=
-github.com/danwakefield/fnmatch v0.0.0-20160403171240-cbb64ac3d964/go.mod h1:Xd9hchkHSWYkEqJwUGisez3G1QY8Ryz0sdWrLPMGjLk=
-github.com/davecgh/go-spew v1.1.0/go.mod h1:J7Y8YcW2NihsgmVo/mv3lAwl/skON4iLHjSsI+c5H38=
-github.com/davecgh/go-spew v1.1.1 h1:vj9j/u1bqnvCEfJOwUhtlOARqs3+rkHYY13jYWTU97c=
-github.com/davecgh/go-spew v1.1.1/go.mod h1:J7Y8YcW2NihsgmVo/mv3lAwl/skON4iLHjSsI+c5H38=
-github.com/dlclark/regexp2 v1.2.0 h1:8sAhBGEM0dRWogWqWyQeIJnxjWO6oIjl8FKqREDsGfk=
-github.com/dlclark/regexp2 v1.2.0/go.mod h1:2pZnwuY/m+8K6iRw6wQdMtk+rH5tNGR1i55kozfMjCc=
-github.com/gorilla/css v1.0.0 h1:BQqNyPTi50JCFMTw/b67hByjMVXZRwGha6wxVGkeihY=
-github.com/gorilla/css v1.0.0/go.mod h1:Dn721qIggHpt4+EFCcTLTU/vk5ySda2ReITrtgBl60c=
-github.com/lucasb-eyer/go-colorful v1.2.0 h1:1nnpGOrhyZZuNyfu1QjKiUICQ74+3FNCN69Aj6K7nkY=
-github.com/lucasb-eyer/go-colorful v1.2.0/go.mod h1:R4dSotOR9KMtayYi1e77YzuveK+i7ruzyGqttikkLy0=
-github.com/mattn/go-colorable v0.1.6/go.mod h1:u6P/XSegPjTcexA+o6vUJrdnUu04hMope9wVRipJSqc=
-github.com/mattn/go-isatty v0.0.12 h1:wuysRhFDzyxgEmMf5xjvJ2M9dZoWAXNNr5LSBS7uHXY=
-github.com/mattn/go-isatty v0.0.12/go.mod h1:cbi8OIDigv2wuxKPP5vlRcQ1OAZbq2CE4Kysco4FUpU=
-github.com/mattn/go-runewidth v0.0.9/go.mod h1:H031xJmbD/WCDINGzjvQ9THkh0rPKHF+m2gUSrubnMI=
-github.com/mattn/go-runewidth v0.0.10 h1:CoZ3S2P7pvtP45xOtBw+/mDL2z0RKI576gSkzRRpdGg=
-github.com/mattn/go-runewidth v0.0.10/go.mod h1:RAqKPSqVFrSLVXbA8x7dzmKdmGzieGRCM46jaSJTDAk=
-github.com/microcosm-cc/bluemonday v1.0.6 h1:ZOvqHKtnx0fUpnbQm3m3zKFWE+DRC+XB1onh8JoEObE=
-github.com/microcosm-cc/bluemonday v1.0.6/go.mod h1:HOT/6NaBlR0f9XlxD3zolN6Z3N8Lp4pvhp+jLS5ihnI=
-github.com/muesli/reflow v0.2.0 h1:2o0UBJPHHH4fa2GCXU4Rg4DwOtWPMekCeyc5EWbAQp0=
-github.com/muesli/reflow v0.2.0/go.mod h1:qT22vjVmM9MIUeLgsVYe/Ye7eZlbv9dZjL3dVhUqLX8=
-github.com/muesli/termenv v0.8.1 h1:9q230czSP3DHVpkaPDXGp0TOfAwyjyYwXlUCQxQSaBk=
-github.com/muesli/termenv v0.8.1/go.mod h1:kzt/D/4a88RoheZmwfqorY3A+tnsSMA9HJC/fQSFKo0=
-github.com/olekukonko/tablewriter v0.0.5 h1:P2Ga83D34wi1o9J6Wh1mRuqd4mF/x/lgBS7N7AbDhec=
-github.com/olekukonko/tablewriter v0.0.5/go.mod h1:hPp6KlRPjbx+hW8ykQs1w3UBbZlj6HuIJcUGPhkA7kY=
-github.com/pkg/errors v0.8.1/go.mod h1:bwawxfHBFNV+L2hUp1rHADufV3IMtnDRdf1r5NINEl0=
-github.com/pkg/errors v0.9.1/go.mod h1:bwawxfHBFNV+L2hUp1rHADufV3IMtnDRdf1r5NINEl0=
-github.com/pmezard/go-difflib v1.0.0 h1:4DBwDE0NGyQoBHbLQYPwSUPoCMWR5BEzIk/f1lZbAQM=
-github.com/pmezard/go-difflib v1.0.0/go.mod h1:iKH77koFhYxTK1pcRnkKkqfTogsbg7gZNVY4sRDYZ/4=
-github.com/rivo/uniseg v0.1.0 h1:+2KBaVoUmb9XzDsrx/Ct0W/EYOSFf/nWTauy++DprtY=
-github.com/rivo/uniseg v0.1.0/go.mod h1:J6wj4VEh+S6ZtnVlnTBMWIodfgj8LQOQFoIToxlJtxc=
-github.com/sergi/go-diff v1.0.0 h1:Kpca3qRNrduNnOQeazBd0ysaKrUJiIuISHxogkT9RPQ=
-github.com/sergi/go-diff v1.0.0/go.mod h1:0CfEIISq7TuYL3j771MWULgwwjU+GofnZX9QAmXWZgo=
-github.com/stretchr/objx v0.1.0/go.mod h1:HFkY916IF+rwdDfMAkV7OtwuqBVzrE8GR6GFx+wExME=
-github.com/stretchr/testify v1.2.2/go.mod h1:a8OnRcib4nhh0OaRAV+Yts87kKdq0PP7pXfy6kDkUVs=
-github.com/stretchr/testify v1.3.0 h1:TivCn/peBQ7UY8ooIcPgZFpTNSz0Q2U6UrFlUfqbe0Q=
-github.com/stretchr/testify v1.3.0/go.mod h1:M5WIy9Dh21IEIfnGCwXGc5bZfKNJtfHm1UVUgZn+9EI=
-github.com/yuin/goldmark v1.2.1/go.mod h1:3hX8gzYuyVAZsxl0MRgGTJEmQBFcNTphYh9decYSb74=
-github.com/yuin/goldmark v1.3.3 h1:37BdQwPx8VOSic8eDSWee6QL9mRpZRm9VJp/QugNrW0=
-github.com/yuin/goldmark v1.3.3/go.mod h1:mwnBkeHKe2W/ZEtQ+71ViKU8L12m81fl3OWwC1Zlc8k=
-github.com/yuin/goldmark-emoji v1.0.1 h1:ctuWEyzGBwiucEqxzwe0SOYDXPAucOrE9NQC18Wa1os=
-github.com/yuin/goldmark-emoji v1.0.1/go.mod h1:2w1E6FEWLcDQkoTE+7HU6QF1F6SLlNGjRIBbIZQFqkQ=
-golang.org/x/net v0.0.0-20210331212208-0fccb6fa2b5c h1:KHUzaHIpjWVlVVNh65G3hhuj3KB1HnjY6Cq5cTvRQT8=
-golang.org/x/net v0.0.0-20210331212208-0fccb6fa2b5c/go.mod h1:p54w0d4576C0XHj96bSt6lcn1PtDYWL6XObtHCRCNQM=
-golang.org/x/sys v0.0.0-20200116001909-b77594299b42/go.mod h1:h1NjWce9XRLGQEsW7wpKNCjG9DtNlClVuFLEZdDNbEs=
-golang.org/x/sys v0.0.0-20200223170610-d5e6a3e2c0ae/go.mod h1:h1NjWce9XRLGQEsW7wpKNCjG9DtNlClVuFLEZdDNbEs=
-golang.org/x/sys v0.0.0-20200413165638-669c56c373c4/go.mod h1:h1NjWce9XRLGQEsW7wpKNCjG9DtNlClVuFLEZdDNbEs=
-golang.org/x/sys v0.0.0-20201119102817-f84b799fce68/go.mod h1:h1NjWce9XRLGQEsW7wpKNCjG9DtNlClVuFLEZdDNbEs=
-golang.org/x/sys v0.0.0-20210330210617-4fbd30eecc44 h1:Bli41pIlzTzf3KEY06n+xnzK/BESIg2ze4Pgfh/aI8c=
-golang.org/x/sys v0.0.0-20210330210617-4fbd30eecc44/go.mod h1:h1NjWce9XRLGQEsW7wpKNCjG9DtNlClVuFLEZdDNbEs=
-golang.org/x/term v0.0.0-20201126162022-7de9c90e9dd1/go.mod h1:bj7SfCRtBDWHUb9snDiAeCFNEtKQo2Wmx5Cou7ajbmo=
-golang.org/x/text v0.3.3/go.mod h1:5Zoc/QRtKVWzQhOtBMvqHzDpF6irO9z98xDceosuGiQ=
-golang.org/x/tools v0.0.0-20180917221912-90fa682c2a6e/go.mod h1:n7NCudcB/nEzxVGmLbDWY5pfWTLqBcC2KZ6jyYvM4mQ=
diff --git a/vendor/github.com/charmbracelet/glamour/styles.go b/vendor/github.com/charmbracelet/glamour/styles.go
deleted file mode 100644
index caac382..0000000
--- a/vendor/github.com/charmbracelet/glamour/styles.go
+++ /dev/null
@@ -1,660 +0,0 @@
-package glamour
-
-//go:generate go run ./internal/generate-style-json
-
-import (
- "github.com/charmbracelet/glamour/ansi"
-)
-
-var (
- // ASCIIStyleConfig uses only ASCII characters.
- ASCIIStyleConfig = ansi.StyleConfig{
- Document: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- BlockPrefix: "\n",
- BlockSuffix: "\n",
- },
- Margin: uintPtr(2),
- },
- BlockQuote: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{},
- Indent: uintPtr(1),
- IndentToken: stringPtr("| "),
- },
- Paragraph: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{},
- },
- List: ansi.StyleList{
- StyleBlock: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{},
- },
- LevelIndent: 4,
- },
- Heading: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- BlockSuffix: "\n",
- },
- },
- H1: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- Prefix: "# ",
- },
- },
- H2: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- Prefix: "## ",
- },
- },
- H3: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- Prefix: "### ",
- },
- },
- H4: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- Prefix: "#### ",
- },
- },
- H5: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- Prefix: "##### ",
- },
- },
- H6: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- Prefix: "###### ",
- },
- },
- Strikethrough: ansi.StylePrimitive{
- BlockPrefix: "~~",
- BlockSuffix: "~~",
- },
- Emph: ansi.StylePrimitive{
- BlockPrefix: "*",
- BlockSuffix: "*",
- },
- Strong: ansi.StylePrimitive{
- BlockPrefix: "**",
- BlockSuffix: "**",
- },
- HorizontalRule: ansi.StylePrimitive{
- Format: "\n--------\n",
- },
- Item: ansi.StylePrimitive{
- BlockPrefix: "• ",
- },
- Enumeration: ansi.StylePrimitive{
- BlockPrefix: ". ",
- },
- Task: ansi.StyleTask{
- Ticked: "[x] ",
- Unticked: "[ ] ",
- },
- ImageText: ansi.StylePrimitive{
- Format: "Image: {{.text}} →",
- },
- Code: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- BlockPrefix: "`",
- BlockSuffix: "`",
- },
- },
- CodeBlock: ansi.StyleCodeBlock{
- StyleBlock: ansi.StyleBlock{
- Margin: uintPtr(2),
- },
- },
- Table: ansi.StyleTable{
- CenterSeparator: stringPtr("+"),
- ColumnSeparator: stringPtr("|"),
- RowSeparator: stringPtr("-"),
- },
- DefinitionDescription: ansi.StylePrimitive{
- BlockPrefix: "\n* ",
- },
- }
-
- // DarkStyleConfig is the default dark style.
- DarkStyleConfig = ansi.StyleConfig{
- Document: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- BlockPrefix: "\n",
- BlockSuffix: "\n",
- Color: stringPtr("252"),
- },
- Margin: uintPtr(2),
- },
- BlockQuote: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{},
- Indent: uintPtr(1),
- IndentToken: stringPtr("│ "),
- },
- List: ansi.StyleList{
- LevelIndent: 2,
- },
- Heading: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- BlockSuffix: "\n",
- Color: stringPtr("39"),
- Bold: boolPtr(true),
- },
- },
- H1: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- Prefix: " ",
- Suffix: " ",
- Color: stringPtr("228"),
- BackgroundColor: stringPtr("63"),
- Bold: boolPtr(true),
- },
- },
- H2: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- Prefix: "## ",
- },
- },
- H3: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- Prefix: "### ",
- },
- },
- H4: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- Prefix: "#### ",
- },
- },
- H5: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- Prefix: "##### ",
- },
- },
- H6: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- Prefix: "###### ",
- Color: stringPtr("35"),
- Bold: boolPtr(false),
- },
- },
- Strikethrough: ansi.StylePrimitive{
- CrossedOut: boolPtr(true),
- },
- Emph: ansi.StylePrimitive{
- Italic: boolPtr(true),
- },
- Strong: ansi.StylePrimitive{
- Bold: boolPtr(true),
- },
- HorizontalRule: ansi.StylePrimitive{
- Color: stringPtr("240"),
- Format: "\n--------\n",
- },
- Item: ansi.StylePrimitive{
- BlockPrefix: "• ",
- },
- Enumeration: ansi.StylePrimitive{
- BlockPrefix: ". ",
- },
- Task: ansi.StyleTask{
- StylePrimitive: ansi.StylePrimitive{},
- Ticked: "[✓] ",
- Unticked: "[ ] ",
- },
- Link: ansi.StylePrimitive{
- Color: stringPtr("30"),
- Underline: boolPtr(true),
- },
- LinkText: ansi.StylePrimitive{
- Color: stringPtr("35"),
- Bold: boolPtr(true),
- },
- Image: ansi.StylePrimitive{
- Color: stringPtr("212"),
- Underline: boolPtr(true),
- },
- ImageText: ansi.StylePrimitive{
- Color: stringPtr("243"),
- Format: "Image: {{.text}} →",
- },
- Code: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- Prefix: " ",
- Suffix: " ",
- Color: stringPtr("203"),
- BackgroundColor: stringPtr("236"),
- },
- },
- CodeBlock: ansi.StyleCodeBlock{
- StyleBlock: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- Color: stringPtr("244"),
- },
- Margin: uintPtr(2),
- },
- Chroma: &ansi.Chroma{
- Text: ansi.StylePrimitive{
- Color: stringPtr("#C4C4C4"),
- },
- Error: ansi.StylePrimitive{
- Color: stringPtr("#F1F1F1"),
- BackgroundColor: stringPtr("#F05B5B"),
- },
- Comment: ansi.StylePrimitive{
- Color: stringPtr("#676767"),
- },
- CommentPreproc: ansi.StylePrimitive{
- Color: stringPtr("#FF875F"),
- },
- Keyword: ansi.StylePrimitive{
- Color: stringPtr("#00AAFF"),
- },
- KeywordReserved: ansi.StylePrimitive{
- Color: stringPtr("#FF5FD2"),
- },
- KeywordNamespace: ansi.StylePrimitive{
- Color: stringPtr("#FF5F87"),
- },
- KeywordType: ansi.StylePrimitive{
- Color: stringPtr("#6E6ED8"),
- },
- Operator: ansi.StylePrimitive{
- Color: stringPtr("#EF8080"),
- },
- Punctuation: ansi.StylePrimitive{
- Color: stringPtr("#E8E8A8"),
- },
- Name: ansi.StylePrimitive{
- Color: stringPtr("#C4C4C4"),
- },
- NameBuiltin: ansi.StylePrimitive{
- Color: stringPtr("#FF8EC7"),
- },
- NameTag: ansi.StylePrimitive{
- Color: stringPtr("#B083EA"),
- },
- NameAttribute: ansi.StylePrimitive{
- Color: stringPtr("#7A7AE6"),
- },
- NameClass: ansi.StylePrimitive{
- Color: stringPtr("#F1F1F1"),
- Underline: boolPtr(true),
- Bold: boolPtr(true),
- },
- NameDecorator: ansi.StylePrimitive{
- Color: stringPtr("#FFFF87"),
- },
- NameFunction: ansi.StylePrimitive{
- Color: stringPtr("#00D787"),
- },
- LiteralNumber: ansi.StylePrimitive{
- Color: stringPtr("#6EEFC0"),
- },
- LiteralString: ansi.StylePrimitive{
- Color: stringPtr("#C69669"),
- },
- LiteralStringEscape: ansi.StylePrimitive{
- Color: stringPtr("#AFFFD7"),
- },
- GenericDeleted: ansi.StylePrimitive{
- Color: stringPtr("#FD5B5B"),
- },
- GenericEmph: ansi.StylePrimitive{
- Italic: boolPtr(true),
- },
- GenericInserted: ansi.StylePrimitive{
- Color: stringPtr("#00D787"),
- },
- GenericStrong: ansi.StylePrimitive{
- Bold: boolPtr(true),
- },
- GenericSubheading: ansi.StylePrimitive{
- Color: stringPtr("#777777"),
- },
- Background: ansi.StylePrimitive{
- BackgroundColor: stringPtr("#373737"),
- },
- },
- },
- Table: ansi.StyleTable{
- StyleBlock: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{},
- },
- CenterSeparator: stringPtr("┼"),
- ColumnSeparator: stringPtr("│"),
- RowSeparator: stringPtr("─"),
- },
- DefinitionDescription: ansi.StylePrimitive{
- BlockPrefix: "\n🠶 ",
- },
- }
-
- // LightStyleConfig is the default light style.
- LightStyleConfig = ansi.StyleConfig{
- Document: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- BlockPrefix: "\n",
- BlockSuffix: "\n",
- Color: stringPtr("234"),
- },
- Margin: uintPtr(2),
- },
- BlockQuote: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{},
- Indent: uintPtr(1),
- IndentToken: stringPtr("│ "),
- },
- List: ansi.StyleList{
- LevelIndent: 2,
- },
- Heading: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- BlockSuffix: "\n",
- Color: stringPtr("27"),
- Bold: boolPtr(true),
- },
- },
- H1: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- Prefix: " ",
- Suffix: " ",
- Color: stringPtr("228"),
- BackgroundColor: stringPtr("63"),
- Bold: boolPtr(true),
- },
- },
- H2: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- Prefix: "## ",
- },
- },
- H3: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- Prefix: "### ",
- },
- },
- H4: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- Prefix: "#### ",
- },
- },
- H5: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- Prefix: "##### ",
- },
- },
- H6: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- Prefix: "###### ",
- Bold: boolPtr(false),
- },
- },
- Strikethrough: ansi.StylePrimitive{
- CrossedOut: boolPtr(true),
- },
- Emph: ansi.StylePrimitive{
- Italic: boolPtr(true),
- },
- Strong: ansi.StylePrimitive{
- Bold: boolPtr(true),
- },
- HorizontalRule: ansi.StylePrimitive{
- Color: stringPtr("249"),
- Format: "\n--------\n",
- },
- Item: ansi.StylePrimitive{
- BlockPrefix: "• ",
- },
- Enumeration: ansi.StylePrimitive{
- BlockPrefix: ". ",
- },
- Task: ansi.StyleTask{
- StylePrimitive: ansi.StylePrimitive{},
- Ticked: "[✓] ",
- Unticked: "[ ] ",
- },
- Link: ansi.StylePrimitive{
- Color: stringPtr("36"),
- Underline: boolPtr(true),
- },
- LinkText: ansi.StylePrimitive{
- Color: stringPtr("29"),
- Bold: boolPtr(true),
- },
- Image: ansi.StylePrimitive{
- Color: stringPtr("205"),
- Underline: boolPtr(true),
- },
- ImageText: ansi.StylePrimitive{
- Color: stringPtr("243"),
- Format: "Image: {{.text}} →",
- },
- Code: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- Prefix: " ",
- Suffix: " ",
- Color: stringPtr("203"),
- BackgroundColor: stringPtr("254"),
- },
- },
- CodeBlock: ansi.StyleCodeBlock{
- StyleBlock: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- Color: stringPtr("242"),
- },
- Margin: uintPtr(2),
- },
- Chroma: &ansi.Chroma{
- Text: ansi.StylePrimitive{
- Color: stringPtr("#2A2A2A"),
- },
- Error: ansi.StylePrimitive{
- Color: stringPtr("#F1F1F1"),
- BackgroundColor: stringPtr("#FF5555"),
- },
- Comment: ansi.StylePrimitive{
- Color: stringPtr("#8D8D8D"),
- },
- CommentPreproc: ansi.StylePrimitive{
- Color: stringPtr("#FF875F"),
- },
- Keyword: ansi.StylePrimitive{
- Color: stringPtr("#279EFC"),
- },
- KeywordReserved: ansi.StylePrimitive{
- Color: stringPtr("#FF5FD2"),
- },
- KeywordNamespace: ansi.StylePrimitive{
- Color: stringPtr("#FB406F"),
- },
- KeywordType: ansi.StylePrimitive{
- Color: stringPtr("#7049C2"),
- },
- Operator: ansi.StylePrimitive{
- Color: stringPtr("#FF2626"),
- },
- Punctuation: ansi.StylePrimitive{
- Color: stringPtr("#FA7878"),
- },
- NameBuiltin: ansi.StylePrimitive{
- Color: stringPtr("#0A1BB1"),
- },
- NameTag: ansi.StylePrimitive{
- Color: stringPtr("#581290"),
- },
- NameAttribute: ansi.StylePrimitive{
- Color: stringPtr("#8362CB"),
- },
- NameClass: ansi.StylePrimitive{
- Color: stringPtr("#212121"),
- Underline: boolPtr(true),
- Bold: boolPtr(true),
- },
- NameConstant: ansi.StylePrimitive{
- Color: stringPtr("#581290"),
- },
- NameDecorator: ansi.StylePrimitive{
- Color: stringPtr("#A3A322"),
- },
- NameFunction: ansi.StylePrimitive{
- Color: stringPtr("#019F57"),
- },
- LiteralNumber: ansi.StylePrimitive{
- Color: stringPtr("#22CCAE"),
- },
- LiteralString: ansi.StylePrimitive{
- Color: stringPtr("#7E5B38"),
- },
- LiteralStringEscape: ansi.StylePrimitive{
- Color: stringPtr("#00AEAE"),
- },
- GenericDeleted: ansi.StylePrimitive{
- Color: stringPtr("#FD5B5B"),
- },
- GenericEmph: ansi.StylePrimitive{
- Italic: boolPtr(true),
- },
- GenericInserted: ansi.StylePrimitive{
- Color: stringPtr("#00D787"),
- },
- GenericStrong: ansi.StylePrimitive{
- Bold: boolPtr(true),
- },
- GenericSubheading: ansi.StylePrimitive{
- Color: stringPtr("#777777"),
- },
- Background: ansi.StylePrimitive{
- BackgroundColor: stringPtr("#373737"),
- },
- },
- },
- Table: ansi.StyleTable{
- StyleBlock: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{},
- },
- CenterSeparator: stringPtr("┼"),
- ColumnSeparator: stringPtr("│"),
- RowSeparator: stringPtr("─"),
- },
- DefinitionDescription: ansi.StylePrimitive{
- BlockPrefix: "\n🠶 ",
- },
- }
-
- // NoTTYStyleConfig is the default notty style.
- NoTTYStyleConfig = ansi.StyleConfig{
- Document: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- BlockPrefix: "\n",
- BlockSuffix: "\n",
- },
- Margin: uintPtr(2),
- },
- BlockQuote: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{},
- Indent: uintPtr(1),
- IndentToken: stringPtr("│ "),
- },
- Paragraph: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{},
- },
- List: ansi.StyleList{
- StyleBlock: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{},
- },
- LevelIndent: 4,
- },
- Heading: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- BlockSuffix: "\n",
- },
- },
- H1: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- Prefix: "# ",
- },
- },
- H2: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- Prefix: "## ",
- },
- },
- H3: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- Prefix: "### ",
- },
- },
- H4: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- Prefix: "#### ",
- },
- },
- H5: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- Prefix: "##### ",
- },
- },
- H6: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- Prefix: "###### ",
- },
- },
- Strikethrough: ansi.StylePrimitive{
- BlockPrefix: "~~",
- BlockSuffix: "~~",
- },
- Emph: ansi.StylePrimitive{
- BlockPrefix: "*",
- BlockSuffix: "*",
- },
- Strong: ansi.StylePrimitive{
- BlockPrefix: "**",
- BlockSuffix: "**",
- },
- HorizontalRule: ansi.StylePrimitive{
- Format: "\n--------\n",
- },
- Item: ansi.StylePrimitive{
- BlockPrefix: "• ",
- },
- Enumeration: ansi.StylePrimitive{
- BlockPrefix: ". ",
- },
- Task: ansi.StyleTask{
- Ticked: "[✓] ",
- Unticked: "[ ] ",
- },
- ImageText: ansi.StylePrimitive{
- Format: "Image: {{.text}} →",
- },
- Code: ansi.StyleBlock{
- StylePrimitive: ansi.StylePrimitive{
- BlockPrefix: "`",
- BlockSuffix: "`",
- },
- },
- CodeBlock: ansi.StyleCodeBlock{
- StyleBlock: ansi.StyleBlock{
- Margin: uintPtr(2),
- },
- },
- Table: ansi.StyleTable{
- CenterSeparator: stringPtr("┼"),
- ColumnSeparator: stringPtr("│"),
- RowSeparator: stringPtr("─"),
- },
- DefinitionDescription: ansi.StylePrimitive{
- BlockPrefix: "\n🠶 ",
- },
- }
-
- // DefaultStyles are the default styles.
- DefaultStyles = map[string]*ansi.StyleConfig{
- "ascii": &ASCIIStyleConfig,
- "dark": &DarkStyleConfig,
- "light": &LightStyleConfig,
- "notty": &NoTTYStyleConfig,
- }
-)
-
-func boolPtr(b bool) *bool { return &b }
-func stringPtr(s string) *string { return &s }
-func uintPtr(u uint) *uint { return &u }
diff --git a/vendor/github.com/cpuguy83/go-md2man/v2/LICENSE.md b/vendor/github.com/cpuguy83/go-md2man/v2/LICENSE.md
deleted file mode 100644
index 1cade6c..0000000
--- a/vendor/github.com/cpuguy83/go-md2man/v2/LICENSE.md
+++ /dev/null
@@ -1,21 +0,0 @@
-The MIT License (MIT)
-
-Copyright (c) 2014 Brian Goff
-
-Permission is hereby granted, free of charge, to any person obtaining a copy
-of this software and associated documentation files (the "Software"), to deal
-in the Software without restriction, including without limitation the rights
-to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
-copies of the Software, and to permit persons to whom the Software is
-furnished to do so, subject to the following conditions:
-
-The above copyright notice and this permission notice shall be included in all
-copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
-IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
-FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
-AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
-LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
-OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
-SOFTWARE.
diff --git a/vendor/github.com/cpuguy83/go-md2man/v2/md2man/md2man.go b/vendor/github.com/cpuguy83/go-md2man/v2/md2man/md2man.go
deleted file mode 100644
index b480056..0000000
--- a/vendor/github.com/cpuguy83/go-md2man/v2/md2man/md2man.go
+++ /dev/null
@@ -1,14 +0,0 @@
-package md2man
-
-import (
- "github.com/russross/blackfriday/v2"
-)
-
-// Render converts a markdown document into a roff formatted document.
-func Render(doc []byte) []byte {
- renderer := NewRoffRenderer()
-
- return blackfriday.Run(doc,
- []blackfriday.Option{blackfriday.WithRenderer(renderer),
- blackfriday.WithExtensions(renderer.GetExtensions())}...)
-}
diff --git a/vendor/github.com/cpuguy83/go-md2man/v2/md2man/roff.go b/vendor/github.com/cpuguy83/go-md2man/v2/md2man/roff.go
deleted file mode 100644
index be2b343..0000000
--- a/vendor/github.com/cpuguy83/go-md2man/v2/md2man/roff.go
+++ /dev/null
@@ -1,336 +0,0 @@
-package md2man
-
-import (
- "fmt"
- "io"
- "os"
- "strings"
-
- "github.com/russross/blackfriday/v2"
-)
-
-// roffRenderer implements the blackfriday.Renderer interface for creating
-// roff format (manpages) from markdown text
-type roffRenderer struct {
- extensions blackfriday.Extensions
- listCounters []int
- firstHeader bool
- firstDD bool
- listDepth int
-}
-
-const (
- titleHeader = ".TH "
- topLevelHeader = "\n\n.SH "
- secondLevelHdr = "\n.SH "
- otherHeader = "\n.SS "
- crTag = "\n"
- emphTag = "\\fI"
- emphCloseTag = "\\fP"
- strongTag = "\\fB"
- strongCloseTag = "\\fP"
- breakTag = "\n.br\n"
- paraTag = "\n.PP\n"
- hruleTag = "\n.ti 0\n\\l'\\n(.lu'\n"
- linkTag = "\n\\[la]"
- linkCloseTag = "\\[ra]"
- codespanTag = "\\fB\\fC"
- codespanCloseTag = "\\fR"
- codeTag = "\n.PP\n.RS\n\n.nf\n"
- codeCloseTag = "\n.fi\n.RE\n"
- quoteTag = "\n.PP\n.RS\n"
- quoteCloseTag = "\n.RE\n"
- listTag = "\n.RS\n"
- listCloseTag = "\n.RE\n"
- dtTag = "\n.TP\n"
- dd2Tag = "\n"
- tableStart = "\n.TS\nallbox;\n"
- tableEnd = ".TE\n"
- tableCellStart = "T{\n"
- tableCellEnd = "\nT}\n"
-)
-
-// NewRoffRenderer creates a new blackfriday Renderer for generating roff documents
-// from markdown
-func NewRoffRenderer() *roffRenderer { // nolint: golint
- var extensions blackfriday.Extensions
-
- extensions |= blackfriday.NoIntraEmphasis
- extensions |= blackfriday.Tables
- extensions |= blackfriday.FencedCode
- extensions |= blackfriday.SpaceHeadings
- extensions |= blackfriday.Footnotes
- extensions |= blackfriday.Titleblock
- extensions |= blackfriday.DefinitionLists
- return &roffRenderer{
- extensions: extensions,
- }
-}
-
-// GetExtensions returns the list of extensions used by this renderer implementation
-func (r *roffRenderer) GetExtensions() blackfriday.Extensions {
- return r.extensions
-}
-
-// RenderHeader handles outputting the header at document start
-func (r *roffRenderer) RenderHeader(w io.Writer, ast *blackfriday.Node) {
- // disable hyphenation
- out(w, ".nh\n")
-}
-
-// RenderFooter handles outputting the footer at the document end; the roff
-// renderer has no footer information
-func (r *roffRenderer) RenderFooter(w io.Writer, ast *blackfriday.Node) {
-}
-
-// RenderNode is called for each node in a markdown document; based on the node
-// type the equivalent roff output is sent to the writer
-func (r *roffRenderer) RenderNode(w io.Writer, node *blackfriday.Node, entering bool) blackfriday.WalkStatus {
-
- var walkAction = blackfriday.GoToNext
-
- switch node.Type {
- case blackfriday.Text:
- escapeSpecialChars(w, node.Literal)
- case blackfriday.Softbreak:
- out(w, crTag)
- case blackfriday.Hardbreak:
- out(w, breakTag)
- case blackfriday.Emph:
- if entering {
- out(w, emphTag)
- } else {
- out(w, emphCloseTag)
- }
- case blackfriday.Strong:
- if entering {
- out(w, strongTag)
- } else {
- out(w, strongCloseTag)
- }
- case blackfriday.Link:
- if !entering {
- out(w, linkTag+string(node.LinkData.Destination)+linkCloseTag)
- }
- case blackfriday.Image:
- // ignore images
- walkAction = blackfriday.SkipChildren
- case blackfriday.Code:
- out(w, codespanTag)
- escapeSpecialChars(w, node.Literal)
- out(w, codespanCloseTag)
- case blackfriday.Document:
- break
- case blackfriday.Paragraph:
- // roff .PP markers break lists
- if r.listDepth > 0 {
- return blackfriday.GoToNext
- }
- if entering {
- out(w, paraTag)
- } else {
- out(w, crTag)
- }
- case blackfriday.BlockQuote:
- if entering {
- out(w, quoteTag)
- } else {
- out(w, quoteCloseTag)
- }
- case blackfriday.Heading:
- r.handleHeading(w, node, entering)
- case blackfriday.HorizontalRule:
- out(w, hruleTag)
- case blackfriday.List:
- r.handleList(w, node, entering)
- case blackfriday.Item:
- r.handleItem(w, node, entering)
- case blackfriday.CodeBlock:
- out(w, codeTag)
- escapeSpecialChars(w, node.Literal)
- out(w, codeCloseTag)
- case blackfriday.Table:
- r.handleTable(w, node, entering)
- case blackfriday.TableHead:
- case blackfriday.TableBody:
- case blackfriday.TableRow:
- // no action as cell entries do all the nroff formatting
- return blackfriday.GoToNext
- case blackfriday.TableCell:
- r.handleTableCell(w, node, entering)
- case blackfriday.HTMLSpan:
- // ignore other HTML tags
- default:
- fmt.Fprintln(os.Stderr, "WARNING: go-md2man does not handle node type "+node.Type.String())
- }
- return walkAction
-}
-
-func (r *roffRenderer) handleHeading(w io.Writer, node *blackfriday.Node, entering bool) {
- if entering {
- switch node.Level {
- case 1:
- if !r.firstHeader {
- out(w, titleHeader)
- r.firstHeader = true
- break
- }
- out(w, topLevelHeader)
- case 2:
- out(w, secondLevelHdr)
- default:
- out(w, otherHeader)
- }
- }
-}
-
-func (r *roffRenderer) handleList(w io.Writer, node *blackfriday.Node, entering bool) {
- openTag := listTag
- closeTag := listCloseTag
- if node.ListFlags&blackfriday.ListTypeDefinition != 0 {
- // tags for definition lists handled within Item node
- openTag = ""
- closeTag = ""
- }
- if entering {
- r.listDepth++
- if node.ListFlags&blackfriday.ListTypeOrdered != 0 {
- r.listCounters = append(r.listCounters, 1)
- }
- out(w, openTag)
- } else {
- if node.ListFlags&blackfriday.ListTypeOrdered != 0 {
- r.listCounters = r.listCounters[:len(r.listCounters)-1]
- }
- out(w, closeTag)
- r.listDepth--
- }
-}
-
-func (r *roffRenderer) handleItem(w io.Writer, node *blackfriday.Node, entering bool) {
- if entering {
- if node.ListFlags&blackfriday.ListTypeOrdered != 0 {
- out(w, fmt.Sprintf(".IP \"%3d.\" 5\n", r.listCounters[len(r.listCounters)-1]))
- r.listCounters[len(r.listCounters)-1]++
- } else if node.ListFlags&blackfriday.ListTypeTerm != 0 {
- // DT (definition term): line just before DD (see below).
- out(w, dtTag)
- r.firstDD = true
- } else if node.ListFlags&blackfriday.ListTypeDefinition != 0 {
- // DD (definition description): line that starts with ": ".
- //
- // We have to distinguish between the first DD and the
- // subsequent ones, as there should be no vertical
- // whitespace between the DT and the first DD.
- if r.firstDD {
- r.firstDD = false
- } else {
- out(w, dd2Tag)
- }
- } else {
- out(w, ".IP \\(bu 2\n")
- }
- } else {
- out(w, "\n")
- }
-}
-
-func (r *roffRenderer) handleTable(w io.Writer, node *blackfriday.Node, entering bool) {
- if entering {
- out(w, tableStart)
- // call walker to count cells (and rows?) so format section can be produced
- columns := countColumns(node)
- out(w, strings.Repeat("l ", columns)+"\n")
- out(w, strings.Repeat("l ", columns)+".\n")
- } else {
- out(w, tableEnd)
- }
-}
-
-func (r *roffRenderer) handleTableCell(w io.Writer, node *blackfriday.Node, entering bool) {
- if entering {
- var start string
- if node.Prev != nil && node.Prev.Type == blackfriday.TableCell {
- start = "\t"
- }
- if node.IsHeader {
- start += codespanTag
- } else if nodeLiteralSize(node) > 30 {
- start += tableCellStart
- }
- out(w, start)
- } else {
- var end string
- if node.IsHeader {
- end = codespanCloseTag
- } else if nodeLiteralSize(node) > 30 {
- end = tableCellEnd
- }
- if node.Next == nil && end != tableCellEnd {
- // Last cell: need to carriage return if we are at the end of the
- // header row and content isn't wrapped in a "tablecell"
- end += crTag
- }
- out(w, end)
- }
-}
-
-func nodeLiteralSize(node *blackfriday.Node) int {
- total := 0
- for n := node.FirstChild; n != nil; n = n.FirstChild {
- total += len(n.Literal)
- }
- return total
-}
-
-// because roff format requires knowing the column count before outputting any table
-// data we need to walk a table tree and count the columns
-func countColumns(node *blackfriday.Node) int {
- var columns int
-
- node.Walk(func(node *blackfriday.Node, entering bool) blackfriday.WalkStatus {
- switch node.Type {
- case blackfriday.TableRow:
- if !entering {
- return blackfriday.Terminate
- }
- case blackfriday.TableCell:
- if entering {
- columns++
- }
- default:
- }
- return blackfriday.GoToNext
- })
- return columns
-}
-
-func out(w io.Writer, output string) {
- io.WriteString(w, output) // nolint: errcheck
-}
-
-func escapeSpecialChars(w io.Writer, text []byte) {
- for i := 0; i < len(text); i++ {
- // escape initial apostrophe or period
- if len(text) >= 1 && (text[0] == '\'' || text[0] == '.') {
- out(w, "\\&")
- }
-
- // directly copy normal characters
- org := i
-
- for i < len(text) && text[i] != '\\' {
- i++
- }
- if i > org {
- w.Write(text[org:i]) // nolint: errcheck
- }
-
- // escape a character
- if i >= len(text) {
- break
- }
-
- w.Write([]byte{'\\', text[i]}) // nolint: errcheck
- }
-}
diff --git a/vendor/github.com/danwakefield/fnmatch/.gitignore b/vendor/github.com/danwakefield/fnmatch/.gitignore
deleted file mode 100644
index daf913b..0000000
--- a/vendor/github.com/danwakefield/fnmatch/.gitignore
+++ /dev/null
@@ -1,24 +0,0 @@
-# Compiled Object files, Static and Dynamic libs (Shared Objects)
-*.o
-*.a
-*.so
-
-# Folders
-_obj
-_test
-
-# Architecture specific extensions/prefixes
-*.[568vq]
-[568vq].out
-
-*.cgo1.go
-*.cgo2.c
-_cgo_defun.c
-_cgo_gotypes.go
-_cgo_export.*
-
-_testmain.go
-
-*.exe
-*.test
-*.prof
diff --git a/vendor/github.com/danwakefield/fnmatch/LICENSE b/vendor/github.com/danwakefield/fnmatch/LICENSE
deleted file mode 100644
index 0dc9851..0000000
--- a/vendor/github.com/danwakefield/fnmatch/LICENSE
+++ /dev/null
@@ -1,23 +0,0 @@
-Copyright (c) 2016, Daniel Wakefield
-All rights reserved.
-
-Redistribution and use in source and binary forms, with or without
-modification, are permitted provided that the following conditions are met:
-
-* Redistributions of source code must retain the above copyright notice, this
- list of conditions and the following disclaimer.
-
-* Redistributions in binary form must reproduce the above copyright notice,
- this list of conditions and the following disclaimer in the documentation
- and/or other materials provided with the distribution.
-
-THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
-AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
-IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
-DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE
-FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
-DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
-SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
-CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY,
-OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
-OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
diff --git a/vendor/github.com/danwakefield/fnmatch/README.md b/vendor/github.com/danwakefield/fnmatch/README.md
deleted file mode 100644
index b8d7156..0000000
--- a/vendor/github.com/danwakefield/fnmatch/README.md
+++ /dev/null
@@ -1,4 +0,0 @@
-# fnmatch
-Updated clone of kballards golang fnmatch gist (https://gist.github.com/kballard/272720)
-
-
diff --git a/vendor/github.com/danwakefield/fnmatch/fnmatch.go b/vendor/github.com/danwakefield/fnmatch/fnmatch.go
deleted file mode 100644
index 07ac7b3..0000000
--- a/vendor/github.com/danwakefield/fnmatch/fnmatch.go
+++ /dev/null
@@ -1,219 +0,0 @@
-// Provide string-matching based on fnmatch.3
-package fnmatch
-
-// There are a few issues that I believe to be bugs, but this implementation is
-// based as closely as possible on BSD fnmatch. These bugs are present in the
-// source of BSD fnmatch, and so are replicated here. The issues are as follows:
-//
-// * FNM_PERIOD is no longer observed after the first * in a pattern
-// This only applies to matches done with FNM_PATHNAME as well
-// * FNM_PERIOD doesn't apply to ranges. According to the documentation,
-// a period must be matched explicitly, but a range will match it too
-
-import (
- "unicode"
- "unicode/utf8"
-)
-
-const (
- FNM_NOESCAPE = (1 << iota)
- FNM_PATHNAME
- FNM_PERIOD
-
- FNM_LEADING_DIR
- FNM_CASEFOLD
-
- FNM_IGNORECASE = FNM_CASEFOLD
- FNM_FILE_NAME = FNM_PATHNAME
-)
-
-func unpackRune(str *string) rune {
- rune, size := utf8.DecodeRuneInString(*str)
- *str = (*str)[size:]
- return rune
-}
-
-// Matches the pattern against the string, with the given flags,
-// and returns true if the match is successful.
-// This function should match fnmatch.3 as closely as possible.
-func Match(pattern, s string, flags int) bool {
- // The implementation for this function was patterned after the BSD fnmatch.c
- // source found at http://src.gnu-darwin.org/src/contrib/csup/fnmatch.c.html
- noescape := (flags&FNM_NOESCAPE != 0)
- pathname := (flags&FNM_PATHNAME != 0)
- period := (flags&FNM_PERIOD != 0)
- leadingdir := (flags&FNM_LEADING_DIR != 0)
- casefold := (flags&FNM_CASEFOLD != 0)
- // the following is some bookkeeping that the original fnmatch.c implementation did not do
- // We are forced to do this because we're not keeping indexes into C strings but rather
- // processing utf8-encoded strings. Use a custom unpacker to maintain our state for us
- sAtStart := true
- sLastAtStart := true
- sLastSlash := false
- sLastUnpacked := rune(0)
- unpackS := func() rune {
- sLastSlash = (sLastUnpacked == '/')
- sLastUnpacked = unpackRune(&s)
- sLastAtStart = sAtStart
- sAtStart = false
- return sLastUnpacked
- }
- for len(pattern) > 0 {
- c := unpackRune(&pattern)
- switch c {
- case '?':
- if len(s) == 0 {
- return false
- }
- sc := unpackS()
- if pathname && sc == '/' {
- return false
- }
- if period && sc == '.' && (sLastAtStart || (pathname && sLastSlash)) {
- return false
- }
- case '*':
- // collapse multiple *'s
- // don't use unpackRune here, the only char we care to detect is ASCII
- for len(pattern) > 0 && pattern[0] == '*' {
- pattern = pattern[1:]
- }
- if period && s[0] == '.' && (sAtStart || (pathname && sLastUnpacked == '/')) {
- return false
- }
- // optimize for patterns with * at end or before /
- if len(pattern) == 0 {
- if pathname {
- return leadingdir || (strchr(s, '/') == -1)
- } else {
- return true
- }
- return !(pathname && strchr(s, '/') >= 0)
- } else if pathname && pattern[0] == '/' {
- offset := strchr(s, '/')
- if offset == -1 {
- return false
- } else {
- // we already know our pattern and string have a /, skip past it
- s = s[offset:] // use unpackS here to maintain our bookkeeping state
- unpackS()
- pattern = pattern[1:] // we know / is one byte long
- break
- }
- }
- // general case, recurse
- for test := s; len(test) > 0; unpackRune(&test) {
- // I believe the (flags &^ FNM_PERIOD) is a bug when FNM_PATHNAME is specified
- // but this follows exactly from how fnmatch.c implements it
- if Match(pattern, test, (flags &^ FNM_PERIOD)) {
- return true
- } else if pathname && test[0] == '/' {
- break
- }
- }
- return false
- case '[':
- if len(s) == 0 {
- return false
- }
- if pathname && s[0] == '/' {
- return false
- }
- sc := unpackS()
- if !rangematch(&pattern, sc, flags) {
- return false
- }
- case '\\':
- if !noescape {
- if len(pattern) > 0 {
- c = unpackRune(&pattern)
- }
- }
- fallthrough
- default:
- if len(s) == 0 {
- return false
- }
- sc := unpackS()
- switch {
- case sc == c:
- case casefold && unicode.ToLower(sc) == unicode.ToLower(c):
- default:
- return false
- }
- }
- }
- return len(s) == 0 || (leadingdir && s[0] == '/')
-}
-
-func rangematch(pattern *string, test rune, flags int) bool {
- if len(*pattern) == 0 {
- return false
- }
- casefold := (flags&FNM_CASEFOLD != 0)
- noescape := (flags&FNM_NOESCAPE != 0)
- if casefold {
- test = unicode.ToLower(test)
- }
- var negate, matched bool
- if (*pattern)[0] == '^' || (*pattern)[0] == '!' {
- negate = true
- (*pattern) = (*pattern)[1:]
- }
- for !matched && len(*pattern) > 1 && (*pattern)[0] != ']' {
- c := unpackRune(pattern)
- if !noescape && c == '\\' {
- if len(*pattern) > 1 {
- c = unpackRune(pattern)
- } else {
- return false
- }
- }
- if casefold {
- c = unicode.ToLower(c)
- }
- if (*pattern)[0] == '-' && len(*pattern) > 1 && (*pattern)[1] != ']' {
- unpackRune(pattern) // skip the -
- c2 := unpackRune(pattern)
- if !noescape && c2 == '\\' {
- if len(*pattern) > 0 {
- c2 = unpackRune(pattern)
- } else {
- return false
- }
- }
- if casefold {
- c2 = unicode.ToLower(c2)
- }
- // this really should be more intelligent, but it looks like
- // fnmatch.c does simple int comparisons, therefore we will as well
- if c <= test && test <= c2 {
- matched = true
- }
- } else if c == test {
- matched = true
- }
- }
- // skip past the rest of the pattern
- ok := false
- for !ok && len(*pattern) > 0 {
- c := unpackRune(pattern)
- if c == '\\' && len(*pattern) > 0 {
- unpackRune(pattern)
- } else if c == ']' {
- ok = true
- }
- }
- return ok && matched != negate
-}
-
-// define strchr because strings.Index() seems a bit overkill
-// returns the index of c in s, or -1 if there is no match
-func strchr(s string, c rune) int {
- for i, sc := range s {
- if sc == c {
- return i
- }
- }
- return -1
-}
diff --git a/vendor/github.com/davecgh/go-spew/LICENSE b/vendor/github.com/davecgh/go-spew/LICENSE
deleted file mode 100644
index bc52e96..0000000
--- a/vendor/github.com/davecgh/go-spew/LICENSE
+++ /dev/null
@@ -1,15 +0,0 @@
-ISC License
-
-Copyright (c) 2012-2016 Dave Collins
-
-Permission to use, copy, modify, and/or distribute this software for any
-purpose with or without fee is hereby granted, provided that the above
-copyright notice and this permission notice appear in all copies.
-
-THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES
-WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF
-MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR
-ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
-WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN
-ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF
-OR IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
diff --git a/vendor/github.com/davecgh/go-spew/spew/bypass.go b/vendor/github.com/davecgh/go-spew/spew/bypass.go
deleted file mode 100644
index 7929947..0000000
--- a/vendor/github.com/davecgh/go-spew/spew/bypass.go
+++ /dev/null
@@ -1,145 +0,0 @@
-// Copyright (c) 2015-2016 Dave Collins
-//
-// Permission to use, copy, modify, and distribute this software for any
-// purpose with or without fee is hereby granted, provided that the above
-// copyright notice and this permission notice appear in all copies.
-//
-// THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES
-// WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF
-// MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR
-// ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
-// WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN
-// ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF
-// OR IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
-
-// NOTE: Due to the following build constraints, this file will only be compiled
-// when the code is not running on Google App Engine, compiled by GopherJS, and
-// "-tags safe" is not added to the go build command line. The "disableunsafe"
-// tag is deprecated and thus should not be used.
-// Go versions prior to 1.4 are disabled because they use a different layout
-// for interfaces which make the implementation of unsafeReflectValue more complex.
-// +build !js,!appengine,!safe,!disableunsafe,go1.4
-
-package spew
-
-import (
- "reflect"
- "unsafe"
-)
-
-const (
- // UnsafeDisabled is a build-time constant which specifies whether or
- // not access to the unsafe package is available.
- UnsafeDisabled = false
-
- // ptrSize is the size of a pointer on the current arch.
- ptrSize = unsafe.Sizeof((*byte)(nil))
-)
-
-type flag uintptr
-
-var (
- // flagRO indicates whether the value field of a reflect.Value
- // is read-only.
- flagRO flag
-
- // flagAddr indicates whether the address of the reflect.Value's
- // value may be taken.
- flagAddr flag
-)
-
-// flagKindMask holds the bits that make up the kind
-// part of the flags field. In all the supported versions,
-// it is in the lower 5 bits.
-const flagKindMask = flag(0x1f)
-
-// Different versions of Go have used different
-// bit layouts for the flags type. This table
-// records the known combinations.
-var okFlags = []struct {
- ro, addr flag
-}{{
- // From Go 1.4 to 1.5
- ro: 1 << 5,
- addr: 1 << 7,
-}, {
- // Up to Go tip.
- ro: 1<<5 | 1<<6,
- addr: 1 << 8,
-}}
-
-var flagValOffset = func() uintptr {
- field, ok := reflect.TypeOf(reflect.Value{}).FieldByName("flag")
- if !ok {
- panic("reflect.Value has no flag field")
- }
- return field.Offset
-}()
-
-// flagField returns a pointer to the flag field of a reflect.Value.
-func flagField(v *reflect.Value) *flag {
- return (*flag)(unsafe.Pointer(uintptr(unsafe.Pointer(v)) + flagValOffset))
-}
-
-// unsafeReflectValue converts the passed reflect.Value into a one that bypasses
-// the typical safety restrictions preventing access to unaddressable and
-// unexported data. It works by digging the raw pointer to the underlying
-// value out of the protected value and generating a new unprotected (unsafe)
-// reflect.Value to it.
-//
-// This allows us to check for implementations of the Stringer and error
-// interfaces to be used for pretty printing ordinarily unaddressable and
-// inaccessible values such as unexported struct fields.
-func unsafeReflectValue(v reflect.Value) reflect.Value {
- if !v.IsValid() || (v.CanInterface() && v.CanAddr()) {
- return v
- }
- flagFieldPtr := flagField(&v)
- *flagFieldPtr &^= flagRO
- *flagFieldPtr |= flagAddr
- return v
-}
-
-// Sanity checks against future reflect package changes
-// to the type or semantics of the Value.flag field.
-func init() {
- field, ok := reflect.TypeOf(reflect.Value{}).FieldByName("flag")
- if !ok {
- panic("reflect.Value has no flag field")
- }
- if field.Type.Kind() != reflect.TypeOf(flag(0)).Kind() {
- panic("reflect.Value flag field has changed kind")
- }
- type t0 int
- var t struct {
- A t0
- // t0 will have flagEmbedRO set.
- t0
- // a will have flagStickyRO set
- a t0
- }
- vA := reflect.ValueOf(t).FieldByName("A")
- va := reflect.ValueOf(t).FieldByName("a")
- vt0 := reflect.ValueOf(t).FieldByName("t0")
-
- // Infer flagRO from the difference between the flags
- // for the (otherwise identical) fields in t.
- flagPublic := *flagField(&vA)
- flagWithRO := *flagField(&va) | *flagField(&vt0)
- flagRO = flagPublic ^ flagWithRO
-
- // Infer flagAddr from the difference between a value
- // taken from a pointer and not.
- vPtrA := reflect.ValueOf(&t).Elem().FieldByName("A")
- flagNoPtr := *flagField(&vA)
- flagPtr := *flagField(&vPtrA)
- flagAddr = flagNoPtr ^ flagPtr
-
- // Check that the inferred flags tally with one of the known versions.
- for _, f := range okFlags {
- if flagRO == f.ro && flagAddr == f.addr {
- return
- }
- }
- panic("reflect.Value read-only flag has changed semantics")
-}
diff --git a/vendor/github.com/davecgh/go-spew/spew/bypasssafe.go b/vendor/github.com/davecgh/go-spew/spew/bypasssafe.go
deleted file mode 100644
index 205c28d..0000000
--- a/vendor/github.com/davecgh/go-spew/spew/bypasssafe.go
+++ /dev/null
@@ -1,38 +0,0 @@
-// Copyright (c) 2015-2016 Dave Collins
-//
-// Permission to use, copy, modify, and distribute this software for any
-// purpose with or without fee is hereby granted, provided that the above
-// copyright notice and this permission notice appear in all copies.
-//
-// THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES
-// WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF
-// MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR
-// ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
-// WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN
-// ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF
-// OR IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
-
-// NOTE: Due to the following build constraints, this file will only be compiled
-// when the code is running on Google App Engine, compiled by GopherJS, or
-// "-tags safe" is added to the go build command line. The "disableunsafe"
-// tag is deprecated and thus should not be used.
-// +build js appengine safe disableunsafe !go1.4
-
-package spew
-
-import "reflect"
-
-const (
- // UnsafeDisabled is a build-time constant which specifies whether or
- // not access to the unsafe package is available.
- UnsafeDisabled = true
-)
-
-// unsafeReflectValue typically converts the passed reflect.Value into a one
-// that bypasses the typical safety restrictions preventing access to
-// unaddressable and unexported data. However, doing this relies on access to
-// the unsafe package. This is a stub version which simply returns the passed
-// reflect.Value when the unsafe package is not available.
-func unsafeReflectValue(v reflect.Value) reflect.Value {
- return v
-}
diff --git a/vendor/github.com/davecgh/go-spew/spew/common.go b/vendor/github.com/davecgh/go-spew/spew/common.go
deleted file mode 100644
index 1be8ce9..0000000
--- a/vendor/github.com/davecgh/go-spew/spew/common.go
+++ /dev/null
@@ -1,341 +0,0 @@
-/*
- * Copyright (c) 2013-2016 Dave Collins
- *
- * Permission to use, copy, modify, and distribute this software for any
- * purpose with or without fee is hereby granted, provided that the above
- * copyright notice and this permission notice appear in all copies.
- *
- * THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES
- * WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF
- * MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR
- * ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
- * WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN
- * ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF
- * OR IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
- */
-
-package spew
-
-import (
- "bytes"
- "fmt"
- "io"
- "reflect"
- "sort"
- "strconv"
-)
-
-// Some constants in the form of bytes to avoid string overhead. This mirrors
-// the technique used in the fmt package.
-var (
- panicBytes = []byte("(PANIC=")
- plusBytes = []byte("+")
- iBytes = []byte("i")
- trueBytes = []byte("true")
- falseBytes = []byte("false")
- interfaceBytes = []byte("(interface {})")
- commaNewlineBytes = []byte(",\n")
- newlineBytes = []byte("\n")
- openBraceBytes = []byte("{")
- openBraceNewlineBytes = []byte("{\n")
- closeBraceBytes = []byte("}")
- asteriskBytes = []byte("*")
- colonBytes = []byte(":")
- colonSpaceBytes = []byte(": ")
- openParenBytes = []byte("(")
- closeParenBytes = []byte(")")
- spaceBytes = []byte(" ")
- pointerChainBytes = []byte("->")
- nilAngleBytes = []byte("")
- maxNewlineBytes = []byte("\n")
- maxShortBytes = []byte("")
- circularBytes = []byte("")
- circularShortBytes = []byte("")
- invalidAngleBytes = []byte("")
- openBracketBytes = []byte("[")
- closeBracketBytes = []byte("]")
- percentBytes = []byte("%")
- precisionBytes = []byte(".")
- openAngleBytes = []byte("<")
- closeAngleBytes = []byte(">")
- openMapBytes = []byte("map[")
- closeMapBytes = []byte("]")
- lenEqualsBytes = []byte("len=")
- capEqualsBytes = []byte("cap=")
-)
-
-// hexDigits is used to map a decimal value to a hex digit.
-var hexDigits = "0123456789abcdef"
-
-// catchPanic handles any panics that might occur during the handleMethods
-// calls.
-func catchPanic(w io.Writer, v reflect.Value) {
- if err := recover(); err != nil {
- w.Write(panicBytes)
- fmt.Fprintf(w, "%v", err)
- w.Write(closeParenBytes)
- }
-}
-
-// handleMethods attempts to call the Error and String methods on the underlying
-// type the passed reflect.Value represents and outputes the result to Writer w.
-//
-// It handles panics in any called methods by catching and displaying the error
-// as the formatted value.
-func handleMethods(cs *ConfigState, w io.Writer, v reflect.Value) (handled bool) {
- // We need an interface to check if the type implements the error or
- // Stringer interface. However, the reflect package won't give us an
- // interface on certain things like unexported struct fields in order
- // to enforce visibility rules. We use unsafe, when it's available,
- // to bypass these restrictions since this package does not mutate the
- // values.
- if !v.CanInterface() {
- if UnsafeDisabled {
- return false
- }
-
- v = unsafeReflectValue(v)
- }
-
- // Choose whether or not to do error and Stringer interface lookups against
- // the base type or a pointer to the base type depending on settings.
- // Technically calling one of these methods with a pointer receiver can
- // mutate the value, however, types which choose to satisify an error or
- // Stringer interface with a pointer receiver should not be mutating their
- // state inside these interface methods.
- if !cs.DisablePointerMethods && !UnsafeDisabled && !v.CanAddr() {
- v = unsafeReflectValue(v)
- }
- if v.CanAddr() {
- v = v.Addr()
- }
-
- // Is it an error or Stringer?
- switch iface := v.Interface().(type) {
- case error:
- defer catchPanic(w, v)
- if cs.ContinueOnMethod {
- w.Write(openParenBytes)
- w.Write([]byte(iface.Error()))
- w.Write(closeParenBytes)
- w.Write(spaceBytes)
- return false
- }
-
- w.Write([]byte(iface.Error()))
- return true
-
- case fmt.Stringer:
- defer catchPanic(w, v)
- if cs.ContinueOnMethod {
- w.Write(openParenBytes)
- w.Write([]byte(iface.String()))
- w.Write(closeParenBytes)
- w.Write(spaceBytes)
- return false
- }
- w.Write([]byte(iface.String()))
- return true
- }
- return false
-}
-
-// printBool outputs a boolean value as true or false to Writer w.
-func printBool(w io.Writer, val bool) {
- if val {
- w.Write(trueBytes)
- } else {
- w.Write(falseBytes)
- }
-}
-
-// printInt outputs a signed integer value to Writer w.
-func printInt(w io.Writer, val int64, base int) {
- w.Write([]byte(strconv.FormatInt(val, base)))
-}
-
-// printUint outputs an unsigned integer value to Writer w.
-func printUint(w io.Writer, val uint64, base int) {
- w.Write([]byte(strconv.FormatUint(val, base)))
-}
-
-// printFloat outputs a floating point value using the specified precision,
-// which is expected to be 32 or 64bit, to Writer w.
-func printFloat(w io.Writer, val float64, precision int) {
- w.Write([]byte(strconv.FormatFloat(val, 'g', -1, precision)))
-}
-
-// printComplex outputs a complex value using the specified float precision
-// for the real and imaginary parts to Writer w.
-func printComplex(w io.Writer, c complex128, floatPrecision int) {
- r := real(c)
- w.Write(openParenBytes)
- w.Write([]byte(strconv.FormatFloat(r, 'g', -1, floatPrecision)))
- i := imag(c)
- if i >= 0 {
- w.Write(plusBytes)
- }
- w.Write([]byte(strconv.FormatFloat(i, 'g', -1, floatPrecision)))
- w.Write(iBytes)
- w.Write(closeParenBytes)
-}
-
-// printHexPtr outputs a uintptr formatted as hexadecimal with a leading '0x'
-// prefix to Writer w.
-func printHexPtr(w io.Writer, p uintptr) {
- // Null pointer.
- num := uint64(p)
- if num == 0 {
- w.Write(nilAngleBytes)
- return
- }
-
- // Max uint64 is 16 bytes in hex + 2 bytes for '0x' prefix
- buf := make([]byte, 18)
-
- // It's simpler to construct the hex string right to left.
- base := uint64(16)
- i := len(buf) - 1
- for num >= base {
- buf[i] = hexDigits[num%base]
- num /= base
- i--
- }
- buf[i] = hexDigits[num]
-
- // Add '0x' prefix.
- i--
- buf[i] = 'x'
- i--
- buf[i] = '0'
-
- // Strip unused leading bytes.
- buf = buf[i:]
- w.Write(buf)
-}
-
-// valuesSorter implements sort.Interface to allow a slice of reflect.Value
-// elements to be sorted.
-type valuesSorter struct {
- values []reflect.Value
- strings []string // either nil or same len and values
- cs *ConfigState
-}
-
-// newValuesSorter initializes a valuesSorter instance, which holds a set of
-// surrogate keys on which the data should be sorted. It uses flags in
-// ConfigState to decide if and how to populate those surrogate keys.
-func newValuesSorter(values []reflect.Value, cs *ConfigState) sort.Interface {
- vs := &valuesSorter{values: values, cs: cs}
- if canSortSimply(vs.values[0].Kind()) {
- return vs
- }
- if !cs.DisableMethods {
- vs.strings = make([]string, len(values))
- for i := range vs.values {
- b := bytes.Buffer{}
- if !handleMethods(cs, &b, vs.values[i]) {
- vs.strings = nil
- break
- }
- vs.strings[i] = b.String()
- }
- }
- if vs.strings == nil && cs.SpewKeys {
- vs.strings = make([]string, len(values))
- for i := range vs.values {
- vs.strings[i] = Sprintf("%#v", vs.values[i].Interface())
- }
- }
- return vs
-}
-
-// canSortSimply tests whether a reflect.Kind is a primitive that can be sorted
-// directly, or whether it should be considered for sorting by surrogate keys
-// (if the ConfigState allows it).
-func canSortSimply(kind reflect.Kind) bool {
- // This switch parallels valueSortLess, except for the default case.
- switch kind {
- case reflect.Bool:
- return true
- case reflect.Int8, reflect.Int16, reflect.Int32, reflect.Int64, reflect.Int:
- return true
- case reflect.Uint8, reflect.Uint16, reflect.Uint32, reflect.Uint64, reflect.Uint:
- return true
- case reflect.Float32, reflect.Float64:
- return true
- case reflect.String:
- return true
- case reflect.Uintptr:
- return true
- case reflect.Array:
- return true
- }
- return false
-}
-
-// Len returns the number of values in the slice. It is part of the
-// sort.Interface implementation.
-func (s *valuesSorter) Len() int {
- return len(s.values)
-}
-
-// Swap swaps the values at the passed indices. It is part of the
-// sort.Interface implementation.
-func (s *valuesSorter) Swap(i, j int) {
- s.values[i], s.values[j] = s.values[j], s.values[i]
- if s.strings != nil {
- s.strings[i], s.strings[j] = s.strings[j], s.strings[i]
- }
-}
-
-// valueSortLess returns whether the first value should sort before the second
-// value. It is used by valueSorter.Less as part of the sort.Interface
-// implementation.
-func valueSortLess(a, b reflect.Value) bool {
- switch a.Kind() {
- case reflect.Bool:
- return !a.Bool() && b.Bool()
- case reflect.Int8, reflect.Int16, reflect.Int32, reflect.Int64, reflect.Int:
- return a.Int() < b.Int()
- case reflect.Uint8, reflect.Uint16, reflect.Uint32, reflect.Uint64, reflect.Uint:
- return a.Uint() < b.Uint()
- case reflect.Float32, reflect.Float64:
- return a.Float() < b.Float()
- case reflect.String:
- return a.String() < b.String()
- case reflect.Uintptr:
- return a.Uint() < b.Uint()
- case reflect.Array:
- // Compare the contents of both arrays.
- l := a.Len()
- for i := 0; i < l; i++ {
- av := a.Index(i)
- bv := b.Index(i)
- if av.Interface() == bv.Interface() {
- continue
- }
- return valueSortLess(av, bv)
- }
- }
- return a.String() < b.String()
-}
-
-// Less returns whether the value at index i should sort before the
-// value at index j. It is part of the sort.Interface implementation.
-func (s *valuesSorter) Less(i, j int) bool {
- if s.strings == nil {
- return valueSortLess(s.values[i], s.values[j])
- }
- return s.strings[i] < s.strings[j]
-}
-
-// sortValues is a sort function that handles both native types and any type that
-// can be converted to error or Stringer. Other inputs are sorted according to
-// their Value.String() value to ensure display stability.
-func sortValues(values []reflect.Value, cs *ConfigState) {
- if len(values) == 0 {
- return
- }
- sort.Sort(newValuesSorter(values, cs))
-}
diff --git a/vendor/github.com/davecgh/go-spew/spew/config.go b/vendor/github.com/davecgh/go-spew/spew/config.go
deleted file mode 100644
index 2e3d22f..0000000
--- a/vendor/github.com/davecgh/go-spew/spew/config.go
+++ /dev/null
@@ -1,306 +0,0 @@
-/*
- * Copyright (c) 2013-2016 Dave Collins
- *
- * Permission to use, copy, modify, and distribute this software for any
- * purpose with or without fee is hereby granted, provided that the above
- * copyright notice and this permission notice appear in all copies.
- *
- * THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES
- * WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF
- * MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR
- * ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
- * WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN
- * ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF
- * OR IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
- */
-
-package spew
-
-import (
- "bytes"
- "fmt"
- "io"
- "os"
-)
-
-// ConfigState houses the configuration options used by spew to format and
-// display values. There is a global instance, Config, that is used to control
-// all top-level Formatter and Dump functionality. Each ConfigState instance
-// provides methods equivalent to the top-level functions.
-//
-// The zero value for ConfigState provides no indentation. You would typically
-// want to set it to a space or a tab.
-//
-// Alternatively, you can use NewDefaultConfig to get a ConfigState instance
-// with default settings. See the documentation of NewDefaultConfig for default
-// values.
-type ConfigState struct {
- // Indent specifies the string to use for each indentation level. The
- // global config instance that all top-level functions use set this to a
- // single space by default. If you would like more indentation, you might
- // set this to a tab with "\t" or perhaps two spaces with " ".
- Indent string
-
- // MaxDepth controls the maximum number of levels to descend into nested
- // data structures. The default, 0, means there is no limit.
- //
- // NOTE: Circular data structures are properly detected, so it is not
- // necessary to set this value unless you specifically want to limit deeply
- // nested data structures.
- MaxDepth int
-
- // DisableMethods specifies whether or not error and Stringer interfaces are
- // invoked for types that implement them.
- DisableMethods bool
-
- // DisablePointerMethods specifies whether or not to check for and invoke
- // error and Stringer interfaces on types which only accept a pointer
- // receiver when the current type is not a pointer.
- //
- // NOTE: This might be an unsafe action since calling one of these methods
- // with a pointer receiver could technically mutate the value, however,
- // in practice, types which choose to satisify an error or Stringer
- // interface with a pointer receiver should not be mutating their state
- // inside these interface methods. As a result, this option relies on
- // access to the unsafe package, so it will not have any effect when
- // running in environments without access to the unsafe package such as
- // Google App Engine or with the "safe" build tag specified.
- DisablePointerMethods bool
-
- // DisablePointerAddresses specifies whether to disable the printing of
- // pointer addresses. This is useful when diffing data structures in tests.
- DisablePointerAddresses bool
-
- // DisableCapacities specifies whether to disable the printing of capacities
- // for arrays, slices, maps and channels. This is useful when diffing
- // data structures in tests.
- DisableCapacities bool
-
- // ContinueOnMethod specifies whether or not recursion should continue once
- // a custom error or Stringer interface is invoked. The default, false,
- // means it will print the results of invoking the custom error or Stringer
- // interface and return immediately instead of continuing to recurse into
- // the internals of the data type.
- //
- // NOTE: This flag does not have any effect if method invocation is disabled
- // via the DisableMethods or DisablePointerMethods options.
- ContinueOnMethod bool
-
- // SortKeys specifies map keys should be sorted before being printed. Use
- // this to have a more deterministic, diffable output. Note that only
- // native types (bool, int, uint, floats, uintptr and string) and types
- // that support the error or Stringer interfaces (if methods are
- // enabled) are supported, with other types sorted according to the
- // reflect.Value.String() output which guarantees display stability.
- SortKeys bool
-
- // SpewKeys specifies that, as a last resort attempt, map keys should
- // be spewed to strings and sorted by those strings. This is only
- // considered if SortKeys is true.
- SpewKeys bool
-}
-
-// Config is the active configuration of the top-level functions.
-// The configuration can be changed by modifying the contents of spew.Config.
-var Config = ConfigState{Indent: " "}
-
-// Errorf is a wrapper for fmt.Errorf that treats each argument as if it were
-// passed with a Formatter interface returned by c.NewFormatter. It returns
-// the formatted string as a value that satisfies error. See NewFormatter
-// for formatting details.
-//
-// This function is shorthand for the following syntax:
-//
-// fmt.Errorf(format, c.NewFormatter(a), c.NewFormatter(b))
-func (c *ConfigState) Errorf(format string, a ...interface{}) (err error) {
- return fmt.Errorf(format, c.convertArgs(a)...)
-}
-
-// Fprint is a wrapper for fmt.Fprint that treats each argument as if it were
-// passed with a Formatter interface returned by c.NewFormatter. It returns
-// the number of bytes written and any write error encountered. See
-// NewFormatter for formatting details.
-//
-// This function is shorthand for the following syntax:
-//
-// fmt.Fprint(w, c.NewFormatter(a), c.NewFormatter(b))
-func (c *ConfigState) Fprint(w io.Writer, a ...interface{}) (n int, err error) {
- return fmt.Fprint(w, c.convertArgs(a)...)
-}
-
-// Fprintf is a wrapper for fmt.Fprintf that treats each argument as if it were
-// passed with a Formatter interface returned by c.NewFormatter. It returns
-// the number of bytes written and any write error encountered. See
-// NewFormatter for formatting details.
-//
-// This function is shorthand for the following syntax:
-//
-// fmt.Fprintf(w, format, c.NewFormatter(a), c.NewFormatter(b))
-func (c *ConfigState) Fprintf(w io.Writer, format string, a ...interface{}) (n int, err error) {
- return fmt.Fprintf(w, format, c.convertArgs(a)...)
-}
-
-// Fprintln is a wrapper for fmt.Fprintln that treats each argument as if it
-// passed with a Formatter interface returned by c.NewFormatter. See
-// NewFormatter for formatting details.
-//
-// This function is shorthand for the following syntax:
-//
-// fmt.Fprintln(w, c.NewFormatter(a), c.NewFormatter(b))
-func (c *ConfigState) Fprintln(w io.Writer, a ...interface{}) (n int, err error) {
- return fmt.Fprintln(w, c.convertArgs(a)...)
-}
-
-// Print is a wrapper for fmt.Print that treats each argument as if it were
-// passed with a Formatter interface returned by c.NewFormatter. It returns
-// the number of bytes written and any write error encountered. See
-// NewFormatter for formatting details.
-//
-// This function is shorthand for the following syntax:
-//
-// fmt.Print(c.NewFormatter(a), c.NewFormatter(b))
-func (c *ConfigState) Print(a ...interface{}) (n int, err error) {
- return fmt.Print(c.convertArgs(a)...)
-}
-
-// Printf is a wrapper for fmt.Printf that treats each argument as if it were
-// passed with a Formatter interface returned by c.NewFormatter. It returns
-// the number of bytes written and any write error encountered. See
-// NewFormatter for formatting details.
-//
-// This function is shorthand for the following syntax:
-//
-// fmt.Printf(format, c.NewFormatter(a), c.NewFormatter(b))
-func (c *ConfigState) Printf(format string, a ...interface{}) (n int, err error) {
- return fmt.Printf(format, c.convertArgs(a)...)
-}
-
-// Println is a wrapper for fmt.Println that treats each argument as if it were
-// passed with a Formatter interface returned by c.NewFormatter. It returns
-// the number of bytes written and any write error encountered. See
-// NewFormatter for formatting details.
-//
-// This function is shorthand for the following syntax:
-//
-// fmt.Println(c.NewFormatter(a), c.NewFormatter(b))
-func (c *ConfigState) Println(a ...interface{}) (n int, err error) {
- return fmt.Println(c.convertArgs(a)...)
-}
-
-// Sprint is a wrapper for fmt.Sprint that treats each argument as if it were
-// passed with a Formatter interface returned by c.NewFormatter. It returns
-// the resulting string. See NewFormatter for formatting details.
-//
-// This function is shorthand for the following syntax:
-//
-// fmt.Sprint(c.NewFormatter(a), c.NewFormatter(b))
-func (c *ConfigState) Sprint(a ...interface{}) string {
- return fmt.Sprint(c.convertArgs(a)...)
-}
-
-// Sprintf is a wrapper for fmt.Sprintf that treats each argument as if it were
-// passed with a Formatter interface returned by c.NewFormatter. It returns
-// the resulting string. See NewFormatter for formatting details.
-//
-// This function is shorthand for the following syntax:
-//
-// fmt.Sprintf(format, c.NewFormatter(a), c.NewFormatter(b))
-func (c *ConfigState) Sprintf(format string, a ...interface{}) string {
- return fmt.Sprintf(format, c.convertArgs(a)...)
-}
-
-// Sprintln is a wrapper for fmt.Sprintln that treats each argument as if it
-// were passed with a Formatter interface returned by c.NewFormatter. It
-// returns the resulting string. See NewFormatter for formatting details.
-//
-// This function is shorthand for the following syntax:
-//
-// fmt.Sprintln(c.NewFormatter(a), c.NewFormatter(b))
-func (c *ConfigState) Sprintln(a ...interface{}) string {
- return fmt.Sprintln(c.convertArgs(a)...)
-}
-
-/*
-NewFormatter returns a custom formatter that satisfies the fmt.Formatter
-interface. As a result, it integrates cleanly with standard fmt package
-printing functions. The formatter is useful for inline printing of smaller data
-types similar to the standard %v format specifier.
-
-The custom formatter only responds to the %v (most compact), %+v (adds pointer
-addresses), %#v (adds types), and %#+v (adds types and pointer addresses) verb
-combinations. Any other verbs such as %x and %q will be sent to the the
-standard fmt package for formatting. In addition, the custom formatter ignores
-the width and precision arguments (however they will still work on the format
-specifiers not handled by the custom formatter).
-
-Typically this function shouldn't be called directly. It is much easier to make
-use of the custom formatter by calling one of the convenience functions such as
-c.Printf, c.Println, or c.Printf.
-*/
-func (c *ConfigState) NewFormatter(v interface{}) fmt.Formatter {
- return newFormatter(c, v)
-}
-
-// Fdump formats and displays the passed arguments to io.Writer w. It formats
-// exactly the same as Dump.
-func (c *ConfigState) Fdump(w io.Writer, a ...interface{}) {
- fdump(c, w, a...)
-}
-
-/*
-Dump displays the passed parameters to standard out with newlines, customizable
-indentation, and additional debug information such as complete types and all
-pointer addresses used to indirect to the final value. It provides the
-following features over the built-in printing facilities provided by the fmt
-package:
-
- * Pointers are dereferenced and followed
- * Circular data structures are detected and handled properly
- * Custom Stringer/error interfaces are optionally invoked, including
- on unexported types
- * Custom types which only implement the Stringer/error interfaces via
- a pointer receiver are optionally invoked when passing non-pointer
- variables
- * Byte arrays and slices are dumped like the hexdump -C command which
- includes offsets, byte values in hex, and ASCII output
-
-The configuration options are controlled by modifying the public members
-of c. See ConfigState for options documentation.
-
-See Fdump if you would prefer dumping to an arbitrary io.Writer or Sdump to
-get the formatted result as a string.
-*/
-func (c *ConfigState) Dump(a ...interface{}) {
- fdump(c, os.Stdout, a...)
-}
-
-// Sdump returns a string with the passed arguments formatted exactly the same
-// as Dump.
-func (c *ConfigState) Sdump(a ...interface{}) string {
- var buf bytes.Buffer
- fdump(c, &buf, a...)
- return buf.String()
-}
-
-// convertArgs accepts a slice of arguments and returns a slice of the same
-// length with each argument converted to a spew Formatter interface using
-// the ConfigState associated with s.
-func (c *ConfigState) convertArgs(args []interface{}) (formatters []interface{}) {
- formatters = make([]interface{}, len(args))
- for index, arg := range args {
- formatters[index] = newFormatter(c, arg)
- }
- return formatters
-}
-
-// NewDefaultConfig returns a ConfigState with the following default settings.
-//
-// Indent: " "
-// MaxDepth: 0
-// DisableMethods: false
-// DisablePointerMethods: false
-// ContinueOnMethod: false
-// SortKeys: false
-func NewDefaultConfig() *ConfigState {
- return &ConfigState{Indent: " "}
-}
diff --git a/vendor/github.com/davecgh/go-spew/spew/doc.go b/vendor/github.com/davecgh/go-spew/spew/doc.go
deleted file mode 100644
index aacaac6..0000000
--- a/vendor/github.com/davecgh/go-spew/spew/doc.go
+++ /dev/null
@@ -1,211 +0,0 @@
-/*
- * Copyright (c) 2013-2016 Dave Collins
- *
- * Permission to use, copy, modify, and distribute this software for any
- * purpose with or without fee is hereby granted, provided that the above
- * copyright notice and this permission notice appear in all copies.
- *
- * THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES
- * WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF
- * MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR
- * ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
- * WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN
- * ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF
- * OR IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
- */
-
-/*
-Package spew implements a deep pretty printer for Go data structures to aid in
-debugging.
-
-A quick overview of the additional features spew provides over the built-in
-printing facilities for Go data types are as follows:
-
- * Pointers are dereferenced and followed
- * Circular data structures are detected and handled properly
- * Custom Stringer/error interfaces are optionally invoked, including
- on unexported types
- * Custom types which only implement the Stringer/error interfaces via
- a pointer receiver are optionally invoked when passing non-pointer
- variables
- * Byte arrays and slices are dumped like the hexdump -C command which
- includes offsets, byte values in hex, and ASCII output (only when using
- Dump style)
-
-There are two different approaches spew allows for dumping Go data structures:
-
- * Dump style which prints with newlines, customizable indentation,
- and additional debug information such as types and all pointer addresses
- used to indirect to the final value
- * A custom Formatter interface that integrates cleanly with the standard fmt
- package and replaces %v, %+v, %#v, and %#+v to provide inline printing
- similar to the default %v while providing the additional functionality
- outlined above and passing unsupported format verbs such as %x and %q
- along to fmt
-
-Quick Start
-
-This section demonstrates how to quickly get started with spew. See the
-sections below for further details on formatting and configuration options.
-
-To dump a variable with full newlines, indentation, type, and pointer
-information use Dump, Fdump, or Sdump:
- spew.Dump(myVar1, myVar2, ...)
- spew.Fdump(someWriter, myVar1, myVar2, ...)
- str := spew.Sdump(myVar1, myVar2, ...)
-
-Alternatively, if you would prefer to use format strings with a compacted inline
-printing style, use the convenience wrappers Printf, Fprintf, etc with
-%v (most compact), %+v (adds pointer addresses), %#v (adds types), or
-%#+v (adds types and pointer addresses):
- spew.Printf("myVar1: %v -- myVar2: %+v", myVar1, myVar2)
- spew.Printf("myVar3: %#v -- myVar4: %#+v", myVar3, myVar4)
- spew.Fprintf(someWriter, "myVar1: %v -- myVar2: %+v", myVar1, myVar2)
- spew.Fprintf(someWriter, "myVar3: %#v -- myVar4: %#+v", myVar3, myVar4)
-
-Configuration Options
-
-Configuration of spew is handled by fields in the ConfigState type. For
-convenience, all of the top-level functions use a global state available
-via the spew.Config global.
-
-It is also possible to create a ConfigState instance that provides methods
-equivalent to the top-level functions. This allows concurrent configuration
-options. See the ConfigState documentation for more details.
-
-The following configuration options are available:
- * Indent
- String to use for each indentation level for Dump functions.
- It is a single space by default. A popular alternative is "\t".
-
- * MaxDepth
- Maximum number of levels to descend into nested data structures.
- There is no limit by default.
-
- * DisableMethods
- Disables invocation of error and Stringer interface methods.
- Method invocation is enabled by default.
-
- * DisablePointerMethods
- Disables invocation of error and Stringer interface methods on types
- which only accept pointer receivers from non-pointer variables.
- Pointer method invocation is enabled by default.
-
- * DisablePointerAddresses
- DisablePointerAddresses specifies whether to disable the printing of
- pointer addresses. This is useful when diffing data structures in tests.
-
- * DisableCapacities
- DisableCapacities specifies whether to disable the printing of
- capacities for arrays, slices, maps and channels. This is useful when
- diffing data structures in tests.
-
- * ContinueOnMethod
- Enables recursion into types after invoking error and Stringer interface
- methods. Recursion after method invocation is disabled by default.
-
- * SortKeys
- Specifies map keys should be sorted before being printed. Use
- this to have a more deterministic, diffable output. Note that
- only native types (bool, int, uint, floats, uintptr and string)
- and types which implement error or Stringer interfaces are
- supported with other types sorted according to the
- reflect.Value.String() output which guarantees display
- stability. Natural map order is used by default.
-
- * SpewKeys
- Specifies that, as a last resort attempt, map keys should be
- spewed to strings and sorted by those strings. This is only
- considered if SortKeys is true.
-
-Dump Usage
-
-Simply call spew.Dump with a list of variables you want to dump:
-
- spew.Dump(myVar1, myVar2, ...)
-
-You may also call spew.Fdump if you would prefer to output to an arbitrary
-io.Writer. For example, to dump to standard error:
-
- spew.Fdump(os.Stderr, myVar1, myVar2, ...)
-
-A third option is to call spew.Sdump to get the formatted output as a string:
-
- str := spew.Sdump(myVar1, myVar2, ...)
-
-Sample Dump Output
-
-See the Dump example for details on the setup of the types and variables being
-shown here.
-
- (main.Foo) {
- unexportedField: (*main.Bar)(0xf84002e210)({
- flag: (main.Flag) flagTwo,
- data: (uintptr)
- }),
- ExportedField: (map[interface {}]interface {}) (len=1) {
- (string) (len=3) "one": (bool) true
- }
- }
-
-Byte (and uint8) arrays and slices are displayed uniquely like the hexdump -C
-command as shown.
- ([]uint8) (len=32 cap=32) {
- 00000000 11 12 13 14 15 16 17 18 19 1a 1b 1c 1d 1e 1f 20 |............... |
- 00000010 21 22 23 24 25 26 27 28 29 2a 2b 2c 2d 2e 2f 30 |!"#$%&'()*+,-./0|
- 00000020 31 32 |12|
- }
-
-Custom Formatter
-
-Spew provides a custom formatter that implements the fmt.Formatter interface
-so that it integrates cleanly with standard fmt package printing functions. The
-formatter is useful for inline printing of smaller data types similar to the
-standard %v format specifier.
-
-The custom formatter only responds to the %v (most compact), %+v (adds pointer
-addresses), %#v (adds types), or %#+v (adds types and pointer addresses) verb
-combinations. Any other verbs such as %x and %q will be sent to the the
-standard fmt package for formatting. In addition, the custom formatter ignores
-the width and precision arguments (however they will still work on the format
-specifiers not handled by the custom formatter).
-
-Custom Formatter Usage
-
-The simplest way to make use of the spew custom formatter is to call one of the
-convenience functions such as spew.Printf, spew.Println, or spew.Printf. The
-functions have syntax you are most likely already familiar with:
-
- spew.Printf("myVar1: %v -- myVar2: %+v", myVar1, myVar2)
- spew.Printf("myVar3: %#v -- myVar4: %#+v", myVar3, myVar4)
- spew.Println(myVar, myVar2)
- spew.Fprintf(os.Stderr, "myVar1: %v -- myVar2: %+v", myVar1, myVar2)
- spew.Fprintf(os.Stderr, "myVar3: %#v -- myVar4: %#+v", myVar3, myVar4)
-
-See the Index for the full list convenience functions.
-
-Sample Formatter Output
-
-Double pointer to a uint8:
- %v: <**>5
- %+v: <**>(0xf8400420d0->0xf8400420c8)5
- %#v: (**uint8)5
- %#+v: (**uint8)(0xf8400420d0->0xf8400420c8)5
-
-Pointer to circular struct with a uint8 field and a pointer to itself:
- %v: <*>{1 <*>}
- %+v: <*>(0xf84003e260){ui8:1 c:<*>(0xf84003e260)}
- %#v: (*main.circular){ui8:(uint8)1 c:(*main.circular)}
- %#+v: (*main.circular)(0xf84003e260){ui8:(uint8)1 c:(*main.circular)(0xf84003e260)}
-
-See the Printf example for details on the setup of variables being shown
-here.
-
-Errors
-
-Since it is possible for custom Stringer/error interfaces to panic, spew
-detects them and handles them internally by printing the panic information
-inline with the output. Since spew is intended to provide deep pretty printing
-capabilities on structures, it intentionally does not return any errors.
-*/
-package spew
diff --git a/vendor/github.com/davecgh/go-spew/spew/dump.go b/vendor/github.com/davecgh/go-spew/spew/dump.go
deleted file mode 100644
index f78d89f..0000000
--- a/vendor/github.com/davecgh/go-spew/spew/dump.go
+++ /dev/null
@@ -1,509 +0,0 @@
-/*
- * Copyright (c) 2013-2016 Dave Collins
- *
- * Permission to use, copy, modify, and distribute this software for any
- * purpose with or without fee is hereby granted, provided that the above
- * copyright notice and this permission notice appear in all copies.
- *
- * THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES
- * WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF
- * MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR
- * ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
- * WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN
- * ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF
- * OR IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
- */
-
-package spew
-
-import (
- "bytes"
- "encoding/hex"
- "fmt"
- "io"
- "os"
- "reflect"
- "regexp"
- "strconv"
- "strings"
-)
-
-var (
- // uint8Type is a reflect.Type representing a uint8. It is used to
- // convert cgo types to uint8 slices for hexdumping.
- uint8Type = reflect.TypeOf(uint8(0))
-
- // cCharRE is a regular expression that matches a cgo char.
- // It is used to detect character arrays to hexdump them.
- cCharRE = regexp.MustCompile(`^.*\._Ctype_char$`)
-
- // cUnsignedCharRE is a regular expression that matches a cgo unsigned
- // char. It is used to detect unsigned character arrays to hexdump
- // them.
- cUnsignedCharRE = regexp.MustCompile(`^.*\._Ctype_unsignedchar$`)
-
- // cUint8tCharRE is a regular expression that matches a cgo uint8_t.
- // It is used to detect uint8_t arrays to hexdump them.
- cUint8tCharRE = regexp.MustCompile(`^.*\._Ctype_uint8_t$`)
-)
-
-// dumpState contains information about the state of a dump operation.
-type dumpState struct {
- w io.Writer
- depth int
- pointers map[uintptr]int
- ignoreNextType bool
- ignoreNextIndent bool
- cs *ConfigState
-}
-
-// indent performs indentation according to the depth level and cs.Indent
-// option.
-func (d *dumpState) indent() {
- if d.ignoreNextIndent {
- d.ignoreNextIndent = false
- return
- }
- d.w.Write(bytes.Repeat([]byte(d.cs.Indent), d.depth))
-}
-
-// unpackValue returns values inside of non-nil interfaces when possible.
-// This is useful for data types like structs, arrays, slices, and maps which
-// can contain varying types packed inside an interface.
-func (d *dumpState) unpackValue(v reflect.Value) reflect.Value {
- if v.Kind() == reflect.Interface && !v.IsNil() {
- v = v.Elem()
- }
- return v
-}
-
-// dumpPtr handles formatting of pointers by indirecting them as necessary.
-func (d *dumpState) dumpPtr(v reflect.Value) {
- // Remove pointers at or below the current depth from map used to detect
- // circular refs.
- for k, depth := range d.pointers {
- if depth >= d.depth {
- delete(d.pointers, k)
- }
- }
-
- // Keep list of all dereferenced pointers to show later.
- pointerChain := make([]uintptr, 0)
-
- // Figure out how many levels of indirection there are by dereferencing
- // pointers and unpacking interfaces down the chain while detecting circular
- // references.
- nilFound := false
- cycleFound := false
- indirects := 0
- ve := v
- for ve.Kind() == reflect.Ptr {
- if ve.IsNil() {
- nilFound = true
- break
- }
- indirects++
- addr := ve.Pointer()
- pointerChain = append(pointerChain, addr)
- if pd, ok := d.pointers[addr]; ok && pd < d.depth {
- cycleFound = true
- indirects--
- break
- }
- d.pointers[addr] = d.depth
-
- ve = ve.Elem()
- if ve.Kind() == reflect.Interface {
- if ve.IsNil() {
- nilFound = true
- break
- }
- ve = ve.Elem()
- }
- }
-
- // Display type information.
- d.w.Write(openParenBytes)
- d.w.Write(bytes.Repeat(asteriskBytes, indirects))
- d.w.Write([]byte(ve.Type().String()))
- d.w.Write(closeParenBytes)
-
- // Display pointer information.
- if !d.cs.DisablePointerAddresses && len(pointerChain) > 0 {
- d.w.Write(openParenBytes)
- for i, addr := range pointerChain {
- if i > 0 {
- d.w.Write(pointerChainBytes)
- }
- printHexPtr(d.w, addr)
- }
- d.w.Write(closeParenBytes)
- }
-
- // Display dereferenced value.
- d.w.Write(openParenBytes)
- switch {
- case nilFound:
- d.w.Write(nilAngleBytes)
-
- case cycleFound:
- d.w.Write(circularBytes)
-
- default:
- d.ignoreNextType = true
- d.dump(ve)
- }
- d.w.Write(closeParenBytes)
-}
-
-// dumpSlice handles formatting of arrays and slices. Byte (uint8 under
-// reflection) arrays and slices are dumped in hexdump -C fashion.
-func (d *dumpState) dumpSlice(v reflect.Value) {
- // Determine whether this type should be hex dumped or not. Also,
- // for types which should be hexdumped, try to use the underlying data
- // first, then fall back to trying to convert them to a uint8 slice.
- var buf []uint8
- doConvert := false
- doHexDump := false
- numEntries := v.Len()
- if numEntries > 0 {
- vt := v.Index(0).Type()
- vts := vt.String()
- switch {
- // C types that need to be converted.
- case cCharRE.MatchString(vts):
- fallthrough
- case cUnsignedCharRE.MatchString(vts):
- fallthrough
- case cUint8tCharRE.MatchString(vts):
- doConvert = true
-
- // Try to use existing uint8 slices and fall back to converting
- // and copying if that fails.
- case vt.Kind() == reflect.Uint8:
- // We need an addressable interface to convert the type
- // to a byte slice. However, the reflect package won't
- // give us an interface on certain things like
- // unexported struct fields in order to enforce
- // visibility rules. We use unsafe, when available, to
- // bypass these restrictions since this package does not
- // mutate the values.
- vs := v
- if !vs.CanInterface() || !vs.CanAddr() {
- vs = unsafeReflectValue(vs)
- }
- if !UnsafeDisabled {
- vs = vs.Slice(0, numEntries)
-
- // Use the existing uint8 slice if it can be
- // type asserted.
- iface := vs.Interface()
- if slice, ok := iface.([]uint8); ok {
- buf = slice
- doHexDump = true
- break
- }
- }
-
- // The underlying data needs to be converted if it can't
- // be type asserted to a uint8 slice.
- doConvert = true
- }
-
- // Copy and convert the underlying type if needed.
- if doConvert && vt.ConvertibleTo(uint8Type) {
- // Convert and copy each element into a uint8 byte
- // slice.
- buf = make([]uint8, numEntries)
- for i := 0; i < numEntries; i++ {
- vv := v.Index(i)
- buf[i] = uint8(vv.Convert(uint8Type).Uint())
- }
- doHexDump = true
- }
- }
-
- // Hexdump the entire slice as needed.
- if doHexDump {
- indent := strings.Repeat(d.cs.Indent, d.depth)
- str := indent + hex.Dump(buf)
- str = strings.Replace(str, "\n", "\n"+indent, -1)
- str = strings.TrimRight(str, d.cs.Indent)
- d.w.Write([]byte(str))
- return
- }
-
- // Recursively call dump for each item.
- for i := 0; i < numEntries; i++ {
- d.dump(d.unpackValue(v.Index(i)))
- if i < (numEntries - 1) {
- d.w.Write(commaNewlineBytes)
- } else {
- d.w.Write(newlineBytes)
- }
- }
-}
-
-// dump is the main workhorse for dumping a value. It uses the passed reflect
-// value to figure out what kind of object we are dealing with and formats it
-// appropriately. It is a recursive function, however circular data structures
-// are detected and handled properly.
-func (d *dumpState) dump(v reflect.Value) {
- // Handle invalid reflect values immediately.
- kind := v.Kind()
- if kind == reflect.Invalid {
- d.w.Write(invalidAngleBytes)
- return
- }
-
- // Handle pointers specially.
- if kind == reflect.Ptr {
- d.indent()
- d.dumpPtr(v)
- return
- }
-
- // Print type information unless already handled elsewhere.
- if !d.ignoreNextType {
- d.indent()
- d.w.Write(openParenBytes)
- d.w.Write([]byte(v.Type().String()))
- d.w.Write(closeParenBytes)
- d.w.Write(spaceBytes)
- }
- d.ignoreNextType = false
-
- // Display length and capacity if the built-in len and cap functions
- // work with the value's kind and the len/cap itself is non-zero.
- valueLen, valueCap := 0, 0
- switch v.Kind() {
- case reflect.Array, reflect.Slice, reflect.Chan:
- valueLen, valueCap = v.Len(), v.Cap()
- case reflect.Map, reflect.String:
- valueLen = v.Len()
- }
- if valueLen != 0 || !d.cs.DisableCapacities && valueCap != 0 {
- d.w.Write(openParenBytes)
- if valueLen != 0 {
- d.w.Write(lenEqualsBytes)
- printInt(d.w, int64(valueLen), 10)
- }
- if !d.cs.DisableCapacities && valueCap != 0 {
- if valueLen != 0 {
- d.w.Write(spaceBytes)
- }
- d.w.Write(capEqualsBytes)
- printInt(d.w, int64(valueCap), 10)
- }
- d.w.Write(closeParenBytes)
- d.w.Write(spaceBytes)
- }
-
- // Call Stringer/error interfaces if they exist and the handle methods flag
- // is enabled
- if !d.cs.DisableMethods {
- if (kind != reflect.Invalid) && (kind != reflect.Interface) {
- if handled := handleMethods(d.cs, d.w, v); handled {
- return
- }
- }
- }
-
- switch kind {
- case reflect.Invalid:
- // Do nothing. We should never get here since invalid has already
- // been handled above.
-
- case reflect.Bool:
- printBool(d.w, v.Bool())
-
- case reflect.Int8, reflect.Int16, reflect.Int32, reflect.Int64, reflect.Int:
- printInt(d.w, v.Int(), 10)
-
- case reflect.Uint8, reflect.Uint16, reflect.Uint32, reflect.Uint64, reflect.Uint:
- printUint(d.w, v.Uint(), 10)
-
- case reflect.Float32:
- printFloat(d.w, v.Float(), 32)
-
- case reflect.Float64:
- printFloat(d.w, v.Float(), 64)
-
- case reflect.Complex64:
- printComplex(d.w, v.Complex(), 32)
-
- case reflect.Complex128:
- printComplex(d.w, v.Complex(), 64)
-
- case reflect.Slice:
- if v.IsNil() {
- d.w.Write(nilAngleBytes)
- break
- }
- fallthrough
-
- case reflect.Array:
- d.w.Write(openBraceNewlineBytes)
- d.depth++
- if (d.cs.MaxDepth != 0) && (d.depth > d.cs.MaxDepth) {
- d.indent()
- d.w.Write(maxNewlineBytes)
- } else {
- d.dumpSlice(v)
- }
- d.depth--
- d.indent()
- d.w.Write(closeBraceBytes)
-
- case reflect.String:
- d.w.Write([]byte(strconv.Quote(v.String())))
-
- case reflect.Interface:
- // The only time we should get here is for nil interfaces due to
- // unpackValue calls.
- if v.IsNil() {
- d.w.Write(nilAngleBytes)
- }
-
- case reflect.Ptr:
- // Do nothing. We should never get here since pointers have already
- // been handled above.
-
- case reflect.Map:
- // nil maps should be indicated as different than empty maps
- if v.IsNil() {
- d.w.Write(nilAngleBytes)
- break
- }
-
- d.w.Write(openBraceNewlineBytes)
- d.depth++
- if (d.cs.MaxDepth != 0) && (d.depth > d.cs.MaxDepth) {
- d.indent()
- d.w.Write(maxNewlineBytes)
- } else {
- numEntries := v.Len()
- keys := v.MapKeys()
- if d.cs.SortKeys {
- sortValues(keys, d.cs)
- }
- for i, key := range keys {
- d.dump(d.unpackValue(key))
- d.w.Write(colonSpaceBytes)
- d.ignoreNextIndent = true
- d.dump(d.unpackValue(v.MapIndex(key)))
- if i < (numEntries - 1) {
- d.w.Write(commaNewlineBytes)
- } else {
- d.w.Write(newlineBytes)
- }
- }
- }
- d.depth--
- d.indent()
- d.w.Write(closeBraceBytes)
-
- case reflect.Struct:
- d.w.Write(openBraceNewlineBytes)
- d.depth++
- if (d.cs.MaxDepth != 0) && (d.depth > d.cs.MaxDepth) {
- d.indent()
- d.w.Write(maxNewlineBytes)
- } else {
- vt := v.Type()
- numFields := v.NumField()
- for i := 0; i < numFields; i++ {
- d.indent()
- vtf := vt.Field(i)
- d.w.Write([]byte(vtf.Name))
- d.w.Write(colonSpaceBytes)
- d.ignoreNextIndent = true
- d.dump(d.unpackValue(v.Field(i)))
- if i < (numFields - 1) {
- d.w.Write(commaNewlineBytes)
- } else {
- d.w.Write(newlineBytes)
- }
- }
- }
- d.depth--
- d.indent()
- d.w.Write(closeBraceBytes)
-
- case reflect.Uintptr:
- printHexPtr(d.w, uintptr(v.Uint()))
-
- case reflect.UnsafePointer, reflect.Chan, reflect.Func:
- printHexPtr(d.w, v.Pointer())
-
- // There were not any other types at the time this code was written, but
- // fall back to letting the default fmt package handle it in case any new
- // types are added.
- default:
- if v.CanInterface() {
- fmt.Fprintf(d.w, "%v", v.Interface())
- } else {
- fmt.Fprintf(d.w, "%v", v.String())
- }
- }
-}
-
-// fdump is a helper function to consolidate the logic from the various public
-// methods which take varying writers and config states.
-func fdump(cs *ConfigState, w io.Writer, a ...interface{}) {
- for _, arg := range a {
- if arg == nil {
- w.Write(interfaceBytes)
- w.Write(spaceBytes)
- w.Write(nilAngleBytes)
- w.Write(newlineBytes)
- continue
- }
-
- d := dumpState{w: w, cs: cs}
- d.pointers = make(map[uintptr]int)
- d.dump(reflect.ValueOf(arg))
- d.w.Write(newlineBytes)
- }
-}
-
-// Fdump formats and displays the passed arguments to io.Writer w. It formats
-// exactly the same as Dump.
-func Fdump(w io.Writer, a ...interface{}) {
- fdump(&Config, w, a...)
-}
-
-// Sdump returns a string with the passed arguments formatted exactly the same
-// as Dump.
-func Sdump(a ...interface{}) string {
- var buf bytes.Buffer
- fdump(&Config, &buf, a...)
- return buf.String()
-}
-
-/*
-Dump displays the passed parameters to standard out with newlines, customizable
-indentation, and additional debug information such as complete types and all
-pointer addresses used to indirect to the final value. It provides the
-following features over the built-in printing facilities provided by the fmt
-package:
-
- * Pointers are dereferenced and followed
- * Circular data structures are detected and handled properly
- * Custom Stringer/error interfaces are optionally invoked, including
- on unexported types
- * Custom types which only implement the Stringer/error interfaces via
- a pointer receiver are optionally invoked when passing non-pointer
- variables
- * Byte arrays and slices are dumped like the hexdump -C command which
- includes offsets, byte values in hex, and ASCII output
-
-The configuration options are controlled by an exported package global,
-spew.Config. See ConfigState for options documentation.
-
-See Fdump if you would prefer dumping to an arbitrary io.Writer or Sdump to
-get the formatted result as a string.
-*/
-func Dump(a ...interface{}) {
- fdump(&Config, os.Stdout, a...)
-}
diff --git a/vendor/github.com/davecgh/go-spew/spew/format.go b/vendor/github.com/davecgh/go-spew/spew/format.go
deleted file mode 100644
index b04edb7..0000000
--- a/vendor/github.com/davecgh/go-spew/spew/format.go
+++ /dev/null
@@ -1,419 +0,0 @@
-/*
- * Copyright (c) 2013-2016 Dave Collins
- *
- * Permission to use, copy, modify, and distribute this software for any
- * purpose with or without fee is hereby granted, provided that the above
- * copyright notice and this permission notice appear in all copies.
- *
- * THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES
- * WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF
- * MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR
- * ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
- * WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN
- * ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF
- * OR IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
- */
-
-package spew
-
-import (
- "bytes"
- "fmt"
- "reflect"
- "strconv"
- "strings"
-)
-
-// supportedFlags is a list of all the character flags supported by fmt package.
-const supportedFlags = "0-+# "
-
-// formatState implements the fmt.Formatter interface and contains information
-// about the state of a formatting operation. The NewFormatter function can
-// be used to get a new Formatter which can be used directly as arguments
-// in standard fmt package printing calls.
-type formatState struct {
- value interface{}
- fs fmt.State
- depth int
- pointers map[uintptr]int
- ignoreNextType bool
- cs *ConfigState
-}
-
-// buildDefaultFormat recreates the original format string without precision
-// and width information to pass in to fmt.Sprintf in the case of an
-// unrecognized type. Unless new types are added to the language, this
-// function won't ever be called.
-func (f *formatState) buildDefaultFormat() (format string) {
- buf := bytes.NewBuffer(percentBytes)
-
- for _, flag := range supportedFlags {
- if f.fs.Flag(int(flag)) {
- buf.WriteRune(flag)
- }
- }
-
- buf.WriteRune('v')
-
- format = buf.String()
- return format
-}
-
-// constructOrigFormat recreates the original format string including precision
-// and width information to pass along to the standard fmt package. This allows
-// automatic deferral of all format strings this package doesn't support.
-func (f *formatState) constructOrigFormat(verb rune) (format string) {
- buf := bytes.NewBuffer(percentBytes)
-
- for _, flag := range supportedFlags {
- if f.fs.Flag(int(flag)) {
- buf.WriteRune(flag)
- }
- }
-
- if width, ok := f.fs.Width(); ok {
- buf.WriteString(strconv.Itoa(width))
- }
-
- if precision, ok := f.fs.Precision(); ok {
- buf.Write(precisionBytes)
- buf.WriteString(strconv.Itoa(precision))
- }
-
- buf.WriteRune(verb)
-
- format = buf.String()
- return format
-}
-
-// unpackValue returns values inside of non-nil interfaces when possible and
-// ensures that types for values which have been unpacked from an interface
-// are displayed when the show types flag is also set.
-// This is useful for data types like structs, arrays, slices, and maps which
-// can contain varying types packed inside an interface.
-func (f *formatState) unpackValue(v reflect.Value) reflect.Value {
- if v.Kind() == reflect.Interface {
- f.ignoreNextType = false
- if !v.IsNil() {
- v = v.Elem()
- }
- }
- return v
-}
-
-// formatPtr handles formatting of pointers by indirecting them as necessary.
-func (f *formatState) formatPtr(v reflect.Value) {
- // Display nil if top level pointer is nil.
- showTypes := f.fs.Flag('#')
- if v.IsNil() && (!showTypes || f.ignoreNextType) {
- f.fs.Write(nilAngleBytes)
- return
- }
-
- // Remove pointers at or below the current depth from map used to detect
- // circular refs.
- for k, depth := range f.pointers {
- if depth >= f.depth {
- delete(f.pointers, k)
- }
- }
-
- // Keep list of all dereferenced pointers to possibly show later.
- pointerChain := make([]uintptr, 0)
-
- // Figure out how many levels of indirection there are by derferencing
- // pointers and unpacking interfaces down the chain while detecting circular
- // references.
- nilFound := false
- cycleFound := false
- indirects := 0
- ve := v
- for ve.Kind() == reflect.Ptr {
- if ve.IsNil() {
- nilFound = true
- break
- }
- indirects++
- addr := ve.Pointer()
- pointerChain = append(pointerChain, addr)
- if pd, ok := f.pointers[addr]; ok && pd < f.depth {
- cycleFound = true
- indirects--
- break
- }
- f.pointers[addr] = f.depth
-
- ve = ve.Elem()
- if ve.Kind() == reflect.Interface {
- if ve.IsNil() {
- nilFound = true
- break
- }
- ve = ve.Elem()
- }
- }
-
- // Display type or indirection level depending on flags.
- if showTypes && !f.ignoreNextType {
- f.fs.Write(openParenBytes)
- f.fs.Write(bytes.Repeat(asteriskBytes, indirects))
- f.fs.Write([]byte(ve.Type().String()))
- f.fs.Write(closeParenBytes)
- } else {
- if nilFound || cycleFound {
- indirects += strings.Count(ve.Type().String(), "*")
- }
- f.fs.Write(openAngleBytes)
- f.fs.Write([]byte(strings.Repeat("*", indirects)))
- f.fs.Write(closeAngleBytes)
- }
-
- // Display pointer information depending on flags.
- if f.fs.Flag('+') && (len(pointerChain) > 0) {
- f.fs.Write(openParenBytes)
- for i, addr := range pointerChain {
- if i > 0 {
- f.fs.Write(pointerChainBytes)
- }
- printHexPtr(f.fs, addr)
- }
- f.fs.Write(closeParenBytes)
- }
-
- // Display dereferenced value.
- switch {
- case nilFound:
- f.fs.Write(nilAngleBytes)
-
- case cycleFound:
- f.fs.Write(circularShortBytes)
-
- default:
- f.ignoreNextType = true
- f.format(ve)
- }
-}
-
-// format is the main workhorse for providing the Formatter interface. It
-// uses the passed reflect value to figure out what kind of object we are
-// dealing with and formats it appropriately. It is a recursive function,
-// however circular data structures are detected and handled properly.
-func (f *formatState) format(v reflect.Value) {
- // Handle invalid reflect values immediately.
- kind := v.Kind()
- if kind == reflect.Invalid {
- f.fs.Write(invalidAngleBytes)
- return
- }
-
- // Handle pointers specially.
- if kind == reflect.Ptr {
- f.formatPtr(v)
- return
- }
-
- // Print type information unless already handled elsewhere.
- if !f.ignoreNextType && f.fs.Flag('#') {
- f.fs.Write(openParenBytes)
- f.fs.Write([]byte(v.Type().String()))
- f.fs.Write(closeParenBytes)
- }
- f.ignoreNextType = false
-
- // Call Stringer/error interfaces if they exist and the handle methods
- // flag is enabled.
- if !f.cs.DisableMethods {
- if (kind != reflect.Invalid) && (kind != reflect.Interface) {
- if handled := handleMethods(f.cs, f.fs, v); handled {
- return
- }
- }
- }
-
- switch kind {
- case reflect.Invalid:
- // Do nothing. We should never get here since invalid has already
- // been handled above.
-
- case reflect.Bool:
- printBool(f.fs, v.Bool())
-
- case reflect.Int8, reflect.Int16, reflect.Int32, reflect.Int64, reflect.Int:
- printInt(f.fs, v.Int(), 10)
-
- case reflect.Uint8, reflect.Uint16, reflect.Uint32, reflect.Uint64, reflect.Uint:
- printUint(f.fs, v.Uint(), 10)
-
- case reflect.Float32:
- printFloat(f.fs, v.Float(), 32)
-
- case reflect.Float64:
- printFloat(f.fs, v.Float(), 64)
-
- case reflect.Complex64:
- printComplex(f.fs, v.Complex(), 32)
-
- case reflect.Complex128:
- printComplex(f.fs, v.Complex(), 64)
-
- case reflect.Slice:
- if v.IsNil() {
- f.fs.Write(nilAngleBytes)
- break
- }
- fallthrough
-
- case reflect.Array:
- f.fs.Write(openBracketBytes)
- f.depth++
- if (f.cs.MaxDepth != 0) && (f.depth > f.cs.MaxDepth) {
- f.fs.Write(maxShortBytes)
- } else {
- numEntries := v.Len()
- for i := 0; i < numEntries; i++ {
- if i > 0 {
- f.fs.Write(spaceBytes)
- }
- f.ignoreNextType = true
- f.format(f.unpackValue(v.Index(i)))
- }
- }
- f.depth--
- f.fs.Write(closeBracketBytes)
-
- case reflect.String:
- f.fs.Write([]byte(v.String()))
-
- case reflect.Interface:
- // The only time we should get here is for nil interfaces due to
- // unpackValue calls.
- if v.IsNil() {
- f.fs.Write(nilAngleBytes)
- }
-
- case reflect.Ptr:
- // Do nothing. We should never get here since pointers have already
- // been handled above.
-
- case reflect.Map:
- // nil maps should be indicated as different than empty maps
- if v.IsNil() {
- f.fs.Write(nilAngleBytes)
- break
- }
-
- f.fs.Write(openMapBytes)
- f.depth++
- if (f.cs.MaxDepth != 0) && (f.depth > f.cs.MaxDepth) {
- f.fs.Write(maxShortBytes)
- } else {
- keys := v.MapKeys()
- if f.cs.SortKeys {
- sortValues(keys, f.cs)
- }
- for i, key := range keys {
- if i > 0 {
- f.fs.Write(spaceBytes)
- }
- f.ignoreNextType = true
- f.format(f.unpackValue(key))
- f.fs.Write(colonBytes)
- f.ignoreNextType = true
- f.format(f.unpackValue(v.MapIndex(key)))
- }
- }
- f.depth--
- f.fs.Write(closeMapBytes)
-
- case reflect.Struct:
- numFields := v.NumField()
- f.fs.Write(openBraceBytes)
- f.depth++
- if (f.cs.MaxDepth != 0) && (f.depth > f.cs.MaxDepth) {
- f.fs.Write(maxShortBytes)
- } else {
- vt := v.Type()
- for i := 0; i < numFields; i++ {
- if i > 0 {
- f.fs.Write(spaceBytes)
- }
- vtf := vt.Field(i)
- if f.fs.Flag('+') || f.fs.Flag('#') {
- f.fs.Write([]byte(vtf.Name))
- f.fs.Write(colonBytes)
- }
- f.format(f.unpackValue(v.Field(i)))
- }
- }
- f.depth--
- f.fs.Write(closeBraceBytes)
-
- case reflect.Uintptr:
- printHexPtr(f.fs, uintptr(v.Uint()))
-
- case reflect.UnsafePointer, reflect.Chan, reflect.Func:
- printHexPtr(f.fs, v.Pointer())
-
- // There were not any other types at the time this code was written, but
- // fall back to letting the default fmt package handle it if any get added.
- default:
- format := f.buildDefaultFormat()
- if v.CanInterface() {
- fmt.Fprintf(f.fs, format, v.Interface())
- } else {
- fmt.Fprintf(f.fs, format, v.String())
- }
- }
-}
-
-// Format satisfies the fmt.Formatter interface. See NewFormatter for usage
-// details.
-func (f *formatState) Format(fs fmt.State, verb rune) {
- f.fs = fs
-
- // Use standard formatting for verbs that are not v.
- if verb != 'v' {
- format := f.constructOrigFormat(verb)
- fmt.Fprintf(fs, format, f.value)
- return
- }
-
- if f.value == nil {
- if fs.Flag('#') {
- fs.Write(interfaceBytes)
- }
- fs.Write(nilAngleBytes)
- return
- }
-
- f.format(reflect.ValueOf(f.value))
-}
-
-// newFormatter is a helper function to consolidate the logic from the various
-// public methods which take varying config states.
-func newFormatter(cs *ConfigState, v interface{}) fmt.Formatter {
- fs := &formatState{value: v, cs: cs}
- fs.pointers = make(map[uintptr]int)
- return fs
-}
-
-/*
-NewFormatter returns a custom formatter that satisfies the fmt.Formatter
-interface. As a result, it integrates cleanly with standard fmt package
-printing functions. The formatter is useful for inline printing of smaller data
-types similar to the standard %v format specifier.
-
-The custom formatter only responds to the %v (most compact), %+v (adds pointer
-addresses), %#v (adds types), or %#+v (adds types and pointer addresses) verb
-combinations. Any other verbs such as %x and %q will be sent to the the
-standard fmt package for formatting. In addition, the custom formatter ignores
-the width and precision arguments (however they will still work on the format
-specifiers not handled by the custom formatter).
-
-Typically this function shouldn't be called directly. It is much easier to make
-use of the custom formatter by calling one of the convenience functions such as
-Printf, Println, or Fprintf.
-*/
-func NewFormatter(v interface{}) fmt.Formatter {
- return newFormatter(&Config, v)
-}
diff --git a/vendor/github.com/davecgh/go-spew/spew/spew.go b/vendor/github.com/davecgh/go-spew/spew/spew.go
deleted file mode 100644
index 32c0e33..0000000
--- a/vendor/github.com/davecgh/go-spew/spew/spew.go
+++ /dev/null
@@ -1,148 +0,0 @@
-/*
- * Copyright (c) 2013-2016 Dave Collins
- *
- * Permission to use, copy, modify, and distribute this software for any
- * purpose with or without fee is hereby granted, provided that the above
- * copyright notice and this permission notice appear in all copies.
- *
- * THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES
- * WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF
- * MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR
- * ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
- * WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN
- * ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF
- * OR IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
- */
-
-package spew
-
-import (
- "fmt"
- "io"
-)
-
-// Errorf is a wrapper for fmt.Errorf that treats each argument as if it were
-// passed with a default Formatter interface returned by NewFormatter. It
-// returns the formatted string as a value that satisfies error. See
-// NewFormatter for formatting details.
-//
-// This function is shorthand for the following syntax:
-//
-// fmt.Errorf(format, spew.NewFormatter(a), spew.NewFormatter(b))
-func Errorf(format string, a ...interface{}) (err error) {
- return fmt.Errorf(format, convertArgs(a)...)
-}
-
-// Fprint is a wrapper for fmt.Fprint that treats each argument as if it were
-// passed with a default Formatter interface returned by NewFormatter. It
-// returns the number of bytes written and any write error encountered. See
-// NewFormatter for formatting details.
-//
-// This function is shorthand for the following syntax:
-//
-// fmt.Fprint(w, spew.NewFormatter(a), spew.NewFormatter(b))
-func Fprint(w io.Writer, a ...interface{}) (n int, err error) {
- return fmt.Fprint(w, convertArgs(a)...)
-}
-
-// Fprintf is a wrapper for fmt.Fprintf that treats each argument as if it were
-// passed with a default Formatter interface returned by NewFormatter. It
-// returns the number of bytes written and any write error encountered. See
-// NewFormatter for formatting details.
-//
-// This function is shorthand for the following syntax:
-//
-// fmt.Fprintf(w, format, spew.NewFormatter(a), spew.NewFormatter(b))
-func Fprintf(w io.Writer, format string, a ...interface{}) (n int, err error) {
- return fmt.Fprintf(w, format, convertArgs(a)...)
-}
-
-// Fprintln is a wrapper for fmt.Fprintln that treats each argument as if it
-// passed with a default Formatter interface returned by NewFormatter. See
-// NewFormatter for formatting details.
-//
-// This function is shorthand for the following syntax:
-//
-// fmt.Fprintln(w, spew.NewFormatter(a), spew.NewFormatter(b))
-func Fprintln(w io.Writer, a ...interface{}) (n int, err error) {
- return fmt.Fprintln(w, convertArgs(a)...)
-}
-
-// Print is a wrapper for fmt.Print that treats each argument as if it were
-// passed with a default Formatter interface returned by NewFormatter. It
-// returns the number of bytes written and any write error encountered. See
-// NewFormatter for formatting details.
-//
-// This function is shorthand for the following syntax:
-//
-// fmt.Print(spew.NewFormatter(a), spew.NewFormatter(b))
-func Print(a ...interface{}) (n int, err error) {
- return fmt.Print(convertArgs(a)...)
-}
-
-// Printf is a wrapper for fmt.Printf that treats each argument as if it were
-// passed with a default Formatter interface returned by NewFormatter. It
-// returns the number of bytes written and any write error encountered. See
-// NewFormatter for formatting details.
-//
-// This function is shorthand for the following syntax:
-//
-// fmt.Printf(format, spew.NewFormatter(a), spew.NewFormatter(b))
-func Printf(format string, a ...interface{}) (n int, err error) {
- return fmt.Printf(format, convertArgs(a)...)
-}
-
-// Println is a wrapper for fmt.Println that treats each argument as if it were
-// passed with a default Formatter interface returned by NewFormatter. It
-// returns the number of bytes written and any write error encountered. See
-// NewFormatter for formatting details.
-//
-// This function is shorthand for the following syntax:
-//
-// fmt.Println(spew.NewFormatter(a), spew.NewFormatter(b))
-func Println(a ...interface{}) (n int, err error) {
- return fmt.Println(convertArgs(a)...)
-}
-
-// Sprint is a wrapper for fmt.Sprint that treats each argument as if it were
-// passed with a default Formatter interface returned by NewFormatter. It
-// returns the resulting string. See NewFormatter for formatting details.
-//
-// This function is shorthand for the following syntax:
-//
-// fmt.Sprint(spew.NewFormatter(a), spew.NewFormatter(b))
-func Sprint(a ...interface{}) string {
- return fmt.Sprint(convertArgs(a)...)
-}
-
-// Sprintf is a wrapper for fmt.Sprintf that treats each argument as if it were
-// passed with a default Formatter interface returned by NewFormatter. It
-// returns the resulting string. See NewFormatter for formatting details.
-//
-// This function is shorthand for the following syntax:
-//
-// fmt.Sprintf(format, spew.NewFormatter(a), spew.NewFormatter(b))
-func Sprintf(format string, a ...interface{}) string {
- return fmt.Sprintf(format, convertArgs(a)...)
-}
-
-// Sprintln is a wrapper for fmt.Sprintln that treats each argument as if it
-// were passed with a default Formatter interface returned by NewFormatter. It
-// returns the resulting string. See NewFormatter for formatting details.
-//
-// This function is shorthand for the following syntax:
-//
-// fmt.Sprintln(spew.NewFormatter(a), spew.NewFormatter(b))
-func Sprintln(a ...interface{}) string {
- return fmt.Sprintln(convertArgs(a)...)
-}
-
-// convertArgs accepts a slice of arguments and returns a slice of the same
-// length with each argument converted to a default spew Formatter interface.
-func convertArgs(args []interface{}) (formatters []interface{}) {
- formatters = make([]interface{}, len(args))
- for index, arg := range args {
- formatters[index] = NewFormatter(arg)
- }
- return formatters
-}
diff --git a/vendor/github.com/dlclark/regexp2/.gitignore b/vendor/github.com/dlclark/regexp2/.gitignore
deleted file mode 100644
index fb844c3..0000000
--- a/vendor/github.com/dlclark/regexp2/.gitignore
+++ /dev/null
@@ -1,27 +0,0 @@
-# Compiled Object files, Static and Dynamic libs (Shared Objects)
-*.o
-*.a
-*.so
-
-# Folders
-_obj
-_test
-
-# Architecture specific extensions/prefixes
-*.[568vq]
-[568vq].out
-
-*.cgo1.go
-*.cgo2.c
-_cgo_defun.c
-_cgo_gotypes.go
-_cgo_export.*
-
-_testmain.go
-
-*.exe
-*.test
-*.prof
-*.out
-
-.DS_Store
diff --git a/vendor/github.com/dlclark/regexp2/.travis.yml b/vendor/github.com/dlclark/regexp2/.travis.yml
deleted file mode 100644
index 2aa5ea1..0000000
--- a/vendor/github.com/dlclark/regexp2/.travis.yml
+++ /dev/null
@@ -1,5 +0,0 @@
-language: go
-
-go:
- - 1.9
- - tip
\ No newline at end of file
diff --git a/vendor/github.com/dlclark/regexp2/ATTRIB b/vendor/github.com/dlclark/regexp2/ATTRIB
deleted file mode 100644
index cdf4560..0000000
--- a/vendor/github.com/dlclark/regexp2/ATTRIB
+++ /dev/null
@@ -1,133 +0,0 @@
-============
-These pieces of code were ported from dotnet/corefx:
-
-syntax/charclass.go (from RegexCharClass.cs): ported to use the built-in Go unicode classes. Canonicalize is
- a direct port, but most of the other code required large changes because the C# implementation
- used a string to represent the CharSet data structure and I cleaned that up in my implementation.
-
-syntax/code.go (from RegexCode.cs): ported literally with various cleanups and layout to make it more Go-ish.
-
-syntax/escape.go (from RegexParser.cs): ported Escape method and added some optimizations. Unescape is inspired by
- the C# implementation but couldn't be directly ported because of the lack of do-while syntax in Go.
-
-syntax/parser.go (from RegexpParser.cs and RegexOptions.cs): ported parser struct and associated methods as
- literally as possible. Several language differences required changes. E.g. lack pre/post-fix increments as
- expressions, lack of do-while loops, lack of overloads, etc.
-
-syntax/prefix.go (from RegexFCD.cs and RegexBoyerMoore.cs): ported as literally as possible and added support
- for unicode chars that are longer than the 16-bit char in C# for the 32-bit rune in Go.
-
-syntax/replacerdata.go (from RegexReplacement.cs): conceptually ported and re-organized to handle differences
- in charclass implementation, and fix odd code layout between RegexParser.cs, Regex.cs, and RegexReplacement.cs.
-
-syntax/tree.go (from RegexTree.cs and RegexNode.cs): ported literally as possible.
-
-syntax/writer.go (from RegexWriter.cs): ported literally with minor changes to make it more Go-ish.
-
-match.go (from RegexMatch.cs): ported, simplified, and changed to handle Go's lack of inheritence.
-
-regexp.go (from Regex.cs and RegexOptions.cs): conceptually serves the same "starting point", but is simplified
- and changed to handle differences in C# strings and Go strings/runes.
-
-replace.go (from RegexReplacement.cs): ported closely and then cleaned up to combine the MatchEvaluator and
- simple string replace implementations.
-
-runner.go (from RegexRunner.cs): ported literally as possible.
-
-regexp_test.go (from CaptureTests.cs and GroupNamesAndNumbers.cs): conceptually ported, but the code was
- manually structured like Go tests.
-
-replace_test.go (from RegexReplaceStringTest0.cs): conceptually ported
-
-rtl_test.go (from RightToLeft.cs): conceptually ported
----
-dotnet/corefx was released under this license:
-
-The MIT License (MIT)
-
-Copyright (c) Microsoft Corporation
-
-Permission is hereby granted, free of charge, to any person obtaining a copy
-of this software and associated documentation files (the "Software"), to deal
-in the Software without restriction, including without limitation the rights
-to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
-copies of the Software, and to permit persons to whom the Software is
-furnished to do so, subject to the following conditions:
-
-The above copyright notice and this permission notice shall be included in all
-copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
-IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
-FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
-AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
-LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
-OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
-SOFTWARE.
-
-============
-These pieces of code are copied from the Go framework:
-
-- The overall directory structure of regexp2 was inspired by the Go runtime regexp package.
-- The optimization in the escape method of syntax/escape.go is from the Go runtime QuoteMeta() func in regexp/regexp.go
-- The method signatures in regexp.go are designed to match the Go framework regexp methods closely
-- func regexp2.MustCompile and func quote are almost identifical to the regexp package versions
-- BenchmarkMatch* and TestProgramTooLong* funcs in regexp_performance_test.go were copied from the framework
- regexp/exec_test.go
----
-The Go framework was released under this license:
-
-Copyright (c) 2012 The Go Authors. All rights reserved.
-
-Redistribution and use in source and binary forms, with or without
-modification, are permitted provided that the following conditions are
-met:
-
- * Redistributions of source code must retain the above copyright
-notice, this list of conditions and the following disclaimer.
- * Redistributions in binary form must reproduce the above
-copyright notice, this list of conditions and the following disclaimer
-in the documentation and/or other materials provided with the
-distribution.
- * Neither the name of Google Inc. nor the names of its
-contributors may be used to endorse or promote products derived from
-this software without specific prior written permission.
-
-THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
-"AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
-LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR
-A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT
-OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
-SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT
-LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
-DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
-THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
-(INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
-OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
-
-============
-Some test data were gathered from the Mono project.
-
-regexp_mono_test.go: ported from https://github.com/mono/mono/blob/master/mcs/class/System/Test/System.Text.RegularExpressions/PerlTrials.cs
----
-Mono tests released under this license:
-
-Permission is hereby granted, free of charge, to any person obtaining
-a copy of this software and associated documentation files (the
-"Software"), to deal in the Software without restriction, including
-without limitation the rights to use, copy, modify, merge, publish,
-distribute, sublicense, and/or sell copies of the Software, and to
-permit persons to whom the Software is furnished to do so, subject to
-the following conditions:
-
-The above copyright notice and this permission notice shall be
-included in all copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
-EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
-MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
-NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
-LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
-OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
-WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
-
diff --git a/vendor/github.com/dlclark/regexp2/LICENSE b/vendor/github.com/dlclark/regexp2/LICENSE
deleted file mode 100644
index fe83dfd..0000000
--- a/vendor/github.com/dlclark/regexp2/LICENSE
+++ /dev/null
@@ -1,21 +0,0 @@
-The MIT License (MIT)
-
-Copyright (c) Doug Clark
-
-Permission is hereby granted, free of charge, to any person obtaining a copy
-of this software and associated documentation files (the "Software"), to deal
-in the Software without restriction, including without limitation the rights
-to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
-copies of the Software, and to permit persons to whom the Software is
-furnished to do so, subject to the following conditions:
-
-The above copyright notice and this permission notice shall be included in all
-copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
-IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
-FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
-AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
-LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
-OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
-SOFTWARE.
diff --git a/vendor/github.com/dlclark/regexp2/README.md b/vendor/github.com/dlclark/regexp2/README.md
deleted file mode 100644
index 4e4abb4..0000000
--- a/vendor/github.com/dlclark/regexp2/README.md
+++ /dev/null
@@ -1,83 +0,0 @@
-# regexp2 - full featured regular expressions for Go
-Regexp2 is a feature-rich RegExp engine for Go. It doesn't have constant time guarantees like the built-in `regexp` package, but it allows backtracking and is compatible with Perl5 and .NET. You'll likely be better off with the RE2 engine from the `regexp` package and should only use this if you need to write very complex patterns or require compatibility with .NET.
-
-## Basis of the engine
-The engine is ported from the .NET framework's System.Text.RegularExpressions.Regex engine. That engine was open sourced in 2015 under the MIT license. There are some fundamental differences between .NET strings and Go strings that required a bit of borrowing from the Go framework regex engine as well. I cleaned up a couple of the dirtier bits during the port (regexcharclass.cs was terrible), but the parse tree, code emmitted, and therefore patterns matched should be identical.
-
-## Installing
-This is a go-gettable library, so install is easy:
-
- go get github.com/dlclark/regexp2/...
-
-## Usage
-Usage is similar to the Go `regexp` package. Just like in `regexp`, you start by converting a regex into a state machine via the `Compile` or `MustCompile` methods. They ultimately do the same thing, but `MustCompile` will panic if the regex is invalid. You can then use the provided `Regexp` struct to find matches repeatedly. A `Regexp` struct is safe to use across goroutines.
-
-```go
-re := regexp2.MustCompile(`Your pattern`, 0)
-if isMatch, _ := re.MatchString(`Something to match`); isMatch {
- //do something
-}
-```
-
-The only error that the `*Match*` methods *should* return is a Timeout if you set the `re.MatchTimeout` field. Any other error is a bug in the `regexp2` package. If you need more details about capture groups in a match then use the `FindStringMatch` method, like so:
-
-```go
-if m, _ := re.FindStringMatch(`Something to match`); m != nil {
- // the whole match is always group 0
- fmt.Printf("Group 0: %v\n", m.String())
-
- // you can get all the groups too
- gps := m.Groups()
-
- // a group can be captured multiple times, so each cap is separately addressable
- fmt.Printf("Group 1, first capture", gps[1].Captures[0].String())
- fmt.Printf("Group 1, second capture", gps[1].Captures[1].String())
-}
-```
-
-Group 0 is embedded in the Match. Group 0 is an automatically-assigned group that encompasses the whole pattern. This means that `m.String()` is the same as `m.Group.String()` and `m.Groups()[0].String()`
-
-The __last__ capture is embedded in each group, so `g.String()` will return the same thing as `g.Capture.String()` and `g.Captures[len(g.Captures)-1].String()`.
-
-## Compare `regexp` and `regexp2`
-| Category | regexp | regexp2 |
-| --- | --- | --- |
-| Catastrophic backtracking possible | no, constant execution time guarantees | yes, if your pattern is at risk you can use the `re.MatchTimeout` field |
-| Python-style capture groups `(?Pre)` | yes | no (yes in RE2 compat mode) |
-| .NET-style capture groups `(?re)` or `(?'name're)` | no | yes |
-| comments `(?#comment)` | no | yes |
-| branch numbering reset `(?\|a\|b)` | no | no |
-| possessive match `(?>re)` | no | yes |
-| positive lookahead `(?=re)` | no | yes |
-| negative lookahead `(?!re)` | no | yes |
-| positive lookbehind `(?<=re)` | no | yes |
-| negative lookbehind `(?re)`)
-* change singleline behavior for `$` to only match end of string (like RE2) (see [#24](https://github.com/dlclark/regexp2/issues/24))
-
-```go
-re := regexp2.MustCompile(`Your RE2-compatible pattern`, regexp2.RE2)
-if isMatch, _ := re.MatchString(`Something to match`); isMatch {
- //do something
-}
-```
-
-This feature is a work in progress and I'm open to ideas for more things to put here (maybe more relaxed character escaping rules?).
-
-
-## Library features that I'm still working on
-- Regex split
-
-## Potential bugs
-I've run a battery of tests against regexp2 from various sources and found the debug output matches the .NET engine, but .NET and Go handle strings very differently. I've attempted to handle these differences, but most of my testing deals with basic ASCII with a little bit of multi-byte Unicode. There's a chance that there are bugs in the string handling related to character sets with supplementary Unicode chars. Right-to-Left support is coded, but not well tested either.
-
-## Find a bug?
-I'm open to new issues and pull requests with tests if you find something odd!
diff --git a/vendor/github.com/dlclark/regexp2/match.go b/vendor/github.com/dlclark/regexp2/match.go
deleted file mode 100644
index 1871cff..0000000
--- a/vendor/github.com/dlclark/regexp2/match.go
+++ /dev/null
@@ -1,347 +0,0 @@
-package regexp2
-
-import (
- "bytes"
- "fmt"
-)
-
-// Match is a single regex result match that contains groups and repeated captures
-// -Groups
-// -Capture
-type Match struct {
- Group //embeded group 0
-
- regex *Regexp
- otherGroups []Group
-
- // input to the match
- textpos int
- textstart int
-
- capcount int
- caps []int
- sparseCaps map[int]int
-
- // output from the match
- matches [][]int
- matchcount []int
-
- // whether we've done any balancing with this match. If we
- // have done balancing, we'll need to do extra work in Tidy().
- balancing bool
-}
-
-// Group is an explicit or implit (group 0) matched group within the pattern
-type Group struct {
- Capture // the last capture of this group is embeded for ease of use
-
- Name string // group name
- Captures []Capture // captures of this group
-}
-
-// Capture is a single capture of text within the larger original string
-type Capture struct {
- // the original string
- text []rune
- // the position in the original string where the first character of
- // captured substring was found.
- Index int
- // the length of the captured substring.
- Length int
-}
-
-// String returns the captured text as a String
-func (c *Capture) String() string {
- return string(c.text[c.Index : c.Index+c.Length])
-}
-
-// Runes returns the captured text as a rune slice
-func (c *Capture) Runes() []rune {
- return c.text[c.Index : c.Index+c.Length]
-}
-
-func newMatch(regex *Regexp, capcount int, text []rune, startpos int) *Match {
- m := Match{
- regex: regex,
- matchcount: make([]int, capcount),
- matches: make([][]int, capcount),
- textstart: startpos,
- balancing: false,
- }
- m.Name = "0"
- m.text = text
- m.matches[0] = make([]int, 2)
- return &m
-}
-
-func newMatchSparse(regex *Regexp, caps map[int]int, capcount int, text []rune, startpos int) *Match {
- m := newMatch(regex, capcount, text, startpos)
- m.sparseCaps = caps
- return m
-}
-
-func (m *Match) reset(text []rune, textstart int) {
- m.text = text
- m.textstart = textstart
- for i := 0; i < len(m.matchcount); i++ {
- m.matchcount[i] = 0
- }
- m.balancing = false
-}
-
-func (m *Match) tidy(textpos int) {
-
- interval := m.matches[0]
- m.Index = interval[0]
- m.Length = interval[1]
- m.textpos = textpos
- m.capcount = m.matchcount[0]
- //copy our root capture to the list
- m.Group.Captures = []Capture{m.Group.Capture}
-
- if m.balancing {
- // The idea here is that we want to compact all of our unbalanced captures. To do that we
- // use j basically as a count of how many unbalanced captures we have at any given time
- // (really j is an index, but j/2 is the count). First we skip past all of the real captures
- // until we find a balance captures. Then we check each subsequent entry. If it's a balance
- // capture (it's negative), we decrement j. If it's a real capture, we increment j and copy
- // it down to the last free position.
- for cap := 0; cap < len(m.matchcount); cap++ {
- limit := m.matchcount[cap] * 2
- matcharray := m.matches[cap]
-
- var i, j int
-
- for i = 0; i < limit; i++ {
- if matcharray[i] < 0 {
- break
- }
- }
-
- for j = i; i < limit; i++ {
- if matcharray[i] < 0 {
- // skip negative values
- j--
- } else {
- // but if we find something positive (an actual capture), copy it back to the last
- // unbalanced position.
- if i != j {
- matcharray[j] = matcharray[i]
- }
- j++
- }
- }
-
- m.matchcount[cap] = j / 2
- }
-
- m.balancing = false
- }
-}
-
-// isMatched tells if a group was matched by capnum
-func (m *Match) isMatched(cap int) bool {
- return cap < len(m.matchcount) && m.matchcount[cap] > 0 && m.matches[cap][m.matchcount[cap]*2-1] != (-3+1)
-}
-
-// matchIndex returns the index of the last specified matched group by capnum
-func (m *Match) matchIndex(cap int) int {
- i := m.matches[cap][m.matchcount[cap]*2-2]
- if i >= 0 {
- return i
- }
-
- return m.matches[cap][-3-i]
-}
-
-// matchLength returns the length of the last specified matched group by capnum
-func (m *Match) matchLength(cap int) int {
- i := m.matches[cap][m.matchcount[cap]*2-1]
- if i >= 0 {
- return i
- }
-
- return m.matches[cap][-3-i]
-}
-
-// Nonpublic builder: add a capture to the group specified by "c"
-func (m *Match) addMatch(c, start, l int) {
-
- if m.matches[c] == nil {
- m.matches[c] = make([]int, 2)
- }
-
- capcount := m.matchcount[c]
-
- if capcount*2+2 > len(m.matches[c]) {
- oldmatches := m.matches[c]
- newmatches := make([]int, capcount*8)
- copy(newmatches, oldmatches[:capcount*2])
- m.matches[c] = newmatches
- }
-
- m.matches[c][capcount*2] = start
- m.matches[c][capcount*2+1] = l
- m.matchcount[c] = capcount + 1
- //log.Printf("addMatch: c=%v, i=%v, l=%v ... matches: %v", c, start, l, m.matches)
-}
-
-// Nonpublic builder: Add a capture to balance the specified group. This is used by the
-// balanced match construct. (?...)
-//
-// If there were no such thing as backtracking, this would be as simple as calling RemoveMatch(c).
-// However, since we have backtracking, we need to keep track of everything.
-func (m *Match) balanceMatch(c int) {
- m.balancing = true
-
- // we'll look at the last capture first
- capcount := m.matchcount[c]
- target := capcount*2 - 2
-
- // first see if it is negative, and therefore is a reference to the next available
- // capture group for balancing. If it is, we'll reset target to point to that capture.
- if m.matches[c][target] < 0 {
- target = -3 - m.matches[c][target]
- }
-
- // move back to the previous capture
- target -= 2
-
- // if the previous capture is a reference, just copy that reference to the end. Otherwise, point to it.
- if target >= 0 && m.matches[c][target] < 0 {
- m.addMatch(c, m.matches[c][target], m.matches[c][target+1])
- } else {
- m.addMatch(c, -3-target, -4-target /* == -3 - (target + 1) */)
- }
-}
-
-// Nonpublic builder: removes a group match by capnum
-func (m *Match) removeMatch(c int) {
- m.matchcount[c]--
-}
-
-// GroupCount returns the number of groups this match has matched
-func (m *Match) GroupCount() int {
- return len(m.matchcount)
-}
-
-// GroupByName returns a group based on the name of the group, or nil if the group name does not exist
-func (m *Match) GroupByName(name string) *Group {
- num := m.regex.GroupNumberFromName(name)
- if num < 0 {
- return nil
- }
- return m.GroupByNumber(num)
-}
-
-// GroupByNumber returns a group based on the number of the group, or nil if the group number does not exist
-func (m *Match) GroupByNumber(num int) *Group {
- // check our sparse map
- if m.sparseCaps != nil {
- if newNum, ok := m.sparseCaps[num]; ok {
- num = newNum
- }
- }
- if num >= len(m.matchcount) || num < 0 {
- return nil
- }
-
- if num == 0 {
- return &m.Group
- }
-
- m.populateOtherGroups()
-
- return &m.otherGroups[num-1]
-}
-
-// Groups returns all the capture groups, starting with group 0 (the full match)
-func (m *Match) Groups() []Group {
- m.populateOtherGroups()
- g := make([]Group, len(m.otherGroups)+1)
- g[0] = m.Group
- copy(g[1:], m.otherGroups)
- return g
-}
-
-func (m *Match) populateOtherGroups() {
- // Construct all the Group objects first time called
- if m.otherGroups == nil {
- m.otherGroups = make([]Group, len(m.matchcount)-1)
- for i := 0; i < len(m.otherGroups); i++ {
- m.otherGroups[i] = newGroup(m.regex.GroupNameFromNumber(i+1), m.text, m.matches[i+1], m.matchcount[i+1])
- }
- }
-}
-
-func (m *Match) groupValueAppendToBuf(groupnum int, buf *bytes.Buffer) {
- c := m.matchcount[groupnum]
- if c == 0 {
- return
- }
-
- matches := m.matches[groupnum]
-
- index := matches[(c-1)*2]
- last := index + matches[(c*2)-1]
-
- for ; index < last; index++ {
- buf.WriteRune(m.text[index])
- }
-}
-
-func newGroup(name string, text []rune, caps []int, capcount int) Group {
- g := Group{}
- g.text = text
- if capcount > 0 {
- g.Index = caps[(capcount-1)*2]
- g.Length = caps[(capcount*2)-1]
- }
- g.Name = name
- g.Captures = make([]Capture, capcount)
- for i := 0; i < capcount; i++ {
- g.Captures[i] = Capture{
- text: text,
- Index: caps[i*2],
- Length: caps[i*2+1],
- }
- }
- //log.Printf("newGroup! capcount %v, %+v", capcount, g)
-
- return g
-}
-
-func (m *Match) dump() string {
- buf := &bytes.Buffer{}
- buf.WriteRune('\n')
- if len(m.sparseCaps) > 0 {
- for k, v := range m.sparseCaps {
- fmt.Fprintf(buf, "Slot %v -> %v\n", k, v)
- }
- }
-
- for i, g := range m.Groups() {
- fmt.Fprintf(buf, "Group %v (%v), %v caps:\n", i, g.Name, len(g.Captures))
-
- for _, c := range g.Captures {
- fmt.Fprintf(buf, " (%v, %v) %v\n", c.Index, c.Length, c.String())
- }
- }
- /*
- for i := 0; i < len(m.matchcount); i++ {
- fmt.Fprintf(buf, "\nGroup %v (%v):\n", i, m.regex.GroupNameFromNumber(i))
-
- for j := 0; j < m.matchcount[i]; j++ {
- text := ""
-
- if m.matches[i][j*2] >= 0 {
- start := m.matches[i][j*2]
- text = m.text[start : start+m.matches[i][j*2+1]]
- }
-
- fmt.Fprintf(buf, " (%v, %v) %v\n", m.matches[i][j*2], m.matches[i][j*2+1], text)
- }
- }
- */
- return buf.String()
-}
diff --git a/vendor/github.com/dlclark/regexp2/regexp.go b/vendor/github.com/dlclark/regexp2/regexp.go
deleted file mode 100644
index 7c7b01d..0000000
--- a/vendor/github.com/dlclark/regexp2/regexp.go
+++ /dev/null
@@ -1,355 +0,0 @@
-/*
-Package regexp2 is a regexp package that has an interface similar to Go's framework regexp engine but uses a
-more feature full regex engine behind the scenes.
-
-It doesn't have constant time guarantees, but it allows backtracking and is compatible with Perl5 and .NET.
-You'll likely be better off with the RE2 engine from the regexp package and should only use this if you
-need to write very complex patterns or require compatibility with .NET.
-*/
-package regexp2
-
-import (
- "errors"
- "math"
- "strconv"
- "sync"
- "time"
-
- "github.com/dlclark/regexp2/syntax"
-)
-
-// Default timeout used when running regexp matches -- "forever"
-var DefaultMatchTimeout = time.Duration(math.MaxInt64)
-
-// Regexp is the representation of a compiled regular expression.
-// A Regexp is safe for concurrent use by multiple goroutines.
-type Regexp struct {
- //timeout when trying to find matches
- MatchTimeout time.Duration
-
- // read-only after Compile
- pattern string // as passed to Compile
- options RegexOptions // options
-
- caps map[int]int // capnum->index
- capnames map[string]int //capture group name -> index
- capslist []string //sorted list of capture group names
- capsize int // size of the capture array
-
- code *syntax.Code // compiled program
-
- // cache of machines for running regexp
- muRun sync.Mutex
- runner []*runner
-}
-
-// Compile parses a regular expression and returns, if successful,
-// a Regexp object that can be used to match against text.
-func Compile(expr string, opt RegexOptions) (*Regexp, error) {
- // parse it
- tree, err := syntax.Parse(expr, syntax.RegexOptions(opt))
- if err != nil {
- return nil, err
- }
-
- // translate it to code
- code, err := syntax.Write(tree)
- if err != nil {
- return nil, err
- }
-
- // return it
- return &Regexp{
- pattern: expr,
- options: opt,
- caps: code.Caps,
- capnames: tree.Capnames,
- capslist: tree.Caplist,
- capsize: code.Capsize,
- code: code,
- MatchTimeout: DefaultMatchTimeout,
- }, nil
-}
-
-// MustCompile is like Compile but panics if the expression cannot be parsed.
-// It simplifies safe initialization of global variables holding compiled regular
-// expressions.
-func MustCompile(str string, opt RegexOptions) *Regexp {
- regexp, error := Compile(str, opt)
- if error != nil {
- panic(`regexp2: Compile(` + quote(str) + `): ` + error.Error())
- }
- return regexp
-}
-
-// Escape adds backslashes to any special characters in the input string
-func Escape(input string) string {
- return syntax.Escape(input)
-}
-
-// Unescape removes any backslashes from previously-escaped special characters in the input string
-func Unescape(input string) (string, error) {
- return syntax.Unescape(input)
-}
-
-// String returns the source text used to compile the regular expression.
-func (re *Regexp) String() string {
- return re.pattern
-}
-
-func quote(s string) string {
- if strconv.CanBackquote(s) {
- return "`" + s + "`"
- }
- return strconv.Quote(s)
-}
-
-// RegexOptions impact the runtime and parsing behavior
-// for each specific regex. They are setable in code as well
-// as in the regex pattern itself.
-type RegexOptions int32
-
-const (
- None RegexOptions = 0x0
- IgnoreCase = 0x0001 // "i"
- Multiline = 0x0002 // "m"
- ExplicitCapture = 0x0004 // "n"
- Compiled = 0x0008 // "c"
- Singleline = 0x0010 // "s"
- IgnorePatternWhitespace = 0x0020 // "x"
- RightToLeft = 0x0040 // "r"
- Debug = 0x0080 // "d"
- ECMAScript = 0x0100 // "e"
- RE2 = 0x0200 // RE2 (regexp package) compatibility mode
-)
-
-func (re *Regexp) RightToLeft() bool {
- return re.options&RightToLeft != 0
-}
-
-func (re *Regexp) Debug() bool {
- return re.options&Debug != 0
-}
-
-// Replace searches the input string and replaces each match found with the replacement text.
-// Count will limit the number of matches attempted and startAt will allow
-// us to skip past possible matches at the start of the input (left or right depending on RightToLeft option).
-// Set startAt and count to -1 to go through the whole string
-func (re *Regexp) Replace(input, replacement string, startAt, count int) (string, error) {
- data, err := syntax.NewReplacerData(replacement, re.caps, re.capsize, re.capnames, syntax.RegexOptions(re.options))
- if err != nil {
- return "", err
- }
- //TODO: cache ReplacerData
-
- return replace(re, data, nil, input, startAt, count)
-}
-
-// ReplaceFunc searches the input string and replaces each match found using the string from the evaluator
-// Count will limit the number of matches attempted and startAt will allow
-// us to skip past possible matches at the start of the input (left or right depending on RightToLeft option).
-// Set startAt and count to -1 to go through the whole string.
-func (re *Regexp) ReplaceFunc(input string, evaluator MatchEvaluator, startAt, count int) (string, error) {
- return replace(re, nil, evaluator, input, startAt, count)
-}
-
-// FindStringMatch searches the input string for a Regexp match
-func (re *Regexp) FindStringMatch(s string) (*Match, error) {
- // convert string to runes
- return re.run(false, -1, getRunes(s))
-}
-
-// FindRunesMatch searches the input rune slice for a Regexp match
-func (re *Regexp) FindRunesMatch(r []rune) (*Match, error) {
- return re.run(false, -1, r)
-}
-
-// FindStringMatchStartingAt searches the input string for a Regexp match starting at the startAt index
-func (re *Regexp) FindStringMatchStartingAt(s string, startAt int) (*Match, error) {
- if startAt > len(s) {
- return nil, errors.New("startAt must be less than the length of the input string")
- }
- r, startAt := re.getRunesAndStart(s, startAt)
- if startAt == -1 {
- // we didn't find our start index in the string -- that's a problem
- return nil, errors.New("startAt must align to the start of a valid rune in the input string")
- }
-
- return re.run(false, startAt, r)
-}
-
-// FindRunesMatchStartingAt searches the input rune slice for a Regexp match starting at the startAt index
-func (re *Regexp) FindRunesMatchStartingAt(r []rune, startAt int) (*Match, error) {
- return re.run(false, startAt, r)
-}
-
-// FindNextMatch returns the next match in the same input string as the match parameter.
-// Will return nil if there is no next match or if given a nil match.
-func (re *Regexp) FindNextMatch(m *Match) (*Match, error) {
- if m == nil {
- return nil, nil
- }
-
- // If previous match was empty, advance by one before matching to prevent
- // infinite loop
- startAt := m.textpos
- if m.Length == 0 {
- if m.textpos == len(m.text) {
- return nil, nil
- }
-
- if re.RightToLeft() {
- startAt--
- } else {
- startAt++
- }
- }
- return re.run(false, startAt, m.text)
-}
-
-// MatchString return true if the string matches the regex
-// error will be set if a timeout occurs
-func (re *Regexp) MatchString(s string) (bool, error) {
- m, err := re.run(true, -1, getRunes(s))
- if err != nil {
- return false, err
- }
- return m != nil, nil
-}
-
-func (re *Regexp) getRunesAndStart(s string, startAt int) ([]rune, int) {
- if startAt < 0 {
- if re.RightToLeft() {
- r := getRunes(s)
- return r, len(r)
- }
- return getRunes(s), 0
- }
- ret := make([]rune, len(s))
- i := 0
- runeIdx := -1
- for strIdx, r := range s {
- if strIdx == startAt {
- runeIdx = i
- }
- ret[i] = r
- i++
- }
- if startAt == len(s) {
- runeIdx = i
- }
- return ret[:i], runeIdx
-}
-
-func getRunes(s string) []rune {
- return []rune(s)
-}
-
-// MatchRunes return true if the runes matches the regex
-// error will be set if a timeout occurs
-func (re *Regexp) MatchRunes(r []rune) (bool, error) {
- m, err := re.run(true, -1, r)
- if err != nil {
- return false, err
- }
- return m != nil, nil
-}
-
-// GetGroupNames Returns the set of strings used to name capturing groups in the expression.
-func (re *Regexp) GetGroupNames() []string {
- var result []string
-
- if re.capslist == nil {
- result = make([]string, re.capsize)
-
- for i := 0; i < len(result); i++ {
- result[i] = strconv.Itoa(i)
- }
- } else {
- result = make([]string, len(re.capslist))
- copy(result, re.capslist)
- }
-
- return result
-}
-
-// GetGroupNumbers returns the integer group numbers corresponding to a group name.
-func (re *Regexp) GetGroupNumbers() []int {
- var result []int
-
- if re.caps == nil {
- result = make([]int, re.capsize)
-
- for i := 0; i < len(result); i++ {
- result[i] = i
- }
- } else {
- result = make([]int, len(re.caps))
-
- for k, v := range re.caps {
- result[v] = k
- }
- }
-
- return result
-}
-
-// GroupNameFromNumber retrieves a group name that corresponds to a group number.
-// It will return "" for and unknown group number. Unnamed groups automatically
-// receive a name that is the decimal string equivalent of its number.
-func (re *Regexp) GroupNameFromNumber(i int) string {
- if re.capslist == nil {
- if i >= 0 && i < re.capsize {
- return strconv.Itoa(i)
- }
-
- return ""
- }
-
- if re.caps != nil {
- var ok bool
- if i, ok = re.caps[i]; !ok {
- return ""
- }
- }
-
- if i >= 0 && i < len(re.capslist) {
- return re.capslist[i]
- }
-
- return ""
-}
-
-// GroupNumberFromName returns a group number that corresponds to a group name.
-// Returns -1 if the name is not a recognized group name. Numbered groups
-// automatically get a group name that is the decimal string equivalent of its number.
-func (re *Regexp) GroupNumberFromName(name string) int {
- // look up name if we have a hashtable of names
- if re.capnames != nil {
- if k, ok := re.capnames[name]; ok {
- return k
- }
-
- return -1
- }
-
- // convert to an int if it looks like a number
- result := 0
- for i := 0; i < len(name); i++ {
- ch := name[i]
-
- if ch > '9' || ch < '0' {
- return -1
- }
-
- result *= 10
- result += int(ch - '0')
- }
-
- // return int if it's in range
- if result >= 0 && result < re.capsize {
- return result
- }
-
- return -1
-}
diff --git a/vendor/github.com/dlclark/regexp2/replace.go b/vendor/github.com/dlclark/regexp2/replace.go
deleted file mode 100644
index 0376bd9..0000000
--- a/vendor/github.com/dlclark/regexp2/replace.go
+++ /dev/null
@@ -1,177 +0,0 @@
-package regexp2
-
-import (
- "bytes"
- "errors"
-
- "github.com/dlclark/regexp2/syntax"
-)
-
-const (
- replaceSpecials = 4
- replaceLeftPortion = -1
- replaceRightPortion = -2
- replaceLastGroup = -3
- replaceWholeString = -4
-)
-
-// MatchEvaluator is a function that takes a match and returns a replacement string to be used
-type MatchEvaluator func(Match) string
-
-// Three very similar algorithms appear below: replace (pattern),
-// replace (evaluator), and split.
-
-// Replace Replaces all occurrences of the regex in the string with the
-// replacement pattern.
-//
-// Note that the special case of no matches is handled on its own:
-// with no matches, the input string is returned unchanged.
-// The right-to-left case is split out because StringBuilder
-// doesn't handle right-to-left string building directly very well.
-func replace(regex *Regexp, data *syntax.ReplacerData, evaluator MatchEvaluator, input string, startAt, count int) (string, error) {
- if count < -1 {
- return "", errors.New("Count too small")
- }
- if count == 0 {
- return "", nil
- }
-
- m, err := regex.FindStringMatchStartingAt(input, startAt)
-
- if err != nil {
- return "", err
- }
- if m == nil {
- return input, nil
- }
-
- buf := &bytes.Buffer{}
- text := m.text
-
- if !regex.RightToLeft() {
- prevat := 0
- for m != nil {
- if m.Index != prevat {
- buf.WriteString(string(text[prevat:m.Index]))
- }
- prevat = m.Index + m.Length
- if evaluator == nil {
- replacementImpl(data, buf, m)
- } else {
- buf.WriteString(evaluator(*m))
- }
-
- count--
- if count == 0 {
- break
- }
- m, err = regex.FindNextMatch(m)
- if err != nil {
- return "", nil
- }
- }
-
- if prevat < len(text) {
- buf.WriteString(string(text[prevat:]))
- }
- } else {
- prevat := len(text)
- var al []string
-
- for m != nil {
- if m.Index+m.Length != prevat {
- al = append(al, string(text[m.Index+m.Length:prevat]))
- }
- prevat = m.Index
- if evaluator == nil {
- replacementImplRTL(data, &al, m)
- } else {
- al = append(al, evaluator(*m))
- }
-
- count--
- if count == 0 {
- break
- }
- m, err = regex.FindNextMatch(m)
- if err != nil {
- return "", nil
- }
- }
-
- if prevat > 0 {
- buf.WriteString(string(text[:prevat]))
- }
-
- for i := len(al) - 1; i >= 0; i-- {
- buf.WriteString(al[i])
- }
- }
-
- return buf.String(), nil
-}
-
-// Given a Match, emits into the StringBuilder the evaluated
-// substitution pattern.
-func replacementImpl(data *syntax.ReplacerData, buf *bytes.Buffer, m *Match) {
- for _, r := range data.Rules {
-
- if r >= 0 { // string lookup
- buf.WriteString(data.Strings[r])
- } else if r < -replaceSpecials { // group lookup
- m.groupValueAppendToBuf(-replaceSpecials-1-r, buf)
- } else {
- switch -replaceSpecials - 1 - r { // special insertion patterns
- case replaceLeftPortion:
- for i := 0; i < m.Index; i++ {
- buf.WriteRune(m.text[i])
- }
- case replaceRightPortion:
- for i := m.Index + m.Length; i < len(m.text); i++ {
- buf.WriteRune(m.text[i])
- }
- case replaceLastGroup:
- m.groupValueAppendToBuf(m.GroupCount()-1, buf)
- case replaceWholeString:
- for i := 0; i < len(m.text); i++ {
- buf.WriteRune(m.text[i])
- }
- }
- }
- }
-}
-
-func replacementImplRTL(data *syntax.ReplacerData, al *[]string, m *Match) {
- l := *al
- buf := &bytes.Buffer{}
-
- for _, r := range data.Rules {
- buf.Reset()
- if r >= 0 { // string lookup
- l = append(l, data.Strings[r])
- } else if r < -replaceSpecials { // group lookup
- m.groupValueAppendToBuf(-replaceSpecials-1-r, buf)
- l = append(l, buf.String())
- } else {
- switch -replaceSpecials - 1 - r { // special insertion patterns
- case replaceLeftPortion:
- for i := 0; i < m.Index; i++ {
- buf.WriteRune(m.text[i])
- }
- case replaceRightPortion:
- for i := m.Index + m.Length; i < len(m.text); i++ {
- buf.WriteRune(m.text[i])
- }
- case replaceLastGroup:
- m.groupValueAppendToBuf(m.GroupCount()-1, buf)
- case replaceWholeString:
- for i := 0; i < len(m.text); i++ {
- buf.WriteRune(m.text[i])
- }
- }
- l = append(l, buf.String())
- }
- }
-
- *al = l
-}
diff --git a/vendor/github.com/dlclark/regexp2/runner.go b/vendor/github.com/dlclark/regexp2/runner.go
deleted file mode 100644
index 4d7f9b0..0000000
--- a/vendor/github.com/dlclark/regexp2/runner.go
+++ /dev/null
@@ -1,1634 +0,0 @@
-package regexp2
-
-import (
- "bytes"
- "errors"
- "fmt"
- "math"
- "strconv"
- "strings"
- "time"
- "unicode"
-
- "github.com/dlclark/regexp2/syntax"
-)
-
-type runner struct {
- re *Regexp
- code *syntax.Code
-
- runtextstart int // starting point for search
-
- runtext []rune // text to search
- runtextpos int // current position in text
- runtextend int
-
- // The backtracking stack. Opcodes use this to store data regarding
- // what they have matched and where to backtrack to. Each "frame" on
- // the stack takes the form of [CodePosition Data1 Data2...], where
- // CodePosition is the position of the current opcode and
- // the data values are all optional. The CodePosition can be negative, and
- // these values (also called "back2") are used by the BranchMark family of opcodes
- // to indicate whether they are backtracking after a successful or failed
- // match.
- // When we backtrack, we pop the CodePosition off the stack, set the current
- // instruction pointer to that code position, and mark the opcode
- // with a backtracking flag ("Back"). Each opcode then knows how to
- // handle its own data.
- runtrack []int
- runtrackpos int
-
- // This stack is used to track text positions across different opcodes.
- // For example, in /(a*b)+/, the parentheses result in a SetMark/CaptureMark
- // pair. SetMark records the text position before we match a*b. Then
- // CaptureMark uses that position to figure out where the capture starts.
- // Opcodes which push onto this stack are always paired with other opcodes
- // which will pop the value from it later. A successful match should mean
- // that this stack is empty.
- runstack []int
- runstackpos int
-
- // The crawl stack is used to keep track of captures. Every time a group
- // has a capture, we push its group number onto the runcrawl stack. In
- // the case of a balanced match, we push BOTH groups onto the stack.
- runcrawl []int
- runcrawlpos int
-
- runtrackcount int // count of states that may do backtracking
-
- runmatch *Match // result object
-
- ignoreTimeout bool
- timeout time.Duration // timeout in milliseconds (needed for actual)
- timeoutChecksToSkip int
- timeoutAt time.Time
-
- operator syntax.InstOp
- codepos int
- rightToLeft bool
- caseInsensitive bool
-}
-
-// run searches for matches and can continue from the previous match
-//
-// quick is usually false, but can be true to not return matches, just put it in caches
-// textstart is -1 to start at the "beginning" (depending on Right-To-Left), otherwise an index in input
-// input is the string to search for our regex pattern
-func (re *Regexp) run(quick bool, textstart int, input []rune) (*Match, error) {
-
- // get a cached runner
- runner := re.getRunner()
- defer re.putRunner(runner)
-
- if textstart < 0 {
- if re.RightToLeft() {
- textstart = len(input)
- } else {
- textstart = 0
- }
- }
-
- return runner.scan(input, textstart, quick, re.MatchTimeout)
-}
-
-// Scans the string to find the first match. Uses the Match object
-// both to feed text in and as a place to store matches that come out.
-//
-// All the action is in the Go() method. Our
-// responsibility is to load up the class members before
-// calling Go.
-//
-// The optimizer can compute a set of candidate starting characters,
-// and we could use a separate method Skip() that will quickly scan past
-// any characters that we know can't match.
-func (r *runner) scan(rt []rune, textstart int, quick bool, timeout time.Duration) (*Match, error) {
- r.timeout = timeout
- r.ignoreTimeout = (time.Duration(math.MaxInt64) == timeout)
- r.runtextstart = textstart
- r.runtext = rt
- r.runtextend = len(rt)
-
- stoppos := r.runtextend
- bump := 1
-
- if r.re.RightToLeft() {
- bump = -1
- stoppos = 0
- }
-
- r.runtextpos = textstart
- initted := false
-
- r.startTimeoutWatch()
- for {
- if r.re.Debug() {
- //fmt.Printf("\nSearch content: %v\n", string(r.runtext))
- fmt.Printf("\nSearch range: from 0 to %v\n", r.runtextend)
- fmt.Printf("Firstchar search starting at %v stopping at %v\n", r.runtextpos, stoppos)
- }
-
- if r.findFirstChar() {
- if err := r.checkTimeout(); err != nil {
- return nil, err
- }
-
- if !initted {
- r.initMatch()
- initted = true
- }
-
- if r.re.Debug() {
- fmt.Printf("Executing engine starting at %v\n\n", r.runtextpos)
- }
-
- if err := r.execute(); err != nil {
- return nil, err
- }
-
- if r.runmatch.matchcount[0] > 0 {
- // We'll return a match even if it touches a previous empty match
- return r.tidyMatch(quick), nil
- }
-
- // reset state for another go
- r.runtrackpos = len(r.runtrack)
- r.runstackpos = len(r.runstack)
- r.runcrawlpos = len(r.runcrawl)
- }
-
- // failure!
-
- if r.runtextpos == stoppos {
- r.tidyMatch(true)
- return nil, nil
- }
-
- // Recognize leading []* and various anchors, and bump on failure accordingly
-
- // r.bump by one and start again
-
- r.runtextpos += bump
- }
- // We never get here
-}
-
-func (r *runner) execute() error {
-
- r.goTo(0)
-
- for {
-
- if r.re.Debug() {
- r.dumpState()
- }
-
- if err := r.checkTimeout(); err != nil {
- return err
- }
-
- switch r.operator {
- case syntax.Stop:
- return nil
-
- case syntax.Nothing:
- break
-
- case syntax.Goto:
- r.goTo(r.operand(0))
- continue
-
- case syntax.Testref:
- if !r.runmatch.isMatched(r.operand(0)) {
- break
- }
- r.advance(1)
- continue
-
- case syntax.Lazybranch:
- r.trackPush1(r.textPos())
- r.advance(1)
- continue
-
- case syntax.Lazybranch | syntax.Back:
- r.trackPop()
- r.textto(r.trackPeek())
- r.goTo(r.operand(0))
- continue
-
- case syntax.Setmark:
- r.stackPush(r.textPos())
- r.trackPush()
- r.advance(0)
- continue
-
- case syntax.Nullmark:
- r.stackPush(-1)
- r.trackPush()
- r.advance(0)
- continue
-
- case syntax.Setmark | syntax.Back, syntax.Nullmark | syntax.Back:
- r.stackPop()
- break
-
- case syntax.Getmark:
- r.stackPop()
- r.trackPush1(r.stackPeek())
- r.textto(r.stackPeek())
- r.advance(0)
- continue
-
- case syntax.Getmark | syntax.Back:
- r.trackPop()
- r.stackPush(r.trackPeek())
- break
-
- case syntax.Capturemark:
- if r.operand(1) != -1 && !r.runmatch.isMatched(r.operand(1)) {
- break
- }
- r.stackPop()
- if r.operand(1) != -1 {
- r.transferCapture(r.operand(0), r.operand(1), r.stackPeek(), r.textPos())
- } else {
- r.capture(r.operand(0), r.stackPeek(), r.textPos())
- }
- r.trackPush1(r.stackPeek())
-
- r.advance(2)
-
- continue
-
- case syntax.Capturemark | syntax.Back:
- r.trackPop()
- r.stackPush(r.trackPeek())
- r.uncapture()
- if r.operand(0) != -1 && r.operand(1) != -1 {
- r.uncapture()
- }
-
- break
-
- case syntax.Branchmark:
- r.stackPop()
-
- matched := r.textPos() - r.stackPeek()
-
- if matched != 0 { // Nonempty match -> loop now
- r.trackPush2(r.stackPeek(), r.textPos()) // Save old mark, textpos
- r.stackPush(r.textPos()) // Make new mark
- r.goTo(r.operand(0)) // Loop
- } else { // Empty match -> straight now
- r.trackPushNeg1(r.stackPeek()) // Save old mark
- r.advance(1) // Straight
- }
- continue
-
- case syntax.Branchmark | syntax.Back:
- r.trackPopN(2)
- r.stackPop()
- r.textto(r.trackPeekN(1)) // Recall position
- r.trackPushNeg1(r.trackPeek()) // Save old mark
- r.advance(1) // Straight
- continue
-
- case syntax.Branchmark | syntax.Back2:
- r.trackPop()
- r.stackPush(r.trackPeek()) // Recall old mark
- break // Backtrack
-
- case syntax.Lazybranchmark:
- {
- // We hit this the first time through a lazy loop and after each
- // successful match of the inner expression. It simply continues
- // on and doesn't loop.
- r.stackPop()
-
- oldMarkPos := r.stackPeek()
-
- if r.textPos() != oldMarkPos { // Nonempty match -> try to loop again by going to 'back' state
- if oldMarkPos != -1 {
- r.trackPush2(oldMarkPos, r.textPos()) // Save old mark, textpos
- } else {
- r.trackPush2(r.textPos(), r.textPos())
- }
- } else {
- // The inner expression found an empty match, so we'll go directly to 'back2' if we
- // backtrack. In this case, we need to push something on the stack, since back2 pops.
- // However, in the case of ()+? or similar, this empty match may be legitimate, so push the text
- // position associated with that empty match.
- r.stackPush(oldMarkPos)
-
- r.trackPushNeg1(r.stackPeek()) // Save old mark
- }
- r.advance(1)
- continue
- }
-
- case syntax.Lazybranchmark | syntax.Back:
-
- // After the first time, Lazybranchmark | syntax.Back occurs
- // with each iteration of the loop, and therefore with every attempted
- // match of the inner expression. We'll try to match the inner expression,
- // then go back to Lazybranchmark if successful. If the inner expression
- // fails, we go to Lazybranchmark | syntax.Back2
-
- r.trackPopN(2)
- pos := r.trackPeekN(1)
- r.trackPushNeg1(r.trackPeek()) // Save old mark
- r.stackPush(pos) // Make new mark
- r.textto(pos) // Recall position
- r.goTo(r.operand(0)) // Loop
- continue
-
- case syntax.Lazybranchmark | syntax.Back2:
- // The lazy loop has failed. We'll do a true backtrack and
- // start over before the lazy loop.
- r.stackPop()
- r.trackPop()
- r.stackPush(r.trackPeek()) // Recall old mark
- break
-
- case syntax.Setcount:
- r.stackPush2(r.textPos(), r.operand(0))
- r.trackPush()
- r.advance(1)
- continue
-
- case syntax.Nullcount:
- r.stackPush2(-1, r.operand(0))
- r.trackPush()
- r.advance(1)
- continue
-
- case syntax.Setcount | syntax.Back:
- r.stackPopN(2)
- break
-
- case syntax.Nullcount | syntax.Back:
- r.stackPopN(2)
- break
-
- case syntax.Branchcount:
- // r.stackPush:
- // 0: Mark
- // 1: Count
-
- r.stackPopN(2)
- mark := r.stackPeek()
- count := r.stackPeekN(1)
- matched := r.textPos() - mark
-
- if count >= r.operand(1) || (matched == 0 && count >= 0) { // Max loops or empty match -> straight now
- r.trackPushNeg2(mark, count) // Save old mark, count
- r.advance(2) // Straight
- } else { // Nonempty match -> count+loop now
- r.trackPush1(mark) // remember mark
- r.stackPush2(r.textPos(), count+1) // Make new mark, incr count
- r.goTo(r.operand(0)) // Loop
- }
- continue
-
- case syntax.Branchcount | syntax.Back:
- // r.trackPush:
- // 0: Previous mark
- // r.stackPush:
- // 0: Mark (= current pos, discarded)
- // 1: Count
- r.trackPop()
- r.stackPopN(2)
- if r.stackPeekN(1) > 0 { // Positive -> can go straight
- r.textto(r.stackPeek()) // Zap to mark
- r.trackPushNeg2(r.trackPeek(), r.stackPeekN(1)-1) // Save old mark, old count
- r.advance(2) // Straight
- continue
- }
- r.stackPush2(r.trackPeek(), r.stackPeekN(1)-1) // recall old mark, old count
- break
-
- case syntax.Branchcount | syntax.Back2:
- // r.trackPush:
- // 0: Previous mark
- // 1: Previous count
- r.trackPopN(2)
- r.stackPush2(r.trackPeek(), r.trackPeekN(1)) // Recall old mark, old count
- break // Backtrack
-
- case syntax.Lazybranchcount:
- // r.stackPush:
- // 0: Mark
- // 1: Count
-
- r.stackPopN(2)
- mark := r.stackPeek()
- count := r.stackPeekN(1)
-
- if count < 0 { // Negative count -> loop now
- r.trackPushNeg1(mark) // Save old mark
- r.stackPush2(r.textPos(), count+1) // Make new mark, incr count
- r.goTo(r.operand(0)) // Loop
- } else { // Nonneg count -> straight now
- r.trackPush3(mark, count, r.textPos()) // Save mark, count, position
- r.advance(2) // Straight
- }
- continue
-
- case syntax.Lazybranchcount | syntax.Back:
- // r.trackPush:
- // 0: Mark
- // 1: Count
- // 2: r.textPos
-
- r.trackPopN(3)
- mark := r.trackPeek()
- textpos := r.trackPeekN(2)
-
- if r.trackPeekN(1) < r.operand(1) && textpos != mark { // Under limit and not empty match -> loop
- r.textto(textpos) // Recall position
- r.stackPush2(textpos, r.trackPeekN(1)+1) // Make new mark, incr count
- r.trackPushNeg1(mark) // Save old mark
- r.goTo(r.operand(0)) // Loop
- continue
- } else { // Max loops or empty match -> backtrack
- r.stackPush2(r.trackPeek(), r.trackPeekN(1)) // Recall old mark, count
- break // backtrack
- }
-
- case syntax.Lazybranchcount | syntax.Back2:
- // r.trackPush:
- // 0: Previous mark
- // r.stackPush:
- // 0: Mark (== current pos, discarded)
- // 1: Count
- r.trackPop()
- r.stackPopN(2)
- r.stackPush2(r.trackPeek(), r.stackPeekN(1)-1) // Recall old mark, count
- break // Backtrack
-
- case syntax.Setjump:
- r.stackPush2(r.trackpos(), r.crawlpos())
- r.trackPush()
- r.advance(0)
- continue
-
- case syntax.Setjump | syntax.Back:
- r.stackPopN(2)
- break
-
- case syntax.Backjump:
- // r.stackPush:
- // 0: Saved trackpos
- // 1: r.crawlpos
- r.stackPopN(2)
- r.trackto(r.stackPeek())
-
- for r.crawlpos() != r.stackPeekN(1) {
- r.uncapture()
- }
-
- break
-
- case syntax.Forejump:
- // r.stackPush:
- // 0: Saved trackpos
- // 1: r.crawlpos
- r.stackPopN(2)
- r.trackto(r.stackPeek())
- r.trackPush1(r.stackPeekN(1))
- r.advance(0)
- continue
-
- case syntax.Forejump | syntax.Back:
- // r.trackPush:
- // 0: r.crawlpos
- r.trackPop()
-
- for r.crawlpos() != r.trackPeek() {
- r.uncapture()
- }
-
- break
-
- case syntax.Bol:
- if r.leftchars() > 0 && r.charAt(r.textPos()-1) != '\n' {
- break
- }
- r.advance(0)
- continue
-
- case syntax.Eol:
- if r.rightchars() > 0 && r.charAt(r.textPos()) != '\n' {
- break
- }
- r.advance(0)
- continue
-
- case syntax.Boundary:
- if !r.isBoundary(r.textPos(), 0, r.runtextend) {
- break
- }
- r.advance(0)
- continue
-
- case syntax.Nonboundary:
- if r.isBoundary(r.textPos(), 0, r.runtextend) {
- break
- }
- r.advance(0)
- continue
-
- case syntax.ECMABoundary:
- if !r.isECMABoundary(r.textPos(), 0, r.runtextend) {
- break
- }
- r.advance(0)
- continue
-
- case syntax.NonECMABoundary:
- if r.isECMABoundary(r.textPos(), 0, r.runtextend) {
- break
- }
- r.advance(0)
- continue
-
- case syntax.Beginning:
- if r.leftchars() > 0 {
- break
- }
- r.advance(0)
- continue
-
- case syntax.Start:
- if r.textPos() != r.textstart() {
- break
- }
- r.advance(0)
- continue
-
- case syntax.EndZ:
- rchars := r.rightchars()
- if rchars > 1 {
- break
- }
- // RE2 and EcmaScript define $ as "asserts position at the end of the string"
- // PCRE/.NET adds "or before the line terminator right at the end of the string (if any)"
- if (r.re.options & (RE2 | ECMAScript)) != 0 {
- // RE2/Ecmascript mode
- if rchars > 0 {
- break
- }
- } else if rchars == 1 && r.charAt(r.textPos()) != '\n' {
- // "regular" mode
- break
- }
-
- r.advance(0)
- continue
-
- case syntax.End:
- if r.rightchars() > 0 {
- break
- }
- r.advance(0)
- continue
-
- case syntax.One:
- if r.forwardchars() < 1 || r.forwardcharnext() != rune(r.operand(0)) {
- break
- }
-
- r.advance(1)
- continue
-
- case syntax.Notone:
- if r.forwardchars() < 1 || r.forwardcharnext() == rune(r.operand(0)) {
- break
- }
-
- r.advance(1)
- continue
-
- case syntax.Set:
-
- if r.forwardchars() < 1 || !r.code.Sets[r.operand(0)].CharIn(r.forwardcharnext()) {
- break
- }
-
- r.advance(1)
- continue
-
- case syntax.Multi:
- if !r.runematch(r.code.Strings[r.operand(0)]) {
- break
- }
-
- r.advance(1)
- continue
-
- case syntax.Ref:
-
- capnum := r.operand(0)
-
- if r.runmatch.isMatched(capnum) {
- if !r.refmatch(r.runmatch.matchIndex(capnum), r.runmatch.matchLength(capnum)) {
- break
- }
- } else {
- if (r.re.options & ECMAScript) == 0 {
- break
- }
- }
-
- r.advance(1)
- continue
-
- case syntax.Onerep:
-
- c := r.operand(1)
-
- if r.forwardchars() < c {
- break
- }
-
- ch := rune(r.operand(0))
-
- for c > 0 {
- if r.forwardcharnext() != ch {
- goto BreakBackward
- }
- c--
- }
-
- r.advance(2)
- continue
-
- case syntax.Notonerep:
-
- c := r.operand(1)
-
- if r.forwardchars() < c {
- break
- }
- ch := rune(r.operand(0))
-
- for c > 0 {
- if r.forwardcharnext() == ch {
- goto BreakBackward
- }
- c--
- }
-
- r.advance(2)
- continue
-
- case syntax.Setrep:
-
- c := r.operand(1)
-
- if r.forwardchars() < c {
- break
- }
-
- set := r.code.Sets[r.operand(0)]
-
- for c > 0 {
- if !set.CharIn(r.forwardcharnext()) {
- goto BreakBackward
- }
- c--
- }
-
- r.advance(2)
- continue
-
- case syntax.Oneloop:
-
- c := r.operand(1)
-
- if c > r.forwardchars() {
- c = r.forwardchars()
- }
-
- ch := rune(r.operand(0))
- i := c
-
- for ; i > 0; i-- {
- if r.forwardcharnext() != ch {
- r.backwardnext()
- break
- }
- }
-
- if c > i {
- r.trackPush2(c-i-1, r.textPos()-r.bump())
- }
-
- r.advance(2)
- continue
-
- case syntax.Notoneloop:
-
- c := r.operand(1)
-
- if c > r.forwardchars() {
- c = r.forwardchars()
- }
-
- ch := rune(r.operand(0))
- i := c
-
- for ; i > 0; i-- {
- if r.forwardcharnext() == ch {
- r.backwardnext()
- break
- }
- }
-
- if c > i {
- r.trackPush2(c-i-1, r.textPos()-r.bump())
- }
-
- r.advance(2)
- continue
-
- case syntax.Setloop:
-
- c := r.operand(1)
-
- if c > r.forwardchars() {
- c = r.forwardchars()
- }
-
- set := r.code.Sets[r.operand(0)]
- i := c
-
- for ; i > 0; i-- {
- if !set.CharIn(r.forwardcharnext()) {
- r.backwardnext()
- break
- }
- }
-
- if c > i {
- r.trackPush2(c-i-1, r.textPos()-r.bump())
- }
-
- r.advance(2)
- continue
-
- case syntax.Oneloop | syntax.Back, syntax.Notoneloop | syntax.Back:
-
- r.trackPopN(2)
- i := r.trackPeek()
- pos := r.trackPeekN(1)
-
- r.textto(pos)
-
- if i > 0 {
- r.trackPush2(i-1, pos-r.bump())
- }
-
- r.advance(2)
- continue
-
- case syntax.Setloop | syntax.Back:
-
- r.trackPopN(2)
- i := r.trackPeek()
- pos := r.trackPeekN(1)
-
- r.textto(pos)
-
- if i > 0 {
- r.trackPush2(i-1, pos-r.bump())
- }
-
- r.advance(2)
- continue
-
- case syntax.Onelazy, syntax.Notonelazy:
-
- c := r.operand(1)
-
- if c > r.forwardchars() {
- c = r.forwardchars()
- }
-
- if c > 0 {
- r.trackPush2(c-1, r.textPos())
- }
-
- r.advance(2)
- continue
-
- case syntax.Setlazy:
-
- c := r.operand(1)
-
- if c > r.forwardchars() {
- c = r.forwardchars()
- }
-
- if c > 0 {
- r.trackPush2(c-1, r.textPos())
- }
-
- r.advance(2)
- continue
-
- case syntax.Onelazy | syntax.Back:
-
- r.trackPopN(2)
- pos := r.trackPeekN(1)
- r.textto(pos)
-
- if r.forwardcharnext() != rune(r.operand(0)) {
- break
- }
-
- i := r.trackPeek()
-
- if i > 0 {
- r.trackPush2(i-1, pos+r.bump())
- }
-
- r.advance(2)
- continue
-
- case syntax.Notonelazy | syntax.Back:
-
- r.trackPopN(2)
- pos := r.trackPeekN(1)
- r.textto(pos)
-
- if r.forwardcharnext() == rune(r.operand(0)) {
- break
- }
-
- i := r.trackPeek()
-
- if i > 0 {
- r.trackPush2(i-1, pos+r.bump())
- }
-
- r.advance(2)
- continue
-
- case syntax.Setlazy | syntax.Back:
-
- r.trackPopN(2)
- pos := r.trackPeekN(1)
- r.textto(pos)
-
- if !r.code.Sets[r.operand(0)].CharIn(r.forwardcharnext()) {
- break
- }
-
- i := r.trackPeek()
-
- if i > 0 {
- r.trackPush2(i-1, pos+r.bump())
- }
-
- r.advance(2)
- continue
-
- default:
- return errors.New("unknown state in regex runner")
- }
-
- BreakBackward:
- ;
-
- // "break Backward" comes here:
- r.backtrack()
- }
-}
-
-// increase the size of stack and track storage
-func (r *runner) ensureStorage() {
- if r.runstackpos < r.runtrackcount*4 {
- doubleIntSlice(&r.runstack, &r.runstackpos)
- }
- if r.runtrackpos < r.runtrackcount*4 {
- doubleIntSlice(&r.runtrack, &r.runtrackpos)
- }
-}
-
-func doubleIntSlice(s *[]int, pos *int) {
- oldLen := len(*s)
- newS := make([]int, oldLen*2)
-
- copy(newS[oldLen:], *s)
- *pos += oldLen
- *s = newS
-}
-
-// Save a number on the longjump unrolling stack
-func (r *runner) crawl(i int) {
- if r.runcrawlpos == 0 {
- doubleIntSlice(&r.runcrawl, &r.runcrawlpos)
- }
- r.runcrawlpos--
- r.runcrawl[r.runcrawlpos] = i
-}
-
-// Remove a number from the longjump unrolling stack
-func (r *runner) popcrawl() int {
- val := r.runcrawl[r.runcrawlpos]
- r.runcrawlpos++
- return val
-}
-
-// Get the height of the stack
-func (r *runner) crawlpos() int {
- return len(r.runcrawl) - r.runcrawlpos
-}
-
-func (r *runner) advance(i int) {
- r.codepos += (i + 1)
- r.setOperator(r.code.Codes[r.codepos])
-}
-
-func (r *runner) goTo(newpos int) {
- // when branching backward or in place, ensure storage
- if newpos <= r.codepos {
- r.ensureStorage()
- }
-
- r.setOperator(r.code.Codes[newpos])
- r.codepos = newpos
-}
-
-func (r *runner) textto(newpos int) {
- r.runtextpos = newpos
-}
-
-func (r *runner) trackto(newpos int) {
- r.runtrackpos = len(r.runtrack) - newpos
-}
-
-func (r *runner) textstart() int {
- return r.runtextstart
-}
-
-func (r *runner) textPos() int {
- return r.runtextpos
-}
-
-// push onto the backtracking stack
-func (r *runner) trackpos() int {
- return len(r.runtrack) - r.runtrackpos
-}
-
-func (r *runner) trackPush() {
- r.runtrackpos--
- r.runtrack[r.runtrackpos] = r.codepos
-}
-
-func (r *runner) trackPush1(I1 int) {
- r.runtrackpos--
- r.runtrack[r.runtrackpos] = I1
- r.runtrackpos--
- r.runtrack[r.runtrackpos] = r.codepos
-}
-
-func (r *runner) trackPush2(I1, I2 int) {
- r.runtrackpos--
- r.runtrack[r.runtrackpos] = I1
- r.runtrackpos--
- r.runtrack[r.runtrackpos] = I2
- r.runtrackpos--
- r.runtrack[r.runtrackpos] = r.codepos
-}
-
-func (r *runner) trackPush3(I1, I2, I3 int) {
- r.runtrackpos--
- r.runtrack[r.runtrackpos] = I1
- r.runtrackpos--
- r.runtrack[r.runtrackpos] = I2
- r.runtrackpos--
- r.runtrack[r.runtrackpos] = I3
- r.runtrackpos--
- r.runtrack[r.runtrackpos] = r.codepos
-}
-
-func (r *runner) trackPushNeg1(I1 int) {
- r.runtrackpos--
- r.runtrack[r.runtrackpos] = I1
- r.runtrackpos--
- r.runtrack[r.runtrackpos] = -r.codepos
-}
-
-func (r *runner) trackPushNeg2(I1, I2 int) {
- r.runtrackpos--
- r.runtrack[r.runtrackpos] = I1
- r.runtrackpos--
- r.runtrack[r.runtrackpos] = I2
- r.runtrackpos--
- r.runtrack[r.runtrackpos] = -r.codepos
-}
-
-func (r *runner) backtrack() {
- newpos := r.runtrack[r.runtrackpos]
- r.runtrackpos++
-
- if r.re.Debug() {
- if newpos < 0 {
- fmt.Printf(" Backtracking (back2) to code position %v\n", -newpos)
- } else {
- fmt.Printf(" Backtracking to code position %v\n", newpos)
- }
- }
-
- if newpos < 0 {
- newpos = -newpos
- r.setOperator(r.code.Codes[newpos] | syntax.Back2)
- } else {
- r.setOperator(r.code.Codes[newpos] | syntax.Back)
- }
-
- // When branching backward, ensure storage
- if newpos < r.codepos {
- r.ensureStorage()
- }
-
- r.codepos = newpos
-}
-
-func (r *runner) setOperator(op int) {
- r.caseInsensitive = (0 != (op & syntax.Ci))
- r.rightToLeft = (0 != (op & syntax.Rtl))
- r.operator = syntax.InstOp(op & ^(syntax.Rtl | syntax.Ci))
-}
-
-func (r *runner) trackPop() {
- r.runtrackpos++
-}
-
-// pop framesize items from the backtracking stack
-func (r *runner) trackPopN(framesize int) {
- r.runtrackpos += framesize
-}
-
-// Technically we are actually peeking at items already popped. So if you want to
-// get and pop the top item from the stack, you do
-// r.trackPop();
-// r.trackPeek();
-func (r *runner) trackPeek() int {
- return r.runtrack[r.runtrackpos-1]
-}
-
-// get the ith element down on the backtracking stack
-func (r *runner) trackPeekN(i int) int {
- return r.runtrack[r.runtrackpos-i-1]
-}
-
-// Push onto the grouping stack
-func (r *runner) stackPush(I1 int) {
- r.runstackpos--
- r.runstack[r.runstackpos] = I1
-}
-
-func (r *runner) stackPush2(I1, I2 int) {
- r.runstackpos--
- r.runstack[r.runstackpos] = I1
- r.runstackpos--
- r.runstack[r.runstackpos] = I2
-}
-
-func (r *runner) stackPop() {
- r.runstackpos++
-}
-
-// pop framesize items from the grouping stack
-func (r *runner) stackPopN(framesize int) {
- r.runstackpos += framesize
-}
-
-// Technically we are actually peeking at items already popped. So if you want to
-// get and pop the top item from the stack, you do
-// r.stackPop();
-// r.stackPeek();
-func (r *runner) stackPeek() int {
- return r.runstack[r.runstackpos-1]
-}
-
-// get the ith element down on the grouping stack
-func (r *runner) stackPeekN(i int) int {
- return r.runstack[r.runstackpos-i-1]
-}
-
-func (r *runner) operand(i int) int {
- return r.code.Codes[r.codepos+i+1]
-}
-
-func (r *runner) leftchars() int {
- return r.runtextpos
-}
-
-func (r *runner) rightchars() int {
- return r.runtextend - r.runtextpos
-}
-
-func (r *runner) bump() int {
- if r.rightToLeft {
- return -1
- }
- return 1
-}
-
-func (r *runner) forwardchars() int {
- if r.rightToLeft {
- return r.runtextpos
- }
- return r.runtextend - r.runtextpos
-}
-
-func (r *runner) forwardcharnext() rune {
- var ch rune
- if r.rightToLeft {
- r.runtextpos--
- ch = r.runtext[r.runtextpos]
- } else {
- ch = r.runtext[r.runtextpos]
- r.runtextpos++
- }
-
- if r.caseInsensitive {
- return unicode.ToLower(ch)
- }
- return ch
-}
-
-func (r *runner) runematch(str []rune) bool {
- var pos int
-
- c := len(str)
- if !r.rightToLeft {
- if r.runtextend-r.runtextpos < c {
- return false
- }
-
- pos = r.runtextpos + c
- } else {
- if r.runtextpos-0 < c {
- return false
- }
-
- pos = r.runtextpos
- }
-
- if !r.caseInsensitive {
- for c != 0 {
- c--
- pos--
- if str[c] != r.runtext[pos] {
- return false
- }
- }
- } else {
- for c != 0 {
- c--
- pos--
- if str[c] != unicode.ToLower(r.runtext[pos]) {
- return false
- }
- }
- }
-
- if !r.rightToLeft {
- pos += len(str)
- }
-
- r.runtextpos = pos
-
- return true
-}
-
-func (r *runner) refmatch(index, len int) bool {
- var c, pos, cmpos int
-
- if !r.rightToLeft {
- if r.runtextend-r.runtextpos < len {
- return false
- }
-
- pos = r.runtextpos + len
- } else {
- if r.runtextpos-0 < len {
- return false
- }
-
- pos = r.runtextpos
- }
- cmpos = index + len
-
- c = len
-
- if !r.caseInsensitive {
- for c != 0 {
- c--
- cmpos--
- pos--
- if r.runtext[cmpos] != r.runtext[pos] {
- return false
- }
-
- }
- } else {
- for c != 0 {
- c--
- cmpos--
- pos--
-
- if unicode.ToLower(r.runtext[cmpos]) != unicode.ToLower(r.runtext[pos]) {
- return false
- }
- }
- }
-
- if !r.rightToLeft {
- pos += len
- }
-
- r.runtextpos = pos
-
- return true
-}
-
-func (r *runner) backwardnext() {
- if r.rightToLeft {
- r.runtextpos++
- } else {
- r.runtextpos--
- }
-}
-
-func (r *runner) charAt(j int) rune {
- return r.runtext[j]
-}
-
-func (r *runner) findFirstChar() bool {
-
- if 0 != (r.code.Anchors & (syntax.AnchorBeginning | syntax.AnchorStart | syntax.AnchorEndZ | syntax.AnchorEnd)) {
- if !r.code.RightToLeft {
- if (0 != (r.code.Anchors&syntax.AnchorBeginning) && r.runtextpos > 0) ||
- (0 != (r.code.Anchors&syntax.AnchorStart) && r.runtextpos > r.runtextstart) {
- r.runtextpos = r.runtextend
- return false
- }
- if 0 != (r.code.Anchors&syntax.AnchorEndZ) && r.runtextpos < r.runtextend-1 {
- r.runtextpos = r.runtextend - 1
- } else if 0 != (r.code.Anchors&syntax.AnchorEnd) && r.runtextpos < r.runtextend {
- r.runtextpos = r.runtextend
- }
- } else {
- if (0 != (r.code.Anchors&syntax.AnchorEnd) && r.runtextpos < r.runtextend) ||
- (0 != (r.code.Anchors&syntax.AnchorEndZ) && (r.runtextpos < r.runtextend-1 ||
- (r.runtextpos == r.runtextend-1 && r.charAt(r.runtextpos) != '\n'))) ||
- (0 != (r.code.Anchors&syntax.AnchorStart) && r.runtextpos < r.runtextstart) {
- r.runtextpos = 0
- return false
- }
- if 0 != (r.code.Anchors&syntax.AnchorBeginning) && r.runtextpos > 0 {
- r.runtextpos = 0
- }
- }
-
- if r.code.BmPrefix != nil {
- return r.code.BmPrefix.IsMatch(r.runtext, r.runtextpos, 0, r.runtextend)
- }
-
- return true // found a valid start or end anchor
- } else if r.code.BmPrefix != nil {
- r.runtextpos = r.code.BmPrefix.Scan(r.runtext, r.runtextpos, 0, r.runtextend)
-
- if r.runtextpos == -1 {
- if r.code.RightToLeft {
- r.runtextpos = 0
- } else {
- r.runtextpos = r.runtextend
- }
- return false
- }
-
- return true
- } else if r.code.FcPrefix == nil {
- return true
- }
-
- r.rightToLeft = r.code.RightToLeft
- r.caseInsensitive = r.code.FcPrefix.CaseInsensitive
-
- set := r.code.FcPrefix.PrefixSet
- if set.IsSingleton() {
- ch := set.SingletonChar()
- for i := r.forwardchars(); i > 0; i-- {
- if ch == r.forwardcharnext() {
- r.backwardnext()
- return true
- }
- }
- } else {
- for i := r.forwardchars(); i > 0; i-- {
- n := r.forwardcharnext()
- //fmt.Printf("%v in %v: %v\n", string(n), set.String(), set.CharIn(n))
- if set.CharIn(n) {
- r.backwardnext()
- return true
- }
- }
- }
-
- return false
-}
-
-func (r *runner) initMatch() {
- // Use a hashtable'ed Match object if the capture numbers are sparse
-
- if r.runmatch == nil {
- if r.re.caps != nil {
- r.runmatch = newMatchSparse(r.re, r.re.caps, r.re.capsize, r.runtext, r.runtextstart)
- } else {
- r.runmatch = newMatch(r.re, r.re.capsize, r.runtext, r.runtextstart)
- }
- } else {
- r.runmatch.reset(r.runtext, r.runtextstart)
- }
-
- // note we test runcrawl, because it is the last one to be allocated
- // If there is an alloc failure in the middle of the three allocations,
- // we may still return to reuse this instance, and we want to behave
- // as if the allocations didn't occur. (we used to test _trackcount != 0)
-
- if r.runcrawl != nil {
- r.runtrackpos = len(r.runtrack)
- r.runstackpos = len(r.runstack)
- r.runcrawlpos = len(r.runcrawl)
- return
- }
-
- r.initTrackCount()
-
- tracksize := r.runtrackcount * 8
- stacksize := r.runtrackcount * 8
-
- if tracksize < 32 {
- tracksize = 32
- }
- if stacksize < 16 {
- stacksize = 16
- }
-
- r.runtrack = make([]int, tracksize)
- r.runtrackpos = tracksize
-
- r.runstack = make([]int, stacksize)
- r.runstackpos = stacksize
-
- r.runcrawl = make([]int, 32)
- r.runcrawlpos = 32
-}
-
-func (r *runner) tidyMatch(quick bool) *Match {
- if !quick {
- match := r.runmatch
-
- r.runmatch = nil
-
- match.tidy(r.runtextpos)
- return match
- } else {
- // send back our match -- it's not leaving the package, so it's safe to not clean it up
- // this reduces allocs for frequent calls to the "IsMatch" bool-only functions
- return r.runmatch
- }
-}
-
-// capture captures a subexpression. Note that the
-// capnum used here has already been mapped to a non-sparse
-// index (by the code generator RegexWriter).
-func (r *runner) capture(capnum, start, end int) {
- if end < start {
- T := end
- end = start
- start = T
- }
-
- r.crawl(capnum)
- r.runmatch.addMatch(capnum, start, end-start)
-}
-
-// transferCapture captures a subexpression. Note that the
-// capnum used here has already been mapped to a non-sparse
-// index (by the code generator RegexWriter).
-func (r *runner) transferCapture(capnum, uncapnum, start, end int) {
- var start2, end2 int
-
- // these are the two intervals that are cancelling each other
-
- if end < start {
- T := end
- end = start
- start = T
- }
-
- start2 = r.runmatch.matchIndex(uncapnum)
- end2 = start2 + r.runmatch.matchLength(uncapnum)
-
- // The new capture gets the innermost defined interval
-
- if start >= end2 {
- end = start
- start = end2
- } else if end <= start2 {
- start = start2
- } else {
- if end > end2 {
- end = end2
- }
- if start2 > start {
- start = start2
- }
- }
-
- r.crawl(uncapnum)
- r.runmatch.balanceMatch(uncapnum)
-
- if capnum != -1 {
- r.crawl(capnum)
- r.runmatch.addMatch(capnum, start, end-start)
- }
-}
-
-// revert the last capture
-func (r *runner) uncapture() {
- capnum := r.popcrawl()
- r.runmatch.removeMatch(capnum)
-}
-
-//debug
-
-func (r *runner) dumpState() {
- back := ""
- if r.operator&syntax.Back != 0 {
- back = " Back"
- }
- if r.operator&syntax.Back2 != 0 {
- back += " Back2"
- }
- fmt.Printf("Text: %v\nTrack: %v\nStack: %v\n %s%s\n\n",
- r.textposDescription(),
- r.stackDescription(r.runtrack, r.runtrackpos),
- r.stackDescription(r.runstack, r.runstackpos),
- r.code.OpcodeDescription(r.codepos),
- back)
-}
-
-func (r *runner) stackDescription(a []int, index int) string {
- buf := &bytes.Buffer{}
-
- fmt.Fprintf(buf, "%v/%v", len(a)-index, len(a))
- if buf.Len() < 8 {
- buf.WriteString(strings.Repeat(" ", 8-buf.Len()))
- }
-
- buf.WriteRune('(')
- for i := index; i < len(a); i++ {
- if i > index {
- buf.WriteRune(' ')
- }
-
- buf.WriteString(strconv.Itoa(a[i]))
- }
-
- buf.WriteRune(')')
-
- return buf.String()
-}
-
-func (r *runner) textposDescription() string {
- buf := &bytes.Buffer{}
-
- buf.WriteString(strconv.Itoa(r.runtextpos))
-
- if buf.Len() < 8 {
- buf.WriteString(strings.Repeat(" ", 8-buf.Len()))
- }
-
- if r.runtextpos > 0 {
- buf.WriteString(syntax.CharDescription(r.runtext[r.runtextpos-1]))
- } else {
- buf.WriteRune('^')
- }
-
- buf.WriteRune('>')
-
- for i := r.runtextpos; i < r.runtextend; i++ {
- buf.WriteString(syntax.CharDescription(r.runtext[i]))
- }
- if buf.Len() >= 64 {
- buf.Truncate(61)
- buf.WriteString("...")
- } else {
- buf.WriteRune('$')
- }
-
- return buf.String()
-}
-
-// decide whether the pos
-// at the specified index is a boundary or not. It's just not worth
-// emitting inline code for this logic.
-func (r *runner) isBoundary(index, startpos, endpos int) bool {
- return (index > startpos && syntax.IsWordChar(r.runtext[index-1])) !=
- (index < endpos && syntax.IsWordChar(r.runtext[index]))
-}
-
-func (r *runner) isECMABoundary(index, startpos, endpos int) bool {
- return (index > startpos && syntax.IsECMAWordChar(r.runtext[index-1])) !=
- (index < endpos && syntax.IsECMAWordChar(r.runtext[index]))
-}
-
-// this seems like a comment to justify randomly picking 1000 :-P
-// We have determined this value in a series of experiments where x86 retail
-// builds (ono-lab-optimized) were run on different pattern/input pairs. Larger values
-// of TimeoutCheckFrequency did not tend to increase performance; smaller values
-// of TimeoutCheckFrequency tended to slow down the execution.
-const timeoutCheckFrequency int = 1000
-
-func (r *runner) startTimeoutWatch() {
- if r.ignoreTimeout {
- return
- }
-
- r.timeoutChecksToSkip = timeoutCheckFrequency
- r.timeoutAt = time.Now().Add(r.timeout)
-}
-
-func (r *runner) checkTimeout() error {
- if r.ignoreTimeout {
- return nil
- }
- r.timeoutChecksToSkip--
- if r.timeoutChecksToSkip != 0 {
- return nil
- }
-
- r.timeoutChecksToSkip = timeoutCheckFrequency
- return r.doCheckTimeout()
-}
-
-func (r *runner) doCheckTimeout() error {
- current := time.Now()
-
- if current.Before(r.timeoutAt) {
- return nil
- }
-
- if r.re.Debug() {
- //Debug.WriteLine("")
- //Debug.WriteLine("RegEx match timeout occurred!")
- //Debug.WriteLine("Specified timeout: " + TimeSpan.FromMilliseconds(_timeout).ToString())
- //Debug.WriteLine("Timeout check frequency: " + TimeoutCheckFrequency)
- //Debug.WriteLine("Search pattern: " + _runregex._pattern)
- //Debug.WriteLine("Input: " + r.runtext)
- //Debug.WriteLine("About to throw RegexMatchTimeoutException.")
- }
-
- return fmt.Errorf("match timeout after %v on input `%v`", r.timeout, string(r.runtext))
-}
-
-func (r *runner) initTrackCount() {
- r.runtrackcount = r.code.TrackCount
-}
-
-// getRunner returns a run to use for matching re.
-// It uses the re's runner cache if possible, to avoid
-// unnecessary allocation.
-func (re *Regexp) getRunner() *runner {
- re.muRun.Lock()
- if n := len(re.runner); n > 0 {
- z := re.runner[n-1]
- re.runner = re.runner[:n-1]
- re.muRun.Unlock()
- return z
- }
- re.muRun.Unlock()
- z := &runner{
- re: re,
- code: re.code,
- }
- return z
-}
-
-// putRunner returns a runner to the re's cache.
-// There is no attempt to limit the size of the cache, so it will
-// grow to the maximum number of simultaneous matches
-// run using re. (The cache empties when re gets garbage collected.)
-func (re *Regexp) putRunner(r *runner) {
- re.muRun.Lock()
- re.runner = append(re.runner, r)
- re.muRun.Unlock()
-}
diff --git a/vendor/github.com/dlclark/regexp2/syntax/charclass.go b/vendor/github.com/dlclark/regexp2/syntax/charclass.go
deleted file mode 100644
index 53974d1..0000000
--- a/vendor/github.com/dlclark/regexp2/syntax/charclass.go
+++ /dev/null
@@ -1,854 +0,0 @@
-package syntax
-
-import (
- "bytes"
- "encoding/binary"
- "fmt"
- "sort"
- "unicode"
- "unicode/utf8"
-)
-
-// CharSet combines start-end rune ranges and unicode categories representing a set of characters
-type CharSet struct {
- ranges []singleRange
- categories []category
- sub *CharSet //optional subtractor
- negate bool
- anything bool
-}
-
-type category struct {
- negate bool
- cat string
-}
-
-type singleRange struct {
- first rune
- last rune
-}
-
-const (
- spaceCategoryText = " "
- wordCategoryText = "W"
-)
-
-var (
- ecmaSpace = []rune{0x0009, 0x000e, 0x0020, 0x0021, 0x00a0, 0x00a1, 0x1680, 0x1681, 0x2000, 0x200b, 0x2028, 0x202a, 0x202f, 0x2030, 0x205f, 0x2060, 0x3000, 0x3001, 0xfeff, 0xff00}
- ecmaWord = []rune{0x0030, 0x003a, 0x0041, 0x005b, 0x005f, 0x0060, 0x0061, 0x007b}
- ecmaDigit = []rune{0x0030, 0x003a}
-)
-
-var (
- AnyClass = getCharSetFromOldString([]rune{0}, false)
- ECMAAnyClass = getCharSetFromOldString([]rune{0, 0x000a, 0x000b, 0x000d, 0x000e}, false)
- NoneClass = getCharSetFromOldString(nil, false)
- ECMAWordClass = getCharSetFromOldString(ecmaWord, false)
- NotECMAWordClass = getCharSetFromOldString(ecmaWord, true)
- ECMASpaceClass = getCharSetFromOldString(ecmaSpace, false)
- NotECMASpaceClass = getCharSetFromOldString(ecmaSpace, true)
- ECMADigitClass = getCharSetFromOldString(ecmaDigit, false)
- NotECMADigitClass = getCharSetFromOldString(ecmaDigit, true)
-
- WordClass = getCharSetFromCategoryString(false, false, wordCategoryText)
- NotWordClass = getCharSetFromCategoryString(true, false, wordCategoryText)
- SpaceClass = getCharSetFromCategoryString(false, false, spaceCategoryText)
- NotSpaceClass = getCharSetFromCategoryString(true, false, spaceCategoryText)
- DigitClass = getCharSetFromCategoryString(false, false, "Nd")
- NotDigitClass = getCharSetFromCategoryString(false, true, "Nd")
-)
-
-var unicodeCategories = func() map[string]*unicode.RangeTable {
- retVal := make(map[string]*unicode.RangeTable)
- for k, v := range unicode.Scripts {
- retVal[k] = v
- }
- for k, v := range unicode.Categories {
- retVal[k] = v
- }
- for k, v := range unicode.Properties {
- retVal[k] = v
- }
- return retVal
-}()
-
-func getCharSetFromCategoryString(negateSet bool, negateCat bool, cats ...string) func() *CharSet {
- if negateCat && negateSet {
- panic("BUG! You should only negate the set OR the category in a constant setup, but not both")
- }
-
- c := CharSet{negate: negateSet}
-
- c.categories = make([]category, len(cats))
- for i, cat := range cats {
- c.categories[i] = category{cat: cat, negate: negateCat}
- }
- return func() *CharSet {
- //make a copy each time
- local := c
- //return that address
- return &local
- }
-}
-
-func getCharSetFromOldString(setText []rune, negate bool) func() *CharSet {
- c := CharSet{}
- if len(setText) > 0 {
- fillFirst := false
- l := len(setText)
- if negate {
- if setText[0] == 0 {
- setText = setText[1:]
- } else {
- l++
- fillFirst = true
- }
- }
-
- if l%2 == 0 {
- c.ranges = make([]singleRange, l/2)
- } else {
- c.ranges = make([]singleRange, l/2+1)
- }
-
- first := true
- if fillFirst {
- c.ranges[0] = singleRange{first: 0}
- first = false
- }
-
- i := 0
- for _, r := range setText {
- if first {
- // lower bound in a new range
- c.ranges[i] = singleRange{first: r}
- first = false
- } else {
- c.ranges[i].last = r - 1
- i++
- first = true
- }
- }
- if !first {
- c.ranges[i].last = utf8.MaxRune
- }
- }
-
- return func() *CharSet {
- local := c
- return &local
- }
-}
-
-// Copy makes a deep copy to prevent accidental mutation of a set
-func (c CharSet) Copy() CharSet {
- ret := CharSet{
- anything: c.anything,
- negate: c.negate,
- }
-
- ret.ranges = append(ret.ranges, c.ranges...)
- ret.categories = append(ret.categories, c.categories...)
-
- if c.sub != nil {
- sub := c.sub.Copy()
- ret.sub = &sub
- }
-
- return ret
-}
-
-// gets a human-readable description for a set string
-func (c CharSet) String() string {
- buf := &bytes.Buffer{}
- buf.WriteRune('[')
-
- if c.IsNegated() {
- buf.WriteRune('^')
- }
-
- for _, r := range c.ranges {
-
- buf.WriteString(CharDescription(r.first))
- if r.first != r.last {
- if r.last-r.first != 1 {
- //groups that are 1 char apart skip the dash
- buf.WriteRune('-')
- }
- buf.WriteString(CharDescription(r.last))
- }
- }
-
- for _, c := range c.categories {
- buf.WriteString(c.String())
- }
-
- if c.sub != nil {
- buf.WriteRune('-')
- buf.WriteString(c.sub.String())
- }
-
- buf.WriteRune(']')
-
- return buf.String()
-}
-
-// mapHashFill converts a charset into a buffer for use in maps
-func (c CharSet) mapHashFill(buf *bytes.Buffer) {
- if c.negate {
- buf.WriteByte(0)
- } else {
- buf.WriteByte(1)
- }
-
- binary.Write(buf, binary.LittleEndian, len(c.ranges))
- binary.Write(buf, binary.LittleEndian, len(c.categories))
- for _, r := range c.ranges {
- buf.WriteRune(r.first)
- buf.WriteRune(r.last)
- }
- for _, ct := range c.categories {
- buf.WriteString(ct.cat)
- if ct.negate {
- buf.WriteByte(1)
- } else {
- buf.WriteByte(0)
- }
- }
-
- if c.sub != nil {
- c.sub.mapHashFill(buf)
- }
-}
-
-// CharIn returns true if the rune is in our character set (either ranges or categories).
-// It handles negations and subtracted sub-charsets.
-func (c CharSet) CharIn(ch rune) bool {
- val := false
- // in s && !s.subtracted
-
- //check ranges
- for _, r := range c.ranges {
- if ch < r.first {
- continue
- }
- if ch <= r.last {
- val = true
- break
- }
- }
-
- //check categories if we haven't already found a range
- if !val && len(c.categories) > 0 {
- for _, ct := range c.categories {
- // special categories...then unicode
- if ct.cat == spaceCategoryText {
- if unicode.IsSpace(ch) {
- // we found a space so we're done
- // negate means this is a "bad" thing
- val = !ct.negate
- break
- } else if ct.negate {
- val = true
- break
- }
- } else if ct.cat == wordCategoryText {
- if IsWordChar(ch) {
- val = !ct.negate
- break
- } else if ct.negate {
- val = true
- break
- }
- } else if unicode.Is(unicodeCategories[ct.cat], ch) {
- // if we're in this unicode category then we're done
- // if negate=true on this category then we "failed" our test
- // otherwise we're good that we found it
- val = !ct.negate
- break
- } else if ct.negate {
- val = true
- break
- }
- }
- }
-
- // negate the whole char set
- if c.negate {
- val = !val
- }
-
- // get subtracted recurse
- if val && c.sub != nil {
- val = !c.sub.CharIn(ch)
- }
-
- //log.Printf("Char '%v' in %v == %v", string(ch), c.String(), val)
- return val
-}
-
-func (c category) String() string {
- switch c.cat {
- case spaceCategoryText:
- if c.negate {
- return "\\S"
- }
- return "\\s"
- case wordCategoryText:
- if c.negate {
- return "\\W"
- }
- return "\\w"
- }
- if _, ok := unicodeCategories[c.cat]; ok {
-
- if c.negate {
- return "\\P{" + c.cat + "}"
- }
- return "\\p{" + c.cat + "}"
- }
- return "Unknown category: " + c.cat
-}
-
-// CharDescription Produces a human-readable description for a single character.
-func CharDescription(ch rune) string {
- /*if ch == '\\' {
- return "\\\\"
- }
-
- if ch > ' ' && ch <= '~' {
- return string(ch)
- } else if ch == '\n' {
- return "\\n"
- } else if ch == ' ' {
- return "\\ "
- }*/
-
- b := &bytes.Buffer{}
- escape(b, ch, false) //fmt.Sprintf("%U", ch)
- return b.String()
-}
-
-// According to UTS#18 Unicode Regular Expressions (http://www.unicode.org/reports/tr18/)
-// RL 1.4 Simple Word Boundaries The class of includes all Alphabetic
-// values from the Unicode character database, from UnicodeData.txt [UData], plus the U+200C
-// ZERO WIDTH NON-JOINER and U+200D ZERO WIDTH JOINER.
-func IsWordChar(r rune) bool {
- //"L", "Mn", "Nd", "Pc"
- return unicode.In(r,
- unicode.Categories["L"], unicode.Categories["Mn"],
- unicode.Categories["Nd"], unicode.Categories["Pc"]) || r == '\u200D' || r == '\u200C'
- //return 'A' <= r && r <= 'Z' || 'a' <= r && r <= 'z' || '0' <= r && r <= '9' || r == '_'
-}
-
-func IsECMAWordChar(r rune) bool {
- return unicode.In(r,
- unicode.Categories["L"], unicode.Categories["Mn"],
- unicode.Categories["Nd"], unicode.Categories["Pc"])
-
- //return 'A' <= r && r <= 'Z' || 'a' <= r && r <= 'z' || '0' <= r && r <= '9' || r == '_'
-}
-
-// SingletonChar will return the char from the first range without validation.
-// It assumes you have checked for IsSingleton or IsSingletonInverse and will panic given bad input
-func (c CharSet) SingletonChar() rune {
- return c.ranges[0].first
-}
-
-func (c CharSet) IsSingleton() bool {
- return !c.negate && //negated is multiple chars
- len(c.categories) == 0 && len(c.ranges) == 1 && // multiple ranges and unicode classes represent multiple chars
- c.sub == nil && // subtraction means we've got multiple chars
- c.ranges[0].first == c.ranges[0].last // first and last equal means we're just 1 char
-}
-
-func (c CharSet) IsSingletonInverse() bool {
- return c.negate && //same as above, but requires negated
- len(c.categories) == 0 && len(c.ranges) == 1 && // multiple ranges and unicode classes represent multiple chars
- c.sub == nil && // subtraction means we've got multiple chars
- c.ranges[0].first == c.ranges[0].last // first and last equal means we're just 1 char
-}
-
-func (c CharSet) IsMergeable() bool {
- return !c.IsNegated() && !c.HasSubtraction()
-}
-
-func (c CharSet) IsNegated() bool {
- return c.negate
-}
-
-func (c CharSet) HasSubtraction() bool {
- return c.sub != nil
-}
-
-func (c CharSet) IsEmpty() bool {
- return len(c.ranges) == 0 && len(c.categories) == 0 && c.sub == nil
-}
-
-func (c *CharSet) addDigit(ecma, negate bool, pattern string) {
- if ecma {
- if negate {
- c.addRanges(NotECMADigitClass().ranges)
- } else {
- c.addRanges(ECMADigitClass().ranges)
- }
- } else {
- c.addCategories(category{cat: "Nd", negate: negate})
- }
-}
-
-func (c *CharSet) addChar(ch rune) {
- c.addRange(ch, ch)
-}
-
-func (c *CharSet) addSpace(ecma, negate bool) {
- if ecma {
- if negate {
- c.addRanges(NotECMASpaceClass().ranges)
- } else {
- c.addRanges(ECMASpaceClass().ranges)
- }
- } else {
- c.addCategories(category{cat: spaceCategoryText, negate: negate})
- }
-}
-
-func (c *CharSet) addWord(ecma, negate bool) {
- if ecma {
- if negate {
- c.addRanges(NotECMAWordClass().ranges)
- } else {
- c.addRanges(ECMAWordClass().ranges)
- }
- } else {
- c.addCategories(category{cat: wordCategoryText, negate: negate})
- }
-}
-
-// Add set ranges and categories into ours -- no deduping or anything
-func (c *CharSet) addSet(set CharSet) {
- if c.anything {
- return
- }
- if set.anything {
- c.makeAnything()
- return
- }
- // just append here to prevent double-canon
- c.ranges = append(c.ranges, set.ranges...)
- c.addCategories(set.categories...)
- c.canonicalize()
-}
-
-func (c *CharSet) makeAnything() {
- c.anything = true
- c.categories = []category{}
- c.ranges = AnyClass().ranges
-}
-
-func (c *CharSet) addCategories(cats ...category) {
- // don't add dupes and remove positive+negative
- if c.anything {
- // if we've had a previous positive+negative group then
- // just return, we're as broad as we can get
- return
- }
-
- for _, ct := range cats {
- found := false
- for _, ct2 := range c.categories {
- if ct.cat == ct2.cat {
- if ct.negate != ct2.negate {
- // oposite negations...this mean we just
- // take us as anything and move on
- c.makeAnything()
- return
- }
- found = true
- break
- }
- }
-
- if !found {
- c.categories = append(c.categories, ct)
- }
- }
-}
-
-// Merges new ranges to our own
-func (c *CharSet) addRanges(ranges []singleRange) {
- if c.anything {
- return
- }
- c.ranges = append(c.ranges, ranges...)
- c.canonicalize()
-}
-
-// Merges everything but the new ranges into our own
-func (c *CharSet) addNegativeRanges(ranges []singleRange) {
- if c.anything {
- return
- }
-
- var hi rune
-
- // convert incoming ranges into opposites, assume they are in order
- for _, r := range ranges {
- if hi < r.first {
- c.ranges = append(c.ranges, singleRange{hi, r.first - 1})
- }
- hi = r.last + 1
- }
-
- if hi < utf8.MaxRune {
- c.ranges = append(c.ranges, singleRange{hi, utf8.MaxRune})
- }
-
- c.canonicalize()
-}
-
-func isValidUnicodeCat(catName string) bool {
- _, ok := unicodeCategories[catName]
- return ok
-}
-
-func (c *CharSet) addCategory(categoryName string, negate, caseInsensitive bool, pattern string) {
- if !isValidUnicodeCat(categoryName) {
- // unknown unicode category, script, or property "blah"
- panic(fmt.Errorf("Unknown unicode category, script, or property '%v'", categoryName))
-
- }
-
- if caseInsensitive && (categoryName == "Ll" || categoryName == "Lu" || categoryName == "Lt") {
- // when RegexOptions.IgnoreCase is specified then {Ll} {Lu} and {Lt} cases should all match
- c.addCategories(
- category{cat: "Ll", negate: negate},
- category{cat: "Lu", negate: negate},
- category{cat: "Lt", negate: negate})
- }
- c.addCategories(category{cat: categoryName, negate: negate})
-}
-
-func (c *CharSet) addSubtraction(sub *CharSet) {
- c.sub = sub
-}
-
-func (c *CharSet) addRange(chMin, chMax rune) {
- c.ranges = append(c.ranges, singleRange{first: chMin, last: chMax})
- c.canonicalize()
-}
-
-func (c *CharSet) addNamedASCII(name string, negate bool) bool {
- var rs []singleRange
-
- switch name {
- case "alnum":
- rs = []singleRange{singleRange{'0', '9'}, singleRange{'A', 'Z'}, singleRange{'a', 'z'}}
- case "alpha":
- rs = []singleRange{singleRange{'A', 'Z'}, singleRange{'a', 'z'}}
- case "ascii":
- rs = []singleRange{singleRange{0, 0x7f}}
- case "blank":
- rs = []singleRange{singleRange{'\t', '\t'}, singleRange{' ', ' '}}
- case "cntrl":
- rs = []singleRange{singleRange{0, 0x1f}, singleRange{0x7f, 0x7f}}
- case "digit":
- c.addDigit(false, negate, "")
- case "graph":
- rs = []singleRange{singleRange{'!', '~'}}
- case "lower":
- rs = []singleRange{singleRange{'a', 'z'}}
- case "print":
- rs = []singleRange{singleRange{' ', '~'}}
- case "punct": //[!-/:-@[-`{-~]
- rs = []singleRange{singleRange{'!', '/'}, singleRange{':', '@'}, singleRange{'[', '`'}, singleRange{'{', '~'}}
- case "space":
- c.addSpace(true, negate)
- case "upper":
- rs = []singleRange{singleRange{'A', 'Z'}}
- case "word":
- c.addWord(true, negate)
- case "xdigit":
- rs = []singleRange{singleRange{'0', '9'}, singleRange{'A', 'F'}, singleRange{'a', 'f'}}
- default:
- return false
- }
-
- if len(rs) > 0 {
- if negate {
- c.addNegativeRanges(rs)
- } else {
- c.addRanges(rs)
- }
- }
-
- return true
-}
-
-type singleRangeSorter []singleRange
-
-func (p singleRangeSorter) Len() int { return len(p) }
-func (p singleRangeSorter) Less(i, j int) bool { return p[i].first < p[j].first }
-func (p singleRangeSorter) Swap(i, j int) { p[i], p[j] = p[j], p[i] }
-
-// Logic to reduce a character class to a unique, sorted form.
-func (c *CharSet) canonicalize() {
- var i, j int
- var last rune
-
- //
- // Find and eliminate overlapping or abutting ranges
- //
-
- if len(c.ranges) > 1 {
- sort.Sort(singleRangeSorter(c.ranges))
-
- done := false
-
- for i, j = 1, 0; ; i++ {
- for last = c.ranges[j].last; ; i++ {
- if i == len(c.ranges) || last == utf8.MaxRune {
- done = true
- break
- }
-
- CurrentRange := c.ranges[i]
- if CurrentRange.first > last+1 {
- break
- }
-
- if last < CurrentRange.last {
- last = CurrentRange.last
- }
- }
-
- c.ranges[j] = singleRange{first: c.ranges[j].first, last: last}
-
- j++
-
- if done {
- break
- }
-
- if j < i {
- c.ranges[j] = c.ranges[i]
- }
- }
-
- c.ranges = append(c.ranges[:j], c.ranges[len(c.ranges):]...)
- }
-}
-
-// Adds to the class any lowercase versions of characters already
-// in the class. Used for case-insensitivity.
-func (c *CharSet) addLowercase() {
- if c.anything {
- return
- }
- toAdd := []singleRange{}
- for i := 0; i < len(c.ranges); i++ {
- r := c.ranges[i]
- if r.first == r.last {
- lower := unicode.ToLower(r.first)
- c.ranges[i] = singleRange{first: lower, last: lower}
- } else {
- toAdd = append(toAdd, r)
- }
- }
-
- for _, r := range toAdd {
- c.addLowercaseRange(r.first, r.last)
- }
- c.canonicalize()
-}
-
-/**************************************************************************
- Let U be the set of Unicode character values and let L be the lowercase
- function, mapping from U to U. To perform case insensitive matching of
- character sets, we need to be able to map an interval I in U, say
-
- I = [chMin, chMax] = { ch : chMin <= ch <= chMax }
-
- to a set A such that A contains L(I) and A is contained in the union of
- I and L(I).
-
- The table below partitions U into intervals on which L is non-decreasing.
- Thus, for any interval J = [a, b] contained in one of these intervals,
- L(J) is contained in [L(a), L(b)].
-
- It is also true that for any such J, [L(a), L(b)] is contained in the
- union of J and L(J). This does not follow from L being non-decreasing on
- these intervals. It follows from the nature of the L on each interval.
- On each interval, L has one of the following forms:
-
- (1) L(ch) = constant (LowercaseSet)
- (2) L(ch) = ch + offset (LowercaseAdd)
- (3) L(ch) = ch | 1 (LowercaseBor)
- (4) L(ch) = ch + (ch & 1) (LowercaseBad)
-
- It is easy to verify that for any of these forms [L(a), L(b)] is
- contained in the union of [a, b] and L([a, b]).
-***************************************************************************/
-
-const (
- LowercaseSet = 0 // Set to arg.
- LowercaseAdd = 1 // Add arg.
- LowercaseBor = 2 // Bitwise or with 1.
- LowercaseBad = 3 // Bitwise and with 1 and add original.
-)
-
-type lcMap struct {
- chMin, chMax rune
- op, data int32
-}
-
-var lcTable = []lcMap{
- lcMap{'\u0041', '\u005A', LowercaseAdd, 32},
- lcMap{'\u00C0', '\u00DE', LowercaseAdd, 32},
- lcMap{'\u0100', '\u012E', LowercaseBor, 0},
- lcMap{'\u0130', '\u0130', LowercaseSet, 0x0069},
- lcMap{'\u0132', '\u0136', LowercaseBor, 0},
- lcMap{'\u0139', '\u0147', LowercaseBad, 0},
- lcMap{'\u014A', '\u0176', LowercaseBor, 0},
- lcMap{'\u0178', '\u0178', LowercaseSet, 0x00FF},
- lcMap{'\u0179', '\u017D', LowercaseBad, 0},
- lcMap{'\u0181', '\u0181', LowercaseSet, 0x0253},
- lcMap{'\u0182', '\u0184', LowercaseBor, 0},
- lcMap{'\u0186', '\u0186', LowercaseSet, 0x0254},
- lcMap{'\u0187', '\u0187', LowercaseSet, 0x0188},
- lcMap{'\u0189', '\u018A', LowercaseAdd, 205},
- lcMap{'\u018B', '\u018B', LowercaseSet, 0x018C},
- lcMap{'\u018E', '\u018E', LowercaseSet, 0x01DD},
- lcMap{'\u018F', '\u018F', LowercaseSet, 0x0259},
- lcMap{'\u0190', '\u0190', LowercaseSet, 0x025B},
- lcMap{'\u0191', '\u0191', LowercaseSet, 0x0192},
- lcMap{'\u0193', '\u0193', LowercaseSet, 0x0260},
- lcMap{'\u0194', '\u0194', LowercaseSet, 0x0263},
- lcMap{'\u0196', '\u0196', LowercaseSet, 0x0269},
- lcMap{'\u0197', '\u0197', LowercaseSet, 0x0268},
- lcMap{'\u0198', '\u0198', LowercaseSet, 0x0199},
- lcMap{'\u019C', '\u019C', LowercaseSet, 0x026F},
- lcMap{'\u019D', '\u019D', LowercaseSet, 0x0272},
- lcMap{'\u019F', '\u019F', LowercaseSet, 0x0275},
- lcMap{'\u01A0', '\u01A4', LowercaseBor, 0},
- lcMap{'\u01A7', '\u01A7', LowercaseSet, 0x01A8},
- lcMap{'\u01A9', '\u01A9', LowercaseSet, 0x0283},
- lcMap{'\u01AC', '\u01AC', LowercaseSet, 0x01AD},
- lcMap{'\u01AE', '\u01AE', LowercaseSet, 0x0288},
- lcMap{'\u01AF', '\u01AF', LowercaseSet, 0x01B0},
- lcMap{'\u01B1', '\u01B2', LowercaseAdd, 217},
- lcMap{'\u01B3', '\u01B5', LowercaseBad, 0},
- lcMap{'\u01B7', '\u01B7', LowercaseSet, 0x0292},
- lcMap{'\u01B8', '\u01B8', LowercaseSet, 0x01B9},
- lcMap{'\u01BC', '\u01BC', LowercaseSet, 0x01BD},
- lcMap{'\u01C4', '\u01C5', LowercaseSet, 0x01C6},
- lcMap{'\u01C7', '\u01C8', LowercaseSet, 0x01C9},
- lcMap{'\u01CA', '\u01CB', LowercaseSet, 0x01CC},
- lcMap{'\u01CD', '\u01DB', LowercaseBad, 0},
- lcMap{'\u01DE', '\u01EE', LowercaseBor, 0},
- lcMap{'\u01F1', '\u01F2', LowercaseSet, 0x01F3},
- lcMap{'\u01F4', '\u01F4', LowercaseSet, 0x01F5},
- lcMap{'\u01FA', '\u0216', LowercaseBor, 0},
- lcMap{'\u0386', '\u0386', LowercaseSet, 0x03AC},
- lcMap{'\u0388', '\u038A', LowercaseAdd, 37},
- lcMap{'\u038C', '\u038C', LowercaseSet, 0x03CC},
- lcMap{'\u038E', '\u038F', LowercaseAdd, 63},
- lcMap{'\u0391', '\u03AB', LowercaseAdd, 32},
- lcMap{'\u03E2', '\u03EE', LowercaseBor, 0},
- lcMap{'\u0401', '\u040F', LowercaseAdd, 80},
- lcMap{'\u0410', '\u042F', LowercaseAdd, 32},
- lcMap{'\u0460', '\u0480', LowercaseBor, 0},
- lcMap{'\u0490', '\u04BE', LowercaseBor, 0},
- lcMap{'\u04C1', '\u04C3', LowercaseBad, 0},
- lcMap{'\u04C7', '\u04C7', LowercaseSet, 0x04C8},
- lcMap{'\u04CB', '\u04CB', LowercaseSet, 0x04CC},
- lcMap{'\u04D0', '\u04EA', LowercaseBor, 0},
- lcMap{'\u04EE', '\u04F4', LowercaseBor, 0},
- lcMap{'\u04F8', '\u04F8', LowercaseSet, 0x04F9},
- lcMap{'\u0531', '\u0556', LowercaseAdd, 48},
- lcMap{'\u10A0', '\u10C5', LowercaseAdd, 48},
- lcMap{'\u1E00', '\u1EF8', LowercaseBor, 0},
- lcMap{'\u1F08', '\u1F0F', LowercaseAdd, -8},
- lcMap{'\u1F18', '\u1F1F', LowercaseAdd, -8},
- lcMap{'\u1F28', '\u1F2F', LowercaseAdd, -8},
- lcMap{'\u1F38', '\u1F3F', LowercaseAdd, -8},
- lcMap{'\u1F48', '\u1F4D', LowercaseAdd, -8},
- lcMap{'\u1F59', '\u1F59', LowercaseSet, 0x1F51},
- lcMap{'\u1F5B', '\u1F5B', LowercaseSet, 0x1F53},
- lcMap{'\u1F5D', '\u1F5D', LowercaseSet, 0x1F55},
- lcMap{'\u1F5F', '\u1F5F', LowercaseSet, 0x1F57},
- lcMap{'\u1F68', '\u1F6F', LowercaseAdd, -8},
- lcMap{'\u1F88', '\u1F8F', LowercaseAdd, -8},
- lcMap{'\u1F98', '\u1F9F', LowercaseAdd, -8},
- lcMap{'\u1FA8', '\u1FAF', LowercaseAdd, -8},
- lcMap{'\u1FB8', '\u1FB9', LowercaseAdd, -8},
- lcMap{'\u1FBA', '\u1FBB', LowercaseAdd, -74},
- lcMap{'\u1FBC', '\u1FBC', LowercaseSet, 0x1FB3},
- lcMap{'\u1FC8', '\u1FCB', LowercaseAdd, -86},
- lcMap{'\u1FCC', '\u1FCC', LowercaseSet, 0x1FC3},
- lcMap{'\u1FD8', '\u1FD9', LowercaseAdd, -8},
- lcMap{'\u1FDA', '\u1FDB', LowercaseAdd, -100},
- lcMap{'\u1FE8', '\u1FE9', LowercaseAdd, -8},
- lcMap{'\u1FEA', '\u1FEB', LowercaseAdd, -112},
- lcMap{'\u1FEC', '\u1FEC', LowercaseSet, 0x1FE5},
- lcMap{'\u1FF8', '\u1FF9', LowercaseAdd, -128},
- lcMap{'\u1FFA', '\u1FFB', LowercaseAdd, -126},
- lcMap{'\u1FFC', '\u1FFC', LowercaseSet, 0x1FF3},
- lcMap{'\u2160', '\u216F', LowercaseAdd, 16},
- lcMap{'\u24B6', '\u24D0', LowercaseAdd, 26},
- lcMap{'\uFF21', '\uFF3A', LowercaseAdd, 32},
-}
-
-func (c *CharSet) addLowercaseRange(chMin, chMax rune) {
- var i, iMax, iMid int
- var chMinT, chMaxT rune
- var lc lcMap
-
- for i, iMax = 0, len(lcTable); i < iMax; {
- iMid = (i + iMax) / 2
- if lcTable[iMid].chMax < chMin {
- i = iMid + 1
- } else {
- iMax = iMid
- }
- }
-
- for ; i < len(lcTable); i++ {
- lc = lcTable[i]
- if lc.chMin > chMax {
- return
- }
- chMinT = lc.chMin
- if chMinT < chMin {
- chMinT = chMin
- }
-
- chMaxT = lc.chMax
- if chMaxT > chMax {
- chMaxT = chMax
- }
-
- switch lc.op {
- case LowercaseSet:
- chMinT = rune(lc.data)
- chMaxT = rune(lc.data)
- break
- case LowercaseAdd:
- chMinT += lc.data
- chMaxT += lc.data
- break
- case LowercaseBor:
- chMinT |= 1
- chMaxT |= 1
- break
- case LowercaseBad:
- chMinT += (chMinT & 1)
- chMaxT += (chMaxT & 1)
- break
- }
-
- if chMinT < chMin || chMaxT > chMax {
- c.addRange(chMinT, chMaxT)
- }
- }
-}
diff --git a/vendor/github.com/dlclark/regexp2/syntax/code.go b/vendor/github.com/dlclark/regexp2/syntax/code.go
deleted file mode 100644
index 686e822..0000000
--- a/vendor/github.com/dlclark/regexp2/syntax/code.go
+++ /dev/null
@@ -1,274 +0,0 @@
-package syntax
-
-import (
- "bytes"
- "fmt"
- "math"
-)
-
-// similar to prog.go in the go regex package...also with comment 'may not belong in this package'
-
-// File provides operator constants for use by the Builder and the Machine.
-
-// Implementation notes:
-//
-// Regexps are built into RegexCodes, which contain an operation array,
-// a string table, and some constants.
-//
-// Each operation is one of the codes below, followed by the integer
-// operands specified for each op.
-//
-// Strings and sets are indices into a string table.
-
-type InstOp int
-
-const (
- // lef/back operands description
-
- Onerep InstOp = 0 // lef,back char,min,max a {n}
- Notonerep = 1 // lef,back char,min,max .{n}
- Setrep = 2 // lef,back set,min,max [\d]{n}
-
- Oneloop = 3 // lef,back char,min,max a {,n}
- Notoneloop = 4 // lef,back char,min,max .{,n}
- Setloop = 5 // lef,back set,min,max [\d]{,n}
-
- Onelazy = 6 // lef,back char,min,max a {,n}?
- Notonelazy = 7 // lef,back char,min,max .{,n}?
- Setlazy = 8 // lef,back set,min,max [\d]{,n}?
-
- One = 9 // lef char a
- Notone = 10 // lef char [^a]
- Set = 11 // lef set [a-z\s] \w \s \d
-
- Multi = 12 // lef string abcd
- Ref = 13 // lef group \#
-
- Bol = 14 // ^
- Eol = 15 // $
- Boundary = 16 // \b
- Nonboundary = 17 // \B
- Beginning = 18 // \A
- Start = 19 // \G
- EndZ = 20 // \Z
- End = 21 // \Z
-
- Nothing = 22 // Reject!
-
- // Primitive control structures
-
- Lazybranch = 23 // back jump straight first
- Branchmark = 24 // back jump branch first for loop
- Lazybranchmark = 25 // back jump straight first for loop
- Nullcount = 26 // back val set counter, null mark
- Setcount = 27 // back val set counter, make mark
- Branchcount = 28 // back jump,limit branch++ if zero<=c impl group slots
- Capsize int // number of impl group slots
- FcPrefix *Prefix // the set of candidate first characters (may be null)
- BmPrefix *BmPrefix // the fixed prefix string as a Boyer-Moore machine (may be null)
- Anchors AnchorLoc // the set of zero-length start anchors (RegexFCD.Bol, etc)
- RightToLeft bool // true if right to left
-}
-
-func opcodeBacktracks(op InstOp) bool {
- op &= Mask
-
- switch op {
- case Oneloop, Notoneloop, Setloop, Onelazy, Notonelazy, Setlazy, Lazybranch, Branchmark, Lazybranchmark,
- Nullcount, Setcount, Branchcount, Lazybranchcount, Setmark, Capturemark, Getmark, Setjump, Backjump,
- Forejump, Goto:
- return true
-
- default:
- return false
- }
-}
-
-func opcodeSize(op InstOp) int {
- op &= Mask
-
- switch op {
- case Nothing, Bol, Eol, Boundary, Nonboundary, ECMABoundary, NonECMABoundary, Beginning, Start, EndZ,
- End, Nullmark, Setmark, Getmark, Setjump, Backjump, Forejump, Stop:
- return 1
-
- case One, Notone, Multi, Ref, Testref, Goto, Nullcount, Setcount, Lazybranch, Branchmark, Lazybranchmark,
- Prune, Set:
- return 2
-
- case Capturemark, Branchcount, Lazybranchcount, Onerep, Notonerep, Oneloop, Notoneloop, Onelazy, Notonelazy,
- Setlazy, Setrep, Setloop:
- return 3
-
- default:
- panic(fmt.Errorf("Unexpected op code: %v", op))
- }
-}
-
-var codeStr = []string{
- "Onerep", "Notonerep", "Setrep",
- "Oneloop", "Notoneloop", "Setloop",
- "Onelazy", "Notonelazy", "Setlazy",
- "One", "Notone", "Set",
- "Multi", "Ref",
- "Bol", "Eol", "Boundary", "Nonboundary", "Beginning", "Start", "EndZ", "End",
- "Nothing",
- "Lazybranch", "Branchmark", "Lazybranchmark",
- "Nullcount", "Setcount", "Branchcount", "Lazybranchcount",
- "Nullmark", "Setmark", "Capturemark", "Getmark",
- "Setjump", "Backjump", "Forejump", "Testref", "Goto",
- "Prune", "Stop",
- "ECMABoundary", "NonECMABoundary",
-}
-
-func operatorDescription(op InstOp) string {
- desc := codeStr[op&Mask]
- if (op & Ci) != 0 {
- desc += "-Ci"
- }
- if (op & Rtl) != 0 {
- desc += "-Rtl"
- }
- if (op & Back) != 0 {
- desc += "-Back"
- }
- if (op & Back2) != 0 {
- desc += "-Back2"
- }
-
- return desc
-}
-
-// OpcodeDescription is a humman readable string of the specific offset
-func (c *Code) OpcodeDescription(offset int) string {
- buf := &bytes.Buffer{}
-
- op := InstOp(c.Codes[offset])
- fmt.Fprintf(buf, "%06d ", offset)
-
- if opcodeBacktracks(op & Mask) {
- buf.WriteString("*")
- } else {
- buf.WriteString(" ")
- }
- buf.WriteString(operatorDescription(op))
- buf.WriteString("(")
- op &= Mask
-
- switch op {
- case One, Notone, Onerep, Notonerep, Oneloop, Notoneloop, Onelazy, Notonelazy:
- buf.WriteString("Ch = ")
- buf.WriteString(CharDescription(rune(c.Codes[offset+1])))
-
- case Set, Setrep, Setloop, Setlazy:
- buf.WriteString("Set = ")
- buf.WriteString(c.Sets[c.Codes[offset+1]].String())
-
- case Multi:
- fmt.Fprintf(buf, "String = %s", string(c.Strings[c.Codes[offset+1]]))
-
- case Ref, Testref:
- fmt.Fprintf(buf, "Index = %d", c.Codes[offset+1])
-
- case Capturemark:
- fmt.Fprintf(buf, "Index = %d", c.Codes[offset+1])
- if c.Codes[offset+2] != -1 {
- fmt.Fprintf(buf, ", Unindex = %d", c.Codes[offset+2])
- }
-
- case Nullcount, Setcount:
- fmt.Fprintf(buf, "Value = %d", c.Codes[offset+1])
-
- case Goto, Lazybranch, Branchmark, Lazybranchmark, Branchcount, Lazybranchcount:
- fmt.Fprintf(buf, "Addr = %d", c.Codes[offset+1])
- }
-
- switch op {
- case Onerep, Notonerep, Oneloop, Notoneloop, Onelazy, Notonelazy, Setrep, Setloop, Setlazy:
- buf.WriteString(", Rep = ")
- if c.Codes[offset+2] == math.MaxInt32 {
- buf.WriteString("inf")
- } else {
- fmt.Fprintf(buf, "%d", c.Codes[offset+2])
- }
-
- case Branchcount, Lazybranchcount:
- buf.WriteString(", Limit = ")
- if c.Codes[offset+2] == math.MaxInt32 {
- buf.WriteString("inf")
- } else {
- fmt.Fprintf(buf, "%d", c.Codes[offset+2])
- }
-
- }
-
- buf.WriteString(")")
-
- return buf.String()
-}
-
-func (c *Code) Dump() string {
- buf := &bytes.Buffer{}
-
- if c.RightToLeft {
- fmt.Fprintln(buf, "Direction: right-to-left")
- } else {
- fmt.Fprintln(buf, "Direction: left-to-right")
- }
- if c.FcPrefix == nil {
- fmt.Fprintln(buf, "Firstchars: n/a")
- } else {
- fmt.Fprintf(buf, "Firstchars: %v\n", c.FcPrefix.PrefixSet.String())
- }
-
- if c.BmPrefix == nil {
- fmt.Fprintln(buf, "Prefix: n/a")
- } else {
- fmt.Fprintf(buf, "Prefix: %v\n", Escape(c.BmPrefix.String()))
- }
-
- fmt.Fprintf(buf, "Anchors: %v\n", c.Anchors)
- fmt.Fprintln(buf)
-
- if c.BmPrefix != nil {
- fmt.Fprintln(buf, "BoyerMoore:")
- fmt.Fprintln(buf, c.BmPrefix.Dump(" "))
- }
- for i := 0; i < len(c.Codes); i += opcodeSize(InstOp(c.Codes[i])) {
- fmt.Fprintln(buf, c.OpcodeDescription(i))
- }
-
- return buf.String()
-}
diff --git a/vendor/github.com/dlclark/regexp2/syntax/escape.go b/vendor/github.com/dlclark/regexp2/syntax/escape.go
deleted file mode 100644
index 609df10..0000000
--- a/vendor/github.com/dlclark/regexp2/syntax/escape.go
+++ /dev/null
@@ -1,94 +0,0 @@
-package syntax
-
-import (
- "bytes"
- "strconv"
- "strings"
- "unicode"
-)
-
-func Escape(input string) string {
- b := &bytes.Buffer{}
- for _, r := range input {
- escape(b, r, false)
- }
- return b.String()
-}
-
-const meta = `\.+*?()|[]{}^$# `
-
-func escape(b *bytes.Buffer, r rune, force bool) {
- if unicode.IsPrint(r) {
- if strings.IndexRune(meta, r) >= 0 || force {
- b.WriteRune('\\')
- }
- b.WriteRune(r)
- return
- }
-
- switch r {
- case '\a':
- b.WriteString(`\a`)
- case '\f':
- b.WriteString(`\f`)
- case '\n':
- b.WriteString(`\n`)
- case '\r':
- b.WriteString(`\r`)
- case '\t':
- b.WriteString(`\t`)
- case '\v':
- b.WriteString(`\v`)
- default:
- if r < 0x100 {
- b.WriteString(`\x`)
- s := strconv.FormatInt(int64(r), 16)
- if len(s) == 1 {
- b.WriteRune('0')
- }
- b.WriteString(s)
- break
- }
- b.WriteString(`\u`)
- b.WriteString(strconv.FormatInt(int64(r), 16))
- }
-}
-
-func Unescape(input string) (string, error) {
- idx := strings.IndexRune(input, '\\')
- // no slashes means no unescape needed
- if idx == -1 {
- return input, nil
- }
-
- buf := bytes.NewBufferString(input[:idx])
- // get the runes for the rest of the string -- we're going full parser scan on this
-
- p := parser{}
- p.setPattern(input[idx+1:])
- for {
- if p.rightMost() {
- return "", p.getErr(ErrIllegalEndEscape)
- }
- r, err := p.scanCharEscape()
- if err != nil {
- return "", err
- }
- buf.WriteRune(r)
- // are we done?
- if p.rightMost() {
- return buf.String(), nil
- }
-
- r = p.moveRightGetChar()
- for r != '\\' {
- buf.WriteRune(r)
- if p.rightMost() {
- // we're done, no more slashes
- return buf.String(), nil
- }
- // keep scanning until we get another slash
- r = p.moveRightGetChar()
- }
- }
-}
diff --git a/vendor/github.com/dlclark/regexp2/syntax/fuzz.go b/vendor/github.com/dlclark/regexp2/syntax/fuzz.go
deleted file mode 100644
index ee86386..0000000
--- a/vendor/github.com/dlclark/regexp2/syntax/fuzz.go
+++ /dev/null
@@ -1,20 +0,0 @@
-// +build gofuzz
-
-package syntax
-
-// Fuzz is the input point for go-fuzz
-func Fuzz(data []byte) int {
- sdata := string(data)
- tree, err := Parse(sdata, RegexOptions(0))
- if err != nil {
- return 0
- }
-
- // translate it to code
- _, err = Write(tree)
- if err != nil {
- panic(err)
- }
-
- return 1
-}
diff --git a/vendor/github.com/dlclark/regexp2/syntax/parser.go b/vendor/github.com/dlclark/regexp2/syntax/parser.go
deleted file mode 100644
index da14f98..0000000
--- a/vendor/github.com/dlclark/regexp2/syntax/parser.go
+++ /dev/null
@@ -1,2213 +0,0 @@
-package syntax
-
-import (
- "fmt"
- "math"
- "os"
- "sort"
- "strconv"
- "unicode"
-)
-
-type RegexOptions int32
-
-const (
- IgnoreCase RegexOptions = 0x0001 // "i"
- Multiline = 0x0002 // "m"
- ExplicitCapture = 0x0004 // "n"
- Compiled = 0x0008 // "c"
- Singleline = 0x0010 // "s"
- IgnorePatternWhitespace = 0x0020 // "x"
- RightToLeft = 0x0040 // "r"
- Debug = 0x0080 // "d"
- ECMAScript = 0x0100 // "e"
- RE2 = 0x0200 // RE2 compat mode
-)
-
-func optionFromCode(ch rune) RegexOptions {
- // case-insensitive
- switch ch {
- case 'i', 'I':
- return IgnoreCase
- case 'r', 'R':
- return RightToLeft
- case 'm', 'M':
- return Multiline
- case 'n', 'N':
- return ExplicitCapture
- case 's', 'S':
- return Singleline
- case 'x', 'X':
- return IgnorePatternWhitespace
- case 'd', 'D':
- return Debug
- case 'e', 'E':
- return ECMAScript
- default:
- return 0
- }
-}
-
-// An Error describes a failure to parse a regular expression
-// and gives the offending expression.
-type Error struct {
- Code ErrorCode
- Expr string
- Args []interface{}
-}
-
-func (e *Error) Error() string {
- if len(e.Args) == 0 {
- return "error parsing regexp: " + e.Code.String() + " in `" + e.Expr + "`"
- }
- return "error parsing regexp: " + fmt.Sprintf(e.Code.String(), e.Args...) + " in `" + e.Expr + "`"
-}
-
-// An ErrorCode describes a failure to parse a regular expression.
-type ErrorCode string
-
-const (
- // internal issue
- ErrInternalError ErrorCode = "regexp/syntax: internal error"
- // Parser errors
- ErrUnterminatedComment = "unterminated comment"
- ErrInvalidCharRange = "invalid character class range"
- ErrInvalidRepeatSize = "invalid repeat count"
- ErrInvalidUTF8 = "invalid UTF-8"
- ErrCaptureGroupOutOfRange = "capture group number out of range"
- ErrUnexpectedParen = "unexpected )"
- ErrMissingParen = "missing closing )"
- ErrMissingBrace = "missing closing }"
- ErrInvalidRepeatOp = "invalid nested repetition operator"
- ErrMissingRepeatArgument = "missing argument to repetition operator"
- ErrConditionalExpression = "illegal conditional (?(...)) expression"
- ErrTooManyAlternates = "too many | in (?()|)"
- ErrUnrecognizedGrouping = "unrecognized grouping construct: (%v"
- ErrInvalidGroupName = "invalid group name: group names must begin with a word character and have a matching terminator"
- ErrCapNumNotZero = "capture number cannot be zero"
- ErrUndefinedBackRef = "reference to undefined group number %v"
- ErrUndefinedNameRef = "reference to undefined group name %v"
- ErrAlternationCantCapture = "alternation conditions do not capture and cannot be named"
- ErrAlternationCantHaveComment = "alternation conditions cannot be comments"
- ErrMalformedReference = "(?(%v) ) malformed"
- ErrUndefinedReference = "(?(%v) ) reference to undefined group"
- ErrIllegalEndEscape = "illegal \\ at end of pattern"
- ErrMalformedSlashP = "malformed \\p{X} character escape"
- ErrIncompleteSlashP = "incomplete \\p{X} character escape"
- ErrUnknownSlashP = "unknown unicode category, script, or property '%v'"
- ErrUnrecognizedEscape = "unrecognized escape sequence \\%v"
- ErrMissingControl = "missing control character"
- ErrUnrecognizedControl = "unrecognized control character"
- ErrTooFewHex = "insufficient hexadecimal digits"
- ErrInvalidHex = "hex values may not be larger than 0x10FFFF"
- ErrMalformedNameRef = "malformed \\k<...> named back reference"
- ErrBadClassInCharRange = "cannot include class \\%v in character range"
- ErrUnterminatedBracket = "unterminated [] set"
- ErrSubtractionMustBeLast = "a subtraction must be the last element in a character class"
- ErrReversedCharRange = "[x-y] range in reverse order"
-)
-
-func (e ErrorCode) String() string {
- return string(e)
-}
-
-type parser struct {
- stack *regexNode
- group *regexNode
- alternation *regexNode
- concatenation *regexNode
- unit *regexNode
-
- patternRaw string
- pattern []rune
-
- currentPos int
- specialCase *unicode.SpecialCase
-
- autocap int
- capcount int
- captop int
- capsize int
-
- caps map[int]int
- capnames map[string]int
-
- capnumlist []int
- capnamelist []string
-
- options RegexOptions
- optionsStack []RegexOptions
- ignoreNextParen bool
-}
-
-const (
- maxValueDiv10 int = math.MaxInt32 / 10
- maxValueMod10 = math.MaxInt32 % 10
-)
-
-// Parse converts a regex string into a parse tree
-func Parse(re string, op RegexOptions) (*RegexTree, error) {
- p := parser{
- options: op,
- caps: make(map[int]int),
- }
- p.setPattern(re)
-
- if err := p.countCaptures(); err != nil {
- return nil, err
- }
-
- p.reset(op)
- root, err := p.scanRegex()
-
- if err != nil {
- return nil, err
- }
- tree := &RegexTree{
- root: root,
- caps: p.caps,
- capnumlist: p.capnumlist,
- captop: p.captop,
- Capnames: p.capnames,
- Caplist: p.capnamelist,
- options: op,
- }
-
- if tree.options&Debug > 0 {
- os.Stdout.WriteString(tree.Dump())
- }
-
- return tree, nil
-}
-
-func (p *parser) setPattern(pattern string) {
- p.patternRaw = pattern
- p.pattern = make([]rune, 0, len(pattern))
-
- //populate our rune array to handle utf8 encoding
- for _, r := range pattern {
- p.pattern = append(p.pattern, r)
- }
-}
-func (p *parser) getErr(code ErrorCode, args ...interface{}) error {
- return &Error{Code: code, Expr: p.patternRaw, Args: args}
-}
-
-func (p *parser) noteCaptureSlot(i, pos int) {
- if _, ok := p.caps[i]; !ok {
- // the rhs of the hashtable isn't used in the parser
- p.caps[i] = pos
- p.capcount++
-
- if p.captop <= i {
- if i == math.MaxInt32 {
- p.captop = i
- } else {
- p.captop = i + 1
- }
- }
- }
-}
-
-func (p *parser) noteCaptureName(name string, pos int) {
- if p.capnames == nil {
- p.capnames = make(map[string]int)
- }
-
- if _, ok := p.capnames[name]; !ok {
- p.capnames[name] = pos
- p.capnamelist = append(p.capnamelist, name)
- }
-}
-
-func (p *parser) assignNameSlots() {
- if p.capnames != nil {
- for _, name := range p.capnamelist {
- for p.isCaptureSlot(p.autocap) {
- p.autocap++
- }
- pos := p.capnames[name]
- p.capnames[name] = p.autocap
- p.noteCaptureSlot(p.autocap, pos)
-
- p.autocap++
- }
- }
-
- // if the caps array has at least one gap, construct the list of used slots
- if p.capcount < p.captop {
- p.capnumlist = make([]int, p.capcount)
- i := 0
-
- for k := range p.caps {
- p.capnumlist[i] = k
- i++
- }
-
- sort.Ints(p.capnumlist)
- }
-
- // merge capsnumlist into capnamelist
- if p.capnames != nil || p.capnumlist != nil {
- var oldcapnamelist []string
- var next int
- var k int
-
- if p.capnames == nil {
- oldcapnamelist = nil
- p.capnames = make(map[string]int)
- p.capnamelist = []string{}
- next = -1
- } else {
- oldcapnamelist = p.capnamelist
- p.capnamelist = []string{}
- next = p.capnames[oldcapnamelist[0]]
- }
-
- for i := 0; i < p.capcount; i++ {
- j := i
- if p.capnumlist != nil {
- j = p.capnumlist[i]
- }
-
- if next == j {
- p.capnamelist = append(p.capnamelist, oldcapnamelist[k])
- k++
-
- if k == len(oldcapnamelist) {
- next = -1
- } else {
- next = p.capnames[oldcapnamelist[k]]
- }
-
- } else {
- //feature: culture?
- str := strconv.Itoa(j)
- p.capnamelist = append(p.capnamelist, str)
- p.capnames[str] = j
- }
- }
- }
-}
-
-func (p *parser) consumeAutocap() int {
- r := p.autocap
- p.autocap++
- return r
-}
-
-// CountCaptures is a prescanner for deducing the slots used for
-// captures by doing a partial tokenization of the pattern.
-func (p *parser) countCaptures() error {
- var ch rune
-
- p.noteCaptureSlot(0, 0)
-
- p.autocap = 1
-
- for p.charsRight() > 0 {
- pos := p.textpos()
- ch = p.moveRightGetChar()
- switch ch {
- case '\\':
- if p.charsRight() > 0 {
- p.scanBackslash(true)
- }
-
- case '#':
- if p.useOptionX() {
- p.moveLeft()
- p.scanBlank()
- }
-
- case '[':
- p.scanCharSet(false, true)
-
- case ')':
- if !p.emptyOptionsStack() {
- p.popOptions()
- }
-
- case '(':
- if p.charsRight() >= 2 && p.rightChar(1) == '#' && p.rightChar(0) == '?' {
- p.moveLeft()
- p.scanBlank()
- } else {
- p.pushOptions()
- if p.charsRight() > 0 && p.rightChar(0) == '?' {
- // we have (?...
- p.moveRight(1)
-
- if p.charsRight() > 1 && (p.rightChar(0) == '<' || p.rightChar(0) == '\'') {
- // named group: (?<... or (?'...
-
- p.moveRight(1)
- ch = p.rightChar(0)
-
- if ch != '0' && IsWordChar(ch) {
- if ch >= '1' && ch <= '9' {
- dec, err := p.scanDecimal()
- if err != nil {
- return err
- }
- p.noteCaptureSlot(dec, pos)
- } else {
- p.noteCaptureName(p.scanCapname(), pos)
- }
- }
- } else if p.useRE2() && p.charsRight() > 2 && (p.rightChar(0) == 'P' && p.rightChar(1) == '<') {
- // RE2-compat (?P<)
- p.moveRight(2)
- ch = p.rightChar(0)
- if IsWordChar(ch) {
- p.noteCaptureName(p.scanCapname(), pos)
- }
-
- } else {
- // (?...
-
- // get the options if it's an option construct (?cimsx-cimsx...)
- p.scanOptions()
-
- if p.charsRight() > 0 {
- if p.rightChar(0) == ')' {
- // (?cimsx-cimsx)
- p.moveRight(1)
- p.popKeepOptions()
- } else if p.rightChar(0) == '(' {
- // alternation construct: (?(foo)yes|no)
- // ignore the next paren so we don't capture the condition
- p.ignoreNextParen = true
-
- // break from here so we don't reset ignoreNextParen
- continue
- }
- }
- }
- } else {
- if !p.useOptionN() && !p.ignoreNextParen {
- p.noteCaptureSlot(p.consumeAutocap(), pos)
- }
- }
- }
-
- p.ignoreNextParen = false
-
- }
- }
-
- p.assignNameSlots()
- return nil
-}
-
-func (p *parser) reset(topopts RegexOptions) {
- p.currentPos = 0
- p.autocap = 1
- p.ignoreNextParen = false
-
- if len(p.optionsStack) > 0 {
- p.optionsStack = p.optionsStack[:0]
- }
-
- p.options = topopts
- p.stack = nil
-}
-
-func (p *parser) scanRegex() (*regexNode, error) {
- ch := '@' // nonspecial ch, means at beginning
- isQuant := false
-
- p.startGroup(newRegexNodeMN(ntCapture, p.options, 0, -1))
-
- for p.charsRight() > 0 {
- wasPrevQuantifier := isQuant
- isQuant = false
-
- if err := p.scanBlank(); err != nil {
- return nil, err
- }
-
- startpos := p.textpos()
-
- // move past all of the normal characters. We'll stop when we hit some kind of control character,
- // or if IgnorePatternWhiteSpace is on, we'll stop when we see some whitespace.
- if p.useOptionX() {
- for p.charsRight() > 0 {
- ch = p.rightChar(0)
- //UGLY: clean up, this is ugly
- if !(!isStopperX(ch) || (ch == '{' && !p.isTrueQuantifier())) {
- break
- }
- p.moveRight(1)
- }
- } else {
- for p.charsRight() > 0 {
- ch = p.rightChar(0)
- if !(!isSpecial(ch) || ch == '{' && !p.isTrueQuantifier()) {
- break
- }
- p.moveRight(1)
- }
- }
-
- endpos := p.textpos()
-
- p.scanBlank()
-
- if p.charsRight() == 0 {
- ch = '!' // nonspecial, means at end
- } else if ch = p.rightChar(0); isSpecial(ch) {
- isQuant = isQuantifier(ch)
- p.moveRight(1)
- } else {
- ch = ' ' // nonspecial, means at ordinary char
- }
-
- if startpos < endpos {
- cchUnquantified := endpos - startpos
- if isQuant {
- cchUnquantified--
- }
- wasPrevQuantifier = false
-
- if cchUnquantified > 0 {
- p.addToConcatenate(startpos, cchUnquantified, false)
- }
-
- if isQuant {
- p.addUnitOne(p.charAt(endpos - 1))
- }
- }
-
- switch ch {
- case '!':
- goto BreakOuterScan
-
- case ' ':
- goto ContinueOuterScan
-
- case '[':
- cc, err := p.scanCharSet(p.useOptionI(), false)
- if err != nil {
- return nil, err
- }
- p.addUnitSet(cc)
-
- case '(':
- p.pushOptions()
-
- if grouper, err := p.scanGroupOpen(); err != nil {
- return nil, err
- } else if grouper == nil {
- p.popKeepOptions()
- } else {
- p.pushGroup()
- p.startGroup(grouper)
- }
-
- continue
-
- case '|':
- p.addAlternate()
- goto ContinueOuterScan
-
- case ')':
- if p.emptyStack() {
- return nil, p.getErr(ErrUnexpectedParen)
- }
-
- if err := p.addGroup(); err != nil {
- return nil, err
- }
- if err := p.popGroup(); err != nil {
- return nil, err
- }
- p.popOptions()
-
- if p.unit == nil {
- goto ContinueOuterScan
- }
-
- case '\\':
- n, err := p.scanBackslash(false)
- if err != nil {
- return nil, err
- }
- p.addUnitNode(n)
-
- case '^':
- if p.useOptionM() {
- p.addUnitType(ntBol)
- } else {
- p.addUnitType(ntBeginning)
- }
-
- case '$':
- if p.useOptionM() {
- p.addUnitType(ntEol)
- } else {
- p.addUnitType(ntEndZ)
- }
-
- case '.':
- if p.useOptionE() {
- p.addUnitSet(ECMAAnyClass())
- } else if p.useOptionS() {
- p.addUnitSet(AnyClass())
- } else {
- p.addUnitNotone('\n')
- }
-
- case '{', '*', '+', '?':
- if p.unit == nil {
- if wasPrevQuantifier {
- return nil, p.getErr(ErrInvalidRepeatOp)
- } else {
- return nil, p.getErr(ErrMissingRepeatArgument)
- }
- }
- p.moveLeft()
-
- default:
- return nil, p.getErr(ErrInternalError)
- }
-
- if err := p.scanBlank(); err != nil {
- return nil, err
- }
-
- if p.charsRight() > 0 {
- isQuant = p.isTrueQuantifier()
- }
- if p.charsRight() == 0 || !isQuant {
- //maintain odd C# assignment order -- not sure if required, could clean up?
- p.addConcatenate()
- goto ContinueOuterScan
- }
-
- ch = p.moveRightGetChar()
-
- // Handle quantifiers
- for p.unit != nil {
- var min, max int
- var lazy bool
-
- switch ch {
- case '*':
- min = 0
- max = math.MaxInt32
-
- case '?':
- min = 0
- max = 1
-
- case '+':
- min = 1
- max = math.MaxInt32
-
- case '{':
- {
- var err error
- startpos = p.textpos()
- if min, err = p.scanDecimal(); err != nil {
- return nil, err
- }
- max = min
- if startpos < p.textpos() {
- if p.charsRight() > 0 && p.rightChar(0) == ',' {
- p.moveRight(1)
- if p.charsRight() == 0 || p.rightChar(0) == '}' {
- max = math.MaxInt32
- } else {
- if max, err = p.scanDecimal(); err != nil {
- return nil, err
- }
- }
- }
- }
-
- if startpos == p.textpos() || p.charsRight() == 0 || p.moveRightGetChar() != '}' {
- p.addConcatenate()
- p.textto(startpos - 1)
- goto ContinueOuterScan
- }
- }
-
- default:
- return nil, p.getErr(ErrInternalError)
- }
-
- if err := p.scanBlank(); err != nil {
- return nil, err
- }
-
- if p.charsRight() == 0 || p.rightChar(0) != '?' {
- lazy = false
- } else {
- p.moveRight(1)
- lazy = true
- }
-
- if min > max {
- return nil, p.getErr(ErrInvalidRepeatSize)
- }
-
- p.addConcatenate3(lazy, min, max)
- }
-
- ContinueOuterScan:
- }
-
-BreakOuterScan:
- ;
-
- if !p.emptyStack() {
- return nil, p.getErr(ErrMissingParen)
- }
-
- if err := p.addGroup(); err != nil {
- return nil, err
- }
-
- return p.unit, nil
-
-}
-
-/*
- * Simple parsing for replacement patterns
- */
-func (p *parser) scanReplacement() (*regexNode, error) {
- var c, startpos int
-
- p.concatenation = newRegexNode(ntConcatenate, p.options)
-
- for {
- c = p.charsRight()
- if c == 0 {
- break
- }
-
- startpos = p.textpos()
-
- for c > 0 && p.rightChar(0) != '$' {
- p.moveRight(1)
- c--
- }
-
- p.addToConcatenate(startpos, p.textpos()-startpos, true)
-
- if c > 0 {
- if p.moveRightGetChar() == '$' {
- n, err := p.scanDollar()
- if err != nil {
- return nil, err
- }
- p.addUnitNode(n)
- }
- p.addConcatenate()
- }
- }
-
- return p.concatenation, nil
-}
-
-/*
- * Scans $ patterns recognized within replacement patterns
- */
-func (p *parser) scanDollar() (*regexNode, error) {
- if p.charsRight() == 0 {
- return newRegexNodeCh(ntOne, p.options, '$'), nil
- }
-
- ch := p.rightChar(0)
- angled := false
- backpos := p.textpos()
- lastEndPos := backpos
-
- // Note angle
-
- if ch == '{' && p.charsRight() > 1 {
- angled = true
- p.moveRight(1)
- ch = p.rightChar(0)
- }
-
- // Try to parse backreference: \1 or \{1} or \{cap}
-
- if ch >= '0' && ch <= '9' {
- if !angled && p.useOptionE() {
- capnum := -1
- newcapnum := int(ch - '0')
- p.moveRight(1)
- if p.isCaptureSlot(newcapnum) {
- capnum = newcapnum
- lastEndPos = p.textpos()
- }
-
- for p.charsRight() > 0 {
- ch = p.rightChar(0)
- if ch < '0' || ch > '9' {
- break
- }
- digit := int(ch - '0')
- if newcapnum > maxValueDiv10 || (newcapnum == maxValueDiv10 && digit > maxValueMod10) {
- return nil, p.getErr(ErrCaptureGroupOutOfRange)
- }
-
- newcapnum = newcapnum*10 + digit
-
- p.moveRight(1)
- if p.isCaptureSlot(newcapnum) {
- capnum = newcapnum
- lastEndPos = p.textpos()
- }
- }
- p.textto(lastEndPos)
- if capnum >= 0 {
- return newRegexNodeM(ntRef, p.options, capnum), nil
- }
- } else {
- capnum, err := p.scanDecimal()
- if err != nil {
- return nil, err
- }
- if !angled || p.charsRight() > 0 && p.moveRightGetChar() == '}' {
- if p.isCaptureSlot(capnum) {
- return newRegexNodeM(ntRef, p.options, capnum), nil
- }
- }
- }
- } else if angled && IsWordChar(ch) {
- capname := p.scanCapname()
-
- if p.charsRight() > 0 && p.moveRightGetChar() == '}' {
- if p.isCaptureName(capname) {
- return newRegexNodeM(ntRef, p.options, p.captureSlotFromName(capname)), nil
- }
- }
- } else if !angled {
- capnum := 1
-
- switch ch {
- case '$':
- p.moveRight(1)
- return newRegexNodeCh(ntOne, p.options, '$'), nil
- case '&':
- capnum = 0
- case '`':
- capnum = replaceLeftPortion
- case '\'':
- capnum = replaceRightPortion
- case '+':
- capnum = replaceLastGroup
- case '_':
- capnum = replaceWholeString
- }
-
- if capnum != 1 {
- p.moveRight(1)
- return newRegexNodeM(ntRef, p.options, capnum), nil
- }
- }
-
- // unrecognized $: literalize
-
- p.textto(backpos)
- return newRegexNodeCh(ntOne, p.options, '$'), nil
-}
-
-// scanGroupOpen scans chars following a '(' (not counting the '('), and returns
-// a RegexNode for the type of group scanned, or nil if the group
-// simply changed options (?cimsx-cimsx) or was a comment (#...).
-func (p *parser) scanGroupOpen() (*regexNode, error) {
- var ch rune
- var nt nodeType
- var err error
- close := '>'
- start := p.textpos()
-
- // just return a RegexNode if we have:
- // 1. "(" followed by nothing
- // 2. "(x" where x != ?
- // 3. "(?)"
- if p.charsRight() == 0 || p.rightChar(0) != '?' || (p.rightChar(0) == '?' && (p.charsRight() > 1 && p.rightChar(1) == ')')) {
- if p.useOptionN() || p.ignoreNextParen {
- p.ignoreNextParen = false
- return newRegexNode(ntGroup, p.options), nil
- }
- return newRegexNodeMN(ntCapture, p.options, p.consumeAutocap(), -1), nil
- }
-
- p.moveRight(1)
-
- for {
- if p.charsRight() == 0 {
- break
- }
-
- switch ch = p.moveRightGetChar(); ch {
- case ':':
- nt = ntGroup
-
- case '=':
- p.options &= ^RightToLeft
- nt = ntRequire
-
- case '!':
- p.options &= ^RightToLeft
- nt = ntPrevent
-
- case '>':
- nt = ntGreedy
-
- case '\'':
- close = '\''
- fallthrough
-
- case '<':
- if p.charsRight() == 0 {
- goto BreakRecognize
- }
-
- switch ch = p.moveRightGetChar(); ch {
- case '=':
- if close == '\'' {
- goto BreakRecognize
- }
-
- p.options |= RightToLeft
- nt = ntRequire
-
- case '!':
- if close == '\'' {
- goto BreakRecognize
- }
-
- p.options |= RightToLeft
- nt = ntPrevent
-
- default:
- p.moveLeft()
- capnum := -1
- uncapnum := -1
- proceed := false
-
- // grab part before -
-
- if ch >= '0' && ch <= '9' {
- if capnum, err = p.scanDecimal(); err != nil {
- return nil, err
- }
-
- if !p.isCaptureSlot(capnum) {
- capnum = -1
- }
-
- // check if we have bogus characters after the number
- if p.charsRight() > 0 && !(p.rightChar(0) == close || p.rightChar(0) == '-') {
- return nil, p.getErr(ErrInvalidGroupName)
- }
- if capnum == 0 {
- return nil, p.getErr(ErrCapNumNotZero)
- }
- } else if IsWordChar(ch) {
- capname := p.scanCapname()
-
- if p.isCaptureName(capname) {
- capnum = p.captureSlotFromName(capname)
- }
-
- // check if we have bogus character after the name
- if p.charsRight() > 0 && !(p.rightChar(0) == close || p.rightChar(0) == '-') {
- return nil, p.getErr(ErrInvalidGroupName)
- }
- } else if ch == '-' {
- proceed = true
- } else {
- // bad group name - starts with something other than a word character and isn't a number
- return nil, p.getErr(ErrInvalidGroupName)
- }
-
- // grab part after - if any
-
- if (capnum != -1 || proceed == true) && p.charsRight() > 0 && p.rightChar(0) == '-' {
- p.moveRight(1)
-
- //no more chars left, no closing char, etc
- if p.charsRight() == 0 {
- return nil, p.getErr(ErrInvalidGroupName)
- }
-
- ch = p.rightChar(0)
- if ch >= '0' && ch <= '9' {
- if uncapnum, err = p.scanDecimal(); err != nil {
- return nil, err
- }
-
- if !p.isCaptureSlot(uncapnum) {
- return nil, p.getErr(ErrUndefinedBackRef, uncapnum)
- }
-
- // check if we have bogus characters after the number
- if p.charsRight() > 0 && p.rightChar(0) != close {
- return nil, p.getErr(ErrInvalidGroupName)
- }
- } else if IsWordChar(ch) {
- uncapname := p.scanCapname()
-
- if !p.isCaptureName(uncapname) {
- return nil, p.getErr(ErrUndefinedNameRef, uncapname)
- }
- uncapnum = p.captureSlotFromName(uncapname)
-
- // check if we have bogus character after the name
- if p.charsRight() > 0 && p.rightChar(0) != close {
- return nil, p.getErr(ErrInvalidGroupName)
- }
- } else {
- // bad group name - starts with something other than a word character and isn't a number
- return nil, p.getErr(ErrInvalidGroupName)
- }
- }
-
- // actually make the node
-
- if (capnum != -1 || uncapnum != -1) && p.charsRight() > 0 && p.moveRightGetChar() == close {
- return newRegexNodeMN(ntCapture, p.options, capnum, uncapnum), nil
- }
- goto BreakRecognize
- }
-
- case '(':
- // alternation construct (?(...) | )
-
- parenPos := p.textpos()
- if p.charsRight() > 0 {
- ch = p.rightChar(0)
-
- // check if the alternation condition is a backref
- if ch >= '0' && ch <= '9' {
- var capnum int
- if capnum, err = p.scanDecimal(); err != nil {
- return nil, err
- }
- if p.charsRight() > 0 && p.moveRightGetChar() == ')' {
- if p.isCaptureSlot(capnum) {
- return newRegexNodeM(ntTestref, p.options, capnum), nil
- }
- return nil, p.getErr(ErrUndefinedReference, capnum)
- }
-
- return nil, p.getErr(ErrMalformedReference, capnum)
-
- } else if IsWordChar(ch) {
- capname := p.scanCapname()
-
- if p.isCaptureName(capname) && p.charsRight() > 0 && p.moveRightGetChar() == ')' {
- return newRegexNodeM(ntTestref, p.options, p.captureSlotFromName(capname)), nil
- }
- }
- }
- // not a backref
- nt = ntTestgroup
- p.textto(parenPos - 1) // jump to the start of the parentheses
- p.ignoreNextParen = true // but make sure we don't try to capture the insides
-
- charsRight := p.charsRight()
- if charsRight >= 3 && p.rightChar(1) == '?' {
- rightchar2 := p.rightChar(2)
- // disallow comments in the condition
- if rightchar2 == '#' {
- return nil, p.getErr(ErrAlternationCantHaveComment)
- }
-
- // disallow named capture group (?<..>..) in the condition
- if rightchar2 == '\'' {
- return nil, p.getErr(ErrAlternationCantCapture)
- }
-
- if charsRight >= 4 && (rightchar2 == '<' && p.rightChar(3) != '!' && p.rightChar(3) != '=') {
- return nil, p.getErr(ErrAlternationCantCapture)
- }
- }
-
- case 'P':
- if p.useRE2() {
- // support for P syntax
- if p.charsRight() < 3 {
- goto BreakRecognize
- }
-
- ch = p.moveRightGetChar()
- if ch != '<' {
- goto BreakRecognize
- }
-
- ch = p.moveRightGetChar()
- p.moveLeft()
-
- if IsWordChar(ch) {
- capnum := -1
- capname := p.scanCapname()
-
- if p.isCaptureName(capname) {
- capnum = p.captureSlotFromName(capname)
- }
-
- // check if we have bogus character after the name
- if p.charsRight() > 0 && p.rightChar(0) != '>' {
- return nil, p.getErr(ErrInvalidGroupName)
- }
-
- // actually make the node
-
- if capnum != -1 && p.charsRight() > 0 && p.moveRightGetChar() == '>' {
- return newRegexNodeMN(ntCapture, p.options, capnum, -1), nil
- }
- goto BreakRecognize
-
- } else {
- // bad group name - starts with something other than a word character and isn't a number
- return nil, p.getErr(ErrInvalidGroupName)
- }
- }
- // if we're not using RE2 compat mode then
- // we just behave like normal
- fallthrough
-
- default:
- p.moveLeft()
-
- nt = ntGroup
- // disallow options in the children of a testgroup node
- if p.group.t != ntTestgroup {
- p.scanOptions()
- }
- if p.charsRight() == 0 {
- goto BreakRecognize
- }
-
- if ch = p.moveRightGetChar(); ch == ')' {
- return nil, nil
- }
-
- if ch != ':' {
- goto BreakRecognize
- }
-
- }
-
- return newRegexNode(nt, p.options), nil
- }
-
-BreakRecognize:
-
- // break Recognize comes here
-
- return nil, p.getErr(ErrUnrecognizedGrouping, string(p.pattern[start:p.textpos()]))
-}
-
-// scans backslash specials and basics
-func (p *parser) scanBackslash(scanOnly bool) (*regexNode, error) {
-
- if p.charsRight() == 0 {
- return nil, p.getErr(ErrIllegalEndEscape)
- }
-
- switch ch := p.rightChar(0); ch {
- case 'b', 'B', 'A', 'G', 'Z', 'z':
- p.moveRight(1)
- return newRegexNode(p.typeFromCode(ch), p.options), nil
-
- case 'w':
- p.moveRight(1)
- if p.useOptionE() {
- return newRegexNodeSet(ntSet, p.options, ECMAWordClass()), nil
- }
- return newRegexNodeSet(ntSet, p.options, WordClass()), nil
-
- case 'W':
- p.moveRight(1)
- if p.useOptionE() {
- return newRegexNodeSet(ntSet, p.options, NotECMAWordClass()), nil
- }
- return newRegexNodeSet(ntSet, p.options, NotWordClass()), nil
-
- case 's':
- p.moveRight(1)
- if p.useOptionE() {
- return newRegexNodeSet(ntSet, p.options, ECMASpaceClass()), nil
- }
- return newRegexNodeSet(ntSet, p.options, SpaceClass()), nil
-
- case 'S':
- p.moveRight(1)
- if p.useOptionE() {
- return newRegexNodeSet(ntSet, p.options, NotECMASpaceClass()), nil
- }
- return newRegexNodeSet(ntSet, p.options, NotSpaceClass()), nil
-
- case 'd':
- p.moveRight(1)
- if p.useOptionE() {
- return newRegexNodeSet(ntSet, p.options, ECMADigitClass()), nil
- }
- return newRegexNodeSet(ntSet, p.options, DigitClass()), nil
-
- case 'D':
- p.moveRight(1)
- if p.useOptionE() {
- return newRegexNodeSet(ntSet, p.options, NotECMADigitClass()), nil
- }
- return newRegexNodeSet(ntSet, p.options, NotDigitClass()), nil
-
- case 'p', 'P':
- p.moveRight(1)
- prop, err := p.parseProperty()
- if err != nil {
- return nil, err
- }
- cc := &CharSet{}
- cc.addCategory(prop, (ch != 'p'), p.useOptionI(), p.patternRaw)
- if p.useOptionI() {
- cc.addLowercase()
- }
-
- return newRegexNodeSet(ntSet, p.options, cc), nil
-
- default:
- return p.scanBasicBackslash(scanOnly)
- }
-}
-
-// Scans \-style backreferences and character escapes
-func (p *parser) scanBasicBackslash(scanOnly bool) (*regexNode, error) {
- if p.charsRight() == 0 {
- return nil, p.getErr(ErrIllegalEndEscape)
- }
- angled := false
- close := '\x00'
-
- backpos := p.textpos()
- ch := p.rightChar(0)
-
- // allow \k instead of \, which is now deprecated
-
- if ch == 'k' {
- if p.charsRight() >= 2 {
- p.moveRight(1)
- ch = p.moveRightGetChar()
-
- if ch == '<' || ch == '\'' {
- angled = true
- if ch == '\'' {
- close = '\''
- } else {
- close = '>'
- }
- }
- }
-
- if !angled || p.charsRight() <= 0 {
- return nil, p.getErr(ErrMalformedNameRef)
- }
-
- ch = p.rightChar(0)
-
- } else if (ch == '<' || ch == '\'') && p.charsRight() > 1 { // Note angle without \g
- angled = true
- if ch == '\'' {
- close = '\''
- } else {
- close = '>'
- }
-
- p.moveRight(1)
- ch = p.rightChar(0)
- }
-
- // Try to parse backreference: \<1> or \
-
- if angled && ch >= '0' && ch <= '9' {
- capnum, err := p.scanDecimal()
- if err != nil {
- return nil, err
- }
-
- if p.charsRight() > 0 && p.moveRightGetChar() == close {
- if p.isCaptureSlot(capnum) {
- return newRegexNodeM(ntRef, p.options, capnum), nil
- }
- return nil, p.getErr(ErrUndefinedBackRef, capnum)
- }
- } else if !angled && ch >= '1' && ch <= '9' { // Try to parse backreference or octal: \1
- capnum, err := p.scanDecimal()
- if err != nil {
- return nil, err
- }
-
- if scanOnly {
- return nil, nil
- }
-
- if p.isCaptureSlot(capnum) {
- return newRegexNodeM(ntRef, p.options, capnum), nil
- }
- if capnum <= 9 && !p.useOptionE() {
- return nil, p.getErr(ErrUndefinedBackRef, capnum)
- }
-
- } else if angled && IsWordChar(ch) {
- capname := p.scanCapname()
-
- if p.charsRight() > 0 && p.moveRightGetChar() == close {
- if p.isCaptureName(capname) {
- return newRegexNodeM(ntRef, p.options, p.captureSlotFromName(capname)), nil
- }
- return nil, p.getErr(ErrUndefinedNameRef, capname)
- }
- }
-
- // Not backreference: must be char code
-
- p.textto(backpos)
- ch, err := p.scanCharEscape()
- if err != nil {
- return nil, err
- }
-
- if p.useOptionI() {
- ch = unicode.ToLower(ch)
- }
-
- return newRegexNodeCh(ntOne, p.options, ch), nil
-}
-
-// Scans X for \p{X} or \P{X}
-func (p *parser) parseProperty() (string, error) {
- if p.charsRight() < 3 {
- return "", p.getErr(ErrIncompleteSlashP)
- }
- ch := p.moveRightGetChar()
- if ch != '{' {
- return "", p.getErr(ErrMalformedSlashP)
- }
-
- startpos := p.textpos()
- for p.charsRight() > 0 {
- ch = p.moveRightGetChar()
- if !(IsWordChar(ch) || ch == '-') {
- p.moveLeft()
- break
- }
- }
- capname := string(p.pattern[startpos:p.textpos()])
-
- if p.charsRight() == 0 || p.moveRightGetChar() != '}' {
- return "", p.getErr(ErrIncompleteSlashP)
- }
-
- if !isValidUnicodeCat(capname) {
- return "", p.getErr(ErrUnknownSlashP, capname)
- }
-
- return capname, nil
-}
-
-// Returns ReNode type for zero-length assertions with a \ code.
-func (p *parser) typeFromCode(ch rune) nodeType {
- switch ch {
- case 'b':
- if p.useOptionE() {
- return ntECMABoundary
- }
- return ntBoundary
- case 'B':
- if p.useOptionE() {
- return ntNonECMABoundary
- }
- return ntNonboundary
- case 'A':
- return ntBeginning
- case 'G':
- return ntStart
- case 'Z':
- return ntEndZ
- case 'z':
- return ntEnd
- default:
- return ntNothing
- }
-}
-
-// Scans whitespace or x-mode comments.
-func (p *parser) scanBlank() error {
- if p.useOptionX() {
- for {
- for p.charsRight() > 0 && isSpace(p.rightChar(0)) {
- p.moveRight(1)
- }
-
- if p.charsRight() == 0 {
- break
- }
-
- if p.rightChar(0) == '#' {
- for p.charsRight() > 0 && p.rightChar(0) != '\n' {
- p.moveRight(1)
- }
- } else if p.charsRight() >= 3 && p.rightChar(2) == '#' &&
- p.rightChar(1) == '?' && p.rightChar(0) == '(' {
- for p.charsRight() > 0 && p.rightChar(0) != ')' {
- p.moveRight(1)
- }
- if p.charsRight() == 0 {
- return p.getErr(ErrUnterminatedComment)
- }
- p.moveRight(1)
- } else {
- break
- }
- }
- } else {
- for {
- if p.charsRight() < 3 || p.rightChar(2) != '#' ||
- p.rightChar(1) != '?' || p.rightChar(0) != '(' {
- return nil
- }
-
- for p.charsRight() > 0 && p.rightChar(0) != ')' {
- p.moveRight(1)
- }
- if p.charsRight() == 0 {
- return p.getErr(ErrUnterminatedComment)
- }
- p.moveRight(1)
- }
- }
- return nil
-}
-
-func (p *parser) scanCapname() string {
- startpos := p.textpos()
-
- for p.charsRight() > 0 {
- if !IsWordChar(p.moveRightGetChar()) {
- p.moveLeft()
- break
- }
- }
-
- return string(p.pattern[startpos:p.textpos()])
-}
-
-//Scans contents of [] (not including []'s), and converts to a set.
-func (p *parser) scanCharSet(caseInsensitive, scanOnly bool) (*CharSet, error) {
- ch := '\x00'
- chPrev := '\x00'
- inRange := false
- firstChar := true
- closed := false
-
- var cc *CharSet
- if !scanOnly {
- cc = &CharSet{}
- }
-
- if p.charsRight() > 0 && p.rightChar(0) == '^' {
- p.moveRight(1)
- if !scanOnly {
- cc.negate = true
- }
- }
-
- for ; p.charsRight() > 0; firstChar = false {
- fTranslatedChar := false
- ch = p.moveRightGetChar()
- if ch == ']' {
- if !firstChar {
- closed = true
- break
- } else if p.useOptionE() {
- if !scanOnly {
- cc.addRanges(NoneClass().ranges)
- }
- closed = true
- break
- }
-
- } else if ch == '\\' && p.charsRight() > 0 {
- switch ch = p.moveRightGetChar(); ch {
- case 'D', 'd':
- if !scanOnly {
- if inRange {
- return nil, p.getErr(ErrBadClassInCharRange, ch)
- }
- cc.addDigit(p.useOptionE(), ch == 'D', p.patternRaw)
- }
- continue
-
- case 'S', 's':
- if !scanOnly {
- if inRange {
- return nil, p.getErr(ErrBadClassInCharRange, ch)
- }
- cc.addSpace(p.useOptionE(), ch == 'S')
- }
- continue
-
- case 'W', 'w':
- if !scanOnly {
- if inRange {
- return nil, p.getErr(ErrBadClassInCharRange, ch)
- }
-
- cc.addWord(p.useOptionE(), ch == 'W')
- }
- continue
-
- case 'p', 'P':
- if !scanOnly {
- if inRange {
- return nil, p.getErr(ErrBadClassInCharRange, ch)
- }
- prop, err := p.parseProperty()
- if err != nil {
- return nil, err
- }
- cc.addCategory(prop, (ch != 'p'), caseInsensitive, p.patternRaw)
- } else {
- p.parseProperty()
- }
-
- continue
-
- case '-':
- if !scanOnly {
- cc.addRange(ch, ch)
- }
- continue
-
- default:
- p.moveLeft()
- var err error
- ch, err = p.scanCharEscape() // non-literal character
- if err != nil {
- return nil, err
- }
- fTranslatedChar = true
- break // this break will only break out of the switch
- }
- } else if ch == '[' {
- // This is code for Posix style properties - [:Ll:] or [:IsTibetan:].
- // It currently doesn't do anything other than skip the whole thing!
- if p.charsRight() > 0 && p.rightChar(0) == ':' && !inRange {
- savePos := p.textpos()
-
- p.moveRight(1)
- negate := false
- if p.charsRight() > 1 && p.rightChar(0) == '^' {
- negate = true
- p.moveRight(1)
- }
-
- nm := p.scanCapname() // snag the name
- if !scanOnly && p.useRE2() {
- // look up the name since these are valid for RE2
- // add the group based on the name
- if ok := cc.addNamedASCII(nm, negate); !ok {
- return nil, p.getErr(ErrInvalidCharRange)
- }
- }
- if p.charsRight() < 2 || p.moveRightGetChar() != ':' || p.moveRightGetChar() != ']' {
- p.textto(savePos)
- } else if p.useRE2() {
- // move on
- continue
- }
- }
- }
-
- if inRange {
- inRange = false
- if !scanOnly {
- if ch == '[' && !fTranslatedChar && !firstChar {
- // We thought we were in a range, but we're actually starting a subtraction.
- // In that case, we'll add chPrev to our char class, skip the opening [, and
- // scan the new character class recursively.
- cc.addChar(chPrev)
- sub, err := p.scanCharSet(caseInsensitive, false)
- if err != nil {
- return nil, err
- }
- cc.addSubtraction(sub)
-
- if p.charsRight() > 0 && p.rightChar(0) != ']' {
- return nil, p.getErr(ErrSubtractionMustBeLast)
- }
- } else {
- // a regular range, like a-z
- if chPrev > ch {
- return nil, p.getErr(ErrReversedCharRange)
- }
- cc.addRange(chPrev, ch)
- }
- }
- } else if p.charsRight() >= 2 && p.rightChar(0) == '-' && p.rightChar(1) != ']' {
- // this could be the start of a range
- chPrev = ch
- inRange = true
- p.moveRight(1)
- } else if p.charsRight() >= 1 && ch == '-' && !fTranslatedChar && p.rightChar(0) == '[' && !firstChar {
- // we aren't in a range, and now there is a subtraction. Usually this happens
- // only when a subtraction follows a range, like [a-z-[b]]
- if !scanOnly {
- p.moveRight(1)
- sub, err := p.scanCharSet(caseInsensitive, false)
- if err != nil {
- return nil, err
- }
- cc.addSubtraction(sub)
-
- if p.charsRight() > 0 && p.rightChar(0) != ']' {
- return nil, p.getErr(ErrSubtractionMustBeLast)
- }
- } else {
- p.moveRight(1)
- p.scanCharSet(caseInsensitive, true)
- }
- } else {
- if !scanOnly {
- cc.addRange(ch, ch)
- }
- }
- }
-
- if !closed {
- return nil, p.getErr(ErrUnterminatedBracket)
- }
-
- if !scanOnly && caseInsensitive {
- cc.addLowercase()
- }
-
- return cc, nil
-}
-
-// Scans any number of decimal digits (pegs value at 2^31-1 if too large)
-func (p *parser) scanDecimal() (int, error) {
- i := 0
- var d int
-
- for p.charsRight() > 0 {
- d = int(p.rightChar(0) - '0')
- if d < 0 || d > 9 {
- break
- }
- p.moveRight(1)
-
- if i > maxValueDiv10 || (i == maxValueDiv10 && d > maxValueMod10) {
- return 0, p.getErr(ErrCaptureGroupOutOfRange)
- }
-
- i *= 10
- i += d
- }
-
- return int(i), nil
-}
-
-// Returns true for options allowed only at the top level
-func isOnlyTopOption(option RegexOptions) bool {
- return option == RightToLeft || option == ECMAScript || option == RE2
-}
-
-// Scans cimsx-cimsx option string, stops at the first unrecognized char.
-func (p *parser) scanOptions() {
-
- for off := false; p.charsRight() > 0; p.moveRight(1) {
- ch := p.rightChar(0)
-
- if ch == '-' {
- off = true
- } else if ch == '+' {
- off = false
- } else {
- option := optionFromCode(ch)
- if option == 0 || isOnlyTopOption(option) {
- return
- }
-
- if off {
- p.options &= ^option
- } else {
- p.options |= option
- }
- }
- }
-}
-
-// Scans \ code for escape codes that map to single unicode chars.
-func (p *parser) scanCharEscape() (r rune, err error) {
-
- ch := p.moveRightGetChar()
-
- if ch >= '0' && ch <= '7' {
- p.moveLeft()
- return p.scanOctal(), nil
- }
-
- pos := p.textpos()
-
- switch ch {
- case 'x':
- // support for \x{HEX} syntax from Perl and PCRE
- if p.charsRight() > 0 && p.rightChar(0) == '{' {
- if p.useOptionE() {
- return ch, nil
- }
- p.moveRight(1)
- return p.scanHexUntilBrace()
- } else {
- r, err = p.scanHex(2)
- }
- case 'u':
- r, err = p.scanHex(4)
- case 'a':
- return '\u0007', nil
- case 'b':
- return '\b', nil
- case 'e':
- return '\u001B', nil
- case 'f':
- return '\f', nil
- case 'n':
- return '\n', nil
- case 'r':
- return '\r', nil
- case 't':
- return '\t', nil
- case 'v':
- return '\u000B', nil
- case 'c':
- r, err = p.scanControl()
- default:
- if !p.useOptionE() && IsWordChar(ch) {
- return 0, p.getErr(ErrUnrecognizedEscape, string(ch))
- }
- return ch, nil
- }
- if err != nil && p.useOptionE() {
- p.textto(pos)
- return ch, nil
- }
- return
-}
-
-// Grabs and converts an ascii control character
-func (p *parser) scanControl() (rune, error) {
- if p.charsRight() <= 0 {
- return 0, p.getErr(ErrMissingControl)
- }
-
- ch := p.moveRightGetChar()
-
- // \ca interpreted as \cA
-
- if ch >= 'a' && ch <= 'z' {
- ch = (ch - ('a' - 'A'))
- }
- ch = (ch - '@')
- if ch >= 0 && ch < ' ' {
- return ch, nil
- }
-
- return 0, p.getErr(ErrUnrecognizedControl)
-
-}
-
-// Scan hex digits until we hit a closing brace.
-// Non-hex digits, hex value too large for UTF-8, or running out of chars are errors
-func (p *parser) scanHexUntilBrace() (rune, error) {
- // PCRE spec reads like unlimited hex digits are allowed, but unicode has a limit
- // so we can enforce that
- i := 0
- hasContent := false
-
- for p.charsRight() > 0 {
- ch := p.moveRightGetChar()
- if ch == '}' {
- // hit our close brace, we're done here
- // prevent \x{}
- if !hasContent {
- return 0, p.getErr(ErrTooFewHex)
- }
- return rune(i), nil
- }
- hasContent = true
- // no brace needs to be hex digit
- d := hexDigit(ch)
- if d < 0 {
- return 0, p.getErr(ErrMissingBrace)
- }
-
- i *= 0x10
- i += d
-
- if i > unicode.MaxRune {
- return 0, p.getErr(ErrInvalidHex)
- }
- }
-
- // we only make it here if we run out of digits without finding the brace
- return 0, p.getErr(ErrMissingBrace)
-}
-
-// Scans exactly c hex digits (c=2 for \xFF, c=4 for \uFFFF)
-func (p *parser) scanHex(c int) (rune, error) {
-
- i := 0
-
- if p.charsRight() >= c {
- for c > 0 {
- d := hexDigit(p.moveRightGetChar())
- if d < 0 {
- break
- }
- i *= 0x10
- i += d
- c--
- }
- }
-
- if c > 0 {
- return 0, p.getErr(ErrTooFewHex)
- }
-
- return rune(i), nil
-}
-
-// Returns n <= 0xF for a hex digit.
-func hexDigit(ch rune) int {
-
- if d := uint(ch - '0'); d <= 9 {
- return int(d)
- }
-
- if d := uint(ch - 'a'); d <= 5 {
- return int(d + 0xa)
- }
-
- if d := uint(ch - 'A'); d <= 5 {
- return int(d + 0xa)
- }
-
- return -1
-}
-
-// Scans up to three octal digits (stops before exceeding 0377).
-func (p *parser) scanOctal() rune {
- // Consume octal chars only up to 3 digits and value 0377
-
- c := 3
-
- if c > p.charsRight() {
- c = p.charsRight()
- }
-
- //we know the first char is good because the caller had to check
- i := 0
- d := int(p.rightChar(0) - '0')
- for c > 0 && d <= 7 && d >= 0 {
- if i >= 0x20 && p.useOptionE() {
- break
- }
- i *= 8
- i += d
- c--
-
- p.moveRight(1)
- if !p.rightMost() {
- d = int(p.rightChar(0) - '0')
- }
- }
-
- // Octal codes only go up to 255. Any larger and the behavior that Perl follows
- // is simply to truncate the high bits.
- i &= 0xFF
-
- return rune(i)
-}
-
-// Returns the current parsing position.
-func (p *parser) textpos() int {
- return p.currentPos
-}
-
-// Zaps to a specific parsing position.
-func (p *parser) textto(pos int) {
- p.currentPos = pos
-}
-
-// Returns the char at the right of the current parsing position and advances to the right.
-func (p *parser) moveRightGetChar() rune {
- ch := p.pattern[p.currentPos]
- p.currentPos++
- return ch
-}
-
-// Moves the current position to the right.
-func (p *parser) moveRight(i int) {
- // default would be 1
- p.currentPos += i
-}
-
-// Moves the current parsing position one to the left.
-func (p *parser) moveLeft() {
- p.currentPos--
-}
-
-// Returns the char left of the current parsing position.
-func (p *parser) charAt(i int) rune {
- return p.pattern[i]
-}
-
-// Returns the char i chars right of the current parsing position.
-func (p *parser) rightChar(i int) rune {
- // default would be 0
- return p.pattern[p.currentPos+i]
-}
-
-// Number of characters to the right of the current parsing position.
-func (p *parser) charsRight() int {
- return len(p.pattern) - p.currentPos
-}
-
-func (p *parser) rightMost() bool {
- return p.currentPos == len(p.pattern)
-}
-
-// Looks up the slot number for a given name
-func (p *parser) captureSlotFromName(capname string) int {
- return p.capnames[capname]
-}
-
-// True if the capture slot was noted
-func (p *parser) isCaptureSlot(i int) bool {
- if p.caps != nil {
- _, ok := p.caps[i]
- return ok
- }
-
- return (i >= 0 && i < p.capsize)
-}
-
-// Looks up the slot number for a given name
-func (p *parser) isCaptureName(capname string) bool {
- if p.capnames == nil {
- return false
- }
-
- _, ok := p.capnames[capname]
- return ok
-}
-
-// option shortcuts
-
-// True if N option disabling '(' autocapture is on.
-func (p *parser) useOptionN() bool {
- return (p.options & ExplicitCapture) != 0
-}
-
-// True if I option enabling case-insensitivity is on.
-func (p *parser) useOptionI() bool {
- return (p.options & IgnoreCase) != 0
-}
-
-// True if M option altering meaning of $ and ^ is on.
-func (p *parser) useOptionM() bool {
- return (p.options & Multiline) != 0
-}
-
-// True if S option altering meaning of . is on.
-func (p *parser) useOptionS() bool {
- return (p.options & Singleline) != 0
-}
-
-// True if X option enabling whitespace/comment mode is on.
-func (p *parser) useOptionX() bool {
- return (p.options & IgnorePatternWhitespace) != 0
-}
-
-// True if E option enabling ECMAScript behavior on.
-func (p *parser) useOptionE() bool {
- return (p.options & ECMAScript) != 0
-}
-
-// true to use RE2 compatibility parsing behavior.
-func (p *parser) useRE2() bool {
- return (p.options & RE2) != 0
-}
-
-// True if options stack is empty.
-func (p *parser) emptyOptionsStack() bool {
- return len(p.optionsStack) == 0
-}
-
-// Finish the current quantifiable (when a quantifier is not found or is not possible)
-func (p *parser) addConcatenate() {
- // The first (| inside a Testgroup group goes directly to the group
- p.concatenation.addChild(p.unit)
- p.unit = nil
-}
-
-// Finish the current quantifiable (when a quantifier is found)
-func (p *parser) addConcatenate3(lazy bool, min, max int) {
- p.concatenation.addChild(p.unit.makeQuantifier(lazy, min, max))
- p.unit = nil
-}
-
-// Sets the current unit to a single char node
-func (p *parser) addUnitOne(ch rune) {
- if p.useOptionI() {
- ch = unicode.ToLower(ch)
- }
-
- p.unit = newRegexNodeCh(ntOne, p.options, ch)
-}
-
-// Sets the current unit to a single inverse-char node
-func (p *parser) addUnitNotone(ch rune) {
- if p.useOptionI() {
- ch = unicode.ToLower(ch)
- }
-
- p.unit = newRegexNodeCh(ntNotone, p.options, ch)
-}
-
-// Sets the current unit to a single set node
-func (p *parser) addUnitSet(set *CharSet) {
- p.unit = newRegexNodeSet(ntSet, p.options, set)
-}
-
-// Sets the current unit to a subtree
-func (p *parser) addUnitNode(node *regexNode) {
- p.unit = node
-}
-
-// Sets the current unit to an assertion of the specified type
-func (p *parser) addUnitType(t nodeType) {
- p.unit = newRegexNode(t, p.options)
-}
-
-// Finish the current group (in response to a ')' or end)
-func (p *parser) addGroup() error {
- if p.group.t == ntTestgroup || p.group.t == ntTestref {
- p.group.addChild(p.concatenation.reverseLeft())
- if (p.group.t == ntTestref && len(p.group.children) > 2) || len(p.group.children) > 3 {
- return p.getErr(ErrTooManyAlternates)
- }
- } else {
- p.alternation.addChild(p.concatenation.reverseLeft())
- p.group.addChild(p.alternation)
- }
-
- p.unit = p.group
- return nil
-}
-
-// Pops the option stack, but keeps the current options unchanged.
-func (p *parser) popKeepOptions() {
- lastIdx := len(p.optionsStack) - 1
- p.optionsStack = p.optionsStack[:lastIdx]
-}
-
-// Recalls options from the stack.
-func (p *parser) popOptions() {
- lastIdx := len(p.optionsStack) - 1
- // get the last item on the stack and then remove it by reslicing
- p.options = p.optionsStack[lastIdx]
- p.optionsStack = p.optionsStack[:lastIdx]
-}
-
-// Saves options on a stack.
-func (p *parser) pushOptions() {
- p.optionsStack = append(p.optionsStack, p.options)
-}
-
-// Add a string to the last concatenate.
-func (p *parser) addToConcatenate(pos, cch int, isReplacement bool) {
- var node *regexNode
-
- if cch == 0 {
- return
- }
-
- if cch > 1 {
- str := p.pattern[pos : pos+cch]
-
- if p.useOptionI() && !isReplacement {
- // We do the ToLower character by character for consistency. With surrogate chars, doing
- // a ToLower on the entire string could actually change the surrogate pair. This is more correct
- // linguistically, but since Regex doesn't support surrogates, it's more important to be
- // consistent.
- for i := 0; i < len(str); i++ {
- str[i] = unicode.ToLower(str[i])
- }
- }
-
- node = newRegexNodeStr(ntMulti, p.options, str)
- } else {
- ch := p.charAt(pos)
-
- if p.useOptionI() && !isReplacement {
- ch = unicode.ToLower(ch)
- }
-
- node = newRegexNodeCh(ntOne, p.options, ch)
- }
-
- p.concatenation.addChild(node)
-}
-
-// Push the parser state (in response to an open paren)
-func (p *parser) pushGroup() {
- p.group.next = p.stack
- p.alternation.next = p.group
- p.concatenation.next = p.alternation
- p.stack = p.concatenation
-}
-
-// Remember the pushed state (in response to a ')')
-func (p *parser) popGroup() error {
- p.concatenation = p.stack
- p.alternation = p.concatenation.next
- p.group = p.alternation.next
- p.stack = p.group.next
-
- // The first () inside a Testgroup group goes directly to the group
- if p.group.t == ntTestgroup && len(p.group.children) == 0 {
- if p.unit == nil {
- return p.getErr(ErrConditionalExpression)
- }
-
- p.group.addChild(p.unit)
- p.unit = nil
- }
- return nil
-}
-
-// True if the group stack is empty.
-func (p *parser) emptyStack() bool {
- return p.stack == nil
-}
-
-// Start a new round for the parser state (in response to an open paren or string start)
-func (p *parser) startGroup(openGroup *regexNode) {
- p.group = openGroup
- p.alternation = newRegexNode(ntAlternate, p.options)
- p.concatenation = newRegexNode(ntConcatenate, p.options)
-}
-
-// Finish the current concatenation (in response to a |)
-func (p *parser) addAlternate() {
- // The | parts inside a Testgroup group go directly to the group
-
- if p.group.t == ntTestgroup || p.group.t == ntTestref {
- p.group.addChild(p.concatenation.reverseLeft())
- } else {
- p.alternation.addChild(p.concatenation.reverseLeft())
- }
-
- p.concatenation = newRegexNode(ntConcatenate, p.options)
-}
-
-// For categorizing ascii characters.
-
-const (
- Q byte = 5 // quantifier
- S = 4 // ordinary stopper
- Z = 3 // ScanBlank stopper
- X = 2 // whitespace
- E = 1 // should be escaped
-)
-
-var _category = []byte{
- //01 2 3 4 5 6 7 8 9 A B C D E F 0 1 2 3 4 5 6 7 8 9 A B C D E F
- 0, 0, 0, 0, 0, 0, 0, 0, 0, X, X, X, X, X, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
- // ! " # $ % & ' ( ) * + , - . / 0 1 2 3 4 5 6 7 8 9 : ; < = > ?
- X, 0, 0, Z, S, 0, 0, 0, S, S, Q, Q, 0, 0, S, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, Q,
- //@A B C D E F G H I J K L M N O P Q R S T U V W X Y Z [ \ ] ^ _
- 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, S, S, 0, S, 0,
- //'a b c d e f g h i j k l m n o p q r s t u v w x y z { | } ~
- 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, Q, S, 0, 0, 0,
-}
-
-func isSpace(ch rune) bool {
- return (ch <= ' ' && _category[ch] == X)
-}
-
-// Returns true for those characters that terminate a string of ordinary chars.
-func isSpecial(ch rune) bool {
- return (ch <= '|' && _category[ch] >= S)
-}
-
-// Returns true for those characters that terminate a string of ordinary chars.
-func isStopperX(ch rune) bool {
- return (ch <= '|' && _category[ch] >= X)
-}
-
-// Returns true for those characters that begin a quantifier.
-func isQuantifier(ch rune) bool {
- return (ch <= '{' && _category[ch] >= Q)
-}
-
-func (p *parser) isTrueQuantifier() bool {
- nChars := p.charsRight()
- if nChars == 0 {
- return false
- }
-
- startpos := p.textpos()
- ch := p.charAt(startpos)
- if ch != '{' {
- return ch <= '{' && _category[ch] >= Q
- }
-
- //UGLY: this is ugly -- the original code was ugly too
- pos := startpos
- for {
- nChars--
- if nChars <= 0 {
- break
- }
- pos++
- ch = p.charAt(pos)
- if ch < '0' || ch > '9' {
- break
- }
- }
-
- if nChars == 0 || pos-startpos == 1 {
- return false
- }
- if ch == '}' {
- return true
- }
- if ch != ',' {
- return false
- }
- for {
- nChars--
- if nChars <= 0 {
- break
- }
- pos++
- ch = p.charAt(pos)
- if ch < '0' || ch > '9' {
- break
- }
- }
-
- return nChars > 0 && ch == '}'
-}
diff --git a/vendor/github.com/dlclark/regexp2/syntax/prefix.go b/vendor/github.com/dlclark/regexp2/syntax/prefix.go
deleted file mode 100644
index 011ef0b..0000000
--- a/vendor/github.com/dlclark/regexp2/syntax/prefix.go
+++ /dev/null
@@ -1,896 +0,0 @@
-package syntax
-
-import (
- "bytes"
- "fmt"
- "strconv"
- "unicode"
- "unicode/utf8"
-)
-
-type Prefix struct {
- PrefixStr []rune
- PrefixSet CharSet
- CaseInsensitive bool
-}
-
-// It takes a RegexTree and computes the set of chars that can start it.
-func getFirstCharsPrefix(tree *RegexTree) *Prefix {
- s := regexFcd{
- fcStack: make([]regexFc, 32),
- intStack: make([]int, 32),
- }
- fc := s.regexFCFromRegexTree(tree)
-
- if fc == nil || fc.nullable || fc.cc.IsEmpty() {
- return nil
- }
- fcSet := fc.getFirstChars()
- return &Prefix{PrefixSet: fcSet, CaseInsensitive: fc.caseInsensitive}
-}
-
-type regexFcd struct {
- intStack []int
- intDepth int
- fcStack []regexFc
- fcDepth int
- skipAllChildren bool // don't process any more children at the current level
- skipchild bool // don't process the current child.
- failed bool
-}
-
-/*
- * The main FC computation. It does a shortcutted depth-first walk
- * through the tree and calls CalculateFC to emits code before
- * and after each child of an interior node, and at each leaf.
- */
-func (s *regexFcd) regexFCFromRegexTree(tree *RegexTree) *regexFc {
- curNode := tree.root
- curChild := 0
-
- for {
- if len(curNode.children) == 0 {
- // This is a leaf node
- s.calculateFC(curNode.t, curNode, 0)
- } else if curChild < len(curNode.children) && !s.skipAllChildren {
- // This is an interior node, and we have more children to analyze
- s.calculateFC(curNode.t|beforeChild, curNode, curChild)
-
- if !s.skipchild {
- curNode = curNode.children[curChild]
- // this stack is how we get a depth first walk of the tree.
- s.pushInt(curChild)
- curChild = 0
- } else {
- curChild++
- s.skipchild = false
- }
- continue
- }
-
- // This is an interior node where we've finished analyzing all the children, or
- // the end of a leaf node.
- s.skipAllChildren = false
-
- if s.intIsEmpty() {
- break
- }
-
- curChild = s.popInt()
- curNode = curNode.next
-
- s.calculateFC(curNode.t|afterChild, curNode, curChild)
- if s.failed {
- return nil
- }
-
- curChild++
- }
-
- if s.fcIsEmpty() {
- return nil
- }
-
- return s.popFC()
-}
-
-// To avoid recursion, we use a simple integer stack.
-// This is the push.
-func (s *regexFcd) pushInt(I int) {
- if s.intDepth >= len(s.intStack) {
- expanded := make([]int, s.intDepth*2)
- copy(expanded, s.intStack)
- s.intStack = expanded
- }
-
- s.intStack[s.intDepth] = I
- s.intDepth++
-}
-
-// True if the stack is empty.
-func (s *regexFcd) intIsEmpty() bool {
- return s.intDepth == 0
-}
-
-// This is the pop.
-func (s *regexFcd) popInt() int {
- s.intDepth--
- return s.intStack[s.intDepth]
-}
-
-// We also use a stack of RegexFC objects.
-// This is the push.
-func (s *regexFcd) pushFC(fc regexFc) {
- if s.fcDepth >= len(s.fcStack) {
- expanded := make([]regexFc, s.fcDepth*2)
- copy(expanded, s.fcStack)
- s.fcStack = expanded
- }
-
- s.fcStack[s.fcDepth] = fc
- s.fcDepth++
-}
-
-// True if the stack is empty.
-func (s *regexFcd) fcIsEmpty() bool {
- return s.fcDepth == 0
-}
-
-// This is the pop.
-func (s *regexFcd) popFC() *regexFc {
- s.fcDepth--
- return &s.fcStack[s.fcDepth]
-}
-
-// This is the top.
-func (s *regexFcd) topFC() *regexFc {
- return &s.fcStack[s.fcDepth-1]
-}
-
-// Called in Beforechild to prevent further processing of the current child
-func (s *regexFcd) skipChild() {
- s.skipchild = true
-}
-
-// FC computation and shortcut cases for each node type
-func (s *regexFcd) calculateFC(nt nodeType, node *regexNode, CurIndex int) {
- //fmt.Printf("NodeType: %v, CurIndex: %v, Desc: %v\n", nt, CurIndex, node.description())
- ci := false
- rtl := false
-
- if nt <= ntRef {
- if (node.options & IgnoreCase) != 0 {
- ci = true
- }
- if (node.options & RightToLeft) != 0 {
- rtl = true
- }
- }
-
- switch nt {
- case ntConcatenate | beforeChild, ntAlternate | beforeChild, ntTestref | beforeChild, ntLoop | beforeChild, ntLazyloop | beforeChild:
- break
-
- case ntTestgroup | beforeChild:
- if CurIndex == 0 {
- s.skipChild()
- }
- break
-
- case ntEmpty:
- s.pushFC(regexFc{nullable: true})
- break
-
- case ntConcatenate | afterChild:
- if CurIndex != 0 {
- child := s.popFC()
- cumul := s.topFC()
-
- s.failed = !cumul.addFC(*child, true)
- }
-
- fc := s.topFC()
- if !fc.nullable {
- s.skipAllChildren = true
- }
- break
-
- case ntTestgroup | afterChild:
- if CurIndex > 1 {
- child := s.popFC()
- cumul := s.topFC()
-
- s.failed = !cumul.addFC(*child, false)
- }
- break
-
- case ntAlternate | afterChild, ntTestref | afterChild:
- if CurIndex != 0 {
- child := s.popFC()
- cumul := s.topFC()
-
- s.failed = !cumul.addFC(*child, false)
- }
- break
-
- case ntLoop | afterChild, ntLazyloop | afterChild:
- if node.m == 0 {
- fc := s.topFC()
- fc.nullable = true
- }
- break
-
- case ntGroup | beforeChild, ntGroup | afterChild, ntCapture | beforeChild, ntCapture | afterChild, ntGreedy | beforeChild, ntGreedy | afterChild:
- break
-
- case ntRequire | beforeChild, ntPrevent | beforeChild:
- s.skipChild()
- s.pushFC(regexFc{nullable: true})
- break
-
- case ntRequire | afterChild, ntPrevent | afterChild:
- break
-
- case ntOne, ntNotone:
- s.pushFC(newRegexFc(node.ch, nt == ntNotone, false, ci))
- break
-
- case ntOneloop, ntOnelazy:
- s.pushFC(newRegexFc(node.ch, false, node.m == 0, ci))
- break
-
- case ntNotoneloop, ntNotonelazy:
- s.pushFC(newRegexFc(node.ch, true, node.m == 0, ci))
- break
-
- case ntMulti:
- if len(node.str) == 0 {
- s.pushFC(regexFc{nullable: true})
- } else if !rtl {
- s.pushFC(newRegexFc(node.str[0], false, false, ci))
- } else {
- s.pushFC(newRegexFc(node.str[len(node.str)-1], false, false, ci))
- }
- break
-
- case ntSet:
- s.pushFC(regexFc{cc: node.set.Copy(), nullable: false, caseInsensitive: ci})
- break
-
- case ntSetloop, ntSetlazy:
- s.pushFC(regexFc{cc: node.set.Copy(), nullable: node.m == 0, caseInsensitive: ci})
- break
-
- case ntRef:
- s.pushFC(regexFc{cc: *AnyClass(), nullable: true, caseInsensitive: false})
- break
-
- case ntNothing, ntBol, ntEol, ntBoundary, ntNonboundary, ntECMABoundary, ntNonECMABoundary, ntBeginning, ntStart, ntEndZ, ntEnd:
- s.pushFC(regexFc{nullable: true})
- break
-
- default:
- panic(fmt.Sprintf("unexpected op code: %v", nt))
- }
-}
-
-type regexFc struct {
- cc CharSet
- nullable bool
- caseInsensitive bool
-}
-
-func newRegexFc(ch rune, not, nullable, caseInsensitive bool) regexFc {
- r := regexFc{
- caseInsensitive: caseInsensitive,
- nullable: nullable,
- }
- if not {
- if ch > 0 {
- r.cc.addRange('\x00', ch-1)
- }
- if ch < 0xFFFF {
- r.cc.addRange(ch+1, utf8.MaxRune)
- }
- } else {
- r.cc.addRange(ch, ch)
- }
- return r
-}
-
-func (r *regexFc) getFirstChars() CharSet {
- if r.caseInsensitive {
- r.cc.addLowercase()
- }
-
- return r.cc
-}
-
-func (r *regexFc) addFC(fc regexFc, concatenate bool) bool {
- if !r.cc.IsMergeable() || !fc.cc.IsMergeable() {
- return false
- }
-
- if concatenate {
- if !r.nullable {
- return true
- }
-
- if !fc.nullable {
- r.nullable = false
- }
- } else {
- if fc.nullable {
- r.nullable = true
- }
- }
-
- r.caseInsensitive = r.caseInsensitive || fc.caseInsensitive
- r.cc.addSet(fc.cc)
-
- return true
-}
-
-// This is a related computation: it takes a RegexTree and computes the
-// leading substring if it sees one. It's quite trivial and gives up easily.
-func getPrefix(tree *RegexTree) *Prefix {
- var concatNode *regexNode
- nextChild := 0
-
- curNode := tree.root
-
- for {
- switch curNode.t {
- case ntConcatenate:
- if len(curNode.children) > 0 {
- concatNode = curNode
- nextChild = 0
- }
-
- case ntGreedy, ntCapture:
- curNode = curNode.children[0]
- concatNode = nil
- continue
-
- case ntOneloop, ntOnelazy:
- if curNode.m > 0 {
- return &Prefix{
- PrefixStr: repeat(curNode.ch, curNode.m),
- CaseInsensitive: (curNode.options & IgnoreCase) != 0,
- }
- }
- return nil
-
- case ntOne:
- return &Prefix{
- PrefixStr: []rune{curNode.ch},
- CaseInsensitive: (curNode.options & IgnoreCase) != 0,
- }
-
- case ntMulti:
- return &Prefix{
- PrefixStr: curNode.str,
- CaseInsensitive: (curNode.options & IgnoreCase) != 0,
- }
-
- case ntBol, ntEol, ntBoundary, ntECMABoundary, ntBeginning, ntStart,
- ntEndZ, ntEnd, ntEmpty, ntRequire, ntPrevent:
-
- default:
- return nil
- }
-
- if concatNode == nil || nextChild >= len(concatNode.children) {
- return nil
- }
-
- curNode = concatNode.children[nextChild]
- nextChild++
- }
-}
-
-// repeat the rune r, c times... up to the max of MaxPrefixSize
-func repeat(r rune, c int) []rune {
- if c > MaxPrefixSize {
- c = MaxPrefixSize
- }
-
- ret := make([]rune, c)
-
- // binary growth using copy for speed
- ret[0] = r
- bp := 1
- for bp < len(ret) {
- copy(ret[bp:], ret[:bp])
- bp *= 2
- }
-
- return ret
-}
-
-// BmPrefix precomputes the Boyer-Moore
-// tables for fast string scanning. These tables allow
-// you to scan for the first occurrence of a string within
-// a large body of text without examining every character.
-// The performance of the heuristic depends on the actual
-// string and the text being searched, but usually, the longer
-// the string that is being searched for, the fewer characters
-// need to be examined.
-type BmPrefix struct {
- positive []int
- negativeASCII []int
- negativeUnicode [][]int
- pattern []rune
- lowASCII rune
- highASCII rune
- rightToLeft bool
- caseInsensitive bool
-}
-
-func newBmPrefix(pattern []rune, caseInsensitive, rightToLeft bool) *BmPrefix {
-
- b := &BmPrefix{
- rightToLeft: rightToLeft,
- caseInsensitive: caseInsensitive,
- pattern: pattern,
- }
-
- if caseInsensitive {
- for i := 0; i < len(b.pattern); i++ {
- // We do the ToLower character by character for consistency. With surrogate chars, doing
- // a ToLower on the entire string could actually change the surrogate pair. This is more correct
- // linguistically, but since Regex doesn't support surrogates, it's more important to be
- // consistent.
-
- b.pattern[i] = unicode.ToLower(b.pattern[i])
- }
- }
-
- var beforefirst, last, bump int
- var scan, match int
-
- if !rightToLeft {
- beforefirst = -1
- last = len(b.pattern) - 1
- bump = 1
- } else {
- beforefirst = len(b.pattern)
- last = 0
- bump = -1
- }
-
- // PART I - the good-suffix shift table
- //
- // compute the positive requirement:
- // if char "i" is the first one from the right that doesn't match,
- // then we know the matcher can advance by _positive[i].
- //
- // This algorithm is a simplified variant of the standard
- // Boyer-Moore good suffix calculation.
-
- b.positive = make([]int, len(b.pattern))
-
- examine := last
- ch := b.pattern[examine]
- b.positive[examine] = bump
- examine -= bump
-
-Outerloop:
- for {
- // find an internal char (examine) that matches the tail
-
- for {
- if examine == beforefirst {
- break Outerloop
- }
- if b.pattern[examine] == ch {
- break
- }
- examine -= bump
- }
-
- match = last
- scan = examine
-
- // find the length of the match
- for {
- if scan == beforefirst || b.pattern[match] != b.pattern[scan] {
- // at the end of the match, note the difference in _positive
- // this is not the length of the match, but the distance from the internal match
- // to the tail suffix.
- if b.positive[match] == 0 {
- b.positive[match] = match - scan
- }
-
- // System.Diagnostics.Debug.WriteLine("Set positive[" + match + "] to " + (match - scan));
-
- break
- }
-
- scan -= bump
- match -= bump
- }
-
- examine -= bump
- }
-
- match = last - bump
-
- // scan for the chars for which there are no shifts that yield a different candidate
-
- // The inside of the if statement used to say
- // "_positive[match] = last - beforefirst;"
- // This is slightly less aggressive in how much we skip, but at worst it
- // should mean a little more work rather than skipping a potential match.
- for match != beforefirst {
- if b.positive[match] == 0 {
- b.positive[match] = bump
- }
-
- match -= bump
- }
-
- // PART II - the bad-character shift table
- //
- // compute the negative requirement:
- // if char "ch" is the reject character when testing position "i",
- // we can slide up by _negative[ch];
- // (_negative[ch] = str.Length - 1 - str.LastIndexOf(ch))
- //
- // the lookup table is divided into ASCII and Unicode portions;
- // only those parts of the Unicode 16-bit code set that actually
- // appear in the string are in the table. (Maximum size with
- // Unicode is 65K; ASCII only case is 512 bytes.)
-
- b.negativeASCII = make([]int, 128)
-
- for i := 0; i < len(b.negativeASCII); i++ {
- b.negativeASCII[i] = last - beforefirst
- }
-
- b.lowASCII = 127
- b.highASCII = 0
-
- for examine = last; examine != beforefirst; examine -= bump {
- ch = b.pattern[examine]
-
- switch {
- case ch < 128:
- if b.lowASCII > ch {
- b.lowASCII = ch
- }
-
- if b.highASCII < ch {
- b.highASCII = ch
- }
-
- if b.negativeASCII[ch] == last-beforefirst {
- b.negativeASCII[ch] = last - examine
- }
- case ch <= 0xffff:
- i, j := ch>>8, ch&0xFF
-
- if b.negativeUnicode == nil {
- b.negativeUnicode = make([][]int, 256)
- }
-
- if b.negativeUnicode[i] == nil {
- newarray := make([]int, 256)
-
- for k := 0; k < len(newarray); k++ {
- newarray[k] = last - beforefirst
- }
-
- if i == 0 {
- copy(newarray, b.negativeASCII)
- //TODO: this line needed?
- b.negativeASCII = newarray
- }
-
- b.negativeUnicode[i] = newarray
- }
-
- if b.negativeUnicode[i][j] == last-beforefirst {
- b.negativeUnicode[i][j] = last - examine
- }
- default:
- // we can't do the filter because this algo doesn't support
- // unicode chars >0xffff
- return nil
- }
- }
-
- return b
-}
-
-func (b *BmPrefix) String() string {
- return string(b.pattern)
-}
-
-// Dump returns the contents of the filter as a human readable string
-func (b *BmPrefix) Dump(indent string) string {
- buf := &bytes.Buffer{}
-
- fmt.Fprintf(buf, "%sBM Pattern: %s\n%sPositive: ", indent, string(b.pattern), indent)
- for i := 0; i < len(b.positive); i++ {
- buf.WriteString(strconv.Itoa(b.positive[i]))
- buf.WriteRune(' ')
- }
- buf.WriteRune('\n')
-
- if b.negativeASCII != nil {
- buf.WriteString(indent)
- buf.WriteString("Negative table\n")
- for i := 0; i < len(b.negativeASCII); i++ {
- if b.negativeASCII[i] != len(b.pattern) {
- fmt.Fprintf(buf, "%s %s %s\n", indent, Escape(string(rune(i))), strconv.Itoa(b.negativeASCII[i]))
- }
- }
- }
-
- return buf.String()
-}
-
-// Scan uses the Boyer-Moore algorithm to find the first occurrence
-// of the specified string within text, beginning at index, and
-// constrained within beglimit and endlimit.
-//
-// The direction and case-sensitivity of the match is determined
-// by the arguments to the RegexBoyerMoore constructor.
-func (b *BmPrefix) Scan(text []rune, index, beglimit, endlimit int) int {
- var (
- defadv, test, test2 int
- match, startmatch, endmatch int
- bump, advance int
- chTest rune
- unicodeLookup []int
- )
-
- if !b.rightToLeft {
- defadv = len(b.pattern)
- startmatch = len(b.pattern) - 1
- endmatch = 0
- test = index + defadv - 1
- bump = 1
- } else {
- defadv = -len(b.pattern)
- startmatch = 0
- endmatch = -defadv - 1
- test = index + defadv
- bump = -1
- }
-
- chMatch := b.pattern[startmatch]
-
- for {
- if test >= endlimit || test < beglimit {
- return -1
- }
-
- chTest = text[test]
-
- if b.caseInsensitive {
- chTest = unicode.ToLower(chTest)
- }
-
- if chTest != chMatch {
- if chTest < 128 {
- advance = b.negativeASCII[chTest]
- } else if chTest < 0xffff && len(b.negativeUnicode) > 0 {
- unicodeLookup = b.negativeUnicode[chTest>>8]
- if len(unicodeLookup) > 0 {
- advance = unicodeLookup[chTest&0xFF]
- } else {
- advance = defadv
- }
- } else {
- advance = defadv
- }
-
- test += advance
- } else { // if (chTest == chMatch)
- test2 = test
- match = startmatch
-
- for {
- if match == endmatch {
- if b.rightToLeft {
- return test2 + 1
- } else {
- return test2
- }
- }
-
- match -= bump
- test2 -= bump
-
- chTest = text[test2]
-
- if b.caseInsensitive {
- chTest = unicode.ToLower(chTest)
- }
-
- if chTest != b.pattern[match] {
- advance = b.positive[match]
- if (chTest & 0xFF80) == 0 {
- test2 = (match - startmatch) + b.negativeASCII[chTest]
- } else if chTest < 0xffff && len(b.negativeUnicode) > 0 {
- unicodeLookup = b.negativeUnicode[chTest>>8]
- if len(unicodeLookup) > 0 {
- test2 = (match - startmatch) + unicodeLookup[chTest&0xFF]
- } else {
- test += advance
- break
- }
- } else {
- test += advance
- break
- }
-
- if b.rightToLeft {
- if test2 < advance {
- advance = test2
- }
- } else if test2 > advance {
- advance = test2
- }
-
- test += advance
- break
- }
- }
- }
- }
-}
-
-// When a regex is anchored, we can do a quick IsMatch test instead of a Scan
-func (b *BmPrefix) IsMatch(text []rune, index, beglimit, endlimit int) bool {
- if !b.rightToLeft {
- if index < beglimit || endlimit-index < len(b.pattern) {
- return false
- }
-
- return b.matchPattern(text, index)
- } else {
- if index > endlimit || index-beglimit < len(b.pattern) {
- return false
- }
-
- return b.matchPattern(text, index-len(b.pattern))
- }
-}
-
-func (b *BmPrefix) matchPattern(text []rune, index int) bool {
- if len(text)-index < len(b.pattern) {
- return false
- }
-
- if b.caseInsensitive {
- for i := 0; i < len(b.pattern); i++ {
- //Debug.Assert(textinfo.ToLower(_pattern[i]) == _pattern[i], "pattern should be converted to lower case in constructor!");
- if unicode.ToLower(text[index+i]) != b.pattern[i] {
- return false
- }
- }
- return true
- } else {
- for i := 0; i < len(b.pattern); i++ {
- if text[index+i] != b.pattern[i] {
- return false
- }
- }
- return true
- }
-}
-
-type AnchorLoc int16
-
-// where the regex can be pegged
-const (
- AnchorBeginning AnchorLoc = 0x0001
- AnchorBol = 0x0002
- AnchorStart = 0x0004
- AnchorEol = 0x0008
- AnchorEndZ = 0x0010
- AnchorEnd = 0x0020
- AnchorBoundary = 0x0040
- AnchorECMABoundary = 0x0080
-)
-
-func getAnchors(tree *RegexTree) AnchorLoc {
-
- var concatNode *regexNode
- nextChild, result := 0, AnchorLoc(0)
-
- curNode := tree.root
-
- for {
- switch curNode.t {
- case ntConcatenate:
- if len(curNode.children) > 0 {
- concatNode = curNode
- nextChild = 0
- }
-
- case ntGreedy, ntCapture:
- curNode = curNode.children[0]
- concatNode = nil
- continue
-
- case ntBol, ntEol, ntBoundary, ntECMABoundary, ntBeginning,
- ntStart, ntEndZ, ntEnd:
- return result | anchorFromType(curNode.t)
-
- case ntEmpty, ntRequire, ntPrevent:
-
- default:
- return result
- }
-
- if concatNode == nil || nextChild >= len(concatNode.children) {
- return result
- }
-
- curNode = concatNode.children[nextChild]
- nextChild++
- }
-}
-
-func anchorFromType(t nodeType) AnchorLoc {
- switch t {
- case ntBol:
- return AnchorBol
- case ntEol:
- return AnchorEol
- case ntBoundary:
- return AnchorBoundary
- case ntECMABoundary:
- return AnchorECMABoundary
- case ntBeginning:
- return AnchorBeginning
- case ntStart:
- return AnchorStart
- case ntEndZ:
- return AnchorEndZ
- case ntEnd:
- return AnchorEnd
- default:
- return 0
- }
-}
-
-// anchorDescription returns a human-readable description of the anchors
-func (anchors AnchorLoc) String() string {
- buf := &bytes.Buffer{}
-
- if 0 != (anchors & AnchorBeginning) {
- buf.WriteString(", Beginning")
- }
- if 0 != (anchors & AnchorStart) {
- buf.WriteString(", Start")
- }
- if 0 != (anchors & AnchorBol) {
- buf.WriteString(", Bol")
- }
- if 0 != (anchors & AnchorBoundary) {
- buf.WriteString(", Boundary")
- }
- if 0 != (anchors & AnchorECMABoundary) {
- buf.WriteString(", ECMABoundary")
- }
- if 0 != (anchors & AnchorEol) {
- buf.WriteString(", Eol")
- }
- if 0 != (anchors & AnchorEnd) {
- buf.WriteString(", End")
- }
- if 0 != (anchors & AnchorEndZ) {
- buf.WriteString(", EndZ")
- }
-
- // trim off comma
- if buf.Len() >= 2 {
- return buf.String()[2:]
- }
- return "None"
-}
diff --git a/vendor/github.com/dlclark/regexp2/syntax/replacerdata.go b/vendor/github.com/dlclark/regexp2/syntax/replacerdata.go
deleted file mode 100644
index bcf4d3f..0000000
--- a/vendor/github.com/dlclark/regexp2/syntax/replacerdata.go
+++ /dev/null
@@ -1,87 +0,0 @@
-package syntax
-
-import (
- "bytes"
- "errors"
-)
-
-type ReplacerData struct {
- Rep string
- Strings []string
- Rules []int
-}
-
-const (
- replaceSpecials = 4
- replaceLeftPortion = -1
- replaceRightPortion = -2
- replaceLastGroup = -3
- replaceWholeString = -4
-)
-
-//ErrReplacementError is a general error during parsing the replacement text
-var ErrReplacementError = errors.New("Replacement pattern error.")
-
-// NewReplacerData will populate a reusable replacer data struct based on the given replacement string
-// and the capture group data from a regexp
-func NewReplacerData(rep string, caps map[int]int, capsize int, capnames map[string]int, op RegexOptions) (*ReplacerData, error) {
- p := parser{
- options: op,
- caps: caps,
- capsize: capsize,
- capnames: capnames,
- }
- p.setPattern(rep)
- concat, err := p.scanReplacement()
- if err != nil {
- return nil, err
- }
-
- if concat.t != ntConcatenate {
- panic(ErrReplacementError)
- }
-
- sb := &bytes.Buffer{}
- var (
- strings []string
- rules []int
- )
-
- for _, child := range concat.children {
- switch child.t {
- case ntMulti:
- child.writeStrToBuf(sb)
-
- case ntOne:
- sb.WriteRune(child.ch)
-
- case ntRef:
- if sb.Len() > 0 {
- rules = append(rules, len(strings))
- strings = append(strings, sb.String())
- sb.Reset()
- }
- slot := child.m
-
- if len(caps) > 0 && slot >= 0 {
- slot = caps[slot]
- }
-
- rules = append(rules, -replaceSpecials-1-slot)
-
- default:
- panic(ErrReplacementError)
- }
- }
-
- if sb.Len() > 0 {
- rules = append(rules, len(strings))
- strings = append(strings, sb.String())
- }
-
- return &ReplacerData{
- Rep: rep,
- Strings: strings,
- Rules: rules,
- }, nil
-}
diff --git a/vendor/github.com/dlclark/regexp2/syntax/tree.go b/vendor/github.com/dlclark/regexp2/syntax/tree.go
deleted file mode 100644
index ea28829..0000000
--- a/vendor/github.com/dlclark/regexp2/syntax/tree.go
+++ /dev/null
@@ -1,654 +0,0 @@
-package syntax
-
-import (
- "bytes"
- "fmt"
- "math"
- "strconv"
-)
-
-type RegexTree struct {
- root *regexNode
- caps map[int]int
- capnumlist []int
- captop int
- Capnames map[string]int
- Caplist []string
- options RegexOptions
-}
-
-// It is built into a parsed tree for a regular expression.
-
-// Implementation notes:
-//
-// Since the node tree is a temporary data structure only used
-// during compilation of the regexp to integer codes, it's
-// designed for clarity and convenience rather than
-// space efficiency.
-//
-// RegexNodes are built into a tree, linked by the n.children list.
-// Each node also has a n.parent and n.ichild member indicating
-// its parent and which child # it is in its parent's list.
-//
-// RegexNodes come in as many types as there are constructs in
-// a regular expression, for example, "concatenate", "alternate",
-// "one", "rept", "group". There are also node types for basic
-// peephole optimizations, e.g., "onerep", "notsetrep", etc.
-//
-// Because perl 5 allows "lookback" groups that scan backwards,
-// each node also gets a "direction". Normally the value of
-// boolean n.backward = false.
-//
-// During parsing, top-level nodes are also stacked onto a parse
-// stack (a stack of trees). For this purpose we have a n.next
-// pointer. [Note that to save a few bytes, we could overload the
-// n.parent pointer instead.]
-//
-// On the parse stack, each tree has a "role" - basically, the
-// nonterminal in the grammar that the parser has currently
-// assigned to the tree. That code is stored in n.role.
-//
-// Finally, some of the different kinds of nodes have data.
-// Two integers (for the looping constructs) are stored in
-// n.operands, an an object (either a string or a set)
-// is stored in n.data
-type regexNode struct {
- t nodeType
- children []*regexNode
- str []rune
- set *CharSet
- ch rune
- m int
- n int
- options RegexOptions
- next *regexNode
-}
-
-type nodeType int32
-
-const (
- // The following are leaves, and correspond to primitive operations
-
- ntOnerep nodeType = 0 // lef,back char,min,max a {n}
- ntNotonerep = 1 // lef,back char,min,max .{n}
- ntSetrep = 2 // lef,back set,min,max [\d]{n}
- ntOneloop = 3 // lef,back char,min,max a {,n}
- ntNotoneloop = 4 // lef,back char,min,max .{,n}
- ntSetloop = 5 // lef,back set,min,max [\d]{,n}
- ntOnelazy = 6 // lef,back char,min,max a {,n}?
- ntNotonelazy = 7 // lef,back char,min,max .{,n}?
- ntSetlazy = 8 // lef,back set,min,max [\d]{,n}?
- ntOne = 9 // lef char a
- ntNotone = 10 // lef char [^a]
- ntSet = 11 // lef set [a-z\s] \w \s \d
- ntMulti = 12 // lef string abcd
- ntRef = 13 // lef group \#
- ntBol = 14 // ^
- ntEol = 15 // $
- ntBoundary = 16 // \b
- ntNonboundary = 17 // \B
- ntBeginning = 18 // \A
- ntStart = 19 // \G
- ntEndZ = 20 // \Z
- ntEnd = 21 // \Z
-
- // Interior nodes do not correspond to primitive operations, but
- // control structures compositing other operations
-
- // Concat and alternate take n children, and can run forward or backwards
-
- ntNothing = 22 // []
- ntEmpty = 23 // ()
- ntAlternate = 24 // a|b
- ntConcatenate = 25 // ab
- ntLoop = 26 // m,x * + ? {,}
- ntLazyloop = 27 // m,x *? +? ?? {,}?
- ntCapture = 28 // n ()
- ntGroup = 29 // (?:)
- ntRequire = 30 // (?=) (?<=)
- ntPrevent = 31 // (?!) (?) (?<)
- ntTestref = 33 // (?(n) | )
- ntTestgroup = 34 // (?(...) | )
-
- ntECMABoundary = 41 // \b
- ntNonECMABoundary = 42 // \B
-)
-
-func newRegexNode(t nodeType, opt RegexOptions) *regexNode {
- return ®exNode{
- t: t,
- options: opt,
- }
-}
-
-func newRegexNodeCh(t nodeType, opt RegexOptions, ch rune) *regexNode {
- return ®exNode{
- t: t,
- options: opt,
- ch: ch,
- }
-}
-
-func newRegexNodeStr(t nodeType, opt RegexOptions, str []rune) *regexNode {
- return ®exNode{
- t: t,
- options: opt,
- str: str,
- }
-}
-
-func newRegexNodeSet(t nodeType, opt RegexOptions, set *CharSet) *regexNode {
- return ®exNode{
- t: t,
- options: opt,
- set: set,
- }
-}
-
-func newRegexNodeM(t nodeType, opt RegexOptions, m int) *regexNode {
- return ®exNode{
- t: t,
- options: opt,
- m: m,
- }
-}
-func newRegexNodeMN(t nodeType, opt RegexOptions, m, n int) *regexNode {
- return ®exNode{
- t: t,
- options: opt,
- m: m,
- n: n,
- }
-}
-
-func (n *regexNode) writeStrToBuf(buf *bytes.Buffer) {
- for i := 0; i < len(n.str); i++ {
- buf.WriteRune(n.str[i])
- }
-}
-
-func (n *regexNode) addChild(child *regexNode) {
- reduced := child.reduce()
- n.children = append(n.children, reduced)
- reduced.next = n
-}
-
-func (n *regexNode) insertChildren(afterIndex int, nodes []*regexNode) {
- newChildren := make([]*regexNode, 0, len(n.children)+len(nodes))
- n.children = append(append(append(newChildren, n.children[:afterIndex]...), nodes...), n.children[afterIndex:]...)
-}
-
-// removes children including the start but not the end index
-func (n *regexNode) removeChildren(startIndex, endIndex int) {
- n.children = append(n.children[:startIndex], n.children[endIndex:]...)
-}
-
-// Pass type as OneLazy or OneLoop
-func (n *regexNode) makeRep(t nodeType, min, max int) {
- n.t += (t - ntOne)
- n.m = min
- n.n = max
-}
-
-func (n *regexNode) reduce() *regexNode {
- switch n.t {
- case ntAlternate:
- return n.reduceAlternation()
-
- case ntConcatenate:
- return n.reduceConcatenation()
-
- case ntLoop, ntLazyloop:
- return n.reduceRep()
-
- case ntGroup:
- return n.reduceGroup()
-
- case ntSet, ntSetloop:
- return n.reduceSet()
-
- default:
- return n
- }
-}
-
-// Basic optimization. Single-letter alternations can be replaced
-// by faster set specifications, and nested alternations with no
-// intervening operators can be flattened:
-//
-// a|b|c|def|g|h -> [a-c]|def|[gh]
-// apple|(?:orange|pear)|grape -> apple|orange|pear|grape
-func (n *regexNode) reduceAlternation() *regexNode {
- if len(n.children) == 0 {
- return newRegexNode(ntNothing, n.options)
- }
-
- wasLastSet := false
- lastNodeCannotMerge := false
- var optionsLast RegexOptions
- var i, j int
-
- for i, j = 0, 0; i < len(n.children); i, j = i+1, j+1 {
- at := n.children[i]
-
- if j < i {
- n.children[j] = at
- }
-
- for {
- if at.t == ntAlternate {
- for k := 0; k < len(at.children); k++ {
- at.children[k].next = n
- }
- n.insertChildren(i+1, at.children)
-
- j--
- } else if at.t == ntSet || at.t == ntOne {
- // Cannot merge sets if L or I options differ, or if either are negated.
- optionsAt := at.options & (RightToLeft | IgnoreCase)
-
- if at.t == ntSet {
- if !wasLastSet || optionsLast != optionsAt || lastNodeCannotMerge || !at.set.IsMergeable() {
- wasLastSet = true
- lastNodeCannotMerge = !at.set.IsMergeable()
- optionsLast = optionsAt
- break
- }
- } else if !wasLastSet || optionsLast != optionsAt || lastNodeCannotMerge {
- wasLastSet = true
- lastNodeCannotMerge = false
- optionsLast = optionsAt
- break
- }
-
- // The last node was a Set or a One, we're a Set or One and our options are the same.
- // Merge the two nodes.
- j--
- prev := n.children[j]
-
- var prevCharClass *CharSet
- if prev.t == ntOne {
- prevCharClass = &CharSet{}
- prevCharClass.addChar(prev.ch)
- } else {
- prevCharClass = prev.set
- }
-
- if at.t == ntOne {
- prevCharClass.addChar(at.ch)
- } else {
- prevCharClass.addSet(*at.set)
- }
-
- prev.t = ntSet
- prev.set = prevCharClass
- } else if at.t == ntNothing {
- j--
- } else {
- wasLastSet = false
- lastNodeCannotMerge = false
- }
- break
- }
- }
-
- if j < i {
- n.removeChildren(j, i)
- }
-
- return n.stripEnation(ntNothing)
-}
-
-// Basic optimization. Adjacent strings can be concatenated.
-//
-// (?:abc)(?:def) -> abcdef
-func (n *regexNode) reduceConcatenation() *regexNode {
- // Eliminate empties and concat adjacent strings/chars
-
- var optionsLast RegexOptions
- var optionsAt RegexOptions
- var i, j int
-
- if len(n.children) == 0 {
- return newRegexNode(ntEmpty, n.options)
- }
-
- wasLastString := false
-
- for i, j = 0, 0; i < len(n.children); i, j = i+1, j+1 {
- var at, prev *regexNode
-
- at = n.children[i]
-
- if j < i {
- n.children[j] = at
- }
-
- if at.t == ntConcatenate &&
- ((at.options & RightToLeft) == (n.options & RightToLeft)) {
- for k := 0; k < len(at.children); k++ {
- at.children[k].next = n
- }
-
- //insert at.children at i+1 index in n.children
- n.insertChildren(i+1, at.children)
-
- j--
- } else if at.t == ntMulti || at.t == ntOne {
- // Cannot merge strings if L or I options differ
- optionsAt = at.options & (RightToLeft | IgnoreCase)
-
- if !wasLastString || optionsLast != optionsAt {
- wasLastString = true
- optionsLast = optionsAt
- continue
- }
-
- j--
- prev = n.children[j]
-
- if prev.t == ntOne {
- prev.t = ntMulti
- prev.str = []rune{prev.ch}
- }
-
- if (optionsAt & RightToLeft) == 0 {
- if at.t == ntOne {
- prev.str = append(prev.str, at.ch)
- } else {
- prev.str = append(prev.str, at.str...)
- }
- } else {
- if at.t == ntOne {
- // insert at the front by expanding our slice, copying the data over, and then setting the value
- prev.str = append(prev.str, 0)
- copy(prev.str[1:], prev.str)
- prev.str[0] = at.ch
- } else {
- //insert at the front...this one we'll make a new slice and copy both into it
- merge := make([]rune, len(prev.str)+len(at.str))
- copy(merge, at.str)
- copy(merge[len(at.str):], prev.str)
- prev.str = merge
- }
- }
- } else if at.t == ntEmpty {
- j--
- } else {
- wasLastString = false
- }
- }
-
- if j < i {
- // remove indices j through i from the children
- n.removeChildren(j, i)
- }
-
- return n.stripEnation(ntEmpty)
-}
-
-// Nested repeaters just get multiplied with each other if they're not
-// too lumpy
-func (n *regexNode) reduceRep() *regexNode {
-
- u := n
- t := n.t
- min := n.m
- max := n.n
-
- for {
- if len(u.children) == 0 {
- break
- }
-
- child := u.children[0]
-
- // multiply reps of the same type only
- if child.t != t {
- childType := child.t
-
- if !(childType >= ntOneloop && childType <= ntSetloop && t == ntLoop ||
- childType >= ntOnelazy && childType <= ntSetlazy && t == ntLazyloop) {
- break
- }
- }
-
- // child can be too lumpy to blur, e.g., (a {100,105}) {3} or (a {2,})?
- // [but things like (a {2,})+ are not too lumpy...]
- if u.m == 0 && child.m > 1 || child.n < child.m*2 {
- break
- }
-
- u = child
- if u.m > 0 {
- if (math.MaxInt32-1)/u.m < min {
- u.m = math.MaxInt32
- } else {
- u.m = u.m * min
- }
- }
- if u.n > 0 {
- if (math.MaxInt32-1)/u.n < max {
- u.n = math.MaxInt32
- } else {
- u.n = u.n * max
- }
- }
- }
-
- if math.MaxInt32 == min {
- return newRegexNode(ntNothing, n.options)
- }
- return u
-
-}
-
-// Simple optimization. If a concatenation or alternation has only
-// one child strip out the intermediate node. If it has zero children,
-// turn it into an empty.
-func (n *regexNode) stripEnation(emptyType nodeType) *regexNode {
- switch len(n.children) {
- case 0:
- return newRegexNode(emptyType, n.options)
- case 1:
- return n.children[0]
- default:
- return n
- }
-}
-
-func (n *regexNode) reduceGroup() *regexNode {
- u := n
-
- for u.t == ntGroup {
- u = u.children[0]
- }
-
- return u
-}
-
-// Simple optimization. If a set is a singleton, an inverse singleton,
-// or empty, it's transformed accordingly.
-func (n *regexNode) reduceSet() *regexNode {
- // Extract empty-set, one and not-one case as special
-
- if n.set == nil {
- n.t = ntNothing
- } else if n.set.IsSingleton() {
- n.ch = n.set.SingletonChar()
- n.set = nil
- n.t += (ntOne - ntSet)
- } else if n.set.IsSingletonInverse() {
- n.ch = n.set.SingletonChar()
- n.set = nil
- n.t += (ntNotone - ntSet)
- }
-
- return n
-}
-
-func (n *regexNode) reverseLeft() *regexNode {
- if n.options&RightToLeft != 0 && n.t == ntConcatenate && len(n.children) > 0 {
- //reverse children order
- for left, right := 0, len(n.children)-1; left < right; left, right = left+1, right-1 {
- n.children[left], n.children[right] = n.children[right], n.children[left]
- }
- }
-
- return n
-}
-
-func (n *regexNode) makeQuantifier(lazy bool, min, max int) *regexNode {
- if min == 0 && max == 0 {
- return newRegexNode(ntEmpty, n.options)
- }
-
- if min == 1 && max == 1 {
- return n
- }
-
- switch n.t {
- case ntOne, ntNotone, ntSet:
- if lazy {
- n.makeRep(Onelazy, min, max)
- } else {
- n.makeRep(Oneloop, min, max)
- }
- return n
-
- default:
- var t nodeType
- if lazy {
- t = ntLazyloop
- } else {
- t = ntLoop
- }
- result := newRegexNodeMN(t, n.options, min, max)
- result.addChild(n)
- return result
- }
-}
-
-// debug functions
-
-var typeStr = []string{
- "Onerep", "Notonerep", "Setrep",
- "Oneloop", "Notoneloop", "Setloop",
- "Onelazy", "Notonelazy", "Setlazy",
- "One", "Notone", "Set",
- "Multi", "Ref",
- "Bol", "Eol", "Boundary", "Nonboundary",
- "Beginning", "Start", "EndZ", "End",
- "Nothing", "Empty",
- "Alternate", "Concatenate",
- "Loop", "Lazyloop",
- "Capture", "Group", "Require", "Prevent", "Greedy",
- "Testref", "Testgroup",
- "Unknown", "Unknown", "Unknown",
- "Unknown", "Unknown", "Unknown",
- "ECMABoundary", "NonECMABoundary",
-}
-
-func (n *regexNode) description() string {
- buf := &bytes.Buffer{}
-
- buf.WriteString(typeStr[n.t])
-
- if (n.options & ExplicitCapture) != 0 {
- buf.WriteString("-C")
- }
- if (n.options & IgnoreCase) != 0 {
- buf.WriteString("-I")
- }
- if (n.options & RightToLeft) != 0 {
- buf.WriteString("-L")
- }
- if (n.options & Multiline) != 0 {
- buf.WriteString("-M")
- }
- if (n.options & Singleline) != 0 {
- buf.WriteString("-S")
- }
- if (n.options & IgnorePatternWhitespace) != 0 {
- buf.WriteString("-X")
- }
- if (n.options & ECMAScript) != 0 {
- buf.WriteString("-E")
- }
-
- switch n.t {
- case ntOneloop, ntNotoneloop, ntOnelazy, ntNotonelazy, ntOne, ntNotone:
- buf.WriteString("(Ch = " + CharDescription(n.ch) + ")")
- break
- case ntCapture:
- buf.WriteString("(index = " + strconv.Itoa(n.m) + ", unindex = " + strconv.Itoa(n.n) + ")")
- break
- case ntRef, ntTestref:
- buf.WriteString("(index = " + strconv.Itoa(n.m) + ")")
- break
- case ntMulti:
- fmt.Fprintf(buf, "(String = %s)", string(n.str))
- break
- case ntSet, ntSetloop, ntSetlazy:
- buf.WriteString("(Set = " + n.set.String() + ")")
- break
- }
-
- switch n.t {
- case ntOneloop, ntNotoneloop, ntOnelazy, ntNotonelazy, ntSetloop, ntSetlazy, ntLoop, ntLazyloop:
- buf.WriteString("(Min = ")
- buf.WriteString(strconv.Itoa(n.m))
- buf.WriteString(", Max = ")
- if n.n == math.MaxInt32 {
- buf.WriteString("inf")
- } else {
- buf.WriteString(strconv.Itoa(n.n))
- }
- buf.WriteString(")")
-
- break
- }
-
- return buf.String()
-}
-
-var padSpace = []byte(" ")
-
-func (t *RegexTree) Dump() string {
- return t.root.dump()
-}
-
-func (n *regexNode) dump() string {
- var stack []int
- CurNode := n
- CurChild := 0
-
- buf := bytes.NewBufferString(CurNode.description())
- buf.WriteRune('\n')
-
- for {
- if CurNode.children != nil && CurChild < len(CurNode.children) {
- stack = append(stack, CurChild+1)
- CurNode = CurNode.children[CurChild]
- CurChild = 0
-
- Depth := len(stack)
- if Depth > 32 {
- Depth = 32
- }
- buf.Write(padSpace[:Depth])
- buf.WriteString(CurNode.description())
- buf.WriteRune('\n')
- } else {
- if len(stack) == 0 {
- break
- }
-
- CurChild = stack[len(stack)-1]
- stack = stack[:len(stack)-1]
- CurNode = CurNode.next
- }
- }
- return buf.String()
-}
diff --git a/vendor/github.com/dlclark/regexp2/syntax/writer.go b/vendor/github.com/dlclark/regexp2/syntax/writer.go
deleted file mode 100644
index a5aa11c..0000000
--- a/vendor/github.com/dlclark/regexp2/syntax/writer.go
+++ /dev/null
@@ -1,500 +0,0 @@
-package syntax
-
-import (
- "bytes"
- "fmt"
- "math"
- "os"
-)
-
-func Write(tree *RegexTree) (*Code, error) {
- w := writer{
- intStack: make([]int, 0, 32),
- emitted: make([]int, 2),
- stringhash: make(map[string]int),
- sethash: make(map[string]int),
- }
-
- code, err := w.codeFromTree(tree)
-
- if tree.options&Debug > 0 && code != nil {
- os.Stdout.WriteString(code.Dump())
- os.Stdout.WriteString("\n")
- }
-
- return code, err
-}
-
-type writer struct {
- emitted []int
-
- intStack []int
- curpos int
- stringhash map[string]int
- stringtable [][]rune
- sethash map[string]int
- settable []*CharSet
- counting bool
- count int
- trackcount int
- caps map[int]int
-}
-
-const (
- beforeChild nodeType = 64
- afterChild = 128
- //MaxPrefixSize is the largest number of runes we'll use for a BoyerMoyer prefix
- MaxPrefixSize = 50
-)
-
-// The top level RegexCode generator. It does a depth-first walk
-// through the tree and calls EmitFragment to emits code before
-// and after each child of an interior node, and at each leaf.
-//
-// It runs two passes, first to count the size of the generated
-// code, and second to generate the code.
-//
-// We should time it against the alternative, which is
-// to just generate the code and grow the array as we go.
-func (w *writer) codeFromTree(tree *RegexTree) (*Code, error) {
- var (
- curNode *regexNode
- curChild int
- capsize int
- )
- // construct sparse capnum mapping if some numbers are unused
-
- if tree.capnumlist == nil || tree.captop == len(tree.capnumlist) {
- capsize = tree.captop
- w.caps = nil
- } else {
- capsize = len(tree.capnumlist)
- w.caps = tree.caps
- for i := 0; i < len(tree.capnumlist); i++ {
- w.caps[tree.capnumlist[i]] = i
- }
- }
-
- w.counting = true
-
- for {
- if !w.counting {
- w.emitted = make([]int, w.count)
- }
-
- curNode = tree.root
- curChild = 0
-
- w.emit1(Lazybranch, 0)
-
- for {
- if len(curNode.children) == 0 {
- w.emitFragment(curNode.t, curNode, 0)
- } else if curChild < len(curNode.children) {
- w.emitFragment(curNode.t|beforeChild, curNode, curChild)
-
- curNode = curNode.children[curChild]
-
- w.pushInt(curChild)
- curChild = 0
- continue
- }
-
- if w.emptyStack() {
- break
- }
-
- curChild = w.popInt()
- curNode = curNode.next
-
- w.emitFragment(curNode.t|afterChild, curNode, curChild)
- curChild++
- }
-
- w.patchJump(0, w.curPos())
- w.emit(Stop)
-
- if !w.counting {
- break
- }
-
- w.counting = false
- }
-
- fcPrefix := getFirstCharsPrefix(tree)
- prefix := getPrefix(tree)
- rtl := (tree.options & RightToLeft) != 0
-
- var bmPrefix *BmPrefix
- //TODO: benchmark string prefixes
- if prefix != nil && len(prefix.PrefixStr) > 0 && MaxPrefixSize > 0 {
- if len(prefix.PrefixStr) > MaxPrefixSize {
- // limit prefix changes to 10k
- prefix.PrefixStr = prefix.PrefixStr[:MaxPrefixSize]
- }
- bmPrefix = newBmPrefix(prefix.PrefixStr, prefix.CaseInsensitive, rtl)
- } else {
- bmPrefix = nil
- }
-
- return &Code{
- Codes: w.emitted,
- Strings: w.stringtable,
- Sets: w.settable,
- TrackCount: w.trackcount,
- Caps: w.caps,
- Capsize: capsize,
- FcPrefix: fcPrefix,
- BmPrefix: bmPrefix,
- Anchors: getAnchors(tree),
- RightToLeft: rtl,
- }, nil
-}
-
-// The main RegexCode generator. It does a depth-first walk
-// through the tree and calls EmitFragment to emits code before
-// and after each child of an interior node, and at each leaf.
-func (w *writer) emitFragment(nodetype nodeType, node *regexNode, curIndex int) error {
- bits := InstOp(0)
-
- if nodetype <= ntRef {
- if (node.options & RightToLeft) != 0 {
- bits |= Rtl
- }
- if (node.options & IgnoreCase) != 0 {
- bits |= Ci
- }
- }
- ntBits := nodeType(bits)
-
- switch nodetype {
- case ntConcatenate | beforeChild, ntConcatenate | afterChild, ntEmpty:
- break
-
- case ntAlternate | beforeChild:
- if curIndex < len(node.children)-1 {
- w.pushInt(w.curPos())
- w.emit1(Lazybranch, 0)
- }
-
- case ntAlternate | afterChild:
- if curIndex < len(node.children)-1 {
- lbPos := w.popInt()
- w.pushInt(w.curPos())
- w.emit1(Goto, 0)
- w.patchJump(lbPos, w.curPos())
- } else {
- for i := 0; i < curIndex; i++ {
- w.patchJump(w.popInt(), w.curPos())
- }
- }
- break
-
- case ntTestref | beforeChild:
- if curIndex == 0 {
- w.emit(Setjump)
- w.pushInt(w.curPos())
- w.emit1(Lazybranch, 0)
- w.emit1(Testref, w.mapCapnum(node.m))
- w.emit(Forejump)
- }
-
- case ntTestref | afterChild:
- if curIndex == 0 {
- branchpos := w.popInt()
- w.pushInt(w.curPos())
- w.emit1(Goto, 0)
- w.patchJump(branchpos, w.curPos())
- w.emit(Forejump)
- if len(node.children) <= 1 {
- w.patchJump(w.popInt(), w.curPos())
- }
- } else if curIndex == 1 {
- w.patchJump(w.popInt(), w.curPos())
- }
-
- case ntTestgroup | beforeChild:
- if curIndex == 0 {
- w.emit(Setjump)
- w.emit(Setmark)
- w.pushInt(w.curPos())
- w.emit1(Lazybranch, 0)
- }
-
- case ntTestgroup | afterChild:
- if curIndex == 0 {
- w.emit(Getmark)
- w.emit(Forejump)
- } else if curIndex == 1 {
- Branchpos := w.popInt()
- w.pushInt(w.curPos())
- w.emit1(Goto, 0)
- w.patchJump(Branchpos, w.curPos())
- w.emit(Getmark)
- w.emit(Forejump)
- if len(node.children) <= 2 {
- w.patchJump(w.popInt(), w.curPos())
- }
- } else if curIndex == 2 {
- w.patchJump(w.popInt(), w.curPos())
- }
-
- case ntLoop | beforeChild, ntLazyloop | beforeChild:
-
- if node.n < math.MaxInt32 || node.m > 1 {
- if node.m == 0 {
- w.emit1(Nullcount, 0)
- } else {
- w.emit1(Setcount, 1-node.m)
- }
- } else if node.m == 0 {
- w.emit(Nullmark)
- } else {
- w.emit(Setmark)
- }
-
- if node.m == 0 {
- w.pushInt(w.curPos())
- w.emit1(Goto, 0)
- }
- w.pushInt(w.curPos())
-
- case ntLoop | afterChild, ntLazyloop | afterChild:
-
- startJumpPos := w.curPos()
- lazy := (nodetype - (ntLoop | afterChild))
-
- if node.n < math.MaxInt32 || node.m > 1 {
- if node.n == math.MaxInt32 {
- w.emit2(InstOp(Branchcount+lazy), w.popInt(), math.MaxInt32)
- } else {
- w.emit2(InstOp(Branchcount+lazy), w.popInt(), node.n-node.m)
- }
- } else {
- w.emit1(InstOp(Branchmark+lazy), w.popInt())
- }
-
- if node.m == 0 {
- w.patchJump(w.popInt(), startJumpPos)
- }
-
- case ntGroup | beforeChild, ntGroup | afterChild:
-
- case ntCapture | beforeChild:
- w.emit(Setmark)
-
- case ntCapture | afterChild:
- w.emit2(Capturemark, w.mapCapnum(node.m), w.mapCapnum(node.n))
-
- case ntRequire | beforeChild:
- // NOTE: the following line causes lookahead/lookbehind to be
- // NON-BACKTRACKING. It can be commented out with (*)
- w.emit(Setjump)
-
- w.emit(Setmark)
-
- case ntRequire | afterChild:
- w.emit(Getmark)
-
- // NOTE: the following line causes lookahead/lookbehind to be
- // NON-BACKTRACKING. It can be commented out with (*)
- w.emit(Forejump)
-
- case ntPrevent | beforeChild:
- w.emit(Setjump)
- w.pushInt(w.curPos())
- w.emit1(Lazybranch, 0)
-
- case ntPrevent | afterChild:
- w.emit(Backjump)
- w.patchJump(w.popInt(), w.curPos())
- w.emit(Forejump)
-
- case ntGreedy | beforeChild:
- w.emit(Setjump)
-
- case ntGreedy | afterChild:
- w.emit(Forejump)
-
- case ntOne, ntNotone:
- w.emit1(InstOp(node.t|ntBits), int(node.ch))
-
- case ntNotoneloop, ntNotonelazy, ntOneloop, ntOnelazy:
- if node.m > 0 {
- if node.t == ntOneloop || node.t == ntOnelazy {
- w.emit2(Onerep|bits, int(node.ch), node.m)
- } else {
- w.emit2(Notonerep|bits, int(node.ch), node.m)
- }
- }
- if node.n > node.m {
- if node.n == math.MaxInt32 {
- w.emit2(InstOp(node.t|ntBits), int(node.ch), math.MaxInt32)
- } else {
- w.emit2(InstOp(node.t|ntBits), int(node.ch), node.n-node.m)
- }
- }
-
- case ntSetloop, ntSetlazy:
- if node.m > 0 {
- w.emit2(Setrep|bits, w.setCode(node.set), node.m)
- }
- if node.n > node.m {
- if node.n == math.MaxInt32 {
- w.emit2(InstOp(node.t|ntBits), w.setCode(node.set), math.MaxInt32)
- } else {
- w.emit2(InstOp(node.t|ntBits), w.setCode(node.set), node.n-node.m)
- }
- }
-
- case ntMulti:
- w.emit1(InstOp(node.t|ntBits), w.stringCode(node.str))
-
- case ntSet:
- w.emit1(InstOp(node.t|ntBits), w.setCode(node.set))
-
- case ntRef:
- w.emit1(InstOp(node.t|ntBits), w.mapCapnum(node.m))
-
- case ntNothing, ntBol, ntEol, ntBoundary, ntNonboundary, ntECMABoundary, ntNonECMABoundary, ntBeginning, ntStart, ntEndZ, ntEnd:
- w.emit(InstOp(node.t))
-
- default:
- return fmt.Errorf("unexpected opcode in regular expression generation: %v", nodetype)
- }
-
- return nil
-}
-
-// To avoid recursion, we use a simple integer stack.
-// This is the push.
-func (w *writer) pushInt(i int) {
- w.intStack = append(w.intStack, i)
-}
-
-// Returns true if the stack is empty.
-func (w *writer) emptyStack() bool {
- return len(w.intStack) == 0
-}
-
-// This is the pop.
-func (w *writer) popInt() int {
- //get our item
- idx := len(w.intStack) - 1
- i := w.intStack[idx]
- //trim our slice
- w.intStack = w.intStack[:idx]
- return i
-}
-
-// Returns the current position in the emitted code.
-func (w *writer) curPos() int {
- return w.curpos
-}
-
-// Fixes up a jump instruction at the specified offset
-// so that it jumps to the specified jumpDest.
-func (w *writer) patchJump(offset, jumpDest int) {
- w.emitted[offset+1] = jumpDest
-}
-
-// Returns an index in the set table for a charset
-// uses a map to eliminate duplicates.
-func (w *writer) setCode(set *CharSet) int {
- if w.counting {
- return 0
- }
-
- buf := &bytes.Buffer{}
-
- set.mapHashFill(buf)
- hash := buf.String()
- i, ok := w.sethash[hash]
- if !ok {
- i = len(w.sethash)
- w.sethash[hash] = i
- w.settable = append(w.settable, set)
- }
- return i
-}
-
-// Returns an index in the string table for a string.
-// uses a map to eliminate duplicates.
-func (w *writer) stringCode(str []rune) int {
- if w.counting {
- return 0
- }
-
- hash := string(str)
- i, ok := w.stringhash[hash]
- if !ok {
- i = len(w.stringhash)
- w.stringhash[hash] = i
- w.stringtable = append(w.stringtable, str)
- }
-
- return i
-}
-
-// When generating code on a regex that uses a sparse set
-// of capture slots, we hash them to a dense set of indices
-// for an array of capture slots. Instead of doing the hash
-// at match time, it's done at compile time, here.
-func (w *writer) mapCapnum(capnum int) int {
- if capnum == -1 {
- return -1
- }
-
- if w.caps != nil {
- return w.caps[capnum]
- }
-
- return capnum
-}
-
-// Emits a zero-argument operation. Note that the emit
-// functions all run in two modes: they can emit code, or
-// they can just count the size of the code.
-func (w *writer) emit(op InstOp) {
- if w.counting {
- w.count++
- if opcodeBacktracks(op) {
- w.trackcount++
- }
- return
- }
- w.emitted[w.curpos] = int(op)
- w.curpos++
-}
-
-// Emits a one-argument operation.
-func (w *writer) emit1(op InstOp, opd1 int) {
- if w.counting {
- w.count += 2
- if opcodeBacktracks(op) {
- w.trackcount++
- }
- return
- }
- w.emitted[w.curpos] = int(op)
- w.curpos++
- w.emitted[w.curpos] = opd1
- w.curpos++
-}
-
-// Emits a two-argument operation.
-func (w *writer) emit2(op InstOp, opd1, opd2 int) {
- if w.counting {
- w.count += 3
- if opcodeBacktracks(op) {
- w.trackcount++
- }
- return
- }
- w.emitted[w.curpos] = int(op)
- w.curpos++
- w.emitted[w.curpos] = opd1
- w.curpos++
- w.emitted[w.curpos] = opd2
- w.curpos++
-}
diff --git a/vendor/github.com/dlclark/regexp2/testoutput1 b/vendor/github.com/dlclark/regexp2/testoutput1
deleted file mode 100644
index fbf63fd..0000000
--- a/vendor/github.com/dlclark/regexp2/testoutput1
+++ /dev/null
@@ -1,7061 +0,0 @@
-# This set of tests is for features that are compatible with all versions of
-# Perl >= 5.10, in non-UTF mode. It should run clean for the 8-bit, 16-bit, and
-# 32-bit PCRE libraries, and also using the perltest.pl script.
-
-#forbid_utf
-#newline_default lf any anycrlf
-#perltest
-
-/the quick brown fox/
- the quick brown fox
- 0: the quick brown fox
- What do you know about the quick brown fox?
- 0: the quick brown fox
-\= Expect no match
- The quick brown FOX
-No match
- What do you know about THE QUICK BROWN FOX?
-No match
-
-/The quick brown fox/i
- the quick brown fox
- 0: the quick brown fox
- The quick brown FOX
- 0: The quick brown FOX
- What do you know about the quick brown fox?
- 0: the quick brown fox
- What do you know about THE QUICK BROWN FOX?
- 0: THE QUICK BROWN FOX
-
-/abcd\t\n\r\f\a\e\071\x3b\$\\\?caxyz/
- abcd\t\n\r\f\a\e9;\$\\?caxyz
- 0: abcd\x09\x0a\x0d\x0c\x07\x1b9;$\?caxyz
-
-/a*abc?xyz+pqr{3}ab{2,}xy{4,5}pq{0,6}AB{0,}zz/
- abxyzpqrrrabbxyyyypqAzz
- 0: abxyzpqrrrabbxyyyypqAzz
- abxyzpqrrrabbxyyyypqAzz
- 0: abxyzpqrrrabbxyyyypqAzz
- aabxyzpqrrrabbxyyyypqAzz
- 0: aabxyzpqrrrabbxyyyypqAzz
- aaabxyzpqrrrabbxyyyypqAzz
- 0: aaabxyzpqrrrabbxyyyypqAzz
- aaaabxyzpqrrrabbxyyyypqAzz
- 0: aaaabxyzpqrrrabbxyyyypqAzz
- abcxyzpqrrrabbxyyyypqAzz
- 0: abcxyzpqrrrabbxyyyypqAzz
- aabcxyzpqrrrabbxyyyypqAzz
- 0: aabcxyzpqrrrabbxyyyypqAzz
- aaabcxyzpqrrrabbxyyyypAzz
- 0: aaabcxyzpqrrrabbxyyyypAzz
- aaabcxyzpqrrrabbxyyyypqAzz
- 0: aaabcxyzpqrrrabbxyyyypqAzz
- aaabcxyzpqrrrabbxyyyypqqAzz
- 0: aaabcxyzpqrrrabbxyyyypqqAzz
- aaabcxyzpqrrrabbxyyyypqqqAzz
- 0: aaabcxyzpqrrrabbxyyyypqqqAzz
- aaabcxyzpqrrrabbxyyyypqqqqAzz
- 0: aaabcxyzpqrrrabbxyyyypqqqqAzz
- aaabcxyzpqrrrabbxyyyypqqqqqAzz
- 0: aaabcxyzpqrrrabbxyyyypqqqqqAzz
- aaabcxyzpqrrrabbxyyyypqqqqqqAzz
- 0: aaabcxyzpqrrrabbxyyyypqqqqqqAzz
- aaaabcxyzpqrrrabbxyyyypqAzz
- 0: aaaabcxyzpqrrrabbxyyyypqAzz
- abxyzzpqrrrabbxyyyypqAzz
- 0: abxyzzpqrrrabbxyyyypqAzz
- aabxyzzzpqrrrabbxyyyypqAzz
- 0: aabxyzzzpqrrrabbxyyyypqAzz
- aaabxyzzzzpqrrrabbxyyyypqAzz
- 0: aaabxyzzzzpqrrrabbxyyyypqAzz
- aaaabxyzzzzpqrrrabbxyyyypqAzz
- 0: aaaabxyzzzzpqrrrabbxyyyypqAzz
- abcxyzzpqrrrabbxyyyypqAzz
- 0: abcxyzzpqrrrabbxyyyypqAzz
- aabcxyzzzpqrrrabbxyyyypqAzz
- 0: aabcxyzzzpqrrrabbxyyyypqAzz
- aaabcxyzzzzpqrrrabbxyyyypqAzz
- 0: aaabcxyzzzzpqrrrabbxyyyypqAzz
- aaaabcxyzzzzpqrrrabbxyyyypqAzz
- 0: aaaabcxyzzzzpqrrrabbxyyyypqAzz
- aaaabcxyzzzzpqrrrabbbxyyyypqAzz
- 0: aaaabcxyzzzzpqrrrabbbxyyyypqAzz
- aaaabcxyzzzzpqrrrabbbxyyyyypqAzz
- 0: aaaabcxyzzzzpqrrrabbbxyyyyypqAzz
- aaabcxyzpqrrrabbxyyyypABzz
- 0: aaabcxyzpqrrrabbxyyyypABzz
- aaabcxyzpqrrrabbxyyyypABBzz
- 0: aaabcxyzpqrrrabbxyyyypABBzz
- >>>aaabxyzpqrrrabbxyyyypqAzz
- 0: aaabxyzpqrrrabbxyyyypqAzz
- >aaaabxyzpqrrrabbxyyyypqAzz
- 0: aaaabxyzpqrrrabbxyyyypqAzz
- >>>>abcxyzpqrrrabbxyyyypqAzz
- 0: abcxyzpqrrrabbxyyyypqAzz
-\= Expect no match
- abxyzpqrrabbxyyyypqAzz
-No match
- abxyzpqrrrrabbxyyyypqAzz
-No match
- abxyzpqrrrabxyyyypqAzz
-No match
- aaaabcxyzzzzpqrrrabbbxyyyyyypqAzz
-No match
- aaaabcxyzzzzpqrrrabbbxyyypqAzz
-No match
- aaabcxyzpqrrrabbxyyyypqqqqqqqAzz
-No match
-
-/^(abc){1,2}zz/
- abczz
- 0: abczz
- 1: abc
- abcabczz
- 0: abcabczz
- 1: abc
-\= Expect no match
- zz
-No match
- abcabcabczz
-No match
- >>abczz
-No match
-
-/^(b+?|a){1,2}?c/
- bc
- 0: bc
- 1: b
- bbc
- 0: bbc
- 1: b
- bbbc
- 0: bbbc
- 1: bb
- bac
- 0: bac
- 1: a
- bbac
- 0: bbac
- 1: a
- aac
- 0: aac
- 1: a
- abbbbbbbbbbbc
- 0: abbbbbbbbbbbc
- 1: bbbbbbbbbbb
- bbbbbbbbbbbac
- 0: bbbbbbbbbbbac
- 1: a
-\= Expect no match
- aaac
-No match
- abbbbbbbbbbbac
-No match
-
-/^(b+|a){1,2}c/
- bc
- 0: bc
- 1: b
- bbc
- 0: bbc
- 1: bb
- bbbc
- 0: bbbc
- 1: bbb
- bac
- 0: bac
- 1: a
- bbac
- 0: bbac
- 1: a
- aac
- 0: aac
- 1: a
- abbbbbbbbbbbc
- 0: abbbbbbbbbbbc
- 1: bbbbbbbbbbb
- bbbbbbbbbbbac
- 0: bbbbbbbbbbbac
- 1: a
-\= Expect no match
- aaac
-No match
- abbbbbbbbbbbac
-No match
-
-/^(b+|a){1,2}?bc/
- bbc
- 0: bbc
- 1: b
-
-/^(b*|ba){1,2}?bc/
- babc
- 0: babc
- 1: ba
- bbabc
- 0: bbabc
- 1: ba
- bababc
- 0: bababc
- 1: ba
-\= Expect no match
- bababbc
-No match
- babababc
-No match
-
-/^(ba|b*){1,2}?bc/
- babc
- 0: babc
- 1: ba
- bbabc
- 0: bbabc
- 1: ba
- bababc
- 0: bababc
- 1: ba
-\= Expect no match
- bababbc
-No match
- babababc
-No match
-
-#/^\ca\cA\c[;\c:/
-# \x01\x01\e;z
-# 0: \x01\x01\x1b;z
-
-/^[ab\]cde]/
- athing
- 0: a
- bthing
- 0: b
- ]thing
- 0: ]
- cthing
- 0: c
- dthing
- 0: d
- ething
- 0: e
-\= Expect no match
- fthing
-No match
- [thing
-No match
- \\thing
-No match
-
-/^[]cde]/
- ]thing
- 0: ]
- cthing
- 0: c
- dthing
- 0: d
- ething
- 0: e
-\= Expect no match
- athing
-No match
- fthing
-No match
-
-/^[^ab\]cde]/
- fthing
- 0: f
- [thing
- 0: [
- \\thing
- 0: \
-\= Expect no match
- athing
-No match
- bthing
-No match
- ]thing
-No match
- cthing
-No match
- dthing
-No match
- ething
-No match
-
-/^[^]cde]/
- athing
- 0: a
- fthing
- 0: f
-\= Expect no match
- ]thing
-No match
- cthing
-No match
- dthing
-No match
- ething
-No match
-
-# DLC - I don't get this one
-#/^\/
-#
-# 0: \x81
-
-#updated to handle 16-bits utf8
-/^ÿ/
- ÿ
- 0: \xc3\xbf
-
-/^[0-9]+$/
- 0
- 0: 0
- 1
- 0: 1
- 2
- 0: 2
- 3
- 0: 3
- 4
- 0: 4
- 5
- 0: 5
- 6
- 0: 6
- 7
- 0: 7
- 8
- 0: 8
- 9
- 0: 9
- 10
- 0: 10
- 100
- 0: 100
-\= Expect no match
- abc
-No match
-
-/^.*nter/
- enter
- 0: enter
- inter
- 0: inter
- uponter
- 0: uponter
-
-/^xxx[0-9]+$/
- xxx0
- 0: xxx0
- xxx1234
- 0: xxx1234
-\= Expect no match
- xxx
-No match
-
-/^.+[0-9][0-9][0-9]$/
- x123
- 0: x123
- x1234
- 0: x1234
- xx123
- 0: xx123
- 123456
- 0: 123456
-\= Expect no match
- 123
-No match
-
-/^.+?[0-9][0-9][0-9]$/
- x123
- 0: x123
- x1234
- 0: x1234
- xx123
- 0: xx123
- 123456
- 0: 123456
-\= Expect no match
- 123
-No match
-
-/^([^!]+)!(.+)=apquxz\.ixr\.zzz\.ac\.uk$/
- abc!pqr=apquxz.ixr.zzz.ac.uk
- 0: abc!pqr=apquxz.ixr.zzz.ac.uk
- 1: abc
- 2: pqr
-\= Expect no match
- !pqr=apquxz.ixr.zzz.ac.uk
-No match
- abc!=apquxz.ixr.zzz.ac.uk
-No match
- abc!pqr=apquxz:ixr.zzz.ac.uk
-No match
- abc!pqr=apquxz.ixr.zzz.ac.ukk
-No match
-
-/:/
- Well, we need a colon: somewhere
- 0: :
-\= Expect no match
- Fail without a colon
-No match
-
-/([\da-f:]+)$/i
- 0abc
- 0: 0abc
- 1: 0abc
- abc
- 0: abc
- 1: abc
- fed
- 0: fed
- 1: fed
- E
- 0: E
- 1: E
- ::
- 0: ::
- 1: ::
- 5f03:12C0::932e
- 0: 5f03:12C0::932e
- 1: 5f03:12C0::932e
- fed def
- 0: def
- 1: def
- Any old stuff
- 0: ff
- 1: ff
-\= Expect no match
- 0zzz
-No match
- gzzz
-No match
- fed\x20
-No match
- Any old rubbish
-No match
-
-/^.*\.(\d{1,3})\.(\d{1,3})\.(\d{1,3})$/
- .1.2.3
- 0: .1.2.3
- 1: 1
- 2: 2
- 3: 3
- A.12.123.0
- 0: A.12.123.0
- 1: 12
- 2: 123
- 3: 0
-\= Expect no match
- .1.2.3333
-No match
- 1.2.3
-No match
- 1234.2.3
-No match
-
-/^(\d+)\s+IN\s+SOA\s+(\S+)\s+(\S+)\s*\(\s*$/
- 1 IN SOA non-sp1 non-sp2(
- 0: 1 IN SOA non-sp1 non-sp2(
- 1: 1
- 2: non-sp1
- 3: non-sp2
- 1 IN SOA non-sp1 non-sp2 (
- 0: 1 IN SOA non-sp1 non-sp2 (
- 1: 1
- 2: non-sp1
- 3: non-sp2
-\= Expect no match
- 1IN SOA non-sp1 non-sp2(
-No match
-
-/^[a-zA-Z\d][a-zA-Z\d\-]*(\.[a-zA-Z\d][a-zA-z\d\-]*)*\.$/
- a.
- 0: a.
- Z.
- 0: Z.
- 2.
- 0: 2.
- ab-c.pq-r.
- 0: ab-c.pq-r.
- 1: .pq-r
- sxk.zzz.ac.uk.
- 0: sxk.zzz.ac.uk.
- 1: .uk
- x-.y-.
- 0: x-.y-.
- 1: .y-
-\= Expect no match
- -abc.peq.
-No match
-
-/^\*\.[a-z]([a-z\-\d]*[a-z\d]+)?(\.[a-z]([a-z\-\d]*[a-z\d]+)?)*$/
- *.a
- 0: *.a
- *.b0-a
- 0: *.b0-a
- 1: 0-a
- *.c3-b.c
- 0: *.c3-b.c
- 1: 3-b
- 2: .c
- *.c-a.b-c
- 0: *.c-a.b-c
- 1: -a
- 2: .b-c
- 3: -c
-\= Expect no match
- *.0
-No match
- *.a-
-No match
- *.a-b.c-
-No match
- *.c-a.0-c
-No match
-
-/^(?=ab(de))(abd)(e)/
- abde
- 0: abde
- 1: de
- 2: abd
- 3: e
-
-/^(?!(ab)de|x)(abd)(f)/
- abdf
- 0: abdf
- 1:
- 2: abd
- 3: f
-
-/^(?=(ab(cd)))(ab)/
- abcd
- 0: ab
- 1: abcd
- 2: cd
- 3: ab
-
-/^[\da-f](\.[\da-f])*$/i
- a.b.c.d
- 0: a.b.c.d
- 1: .d
- A.B.C.D
- 0: A.B.C.D
- 1: .D
- a.b.c.1.2.3.C
- 0: a.b.c.1.2.3.C
- 1: .C
-
-/^\".*\"\s*(;.*)?$/
- \"1234\"
- 0: "1234"
- \"abcd\" ;
- 0: "abcd" ;
- 1: ;
- \"\" ; rhubarb
- 0: "" ; rhubarb
- 1: ; rhubarb
-\= Expect no match
- \"1234\" : things
-No match
-
-/^$/
- \
- 0:
-\= Expect no match
- A non-empty line
-No match
-
-/ ^ a (?# begins with a) b\sc (?# then b c) $ (?# then end)/x
- ab c
- 0: ab c
-\= Expect no match
- abc
-No match
- ab cde
-No match
-
-/(?x) ^ a (?# begins with a) b\sc (?# then b c) $ (?# then end)/
- ab c
- 0: ab c
-\= Expect no match
- abc
-No match
- ab cde
-No match
-
-/^ a\ b[c ]d $/x
- a bcd
- 0: a bcd
- a b d
- 0: a b d
-\= Expect no match
- abcd
-No match
- ab d
-No match
-
-/^(a(b(c)))(d(e(f)))(h(i(j)))(k(l(m)))$/
- abcdefhijklm
- 0: abcdefhijklm
- 1: abc
- 2: bc
- 3: c
- 4: def
- 5: ef
- 6: f
- 7: hij
- 8: ij
- 9: j
-10: klm
-11: lm
-12: m
-
-/^(?:a(b(c)))(?:d(e(f)))(?:h(i(j)))(?:k(l(m)))$/
- abcdefhijklm
- 0: abcdefhijklm
- 1: bc
- 2: c
- 3: ef
- 4: f
- 5: ij
- 6: j
- 7: lm
- 8: m
-
-#/^[\w][\W][\s][\S][\d][\D][\b][\n][\c]][\022]/
-# a+ Z0+\x08\n\x1d\x12
-# 0: a+ Z0+\x08\x0a\x1d\x12
-
-/^[.^$|()*+?{,}]+/
- .^\$(*+)|{?,?}
- 0: .^$(*+)|{?,?}
-
-/^a*\w/
- z
- 0: z
- az
- 0: az
- aaaz
- 0: aaaz
- a
- 0: a
- aa
- 0: aa
- aaaa
- 0: aaaa
- a+
- 0: a
- aa+
- 0: aa
-
-/^a*?\w/
- z
- 0: z
- az
- 0: a
- aaaz
- 0: a
- a
- 0: a
- aa
- 0: a
- aaaa
- 0: a
- a+
- 0: a
- aa+
- 0: a
-
-/^a+\w/
- az
- 0: az
- aaaz
- 0: aaaz
- aa
- 0: aa
- aaaa
- 0: aaaa
- aa+
- 0: aa
-
-/^a+?\w/
- az
- 0: az
- aaaz
- 0: aa
- aa
- 0: aa
- aaaa
- 0: aa
- aa+
- 0: aa
-
-/^\d{8}\w{2,}/
- 1234567890
- 0: 1234567890
- 12345678ab
- 0: 12345678ab
- 12345678__
- 0: 12345678__
-\= Expect no match
- 1234567
-No match
-
-/^[aeiou\d]{4,5}$/
- uoie
- 0: uoie
- 1234
- 0: 1234
- 12345
- 0: 12345
- aaaaa
- 0: aaaaa
-\= Expect no match
- 123456
-No match
-
-/^[aeiou\d]{4,5}?/
- uoie
- 0: uoie
- 1234
- 0: 1234
- 12345
- 0: 1234
- aaaaa
- 0: aaaa
- 123456
- 0: 1234
-
-/\A(abc|def)=(\1){2,3}\Z/
- abc=abcabc
- 0: abc=abcabc
- 1: abc
- 2: abc
- def=defdefdef
- 0: def=defdefdef
- 1: def
- 2: def
-\= Expect no match
- abc=defdef
-No match
-
-/^(a)(b)(c)(d)(e)(f)(g)(h)(i)(j)(k)\11*(\3\4)\1(?#)2$/
- abcdefghijkcda2
- 0: abcdefghijkcda2
- 1: a
- 2: b
- 3: c
- 4: d
- 5: e
- 6: f
- 7: g
- 8: h
- 9: i
-10: j
-11: k
-12: cd
- abcdefghijkkkkcda2
- 0: abcdefghijkkkkcda2
- 1: a
- 2: b
- 3: c
- 4: d
- 5: e
- 6: f
- 7: g
- 8: h
- 9: i
-10: j
-11: k
-12: cd
-
-/(cat(a(ract|tonic)|erpillar)) \1()2(3)/
- cataract cataract23
- 0: cataract cataract23
- 1: cataract
- 2: aract
- 3: ract
- 4:
- 5: 3
- catatonic catatonic23
- 0: catatonic catatonic23
- 1: catatonic
- 2: atonic
- 3: tonic
- 4:
- 5: 3
- caterpillar caterpillar23
- 0: caterpillar caterpillar23
- 1: caterpillar
- 2: erpillar
- 3:
- 4:
- 5: 3
-
-
-/^From +([^ ]+) +[a-zA-Z][a-zA-Z][a-zA-Z] +[a-zA-Z][a-zA-Z][a-zA-Z] +[0-9]?[0-9] +[0-9][0-9]:[0-9][0-9]/
- From abcd Mon Sep 01 12:33:02 1997
- 0: From abcd Mon Sep 01 12:33
- 1: abcd
-
-/^From\s+\S+\s+([a-zA-Z]{3}\s+){2}\d{1,2}\s+\d\d:\d\d/
- From abcd Mon Sep 01 12:33:02 1997
- 0: From abcd Mon Sep 01 12:33
- 1: Sep
- From abcd Mon Sep 1 12:33:02 1997
- 0: From abcd Mon Sep 1 12:33
- 1: Sep
-\= Expect no match
- From abcd Sep 01 12:33:02 1997
-No match
-
-/^12.34/s
- 12\n34
- 0: 12\x0a34
- 12\r34
- 0: 12\x0d34
-
-/\w+(?=\t)/
- the quick brown\t fox
- 0: brown
-
-/foo(?!bar)(.*)/
- foobar is foolish see?
- 0: foolish see?
- 1: lish see?
-
-/(?:(?!foo)...|^.{0,2})bar(.*)/
- foobar crowbar etc
- 0: rowbar etc
- 1: etc
- barrel
- 0: barrel
- 1: rel
- 2barrel
- 0: 2barrel
- 1: rel
- A barrel
- 0: A barrel
- 1: rel
-
-/^(\D*)(?=\d)(?!123)/
- abc456
- 0: abc
- 1: abc
-\= Expect no match
- abc123
-No match
-
-/^1234(?# test newlines
- inside)/
- 1234
- 0: 1234
-
-/^1234 #comment in extended re
- /x
- 1234
- 0: 1234
-
-/#rhubarb
- abcd/x
- abcd
- 0: abcd
-
-/^abcd#rhubarb/x
- abcd
- 0: abcd
-
-/^(a)\1{2,3}(.)/
- aaab
- 0: aaab
- 1: a
- 2: b
- aaaab
- 0: aaaab
- 1: a
- 2: b
- aaaaab
- 0: aaaaa
- 1: a
- 2: a
- aaaaaab
- 0: aaaaa
- 1: a
- 2: a
-
-/(?!^)abc/
- the abc
- 0: abc
-\= Expect no match
- abc
-No match
-
-/(?=^)abc/
- abc
- 0: abc
-\= Expect no match
- the abc
-No match
-
-/^[ab]{1,3}(ab*|b)/
- aabbbbb
- 0: aabb
- 1: b
-
-/^[ab]{1,3}?(ab*|b)/
- aabbbbb
- 0: aabbbbb
- 1: abbbbb
-
-/^[ab]{1,3}?(ab*?|b)/
- aabbbbb
- 0: aa
- 1: a
-
-/^[ab]{1,3}(ab*?|b)/
- aabbbbb
- 0: aabb
- 1: b
-
-/ (?: [\040\t] | \(
-(?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] | \( (?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] )* \) )*
-\) )* # optional leading comment
-(?: (?:
-[^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]+ # some number of atom characters...
-(?![^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]) # ..not followed by something that could be part of an atom
-|
-" (?: # opening quote...
-[^\\\x80-\xff\n\015"] # Anything except backslash and quote
-| # or
-\\ [^\x80-\xff] # Escaped something (something != CR)
-)* " # closing quote
-) # initial word
-(?: (?: [\040\t] | \(
-(?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] | \( (?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] )* \) )*
-\) )* \. (?: [\040\t] | \(
-(?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] | \( (?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] )* \) )*
-\) )* (?:
-[^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]+ # some number of atom characters...
-(?![^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]) # ..not followed by something that could be part of an atom
-|
-" (?: # opening quote...
-[^\\\x80-\xff\n\015"] # Anything except backslash and quote
-| # or
-\\ [^\x80-\xff] # Escaped something (something != CR)
-)* " # closing quote
-) )* # further okay, if led by a period
-(?: [\040\t] | \(
-(?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] | \( (?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] )* \) )*
-\) )* @ (?: [\040\t] | \(
-(?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] | \( (?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] )* \) )*
-\) )* (?:
-[^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]+ # some number of atom characters...
-(?![^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]) # ..not followed by something that could be part of an atom
-| \[ # [
-(?: [^\\\x80-\xff\n\015\[\]] | \\ [^\x80-\xff] )* # stuff
-\] # ]
-) # initial subdomain
-(?: #
-(?: [\040\t] | \(
-(?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] | \( (?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] )* \) )*
-\) )* \. # if led by a period...
-(?: [\040\t] | \(
-(?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] | \( (?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] )* \) )*
-\) )* (?:
-[^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]+ # some number of atom characters...
-(?![^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]) # ..not followed by something that could be part of an atom
-| \[ # [
-(?: [^\\\x80-\xff\n\015\[\]] | \\ [^\x80-\xff] )* # stuff
-\] # ]
-) # ...further okay
-)*
-# address
-| # or
-(?:
-[^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]+ # some number of atom characters...
-(?![^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]) # ..not followed by something that could be part of an atom
-|
-" (?: # opening quote...
-[^\\\x80-\xff\n\015"] # Anything except backslash and quote
-| # or
-\\ [^\x80-\xff] # Escaped something (something != CR)
-)* " # closing quote
-) # one word, optionally followed by....
-(?:
-[^()<>@,;:".\\\[\]\x80-\xff\000-\010\012-\037] | # atom and space parts, or...
-\(
-(?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] | \( (?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] )* \) )*
-\) | # comments, or...
-
-" (?: # opening quote...
-[^\\\x80-\xff\n\015"] # Anything except backslash and quote
-| # or
-\\ [^\x80-\xff] # Escaped something (something != CR)
-)* " # closing quote
-# quoted strings
-)*
-< (?: [\040\t] | \(
-(?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] | \( (?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] )* \) )*
-\) )* # leading <
-(?: @ (?: [\040\t] | \(
-(?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] | \( (?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] )* \) )*
-\) )* (?:
-[^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]+ # some number of atom characters...
-(?![^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]) # ..not followed by something that could be part of an atom
-| \[ # [
-(?: [^\\\x80-\xff\n\015\[\]] | \\ [^\x80-\xff] )* # stuff
-\] # ]
-) # initial subdomain
-(?: #
-(?: [\040\t] | \(
-(?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] | \( (?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] )* \) )*
-\) )* \. # if led by a period...
-(?: [\040\t] | \(
-(?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] | \( (?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] )* \) )*
-\) )* (?:
-[^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]+ # some number of atom characters...
-(?![^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]) # ..not followed by something that could be part of an atom
-| \[ # [
-(?: [^\\\x80-\xff\n\015\[\]] | \\ [^\x80-\xff] )* # stuff
-\] # ]
-) # ...further okay
-)*
-
-(?: (?: [\040\t] | \(
-(?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] | \( (?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] )* \) )*
-\) )* , (?: [\040\t] | \(
-(?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] | \( (?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] )* \) )*
-\) )* @ (?: [\040\t] | \(
-(?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] | \( (?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] )* \) )*
-\) )* (?:
-[^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]+ # some number of atom characters...
-(?![^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]) # ..not followed by something that could be part of an atom
-| \[ # [
-(?: [^\\\x80-\xff\n\015\[\]] | \\ [^\x80-\xff] )* # stuff
-\] # ]
-) # initial subdomain
-(?: #
-(?: [\040\t] | \(
-(?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] | \( (?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] )* \) )*
-\) )* \. # if led by a period...
-(?: [\040\t] | \(
-(?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] | \( (?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] )* \) )*
-\) )* (?:
-[^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]+ # some number of atom characters...
-(?![^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]) # ..not followed by something that could be part of an atom
-| \[ # [
-(?: [^\\\x80-\xff\n\015\[\]] | \\ [^\x80-\xff] )* # stuff
-\] # ]
-) # ...further okay
-)*
-)* # further okay, if led by comma
-: # closing colon
-(?: [\040\t] | \(
-(?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] | \( (?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] )* \) )*
-\) )* )? # optional route
-(?:
-[^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]+ # some number of atom characters...
-(?![^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]) # ..not followed by something that could be part of an atom
-|
-" (?: # opening quote...
-[^\\\x80-\xff\n\015"] # Anything except backslash and quote
-| # or
-\\ [^\x80-\xff] # Escaped something (something != CR)
-)* " # closing quote
-) # initial word
-(?: (?: [\040\t] | \(
-(?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] | \( (?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] )* \) )*
-\) )* \. (?: [\040\t] | \(
-(?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] | \( (?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] )* \) )*
-\) )* (?:
-[^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]+ # some number of atom characters...
-(?![^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]) # ..not followed by something that could be part of an atom
-|
-" (?: # opening quote...
-[^\\\x80-\xff\n\015"] # Anything except backslash and quote
-| # or
-\\ [^\x80-\xff] # Escaped something (something != CR)
-)* " # closing quote
-) )* # further okay, if led by a period
-(?: [\040\t] | \(
-(?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] | \( (?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] )* \) )*
-\) )* @ (?: [\040\t] | \(
-(?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] | \( (?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] )* \) )*
-\) )* (?:
-[^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]+ # some number of atom characters...
-(?![^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]) # ..not followed by something that could be part of an atom
-| \[ # [
-(?: [^\\\x80-\xff\n\015\[\]] | \\ [^\x80-\xff] )* # stuff
-\] # ]
-) # initial subdomain
-(?: #
-(?: [\040\t] | \(
-(?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] | \( (?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] )* \) )*
-\) )* \. # if led by a period...
-(?: [\040\t] | \(
-(?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] | \( (?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] )* \) )*
-\) )* (?:
-[^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]+ # some number of atom characters...
-(?![^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]) # ..not followed by something that could be part of an atom
-| \[ # [
-(?: [^\\\x80-\xff\n\015\[\]] | \\ [^\x80-\xff] )* # stuff
-\] # ]
-) # ...further okay
-)*
-# address spec
-(?: [\040\t] | \(
-(?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] | \( (?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] )* \) )*
-\) )* > # trailing >
-# name and address
-) (?: [\040\t] | \(
-(?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] | \( (?: [^\\\x80-\xff\n\015()] | \\ [^\x80-\xff] )* \) )*
-\) )* # optional trailing comment
-/x
- Alan Other
- 0: Alan Other
-
- 0: user@dom.ain
- user\@dom.ain
- 0: user@dom.ain
- \"A. Other\" (a comment)
- 0: "A. Other" (a comment)
- A. Other (a comment)
- 0: Other (a comment)
- \"/s=user/ou=host/o=place/prmd=uu.yy/admd= /c=gb/\"\@x400-re.lay
- 0: "/s=user/ou=host/o=place/prmd=uu.yy/admd= /c=gb/"@x400-re.lay
- A missing angle @,;:".\\\[\]\000-\037\x80-\xff]+ # some number of atom characters...
-(?![^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]) # ..not followed by something that could be part of an atom
-# Atom
-| # or
-" # "
-[^\\\x80-\xff\n\015"] * # normal
-(?: \\ [^\x80-\xff] [^\\\x80-\xff\n\015"] * )* # ( special normal* )*
-" # "
-# Quoted string
-)
-[\040\t]* # Nab whitespace.
-(?:
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: # (
-(?: \\ [^\x80-\xff] |
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: \\ [^\x80-\xff] [^\\\x80-\xff\n\015()] * )* # (special normal*)*
-\) # )
-) # special
-[^\\\x80-\xff\n\015()] * # normal*
-)* # )*
-\) # )
-[\040\t]* )* # If comment found, allow more spaces.
-(?:
-\.
-[\040\t]* # Nab whitespace.
-(?:
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: # (
-(?: \\ [^\x80-\xff] |
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: \\ [^\x80-\xff] [^\\\x80-\xff\n\015()] * )* # (special normal*)*
-\) # )
-) # special
-[^\\\x80-\xff\n\015()] * # normal*
-)* # )*
-\) # )
-[\040\t]* )* # If comment found, allow more spaces.
-(?:
-[^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]+ # some number of atom characters...
-(?![^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]) # ..not followed by something that could be part of an atom
-# Atom
-| # or
-" # "
-[^\\\x80-\xff\n\015"] * # normal
-(?: \\ [^\x80-\xff] [^\\\x80-\xff\n\015"] * )* # ( special normal* )*
-" # "
-# Quoted string
-)
-[\040\t]* # Nab whitespace.
-(?:
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: # (
-(?: \\ [^\x80-\xff] |
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: \\ [^\x80-\xff] [^\\\x80-\xff\n\015()] * )* # (special normal*)*
-\) # )
-) # special
-[^\\\x80-\xff\n\015()] * # normal*
-)* # )*
-\) # )
-[\040\t]* )* # If comment found, allow more spaces.
-# additional words
-)*
-@
-[\040\t]* # Nab whitespace.
-(?:
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: # (
-(?: \\ [^\x80-\xff] |
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: \\ [^\x80-\xff] [^\\\x80-\xff\n\015()] * )* # (special normal*)*
-\) # )
-) # special
-[^\\\x80-\xff\n\015()] * # normal*
-)* # )*
-\) # )
-[\040\t]* )* # If comment found, allow more spaces.
-(?:
-[^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]+ # some number of atom characters...
-(?![^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]) # ..not followed by something that could be part of an atom
-|
-\[ # [
-(?: [^\\\x80-\xff\n\015\[\]] | \\ [^\x80-\xff] )* # stuff
-\] # ]
-)
-[\040\t]* # Nab whitespace.
-(?:
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: # (
-(?: \\ [^\x80-\xff] |
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: \\ [^\x80-\xff] [^\\\x80-\xff\n\015()] * )* # (special normal*)*
-\) # )
-) # special
-[^\\\x80-\xff\n\015()] * # normal*
-)* # )*
-\) # )
-[\040\t]* )* # If comment found, allow more spaces.
-# optional trailing comments
-(?:
-\.
-[\040\t]* # Nab whitespace.
-(?:
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: # (
-(?: \\ [^\x80-\xff] |
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: \\ [^\x80-\xff] [^\\\x80-\xff\n\015()] * )* # (special normal*)*
-\) # )
-) # special
-[^\\\x80-\xff\n\015()] * # normal*
-)* # )*
-\) # )
-[\040\t]* )* # If comment found, allow more spaces.
-(?:
-[^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]+ # some number of atom characters...
-(?![^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]) # ..not followed by something that could be part of an atom
-|
-\[ # [
-(?: [^\\\x80-\xff\n\015\[\]] | \\ [^\x80-\xff] )* # stuff
-\] # ]
-)
-[\040\t]* # Nab whitespace.
-(?:
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: # (
-(?: \\ [^\x80-\xff] |
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: \\ [^\x80-\xff] [^\\\x80-\xff\n\015()] * )* # (special normal*)*
-\) # )
-) # special
-[^\\\x80-\xff\n\015()] * # normal*
-)* # )*
-\) # )
-[\040\t]* )* # If comment found, allow more spaces.
-# optional trailing comments
-)*
-# address
-| # or
-(?:
-[^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]+ # some number of atom characters...
-(?![^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]) # ..not followed by something that could be part of an atom
-# Atom
-| # or
-" # "
-[^\\\x80-\xff\n\015"] * # normal
-(?: \\ [^\x80-\xff] [^\\\x80-\xff\n\015"] * )* # ( special normal* )*
-" # "
-# Quoted string
-)
-# leading word
-[^()<>@,;:".\\\[\]\x80-\xff\000-\010\012-\037] * # "normal" atoms and or spaces
-(?:
-(?:
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: # (
-(?: \\ [^\x80-\xff] |
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: \\ [^\x80-\xff] [^\\\x80-\xff\n\015()] * )* # (special normal*)*
-\) # )
-) # special
-[^\\\x80-\xff\n\015()] * # normal*
-)* # )*
-\) # )
-|
-" # "
-[^\\\x80-\xff\n\015"] * # normal
-(?: \\ [^\x80-\xff] [^\\\x80-\xff\n\015"] * )* # ( special normal* )*
-" # "
-) # "special" comment or quoted string
-[^()<>@,;:".\\\[\]\x80-\xff\000-\010\012-\037] * # more "normal"
-)*
-<
-[\040\t]* # Nab whitespace.
-(?:
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: # (
-(?: \\ [^\x80-\xff] |
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: \\ [^\x80-\xff] [^\\\x80-\xff\n\015()] * )* # (special normal*)*
-\) # )
-) # special
-[^\\\x80-\xff\n\015()] * # normal*
-)* # )*
-\) # )
-[\040\t]* )* # If comment found, allow more spaces.
-# <
-(?:
-@
-[\040\t]* # Nab whitespace.
-(?:
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: # (
-(?: \\ [^\x80-\xff] |
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: \\ [^\x80-\xff] [^\\\x80-\xff\n\015()] * )* # (special normal*)*
-\) # )
-) # special
-[^\\\x80-\xff\n\015()] * # normal*
-)* # )*
-\) # )
-[\040\t]* )* # If comment found, allow more spaces.
-(?:
-[^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]+ # some number of atom characters...
-(?![^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]) # ..not followed by something that could be part of an atom
-|
-\[ # [
-(?: [^\\\x80-\xff\n\015\[\]] | \\ [^\x80-\xff] )* # stuff
-\] # ]
-)
-[\040\t]* # Nab whitespace.
-(?:
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: # (
-(?: \\ [^\x80-\xff] |
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: \\ [^\x80-\xff] [^\\\x80-\xff\n\015()] * )* # (special normal*)*
-\) # )
-) # special
-[^\\\x80-\xff\n\015()] * # normal*
-)* # )*
-\) # )
-[\040\t]* )* # If comment found, allow more spaces.
-# optional trailing comments
-(?:
-\.
-[\040\t]* # Nab whitespace.
-(?:
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: # (
-(?: \\ [^\x80-\xff] |
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: \\ [^\x80-\xff] [^\\\x80-\xff\n\015()] * )* # (special normal*)*
-\) # )
-) # special
-[^\\\x80-\xff\n\015()] * # normal*
-)* # )*
-\) # )
-[\040\t]* )* # If comment found, allow more spaces.
-(?:
-[^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]+ # some number of atom characters...
-(?![^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]) # ..not followed by something that could be part of an atom
-|
-\[ # [
-(?: [^\\\x80-\xff\n\015\[\]] | \\ [^\x80-\xff] )* # stuff
-\] # ]
-)
-[\040\t]* # Nab whitespace.
-(?:
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: # (
-(?: \\ [^\x80-\xff] |
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: \\ [^\x80-\xff] [^\\\x80-\xff\n\015()] * )* # (special normal*)*
-\) # )
-) # special
-[^\\\x80-\xff\n\015()] * # normal*
-)* # )*
-\) # )
-[\040\t]* )* # If comment found, allow more spaces.
-# optional trailing comments
-)*
-(?: ,
-[\040\t]* # Nab whitespace.
-(?:
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: # (
-(?: \\ [^\x80-\xff] |
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: \\ [^\x80-\xff] [^\\\x80-\xff\n\015()] * )* # (special normal*)*
-\) # )
-) # special
-[^\\\x80-\xff\n\015()] * # normal*
-)* # )*
-\) # )
-[\040\t]* )* # If comment found, allow more spaces.
-@
-[\040\t]* # Nab whitespace.
-(?:
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: # (
-(?: \\ [^\x80-\xff] |
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: \\ [^\x80-\xff] [^\\\x80-\xff\n\015()] * )* # (special normal*)*
-\) # )
-) # special
-[^\\\x80-\xff\n\015()] * # normal*
-)* # )*
-\) # )
-[\040\t]* )* # If comment found, allow more spaces.
-(?:
-[^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]+ # some number of atom characters...
-(?![^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]) # ..not followed by something that could be part of an atom
-|
-\[ # [
-(?: [^\\\x80-\xff\n\015\[\]] | \\ [^\x80-\xff] )* # stuff
-\] # ]
-)
-[\040\t]* # Nab whitespace.
-(?:
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: # (
-(?: \\ [^\x80-\xff] |
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: \\ [^\x80-\xff] [^\\\x80-\xff\n\015()] * )* # (special normal*)*
-\) # )
-) # special
-[^\\\x80-\xff\n\015()] * # normal*
-)* # )*
-\) # )
-[\040\t]* )* # If comment found, allow more spaces.
-# optional trailing comments
-(?:
-\.
-[\040\t]* # Nab whitespace.
-(?:
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: # (
-(?: \\ [^\x80-\xff] |
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: \\ [^\x80-\xff] [^\\\x80-\xff\n\015()] * )* # (special normal*)*
-\) # )
-) # special
-[^\\\x80-\xff\n\015()] * # normal*
-)* # )*
-\) # )
-[\040\t]* )* # If comment found, allow more spaces.
-(?:
-[^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]+ # some number of atom characters...
-(?![^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]) # ..not followed by something that could be part of an atom
-|
-\[ # [
-(?: [^\\\x80-\xff\n\015\[\]] | \\ [^\x80-\xff] )* # stuff
-\] # ]
-)
-[\040\t]* # Nab whitespace.
-(?:
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: # (
-(?: \\ [^\x80-\xff] |
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: \\ [^\x80-\xff] [^\\\x80-\xff\n\015()] * )* # (special normal*)*
-\) # )
-) # special
-[^\\\x80-\xff\n\015()] * # normal*
-)* # )*
-\) # )
-[\040\t]* )* # If comment found, allow more spaces.
-# optional trailing comments
-)*
-)* # additional domains
-:
-[\040\t]* # Nab whitespace.
-(?:
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: # (
-(?: \\ [^\x80-\xff] |
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: \\ [^\x80-\xff] [^\\\x80-\xff\n\015()] * )* # (special normal*)*
-\) # )
-) # special
-[^\\\x80-\xff\n\015()] * # normal*
-)* # )*
-\) # )
-[\040\t]* )* # If comment found, allow more spaces.
-# optional trailing comments
-)? # optional route
-(?:
-[^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]+ # some number of atom characters...
-(?![^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]) # ..not followed by something that could be part of an atom
-# Atom
-| # or
-" # "
-[^\\\x80-\xff\n\015"] * # normal
-(?: \\ [^\x80-\xff] [^\\\x80-\xff\n\015"] * )* # ( special normal* )*
-" # "
-# Quoted string
-)
-[\040\t]* # Nab whitespace.
-(?:
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: # (
-(?: \\ [^\x80-\xff] |
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: \\ [^\x80-\xff] [^\\\x80-\xff\n\015()] * )* # (special normal*)*
-\) # )
-) # special
-[^\\\x80-\xff\n\015()] * # normal*
-)* # )*
-\) # )
-[\040\t]* )* # If comment found, allow more spaces.
-(?:
-\.
-[\040\t]* # Nab whitespace.
-(?:
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: # (
-(?: \\ [^\x80-\xff] |
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: \\ [^\x80-\xff] [^\\\x80-\xff\n\015()] * )* # (special normal*)*
-\) # )
-) # special
-[^\\\x80-\xff\n\015()] * # normal*
-)* # )*
-\) # )
-[\040\t]* )* # If comment found, allow more spaces.
-(?:
-[^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]+ # some number of atom characters...
-(?![^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]) # ..not followed by something that could be part of an atom
-# Atom
-| # or
-" # "
-[^\\\x80-\xff\n\015"] * # normal
-(?: \\ [^\x80-\xff] [^\\\x80-\xff\n\015"] * )* # ( special normal* )*
-" # "
-# Quoted string
-)
-[\040\t]* # Nab whitespace.
-(?:
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: # (
-(?: \\ [^\x80-\xff] |
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: \\ [^\x80-\xff] [^\\\x80-\xff\n\015()] * )* # (special normal*)*
-\) # )
-) # special
-[^\\\x80-\xff\n\015()] * # normal*
-)* # )*
-\) # )
-[\040\t]* )* # If comment found, allow more spaces.
-# additional words
-)*
-@
-[\040\t]* # Nab whitespace.
-(?:
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: # (
-(?: \\ [^\x80-\xff] |
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: \\ [^\x80-\xff] [^\\\x80-\xff\n\015()] * )* # (special normal*)*
-\) # )
-) # special
-[^\\\x80-\xff\n\015()] * # normal*
-)* # )*
-\) # )
-[\040\t]* )* # If comment found, allow more spaces.
-(?:
-[^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]+ # some number of atom characters...
-(?![^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]) # ..not followed by something that could be part of an atom
-|
-\[ # [
-(?: [^\\\x80-\xff\n\015\[\]] | \\ [^\x80-\xff] )* # stuff
-\] # ]
-)
-[\040\t]* # Nab whitespace.
-(?:
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: # (
-(?: \\ [^\x80-\xff] |
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: \\ [^\x80-\xff] [^\\\x80-\xff\n\015()] * )* # (special normal*)*
-\) # )
-) # special
-[^\\\x80-\xff\n\015()] * # normal*
-)* # )*
-\) # )
-[\040\t]* )* # If comment found, allow more spaces.
-# optional trailing comments
-(?:
-\.
-[\040\t]* # Nab whitespace.
-(?:
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: # (
-(?: \\ [^\x80-\xff] |
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: \\ [^\x80-\xff] [^\\\x80-\xff\n\015()] * )* # (special normal*)*
-\) # )
-) # special
-[^\\\x80-\xff\n\015()] * # normal*
-)* # )*
-\) # )
-[\040\t]* )* # If comment found, allow more spaces.
-(?:
-[^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]+ # some number of atom characters...
-(?![^(\040)<>@,;:".\\\[\]\000-\037\x80-\xff]) # ..not followed by something that could be part of an atom
-|
-\[ # [
-(?: [^\\\x80-\xff\n\015\[\]] | \\ [^\x80-\xff] )* # stuff
-\] # ]
-)
-[\040\t]* # Nab whitespace.
-(?:
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: # (
-(?: \\ [^\x80-\xff] |
-\( # (
-[^\\\x80-\xff\n\015()] * # normal*
-(?: \\ [^\x80-\xff] [^\\\x80-\xff\n\015()] * )* # (special normal*)*
-\) # )
-) # special
-[^\\\x80-\xff\n\015()] * # normal*
-)* # )*
-\) # )
-[\040\t]* )* # If comment found, allow more spaces.
-# optional trailing comments
-)*
-# address spec
-> # >
-# name and address
-)
-/x
- Alan Other
- 0: Alan Other
-
- 0: user@dom.ain
- user\@dom.ain
- 0: user@dom.ain
- \"A. Other\" (a comment)
- 0: "A. Other"
- A. Other (a comment)
- 0: Other
- \"/s=user/ou=host/o=place/prmd=uu.yy/admd= /c=gb/\"\@x400-re.lay
- 0: "/s=user/ou=host/o=place/prmd=uu.yy/admd= /c=gb/"@x400-re.lay
- A missing angle ?@ABCDEFGHIJKLMNOPQRSTUVWXYZ[\]^_`abcdefghijklmnopqrstuvwxyz{|}~\x7f
-
-/P[^*]TAIRE[^*]{1,6}?LL/
- xxxxxxxxxxxPSTAIREISLLxxxxxxxxx
- 0: PSTAIREISLL
-
-/P[^*]TAIRE[^*]{1,}?LL/
- xxxxxxxxxxxPSTAIREISLLxxxxxxxxx
- 0: PSTAIREISLL
-
-/(\.\d\d[1-9]?)\d+/
- 1.230003938
- 0: .230003938
- 1: .23
- 1.875000282
- 0: .875000282
- 1: .875
- 1.235
- 0: .235
- 1: .23
-
-/(\.\d\d((?=0)|\d(?=\d)))/
- 1.230003938
- 0: .23
- 1: .23
- 2:
- 1.875000282
- 0: .875
- 1: .875
- 2: 5
-\= Expect no match
- 1.235
-No match
-
-/\b(foo)\s+(\w+)/i
- Food is on the foo table
- 0: foo table
- 1: foo
- 2: table
-
-/foo(.*)bar/
- The food is under the bar in the barn.
- 0: food is under the bar in the bar
- 1: d is under the bar in the
-
-/foo(.*?)bar/
- The food is under the bar in the barn.
- 0: food is under the bar
- 1: d is under the
-
-/(.*)(\d*)/
- I have 2 numbers: 53147
- 0: I have 2 numbers: 53147
- 1: I have 2 numbers: 53147
- 2:
-
-/(.*)(\d+)/
- I have 2 numbers: 53147
- 0: I have 2 numbers: 53147
- 1: I have 2 numbers: 5314
- 2: 7
-
-/(.*?)(\d*)/
- I have 2 numbers: 53147
- 0:
- 1:
- 2:
-
-/(.*?)(\d+)/
- I have 2 numbers: 53147
- 0: I have 2
- 1: I have
- 2: 2
-
-/(.*)(\d+)$/
- I have 2 numbers: 53147
- 0: I have 2 numbers: 53147
- 1: I have 2 numbers: 5314
- 2: 7
-
-/(.*?)(\d+)$/
- I have 2 numbers: 53147
- 0: I have 2 numbers: 53147
- 1: I have 2 numbers:
- 2: 53147
-
-/(.*)\b(\d+)$/
- I have 2 numbers: 53147
- 0: I have 2 numbers: 53147
- 1: I have 2 numbers:
- 2: 53147
-
-/(.*\D)(\d+)$/
- I have 2 numbers: 53147
- 0: I have 2 numbers: 53147
- 1: I have 2 numbers:
- 2: 53147
-
-/^\D*(?!123)/
- ABC123
- 0: AB
-
-/^(\D*)(?=\d)(?!123)/
- ABC445
- 0: ABC
- 1: ABC
-\= Expect no match
- ABC123
-No match
-
-/^[W-]46]/
- W46]789
- 0: W46]
- -46]789
- 0: -46]
-\= Expect no match
- Wall
-No match
- Zebra
-No match
- 42
-No match
- [abcd]
-No match
- ]abcd[
-No match
-
-/^[W-\]46]/
- W46]789
- 0: W
- Wall
- 0: W
- Zebra
- 0: Z
- Xylophone
- 0: X
- 42
- 0: 4
- [abcd]
- 0: [
- ]abcd[
- 0: ]
- \\backslash
- 0: \
-\= Expect no match
- -46]789
-No match
- well
-No match
-
-/\d\d\/\d\d\/\d\d\d\d/
- 01/01/2000
- 0: 01/01/2000
-
-/word (?:[a-zA-Z0-9]+ ){0,10}otherword/
- word cat dog elephant mussel cow horse canary baboon snake shark otherword
- 0: word cat dog elephant mussel cow horse canary baboon snake shark otherword
-\= Expect no match
- word cat dog elephant mussel cow horse canary baboon snake shark
-No match
-
-/word (?:[a-zA-Z0-9]+ ){0,300}otherword/
-\= Expect no match
- word cat dog elephant mussel cow horse canary baboon snake shark the quick brown fox and the lazy dog and several other words getting close to thirty by now I hope
-No match
-
-/^(a){0,0}/
- bcd
- 0:
- abc
- 0:
- aab
- 0:
-
-/^(a){0,1}/
- bcd
- 0:
- abc
- 0: a
- 1: a
- aab
- 0: a
- 1: a
-
-/^(a){0,2}/
- bcd
- 0:
- abc
- 0: a
- 1: a
- aab
- 0: aa
- 1: a
-
-/^(a){0,3}/
- bcd
- 0:
- abc
- 0: a
- 1: a
- aab
- 0: aa
- 1: a
- aaa
- 0: aaa
- 1: a
-
-/^(a){0,}/
- bcd
- 0:
- abc
- 0: a
- 1: a
- aab
- 0: aa
- 1: a
- aaa
- 0: aaa
- 1: a
- aaaaaaaa
- 0: aaaaaaaa
- 1: a
-
-/^(a){1,1}/
- abc
- 0: a
- 1: a
- aab
- 0: a
- 1: a
-\= Expect no match
- bcd
-No match
-
-/^(a){1,2}/
- abc
- 0: a
- 1: a
- aab
- 0: aa
- 1: a
-\= Expect no match
- bcd
-No match
-
-/^(a){1,3}/
- abc
- 0: a
- 1: a
- aab
- 0: aa
- 1: a
- aaa
- 0: aaa
- 1: a
-\= Expect no match
- bcd
-No match
-
-/^(a){1,}/
- abc
- 0: a
- 1: a
- aab
- 0: aa
- 1: a
- aaa
- 0: aaa
- 1: a
- aaaaaaaa
- 0: aaaaaaaa
- 1: a
-\= Expect no match
- bcd
-No match
-
-/.*\.gif/
- borfle\nbib.gif\nno
- 0: bib.gif
-
-/.{0,}\.gif/
- borfle\nbib.gif\nno
- 0: bib.gif
-
-/.*\.gif/m
- borfle\nbib.gif\nno
- 0: bib.gif
-
-/.*\.gif/s
- borfle\nbib.gif\nno
- 0: borfle\x0abib.gif
-
-/.*\.gif/ms
- borfle\nbib.gif\nno
- 0: borfle\x0abib.gif
-
-/.*$/
- borfle\nbib.gif\nno
- 0: no
-
-/.*$/m
- borfle\nbib.gif\nno
- 0: borfle
-
-/.*$/s
- borfle\nbib.gif\nno
- 0: borfle\x0abib.gif\x0ano
-
-/.*$/ms
- borfle\nbib.gif\nno
- 0: borfle\x0abib.gif\x0ano
-
-/.*$/
- borfle\nbib.gif\nno\n
- 0: no
-
-/.*$/m
- borfle\nbib.gif\nno\n
- 0: borfle
-
-/.*$/s
- borfle\nbib.gif\nno\n
- 0: borfle\x0abib.gif\x0ano\x0a
-
-/.*$/ms
- borfle\nbib.gif\nno\n
- 0: borfle\x0abib.gif\x0ano\x0a
-
-/(.*X|^B)/
- abcde\n1234Xyz
- 0: 1234X
- 1: 1234X
- BarFoo
- 0: B
- 1: B
-\= Expect no match
- abcde\nBar
-No match
-
-/(.*X|^B)/m
- abcde\n1234Xyz
- 0: 1234X
- 1: 1234X
- BarFoo
- 0: B
- 1: B
- abcde\nBar
- 0: B
- 1: B
-
-/(.*X|^B)/s
- abcde\n1234Xyz
- 0: abcde\x0a1234X
- 1: abcde\x0a1234X
- BarFoo
- 0: B
- 1: B
-\= Expect no match
- abcde\nBar
-No match
-
-/(.*X|^B)/ms
- abcde\n1234Xyz
- 0: abcde\x0a1234X
- 1: abcde\x0a1234X
- BarFoo
- 0: B
- 1: B
- abcde\nBar
- 0: B
- 1: B
-
-/(?s)(.*X|^B)/
- abcde\n1234Xyz
- 0: abcde\x0a1234X
- 1: abcde\x0a1234X
- BarFoo
- 0: B
- 1: B
-\= Expect no match
- abcde\nBar
-No match
-
-/(?s:.*X|^B)/
- abcde\n1234Xyz
- 0: abcde\x0a1234X
- BarFoo
- 0: B
-\= Expect no match
- abcde\nBar
-No match
-
-/^.*B/
-\= Expect no match
- abc\nB
-No match
-
-/(?s)^.*B/
- abc\nB
- 0: abc\x0aB
-
-/(?m)^.*B/
- abc\nB
- 0: B
-
-/(?ms)^.*B/
- abc\nB
- 0: abc\x0aB
-
-/(?ms)^B/
- abc\nB
- 0: B
-
-/(?s)B$/
- B\n
- 0: B
-
-/^[0-9][0-9][0-9][0-9][0-9][0-9][0-9][0-9][0-9][0-9][0-9][0-9]/
- 123456654321
- 0: 123456654321
-
-/^\d\d\d\d\d\d\d\d\d\d\d\d/
- 123456654321
- 0: 123456654321
-
-/^[\d][\d][\d][\d][\d][\d][\d][\d][\d][\d][\d][\d]/
- 123456654321
- 0: 123456654321
-
-/^[abc]{12}/
- abcabcabcabc
- 0: abcabcabcabc
-
-/^[a-c]{12}/
- abcabcabcabc
- 0: abcabcabcabc
-
-/^(a|b|c){12}/
- abcabcabcabc
- 0: abcabcabcabc
- 1: c
-
-/^[abcdefghijklmnopqrstuvwxy0123456789]/
- n
- 0: n
-\= Expect no match
- z
-No match
-
-/abcde{0,0}/
- abcd
- 0: abcd
-\= Expect no match
- abce
-No match
-
-/ab[cd]{0,0}e/
- abe
- 0: abe
-\= Expect no match
- abcde
-No match
-
-/ab(c){0,0}d/
- abd
- 0: abd
-\= Expect no match
- abcd
-No match
-
-/a(b*)/
- a
- 0: a
- 1:
- ab
- 0: ab
- 1: b
- abbbb
- 0: abbbb
- 1: bbbb
-\= Expect no match
- bbbbb
-No match
-
-/ab\d{0}e/
- abe
- 0: abe
-\= Expect no match
- ab1e
-No match
-
-/"([^\\"]+|\\.)*"/
- the \"quick\" brown fox
- 0: "quick"
- 1: quick
- \"the \\\"quick\\\" brown fox\"
- 0: "the \"quick\" brown fox"
- 1: brown fox
-
-/]{0,})>]{0,})>([\d]{0,}\.)(.*)((
([\w\W\s\d][^<>]{0,})|[\s]{0,}))<\/a><\/TD> ]{0,})>([\w\W\s\d][^<>]{0,})<\/TD> ]{0,})>([\w\W\s\d][^<>]{0,})<\/TD><\/TR>/is
- 43.Word Processor
(N-1286) Lega lstaff.com CA - Statewide
- 0: 43.Word Processor
(N-1286) Lega lstaff.com CA - Statewide
- 1: BGCOLOR='#DBE9E9'
- 2: align=left valign=top
- 3: 43.
- 4: Word Processor
(N-1286)
- 5:
- 6:
- 7:
- 8: align=left valign=top
- 9: Lega lstaff.com
-10: align=left valign=top
-11: CA - Statewide
-
-/a[^a]b/
- acb
- 0: acb
- a\nb
- 0: a\x0ab
-
-/a.b/
- acb
- 0: acb
-\= Expect no match
- a\nb
-No match
-
-/a[^a]b/s
- acb
- 0: acb
- a\nb
- 0: a\x0ab
-
-/a.b/s
- acb
- 0: acb
- a\nb
- 0: a\x0ab
-
-/^(b+?|a){1,2}?c/
- bac
- 0: bac
- 1: a
- bbac
- 0: bbac
- 1: a
- bbbac
- 0: bbbac
- 1: a
- bbbbac
- 0: bbbbac
- 1: a
- bbbbbac
- 0: bbbbbac
- 1: a
-
-/^(b+|a){1,2}?c/
- bac
- 0: bac
- 1: a
- bbac
- 0: bbac
- 1: a
- bbbac
- 0: bbbac
- 1: a
- bbbbac
- 0: bbbbac
- 1: a
- bbbbbac
- 0: bbbbbac
- 1: a
-
-/(?!\A)x/m
- a\bx\n
- 0: x
- a\nx\n
- 0: x
-\= Expect no match
- x\nb\n
-No match
-
-/(A|B)*?CD/
- CD
- 0: CD
-
-/(A|B)*CD/
- CD
- 0: CD
-
-/(AB)*?\1/
- ABABAB
- 0: ABAB
- 1: AB
-
-/(AB)*\1/
- ABABAB
- 0: ABABAB
- 1: AB
-
-/(?.*/)foo"
- /this/is/a/very/long/line/in/deed/with/very/many/slashes/in/and/foo
- 0: /this/is/a/very/long/line/in/deed/with/very/many/slashes/in/and/foo
-\= Expect no match
- /this/is/a/very/long/line/in/deed/with/very/many/slashes/in/it/you/see/
-No match
-
-/(?>(\.\d\d[1-9]?))\d+/
- 1.230003938
- 0: .230003938
- 1: .23
- 1.875000282
- 0: .875000282
- 1: .875
-\= Expect no match
- 1.235
-No match
-
-/^((?>\w+)|(?>\s+))*$/
- now is the time for all good men to come to the aid of the party
- 0: now is the time for all good men to come to the aid of the party
- 1: party
-\= Expect no match
- this is not a line with only words and spaces!
-No match
-
-/(\d+)(\w)/
- 12345a
- 0: 12345a
- 1: 12345
- 2: a
- 12345+
- 0: 12345
- 1: 1234
- 2: 5
-
-/((?>\d+))(\w)/
- 12345a
- 0: 12345a
- 1: 12345
- 2: a
-\= Expect no match
- 12345+
-No match
-
-/(?>a+)b/
- aaab
- 0: aaab
-
-/((?>a+)b)/
- aaab
- 0: aaab
- 1: aaab
-
-/(?>(a+))b/
- aaab
- 0: aaab
- 1: aaa
-
-/(?>b)+/
- aaabbbccc
- 0: bbb
-
-/(?>a+|b+|c+)*c/
- aaabbbbccccd
- 0: aaabbbbc
-
-/((?>[^()]+)|\([^()]*\))+/
- ((abc(ade)ufh()()x
- 0: abc(ade)ufh()()x
- 1: x
-
-/\(((?>[^()]+)|\([^()]+\))+\)/
- (abc)
- 0: (abc)
- 1: abc
- (abc(def)xyz)
- 0: (abc(def)xyz)
- 1: xyz
-\= Expect no match
- ((()aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa
-No match
-
-/a(?-i)b/i
- ab
- 0: ab
- Ab
- 0: Ab
-\= Expect no match
- aB
-No match
- AB
-No match
-
-/(a (?x)b c)d e/
- a bcd e
- 0: a bcd e
- 1: a bc
-\= Expect no match
- a b cd e
-No match
- abcd e
-No match
- a bcde
-No match
-
-/(a b(?x)c d (?-x)e f)/
- a bcde f
- 0: a bcde f
- 1: a bcde f
-\= Expect no match
- abcdef
-No match
-
-/(a(?i)b)c/
- abc
- 0: abc
- 1: ab
- aBc
- 0: aBc
- 1: aB
-\= Expect no match
- abC
-No match
- aBC
-No match
- Abc
-No match
- ABc
-No match
- ABC
-No match
- AbC
-No match
-
-/a(?i:b)c/
- abc
- 0: abc
- aBc
- 0: aBc
-\= Expect no match
- ABC
-No match
- abC
-No match
- aBC
-No match
-
-/a(?i:b)*c/
- aBc
- 0: aBc
- aBBc
- 0: aBBc
-\= Expect no match
- aBC
-No match
- aBBC
-No match
-
-/a(?=b(?i)c)\w\wd/
- abcd
- 0: abcd
- abCd
- 0: abCd
-\= Expect no match
- aBCd
-No match
- abcD
-No match
-
-/(?s-i:more.*than).*million/i
- more than million
- 0: more than million
- more than MILLION
- 0: more than MILLION
- more \n than Million
- 0: more \x0a than Million
-\= Expect no match
- MORE THAN MILLION
-No match
- more \n than \n million
-No match
-
-/(?:(?s-i)more.*than).*million/i
- more than million
- 0: more than million
- more than MILLION
- 0: more than MILLION
- more \n than Million
- 0: more \x0a than Million
-\= Expect no match
- MORE THAN MILLION
-No match
- more \n than \n million
-No match
-
-/(?>a(?i)b+)+c/
- abc
- 0: abc
- aBbc
- 0: aBbc
- aBBc
- 0: aBBc
-\= Expect no match
- Abc
-No match
- abAb
-No match
- abbC
-No match
-
-/(?=a(?i)b)\w\wc/
- abc
- 0: abc
- aBc
- 0: aBc
-\= Expect no match
- Ab
-No match
- abC
-No match
- aBC
-No match
-
-/(?<=a(?i)b)(\w\w)c/
- abxxc
- 0: xxc
- 1: xx
- aBxxc
- 0: xxc
- 1: xx
-\= Expect no match
- Abxxc
-No match
- ABxxc
-No match
- abxxC
-No match
-
-/(?:(a)|b)(?(1)A|B)/
- aA
- 0: aA
- 1: a
- bB
- 0: bB
-\= Expect no match
- aB
-No match
- bA
-No match
-
-/^(a)?(?(1)a|b)+$/
- aa
- 0: aa
- 1: a
- b
- 0: b
- bb
- 0: bb
-\= Expect no match
- ab
-No match
-
-# Perl gets this next one wrong if the pattern ends with $; in that case it
-# fails to match "12".
-
-/^(?(?=abc)\w{3}:|\d\d)/
- abc:
- 0: abc:
- 12
- 0: 12
- 123
- 0: 12
-\= Expect no match
- xyz
-No match
-
-/^(?(?!abc)\d\d|\w{3}:)$/
- abc:
- 0: abc:
- 12
- 0: 12
-\= Expect no match
- 123
-No match
- xyz
-No match
-
-/(?(?<=foo)bar|cat)/
- foobar
- 0: bar
- cat
- 0: cat
- fcat
- 0: cat
- focat
- 0: cat
-\= Expect no match
- foocat
-No match
-
-/(?(?a*)*/
- a
- 0: a
- aa
- 0: aa
- aaaa
- 0: aaaa
-
-/(abc|)+/
- abc
- 0: abc
- 1:
- abcabc
- 0: abcabc
- 1:
- abcabcabc
- 0: abcabcabc
- 1:
- xyz
- 0:
- 1:
-
-/([a]*)*/
- a
- 0: a
- 1:
- aaaaa
- 0: aaaaa
- 1:
-
-/([ab]*)*/
- a
- 0: a
- 1:
- b
- 0: b
- 1:
- ababab
- 0: ababab
- 1:
- aaaabcde
- 0: aaaab
- 1:
- bbbb
- 0: bbbb
- 1:
-
-/([^a]*)*/
- b
- 0: b
- 1:
- bbbb
- 0: bbbb
- 1:
- aaa
- 0:
- 1:
-
-/([^ab]*)*/
- cccc
- 0: cccc
- 1:
- abab
- 0:
- 1:
-
-/([a]*?)*/
- a
- 0:
- 1:
- aaaa
- 0:
- 1:
-
-/([ab]*?)*/
- a
- 0:
- 1:
- b
- 0:
- 1:
- abab
- 0:
- 1:
- baba
- 0:
- 1:
-
-/([^a]*?)*/
- b
- 0:
- 1:
- bbbb
- 0:
- 1:
- aaa
- 0:
- 1:
-
-/([^ab]*?)*/
- c
- 0:
- 1:
- cccc
- 0:
- 1:
- baba
- 0:
- 1:
-
-/(?>a*)*/
- a
- 0: a
- aaabcde
- 0: aaa
-
-/((?>a*))*/
- aaaaa
- 0: aaaaa
- 1:
- aabbaa
- 0: aa
- 1:
-
-/((?>a*?))*/
- aaaaa
- 0:
- 1:
- aabbaa
- 0:
- 1:
-
-/(?(?=[^a-z]+[a-z]) \d{2}-[a-z]{3}-\d{2} | \d{2}-\d{2}-\d{2} ) /x
- 12-sep-98
- 0: 12-sep-98
- 12-09-98
- 0: 12-09-98
-\= Expect no match
- sep-12-98
-No match
-
-/(?<=(foo))bar\1/
- foobarfoo
- 0: barfoo
- 1: foo
- foobarfootling
- 0: barfoo
- 1: foo
-\= Expect no match
- foobar
-No match
- barfoo
-No match
-
-/(?i:saturday|sunday)/
- saturday
- 0: saturday
- sunday
- 0: sunday
- Saturday
- 0: Saturday
- Sunday
- 0: Sunday
- SATURDAY
- 0: SATURDAY
- SUNDAY
- 0: SUNDAY
- SunDay
- 0: SunDay
-
-/(a(?i)bc|BB)x/
- abcx
- 0: abcx
- 1: abc
- aBCx
- 0: aBCx
- 1: aBC
- bbx
- 0: bbx
- 1: bb
- BBx
- 0: BBx
- 1: BB
-\= Expect no match
- abcX
-No match
- aBCX
-No match
- bbX
-No match
- BBX
-No match
-
-/^([ab](?i)[cd]|[ef])/
- ac
- 0: ac
- 1: ac
- aC
- 0: aC
- 1: aC
- bD
- 0: bD
- 1: bD
- elephant
- 0: e
- 1: e
- Europe
- 0: E
- 1: E
- frog
- 0: f
- 1: f
- France
- 0: F
- 1: F
-\= Expect no match
- Africa
-No match
-
-/^(ab|a(?i)[b-c](?m-i)d|x(?i)y|z)/
- ab
- 0: ab
- 1: ab
- aBd
- 0: aBd
- 1: aBd
- xy
- 0: xy
- 1: xy
- xY
- 0: xY
- 1: xY
- zebra
- 0: z
- 1: z
- Zambesi
- 0: Z
- 1: Z
-\= Expect no match
- aCD
-No match
- XY
-No match
-
-/(?<=foo\n)^bar/m
- foo\nbar
- 0: bar
-\= Expect no match
- bar
-No match
- baz\nbar
-No match
-
-/(?<=(?]&/
- <&OUT
- 0: <&
-
-/^(a\1?){4}$/
- aaaaaaaaaa
- 0: aaaaaaaaaa
- 1: aaaa
-\= Expect no match
- AB
-No match
- aaaaaaaaa
-No match
- aaaaaaaaaaa
-No match
-
-/^(a(?(1)\1)){4}$/
- aaaaaaaaaa
- 0: aaaaaaaaaa
- 1: aaaa
-\= Expect no match
- aaaaaaaaa
-No match
- aaaaaaaaaaa
-No match
-
-/(?:(f)(o)(o)|(b)(a)(r))*/
- foobar
- 0: foobar
- 1: f
- 2: o
- 3: o
- 4: b
- 5: a
- 6: r
-
-/(?<=a)b/
- ab
- 0: b
-\= Expect no match
- cb
-No match
- b
-No match
-
-/(?
- 2: abcd
- xy:z:::abcd
- 0: xy:z:::abcd
- 1: xy:z:::
- 2: abcd
-
-/^[^bcd]*(c+)/
- aexycd
- 0: aexyc
- 1: c
-
-/(a*)b+/
- caab
- 0: aab
- 1: aa
-
-/([\w:]+::)?(\w+)$/
- abcd
- 0: abcd
- 1:
- 2: abcd
- xy:z:::abcd
- 0: xy:z:::abcd
- 1: xy:z:::
- 2: abcd
-\= Expect no match
- abcd:
-No match
- abcd:
-No match
-
-/^[^bcd]*(c+)/
- aexycd
- 0: aexyc
- 1: c
-
-/(>a+)ab/
-
-/(?>a+)b/
- aaab
- 0: aaab
-
-/([[:]+)/
- a:[b]:
- 0: :[
- 1: :[
-
-/([[=]+)/
- a=[b]=
- 0: =[
- 1: =[
-
-/([[.]+)/
- a.[b].
- 0: .[
- 1: .[
-
-/((?>a+)b)/
- aaab
- 0: aaab
- 1: aaab
-
-/(?>(a+))b/
- aaab
- 0: aaab
- 1: aaa
-
-/((?>[^()]+)|\([^()]*\))+/
- ((abc(ade)ufh()()x
- 0: abc(ade)ufh()()x
- 1: x
-
-/a\Z/
-\= Expect no match
- aaab
-No match
- a\nb\n
-No match
-
-/b\Z/
- a\nb\n
- 0: b
-
-/b\z/
-
-/b\Z/
- a\nb
- 0: b
-
-/b\z/
- a\nb
- 0: b
-
-/^(?>(?(1)\.|())[^\W_](?>[a-z0-9-]*[^\W_])?)+$/
- a
- 0: a
- 1:
- abc
- 0: abc
- 1:
- a-b
- 0: a-b
- 1:
- 0-9
- 0: 0-9
- 1:
- a.b
- 0: a.b
- 1:
- 5.6.7
- 0: 5.6.7
- 1:
- the.quick.brown.fox
- 0: the.quick.brown.fox
- 1:
- a100.b200.300c
- 0: a100.b200.300c
- 1:
- 12-ab.1245
- 0: 12-ab.1245
- 1:
-\= Expect no match
- \
-No match
- .a
-No match
- -a
-No match
- a-
-No match
- a.
-No match
- a_b
-No match
- a.-
-No match
- a..
-No match
- ab..bc
-No match
- the.quick.brown.fox-
-No match
- the.quick.brown.fox.
-No match
- the.quick.brown.fox_
-No match
- the.quick.brown.fox+
-No match
-
-/(?>.*)(?<=(abcd|wxyz))/
- alphabetabcd
- 0: alphabetabcd
- 1: abcd
- endingwxyz
- 0: endingwxyz
- 1: wxyz
-\= Expect no match
- a rather long string that doesn't end with one of them
-No match
-
-/word (?>(?:(?!otherword)[a-zA-Z0-9]+ ){0,30})otherword/
- word cat dog elephant mussel cow horse canary baboon snake shark otherword
- 0: word cat dog elephant mussel cow horse canary baboon snake shark otherword
-\= Expect no match
- word cat dog elephant mussel cow horse canary baboon snake shark
-No match
-
-/word (?>[a-zA-Z0-9]+ ){0,30}otherword/
-\= Expect no match
- word cat dog elephant mussel cow horse canary baboon snake shark the quick brown fox and the lazy dog and several other words getting close to thirty by now I hope
-No match
-
-/(?<=\d{3}(?!999))foo/
- 999foo
- 0: foo
- 123999foo
- 0: foo
-\= Expect no match
- 123abcfoo
-No match
-
-/(?<=(?!...999)\d{3})foo/
- 999foo
- 0: foo
- 123999foo
- 0: foo
-\= Expect no match
- 123abcfoo
-No match
-
-/(?<=\d{3}(?!999)...)foo/
- 123abcfoo
- 0: foo
- 123456foo
- 0: foo
-\= Expect no match
- 123999foo
-No match
-
-/(?<=\d{3}...)(?
- 2:
- 3: abcd
-
- 2:
- 3: abcd
- \s*)=(?>\s*) # find
- 2:
- 3: abcd
- Z)+|A)*/
- ZABCDEFG
- 0: ZA
- 1: A
-
-/((?>)+|A)*/
- ZABCDEFG
- 0:
- 1:
-
-/^[\d-a]/
- abcde
- 0: a
- -things
- 0: -
- 0digit
- 0: 0
-\= Expect no match
- bcdef
-No match
-
-/[\s]+/
- > \x09\x0a\x0c\x0d\x0b<
- 0: \x09\x0a\x0c\x0d\x0b
-
-/\s+/
- > \x09\x0a\x0c\x0d\x0b<
- 0: \x09\x0a\x0c\x0d\x0b
-
-/ab/x
- ab
- 0: ab
-
-/(?!\A)x/m
- a\nxb\n
- 0: x
-
-/(?!^)x/m
-\= Expect no match
- a\nxb\n
-No match
-
-#/abc\Qabc\Eabc/
-# abcabcabc
-# 0: abcabcabc
-
-#/abc\Q(*+|\Eabc/
-# abc(*+|abc
-# 0: abc(*+|abc
-
-#/ abc\Q abc\Eabc/x
-# abc abcabc
-# 0: abc abcabc
-#\= Expect no match
-# abcabcabc
-#No match
-
-#/abc#comment
-# \Q#not comment
-# literal\E/x
-# abc#not comment\n literal
-# 0: abc#not comment\x0a literal
-
-#/abc#comment
-# \Q#not comment
-# literal/x
-# abc#not comment\n literal
-# 0: abc#not comment\x0a literal
-
-#/abc#comment
-# \Q#not comment
-# literal\E #more comment
-# /x
-# abc#not comment\n literal
-# 0: abc#not comment\x0a literal
-
-#/abc#comment
-# \Q#not comment
-# literal\E #more comment/x
-# abc#not comment\n literal
-# 0: abc#not comment\x0a literal
-
-#/\Qabc\$xyz\E/
-# abc\\\$xyz
-# 0: abc\$xyz
-
-#/\Qabc\E\$\Qxyz\E/
-# abc\$xyz
-# 0: abc$xyz
-
-/\Gabc/
- abc
- 0: abc
-\= Expect no match
- xyzabc
-No match
-
-/a(?x: b c )d/
- XabcdY
- 0: abcd
-\= Expect no match
- Xa b c d Y
-No match
-
-/((?x)x y z | a b c)/
- XabcY
- 0: abc
- 1: abc
- AxyzB
- 0: xyz
- 1: xyz
-
-/(?i)AB(?-i)C/
- XabCY
- 0: abC
-\= Expect no match
- XabcY
-No match
-
-/((?i)AB(?-i)C|D)E/
- abCE
- 0: abCE
- 1: abC
- DE
- 0: DE
- 1: D
-\= Expect no match
- abcE
-No match
- abCe
-No match
- dE
-No match
- De
-No match
-
-/(.*)\d+\1/
- abc123abc
- 0: abc123abc
- 1: abc
- abc123bc
- 0: bc123bc
- 1: bc
-
-/(.*)\d+\1/s
- abc123abc
- 0: abc123abc
- 1: abc
- abc123bc
- 0: bc123bc
- 1: bc
-
-/((.*))\d+\1/
- abc123abc
- 0: abc123abc
- 1: abc
- 2: abc
- abc123bc
- 0: bc123bc
- 1: bc
- 2: bc
-
-# This tests for an IPv6 address in the form where it can have up to
-# eight components, one and only one of which is empty. This must be
-# an internal component.
-
-/^(?!:) # colon disallowed at start
- (?: # start of item
- (?: [0-9a-f]{1,4} | # 1-4 hex digits or
- (?(1)0 | () ) ) # if null previously matched, fail; else null
- : # followed by colon
- ){1,7} # end item; 1-7 of them required
- [0-9a-f]{1,4} $ # final hex number at end of string
- (?(1)|.) # check that there was an empty component
- /ix
- a123::a123
- 0: a123::a123
- 1:
- a123:b342::abcd
- 0: a123:b342::abcd
- 1:
- a123:b342::324e:abcd
- 0: a123:b342::324e:abcd
- 1:
- a123:ddde:b342::324e:abcd
- 0: a123:ddde:b342::324e:abcd
- 1:
- a123:ddde:b342::324e:dcba:abcd
- 0: a123:ddde:b342::324e:dcba:abcd
- 1:
- a123:ddde:9999:b342::324e:dcba:abcd
- 0: a123:ddde:9999:b342::324e:dcba:abcd
- 1:
-\= Expect no match
- 1:2:3:4:5:6:7:8
-No match
- a123:bce:ddde:9999:b342::324e:dcba:abcd
-No match
- a123::9999:b342::324e:dcba:abcd
-No match
- abcde:2:3:4:5:6:7:8
-No match
- ::1
-No match
- abcd:fee0:123::
-No match
- :1
-No match
- 1:
-No match
-
-#/[z\Qa-d]\E]/
-# z
-# 0: z
-# a
-# 0: a
-# -
-# 0: -
-# d
-# 0: d
-# ]
-# 0: ]
-#\= Expect no match
-# b
-#No match
-
-#TODO: PCRE has an optimization to make this workable, .NET does not
-#/(a+)*b/
-#\= Expect no match
-# aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa
-#No match
-
-# All these had to be updated because we understand unicode
-# and this looks like it's expecting single byte matches
-
-# .NET generates \xe4...not sure what's up, might just be different code pages
-/(?i)reg(?:ul(?:[aä]|ae)r|ex)/
- REGular
- 0: REGular
- regulaer
- 0: regulaer
- Regex
- 0: Regex
- regulär
- 0: regul\xc3\xa4r
-
-#/Åæåä[à-ÿÀ-ß]+/
-# Åæåäà
-# 0: \xc5\xe6\xe5\xe4\xe0
-# Åæåäÿ
-# 0: \xc5\xe6\xe5\xe4\xff
-# ÅæåäÀ
-# 0: \xc5\xe6\xe5\xe4\xc0
-# Åæåäß
-# 0: \xc5\xe6\xe5\xe4\xdf
-
-/(?<=Z)X./
- \x84XAZXB
- 0: XB
-
-/ab cd (?x) de fg/
- ab cd defg
- 0: ab cd defg
-
-/ab cd(?x) de fg/
- ab cddefg
- 0: ab cddefg
-\= Expect no match
- abcddefg
-No match
-
-/(?
- 2:
- D
- 0: D
- 1:
- 2:
-
-# this is really long with debug -- removing for now
-#/(a|)*\d/
-# aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa4
-# 0: aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa4
-# 1:
-#\= Expect no match
-# aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa
-#No match
-
-/(?>a|)*\d/
- aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa4
- 0: aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa4
-\= Expect no match
- aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa
-No match
-
-/(?:a|)*\d/
- aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa4
- 0: aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa4
-\= Expect no match
- aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa
-No match
-
-/^(?s)(?>.*)(?
- 2: a
-
-/(?>(a))b|(a)c/
- ac
- 0: ac
- 1:
- 2: a
-
-/(?=(a))ab|(a)c/
- ac
- 0: ac
- 1:
- 2: a
-
-/((?>(a))b|(a)c)/
- ac
- 0: ac
- 1: ac
- 2:
- 3: a
-
-/(?=(?>(a))b|(a)c)(..)/
- ac
- 0: ac
- 1:
- 2: a
- 3: ac
-
-/(?>(?>(a))b|(a)c)/
- ac
- 0: ac
- 1:
- 2: a
-
-/((?>(a+)b)+(aabab))/
- aaaabaaabaabab
- 0: aaaabaaabaabab
- 1: aaaabaaabaabab
- 2: aaa
- 3: aabab
-
-/(?>a+|ab)+?c/
-\= Expect no match
- aabc
-No match
-
-/(?>a+|ab)+c/
-\= Expect no match
- aabc
-No match
-
-/(?:a+|ab)+c/
- aabc
- 0: aabc
-
-/^(?:a|ab)+c/
- aaaabc
- 0: aaaabc
-
-/(?=abc){0}xyz/
- xyz
- 0: xyz
-
-/(?=abc){1}xyz/
-\= Expect no match
- xyz
-No match
-
-/(?=(a))?./
- ab
- 0: a
- 1: a
- bc
- 0: b
-
-/(?=(a))??./
- ab
- 0: a
- bc
- 0: b
-
-/^(?!a){0}\w+/
- aaaaa
- 0: aaaaa
-
-/(?<=(abc))?xyz/
- abcxyz
- 0: xyz
- 1: abc
- pqrxyz
- 0: xyz
-
-/^[g]+/
- ggg<<>>
- 0: ggg<<>>
-\= Expect no match
- \\ga
-No match
-
-/^[ga]+/
- gggagagaxyz
- 0: gggagaga
-
-/[:a]xxx[b:]/
- :xxx:
- 0: :xxx:
-
-/(?<=a{2})b/i
- xaabc
- 0: b
-\= Expect no match
- xabc
-No match
-
-/(?
-# 4:
-# 5: c
-# 6: d
-# 7: Y
-
-#/^X(?7)(a)(?|(b|(?|(r)|(t))(s))|(q))(c)(d)(Y)/
-# XYabcdY
-# 0: XYabcdY
-# 1: a
-# 2: b
-# 3:
-# 4:
-# 5: c
-# 6: d
-# 7: Y
-
-/(?'abc'\w+):\k{2}/
- a:aaxyz
- 0: a:aa
- 1: a
- ab:ababxyz
- 0: ab:abab
- 1: ab
-\= Expect no match
- a:axyz
-No match
- ab:abxyz
-No match
-
-/^(?a)? (?(ab)b|c) (?(ab)d|e)/x
- abd
- 0: abd
- 1: a
- ce
- 0: ce
-
-# .NET has more consistent grouping numbers with these dupe groups for the two options
-/(?:a(? (?')|(?")) |b(? (?')|(?")) ) (?(quote)[a-z]+|[0-9]+)/x,dupnames
- a\"aaaaa
- 0: a"aaaaa
- 1: "
- 2:
- 3: "
- b\"aaaaa
- 0: b"aaaaa
- 1: "
- 2:
- 3: "
-\= Expect no match
- b\"11111
-No match
-
-#/(?P(?P0)(?P>L1)|(?P>L2))/
-# 0
-# 0: 0
-# 1: 0
-# 00
-# 0: 00
-# 1: 00
-# 2: 0
-# 0000
-# 0: 0000
-# 1: 0000
-# 2: 0
-
-#/(?P(?P0)|(?P>L2)(?P>L1))/
-# 0
-# 0: 0
-# 1: 0
-# 2: 0
-# 00
-# 0: 0
-# 1: 0
-# 2: 0
-# 0000
-# 0: 0
-# 1: 0
-# 2: 0
-
-# Check the use of names for failure
-
-# Check opening parens in comment when seeking forward reference.
-
-#/(?P(?P=abn)xxx|)+/
-# xxx
-# 0:
-# 1:
-
-#Posses
-/^(a)?(\w)/
- aaaaX
- 0: aa
- 1: a
- 2: a
- YZ
- 0: Y
- 1:
- 2: Y
-
-#Posses
-/^(?:a)?(\w)/
- aaaaX
- 0: aa
- 1: a
- YZ
- 0: Y
- 1: Y
-
-/\A.*?(a|bc)/
- ba
- 0: ba
- 1: a
-
-/\A.*?(?:a|bc|d)/
- ba
- 0: ba
-
-# --------------------------
-
-/(another)?(\1?)test/
- hello world test
- 0: test
- 1:
- 2:
-
-/(another)?(\1+)test/
-\= Expect no match
- hello world test
-No match
-
-/((?:a?)*)*c/
- aac
- 0: aac
- 1:
-
-/((?>a?)*)*c/
- aac
- 0: aac
- 1:
-
-/(?>.*?a)(?<=ba)/
- aba
- 0: ba
-
-/(?:.*?a)(?<=ba)/
- aba
- 0: aba
-
-/(?>.*?a)b/s
- aab
- 0: ab
-
-/(?>.*?a)b/
- aab
- 0: ab
-
-/(?>^a)b/s
-\= Expect no match
- aab
-No match
-
-/(?>.*?)(?<=(abcd)|(wxyz))/
- alphabetabcd
- 0:
- 1: abcd
- endingwxyz
- 0:
- 1:
- 2: wxyz
-
-/(?>.*)(?<=(abcd)|(wxyz))/
- alphabetabcd
- 0: alphabetabcd
- 1: abcd
- endingwxyz
- 0: endingwxyz
- 1:
- 2: wxyz
-
-"(?>.*)foo"
-\= Expect no match
- abcdfooxyz
-No match
-
-"(?>.*?)foo"
- abcdfooxyz
- 0: foo
-
-# Tests that try to figure out how Perl works. My hypothesis is that the first
-# verb that is backtracked onto is the one that acts. This seems to be the case
-# almost all the time, but there is one exception that is perhaps a bug.
-
-/a(?=bc).|abd/
- abd
- 0: abd
- abc
- 0: ab
-
-/a(?>bc)d|abd/
- abceabd
- 0: abd
-
-# These tests were formerly in test 2, but changes in PCRE and Perl have
-# made them compatible.
-
-/^(a)?(?(1)a|b)+$/
-\= Expect no match
- a
-No match
-
-# ----
-
-/^\d*\w{4}/
- 1234
- 0: 1234
-\= Expect no match
- 123
-No match
-
-/^[^b]*\w{4}/
- aaaa
- 0: aaaa
-\= Expect no match
- aaa
-No match
-
-/^[^b]*\w{4}/i
- aaaa
- 0: aaaa
-\= Expect no match
- aaa
-No match
-
-/^a*\w{4}/
- aaaa
- 0: aaaa
-\= Expect no match
- aaa
-No match
-
-/^a*\w{4}/i
- aaaa
- 0: aaaa
-\= Expect no match
- aaa
-No match
-
-/(?:(?foo)|(?bar))\k/dupnames
- foofoo
- 0: foofoo
- 1: foo
- barbar
- 0: barbar
- 1: bar
-
-# A notable difference between PCRE and .NET. According to
-# the PCRE docs:
-# If you make a subroutine call to a non-unique named
-# subpattern, the one that corresponds to the first
-# occurrence of the name is used. In the absence of
-# duplicate numbers (see the previous section) this is
-# the one with the lowest number.
-# .NET takes the most recently captured number according to MSDN:
-# A backreference refers to the most recent definition of
-# a group (the definition most immediately to the left,
-# when matching left to right). When a group makes multiple
-# captures, a backreference refers to the most recent capture.
-
-#/(?A)(?:(?foo)|(?bar))\k/dupnames
-# AfooA
-# 0: AfooA
-# 1: A
-# 2: foo
-# AbarA
-# 0: AbarA
-# 1: A
-# 2:
-# 3: bar
-#\= Expect no match
-# Afoofoo
-#No match
-# Abarbar
-#No match
-
-/^(\d+)\s+IN\s+SOA\s+(\S+)\s+(\S+)\s*\(\s*$/
- 1 IN SOA non-sp1 non-sp2(
- 0: 1 IN SOA non-sp1 non-sp2(
- 1: 1
- 2: non-sp1
- 3: non-sp2
-
-# TODO: .NET's group number ordering here in the second example is a bit odd
-/^ (?:(?A)|(?'B'B)(?A)) (?(A)x) (?(B)y)$/x,dupnames
- Ax
- 0: Ax
- 1: A
- BAxy
- 0: BAxy
- 1: A
- 2: B
-
-/ ^ a + b $ /x
- aaaab
- 0: aaaab
-
-/ ^ a + #comment
- b $ /x
- aaaab
- 0: aaaab
-
-/ ^ a + #comment
- #comment
- b $ /x
- aaaab
- 0: aaaab
-
-/ ^ (?> a + ) b $ /x
- aaaab
- 0: aaaab
-
-/ ^ ( a + ) + \w $ /x
- aaaab
- 0: aaaab
- 1: aaaa
-
-/(?:x|(?:(xx|yy)+|x|x|x|x|x)|a|a|a)bc/
-\= Expect no match
- acb
-No match
-
-#Posses
-#/\A(?:[^\"]+|\"(?:[^\"]*|\"\")*\")+/
-# NON QUOTED \"QUOT\"\"ED\" AFTER \"NOT MATCHED
-# 0: NON QUOTED "QUOT""ED" AFTER
-
-#Posses
-#/\A(?:[^\"]+|\"(?:[^\"]+|\"\")*\")+/
-# NON QUOTED \"QUOT\"\"ED\" AFTER \"NOT MATCHED
-# 0: NON QUOTED "QUOT""ED" AFTER
-
-#Posses
-#/\A(?:[^\"]+|\"(?:[^\"]+|\"\")+\")+/
-# NON QUOTED \"QUOT\"\"ED\" AFTER \"NOT MATCHED
-# 0: NON QUOTED "QUOT""ED" AFTER
-
-#Posses
-#/\A([^\"1]+|[\"2]([^\"3]*|[\"4][\"5])*[\"6])+/
-# NON QUOTED \"QUOT\"\"ED\" AFTER \"NOT MATCHED
-# 0: NON QUOTED "QUOT""ED" AFTER
-# 1: AFTER
-# 2:
-
-/^\w+(?>\s*)(?<=\w)/
- test test
- 0: tes
-
-#/(?Pa)?(?Pb)?(?()c|d)*l/
-# acl
-# 0: acl
-# 1: a
-# bdl
-# 0: bdl
-# 1:
-# 2: b
-# adl
-# 0: dl
-# bcl
-# 0: l
-
-/\sabc/
- \x0babc
- 0: \x0babc
-
-#/[\Qa]\E]+/
-# aa]]
-# 0: aa]]
-
-#/[\Q]a\E]+/
-# aa]]
-# 0: aa]]
-
-/A((((((((a))))))))\8B/
- AaaB
- 0: AaaB
- 1: a
- 2: a
- 3: a
- 4: a
- 5: a
- 6: a
- 7: a
- 8: a
-
-/A(((((((((a)))))))))\9B/
- AaaB
- 0: AaaB
- 1: a
- 2: a
- 3: a
- 4: a
- 5: a
- 6: a
- 7: a
- 8: a
- 9: a
-
-/(|ab)*?d/
- abd
- 0: abd
- 1: ab
- xyd
- 0: d
-
-/(\2|a)(\1)/
- aaa
- 0: aa
- 1: a
- 2: a
-
-/(\2)(\1)/
-
-"Z*(|d*){216}"
-
-/((((((((((((x))))))))))))\12/
- xx
- 0: xx
- 1: x
- 2: x
- 3: x
- 4: x
- 5: x
- 6: x
- 7: x
- 8: x
- 9: x
-10: x
-11: x
-12: x
-
-#"(?|(\k'Pm')|(?'Pm'))"
-# abcd
-# 0:
-# 1:
-
-#/(?|(aaa)|(b))\g{1}/
-# aaaaaa
-# 0: aaaaaa
-# 1: aaa
-# bb
-# 0: bb
-# 1: b
-
-#/(?|(aaa)|(b))(?1)/
-# aaaaaa
-# 0: aaaaaa
-# 1: aaa
-# baaa
-# 0: baaa
-# 1: b
-#\= Expect no match
-# bb
-#No match
-
-#/(?|(aaa)|(b))/
-# xaaa
-# 0: aaa
-# 1: aaa
-# xbc
-# 0: b
-# 1: b
-
-#/(?|(?'a'aaa)|(?'a'b))\k'a'/
-# aaaaaa
-# 0: aaaaaa
-# 1: aaa
-# bb
-# 0: bb
-# 1: b
-
-#/(?|(?'a'aaa)|(?'a'b))(?'a'cccc)\k'a'/dupnames
-# aaaccccaaa
-# 0: aaaccccaaa
-# 1: aaa
-# 2: cccc
-# bccccb
-# 0: bccccb
-# 1: b
-# 2: cccc
-
-# End of testinput1
diff --git a/vendor/github.com/emirpasic/gods/LICENSE b/vendor/github.com/emirpasic/gods/LICENSE
deleted file mode 100644
index e5e449b..0000000
--- a/vendor/github.com/emirpasic/gods/LICENSE
+++ /dev/null
@@ -1,41 +0,0 @@
-Copyright (c) 2015, Emir Pasic
-All rights reserved.
-
-Redistribution and use in source and binary forms, with or without
-modification, are permitted provided that the following conditions are met:
-
-* Redistributions of source code must retain the above copyright notice, this
- list of conditions and the following disclaimer.
-
-* Redistributions in binary form must reproduce the above copyright notice,
- this list of conditions and the following disclaimer in the documentation
- and/or other materials provided with the distribution.
-
-THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
-AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
-IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
-DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE
-FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
-DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
-SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
-CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY,
-OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
-OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
-
--------------------------------------------------------------------------------
-
-AVL Tree:
-
-Copyright (c) 2017 Benjamin Scher Purcell
-
-Permission to use, copy, modify, and distribute this software for any
-purpose with or without fee is hereby granted, provided that the above
-copyright notice and this permission notice appear in all copies.
-
-THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES
-WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF
-MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR
-ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
-WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN
-ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF
-OR IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
diff --git a/vendor/github.com/emirpasic/gods/containers/containers.go b/vendor/github.com/emirpasic/gods/containers/containers.go
deleted file mode 100644
index c35ab36..0000000
--- a/vendor/github.com/emirpasic/gods/containers/containers.go
+++ /dev/null
@@ -1,35 +0,0 @@
-// Copyright (c) 2015, Emir Pasic. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-// Package containers provides core interfaces and functions for data structures.
-//
-// Container is the base interface for all data structures to implement.
-//
-// Iterators provide stateful iterators.
-//
-// Enumerable provides Ruby inspired (each, select, map, find, any?, etc.) container functions.
-//
-// Serialization provides serializers (marshalers) and deserializers (unmarshalers).
-package containers
-
-import "github.com/emirpasic/gods/utils"
-
-// Container is base interface that all data structures implement.
-type Container interface {
- Empty() bool
- Size() int
- Clear()
- Values() []interface{}
-}
-
-// GetSortedValues returns sorted container's elements with respect to the passed comparator.
-// Does not effect the ordering of elements within the container.
-func GetSortedValues(container Container, comparator utils.Comparator) []interface{} {
- values := container.Values()
- if len(values) < 2 {
- return values
- }
- utils.Sort(values, comparator)
- return values
-}
diff --git a/vendor/github.com/emirpasic/gods/containers/enumerable.go b/vendor/github.com/emirpasic/gods/containers/enumerable.go
deleted file mode 100644
index ac48b54..0000000
--- a/vendor/github.com/emirpasic/gods/containers/enumerable.go
+++ /dev/null
@@ -1,61 +0,0 @@
-// Copyright (c) 2015, Emir Pasic. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package containers
-
-// EnumerableWithIndex provides functions for ordered containers whose values can be fetched by an index.
-type EnumerableWithIndex interface {
- // Each calls the given function once for each element, passing that element's index and value.
- Each(func(index int, value interface{}))
-
- // Map invokes the given function once for each element and returns a
- // container containing the values returned by the given function.
- // TODO would appreciate help on how to enforce this in containers (don't want to type assert when chaining)
- // Map(func(index int, value interface{}) interface{}) Container
-
- // Select returns a new container containing all elements for which the given function returns a true value.
- // TODO need help on how to enforce this in containers (don't want to type assert when chaining)
- // Select(func(index int, value interface{}) bool) Container
-
- // Any passes each element of the container to the given function and
- // returns true if the function ever returns true for any element.
- Any(func(index int, value interface{}) bool) bool
-
- // All passes each element of the container to the given function and
- // returns true if the function returns true for all elements.
- All(func(index int, value interface{}) bool) bool
-
- // Find passes each element of the container to the given function and returns
- // the first (index,value) for which the function is true or -1,nil otherwise
- // if no element matches the criteria.
- Find(func(index int, value interface{}) bool) (int, interface{})
-}
-
-// EnumerableWithKey provides functions for ordered containers whose values whose elements are key/value pairs.
-type EnumerableWithKey interface {
- // Each calls the given function once for each element, passing that element's key and value.
- Each(func(key interface{}, value interface{}))
-
- // Map invokes the given function once for each element and returns a container
- // containing the values returned by the given function as key/value pairs.
- // TODO need help on how to enforce this in containers (don't want to type assert when chaining)
- // Map(func(key interface{}, value interface{}) (interface{}, interface{})) Container
-
- // Select returns a new container containing all elements for which the given function returns a true value.
- // TODO need help on how to enforce this in containers (don't want to type assert when chaining)
- // Select(func(key interface{}, value interface{}) bool) Container
-
- // Any passes each element of the container to the given function and
- // returns true if the function ever returns true for any element.
- Any(func(key interface{}, value interface{}) bool) bool
-
- // All passes each element of the container to the given function and
- // returns true if the function returns true for all elements.
- All(func(key interface{}, value interface{}) bool) bool
-
- // Find passes each element of the container to the given function and returns
- // the first (key,value) for which the function is true or nil,nil otherwise if no element
- // matches the criteria.
- Find(func(key interface{}, value interface{}) bool) (interface{}, interface{})
-}
diff --git a/vendor/github.com/emirpasic/gods/containers/iterator.go b/vendor/github.com/emirpasic/gods/containers/iterator.go
deleted file mode 100644
index f1a52a3..0000000
--- a/vendor/github.com/emirpasic/gods/containers/iterator.go
+++ /dev/null
@@ -1,109 +0,0 @@
-// Copyright (c) 2015, Emir Pasic. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package containers
-
-// IteratorWithIndex is stateful iterator for ordered containers whose values can be fetched by an index.
-type IteratorWithIndex interface {
- // Next moves the iterator to the next element and returns true if there was a next element in the container.
- // If Next() returns true, then next element's index and value can be retrieved by Index() and Value().
- // If Next() was called for the first time, then it will point the iterator to the first element if it exists.
- // Modifies the state of the iterator.
- Next() bool
-
- // Value returns the current element's value.
- // Does not modify the state of the iterator.
- Value() interface{}
-
- // Index returns the current element's index.
- // Does not modify the state of the iterator.
- Index() int
-
- // Begin resets the iterator to its initial state (one-before-first)
- // Call Next() to fetch the first element if any.
- Begin()
-
- // First moves the iterator to the first element and returns true if there was a first element in the container.
- // If First() returns true, then first element's index and value can be retrieved by Index() and Value().
- // Modifies the state of the iterator.
- First() bool
-}
-
-// IteratorWithKey is a stateful iterator for ordered containers whose elements are key value pairs.
-type IteratorWithKey interface {
- // Next moves the iterator to the next element and returns true if there was a next element in the container.
- // If Next() returns true, then next element's key and value can be retrieved by Key() and Value().
- // If Next() was called for the first time, then it will point the iterator to the first element if it exists.
- // Modifies the state of the iterator.
- Next() bool
-
- // Value returns the current element's value.
- // Does not modify the state of the iterator.
- Value() interface{}
-
- // Key returns the current element's key.
- // Does not modify the state of the iterator.
- Key() interface{}
-
- // Begin resets the iterator to its initial state (one-before-first)
- // Call Next() to fetch the first element if any.
- Begin()
-
- // First moves the iterator to the first element and returns true if there was a first element in the container.
- // If First() returns true, then first element's key and value can be retrieved by Key() and Value().
- // Modifies the state of the iterator.
- First() bool
-}
-
-// ReverseIteratorWithIndex is stateful iterator for ordered containers whose values can be fetched by an index.
-//
-// Essentially it is the same as IteratorWithIndex, but provides additional:
-//
-// Prev() function to enable traversal in reverse
-//
-// Last() function to move the iterator to the last element.
-//
-// End() function to move the iterator past the last element (one-past-the-end).
-type ReverseIteratorWithIndex interface {
- // Prev moves the iterator to the previous element and returns true if there was a previous element in the container.
- // If Prev() returns true, then previous element's index and value can be retrieved by Index() and Value().
- // Modifies the state of the iterator.
- Prev() bool
-
- // End moves the iterator past the last element (one-past-the-end).
- // Call Prev() to fetch the last element if any.
- End()
-
- // Last moves the iterator to the last element and returns true if there was a last element in the container.
- // If Last() returns true, then last element's index and value can be retrieved by Index() and Value().
- // Modifies the state of the iterator.
- Last() bool
-
- IteratorWithIndex
-}
-
-// ReverseIteratorWithKey is a stateful iterator for ordered containers whose elements are key value pairs.
-//
-// Essentially it is the same as IteratorWithKey, but provides additional:
-//
-// Prev() function to enable traversal in reverse
-//
-// Last() function to move the iterator to the last element.
-type ReverseIteratorWithKey interface {
- // Prev moves the iterator to the previous element and returns true if there was a previous element in the container.
- // If Prev() returns true, then previous element's key and value can be retrieved by Key() and Value().
- // Modifies the state of the iterator.
- Prev() bool
-
- // End moves the iterator past the last element (one-past-the-end).
- // Call Prev() to fetch the last element if any.
- End()
-
- // Last moves the iterator to the last element and returns true if there was a last element in the container.
- // If Last() returns true, then last element's key and value can be retrieved by Key() and Value().
- // Modifies the state of the iterator.
- Last() bool
-
- IteratorWithKey
-}
diff --git a/vendor/github.com/emirpasic/gods/containers/serialization.go b/vendor/github.com/emirpasic/gods/containers/serialization.go
deleted file mode 100644
index d7c90c8..0000000
--- a/vendor/github.com/emirpasic/gods/containers/serialization.go
+++ /dev/null
@@ -1,17 +0,0 @@
-// Copyright (c) 2015, Emir Pasic. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package containers
-
-// JSONSerializer provides JSON serialization
-type JSONSerializer interface {
- // ToJSON outputs the JSON representation of containers's elements.
- ToJSON() ([]byte, error)
-}
-
-// JSONDeserializer provides JSON deserialization
-type JSONDeserializer interface {
- // FromJSON populates containers's elements from the input JSON representation.
- FromJSON([]byte) error
-}
diff --git a/vendor/github.com/emirpasic/gods/lists/arraylist/arraylist.go b/vendor/github.com/emirpasic/gods/lists/arraylist/arraylist.go
deleted file mode 100644
index bfedac9..0000000
--- a/vendor/github.com/emirpasic/gods/lists/arraylist/arraylist.go
+++ /dev/null
@@ -1,228 +0,0 @@
-// Copyright (c) 2015, Emir Pasic. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-// Package arraylist implements the array list.
-//
-// Structure is not thread safe.
-//
-// Reference: https://en.wikipedia.org/wiki/List_%28abstract_data_type%29
-package arraylist
-
-import (
- "fmt"
- "strings"
-
- "github.com/emirpasic/gods/lists"
- "github.com/emirpasic/gods/utils"
-)
-
-func assertListImplementation() {
- var _ lists.List = (*List)(nil)
-}
-
-// List holds the elements in a slice
-type List struct {
- elements []interface{}
- size int
-}
-
-const (
- growthFactor = float32(2.0) // growth by 100%
- shrinkFactor = float32(0.25) // shrink when size is 25% of capacity (0 means never shrink)
-)
-
-// New instantiates a new list and adds the passed values, if any, to the list
-func New(values ...interface{}) *List {
- list := &List{}
- if len(values) > 0 {
- list.Add(values...)
- }
- return list
-}
-
-// Add appends a value at the end of the list
-func (list *List) Add(values ...interface{}) {
- list.growBy(len(values))
- for _, value := range values {
- list.elements[list.size] = value
- list.size++
- }
-}
-
-// Get returns the element at index.
-// Second return parameter is true if index is within bounds of the array and array is not empty, otherwise false.
-func (list *List) Get(index int) (interface{}, bool) {
-
- if !list.withinRange(index) {
- return nil, false
- }
-
- return list.elements[index], true
-}
-
-// Remove removes the element at the given index from the list.
-func (list *List) Remove(index int) {
-
- if !list.withinRange(index) {
- return
- }
-
- list.elements[index] = nil // cleanup reference
- copy(list.elements[index:], list.elements[index+1:list.size]) // shift to the left by one (slow operation, need ways to optimize this)
- list.size--
-
- list.shrink()
-}
-
-// Contains checks if elements (one or more) are present in the set.
-// All elements have to be present in the set for the method to return true.
-// Performance time complexity of n^2.
-// Returns true if no arguments are passed at all, i.e. set is always super-set of empty set.
-func (list *List) Contains(values ...interface{}) bool {
-
- for _, searchValue := range values {
- found := false
- for _, element := range list.elements {
- if element == searchValue {
- found = true
- break
- }
- }
- if !found {
- return false
- }
- }
- return true
-}
-
-// Values returns all elements in the list.
-func (list *List) Values() []interface{} {
- newElements := make([]interface{}, list.size, list.size)
- copy(newElements, list.elements[:list.size])
- return newElements
-}
-
-//IndexOf returns index of provided element
-func (list *List) IndexOf(value interface{}) int {
- if list.size == 0 {
- return -1
- }
- for index, element := range list.elements {
- if element == value {
- return index
- }
- }
- return -1
-}
-
-// Empty returns true if list does not contain any elements.
-func (list *List) Empty() bool {
- return list.size == 0
-}
-
-// Size returns number of elements within the list.
-func (list *List) Size() int {
- return list.size
-}
-
-// Clear removes all elements from the list.
-func (list *List) Clear() {
- list.size = 0
- list.elements = []interface{}{}
-}
-
-// Sort sorts values (in-place) using.
-func (list *List) Sort(comparator utils.Comparator) {
- if len(list.elements) < 2 {
- return
- }
- utils.Sort(list.elements[:list.size], comparator)
-}
-
-// Swap swaps the two values at the specified positions.
-func (list *List) Swap(i, j int) {
- if list.withinRange(i) && list.withinRange(j) {
- list.elements[i], list.elements[j] = list.elements[j], list.elements[i]
- }
-}
-
-// Insert inserts values at specified index position shifting the value at that position (if any) and any subsequent elements to the right.
-// Does not do anything if position is negative or bigger than list's size
-// Note: position equal to list's size is valid, i.e. append.
-func (list *List) Insert(index int, values ...interface{}) {
-
- if !list.withinRange(index) {
- // Append
- if index == list.size {
- list.Add(values...)
- }
- return
- }
-
- l := len(values)
- list.growBy(l)
- list.size += l
- copy(list.elements[index+l:], list.elements[index:list.size-l])
- copy(list.elements[index:], values)
-}
-
-// Set the value at specified index
-// Does not do anything if position is negative or bigger than list's size
-// Note: position equal to list's size is valid, i.e. append.
-func (list *List) Set(index int, value interface{}) {
-
- if !list.withinRange(index) {
- // Append
- if index == list.size {
- list.Add(value)
- }
- return
- }
-
- list.elements[index] = value
-}
-
-// String returns a string representation of container
-func (list *List) String() string {
- str := "ArrayList\n"
- values := []string{}
- for _, value := range list.elements[:list.size] {
- values = append(values, fmt.Sprintf("%v", value))
- }
- str += strings.Join(values, ", ")
- return str
-}
-
-// Check that the index is within bounds of the list
-func (list *List) withinRange(index int) bool {
- return index >= 0 && index < list.size
-}
-
-func (list *List) resize(cap int) {
- newElements := make([]interface{}, cap, cap)
- copy(newElements, list.elements)
- list.elements = newElements
-}
-
-// Expand the array if necessary, i.e. capacity will be reached if we add n elements
-func (list *List) growBy(n int) {
- // When capacity is reached, grow by a factor of growthFactor and add number of elements
- currentCapacity := cap(list.elements)
- if list.size+n >= currentCapacity {
- newCapacity := int(growthFactor * float32(currentCapacity+n))
- list.resize(newCapacity)
- }
-}
-
-// Shrink the array if necessary, i.e. when size is shrinkFactor percent of current capacity
-func (list *List) shrink() {
- if shrinkFactor == 0.0 {
- return
- }
- // Shrink when size is at shrinkFactor * capacity
- currentCapacity := cap(list.elements)
- if list.size <= int(float32(currentCapacity)*shrinkFactor) {
- list.resize(list.size)
- }
-}
diff --git a/vendor/github.com/emirpasic/gods/lists/arraylist/enumerable.go b/vendor/github.com/emirpasic/gods/lists/arraylist/enumerable.go
deleted file mode 100644
index b3a8738..0000000
--- a/vendor/github.com/emirpasic/gods/lists/arraylist/enumerable.go
+++ /dev/null
@@ -1,79 +0,0 @@
-// Copyright (c) 2015, Emir Pasic. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package arraylist
-
-import "github.com/emirpasic/gods/containers"
-
-func assertEnumerableImplementation() {
- var _ containers.EnumerableWithIndex = (*List)(nil)
-}
-
-// Each calls the given function once for each element, passing that element's index and value.
-func (list *List) Each(f func(index int, value interface{})) {
- iterator := list.Iterator()
- for iterator.Next() {
- f(iterator.Index(), iterator.Value())
- }
-}
-
-// Map invokes the given function once for each element and returns a
-// container containing the values returned by the given function.
-func (list *List) Map(f func(index int, value interface{}) interface{}) *List {
- newList := &List{}
- iterator := list.Iterator()
- for iterator.Next() {
- newList.Add(f(iterator.Index(), iterator.Value()))
- }
- return newList
-}
-
-// Select returns a new container containing all elements for which the given function returns a true value.
-func (list *List) Select(f func(index int, value interface{}) bool) *List {
- newList := &List{}
- iterator := list.Iterator()
- for iterator.Next() {
- if f(iterator.Index(), iterator.Value()) {
- newList.Add(iterator.Value())
- }
- }
- return newList
-}
-
-// Any passes each element of the collection to the given function and
-// returns true if the function ever returns true for any element.
-func (list *List) Any(f func(index int, value interface{}) bool) bool {
- iterator := list.Iterator()
- for iterator.Next() {
- if f(iterator.Index(), iterator.Value()) {
- return true
- }
- }
- return false
-}
-
-// All passes each element of the collection to the given function and
-// returns true if the function returns true for all elements.
-func (list *List) All(f func(index int, value interface{}) bool) bool {
- iterator := list.Iterator()
- for iterator.Next() {
- if !f(iterator.Index(), iterator.Value()) {
- return false
- }
- }
- return true
-}
-
-// Find passes each element of the container to the given function and returns
-// the first (index,value) for which the function is true or -1,nil otherwise
-// if no element matches the criteria.
-func (list *List) Find(f func(index int, value interface{}) bool) (int, interface{}) {
- iterator := list.Iterator()
- for iterator.Next() {
- if f(iterator.Index(), iterator.Value()) {
- return iterator.Index(), iterator.Value()
- }
- }
- return -1, nil
-}
diff --git a/vendor/github.com/emirpasic/gods/lists/arraylist/iterator.go b/vendor/github.com/emirpasic/gods/lists/arraylist/iterator.go
deleted file mode 100644
index 38a93f3..0000000
--- a/vendor/github.com/emirpasic/gods/lists/arraylist/iterator.go
+++ /dev/null
@@ -1,83 +0,0 @@
-// Copyright (c) 2015, Emir Pasic. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package arraylist
-
-import "github.com/emirpasic/gods/containers"
-
-func assertIteratorImplementation() {
- var _ containers.ReverseIteratorWithIndex = (*Iterator)(nil)
-}
-
-// Iterator holding the iterator's state
-type Iterator struct {
- list *List
- index int
-}
-
-// Iterator returns a stateful iterator whose values can be fetched by an index.
-func (list *List) Iterator() Iterator {
- return Iterator{list: list, index: -1}
-}
-
-// Next moves the iterator to the next element and returns true if there was a next element in the container.
-// If Next() returns true, then next element's index and value can be retrieved by Index() and Value().
-// If Next() was called for the first time, then it will point the iterator to the first element if it exists.
-// Modifies the state of the iterator.
-func (iterator *Iterator) Next() bool {
- if iterator.index < iterator.list.size {
- iterator.index++
- }
- return iterator.list.withinRange(iterator.index)
-}
-
-// Prev moves the iterator to the previous element and returns true if there was a previous element in the container.
-// If Prev() returns true, then previous element's index and value can be retrieved by Index() and Value().
-// Modifies the state of the iterator.
-func (iterator *Iterator) Prev() bool {
- if iterator.index >= 0 {
- iterator.index--
- }
- return iterator.list.withinRange(iterator.index)
-}
-
-// Value returns the current element's value.
-// Does not modify the state of the iterator.
-func (iterator *Iterator) Value() interface{} {
- return iterator.list.elements[iterator.index]
-}
-
-// Index returns the current element's index.
-// Does not modify the state of the iterator.
-func (iterator *Iterator) Index() int {
- return iterator.index
-}
-
-// Begin resets the iterator to its initial state (one-before-first)
-// Call Next() to fetch the first element if any.
-func (iterator *Iterator) Begin() {
- iterator.index = -1
-}
-
-// End moves the iterator past the last element (one-past-the-end).
-// Call Prev() to fetch the last element if any.
-func (iterator *Iterator) End() {
- iterator.index = iterator.list.size
-}
-
-// First moves the iterator to the first element and returns true if there was a first element in the container.
-// If First() returns true, then first element's index and value can be retrieved by Index() and Value().
-// Modifies the state of the iterator.
-func (iterator *Iterator) First() bool {
- iterator.Begin()
- return iterator.Next()
-}
-
-// Last moves the iterator to the last element and returns true if there was a last element in the container.
-// If Last() returns true, then last element's index and value can be retrieved by Index() and Value().
-// Modifies the state of the iterator.
-func (iterator *Iterator) Last() bool {
- iterator.End()
- return iterator.Prev()
-}
diff --git a/vendor/github.com/emirpasic/gods/lists/arraylist/serialization.go b/vendor/github.com/emirpasic/gods/lists/arraylist/serialization.go
deleted file mode 100644
index 2f283fb..0000000
--- a/vendor/github.com/emirpasic/gods/lists/arraylist/serialization.go
+++ /dev/null
@@ -1,29 +0,0 @@
-// Copyright (c) 2015, Emir Pasic. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package arraylist
-
-import (
- "encoding/json"
- "github.com/emirpasic/gods/containers"
-)
-
-func assertSerializationImplementation() {
- var _ containers.JSONSerializer = (*List)(nil)
- var _ containers.JSONDeserializer = (*List)(nil)
-}
-
-// ToJSON outputs the JSON representation of list's elements.
-func (list *List) ToJSON() ([]byte, error) {
- return json.Marshal(list.elements[:list.size])
-}
-
-// FromJSON populates list's elements from the input JSON representation.
-func (list *List) FromJSON(data []byte) error {
- err := json.Unmarshal(data, &list.elements)
- if err == nil {
- list.size = len(list.elements)
- }
- return err
-}
diff --git a/vendor/github.com/emirpasic/gods/lists/lists.go b/vendor/github.com/emirpasic/gods/lists/lists.go
deleted file mode 100644
index 1f6bb08..0000000
--- a/vendor/github.com/emirpasic/gods/lists/lists.go
+++ /dev/null
@@ -1,33 +0,0 @@
-// Copyright (c) 2015, Emir Pasic. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-// Package lists provides an abstract List interface.
-//
-// In computer science, a list or sequence is an abstract data type that represents an ordered sequence of values, where the same value may occur more than once. An instance of a list is a computer representation of the mathematical concept of a finite sequence; the (potentially) infinite analog of a list is a stream. Lists are a basic example of containers, as they contain other values. If the same value occurs multiple times, each occurrence is considered a distinct item.
-//
-// Reference: https://en.wikipedia.org/wiki/List_%28abstract_data_type%29
-package lists
-
-import (
- "github.com/emirpasic/gods/containers"
- "github.com/emirpasic/gods/utils"
-)
-
-// List interface that all lists implement
-type List interface {
- Get(index int) (interface{}, bool)
- Remove(index int)
- Add(values ...interface{})
- Contains(values ...interface{}) bool
- Sort(comparator utils.Comparator)
- Swap(index1, index2 int)
- Insert(index int, values ...interface{})
- Set(index int, value interface{})
-
- containers.Container
- // Empty() bool
- // Size() int
- // Clear()
- // Values() []interface{}
-}
diff --git a/vendor/github.com/emirpasic/gods/trees/binaryheap/binaryheap.go b/vendor/github.com/emirpasic/gods/trees/binaryheap/binaryheap.go
deleted file mode 100644
index 70b28cf..0000000
--- a/vendor/github.com/emirpasic/gods/trees/binaryheap/binaryheap.go
+++ /dev/null
@@ -1,163 +0,0 @@
-// Copyright (c) 2015, Emir Pasic. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-// Package binaryheap implements a binary heap backed by array list.
-//
-// Comparator defines this heap as either min or max heap.
-//
-// Structure is not thread safe.
-//
-// References: http://en.wikipedia.org/wiki/Binary_heap
-package binaryheap
-
-import (
- "fmt"
- "github.com/emirpasic/gods/lists/arraylist"
- "github.com/emirpasic/gods/trees"
- "github.com/emirpasic/gods/utils"
- "strings"
-)
-
-func assertTreeImplementation() {
- var _ trees.Tree = (*Heap)(nil)
-}
-
-// Heap holds elements in an array-list
-type Heap struct {
- list *arraylist.List
- Comparator utils.Comparator
-}
-
-// NewWith instantiates a new empty heap tree with the custom comparator.
-func NewWith(comparator utils.Comparator) *Heap {
- return &Heap{list: arraylist.New(), Comparator: comparator}
-}
-
-// NewWithIntComparator instantiates a new empty heap with the IntComparator, i.e. elements are of type int.
-func NewWithIntComparator() *Heap {
- return &Heap{list: arraylist.New(), Comparator: utils.IntComparator}
-}
-
-// NewWithStringComparator instantiates a new empty heap with the StringComparator, i.e. elements are of type string.
-func NewWithStringComparator() *Heap {
- return &Heap{list: arraylist.New(), Comparator: utils.StringComparator}
-}
-
-// Push adds a value onto the heap and bubbles it up accordingly.
-func (heap *Heap) Push(values ...interface{}) {
- if len(values) == 1 {
- heap.list.Add(values[0])
- heap.bubbleUp()
- } else {
- // Reference: https://en.wikipedia.org/wiki/Binary_heap#Building_a_heap
- for _, value := range values {
- heap.list.Add(value)
- }
- size := heap.list.Size()/2 + 1
- for i := size; i >= 0; i-- {
- heap.bubbleDownIndex(i)
- }
- }
-}
-
-// Pop removes top element on heap and returns it, or nil if heap is empty.
-// Second return parameter is true, unless the heap was empty and there was nothing to pop.
-func (heap *Heap) Pop() (value interface{}, ok bool) {
- value, ok = heap.list.Get(0)
- if !ok {
- return
- }
- lastIndex := heap.list.Size() - 1
- heap.list.Swap(0, lastIndex)
- heap.list.Remove(lastIndex)
- heap.bubbleDown()
- return
-}
-
-// Peek returns top element on the heap without removing it, or nil if heap is empty.
-// Second return parameter is true, unless the heap was empty and there was nothing to peek.
-func (heap *Heap) Peek() (value interface{}, ok bool) {
- return heap.list.Get(0)
-}
-
-// Empty returns true if heap does not contain any elements.
-func (heap *Heap) Empty() bool {
- return heap.list.Empty()
-}
-
-// Size returns number of elements within the heap.
-func (heap *Heap) Size() int {
- return heap.list.Size()
-}
-
-// Clear removes all elements from the heap.
-func (heap *Heap) Clear() {
- heap.list.Clear()
-}
-
-// Values returns all elements in the heap.
-func (heap *Heap) Values() []interface{} {
- return heap.list.Values()
-}
-
-// String returns a string representation of container
-func (heap *Heap) String() string {
- str := "BinaryHeap\n"
- values := []string{}
- for _, value := range heap.list.Values() {
- values = append(values, fmt.Sprintf("%v", value))
- }
- str += strings.Join(values, ", ")
- return str
-}
-
-// Performs the "bubble down" operation. This is to place the element that is at the root
-// of the heap in its correct place so that the heap maintains the min/max-heap order property.
-func (heap *Heap) bubbleDown() {
- heap.bubbleDownIndex(0)
-}
-
-// Performs the "bubble down" operation. This is to place the element that is at the index
-// of the heap in its correct place so that the heap maintains the min/max-heap order property.
-func (heap *Heap) bubbleDownIndex(index int) {
- size := heap.list.Size()
- for leftIndex := index<<1 + 1; leftIndex < size; leftIndex = index<<1 + 1 {
- rightIndex := index<<1 + 2
- smallerIndex := leftIndex
- leftValue, _ := heap.list.Get(leftIndex)
- rightValue, _ := heap.list.Get(rightIndex)
- if rightIndex < size && heap.Comparator(leftValue, rightValue) > 0 {
- smallerIndex = rightIndex
- }
- indexValue, _ := heap.list.Get(index)
- smallerValue, _ := heap.list.Get(smallerIndex)
- if heap.Comparator(indexValue, smallerValue) > 0 {
- heap.list.Swap(index, smallerIndex)
- } else {
- break
- }
- index = smallerIndex
- }
-}
-
-// Performs the "bubble up" operation. This is to place a newly inserted
-// element (i.e. last element in the list) in its correct place so that
-// the heap maintains the min/max-heap order property.
-func (heap *Heap) bubbleUp() {
- index := heap.list.Size() - 1
- for parentIndex := (index - 1) >> 1; index > 0; parentIndex = (index - 1) >> 1 {
- indexValue, _ := heap.list.Get(index)
- parentValue, _ := heap.list.Get(parentIndex)
- if heap.Comparator(parentValue, indexValue) <= 0 {
- break
- }
- heap.list.Swap(index, parentIndex)
- index = parentIndex
- }
-}
-
-// Check that the index is within bounds of the list
-func (heap *Heap) withinRange(index int) bool {
- return index >= 0 && index < heap.list.Size()
-}
diff --git a/vendor/github.com/emirpasic/gods/trees/binaryheap/iterator.go b/vendor/github.com/emirpasic/gods/trees/binaryheap/iterator.go
deleted file mode 100644
index beeb8d7..0000000
--- a/vendor/github.com/emirpasic/gods/trees/binaryheap/iterator.go
+++ /dev/null
@@ -1,84 +0,0 @@
-// Copyright (c) 2015, Emir Pasic. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package binaryheap
-
-import "github.com/emirpasic/gods/containers"
-
-func assertIteratorImplementation() {
- var _ containers.ReverseIteratorWithIndex = (*Iterator)(nil)
-}
-
-// Iterator returns a stateful iterator whose values can be fetched by an index.
-type Iterator struct {
- heap *Heap
- index int
-}
-
-// Iterator returns a stateful iterator whose values can be fetched by an index.
-func (heap *Heap) Iterator() Iterator {
- return Iterator{heap: heap, index: -1}
-}
-
-// Next moves the iterator to the next element and returns true if there was a next element in the container.
-// If Next() returns true, then next element's index and value can be retrieved by Index() and Value().
-// If Next() was called for the first time, then it will point the iterator to the first element if it exists.
-// Modifies the state of the iterator.
-func (iterator *Iterator) Next() bool {
- if iterator.index < iterator.heap.Size() {
- iterator.index++
- }
- return iterator.heap.withinRange(iterator.index)
-}
-
-// Prev moves the iterator to the previous element and returns true if there was a previous element in the container.
-// If Prev() returns true, then previous element's index and value can be retrieved by Index() and Value().
-// Modifies the state of the iterator.
-func (iterator *Iterator) Prev() bool {
- if iterator.index >= 0 {
- iterator.index--
- }
- return iterator.heap.withinRange(iterator.index)
-}
-
-// Value returns the current element's value.
-// Does not modify the state of the iterator.
-func (iterator *Iterator) Value() interface{} {
- value, _ := iterator.heap.list.Get(iterator.index)
- return value
-}
-
-// Index returns the current element's index.
-// Does not modify the state of the iterator.
-func (iterator *Iterator) Index() int {
- return iterator.index
-}
-
-// Begin resets the iterator to its initial state (one-before-first)
-// Call Next() to fetch the first element if any.
-func (iterator *Iterator) Begin() {
- iterator.index = -1
-}
-
-// End moves the iterator past the last element (one-past-the-end).
-// Call Prev() to fetch the last element if any.
-func (iterator *Iterator) End() {
- iterator.index = iterator.heap.Size()
-}
-
-// First moves the iterator to the first element and returns true if there was a first element in the container.
-// If First() returns true, then first element's index and value can be retrieved by Index() and Value().
-// Modifies the state of the iterator.
-func (iterator *Iterator) First() bool {
- iterator.Begin()
- return iterator.Next()
-}
-
-// Last moves the iterator to the last element and returns true if there was a last element in the container.
-// If Last() returns true, then last element's index and value can be retrieved by Index() and Value().
-// Modifies the state of the iterator.
-func (iterator *Iterator) Last() bool {
- iterator.End()
- return iterator.Prev()
-}
diff --git a/vendor/github.com/emirpasic/gods/trees/binaryheap/serialization.go b/vendor/github.com/emirpasic/gods/trees/binaryheap/serialization.go
deleted file mode 100644
index 00d0c77..0000000
--- a/vendor/github.com/emirpasic/gods/trees/binaryheap/serialization.go
+++ /dev/null
@@ -1,22 +0,0 @@
-// Copyright (c) 2015, Emir Pasic. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package binaryheap
-
-import "github.com/emirpasic/gods/containers"
-
-func assertSerializationImplementation() {
- var _ containers.JSONSerializer = (*Heap)(nil)
- var _ containers.JSONDeserializer = (*Heap)(nil)
-}
-
-// ToJSON outputs the JSON representation of the heap.
-func (heap *Heap) ToJSON() ([]byte, error) {
- return heap.list.ToJSON()
-}
-
-// FromJSON populates the heap from the input JSON representation.
-func (heap *Heap) FromJSON(data []byte) error {
- return heap.list.FromJSON(data)
-}
diff --git a/vendor/github.com/emirpasic/gods/trees/trees.go b/vendor/github.com/emirpasic/gods/trees/trees.go
deleted file mode 100644
index a5a7427..0000000
--- a/vendor/github.com/emirpasic/gods/trees/trees.go
+++ /dev/null
@@ -1,21 +0,0 @@
-// Copyright (c) 2015, Emir Pasic. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-// Package trees provides an abstract Tree interface.
-//
-// In computer science, a tree is a widely used abstract data type (ADT) or data structure implementing this ADT that simulates a hierarchical tree structure, with a root value and subtrees of children with a parent node, represented as a set of linked nodes.
-//
-// Reference: https://en.wikipedia.org/wiki/Tree_%28data_structure%29
-package trees
-
-import "github.com/emirpasic/gods/containers"
-
-// Tree interface that all trees implement
-type Tree interface {
- containers.Container
- // Empty() bool
- // Size() int
- // Clear()
- // Values() []interface{}
-}
diff --git a/vendor/github.com/emirpasic/gods/utils/comparator.go b/vendor/github.com/emirpasic/gods/utils/comparator.go
deleted file mode 100644
index 6a9afbf..0000000
--- a/vendor/github.com/emirpasic/gods/utils/comparator.go
+++ /dev/null
@@ -1,251 +0,0 @@
-// Copyright (c) 2015, Emir Pasic. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package utils
-
-import "time"
-
-// Comparator will make type assertion (see IntComparator for example),
-// which will panic if a or b are not of the asserted type.
-//
-// Should return a number:
-// negative , if a < b
-// zero , if a == b
-// positive , if a > b
-type Comparator func(a, b interface{}) int
-
-// StringComparator provides a fast comparison on strings
-func StringComparator(a, b interface{}) int {
- s1 := a.(string)
- s2 := b.(string)
- min := len(s2)
- if len(s1) < len(s2) {
- min = len(s1)
- }
- diff := 0
- for i := 0; i < min && diff == 0; i++ {
- diff = int(s1[i]) - int(s2[i])
- }
- if diff == 0 {
- diff = len(s1) - len(s2)
- }
- if diff < 0 {
- return -1
- }
- if diff > 0 {
- return 1
- }
- return 0
-}
-
-// IntComparator provides a basic comparison on int
-func IntComparator(a, b interface{}) int {
- aAsserted := a.(int)
- bAsserted := b.(int)
- switch {
- case aAsserted > bAsserted:
- return 1
- case aAsserted < bAsserted:
- return -1
- default:
- return 0
- }
-}
-
-// Int8Comparator provides a basic comparison on int8
-func Int8Comparator(a, b interface{}) int {
- aAsserted := a.(int8)
- bAsserted := b.(int8)
- switch {
- case aAsserted > bAsserted:
- return 1
- case aAsserted < bAsserted:
- return -1
- default:
- return 0
- }
-}
-
-// Int16Comparator provides a basic comparison on int16
-func Int16Comparator(a, b interface{}) int {
- aAsserted := a.(int16)
- bAsserted := b.(int16)
- switch {
- case aAsserted > bAsserted:
- return 1
- case aAsserted < bAsserted:
- return -1
- default:
- return 0
- }
-}
-
-// Int32Comparator provides a basic comparison on int32
-func Int32Comparator(a, b interface{}) int {
- aAsserted := a.(int32)
- bAsserted := b.(int32)
- switch {
- case aAsserted > bAsserted:
- return 1
- case aAsserted < bAsserted:
- return -1
- default:
- return 0
- }
-}
-
-// Int64Comparator provides a basic comparison on int64
-func Int64Comparator(a, b interface{}) int {
- aAsserted := a.(int64)
- bAsserted := b.(int64)
- switch {
- case aAsserted > bAsserted:
- return 1
- case aAsserted < bAsserted:
- return -1
- default:
- return 0
- }
-}
-
-// UIntComparator provides a basic comparison on uint
-func UIntComparator(a, b interface{}) int {
- aAsserted := a.(uint)
- bAsserted := b.(uint)
- switch {
- case aAsserted > bAsserted:
- return 1
- case aAsserted < bAsserted:
- return -1
- default:
- return 0
- }
-}
-
-// UInt8Comparator provides a basic comparison on uint8
-func UInt8Comparator(a, b interface{}) int {
- aAsserted := a.(uint8)
- bAsserted := b.(uint8)
- switch {
- case aAsserted > bAsserted:
- return 1
- case aAsserted < bAsserted:
- return -1
- default:
- return 0
- }
-}
-
-// UInt16Comparator provides a basic comparison on uint16
-func UInt16Comparator(a, b interface{}) int {
- aAsserted := a.(uint16)
- bAsserted := b.(uint16)
- switch {
- case aAsserted > bAsserted:
- return 1
- case aAsserted < bAsserted:
- return -1
- default:
- return 0
- }
-}
-
-// UInt32Comparator provides a basic comparison on uint32
-func UInt32Comparator(a, b interface{}) int {
- aAsserted := a.(uint32)
- bAsserted := b.(uint32)
- switch {
- case aAsserted > bAsserted:
- return 1
- case aAsserted < bAsserted:
- return -1
- default:
- return 0
- }
-}
-
-// UInt64Comparator provides a basic comparison on uint64
-func UInt64Comparator(a, b interface{}) int {
- aAsserted := a.(uint64)
- bAsserted := b.(uint64)
- switch {
- case aAsserted > bAsserted:
- return 1
- case aAsserted < bAsserted:
- return -1
- default:
- return 0
- }
-}
-
-// Float32Comparator provides a basic comparison on float32
-func Float32Comparator(a, b interface{}) int {
- aAsserted := a.(float32)
- bAsserted := b.(float32)
- switch {
- case aAsserted > bAsserted:
- return 1
- case aAsserted < bAsserted:
- return -1
- default:
- return 0
- }
-}
-
-// Float64Comparator provides a basic comparison on float64
-func Float64Comparator(a, b interface{}) int {
- aAsserted := a.(float64)
- bAsserted := b.(float64)
- switch {
- case aAsserted > bAsserted:
- return 1
- case aAsserted < bAsserted:
- return -1
- default:
- return 0
- }
-}
-
-// ByteComparator provides a basic comparison on byte
-func ByteComparator(a, b interface{}) int {
- aAsserted := a.(byte)
- bAsserted := b.(byte)
- switch {
- case aAsserted > bAsserted:
- return 1
- case aAsserted < bAsserted:
- return -1
- default:
- return 0
- }
-}
-
-// RuneComparator provides a basic comparison on rune
-func RuneComparator(a, b interface{}) int {
- aAsserted := a.(rune)
- bAsserted := b.(rune)
- switch {
- case aAsserted > bAsserted:
- return 1
- case aAsserted < bAsserted:
- return -1
- default:
- return 0
- }
-}
-
-// TimeComparator provides a basic comparison on time.Time
-func TimeComparator(a, b interface{}) int {
- aAsserted := a.(time.Time)
- bAsserted := b.(time.Time)
-
- switch {
- case aAsserted.After(bAsserted):
- return 1
- case aAsserted.Before(bAsserted):
- return -1
- default:
- return 0
- }
-}
diff --git a/vendor/github.com/emirpasic/gods/utils/sort.go b/vendor/github.com/emirpasic/gods/utils/sort.go
deleted file mode 100644
index 79ced1f..0000000
--- a/vendor/github.com/emirpasic/gods/utils/sort.go
+++ /dev/null
@@ -1,29 +0,0 @@
-// Copyright (c) 2015, Emir Pasic. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package utils
-
-import "sort"
-
-// Sort sorts values (in-place) with respect to the given comparator.
-//
-// Uses Go's sort (hybrid of quicksort for large and then insertion sort for smaller slices).
-func Sort(values []interface{}, comparator Comparator) {
- sort.Sort(sortable{values, comparator})
-}
-
-type sortable struct {
- values []interface{}
- comparator Comparator
-}
-
-func (s sortable) Len() int {
- return len(s.values)
-}
-func (s sortable) Swap(i, j int) {
- s.values[i], s.values[j] = s.values[j], s.values[i]
-}
-func (s sortable) Less(i, j int) bool {
- return s.comparator(s.values[i], s.values[j]) < 0
-}
diff --git a/vendor/github.com/emirpasic/gods/utils/utils.go b/vendor/github.com/emirpasic/gods/utils/utils.go
deleted file mode 100644
index 1ad49cb..0000000
--- a/vendor/github.com/emirpasic/gods/utils/utils.go
+++ /dev/null
@@ -1,47 +0,0 @@
-// Copyright (c) 2015, Emir Pasic. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-// Package utils provides common utility functions.
-//
-// Provided functionalities:
-// - sorting
-// - comparators
-package utils
-
-import (
- "fmt"
- "strconv"
-)
-
-// ToString converts a value to string.
-func ToString(value interface{}) string {
- switch value.(type) {
- case string:
- return value.(string)
- case int8:
- return strconv.FormatInt(int64(value.(int8)), 10)
- case int16:
- return strconv.FormatInt(int64(value.(int16)), 10)
- case int32:
- return strconv.FormatInt(int64(value.(int32)), 10)
- case int64:
- return strconv.FormatInt(int64(value.(int64)), 10)
- case uint8:
- return strconv.FormatUint(uint64(value.(uint8)), 10)
- case uint16:
- return strconv.FormatUint(uint64(value.(uint16)), 10)
- case uint32:
- return strconv.FormatUint(uint64(value.(uint32)), 10)
- case uint64:
- return strconv.FormatUint(uint64(value.(uint64)), 10)
- case float32:
- return strconv.FormatFloat(float64(value.(float32)), 'g', -1, 64)
- case float64:
- return strconv.FormatFloat(float64(value.(float64)), 'g', -1, 64)
- case bool:
- return strconv.FormatBool(value.(bool))
- default:
- return fmt.Sprintf("%+v", value)
- }
-}
diff --git a/vendor/github.com/enescakir/emoji/.gitignore b/vendor/github.com/enescakir/emoji/.gitignore
deleted file mode 100644
index 66fd13c..0000000
--- a/vendor/github.com/enescakir/emoji/.gitignore
+++ /dev/null
@@ -1,15 +0,0 @@
-# Binaries for programs and plugins
-*.exe
-*.exe~
-*.dll
-*.so
-*.dylib
-
-# Test binary, built with `go test -c`
-*.test
-
-# Output of the go coverage tool, specifically when used with LiteIDE
-*.out
-
-# Dependency directories (remove the comment below to include it)
-# vendor/
diff --git a/vendor/github.com/enescakir/emoji/LICENSE b/vendor/github.com/enescakir/emoji/LICENSE
deleted file mode 100644
index 1bf8496..0000000
--- a/vendor/github.com/enescakir/emoji/LICENSE
+++ /dev/null
@@ -1,21 +0,0 @@
-MIT License
-
-Copyright (c) 2020 Enes Çakır
-
-Permission is hereby granted, free of charge, to any person obtaining a copy
-of this software and associated documentation files (the "Software"), to deal
-in the Software without restriction, including without limitation the rights
-to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
-copies of the Software, and to permit persons to whom the Software is
-furnished to do so, subject to the following conditions:
-
-The above copyright notice and this permission notice shall be included in all
-copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
-IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
-FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
-AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
-LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
-OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
-SOFTWARE.
diff --git a/vendor/github.com/enescakir/emoji/README.md b/vendor/github.com/enescakir/emoji/README.md
deleted file mode 100644
index 1cf572a..0000000
--- a/vendor/github.com/enescakir/emoji/README.md
+++ /dev/null
@@ -1,99 +0,0 @@
-# emoji :rocket: :school_satchel: :tada:
-[](https://github.com/enescakir/emoji/actions)
-[](https://godoc.org/github.com/enescakir/emoji)
-[](https://goreportcard.com/report/github.com/enescakir/emoji)
-[](https://codecov.io/gh/enescakir/emoji)
-[](https://github.com/enescakir/emoji/blob/master/LICENSE)
-
-`emoji` is a minimalistic emoji library for Go. It lets you use emoji characters in strings.
-
-Inspired by [spatie/emoji](https://github.com/spatie/emoji)
-
-## Install :floppy_disk:
-``` bash
-go get github.com/enescakir/emoji
-```
-
-## Usage :surfer:
-```go
-package main
-
-import (
- "fmt"
-
- "github.com/enescakir/emoji"
-)
-
-func main() {
- fmt.Printf("Hello %v\n", emoji.WavingHand)
- fmt.Printf("I am %v from %v\n",
- emoji.ManTechnologist,
- emoji.FlagForTurkey,
- )
- fmt.Printf("Different skin tones.\n default: %v light: %v dark: %v\n",
- emoji.ThumbsUp,
- emoji.OkHand.Tone(emoji.Light),
- emoji.CallMeHand.Tone(emoji.Dark),
- )
- fmt.Printf("Emojis with multiple skin tones.\n both medium: %v light and dark: %v\n",
- emoji.PeopleHoldingHands.Tone(emoji.Medium),
- emoji.PeopleHoldingHands.Tone(emoji.Light, emoji.Dark),
- )
- fmt.Println(emoji.Parse("Emoji aliases are :sunglasses:"))
- emoji.Println("Use fmt wrappers :+1: with emoji support :tada:")
-}
-
-/* OUTPUT
-
- Hello 👋
- I am 👨💻 from 🇹🇷
- Different skin tones.
- default: 👍 light: 👌🏻 dark: 🤙🏿
- Emojis with multiple skin tones.
- both medium: 🧑🏽🤝🧑🏽 light and dark: 🧑🏻🤝🧑🏿
- Emoji aliases are 😎
- Use fmt wrappers 👍 with emoji support 🎉
-*/
-```
-
-This package contains emojis constants based on [Full Emoji List v13.0](https://unicode.org/Public/emoji/13.0/emoji-test.txt).
-```go
-emoji.CallMeHand // 🤙
-emoji.CallMeHand.Tone(emoji.Dark) // 🤙🏿
-```
-Also, it has additional emoji aliases from [github/gemoji](https://github.com/github/gemoji).
-```go
-emoji.Parse(":+1:") // 👍
-emoji.Parse(":100:") // 💯
-```
-
-You can generate country flag emoji with [ISO 3166 Alpha2](https://en.wikipedia.org/wiki/ISO_3166-1_alpha-2) codes:
-```go
-emoji.CountryFlag("tr") // 🇹🇷
-emoji.CountryFlag("US") // 🇺🇸
-emoji.Parse("country flag alias :flag-gb:") // country flag alias 🇬🇧
-```
-
-All constants are generated by `internal/generator`.
-
-## Testing :hammer:
-``` bash
-go test
-```
-
-## Todo :pushpin:
-* Add examples to `godoc`
-
-## Contributing :man_technologist:
-I am accepting PRs that add aliases to the package.
-You have to add it to `customEmojis` list at `internal/generator/main`.
-
-If you think an emoji constant is not correct, open an issue.
-Please use [this list](http://unicode.org/emoji/charts/full-emoji-list.html)
-to look up the correct unicode value and the name of the character.
-
-## Credits :star:
-- [Enes Çakır](https://github.com/enescakir)
-
-## License :scroll:
-The MIT License (MIT). Please see [License File](LICENSE.md) for more information.
diff --git a/vendor/github.com/enescakir/emoji/constants.go b/vendor/github.com/enescakir/emoji/constants.go
deleted file mode 100644
index e64efd5..0000000
--- a/vendor/github.com/enescakir/emoji/constants.go
+++ /dev/null
@@ -1,1944 +0,0 @@
-package emoji
-
-// Code generated by github.com/enescakir/emoji/internal/generator DO NOT EDIT.
-
-// Source: https://unicode.org/Public/emoji/13.0/emoji-test.txt
-// Create at: 2020-03-08T15:58:37+03:00
-
-var (
-
- // GROUP: Smileys & Emotion
- // SUBGROUP: face-smiling
- GrinningFace Emoji = "\U0001f600" // grinning face
- GrinningFaceWithBigEyes Emoji = "\U0001f603" // grinning face with big eyes
- GrinningFaceWithSmilingEyes Emoji = "\U0001f604" // grinning face with smiling eyes
- BeamingFaceWithSmilingEyes Emoji = "\U0001f601" // beaming face with smiling eyes
- GrinningSquintingFace Emoji = "\U0001f606" // grinning squinting face
- GrinningFaceWithSweat Emoji = "\U0001f605" // grinning face with sweat
- RollingOnTheFloorLaughing Emoji = "\U0001f923" // rolling on the floor laughing
- FaceWithTearsOfJoy Emoji = "\U0001f602" // face with tears of joy
- SlightlySmilingFace Emoji = "\U0001f642" // slightly smiling face
- UpsideDownFace Emoji = "\U0001f643" // upside-down face
- WinkingFace Emoji = "\U0001f609" // winking face
- SmilingFaceWithSmilingEyes Emoji = "\U0001f60a" // smiling face with smiling eyes
- SmilingFaceWithHalo Emoji = "\U0001f607" // smiling face with halo
- // SUBGROUP: face-affection
- SmilingFaceWithHearts Emoji = "\U0001f970" // smiling face with hearts
- SmilingFaceWithHeartEyes Emoji = "\U0001f60d" // smiling face with heart-eyes
- StarStruck Emoji = "\U0001f929" // star-struck
- FaceBlowingAKiss Emoji = "\U0001f618" // face blowing a kiss
- KissingFace Emoji = "\U0001f617" // kissing face
- SmilingFace Emoji = "\u263a\ufe0f" // smiling face
- KissingFaceWithClosedEyes Emoji = "\U0001f61a" // kissing face with closed eyes
- KissingFaceWithSmilingEyes Emoji = "\U0001f619" // kissing face with smiling eyes
- SmilingFaceWithTear Emoji = "\U0001f972" // smiling face with tear
- // SUBGROUP: face-tongue
- FaceSavoringFood Emoji = "\U0001f60b" // face savoring food
- FaceWithTongue Emoji = "\U0001f61b" // face with tongue
- WinkingFaceWithTongue Emoji = "\U0001f61c" // winking face with tongue
- ZanyFace Emoji = "\U0001f92a" // zany face
- SquintingFaceWithTongue Emoji = "\U0001f61d" // squinting face with tongue
- MoneyMouthFace Emoji = "\U0001f911" // money-mouth face
- // SUBGROUP: face-hand
- HuggingFace Emoji = "\U0001f917" // hugging face
- FaceWithHandOverMouth Emoji = "\U0001f92d" // face with hand over mouth
- ShushingFace Emoji = "\U0001f92b" // shushing face
- ThinkingFace Emoji = "\U0001f914" // thinking face
- // SUBGROUP: face-neutral-skeptical
- ZipperMouthFace Emoji = "\U0001f910" // zipper-mouth face
- FaceWithRaisedEyebrow Emoji = "\U0001f928" // face with raised eyebrow
- NeutralFace Emoji = "\U0001f610" // neutral face
- ExpressionlessFace Emoji = "\U0001f611" // expressionless face
- FaceWithoutMouth Emoji = "\U0001f636" // face without mouth
- SmirkingFace Emoji = "\U0001f60f" // smirking face
- UnamusedFace Emoji = "\U0001f612" // unamused face
- FaceWithRollingEyes Emoji = "\U0001f644" // face with rolling eyes
- GrimacingFace Emoji = "\U0001f62c" // grimacing face
- LyingFace Emoji = "\U0001f925" // lying face
- // SUBGROUP: face-sleepy
- RelievedFace Emoji = "\U0001f60c" // relieved face
- PensiveFace Emoji = "\U0001f614" // pensive face
- SleepyFace Emoji = "\U0001f62a" // sleepy face
- DroolingFace Emoji = "\U0001f924" // drooling face
- SleepingFace Emoji = "\U0001f634" // sleeping face
- // SUBGROUP: face-unwell
- FaceWithMedicalMask Emoji = "\U0001f637" // face with medical mask
- FaceWithThermometer Emoji = "\U0001f912" // face with thermometer
- FaceWithHeadBandage Emoji = "\U0001f915" // face with head-bandage
- NauseatedFace Emoji = "\U0001f922" // nauseated face
- FaceVomiting Emoji = "\U0001f92e" // face vomiting
- SneezingFace Emoji = "\U0001f927" // sneezing face
- HotFace Emoji = "\U0001f975" // hot face
- ColdFace Emoji = "\U0001f976" // cold face
- WoozyFace Emoji = "\U0001f974" // woozy face
- DizzyFace Emoji = "\U0001f635" // dizzy face
- ExplodingHead Emoji = "\U0001f92f" // exploding head
- // SUBGROUP: face-hat
- CowboyHatFace Emoji = "\U0001f920" // cowboy hat face
- PartyingFace Emoji = "\U0001f973" // partying face
- DisguisedFace Emoji = "\U0001f978" // disguised face
- // SUBGROUP: face-glasses
- SmilingFaceWithSunglasses Emoji = "\U0001f60e" // smiling face with sunglasses
- NerdFace Emoji = "\U0001f913" // nerd face
- FaceWithMonocle Emoji = "\U0001f9d0" // face with monocle
- // SUBGROUP: face-concerned
- ConfusedFace Emoji = "\U0001f615" // confused face
- WorriedFace Emoji = "\U0001f61f" // worried face
- SlightlyFrowningFace Emoji = "\U0001f641" // slightly frowning face
- FrowningFace Emoji = "\u2639\ufe0f" // frowning face
- FaceWithOpenMouth Emoji = "\U0001f62e" // face with open mouth
- HushedFace Emoji = "\U0001f62f" // hushed face
- AstonishedFace Emoji = "\U0001f632" // astonished face
- FlushedFace Emoji = "\U0001f633" // flushed face
- PleadingFace Emoji = "\U0001f97a" // pleading face
- FrowningFaceWithOpenMouth Emoji = "\U0001f626" // frowning face with open mouth
- AnguishedFace Emoji = "\U0001f627" // anguished face
- FearfulFace Emoji = "\U0001f628" // fearful face
- AnxiousFaceWithSweat Emoji = "\U0001f630" // anxious face with sweat
- SadButRelievedFace Emoji = "\U0001f625" // sad but relieved face
- CryingFace Emoji = "\U0001f622" // crying face
- LoudlyCryingFace Emoji = "\U0001f62d" // loudly crying face
- FaceScreamingInFear Emoji = "\U0001f631" // face screaming in fear
- ConfoundedFace Emoji = "\U0001f616" // confounded face
- PerseveringFace Emoji = "\U0001f623" // persevering face
- DisappointedFace Emoji = "\U0001f61e" // disappointed face
- DowncastFaceWithSweat Emoji = "\U0001f613" // downcast face with sweat
- WearyFace Emoji = "\U0001f629" // weary face
- TiredFace Emoji = "\U0001f62b" // tired face
- YawningFace Emoji = "\U0001f971" // yawning face
- // SUBGROUP: face-negative
- FaceWithSteamFromNose Emoji = "\U0001f624" // face with steam from nose
- PoutingFace Emoji = "\U0001f621" // pouting face
- AngryFace Emoji = "\U0001f620" // angry face
- FaceWithSymbolsOnMouth Emoji = "\U0001f92c" // face with symbols on mouth
- SmilingFaceWithHorns Emoji = "\U0001f608" // smiling face with horns
- AngryFaceWithHorns Emoji = "\U0001f47f" // angry face with horns
- Skull Emoji = "\U0001f480" // skull
- SkullAndCrossbones Emoji = "\u2620\ufe0f" // skull and crossbones
- // SUBGROUP: face-costume
- PileOfPoo Emoji = "\U0001f4a9" // pile of poo
- ClownFace Emoji = "\U0001f921" // clown face
- Ogre Emoji = "\U0001f479" // ogre
- Goblin Emoji = "\U0001f47a" // goblin
- Ghost Emoji = "\U0001f47b" // ghost
- Alien Emoji = "\U0001f47d" // alien
- AlienMonster Emoji = "\U0001f47e" // alien monster
- Robot Emoji = "\U0001f916" // robot
- // SUBGROUP: cat-face
- GrinningCat Emoji = "\U0001f63a" // grinning cat
- GrinningCatWithSmilingEyes Emoji = "\U0001f638" // grinning cat with smiling eyes
- CatWithTearsOfJoy Emoji = "\U0001f639" // cat with tears of joy
- SmilingCatWithHeartEyes Emoji = "\U0001f63b" // smiling cat with heart-eyes
- CatWithWrySmile Emoji = "\U0001f63c" // cat with wry smile
- KissingCat Emoji = "\U0001f63d" // kissing cat
- WearyCat Emoji = "\U0001f640" // weary cat
- CryingCat Emoji = "\U0001f63f" // crying cat
- PoutingCat Emoji = "\U0001f63e" // pouting cat
- // SUBGROUP: monkey-face
- SeeNoEvilMonkey Emoji = "\U0001f648" // see-no-evil monkey
- HearNoEvilMonkey Emoji = "\U0001f649" // hear-no-evil monkey
- SpeakNoEvilMonkey Emoji = "\U0001f64a" // speak-no-evil monkey
- // SUBGROUP: emotion
- KissMark Emoji = "\U0001f48b" // kiss mark
- LoveLetter Emoji = "\U0001f48c" // love letter
- HeartWithArrow Emoji = "\U0001f498" // heart with arrow
- HeartWithRibbon Emoji = "\U0001f49d" // heart with ribbon
- SparklingHeart Emoji = "\U0001f496" // sparkling heart
- GrowingHeart Emoji = "\U0001f497" // growing heart
- BeatingHeart Emoji = "\U0001f493" // beating heart
- RevolvingHearts Emoji = "\U0001f49e" // revolving hearts
- TwoHearts Emoji = "\U0001f495" // two hearts
- HeartDecoration Emoji = "\U0001f49f" // heart decoration
- HeartExclamation Emoji = "\u2763\ufe0f" // heart exclamation
- BrokenHeart Emoji = "\U0001f494" // broken heart
- RedHeart Emoji = "\u2764\ufe0f" // red heart
- OrangeHeart Emoji = "\U0001f9e1" // orange heart
- YellowHeart Emoji = "\U0001f49b" // yellow heart
- GreenHeart Emoji = "\U0001f49a" // green heart
- BlueHeart Emoji = "\U0001f499" // blue heart
- PurpleHeart Emoji = "\U0001f49c" // purple heart
- BrownHeart Emoji = "\U0001f90e" // brown heart
- BlackHeart Emoji = "\U0001f5a4" // black heart
- WhiteHeart Emoji = "\U0001f90d" // white heart
- HundredPoints Emoji = "\U0001f4af" // hundred points
- AngerSymbol Emoji = "\U0001f4a2" // anger symbol
- Collision Emoji = "\U0001f4a5" // collision
- Dizzy Emoji = "\U0001f4ab" // dizzy
- SweatDroplets Emoji = "\U0001f4a6" // sweat droplets
- DashingAway Emoji = "\U0001f4a8" // dashing away
- Hole Emoji = "\U0001f573\ufe0f" // hole
- Bomb Emoji = "\U0001f4a3" // bomb
- SpeechBalloon Emoji = "\U0001f4ac" // speech balloon
- EyeInSpeechBubble Emoji = "\U0001f441\ufe0f\u200d\U0001f5e8\ufe0f" // eye in speech bubble
- LeftSpeechBubble Emoji = "\U0001f5e8\ufe0f" // left speech bubble
- RightAngerBubble Emoji = "\U0001f5ef\ufe0f" // right anger bubble
- ThoughtBalloon Emoji = "\U0001f4ad" // thought balloon
- Zzz Emoji = "\U0001f4a4" // zzz
-
- // GROUP: People & Body
- // SUBGROUP: hand-fingers-open
- WavingHand EmojiWithTone = newEmojiWithTone("\U0001f44b@") // waving hand
- RaisedBackOfHand EmojiWithTone = newEmojiWithTone("\U0001f91a@") // raised back of hand
- HandWithFingersSplayed EmojiWithTone = newEmojiWithTone("\U0001f590@").withDefaultTone("\ufe0f") // hand with fingers splayed
- RaisedHand EmojiWithTone = newEmojiWithTone("\u270b@") // raised hand
- VulcanSalute EmojiWithTone = newEmojiWithTone("\U0001f596@") // vulcan salute
- // SUBGROUP: hand-fingers-partial
- OkHand EmojiWithTone = newEmojiWithTone("\U0001f44c@") // OK hand
- PinchedFingers EmojiWithTone = newEmojiWithTone("\U0001f90c@") // pinched fingers
- PinchingHand EmojiWithTone = newEmojiWithTone("\U0001f90f@") // pinching hand
- VictoryHand EmojiWithTone = newEmojiWithTone("\u270c@").withDefaultTone("\ufe0f") // victory hand
- CrossedFingers EmojiWithTone = newEmojiWithTone("\U0001f91e@") // crossed fingers
- LoveYouGesture EmojiWithTone = newEmojiWithTone("\U0001f91f@") // love-you gesture
- SignOfTheHorns EmojiWithTone = newEmojiWithTone("\U0001f918@") // sign of the horns
- CallMeHand EmojiWithTone = newEmojiWithTone("\U0001f919@") // call me hand
- // SUBGROUP: hand-single-finger
- BackhandIndexPointingLeft EmojiWithTone = newEmojiWithTone("\U0001f448@") // backhand index pointing left
- BackhandIndexPointingRight EmojiWithTone = newEmojiWithTone("\U0001f449@") // backhand index pointing right
- BackhandIndexPointingUp EmojiWithTone = newEmojiWithTone("\U0001f446@") // backhand index pointing up
- MiddleFinger EmojiWithTone = newEmojiWithTone("\U0001f595@") // middle finger
- BackhandIndexPointingDown EmojiWithTone = newEmojiWithTone("\U0001f447@") // backhand index pointing down
- IndexPointingUp EmojiWithTone = newEmojiWithTone("\u261d@").withDefaultTone("\ufe0f") // index pointing up
- // SUBGROUP: hand-fingers-closed
- ThumbsUp EmojiWithTone = newEmojiWithTone("\U0001f44d@") // thumbs up
- ThumbsDown EmojiWithTone = newEmojiWithTone("\U0001f44e@") // thumbs down
- RaisedFist EmojiWithTone = newEmojiWithTone("\u270a@") // raised fist
- OncomingFist EmojiWithTone = newEmojiWithTone("\U0001f44a@") // oncoming fist
- LeftFacingFist EmojiWithTone = newEmojiWithTone("\U0001f91b@") // left-facing fist
- RightFacingFist EmojiWithTone = newEmojiWithTone("\U0001f91c@") // right-facing fist
- // SUBGROUP: hands
- ClappingHands EmojiWithTone = newEmojiWithTone("\U0001f44f@") // clapping hands
- RaisingHands EmojiWithTone = newEmojiWithTone("\U0001f64c@") // raising hands
- OpenHands EmojiWithTone = newEmojiWithTone("\U0001f450@") // open hands
- PalmsUpTogether EmojiWithTone = newEmojiWithTone("\U0001f932@") // palms up together
- Handshake Emoji = "\U0001f91d" // handshake
- FoldedHands EmojiWithTone = newEmojiWithTone("\U0001f64f@") // folded hands
- // SUBGROUP: hand-prop
- WritingHand EmojiWithTone = newEmojiWithTone("\u270d@").withDefaultTone("\ufe0f") // writing hand
- NailPolish EmojiWithTone = newEmojiWithTone("\U0001f485@") // nail polish
- Selfie EmojiWithTone = newEmojiWithTone("\U0001f933@") // selfie
- // SUBGROUP: body-parts
- FlexedBiceps EmojiWithTone = newEmojiWithTone("\U0001f4aa@") // flexed biceps
- MechanicalArm Emoji = "\U0001f9be" // mechanical arm
- MechanicalLeg Emoji = "\U0001f9bf" // mechanical leg
- Leg EmojiWithTone = newEmojiWithTone("\U0001f9b5@") // leg
- Foot EmojiWithTone = newEmojiWithTone("\U0001f9b6@") // foot
- Ear EmojiWithTone = newEmojiWithTone("\U0001f442@") // ear
- EarWithHearingAid EmojiWithTone = newEmojiWithTone("\U0001f9bb@") // ear with hearing aid
- Nose EmojiWithTone = newEmojiWithTone("\U0001f443@") // nose
- Brain Emoji = "\U0001f9e0" // brain
- AnatomicalHeart Emoji = "\U0001fac0" // anatomical heart
- Lungs Emoji = "\U0001fac1" // lungs
- Tooth Emoji = "\U0001f9b7" // tooth
- Bone Emoji = "\U0001f9b4" // bone
- Eyes Emoji = "\U0001f440" // eyes
- Eye Emoji = "\U0001f441\ufe0f" // eye
- Tongue Emoji = "\U0001f445" // tongue
- Mouth Emoji = "\U0001f444" // mouth
- // SUBGROUP: person
- Baby EmojiWithTone = newEmojiWithTone("\U0001f476@") // baby
- Child EmojiWithTone = newEmojiWithTone("\U0001f9d2@") // child
- Boy EmojiWithTone = newEmojiWithTone("\U0001f466@") // boy
- Girl EmojiWithTone = newEmojiWithTone("\U0001f467@") // girl
- Person EmojiWithTone = newEmojiWithTone("\U0001f9d1@") // person
- PersonWithBlondHair EmojiWithTone = newEmojiWithTone("\U0001f471@") // person: blond hair
- Man EmojiWithTone = newEmojiWithTone("\U0001f468@") // man
- ManWithBeard EmojiWithTone = newEmojiWithTone("\U0001f9d4@") // man: beard
- ManWithRedHair EmojiWithTone = newEmojiWithTone("\U0001f468@\u200d\U0001f9b0") // man: red hair
- ManWithCurlyHair EmojiWithTone = newEmojiWithTone("\U0001f468@\u200d\U0001f9b1") // man: curly hair
- ManWithWhiteHair EmojiWithTone = newEmojiWithTone("\U0001f468@\u200d\U0001f9b3") // man: white hair
- ManBald EmojiWithTone = newEmojiWithTone("\U0001f468@\u200d\U0001f9b2") // man: bald
- Woman EmojiWithTone = newEmojiWithTone("\U0001f469@") // woman
- WomanWithRedHair EmojiWithTone = newEmojiWithTone("\U0001f469@\u200d\U0001f9b0") // woman: red hair
- PersonWithRedHair EmojiWithTone = newEmojiWithTone("\U0001f9d1@\u200d\U0001f9b0") // person: red hair
- WomanWithCurlyHair EmojiWithTone = newEmojiWithTone("\U0001f469@\u200d\U0001f9b1") // woman: curly hair
- PersonWithCurlyHair EmojiWithTone = newEmojiWithTone("\U0001f9d1@\u200d\U0001f9b1") // person: curly hair
- WomanWithWhiteHair EmojiWithTone = newEmojiWithTone("\U0001f469@\u200d\U0001f9b3") // woman: white hair
- PersonWithWhiteHair EmojiWithTone = newEmojiWithTone("\U0001f9d1@\u200d\U0001f9b3") // person: white hair
- WomanBald EmojiWithTone = newEmojiWithTone("\U0001f469@\u200d\U0001f9b2") // woman: bald
- PersonBald EmojiWithTone = newEmojiWithTone("\U0001f9d1@\u200d\U0001f9b2") // person: bald
- WomanWithBlondHair EmojiWithTone = newEmojiWithTone("\U0001f471@\u200d\u2640\ufe0f") // woman: blond hair
- ManWithBlondHair EmojiWithTone = newEmojiWithTone("\U0001f471@\u200d\u2642\ufe0f") // man: blond hair
- OlderPerson EmojiWithTone = newEmojiWithTone("\U0001f9d3@") // older person
- OldMan EmojiWithTone = newEmojiWithTone("\U0001f474@") // old man
- OldWoman EmojiWithTone = newEmojiWithTone("\U0001f475@") // old woman
- // SUBGROUP: person-gesture
- PersonFrowning EmojiWithTone = newEmojiWithTone("\U0001f64d@") // person frowning
- ManFrowning EmojiWithTone = newEmojiWithTone("\U0001f64d@\u200d\u2642\ufe0f") // man frowning
- WomanFrowning EmojiWithTone = newEmojiWithTone("\U0001f64d@\u200d\u2640\ufe0f") // woman frowning
- PersonPouting EmojiWithTone = newEmojiWithTone("\U0001f64e@") // person pouting
- ManPouting EmojiWithTone = newEmojiWithTone("\U0001f64e@\u200d\u2642\ufe0f") // man pouting
- WomanPouting EmojiWithTone = newEmojiWithTone("\U0001f64e@\u200d\u2640\ufe0f") // woman pouting
- PersonGesturingNo EmojiWithTone = newEmojiWithTone("\U0001f645@") // person gesturing NO
- ManGesturingNo EmojiWithTone = newEmojiWithTone("\U0001f645@\u200d\u2642\ufe0f") // man gesturing NO
- WomanGesturingNo EmojiWithTone = newEmojiWithTone("\U0001f645@\u200d\u2640\ufe0f") // woman gesturing NO
- PersonGesturingOk EmojiWithTone = newEmojiWithTone("\U0001f646@") // person gesturing OK
- ManGesturingOk EmojiWithTone = newEmojiWithTone("\U0001f646@\u200d\u2642\ufe0f") // man gesturing OK
- WomanGesturingOk EmojiWithTone = newEmojiWithTone("\U0001f646@\u200d\u2640\ufe0f") // woman gesturing OK
- PersonTippingHand EmojiWithTone = newEmojiWithTone("\U0001f481@") // person tipping hand
- ManTippingHand EmojiWithTone = newEmojiWithTone("\U0001f481@\u200d\u2642\ufe0f") // man tipping hand
- WomanTippingHand EmojiWithTone = newEmojiWithTone("\U0001f481@\u200d\u2640\ufe0f") // woman tipping hand
- PersonRaisingHand EmojiWithTone = newEmojiWithTone("\U0001f64b@") // person raising hand
- ManRaisingHand EmojiWithTone = newEmojiWithTone("\U0001f64b@\u200d\u2642\ufe0f") // man raising hand
- WomanRaisingHand EmojiWithTone = newEmojiWithTone("\U0001f64b@\u200d\u2640\ufe0f") // woman raising hand
- DeafPerson EmojiWithTone = newEmojiWithTone("\U0001f9cf@") // deaf person
- DeafMan EmojiWithTone = newEmojiWithTone("\U0001f9cf@\u200d\u2642\ufe0f") // deaf man
- DeafWoman EmojiWithTone = newEmojiWithTone("\U0001f9cf@\u200d\u2640\ufe0f") // deaf woman
- PersonBowing EmojiWithTone = newEmojiWithTone("\U0001f647@") // person bowing
- ManBowing EmojiWithTone = newEmojiWithTone("\U0001f647@\u200d\u2642\ufe0f") // man bowing
- WomanBowing EmojiWithTone = newEmojiWithTone("\U0001f647@\u200d\u2640\ufe0f") // woman bowing
- PersonFacepalming EmojiWithTone = newEmojiWithTone("\U0001f926@") // person facepalming
- ManFacepalming EmojiWithTone = newEmojiWithTone("\U0001f926@\u200d\u2642\ufe0f") // man facepalming
- WomanFacepalming EmojiWithTone = newEmojiWithTone("\U0001f926@\u200d\u2640\ufe0f") // woman facepalming
- PersonShrugging EmojiWithTone = newEmojiWithTone("\U0001f937@") // person shrugging
- ManShrugging EmojiWithTone = newEmojiWithTone("\U0001f937@\u200d\u2642\ufe0f") // man shrugging
- WomanShrugging EmojiWithTone = newEmojiWithTone("\U0001f937@\u200d\u2640\ufe0f") // woman shrugging
- // SUBGROUP: person-role
- HealthWorker EmojiWithTone = newEmojiWithTone("\U0001f9d1@\u200d\u2695\ufe0f") // health worker
- ManHealthWorker EmojiWithTone = newEmojiWithTone("\U0001f468@\u200d\u2695\ufe0f") // man health worker
- WomanHealthWorker EmojiWithTone = newEmojiWithTone("\U0001f469@\u200d\u2695\ufe0f") // woman health worker
- Student EmojiWithTone = newEmojiWithTone("\U0001f9d1@\u200d\U0001f393") // student
- ManStudent EmojiWithTone = newEmojiWithTone("\U0001f468@\u200d\U0001f393") // man student
- WomanStudent EmojiWithTone = newEmojiWithTone("\U0001f469@\u200d\U0001f393") // woman student
- Teacher EmojiWithTone = newEmojiWithTone("\U0001f9d1@\u200d\U0001f3eb") // teacher
- ManTeacher EmojiWithTone = newEmojiWithTone("\U0001f468@\u200d\U0001f3eb") // man teacher
- WomanTeacher EmojiWithTone = newEmojiWithTone("\U0001f469@\u200d\U0001f3eb") // woman teacher
- Judge EmojiWithTone = newEmojiWithTone("\U0001f9d1@\u200d\u2696\ufe0f") // judge
- ManJudge EmojiWithTone = newEmojiWithTone("\U0001f468@\u200d\u2696\ufe0f") // man judge
- WomanJudge EmojiWithTone = newEmojiWithTone("\U0001f469@\u200d\u2696\ufe0f") // woman judge
- Farmer EmojiWithTone = newEmojiWithTone("\U0001f9d1@\u200d\U0001f33e") // farmer
- ManFarmer EmojiWithTone = newEmojiWithTone("\U0001f468@\u200d\U0001f33e") // man farmer
- WomanFarmer EmojiWithTone = newEmojiWithTone("\U0001f469@\u200d\U0001f33e") // woman farmer
- Cook EmojiWithTone = newEmojiWithTone("\U0001f9d1@\u200d\U0001f373") // cook
- ManCook EmojiWithTone = newEmojiWithTone("\U0001f468@\u200d\U0001f373") // man cook
- WomanCook EmojiWithTone = newEmojiWithTone("\U0001f469@\u200d\U0001f373") // woman cook
- Mechanic EmojiWithTone = newEmojiWithTone("\U0001f9d1@\u200d\U0001f527") // mechanic
- ManMechanic EmojiWithTone = newEmojiWithTone("\U0001f468@\u200d\U0001f527") // man mechanic
- WomanMechanic EmojiWithTone = newEmojiWithTone("\U0001f469@\u200d\U0001f527") // woman mechanic
- FactoryWorker EmojiWithTone = newEmojiWithTone("\U0001f9d1@\u200d\U0001f3ed") // factory worker
- ManFactoryWorker EmojiWithTone = newEmojiWithTone("\U0001f468@\u200d\U0001f3ed") // man factory worker
- WomanFactoryWorker EmojiWithTone = newEmojiWithTone("\U0001f469@\u200d\U0001f3ed") // woman factory worker
- OfficeWorker EmojiWithTone = newEmojiWithTone("\U0001f9d1@\u200d\U0001f4bc") // office worker
- ManOfficeWorker EmojiWithTone = newEmojiWithTone("\U0001f468@\u200d\U0001f4bc") // man office worker
- WomanOfficeWorker EmojiWithTone = newEmojiWithTone("\U0001f469@\u200d\U0001f4bc") // woman office worker
- Scientist EmojiWithTone = newEmojiWithTone("\U0001f9d1@\u200d\U0001f52c") // scientist
- ManScientist EmojiWithTone = newEmojiWithTone("\U0001f468@\u200d\U0001f52c") // man scientist
- WomanScientist EmojiWithTone = newEmojiWithTone("\U0001f469@\u200d\U0001f52c") // woman scientist
- Technologist EmojiWithTone = newEmojiWithTone("\U0001f9d1@\u200d\U0001f4bb") // technologist
- ManTechnologist EmojiWithTone = newEmojiWithTone("\U0001f468@\u200d\U0001f4bb") // man technologist
- WomanTechnologist EmojiWithTone = newEmojiWithTone("\U0001f469@\u200d\U0001f4bb") // woman technologist
- Singer EmojiWithTone = newEmojiWithTone("\U0001f9d1@\u200d\U0001f3a4") // singer
- ManSinger EmojiWithTone = newEmojiWithTone("\U0001f468@\u200d\U0001f3a4") // man singer
- WomanSinger EmojiWithTone = newEmojiWithTone("\U0001f469@\u200d\U0001f3a4") // woman singer
- Artist EmojiWithTone = newEmojiWithTone("\U0001f9d1@\u200d\U0001f3a8") // artist
- ManArtist EmojiWithTone = newEmojiWithTone("\U0001f468@\u200d\U0001f3a8") // man artist
- WomanArtist EmojiWithTone = newEmojiWithTone("\U0001f469@\u200d\U0001f3a8") // woman artist
- Pilot EmojiWithTone = newEmojiWithTone("\U0001f9d1@\u200d\u2708\ufe0f") // pilot
- ManPilot EmojiWithTone = newEmojiWithTone("\U0001f468@\u200d\u2708\ufe0f") // man pilot
- WomanPilot EmojiWithTone = newEmojiWithTone("\U0001f469@\u200d\u2708\ufe0f") // woman pilot
- Astronaut EmojiWithTone = newEmojiWithTone("\U0001f9d1@\u200d\U0001f680") // astronaut
- ManAstronaut EmojiWithTone = newEmojiWithTone("\U0001f468@\u200d\U0001f680") // man astronaut
- WomanAstronaut EmojiWithTone = newEmojiWithTone("\U0001f469@\u200d\U0001f680") // woman astronaut
- Firefighter EmojiWithTone = newEmojiWithTone("\U0001f9d1@\u200d\U0001f692") // firefighter
- ManFirefighter EmojiWithTone = newEmojiWithTone("\U0001f468@\u200d\U0001f692") // man firefighter
- WomanFirefighter EmojiWithTone = newEmojiWithTone("\U0001f469@\u200d\U0001f692") // woman firefighter
- PoliceOfficer EmojiWithTone = newEmojiWithTone("\U0001f46e@") // police officer
- ManPoliceOfficer EmojiWithTone = newEmojiWithTone("\U0001f46e@\u200d\u2642\ufe0f") // man police officer
- WomanPoliceOfficer EmojiWithTone = newEmojiWithTone("\U0001f46e@\u200d\u2640\ufe0f") // woman police officer
- Detective EmojiWithTone = newEmojiWithTone("\U0001f575@").withDefaultTone("\ufe0f") // detective
- ManDetective EmojiWithTone = newEmojiWithTone("\U0001f575@\u200d\u2642\ufe0f").withDefaultTone("\ufe0f") // man detective
- WomanDetective EmojiWithTone = newEmojiWithTone("\U0001f575@\u200d\u2640\ufe0f").withDefaultTone("\ufe0f") // woman detective
- Guard EmojiWithTone = newEmojiWithTone("\U0001f482@") // guard
- ManGuard EmojiWithTone = newEmojiWithTone("\U0001f482@\u200d\u2642\ufe0f") // man guard
- WomanGuard EmojiWithTone = newEmojiWithTone("\U0001f482@\u200d\u2640\ufe0f") // woman guard
- Ninja EmojiWithTone = newEmojiWithTone("\U0001f977@") // ninja
- ConstructionWorker EmojiWithTone = newEmojiWithTone("\U0001f477@") // construction worker
- ManConstructionWorker EmojiWithTone = newEmojiWithTone("\U0001f477@\u200d\u2642\ufe0f") // man construction worker
- WomanConstructionWorker EmojiWithTone = newEmojiWithTone("\U0001f477@\u200d\u2640\ufe0f") // woman construction worker
- Prince EmojiWithTone = newEmojiWithTone("\U0001f934@") // prince
- Princess EmojiWithTone = newEmojiWithTone("\U0001f478@") // princess
- PersonWearingTurban EmojiWithTone = newEmojiWithTone("\U0001f473@") // person wearing turban
- ManWearingTurban EmojiWithTone = newEmojiWithTone("\U0001f473@\u200d\u2642\ufe0f") // man wearing turban
- WomanWearingTurban EmojiWithTone = newEmojiWithTone("\U0001f473@\u200d\u2640\ufe0f") // woman wearing turban
- PersonWithSkullcap EmojiWithTone = newEmojiWithTone("\U0001f472@") // person with skullcap
- WomanWithHeadscarf EmojiWithTone = newEmojiWithTone("\U0001f9d5@") // woman with headscarf
- PersonInTuxedo EmojiWithTone = newEmojiWithTone("\U0001f935@") // person in tuxedo
- ManInTuxedo EmojiWithTone = newEmojiWithTone("\U0001f935@\u200d\u2642\ufe0f") // man in tuxedo
- WomanInTuxedo EmojiWithTone = newEmojiWithTone("\U0001f935@\u200d\u2640\ufe0f") // woman in tuxedo
- PersonWithVeil EmojiWithTone = newEmojiWithTone("\U0001f470@") // person with veil
- ManWithVeil EmojiWithTone = newEmojiWithTone("\U0001f470@\u200d\u2642\ufe0f") // man with veil
- WomanWithVeil EmojiWithTone = newEmojiWithTone("\U0001f470@\u200d\u2640\ufe0f") // woman with veil
- PregnantWoman EmojiWithTone = newEmojiWithTone("\U0001f930@") // pregnant woman
- BreastFeeding EmojiWithTone = newEmojiWithTone("\U0001f931@") // breast-feeding
- WomanFeedingBaby EmojiWithTone = newEmojiWithTone("\U0001f469@\u200d\U0001f37c") // woman feeding baby
- ManFeedingBaby EmojiWithTone = newEmojiWithTone("\U0001f468@\u200d\U0001f37c") // man feeding baby
- PersonFeedingBaby EmojiWithTone = newEmojiWithTone("\U0001f9d1@\u200d\U0001f37c") // person feeding baby
- // SUBGROUP: person-fantasy
- BabyAngel EmojiWithTone = newEmojiWithTone("\U0001f47c@") // baby angel
- SantaClaus EmojiWithTone = newEmojiWithTone("\U0001f385@") // Santa Claus
- MrsClaus EmojiWithTone = newEmojiWithTone("\U0001f936@") // Mrs. Claus
- MxClaus EmojiWithTone = newEmojiWithTone("\U0001f9d1@\u200d\U0001f384") // mx claus
- Superhero EmojiWithTone = newEmojiWithTone("\U0001f9b8@") // superhero
- ManSuperhero EmojiWithTone = newEmojiWithTone("\U0001f9b8@\u200d\u2642\ufe0f") // man superhero
- WomanSuperhero EmojiWithTone = newEmojiWithTone("\U0001f9b8@\u200d\u2640\ufe0f") // woman superhero
- Supervillain EmojiWithTone = newEmojiWithTone("\U0001f9b9@") // supervillain
- ManSupervillain EmojiWithTone = newEmojiWithTone("\U0001f9b9@\u200d\u2642\ufe0f") // man supervillain
- WomanSupervillain EmojiWithTone = newEmojiWithTone("\U0001f9b9@\u200d\u2640\ufe0f") // woman supervillain
- Mage EmojiWithTone = newEmojiWithTone("\U0001f9d9@") // mage
- ManMage EmojiWithTone = newEmojiWithTone("\U0001f9d9@\u200d\u2642\ufe0f") // man mage
- WomanMage EmojiWithTone = newEmojiWithTone("\U0001f9d9@\u200d\u2640\ufe0f") // woman mage
- Fairy EmojiWithTone = newEmojiWithTone("\U0001f9da@") // fairy
- ManFairy EmojiWithTone = newEmojiWithTone("\U0001f9da@\u200d\u2642\ufe0f") // man fairy
- WomanFairy EmojiWithTone = newEmojiWithTone("\U0001f9da@\u200d\u2640\ufe0f") // woman fairy
- Vampire EmojiWithTone = newEmojiWithTone("\U0001f9db@") // vampire
- ManVampire EmojiWithTone = newEmojiWithTone("\U0001f9db@\u200d\u2642\ufe0f") // man vampire
- WomanVampire EmojiWithTone = newEmojiWithTone("\U0001f9db@\u200d\u2640\ufe0f") // woman vampire
- Merperson EmojiWithTone = newEmojiWithTone("\U0001f9dc@") // merperson
- Merman EmojiWithTone = newEmojiWithTone("\U0001f9dc@\u200d\u2642\ufe0f") // merman
- Mermaid EmojiWithTone = newEmojiWithTone("\U0001f9dc@\u200d\u2640\ufe0f") // mermaid
- Elf EmojiWithTone = newEmojiWithTone("\U0001f9dd@") // elf
- ManElf EmojiWithTone = newEmojiWithTone("\U0001f9dd@\u200d\u2642\ufe0f") // man elf
- WomanElf EmojiWithTone = newEmojiWithTone("\U0001f9dd@\u200d\u2640\ufe0f") // woman elf
- Genie Emoji = "\U0001f9de" // genie
- ManGenie Emoji = "\U0001f9de\u200d\u2642\ufe0f" // man genie
- WomanGenie Emoji = "\U0001f9de\u200d\u2640\ufe0f" // woman genie
- Zombie Emoji = "\U0001f9df" // zombie
- ManZombie Emoji = "\U0001f9df\u200d\u2642\ufe0f" // man zombie
- WomanZombie Emoji = "\U0001f9df\u200d\u2640\ufe0f" // woman zombie
- // SUBGROUP: person-activity
- PersonGettingMassage EmojiWithTone = newEmojiWithTone("\U0001f486@") // person getting massage
- ManGettingMassage EmojiWithTone = newEmojiWithTone("\U0001f486@\u200d\u2642\ufe0f") // man getting massage
- WomanGettingMassage EmojiWithTone = newEmojiWithTone("\U0001f486@\u200d\u2640\ufe0f") // woman getting massage
- PersonGettingHaircut EmojiWithTone = newEmojiWithTone("\U0001f487@") // person getting haircut
- ManGettingHaircut EmojiWithTone = newEmojiWithTone("\U0001f487@\u200d\u2642\ufe0f") // man getting haircut
- WomanGettingHaircut EmojiWithTone = newEmojiWithTone("\U0001f487@\u200d\u2640\ufe0f") // woman getting haircut
- PersonWalking EmojiWithTone = newEmojiWithTone("\U0001f6b6@") // person walking
- ManWalking EmojiWithTone = newEmojiWithTone("\U0001f6b6@\u200d\u2642\ufe0f") // man walking
- WomanWalking EmojiWithTone = newEmojiWithTone("\U0001f6b6@\u200d\u2640\ufe0f") // woman walking
- PersonStanding EmojiWithTone = newEmojiWithTone("\U0001f9cd@") // person standing
- ManStanding EmojiWithTone = newEmojiWithTone("\U0001f9cd@\u200d\u2642\ufe0f") // man standing
- WomanStanding EmojiWithTone = newEmojiWithTone("\U0001f9cd@\u200d\u2640\ufe0f") // woman standing
- PersonKneeling EmojiWithTone = newEmojiWithTone("\U0001f9ce@") // person kneeling
- ManKneeling EmojiWithTone = newEmojiWithTone("\U0001f9ce@\u200d\u2642\ufe0f") // man kneeling
- WomanKneeling EmojiWithTone = newEmojiWithTone("\U0001f9ce@\u200d\u2640\ufe0f") // woman kneeling
- PersonWithWhiteCane EmojiWithTone = newEmojiWithTone("\U0001f9d1@\u200d\U0001f9af") // person with white cane
- ManWithWhiteCane EmojiWithTone = newEmojiWithTone("\U0001f468@\u200d\U0001f9af") // man with white cane
- WomanWithWhiteCane EmojiWithTone = newEmojiWithTone("\U0001f469@\u200d\U0001f9af") // woman with white cane
- PersonInMotorizedWheelchair EmojiWithTone = newEmojiWithTone("\U0001f9d1@\u200d\U0001f9bc") // person in motorized wheelchair
- ManInMotorizedWheelchair EmojiWithTone = newEmojiWithTone("\U0001f468@\u200d\U0001f9bc") // man in motorized wheelchair
- WomanInMotorizedWheelchair EmojiWithTone = newEmojiWithTone("\U0001f469@\u200d\U0001f9bc") // woman in motorized wheelchair
- PersonInManualWheelchair EmojiWithTone = newEmojiWithTone("\U0001f9d1@\u200d\U0001f9bd") // person in manual wheelchair
- ManInManualWheelchair EmojiWithTone = newEmojiWithTone("\U0001f468@\u200d\U0001f9bd") // man in manual wheelchair
- WomanInManualWheelchair EmojiWithTone = newEmojiWithTone("\U0001f469@\u200d\U0001f9bd") // woman in manual wheelchair
- PersonRunning EmojiWithTone = newEmojiWithTone("\U0001f3c3@") // person running
- ManRunning EmojiWithTone = newEmojiWithTone("\U0001f3c3@\u200d\u2642\ufe0f") // man running
- WomanRunning EmojiWithTone = newEmojiWithTone("\U0001f3c3@\u200d\u2640\ufe0f") // woman running
- WomanDancing EmojiWithTone = newEmojiWithTone("\U0001f483@") // woman dancing
- ManDancing EmojiWithTone = newEmojiWithTone("\U0001f57a@") // man dancing
- PersonInSuitLevitating EmojiWithTone = newEmojiWithTone("\U0001f574@").withDefaultTone("\ufe0f") // person in suit levitating
- PeopleWithBunnyEars Emoji = "\U0001f46f" // people with bunny ears
- MenWithBunnyEars Emoji = "\U0001f46f\u200d\u2642\ufe0f" // men with bunny ears
- WomenWithBunnyEars Emoji = "\U0001f46f\u200d\u2640\ufe0f" // women with bunny ears
- PersonInSteamyRoom EmojiWithTone = newEmojiWithTone("\U0001f9d6@") // person in steamy room
- ManInSteamyRoom EmojiWithTone = newEmojiWithTone("\U0001f9d6@\u200d\u2642\ufe0f") // man in steamy room
- WomanInSteamyRoom EmojiWithTone = newEmojiWithTone("\U0001f9d6@\u200d\u2640\ufe0f") // woman in steamy room
- PersonClimbing EmojiWithTone = newEmojiWithTone("\U0001f9d7@") // person climbing
- ManClimbing EmojiWithTone = newEmojiWithTone("\U0001f9d7@\u200d\u2642\ufe0f") // man climbing
- WomanClimbing EmojiWithTone = newEmojiWithTone("\U0001f9d7@\u200d\u2640\ufe0f") // woman climbing
- // SUBGROUP: person-sport
- PersonFencing Emoji = "\U0001f93a" // person fencing
- HorseRacing EmojiWithTone = newEmojiWithTone("\U0001f3c7@") // horse racing
- Skier Emoji = "\u26f7\ufe0f" // skier
- Snowboarder EmojiWithTone = newEmojiWithTone("\U0001f3c2@") // snowboarder
- PersonGolfing EmojiWithTone = newEmojiWithTone("\U0001f3cc@").withDefaultTone("\ufe0f") // person golfing
- ManGolfing EmojiWithTone = newEmojiWithTone("\U0001f3cc@\u200d\u2642\ufe0f").withDefaultTone("\ufe0f") // man golfing
- WomanGolfing EmojiWithTone = newEmojiWithTone("\U0001f3cc@\u200d\u2640\ufe0f").withDefaultTone("\ufe0f") // woman golfing
- PersonSurfing EmojiWithTone = newEmojiWithTone("\U0001f3c4@") // person surfing
- ManSurfing EmojiWithTone = newEmojiWithTone("\U0001f3c4@\u200d\u2642\ufe0f") // man surfing
- WomanSurfing EmojiWithTone = newEmojiWithTone("\U0001f3c4@\u200d\u2640\ufe0f") // woman surfing
- PersonRowingBoat EmojiWithTone = newEmojiWithTone("\U0001f6a3@") // person rowing boat
- ManRowingBoat EmojiWithTone = newEmojiWithTone("\U0001f6a3@\u200d\u2642\ufe0f") // man rowing boat
- WomanRowingBoat EmojiWithTone = newEmojiWithTone("\U0001f6a3@\u200d\u2640\ufe0f") // woman rowing boat
- PersonSwimming EmojiWithTone = newEmojiWithTone("\U0001f3ca@") // person swimming
- ManSwimming EmojiWithTone = newEmojiWithTone("\U0001f3ca@\u200d\u2642\ufe0f") // man swimming
- WomanSwimming EmojiWithTone = newEmojiWithTone("\U0001f3ca@\u200d\u2640\ufe0f") // woman swimming
- PersonBouncingBall EmojiWithTone = newEmojiWithTone("\u26f9@").withDefaultTone("\ufe0f") // person bouncing ball
- ManBouncingBall EmojiWithTone = newEmojiWithTone("\u26f9@\u200d\u2642\ufe0f").withDefaultTone("\ufe0f") // man bouncing ball
- WomanBouncingBall EmojiWithTone = newEmojiWithTone("\u26f9@\u200d\u2640\ufe0f").withDefaultTone("\ufe0f") // woman bouncing ball
- PersonLiftingWeights EmojiWithTone = newEmojiWithTone("\U0001f3cb@").withDefaultTone("\ufe0f") // person lifting weights
- ManLiftingWeights EmojiWithTone = newEmojiWithTone("\U0001f3cb@\u200d\u2642\ufe0f").withDefaultTone("\ufe0f") // man lifting weights
- WomanLiftingWeights EmojiWithTone = newEmojiWithTone("\U0001f3cb@\u200d\u2640\ufe0f").withDefaultTone("\ufe0f") // woman lifting weights
- PersonBiking EmojiWithTone = newEmojiWithTone("\U0001f6b4@") // person biking
- ManBiking EmojiWithTone = newEmojiWithTone("\U0001f6b4@\u200d\u2642\ufe0f") // man biking
- WomanBiking EmojiWithTone = newEmojiWithTone("\U0001f6b4@\u200d\u2640\ufe0f") // woman biking
- PersonMountainBiking EmojiWithTone = newEmojiWithTone("\U0001f6b5@") // person mountain biking
- ManMountainBiking EmojiWithTone = newEmojiWithTone("\U0001f6b5@\u200d\u2642\ufe0f") // man mountain biking
- WomanMountainBiking EmojiWithTone = newEmojiWithTone("\U0001f6b5@\u200d\u2640\ufe0f") // woman mountain biking
- PersonCartwheeling EmojiWithTone = newEmojiWithTone("\U0001f938@") // person cartwheeling
- ManCartwheeling EmojiWithTone = newEmojiWithTone("\U0001f938@\u200d\u2642\ufe0f") // man cartwheeling
- WomanCartwheeling EmojiWithTone = newEmojiWithTone("\U0001f938@\u200d\u2640\ufe0f") // woman cartwheeling
- PeopleWrestling Emoji = "\U0001f93c" // people wrestling
- MenWrestling Emoji = "\U0001f93c\u200d\u2642\ufe0f" // men wrestling
- WomenWrestling Emoji = "\U0001f93c\u200d\u2640\ufe0f" // women wrestling
- PersonPlayingWaterPolo EmojiWithTone = newEmojiWithTone("\U0001f93d@") // person playing water polo
- ManPlayingWaterPolo EmojiWithTone = newEmojiWithTone("\U0001f93d@\u200d\u2642\ufe0f") // man playing water polo
- WomanPlayingWaterPolo EmojiWithTone = newEmojiWithTone("\U0001f93d@\u200d\u2640\ufe0f") // woman playing water polo
- PersonPlayingHandball EmojiWithTone = newEmojiWithTone("\U0001f93e@") // person playing handball
- ManPlayingHandball EmojiWithTone = newEmojiWithTone("\U0001f93e@\u200d\u2642\ufe0f") // man playing handball
- WomanPlayingHandball EmojiWithTone = newEmojiWithTone("\U0001f93e@\u200d\u2640\ufe0f") // woman playing handball
- PersonJuggling EmojiWithTone = newEmojiWithTone("\U0001f939@") // person juggling
- ManJuggling EmojiWithTone = newEmojiWithTone("\U0001f939@\u200d\u2642\ufe0f") // man juggling
- WomanJuggling EmojiWithTone = newEmojiWithTone("\U0001f939@\u200d\u2640\ufe0f") // woman juggling
- // SUBGROUP: person-resting
- PersonInLotusPosition EmojiWithTone = newEmojiWithTone("\U0001f9d8@") // person in lotus position
- ManInLotusPosition EmojiWithTone = newEmojiWithTone("\U0001f9d8@\u200d\u2642\ufe0f") // man in lotus position
- WomanInLotusPosition EmojiWithTone = newEmojiWithTone("\U0001f9d8@\u200d\u2640\ufe0f") // woman in lotus position
- PersonTakingBath EmojiWithTone = newEmojiWithTone("\U0001f6c0@") // person taking bath
- PersonInBed EmojiWithTone = newEmojiWithTone("\U0001f6cc@") // person in bed
- // SUBGROUP: family
- PeopleHoldingHands EmojiWithTone = newEmojiWithTone("\U0001f9d1@\u200d\U0001f91d\u200d\U0001f9d1@", "\U0001f9d1@\u200d\U0001f91d\u200d\U0001f9d1@") // people holding hands
- WomenHoldingHands EmojiWithTone = newEmojiWithTone("\U0001f46d@", "\U0001f469@\u200d\U0001f91d\u200d\U0001f469@") // women holding hands
- WomanAndManHoldingHands EmojiWithTone = newEmojiWithTone("\U0001f46b@", "\U0001f469@\u200d\U0001f91d\u200d\U0001f468@") // woman and man holding hands
- MenHoldingHands EmojiWithTone = newEmojiWithTone("\U0001f46c@", "\U0001f468@\u200d\U0001f91d\u200d\U0001f468@") // men holding hands
- Kiss Emoji = "\U0001f48f" // kiss
- KissWomanMan Emoji = "\U0001f469\u200d\u2764\ufe0f\u200d\U0001f48b\u200d\U0001f468" // kiss: woman, man
- KissManMan Emoji = "\U0001f468\u200d\u2764\ufe0f\u200d\U0001f48b\u200d\U0001f468" // kiss: man, man
- KissWomanWoman Emoji = "\U0001f469\u200d\u2764\ufe0f\u200d\U0001f48b\u200d\U0001f469" // kiss: woman, woman
- CoupleWithHeart Emoji = "\U0001f491" // couple with heart
- CoupleWithHeartWomanMan Emoji = "\U0001f469\u200d\u2764\ufe0f\u200d\U0001f468" // couple with heart: woman, man
- CoupleWithHeartManMan Emoji = "\U0001f468\u200d\u2764\ufe0f\u200d\U0001f468" // couple with heart: man, man
- CoupleWithHeartWomanWoman Emoji = "\U0001f469\u200d\u2764\ufe0f\u200d\U0001f469" // couple with heart: woman, woman
- Family Emoji = "\U0001f46a" // family
- FamilyManWomanBoy Emoji = "\U0001f468\u200d\U0001f469\u200d\U0001f466" // family: man, woman, boy
- FamilyManWomanGirl Emoji = "\U0001f468\u200d\U0001f469\u200d\U0001f467" // family: man, woman, girl
- FamilyManWomanGirlBoy Emoji = "\U0001f468\u200d\U0001f469\u200d\U0001f467\u200d\U0001f466" // family: man, woman, girl, boy
- FamilyManWomanBoyBoy Emoji = "\U0001f468\u200d\U0001f469\u200d\U0001f466\u200d\U0001f466" // family: man, woman, boy, boy
- FamilyManWomanGirlGirl Emoji = "\U0001f468\u200d\U0001f469\u200d\U0001f467\u200d\U0001f467" // family: man, woman, girl, girl
- FamilyManManBoy Emoji = "\U0001f468\u200d\U0001f468\u200d\U0001f466" // family: man, man, boy
- FamilyManManGirl Emoji = "\U0001f468\u200d\U0001f468\u200d\U0001f467" // family: man, man, girl
- FamilyManManGirlBoy Emoji = "\U0001f468\u200d\U0001f468\u200d\U0001f467\u200d\U0001f466" // family: man, man, girl, boy
- FamilyManManBoyBoy Emoji = "\U0001f468\u200d\U0001f468\u200d\U0001f466\u200d\U0001f466" // family: man, man, boy, boy
- FamilyManManGirlGirl Emoji = "\U0001f468\u200d\U0001f468\u200d\U0001f467\u200d\U0001f467" // family: man, man, girl, girl
- FamilyWomanWomanBoy Emoji = "\U0001f469\u200d\U0001f469\u200d\U0001f466" // family: woman, woman, boy
- FamilyWomanWomanGirl Emoji = "\U0001f469\u200d\U0001f469\u200d\U0001f467" // family: woman, woman, girl
- FamilyWomanWomanGirlBoy Emoji = "\U0001f469\u200d\U0001f469\u200d\U0001f467\u200d\U0001f466" // family: woman, woman, girl, boy
- FamilyWomanWomanBoyBoy Emoji = "\U0001f469\u200d\U0001f469\u200d\U0001f466\u200d\U0001f466" // family: woman, woman, boy, boy
- FamilyWomanWomanGirlGirl Emoji = "\U0001f469\u200d\U0001f469\u200d\U0001f467\u200d\U0001f467" // family: woman, woman, girl, girl
- FamilyManBoy Emoji = "\U0001f468\u200d\U0001f466" // family: man, boy
- FamilyManBoyBoy Emoji = "\U0001f468\u200d\U0001f466\u200d\U0001f466" // family: man, boy, boy
- FamilyManGirl Emoji = "\U0001f468\u200d\U0001f467" // family: man, girl
- FamilyManGirlBoy Emoji = "\U0001f468\u200d\U0001f467\u200d\U0001f466" // family: man, girl, boy
- FamilyManGirlGirl Emoji = "\U0001f468\u200d\U0001f467\u200d\U0001f467" // family: man, girl, girl
- FamilyWomanBoy Emoji = "\U0001f469\u200d\U0001f466" // family: woman, boy
- FamilyWomanBoyBoy Emoji = "\U0001f469\u200d\U0001f466\u200d\U0001f466" // family: woman, boy, boy
- FamilyWomanGirl Emoji = "\U0001f469\u200d\U0001f467" // family: woman, girl
- FamilyWomanGirlBoy Emoji = "\U0001f469\u200d\U0001f467\u200d\U0001f466" // family: woman, girl, boy
- FamilyWomanGirlGirl Emoji = "\U0001f469\u200d\U0001f467\u200d\U0001f467" // family: woman, girl, girl
- // SUBGROUP: person-symbol
- SpeakingHead Emoji = "\U0001f5e3\ufe0f" // speaking head
- BustInSilhouette Emoji = "\U0001f464" // bust in silhouette
- BustsInSilhouette Emoji = "\U0001f465" // busts in silhouette
- PeopleHugging Emoji = "\U0001fac2" // people hugging
- Footprints Emoji = "\U0001f463" // footprints
-
- // GROUP: Component
- // SUBGROUP: skin-tone
- LightSkinTone Emoji = "\U0001f3fb" // light skin tone
- MediumLightSkinTone Emoji = "\U0001f3fc" // medium-light skin tone
- MediumSkinTone Emoji = "\U0001f3fd" // medium skin tone
- MediumDarkSkinTone Emoji = "\U0001f3fe" // medium-dark skin tone
- DarkSkinTone Emoji = "\U0001f3ff" // dark skin tone
- // SUBGROUP: hair-style
- RedHair Emoji = "\U0001f9b0" // red hair
- CurlyHair Emoji = "\U0001f9b1" // curly hair
- WhiteHair Emoji = "\U0001f9b3" // white hair
- Bald Emoji = "\U0001f9b2" // bald
-
- // GROUP: Animals & Nature
- // SUBGROUP: animal-mammal
- MonkeyFace Emoji = "\U0001f435" // monkey face
- Monkey Emoji = "\U0001f412" // monkey
- Gorilla Emoji = "\U0001f98d" // gorilla
- Orangutan Emoji = "\U0001f9a7" // orangutan
- DogFace Emoji = "\U0001f436" // dog face
- Dog Emoji = "\U0001f415" // dog
- GuideDog Emoji = "\U0001f9ae" // guide dog
- ServiceDog Emoji = "\U0001f415\u200d\U0001f9ba" // service dog
- Poodle Emoji = "\U0001f429" // poodle
- Wolf Emoji = "\U0001f43a" // wolf
- Fox Emoji = "\U0001f98a" // fox
- Raccoon Emoji = "\U0001f99d" // raccoon
- CatFace Emoji = "\U0001f431" // cat face
- Cat Emoji = "\U0001f408" // cat
- BlackCat Emoji = "\U0001f408\u200d\u2b1b" // black cat
- Lion Emoji = "\U0001f981" // lion
- TigerFace Emoji = "\U0001f42f" // tiger face
- Tiger Emoji = "\U0001f405" // tiger
- Leopard Emoji = "\U0001f406" // leopard
- HorseFace Emoji = "\U0001f434" // horse face
- Horse Emoji = "\U0001f40e" // horse
- Unicorn Emoji = "\U0001f984" // unicorn
- Zebra Emoji = "\U0001f993" // zebra
- Deer Emoji = "\U0001f98c" // deer
- Bison Emoji = "\U0001f9ac" // bison
- CowFace Emoji = "\U0001f42e" // cow face
- Ox Emoji = "\U0001f402" // ox
- WaterBuffalo Emoji = "\U0001f403" // water buffalo
- Cow Emoji = "\U0001f404" // cow
- PigFace Emoji = "\U0001f437" // pig face
- Pig Emoji = "\U0001f416" // pig
- Boar Emoji = "\U0001f417" // boar
- PigNose Emoji = "\U0001f43d" // pig nose
- Ram Emoji = "\U0001f40f" // ram
- Ewe Emoji = "\U0001f411" // ewe
- Goat Emoji = "\U0001f410" // goat
- Camel Emoji = "\U0001f42a" // camel
- TwoHumpCamel Emoji = "\U0001f42b" // two-hump camel
- Llama Emoji = "\U0001f999" // llama
- Giraffe Emoji = "\U0001f992" // giraffe
- Elephant Emoji = "\U0001f418" // elephant
- Mammoth Emoji = "\U0001f9a3" // mammoth
- Rhinoceros Emoji = "\U0001f98f" // rhinoceros
- Hippopotamus Emoji = "\U0001f99b" // hippopotamus
- MouseFace Emoji = "\U0001f42d" // mouse face
- Mouse Emoji = "\U0001f401" // mouse
- Rat Emoji = "\U0001f400" // rat
- Hamster Emoji = "\U0001f439" // hamster
- RabbitFace Emoji = "\U0001f430" // rabbit face
- Rabbit Emoji = "\U0001f407" // rabbit
- Chipmunk Emoji = "\U0001f43f\ufe0f" // chipmunk
- Beaver Emoji = "\U0001f9ab" // beaver
- Hedgehog Emoji = "\U0001f994" // hedgehog
- Bat Emoji = "\U0001f987" // bat
- Bear Emoji = "\U0001f43b" // bear
- PolarBear Emoji = "\U0001f43b\u200d\u2744\ufe0f" // polar bear
- Koala Emoji = "\U0001f428" // koala
- Panda Emoji = "\U0001f43c" // panda
- Sloth Emoji = "\U0001f9a5" // sloth
- Otter Emoji = "\U0001f9a6" // otter
- Skunk Emoji = "\U0001f9a8" // skunk
- Kangaroo Emoji = "\U0001f998" // kangaroo
- Badger Emoji = "\U0001f9a1" // badger
- PawPrints Emoji = "\U0001f43e" // paw prints
- // SUBGROUP: animal-bird
- Turkey Emoji = "\U0001f983" // turkey
- Chicken Emoji = "\U0001f414" // chicken
- Rooster Emoji = "\U0001f413" // rooster
- HatchingChick Emoji = "\U0001f423" // hatching chick
- BabyChick Emoji = "\U0001f424" // baby chick
- FrontFacingBabyChick Emoji = "\U0001f425" // front-facing baby chick
- Bird Emoji = "\U0001f426" // bird
- Penguin Emoji = "\U0001f427" // penguin
- Dove Emoji = "\U0001f54a\ufe0f" // dove
- Eagle Emoji = "\U0001f985" // eagle
- Duck Emoji = "\U0001f986" // duck
- Swan Emoji = "\U0001f9a2" // swan
- Owl Emoji = "\U0001f989" // owl
- Dodo Emoji = "\U0001f9a4" // dodo
- Feather Emoji = "\U0001fab6" // feather
- Flamingo Emoji = "\U0001f9a9" // flamingo
- Peacock Emoji = "\U0001f99a" // peacock
- Parrot Emoji = "\U0001f99c" // parrot
- // SUBGROUP: animal-amphibian
- Frog Emoji = "\U0001f438" // frog
- // SUBGROUP: animal-reptile
- Crocodile Emoji = "\U0001f40a" // crocodile
- Turtle Emoji = "\U0001f422" // turtle
- Lizard Emoji = "\U0001f98e" // lizard
- Snake Emoji = "\U0001f40d" // snake
- DragonFace Emoji = "\U0001f432" // dragon face
- Dragon Emoji = "\U0001f409" // dragon
- Sauropod Emoji = "\U0001f995" // sauropod
- TRex Emoji = "\U0001f996" // T-Rex
- // SUBGROUP: animal-marine
- SpoutingWhale Emoji = "\U0001f433" // spouting whale
- Whale Emoji = "\U0001f40b" // whale
- Dolphin Emoji = "\U0001f42c" // dolphin
- Seal Emoji = "\U0001f9ad" // seal
- Fish Emoji = "\U0001f41f" // fish
- TropicalFish Emoji = "\U0001f420" // tropical fish
- Blowfish Emoji = "\U0001f421" // blowfish
- Shark Emoji = "\U0001f988" // shark
- Octopus Emoji = "\U0001f419" // octopus
- SpiralShell Emoji = "\U0001f41a" // spiral shell
- // SUBGROUP: animal-bug
- Snail Emoji = "\U0001f40c" // snail
- Butterfly Emoji = "\U0001f98b" // butterfly
- Bug Emoji = "\U0001f41b" // bug
- Ant Emoji = "\U0001f41c" // ant
- Honeybee Emoji = "\U0001f41d" // honeybee
- Beetle Emoji = "\U0001fab2" // beetle
- LadyBeetle Emoji = "\U0001f41e" // lady beetle
- Cricket Emoji = "\U0001f997" // cricket
- Cockroach Emoji = "\U0001fab3" // cockroach
- Spider Emoji = "\U0001f577\ufe0f" // spider
- SpiderWeb Emoji = "\U0001f578\ufe0f" // spider web
- Scorpion Emoji = "\U0001f982" // scorpion
- Mosquito Emoji = "\U0001f99f" // mosquito
- Fly Emoji = "\U0001fab0" // fly
- Worm Emoji = "\U0001fab1" // worm
- Microbe Emoji = "\U0001f9a0" // microbe
- // SUBGROUP: plant-flower
- Bouquet Emoji = "\U0001f490" // bouquet
- CherryBlossom Emoji = "\U0001f338" // cherry blossom
- WhiteFlower Emoji = "\U0001f4ae" // white flower
- Rosette Emoji = "\U0001f3f5\ufe0f" // rosette
- Rose Emoji = "\U0001f339" // rose
- WiltedFlower Emoji = "\U0001f940" // wilted flower
- Hibiscus Emoji = "\U0001f33a" // hibiscus
- Sunflower Emoji = "\U0001f33b" // sunflower
- Blossom Emoji = "\U0001f33c" // blossom
- Tulip Emoji = "\U0001f337" // tulip
- // SUBGROUP: plant-other
- Seedling Emoji = "\U0001f331" // seedling
- PottedPlant Emoji = "\U0001fab4" // potted plant
- EvergreenTree Emoji = "\U0001f332" // evergreen tree
- DeciduousTree Emoji = "\U0001f333" // deciduous tree
- PalmTree Emoji = "\U0001f334" // palm tree
- Cactus Emoji = "\U0001f335" // cactus
- SheafOfRice Emoji = "\U0001f33e" // sheaf of rice
- Herb Emoji = "\U0001f33f" // herb
- Shamrock Emoji = "\u2618\ufe0f" // shamrock
- FourLeafClover Emoji = "\U0001f340" // four leaf clover
- MapleLeaf Emoji = "\U0001f341" // maple leaf
- FallenLeaf Emoji = "\U0001f342" // fallen leaf
- LeafFlutteringInWind Emoji = "\U0001f343" // leaf fluttering in wind
-
- // GROUP: Food & Drink
- // SUBGROUP: food-fruit
- Grapes Emoji = "\U0001f347" // grapes
- Melon Emoji = "\U0001f348" // melon
- Watermelon Emoji = "\U0001f349" // watermelon
- Tangerine Emoji = "\U0001f34a" // tangerine
- Lemon Emoji = "\U0001f34b" // lemon
- Banana Emoji = "\U0001f34c" // banana
- Pineapple Emoji = "\U0001f34d" // pineapple
- Mango Emoji = "\U0001f96d" // mango
- RedApple Emoji = "\U0001f34e" // red apple
- GreenApple Emoji = "\U0001f34f" // green apple
- Pear Emoji = "\U0001f350" // pear
- Peach Emoji = "\U0001f351" // peach
- Cherries Emoji = "\U0001f352" // cherries
- Strawberry Emoji = "\U0001f353" // strawberry
- Blueberries Emoji = "\U0001fad0" // blueberries
- KiwiFruit Emoji = "\U0001f95d" // kiwi fruit
- Tomato Emoji = "\U0001f345" // tomato
- Olive Emoji = "\U0001fad2" // olive
- Coconut Emoji = "\U0001f965" // coconut
- // SUBGROUP: food-vegetable
- Avocado Emoji = "\U0001f951" // avocado
- Eggplant Emoji = "\U0001f346" // eggplant
- Potato Emoji = "\U0001f954" // potato
- Carrot Emoji = "\U0001f955" // carrot
- EarOfCorn Emoji = "\U0001f33d" // ear of corn
- HotPepper Emoji = "\U0001f336\ufe0f" // hot pepper
- BellPepper Emoji = "\U0001fad1" // bell pepper
- Cucumber Emoji = "\U0001f952" // cucumber
- LeafyGreen Emoji = "\U0001f96c" // leafy green
- Broccoli Emoji = "\U0001f966" // broccoli
- Garlic Emoji = "\U0001f9c4" // garlic
- Onion Emoji = "\U0001f9c5" // onion
- Mushroom Emoji = "\U0001f344" // mushroom
- Peanuts Emoji = "\U0001f95c" // peanuts
- Chestnut Emoji = "\U0001f330" // chestnut
- // SUBGROUP: food-prepared
- Bread Emoji = "\U0001f35e" // bread
- Croissant Emoji = "\U0001f950" // croissant
- BaguetteBread Emoji = "\U0001f956" // baguette bread
- Flatbread Emoji = "\U0001fad3" // flatbread
- Pretzel Emoji = "\U0001f968" // pretzel
- Bagel Emoji = "\U0001f96f" // bagel
- Pancakes Emoji = "\U0001f95e" // pancakes
- Waffle Emoji = "\U0001f9c7" // waffle
- CheeseWedge Emoji = "\U0001f9c0" // cheese wedge
- MeatOnBone Emoji = "\U0001f356" // meat on bone
- PoultryLeg Emoji = "\U0001f357" // poultry leg
- CutOfMeat Emoji = "\U0001f969" // cut of meat
- Bacon Emoji = "\U0001f953" // bacon
- Hamburger Emoji = "\U0001f354" // hamburger
- FrenchFries Emoji = "\U0001f35f" // french fries
- Pizza Emoji = "\U0001f355" // pizza
- HotDog Emoji = "\U0001f32d" // hot dog
- Sandwich Emoji = "\U0001f96a" // sandwich
- Taco Emoji = "\U0001f32e" // taco
- Burrito Emoji = "\U0001f32f" // burrito
- Tamale Emoji = "\U0001fad4" // tamale
- StuffedFlatbread Emoji = "\U0001f959" // stuffed flatbread
- Falafel Emoji = "\U0001f9c6" // falafel
- Egg Emoji = "\U0001f95a" // egg
- Cooking Emoji = "\U0001f373" // cooking
- ShallowPanOfFood Emoji = "\U0001f958" // shallow pan of food
- PotOfFood Emoji = "\U0001f372" // pot of food
- Fondue Emoji = "\U0001fad5" // fondue
- BowlWithSpoon Emoji = "\U0001f963" // bowl with spoon
- GreenSalad Emoji = "\U0001f957" // green salad
- Popcorn Emoji = "\U0001f37f" // popcorn
- Butter Emoji = "\U0001f9c8" // butter
- Salt Emoji = "\U0001f9c2" // salt
- CannedFood Emoji = "\U0001f96b" // canned food
- // SUBGROUP: food-asian
- BentoBox Emoji = "\U0001f371" // bento box
- RiceCracker Emoji = "\U0001f358" // rice cracker
- RiceBall Emoji = "\U0001f359" // rice ball
- CookedRice Emoji = "\U0001f35a" // cooked rice
- CurryRice Emoji = "\U0001f35b" // curry rice
- SteamingBowl Emoji = "\U0001f35c" // steaming bowl
- Spaghetti Emoji = "\U0001f35d" // spaghetti
- RoastedSweetPotato Emoji = "\U0001f360" // roasted sweet potato
- Oden Emoji = "\U0001f362" // oden
- Sushi Emoji = "\U0001f363" // sushi
- FriedShrimp Emoji = "\U0001f364" // fried shrimp
- FishCakeWithSwirl Emoji = "\U0001f365" // fish cake with swirl
- MoonCake Emoji = "\U0001f96e" // moon cake
- Dango Emoji = "\U0001f361" // dango
- Dumpling Emoji = "\U0001f95f" // dumpling
- FortuneCookie Emoji = "\U0001f960" // fortune cookie
- TakeoutBox Emoji = "\U0001f961" // takeout box
- // SUBGROUP: food-marine
- Crab Emoji = "\U0001f980" // crab
- Lobster Emoji = "\U0001f99e" // lobster
- Shrimp Emoji = "\U0001f990" // shrimp
- Squid Emoji = "\U0001f991" // squid
- Oyster Emoji = "\U0001f9aa" // oyster
- // SUBGROUP: food-sweet
- SoftIceCream Emoji = "\U0001f366" // soft ice cream
- ShavedIce Emoji = "\U0001f367" // shaved ice
- IceCream Emoji = "\U0001f368" // ice cream
- Doughnut Emoji = "\U0001f369" // doughnut
- Cookie Emoji = "\U0001f36a" // cookie
- BirthdayCake Emoji = "\U0001f382" // birthday cake
- Shortcake Emoji = "\U0001f370" // shortcake
- Cupcake Emoji = "\U0001f9c1" // cupcake
- Pie Emoji = "\U0001f967" // pie
- ChocolateBar Emoji = "\U0001f36b" // chocolate bar
- Candy Emoji = "\U0001f36c" // candy
- Lollipop Emoji = "\U0001f36d" // lollipop
- Custard Emoji = "\U0001f36e" // custard
- HoneyPot Emoji = "\U0001f36f" // honey pot
- // SUBGROUP: drink
- BabyBottle Emoji = "\U0001f37c" // baby bottle
- GlassOfMilk Emoji = "\U0001f95b" // glass of milk
- HotBeverage Emoji = "\u2615" // hot beverage
- Teapot Emoji = "\U0001fad6" // teapot
- TeacupWithoutHandle Emoji = "\U0001f375" // teacup without handle
- Sake Emoji = "\U0001f376" // sake
- BottleWithPoppingCork Emoji = "\U0001f37e" // bottle with popping cork
- WineGlass Emoji = "\U0001f377" // wine glass
- CocktailGlass Emoji = "\U0001f378" // cocktail glass
- TropicalDrink Emoji = "\U0001f379" // tropical drink
- BeerMug Emoji = "\U0001f37a" // beer mug
- ClinkingBeerMugs Emoji = "\U0001f37b" // clinking beer mugs
- ClinkingGlasses Emoji = "\U0001f942" // clinking glasses
- TumblerGlass Emoji = "\U0001f943" // tumbler glass
- CupWithStraw Emoji = "\U0001f964" // cup with straw
- BubbleTea Emoji = "\U0001f9cb" // bubble tea
- BeverageBox Emoji = "\U0001f9c3" // beverage box
- Mate Emoji = "\U0001f9c9" // mate
- Ice Emoji = "\U0001f9ca" // ice
- // SUBGROUP: dishware
- Chopsticks Emoji = "\U0001f962" // chopsticks
- ForkAndKnifeWithPlate Emoji = "\U0001f37d\ufe0f" // fork and knife with plate
- ForkAndKnife Emoji = "\U0001f374" // fork and knife
- Spoon Emoji = "\U0001f944" // spoon
- KitchenKnife Emoji = "\U0001f52a" // kitchen knife
- Amphora Emoji = "\U0001f3fa" // amphora
-
- // GROUP: Travel & Places
- // SUBGROUP: place-map
- GlobeShowingEuropeAfrica Emoji = "\U0001f30d" // globe showing Europe-Africa
- GlobeShowingAmericas Emoji = "\U0001f30e" // globe showing Americas
- GlobeShowingAsiaAustralia Emoji = "\U0001f30f" // globe showing Asia-Australia
- GlobeWithMeridians Emoji = "\U0001f310" // globe with meridians
- WorldMap Emoji = "\U0001f5fa\ufe0f" // world map
- MapOfJapan Emoji = "\U0001f5fe" // map of Japan
- Compass Emoji = "\U0001f9ed" // compass
- // SUBGROUP: place-geographic
- SnowCappedMountain Emoji = "\U0001f3d4\ufe0f" // snow-capped mountain
- Mountain Emoji = "\u26f0\ufe0f" // mountain
- Volcano Emoji = "\U0001f30b" // volcano
- MountFuji Emoji = "\U0001f5fb" // mount fuji
- Camping Emoji = "\U0001f3d5\ufe0f" // camping
- BeachWithUmbrella Emoji = "\U0001f3d6\ufe0f" // beach with umbrella
- Desert Emoji = "\U0001f3dc\ufe0f" // desert
- DesertIsland Emoji = "\U0001f3dd\ufe0f" // desert island
- NationalPark Emoji = "\U0001f3de\ufe0f" // national park
- // SUBGROUP: place-building
- Stadium Emoji = "\U0001f3df\ufe0f" // stadium
- ClassicalBuilding Emoji = "\U0001f3db\ufe0f" // classical building
- BuildingConstruction Emoji = "\U0001f3d7\ufe0f" // building construction
- Brick Emoji = "\U0001f9f1" // brick
- Rock Emoji = "\U0001faa8" // rock
- Wood Emoji = "\U0001fab5" // wood
- Hut Emoji = "\U0001f6d6" // hut
- Houses Emoji = "\U0001f3d8\ufe0f" // houses
- DerelictHouse Emoji = "\U0001f3da\ufe0f" // derelict house
- House Emoji = "\U0001f3e0" // house
- HouseWithGarden Emoji = "\U0001f3e1" // house with garden
- OfficeBuilding Emoji = "\U0001f3e2" // office building
- JapanesePostOffice Emoji = "\U0001f3e3" // Japanese post office
- PostOffice Emoji = "\U0001f3e4" // post office
- Hospital Emoji = "\U0001f3e5" // hospital
- Bank Emoji = "\U0001f3e6" // bank
- Hotel Emoji = "\U0001f3e8" // hotel
- LoveHotel Emoji = "\U0001f3e9" // love hotel
- ConvenienceStore Emoji = "\U0001f3ea" // convenience store
- School Emoji = "\U0001f3eb" // school
- DepartmentStore Emoji = "\U0001f3ec" // department store
- Factory Emoji = "\U0001f3ed" // factory
- JapaneseCastle Emoji = "\U0001f3ef" // Japanese castle
- Castle Emoji = "\U0001f3f0" // castle
- Wedding Emoji = "\U0001f492" // wedding
- TokyoTower Emoji = "\U0001f5fc" // Tokyo tower
- StatueOfLiberty Emoji = "\U0001f5fd" // Statue of Liberty
- // SUBGROUP: place-religious
- Church Emoji = "\u26ea" // church
- Mosque Emoji = "\U0001f54c" // mosque
- HinduTemple Emoji = "\U0001f6d5" // hindu temple
- Synagogue Emoji = "\U0001f54d" // synagogue
- ShintoShrine Emoji = "\u26e9\ufe0f" // shinto shrine
- Kaaba Emoji = "\U0001f54b" // kaaba
- // SUBGROUP: place-other
- Fountain Emoji = "\u26f2" // fountain
- Tent Emoji = "\u26fa" // tent
- Foggy Emoji = "\U0001f301" // foggy
- NightWithStars Emoji = "\U0001f303" // night with stars
- Cityscape Emoji = "\U0001f3d9\ufe0f" // cityscape
- SunriseOverMountains Emoji = "\U0001f304" // sunrise over mountains
- Sunrise Emoji = "\U0001f305" // sunrise
- CityscapeAtDusk Emoji = "\U0001f306" // cityscape at dusk
- Sunset Emoji = "\U0001f307" // sunset
- BridgeAtNight Emoji = "\U0001f309" // bridge at night
- HotSprings Emoji = "\u2668\ufe0f" // hot springs
- CarouselHorse Emoji = "\U0001f3a0" // carousel horse
- FerrisWheel Emoji = "\U0001f3a1" // ferris wheel
- RollerCoaster Emoji = "\U0001f3a2" // roller coaster
- BarberPole Emoji = "\U0001f488" // barber pole
- CircusTent Emoji = "\U0001f3aa" // circus tent
- // SUBGROUP: transport-ground
- Locomotive Emoji = "\U0001f682" // locomotive
- RailwayCar Emoji = "\U0001f683" // railway car
- HighSpeedTrain Emoji = "\U0001f684" // high-speed train
- BulletTrain Emoji = "\U0001f685" // bullet train
- Train Emoji = "\U0001f686" // train
- Metro Emoji = "\U0001f687" // metro
- LightRail Emoji = "\U0001f688" // light rail
- Station Emoji = "\U0001f689" // station
- Tram Emoji = "\U0001f68a" // tram
- Monorail Emoji = "\U0001f69d" // monorail
- MountainRailway Emoji = "\U0001f69e" // mountain railway
- TramCar Emoji = "\U0001f68b" // tram car
- Bus Emoji = "\U0001f68c" // bus
- OncomingBus Emoji = "\U0001f68d" // oncoming bus
- Trolleybus Emoji = "\U0001f68e" // trolleybus
- Minibus Emoji = "\U0001f690" // minibus
- Ambulance Emoji = "\U0001f691" // ambulance
- FireEngine Emoji = "\U0001f692" // fire engine
- PoliceCar Emoji = "\U0001f693" // police car
- OncomingPoliceCar Emoji = "\U0001f694" // oncoming police car
- Taxi Emoji = "\U0001f695" // taxi
- OncomingTaxi Emoji = "\U0001f696" // oncoming taxi
- Automobile Emoji = "\U0001f697" // automobile
- OncomingAutomobile Emoji = "\U0001f698" // oncoming automobile
- SportUtilityVehicle Emoji = "\U0001f699" // sport utility vehicle
- PickupTruck Emoji = "\U0001f6fb" // pickup truck
- DeliveryTruck Emoji = "\U0001f69a" // delivery truck
- ArticulatedLorry Emoji = "\U0001f69b" // articulated lorry
- Tractor Emoji = "\U0001f69c" // tractor
- RacingCar Emoji = "\U0001f3ce\ufe0f" // racing car
- Motorcycle Emoji = "\U0001f3cd\ufe0f" // motorcycle
- MotorScooter Emoji = "\U0001f6f5" // motor scooter
- ManualWheelchair Emoji = "\U0001f9bd" // manual wheelchair
- MotorizedWheelchair Emoji = "\U0001f9bc" // motorized wheelchair
- AutoRickshaw Emoji = "\U0001f6fa" // auto rickshaw
- Bicycle Emoji = "\U0001f6b2" // bicycle
- KickScooter Emoji = "\U0001f6f4" // kick scooter
- Skateboard Emoji = "\U0001f6f9" // skateboard
- RollerSkate Emoji = "\U0001f6fc" // roller skate
- BusStop Emoji = "\U0001f68f" // bus stop
- Motorway Emoji = "\U0001f6e3\ufe0f" // motorway
- RailwayTrack Emoji = "\U0001f6e4\ufe0f" // railway track
- OilDrum Emoji = "\U0001f6e2\ufe0f" // oil drum
- FuelPump Emoji = "\u26fd" // fuel pump
- PoliceCarLight Emoji = "\U0001f6a8" // police car light
- HorizontalTrafficLight Emoji = "\U0001f6a5" // horizontal traffic light
- VerticalTrafficLight Emoji = "\U0001f6a6" // vertical traffic light
- StopSign Emoji = "\U0001f6d1" // stop sign
- Construction Emoji = "\U0001f6a7" // construction
- // SUBGROUP: transport-water
- Anchor Emoji = "\u2693" // anchor
- Sailboat Emoji = "\u26f5" // sailboat
- Canoe Emoji = "\U0001f6f6" // canoe
- Speedboat Emoji = "\U0001f6a4" // speedboat
- PassengerShip Emoji = "\U0001f6f3\ufe0f" // passenger ship
- Ferry Emoji = "\u26f4\ufe0f" // ferry
- MotorBoat Emoji = "\U0001f6e5\ufe0f" // motor boat
- Ship Emoji = "\U0001f6a2" // ship
- // SUBGROUP: transport-air
- Airplane Emoji = "\u2708\ufe0f" // airplane
- SmallAirplane Emoji = "\U0001f6e9\ufe0f" // small airplane
- AirplaneDeparture Emoji = "\U0001f6eb" // airplane departure
- AirplaneArrival Emoji = "\U0001f6ec" // airplane arrival
- Parachute Emoji = "\U0001fa82" // parachute
- Seat Emoji = "\U0001f4ba" // seat
- Helicopter Emoji = "\U0001f681" // helicopter
- SuspensionRailway Emoji = "\U0001f69f" // suspension railway
- MountainCableway Emoji = "\U0001f6a0" // mountain cableway
- AerialTramway Emoji = "\U0001f6a1" // aerial tramway
- Satellite Emoji = "\U0001f6f0\ufe0f" // satellite
- Rocket Emoji = "\U0001f680" // rocket
- FlyingSaucer Emoji = "\U0001f6f8" // flying saucer
- // SUBGROUP: hotel
- BellhopBell Emoji = "\U0001f6ce\ufe0f" // bellhop bell
- Luggage Emoji = "\U0001f9f3" // luggage
- // SUBGROUP: time
- HourglassDone Emoji = "\u231b" // hourglass done
- HourglassNotDone Emoji = "\u23f3" // hourglass not done
- Watch Emoji = "\u231a" // watch
- AlarmClock Emoji = "\u23f0" // alarm clock
- Stopwatch Emoji = "\u23f1\ufe0f" // stopwatch
- TimerClock Emoji = "\u23f2\ufe0f" // timer clock
- MantelpieceClock Emoji = "\U0001f570\ufe0f" // mantelpiece clock
- TwelveOClock Emoji = "\U0001f55b" // twelve o’clock
- TwelveThirty Emoji = "\U0001f567" // twelve-thirty
- OneOClock Emoji = "\U0001f550" // one o’clock
- OneThirty Emoji = "\U0001f55c" // one-thirty
- TwoOClock Emoji = "\U0001f551" // two o’clock
- TwoThirty Emoji = "\U0001f55d" // two-thirty
- ThreeOClock Emoji = "\U0001f552" // three o’clock
- ThreeThirty Emoji = "\U0001f55e" // three-thirty
- FourOClock Emoji = "\U0001f553" // four o’clock
- FourThirty Emoji = "\U0001f55f" // four-thirty
- FiveOClock Emoji = "\U0001f554" // five o’clock
- FiveThirty Emoji = "\U0001f560" // five-thirty
- SixOClock Emoji = "\U0001f555" // six o’clock
- SixThirty Emoji = "\U0001f561" // six-thirty
- SevenOClock Emoji = "\U0001f556" // seven o’clock
- SevenThirty Emoji = "\U0001f562" // seven-thirty
- EightOClock Emoji = "\U0001f557" // eight o’clock
- EightThirty Emoji = "\U0001f563" // eight-thirty
- NineOClock Emoji = "\U0001f558" // nine o’clock
- NineThirty Emoji = "\U0001f564" // nine-thirty
- TenOClock Emoji = "\U0001f559" // ten o’clock
- TenThirty Emoji = "\U0001f565" // ten-thirty
- ElevenOClock Emoji = "\U0001f55a" // eleven o’clock
- ElevenThirty Emoji = "\U0001f566" // eleven-thirty
- // SUBGROUP: sky & weather
- NewMoon Emoji = "\U0001f311" // new moon
- WaxingCrescentMoon Emoji = "\U0001f312" // waxing crescent moon
- FirstQuarterMoon Emoji = "\U0001f313" // first quarter moon
- WaxingGibbousMoon Emoji = "\U0001f314" // waxing gibbous moon
- FullMoon Emoji = "\U0001f315" // full moon
- WaningGibbousMoon Emoji = "\U0001f316" // waning gibbous moon
- LastQuarterMoon Emoji = "\U0001f317" // last quarter moon
- WaningCrescentMoon Emoji = "\U0001f318" // waning crescent moon
- CrescentMoon Emoji = "\U0001f319" // crescent moon
- NewMoonFace Emoji = "\U0001f31a" // new moon face
- FirstQuarterMoonFace Emoji = "\U0001f31b" // first quarter moon face
- LastQuarterMoonFace Emoji = "\U0001f31c" // last quarter moon face
- Thermometer Emoji = "\U0001f321\ufe0f" // thermometer
- Sun Emoji = "\u2600\ufe0f" // sun
- FullMoonFace Emoji = "\U0001f31d" // full moon face
- SunWithFace Emoji = "\U0001f31e" // sun with face
- RingedPlanet Emoji = "\U0001fa90" // ringed planet
- Star Emoji = "\u2b50" // star
- GlowingStar Emoji = "\U0001f31f" // glowing star
- ShootingStar Emoji = "\U0001f320" // shooting star
- MilkyWay Emoji = "\U0001f30c" // milky way
- Cloud Emoji = "\u2601\ufe0f" // cloud
- SunBehindCloud Emoji = "\u26c5" // sun behind cloud
- CloudWithLightningAndRain Emoji = "\u26c8\ufe0f" // cloud with lightning and rain
- SunBehindSmallCloud Emoji = "\U0001f324\ufe0f" // sun behind small cloud
- SunBehindLargeCloud Emoji = "\U0001f325\ufe0f" // sun behind large cloud
- SunBehindRainCloud Emoji = "\U0001f326\ufe0f" // sun behind rain cloud
- CloudWithRain Emoji = "\U0001f327\ufe0f" // cloud with rain
- CloudWithSnow Emoji = "\U0001f328\ufe0f" // cloud with snow
- CloudWithLightning Emoji = "\U0001f329\ufe0f" // cloud with lightning
- Tornado Emoji = "\U0001f32a\ufe0f" // tornado
- Fog Emoji = "\U0001f32b\ufe0f" // fog
- WindFace Emoji = "\U0001f32c\ufe0f" // wind face
- Cyclone Emoji = "\U0001f300" // cyclone
- Rainbow Emoji = "\U0001f308" // rainbow
- ClosedUmbrella Emoji = "\U0001f302" // closed umbrella
- Umbrella Emoji = "\u2602\ufe0f" // umbrella
- UmbrellaWithRainDrops Emoji = "\u2614" // umbrella with rain drops
- UmbrellaOnGround Emoji = "\u26f1\ufe0f" // umbrella on ground
- HighVoltage Emoji = "\u26a1" // high voltage
- Snowflake Emoji = "\u2744\ufe0f" // snowflake
- Snowman Emoji = "\u2603\ufe0f" // snowman
- SnowmanWithoutSnow Emoji = "\u26c4" // snowman without snow
- Comet Emoji = "\u2604\ufe0f" // comet
- Fire Emoji = "\U0001f525" // fire
- Droplet Emoji = "\U0001f4a7" // droplet
- WaterWave Emoji = "\U0001f30a" // water wave
-
- // GROUP: Activities
- // SUBGROUP: event
- JackOLantern Emoji = "\U0001f383" // jack-o-lantern
- ChristmasTree Emoji = "\U0001f384" // Christmas tree
- Fireworks Emoji = "\U0001f386" // fireworks
- Sparkler Emoji = "\U0001f387" // sparkler
- Firecracker Emoji = "\U0001f9e8" // firecracker
- Sparkles Emoji = "\u2728" // sparkles
- Balloon Emoji = "\U0001f388" // balloon
- PartyPopper Emoji = "\U0001f389" // party popper
- ConfettiBall Emoji = "\U0001f38a" // confetti ball
- TanabataTree Emoji = "\U0001f38b" // tanabata tree
- PineDecoration Emoji = "\U0001f38d" // pine decoration
- JapaneseDolls Emoji = "\U0001f38e" // Japanese dolls
- CarpStreamer Emoji = "\U0001f38f" // carp streamer
- WindChime Emoji = "\U0001f390" // wind chime
- MoonViewingCeremony Emoji = "\U0001f391" // moon viewing ceremony
- RedEnvelope Emoji = "\U0001f9e7" // red envelope
- Ribbon Emoji = "\U0001f380" // ribbon
- WrappedGift Emoji = "\U0001f381" // wrapped gift
- ReminderRibbon Emoji = "\U0001f397\ufe0f" // reminder ribbon
- AdmissionTickets Emoji = "\U0001f39f\ufe0f" // admission tickets
- Ticket Emoji = "\U0001f3ab" // ticket
- // SUBGROUP: award-medal
- MilitaryMedal Emoji = "\U0001f396\ufe0f" // military medal
- Trophy Emoji = "\U0001f3c6" // trophy
- SportsMedal Emoji = "\U0001f3c5" // sports medal
- FirstPlaceMedal Emoji = "\U0001f947" // 1st place medal
- SecondPlaceMedal Emoji = "\U0001f948" // 2nd place medal
- ThirdPlaceMedal Emoji = "\U0001f949" // 3rd place medal
- // SUBGROUP: sport
- SoccerBall Emoji = "\u26bd" // soccer ball
- Baseball Emoji = "\u26be" // baseball
- Softball Emoji = "\U0001f94e" // softball
- Basketball Emoji = "\U0001f3c0" // basketball
- Volleyball Emoji = "\U0001f3d0" // volleyball
- AmericanFootball Emoji = "\U0001f3c8" // american football
- RugbyFootball Emoji = "\U0001f3c9" // rugby football
- Tennis Emoji = "\U0001f3be" // tennis
- FlyingDisc Emoji = "\U0001f94f" // flying disc
- Bowling Emoji = "\U0001f3b3" // bowling
- CricketGame Emoji = "\U0001f3cf" // cricket game
- FieldHockey Emoji = "\U0001f3d1" // field hockey
- IceHockey Emoji = "\U0001f3d2" // ice hockey
- Lacrosse Emoji = "\U0001f94d" // lacrosse
- PingPong Emoji = "\U0001f3d3" // ping pong
- Badminton Emoji = "\U0001f3f8" // badminton
- BoxingGlove Emoji = "\U0001f94a" // boxing glove
- MartialArtsUniform Emoji = "\U0001f94b" // martial arts uniform
- GoalNet Emoji = "\U0001f945" // goal net
- FlagInHole Emoji = "\u26f3" // flag in hole
- IceSkate Emoji = "\u26f8\ufe0f" // ice skate
- FishingPole Emoji = "\U0001f3a3" // fishing pole
- DivingMask Emoji = "\U0001f93f" // diving mask
- RunningShirt Emoji = "\U0001f3bd" // running shirt
- Skis Emoji = "\U0001f3bf" // skis
- Sled Emoji = "\U0001f6f7" // sled
- CurlingStone Emoji = "\U0001f94c" // curling stone
- // SUBGROUP: game
- DirectHit Emoji = "\U0001f3af" // direct hit
- YoYo Emoji = "\U0001fa80" // yo-yo
- Kite Emoji = "\U0001fa81" // kite
- Pool8Ball Emoji = "\U0001f3b1" // pool 8 ball
- CrystalBall Emoji = "\U0001f52e" // crystal ball
- MagicWand Emoji = "\U0001fa84" // magic wand
- NazarAmulet Emoji = "\U0001f9ff" // nazar amulet
- VideoGame Emoji = "\U0001f3ae" // video game
- Joystick Emoji = "\U0001f579\ufe0f" // joystick
- SlotMachine Emoji = "\U0001f3b0" // slot machine
- GameDie Emoji = "\U0001f3b2" // game die
- PuzzlePiece Emoji = "\U0001f9e9" // puzzle piece
- TeddyBear Emoji = "\U0001f9f8" // teddy bear
- Pinata Emoji = "\U0001fa85" // piñata
- NestingDolls Emoji = "\U0001fa86" // nesting dolls
- SpadeSuit Emoji = "\u2660\ufe0f" // spade suit
- HeartSuit Emoji = "\u2665\ufe0f" // heart suit
- DiamondSuit Emoji = "\u2666\ufe0f" // diamond suit
- ClubSuit Emoji = "\u2663\ufe0f" // club suit
- ChessPawn Emoji = "\u265f\ufe0f" // chess pawn
- Joker Emoji = "\U0001f0cf" // joker
- MahjongRedDragon Emoji = "\U0001f004" // mahjong red dragon
- FlowerPlayingCards Emoji = "\U0001f3b4" // flower playing cards
- // SUBGROUP: arts & crafts
- PerformingArts Emoji = "\U0001f3ad" // performing arts
- FramedPicture Emoji = "\U0001f5bc\ufe0f" // framed picture
- ArtistPalette Emoji = "\U0001f3a8" // artist palette
- Thread Emoji = "\U0001f9f5" // thread
- SewingNeedle Emoji = "\U0001faa1" // sewing needle
- Yarn Emoji = "\U0001f9f6" // yarn
- Knot Emoji = "\U0001faa2" // knot
-
- // GROUP: Objects
- // SUBGROUP: clothing
- Glasses Emoji = "\U0001f453" // glasses
- Sunglasses Emoji = "\U0001f576\ufe0f" // sunglasses
- Goggles Emoji = "\U0001f97d" // goggles
- LabCoat Emoji = "\U0001f97c" // lab coat
- SafetyVest Emoji = "\U0001f9ba" // safety vest
- Necktie Emoji = "\U0001f454" // necktie
- TShirt Emoji = "\U0001f455" // t-shirt
- Jeans Emoji = "\U0001f456" // jeans
- Scarf Emoji = "\U0001f9e3" // scarf
- Gloves Emoji = "\U0001f9e4" // gloves
- Coat Emoji = "\U0001f9e5" // coat
- Socks Emoji = "\U0001f9e6" // socks
- Dress Emoji = "\U0001f457" // dress
- Kimono Emoji = "\U0001f458" // kimono
- Sari Emoji = "\U0001f97b" // sari
- OnePieceSwimsuit Emoji = "\U0001fa71" // one-piece swimsuit
- Briefs Emoji = "\U0001fa72" // briefs
- Shorts Emoji = "\U0001fa73" // shorts
- Bikini Emoji = "\U0001f459" // bikini
- WomanSClothes Emoji = "\U0001f45a" // woman’s clothes
- Purse Emoji = "\U0001f45b" // purse
- Handbag Emoji = "\U0001f45c" // handbag
- ClutchBag Emoji = "\U0001f45d" // clutch bag
- ShoppingBags Emoji = "\U0001f6cd\ufe0f" // shopping bags
- Backpack Emoji = "\U0001f392" // backpack
- ThongSandal Emoji = "\U0001fa74" // thong sandal
- ManSShoe Emoji = "\U0001f45e" // man’s shoe
- RunningShoe Emoji = "\U0001f45f" // running shoe
- HikingBoot Emoji = "\U0001f97e" // hiking boot
- FlatShoe Emoji = "\U0001f97f" // flat shoe
- HighHeeledShoe Emoji = "\U0001f460" // high-heeled shoe
- WomanSSandal Emoji = "\U0001f461" // woman’s sandal
- BalletShoes Emoji = "\U0001fa70" // ballet shoes
- WomanSBoot Emoji = "\U0001f462" // woman’s boot
- Crown Emoji = "\U0001f451" // crown
- WomanSHat Emoji = "\U0001f452" // woman’s hat
- TopHat Emoji = "\U0001f3a9" // top hat
- GraduationCap Emoji = "\U0001f393" // graduation cap
- BilledCap Emoji = "\U0001f9e2" // billed cap
- MilitaryHelmet Emoji = "\U0001fa96" // military helmet
- RescueWorkerSHelmet Emoji = "\u26d1\ufe0f" // rescue worker’s helmet
- PrayerBeads Emoji = "\U0001f4ff" // prayer beads
- Lipstick Emoji = "\U0001f484" // lipstick
- Ring Emoji = "\U0001f48d" // ring
- GemStone Emoji = "\U0001f48e" // gem stone
- // SUBGROUP: sound
- MutedSpeaker Emoji = "\U0001f507" // muted speaker
- SpeakerLowVolume Emoji = "\U0001f508" // speaker low volume
- SpeakerMediumVolume Emoji = "\U0001f509" // speaker medium volume
- SpeakerHighVolume Emoji = "\U0001f50a" // speaker high volume
- Loudspeaker Emoji = "\U0001f4e2" // loudspeaker
- Megaphone Emoji = "\U0001f4e3" // megaphone
- PostalHorn Emoji = "\U0001f4ef" // postal horn
- Bell Emoji = "\U0001f514" // bell
- BellWithSlash Emoji = "\U0001f515" // bell with slash
- // SUBGROUP: music
- MusicalScore Emoji = "\U0001f3bc" // musical score
- MusicalNote Emoji = "\U0001f3b5" // musical note
- MusicalNotes Emoji = "\U0001f3b6" // musical notes
- StudioMicrophone Emoji = "\U0001f399\ufe0f" // studio microphone
- LevelSlider Emoji = "\U0001f39a\ufe0f" // level slider
- ControlKnobs Emoji = "\U0001f39b\ufe0f" // control knobs
- Microphone Emoji = "\U0001f3a4" // microphone
- Headphone Emoji = "\U0001f3a7" // headphone
- Radio Emoji = "\U0001f4fb" // radio
- // SUBGROUP: musical-instrument
- Saxophone Emoji = "\U0001f3b7" // saxophone
- Accordion Emoji = "\U0001fa97" // accordion
- Guitar Emoji = "\U0001f3b8" // guitar
- MusicalKeyboard Emoji = "\U0001f3b9" // musical keyboard
- Trumpet Emoji = "\U0001f3ba" // trumpet
- Violin Emoji = "\U0001f3bb" // violin
- Banjo Emoji = "\U0001fa95" // banjo
- Drum Emoji = "\U0001f941" // drum
- LongDrum Emoji = "\U0001fa98" // long drum
- // SUBGROUP: phone
- MobilePhone Emoji = "\U0001f4f1" // mobile phone
- MobilePhoneWithArrow Emoji = "\U0001f4f2" // mobile phone with arrow
- Telephone Emoji = "\u260e\ufe0f" // telephone
- TelephoneReceiver Emoji = "\U0001f4de" // telephone receiver
- Pager Emoji = "\U0001f4df" // pager
- FaxMachine Emoji = "\U0001f4e0" // fax machine
- // SUBGROUP: computer
- Battery Emoji = "\U0001f50b" // battery
- ElectricPlug Emoji = "\U0001f50c" // electric plug
- Laptop Emoji = "\U0001f4bb" // laptop
- DesktopComputer Emoji = "\U0001f5a5\ufe0f" // desktop computer
- Printer Emoji = "\U0001f5a8\ufe0f" // printer
- Keyboard Emoji = "\u2328\ufe0f" // keyboard
- ComputerMouse Emoji = "\U0001f5b1\ufe0f" // computer mouse
- Trackball Emoji = "\U0001f5b2\ufe0f" // trackball
- ComputerDisk Emoji = "\U0001f4bd" // computer disk
- FloppyDisk Emoji = "\U0001f4be" // floppy disk
- OpticalDisk Emoji = "\U0001f4bf" // optical disk
- Dvd Emoji = "\U0001f4c0" // dvd
- Abacus Emoji = "\U0001f9ee" // abacus
- // SUBGROUP: light & video
- MovieCamera Emoji = "\U0001f3a5" // movie camera
- FilmFrames Emoji = "\U0001f39e\ufe0f" // film frames
- FilmProjector Emoji = "\U0001f4fd\ufe0f" // film projector
- ClapperBoard Emoji = "\U0001f3ac" // clapper board
- Television Emoji = "\U0001f4fa" // television
- Camera Emoji = "\U0001f4f7" // camera
- CameraWithFlash Emoji = "\U0001f4f8" // camera with flash
- VideoCamera Emoji = "\U0001f4f9" // video camera
- Videocassette Emoji = "\U0001f4fc" // videocassette
- MagnifyingGlassTiltedLeft Emoji = "\U0001f50d" // magnifying glass tilted left
- MagnifyingGlassTiltedRight Emoji = "\U0001f50e" // magnifying glass tilted right
- Candle Emoji = "\U0001f56f\ufe0f" // candle
- LightBulb Emoji = "\U0001f4a1" // light bulb
- Flashlight Emoji = "\U0001f526" // flashlight
- RedPaperLantern Emoji = "\U0001f3ee" // red paper lantern
- DiyaLamp Emoji = "\U0001fa94" // diya lamp
- // SUBGROUP: book-paper
- NotebookWithDecorativeCover Emoji = "\U0001f4d4" // notebook with decorative cover
- ClosedBook Emoji = "\U0001f4d5" // closed book
- OpenBook Emoji = "\U0001f4d6" // open book
- GreenBook Emoji = "\U0001f4d7" // green book
- BlueBook Emoji = "\U0001f4d8" // blue book
- OrangeBook Emoji = "\U0001f4d9" // orange book
- Books Emoji = "\U0001f4da" // books
- Notebook Emoji = "\U0001f4d3" // notebook
- Ledger Emoji = "\U0001f4d2" // ledger
- PageWithCurl Emoji = "\U0001f4c3" // page with curl
- Scroll Emoji = "\U0001f4dc" // scroll
- PageFacingUp Emoji = "\U0001f4c4" // page facing up
- Newspaper Emoji = "\U0001f4f0" // newspaper
- RolledUpNewspaper Emoji = "\U0001f5de\ufe0f" // rolled-up newspaper
- BookmarkTabs Emoji = "\U0001f4d1" // bookmark tabs
- Bookmark Emoji = "\U0001f516" // bookmark
- Label Emoji = "\U0001f3f7\ufe0f" // label
- // SUBGROUP: money
- MoneyBag Emoji = "\U0001f4b0" // money bag
- Coin Emoji = "\U0001fa99" // coin
- YenBanknote Emoji = "\U0001f4b4" // yen banknote
- DollarBanknote Emoji = "\U0001f4b5" // dollar banknote
- EuroBanknote Emoji = "\U0001f4b6" // euro banknote
- PoundBanknote Emoji = "\U0001f4b7" // pound banknote
- MoneyWithWings Emoji = "\U0001f4b8" // money with wings
- CreditCard Emoji = "\U0001f4b3" // credit card
- Receipt Emoji = "\U0001f9fe" // receipt
- ChartIncreasingWithYen Emoji = "\U0001f4b9" // chart increasing with yen
- // SUBGROUP: mail
- Envelope Emoji = "\u2709\ufe0f" // envelope
- EMail Emoji = "\U0001f4e7" // e-mail
- IncomingEnvelope Emoji = "\U0001f4e8" // incoming envelope
- EnvelopeWithArrow Emoji = "\U0001f4e9" // envelope with arrow
- OutboxTray Emoji = "\U0001f4e4" // outbox tray
- InboxTray Emoji = "\U0001f4e5" // inbox tray
- Package Emoji = "\U0001f4e6" // package
- ClosedMailboxWithRaisedFlag Emoji = "\U0001f4eb" // closed mailbox with raised flag
- ClosedMailboxWithLoweredFlag Emoji = "\U0001f4ea" // closed mailbox with lowered flag
- OpenMailboxWithRaisedFlag Emoji = "\U0001f4ec" // open mailbox with raised flag
- OpenMailboxWithLoweredFlag Emoji = "\U0001f4ed" // open mailbox with lowered flag
- Postbox Emoji = "\U0001f4ee" // postbox
- BallotBoxWithBallot Emoji = "\U0001f5f3\ufe0f" // ballot box with ballot
- // SUBGROUP: writing
- Pencil Emoji = "\u270f\ufe0f" // pencil
- BlackNib Emoji = "\u2712\ufe0f" // black nib
- FountainPen Emoji = "\U0001f58b\ufe0f" // fountain pen
- Pen Emoji = "\U0001f58a\ufe0f" // pen
- Paintbrush Emoji = "\U0001f58c\ufe0f" // paintbrush
- Crayon Emoji = "\U0001f58d\ufe0f" // crayon
- Memo Emoji = "\U0001f4dd" // memo
- // SUBGROUP: office
- Briefcase Emoji = "\U0001f4bc" // briefcase
- FileFolder Emoji = "\U0001f4c1" // file folder
- OpenFileFolder Emoji = "\U0001f4c2" // open file folder
- CardIndexDividers Emoji = "\U0001f5c2\ufe0f" // card index dividers
- Calendar Emoji = "\U0001f4c5" // calendar
- TearOffCalendar Emoji = "\U0001f4c6" // tear-off calendar
- SpiralNotepad Emoji = "\U0001f5d2\ufe0f" // spiral notepad
- SpiralCalendar Emoji = "\U0001f5d3\ufe0f" // spiral calendar
- CardIndex Emoji = "\U0001f4c7" // card index
- ChartIncreasing Emoji = "\U0001f4c8" // chart increasing
- ChartDecreasing Emoji = "\U0001f4c9" // chart decreasing
- BarChart Emoji = "\U0001f4ca" // bar chart
- Clipboard Emoji = "\U0001f4cb" // clipboard
- Pushpin Emoji = "\U0001f4cc" // pushpin
- RoundPushpin Emoji = "\U0001f4cd" // round pushpin
- Paperclip Emoji = "\U0001f4ce" // paperclip
- LinkedPaperclips Emoji = "\U0001f587\ufe0f" // linked paperclips
- StraightRuler Emoji = "\U0001f4cf" // straight ruler
- TriangularRuler Emoji = "\U0001f4d0" // triangular ruler
- Scissors Emoji = "\u2702\ufe0f" // scissors
- CardFileBox Emoji = "\U0001f5c3\ufe0f" // card file box
- FileCabinet Emoji = "\U0001f5c4\ufe0f" // file cabinet
- Wastebasket Emoji = "\U0001f5d1\ufe0f" // wastebasket
- // SUBGROUP: lock
- Locked Emoji = "\U0001f512" // locked
- Unlocked Emoji = "\U0001f513" // unlocked
- LockedWithPen Emoji = "\U0001f50f" // locked with pen
- LockedWithKey Emoji = "\U0001f510" // locked with key
- Key Emoji = "\U0001f511" // key
- OldKey Emoji = "\U0001f5dd\ufe0f" // old key
- // SUBGROUP: tool
- Hammer Emoji = "\U0001f528" // hammer
- Axe Emoji = "\U0001fa93" // axe
- Pick Emoji = "\u26cf\ufe0f" // pick
- HammerAndPick Emoji = "\u2692\ufe0f" // hammer and pick
- HammerAndWrench Emoji = "\U0001f6e0\ufe0f" // hammer and wrench
- Dagger Emoji = "\U0001f5e1\ufe0f" // dagger
- CrossedSwords Emoji = "\u2694\ufe0f" // crossed swords
- Pistol Emoji = "\U0001f52b" // pistol
- Boomerang Emoji = "\U0001fa83" // boomerang
- BowAndArrow Emoji = "\U0001f3f9" // bow and arrow
- Shield Emoji = "\U0001f6e1\ufe0f" // shield
- CarpentrySaw Emoji = "\U0001fa9a" // carpentry saw
- Wrench Emoji = "\U0001f527" // wrench
- Screwdriver Emoji = "\U0001fa9b" // screwdriver
- NutAndBolt Emoji = "\U0001f529" // nut and bolt
- Gear Emoji = "\u2699\ufe0f" // gear
- Clamp Emoji = "\U0001f5dc\ufe0f" // clamp
- BalanceScale Emoji = "\u2696\ufe0f" // balance scale
- WhiteCane Emoji = "\U0001f9af" // white cane
- Link Emoji = "\U0001f517" // link
- Chains Emoji = "\u26d3\ufe0f" // chains
- Hook Emoji = "\U0001fa9d" // hook
- Toolbox Emoji = "\U0001f9f0" // toolbox
- Magnet Emoji = "\U0001f9f2" // magnet
- Ladder Emoji = "\U0001fa9c" // ladder
- // SUBGROUP: science
- Alembic Emoji = "\u2697\ufe0f" // alembic
- TestTube Emoji = "\U0001f9ea" // test tube
- PetriDish Emoji = "\U0001f9eb" // petri dish
- Dna Emoji = "\U0001f9ec" // dna
- Microscope Emoji = "\U0001f52c" // microscope
- Telescope Emoji = "\U0001f52d" // telescope
- SatelliteAntenna Emoji = "\U0001f4e1" // satellite antenna
- // SUBGROUP: medical
- Syringe Emoji = "\U0001f489" // syringe
- DropOfBlood Emoji = "\U0001fa78" // drop of blood
- Pill Emoji = "\U0001f48a" // pill
- AdhesiveBandage Emoji = "\U0001fa79" // adhesive bandage
- Stethoscope Emoji = "\U0001fa7a" // stethoscope
- // SUBGROUP: household
- Door Emoji = "\U0001f6aa" // door
- Elevator Emoji = "\U0001f6d7" // elevator
- Mirror Emoji = "\U0001fa9e" // mirror
- Window Emoji = "\U0001fa9f" // window
- Bed Emoji = "\U0001f6cf\ufe0f" // bed
- CouchAndLamp Emoji = "\U0001f6cb\ufe0f" // couch and lamp
- Chair Emoji = "\U0001fa91" // chair
- Toilet Emoji = "\U0001f6bd" // toilet
- Plunger Emoji = "\U0001faa0" // plunger
- Shower Emoji = "\U0001f6bf" // shower
- Bathtub Emoji = "\U0001f6c1" // bathtub
- MouseTrap Emoji = "\U0001faa4" // mouse trap
- Razor Emoji = "\U0001fa92" // razor
- LotionBottle Emoji = "\U0001f9f4" // lotion bottle
- SafetyPin Emoji = "\U0001f9f7" // safety pin
- Broom Emoji = "\U0001f9f9" // broom
- Basket Emoji = "\U0001f9fa" // basket
- RollOfPaper Emoji = "\U0001f9fb" // roll of paper
- Bucket Emoji = "\U0001faa3" // bucket
- Soap Emoji = "\U0001f9fc" // soap
- Toothbrush Emoji = "\U0001faa5" // toothbrush
- Sponge Emoji = "\U0001f9fd" // sponge
- FireExtinguisher Emoji = "\U0001f9ef" // fire extinguisher
- ShoppingCart Emoji = "\U0001f6d2" // shopping cart
- // SUBGROUP: other-object
- Cigarette Emoji = "\U0001f6ac" // cigarette
- Coffin Emoji = "\u26b0\ufe0f" // coffin
- Headstone Emoji = "\U0001faa6" // headstone
- FuneralUrn Emoji = "\u26b1\ufe0f" // funeral urn
- Moai Emoji = "\U0001f5ff" // moai
- Placard Emoji = "\U0001faa7" // placard
-
- // GROUP: Symbols
- // SUBGROUP: transport-sign
- AtmSign Emoji = "\U0001f3e7" // ATM sign
- LitterInBinSign Emoji = "\U0001f6ae" // litter in bin sign
- PotableWater Emoji = "\U0001f6b0" // potable water
- WheelchairSymbol Emoji = "\u267f" // wheelchair symbol
- MenSRoom Emoji = "\U0001f6b9" // men’s room
- WomenSRoom Emoji = "\U0001f6ba" // women’s room
- Restroom Emoji = "\U0001f6bb" // restroom
- BabySymbol Emoji = "\U0001f6bc" // baby symbol
- WaterCloset Emoji = "\U0001f6be" // water closet
- PassportControl Emoji = "\U0001f6c2" // passport control
- Customs Emoji = "\U0001f6c3" // customs
- BaggageClaim Emoji = "\U0001f6c4" // baggage claim
- LeftLuggage Emoji = "\U0001f6c5" // left luggage
- // SUBGROUP: warning
- Warning Emoji = "\u26a0\ufe0f" // warning
- ChildrenCrossing Emoji = "\U0001f6b8" // children crossing
- NoEntry Emoji = "\u26d4" // no entry
- Prohibited Emoji = "\U0001f6ab" // prohibited
- NoBicycles Emoji = "\U0001f6b3" // no bicycles
- NoSmoking Emoji = "\U0001f6ad" // no smoking
- NoLittering Emoji = "\U0001f6af" // no littering
- NonPotableWater Emoji = "\U0001f6b1" // non-potable water
- NoPedestrians Emoji = "\U0001f6b7" // no pedestrians
- NoMobilePhones Emoji = "\U0001f4f5" // no mobile phones
- NoOneUnderEighteen Emoji = "\U0001f51e" // no one under eighteen
- Radioactive Emoji = "\u2622\ufe0f" // radioactive
- Biohazard Emoji = "\u2623\ufe0f" // biohazard
- // SUBGROUP: arrow
- UpArrow Emoji = "\u2b06\ufe0f" // up arrow
- UpRightArrow Emoji = "\u2197\ufe0f" // up-right arrow
- RightArrow Emoji = "\u27a1\ufe0f" // right arrow
- DownRightArrow Emoji = "\u2198\ufe0f" // down-right arrow
- DownArrow Emoji = "\u2b07\ufe0f" // down arrow
- DownLeftArrow Emoji = "\u2199\ufe0f" // down-left arrow
- LeftArrow Emoji = "\u2b05\ufe0f" // left arrow
- UpLeftArrow Emoji = "\u2196\ufe0f" // up-left arrow
- UpDownArrow Emoji = "\u2195\ufe0f" // up-down arrow
- LeftRightArrow Emoji = "\u2194\ufe0f" // left-right arrow
- RightArrowCurvingLeft Emoji = "\u21a9\ufe0f" // right arrow curving left
- LeftArrowCurvingRight Emoji = "\u21aa\ufe0f" // left arrow curving right
- RightArrowCurvingUp Emoji = "\u2934\ufe0f" // right arrow curving up
- RightArrowCurvingDown Emoji = "\u2935\ufe0f" // right arrow curving down
- ClockwiseVerticalArrows Emoji = "\U0001f503" // clockwise vertical arrows
- CounterclockwiseArrowsButton Emoji = "\U0001f504" // counterclockwise arrows button
- BackArrow Emoji = "\U0001f519" // BACK arrow
- EndArrow Emoji = "\U0001f51a" // END arrow
- OnArrow Emoji = "\U0001f51b" // ON! arrow
- SoonArrow Emoji = "\U0001f51c" // SOON arrow
- TopArrow Emoji = "\U0001f51d" // TOP arrow
- // SUBGROUP: religion
- PlaceOfWorship Emoji = "\U0001f6d0" // place of worship
- AtomSymbol Emoji = "\u269b\ufe0f" // atom symbol
- Om Emoji = "\U0001f549\ufe0f" // om
- StarOfDavid Emoji = "\u2721\ufe0f" // star of David
- WheelOfDharma Emoji = "\u2638\ufe0f" // wheel of dharma
- YinYang Emoji = "\u262f\ufe0f" // yin yang
- LatinCross Emoji = "\u271d\ufe0f" // latin cross
- OrthodoxCross Emoji = "\u2626\ufe0f" // orthodox cross
- StarAndCrescent Emoji = "\u262a\ufe0f" // star and crescent
- PeaceSymbol Emoji = "\u262e\ufe0f" // peace symbol
- Menorah Emoji = "\U0001f54e" // menorah
- DottedSixPointedStar Emoji = "\U0001f52f" // dotted six-pointed star
- // SUBGROUP: zodiac
- Aries Emoji = "\u2648" // Aries
- Taurus Emoji = "\u2649" // Taurus
- Gemini Emoji = "\u264a" // Gemini
- Cancer Emoji = "\u264b" // Cancer
- Leo Emoji = "\u264c" // Leo
- Virgo Emoji = "\u264d" // Virgo
- Libra Emoji = "\u264e" // Libra
- Scorpio Emoji = "\u264f" // Scorpio
- Sagittarius Emoji = "\u2650" // Sagittarius
- Capricorn Emoji = "\u2651" // Capricorn
- Aquarius Emoji = "\u2652" // Aquarius
- Pisces Emoji = "\u2653" // Pisces
- Ophiuchus Emoji = "\u26ce" // Ophiuchus
- // SUBGROUP: av-symbol
- ShuffleTracksButton Emoji = "\U0001f500" // shuffle tracks button
- RepeatButton Emoji = "\U0001f501" // repeat button
- RepeatSingleButton Emoji = "\U0001f502" // repeat single button
- PlayButton Emoji = "\u25b6\ufe0f" // play button
- FastForwardButton Emoji = "\u23e9" // fast-forward button
- NextTrackButton Emoji = "\u23ed\ufe0f" // next track button
- PlayOrPauseButton Emoji = "\u23ef\ufe0f" // play or pause button
- ReverseButton Emoji = "\u25c0\ufe0f" // reverse button
- FastReverseButton Emoji = "\u23ea" // fast reverse button
- LastTrackButton Emoji = "\u23ee\ufe0f" // last track button
- UpwardsButton Emoji = "\U0001f53c" // upwards button
- FastUpButton Emoji = "\u23eb" // fast up button
- DownwardsButton Emoji = "\U0001f53d" // downwards button
- FastDownButton Emoji = "\u23ec" // fast down button
- PauseButton Emoji = "\u23f8\ufe0f" // pause button
- StopButton Emoji = "\u23f9\ufe0f" // stop button
- RecordButton Emoji = "\u23fa\ufe0f" // record button
- EjectButton Emoji = "\u23cf\ufe0f" // eject button
- Cinema Emoji = "\U0001f3a6" // cinema
- DimButton Emoji = "\U0001f505" // dim button
- BrightButton Emoji = "\U0001f506" // bright button
- AntennaBars Emoji = "\U0001f4f6" // antenna bars
- VibrationMode Emoji = "\U0001f4f3" // vibration mode
- MobilePhoneOff Emoji = "\U0001f4f4" // mobile phone off
- // SUBGROUP: gender
- FemaleSign Emoji = "\u2640\ufe0f" // female sign
- MaleSign Emoji = "\u2642\ufe0f" // male sign
- TransgenderSymbol Emoji = "\u26a7\ufe0f" // transgender symbol
- // SUBGROUP: math
- Multiply Emoji = "\u2716\ufe0f" // multiply
- Plus Emoji = "\u2795" // plus
- Minus Emoji = "\u2796" // minus
- Divide Emoji = "\u2797" // divide
- Infinity Emoji = "\u267e\ufe0f" // infinity
- // SUBGROUP: punctuation
- DoubleExclamationMark Emoji = "\u203c\ufe0f" // double exclamation mark
- ExclamationQuestionMark Emoji = "\u2049\ufe0f" // exclamation question mark
- QuestionMark Emoji = "\u2753" // question mark
- WhiteQuestionMark Emoji = "\u2754" // white question mark
- WhiteExclamationMark Emoji = "\u2755" // white exclamation mark
- ExclamationMark Emoji = "\u2757" // exclamation mark
- WavyDash Emoji = "\u3030\ufe0f" // wavy dash
- // SUBGROUP: currency
- CurrencyExchange Emoji = "\U0001f4b1" // currency exchange
- HeavyDollarSign Emoji = "\U0001f4b2" // heavy dollar sign
- // SUBGROUP: other-symbol
- MedicalSymbol Emoji = "\u2695\ufe0f" // medical symbol
- RecyclingSymbol Emoji = "\u267b\ufe0f" // recycling symbol
- FleurDeLis Emoji = "\u269c\ufe0f" // fleur-de-lis
- TridentEmblem Emoji = "\U0001f531" // trident emblem
- NameBadge Emoji = "\U0001f4db" // name badge
- JapaneseSymbolForBeginner Emoji = "\U0001f530" // Japanese symbol for beginner
- HollowRedCircle Emoji = "\u2b55" // hollow red circle
- CheckMarkButton Emoji = "\u2705" // check mark button
- CheckBoxWithCheck Emoji = "\u2611\ufe0f" // check box with check
- CheckMark Emoji = "\u2714\ufe0f" // check mark
- CrossMark Emoji = "\u274c" // cross mark
- CrossMarkButton Emoji = "\u274e" // cross mark button
- CurlyLoop Emoji = "\u27b0" // curly loop
- DoubleCurlyLoop Emoji = "\u27bf" // double curly loop
- PartAlternationMark Emoji = "\u303d\ufe0f" // part alternation mark
- EightSpokedAsterisk Emoji = "\u2733\ufe0f" // eight-spoked asterisk
- EightPointedStar Emoji = "\u2734\ufe0f" // eight-pointed star
- Sparkle Emoji = "\u2747\ufe0f" // sparkle
- Copyright Emoji = "\u00a9\ufe0f" // copyright
- Registered Emoji = "\u00ae\ufe0f" // registered
- TradeMark Emoji = "\u2122\ufe0f" // trade mark
- // SUBGROUP: keycap
- KeycapHash Emoji = "#\ufe0f\u20e3" // keycap: #
- KeycapAsterisk Emoji = "*\ufe0f\u20e3" // keycap: *
- Keycap0 Emoji = "0\ufe0f\u20e3" // keycap: 0
- Keycap1 Emoji = "1\ufe0f\u20e3" // keycap: 1
- Keycap2 Emoji = "2\ufe0f\u20e3" // keycap: 2
- Keycap3 Emoji = "3\ufe0f\u20e3" // keycap: 3
- Keycap4 Emoji = "4\ufe0f\u20e3" // keycap: 4
- Keycap5 Emoji = "5\ufe0f\u20e3" // keycap: 5
- Keycap6 Emoji = "6\ufe0f\u20e3" // keycap: 6
- Keycap7 Emoji = "7\ufe0f\u20e3" // keycap: 7
- Keycap8 Emoji = "8\ufe0f\u20e3" // keycap: 8
- Keycap9 Emoji = "9\ufe0f\u20e3" // keycap: 9
- Keycap10 Emoji = "\U0001f51f" // keycap: 10
- // SUBGROUP: alphanum
- InputLatinUppercase Emoji = "\U0001f520" // input latin uppercase
- InputLatinLowercase Emoji = "\U0001f521" // input latin lowercase
- InputNumbers Emoji = "\U0001f522" // input numbers
- InputSymbols Emoji = "\U0001f523" // input symbols
- InputLatinLetters Emoji = "\U0001f524" // input latin letters
- AButtonBloodType Emoji = "\U0001f170\ufe0f" // A button (blood type)
- AbButtonBloodType Emoji = "\U0001f18e" // AB button (blood type)
- BButtonBloodType Emoji = "\U0001f171\ufe0f" // B button (blood type)
- ClButton Emoji = "\U0001f191" // CL button
- CoolButton Emoji = "\U0001f192" // COOL button
- FreeButton Emoji = "\U0001f193" // FREE button
- Information Emoji = "\u2139\ufe0f" // information
- IdButton Emoji = "\U0001f194" // ID button
- CircledM Emoji = "\u24c2\ufe0f" // circled M
- NewButton Emoji = "\U0001f195" // NEW button
- NgButton Emoji = "\U0001f196" // NG button
- OButtonBloodType Emoji = "\U0001f17e\ufe0f" // O button (blood type)
- OkButton Emoji = "\U0001f197" // OK button
- PButton Emoji = "\U0001f17f\ufe0f" // P button
- SosButton Emoji = "\U0001f198" // SOS button
- UpButton Emoji = "\U0001f199" // UP! button
- VsButton Emoji = "\U0001f19a" // VS button
- JapaneseHereButton Emoji = "\U0001f201" // Japanese “here” button
- JapaneseServiceChargeButton Emoji = "\U0001f202\ufe0f" // Japanese “service charge” button
- JapaneseMonthlyAmountButton Emoji = "\U0001f237\ufe0f" // Japanese “monthly amount” button
- JapaneseNotFreeOfChargeButton Emoji = "\U0001f236" // Japanese “not free of charge” button
- JapaneseReservedButton Emoji = "\U0001f22f" // Japanese “reserved” button
- JapaneseBargainButton Emoji = "\U0001f250" // Japanese “bargain” button
- JapaneseDiscountButton Emoji = "\U0001f239" // Japanese “discount” button
- JapaneseFreeOfChargeButton Emoji = "\U0001f21a" // Japanese “free of charge” button
- JapaneseProhibitedButton Emoji = "\U0001f232" // Japanese “prohibited” button
- JapaneseAcceptableButton Emoji = "\U0001f251" // Japanese “acceptable” button
- JapaneseApplicationButton Emoji = "\U0001f238" // Japanese “application” button
- JapanesePassingGradeButton Emoji = "\U0001f234" // Japanese “passing grade” button
- JapaneseVacancyButton Emoji = "\U0001f233" // Japanese “vacancy” button
- JapaneseCongratulationsButton Emoji = "\u3297\ufe0f" // Japanese “congratulations” button
- JapaneseSecretButton Emoji = "\u3299\ufe0f" // Japanese “secret” button
- JapaneseOpenForBusinessButton Emoji = "\U0001f23a" // Japanese “open for business” button
- JapaneseNoVacancyButton Emoji = "\U0001f235" // Japanese “no vacancy” button
- // SUBGROUP: geometric
- RedCircle Emoji = "\U0001f534" // red circle
- OrangeCircle Emoji = "\U0001f7e0" // orange circle
- YellowCircle Emoji = "\U0001f7e1" // yellow circle
- GreenCircle Emoji = "\U0001f7e2" // green circle
- BlueCircle Emoji = "\U0001f535" // blue circle
- PurpleCircle Emoji = "\U0001f7e3" // purple circle
- BrownCircle Emoji = "\U0001f7e4" // brown circle
- BlackCircle Emoji = "\u26ab" // black circle
- WhiteCircle Emoji = "\u26aa" // white circle
- RedSquare Emoji = "\U0001f7e5" // red square
- OrangeSquare Emoji = "\U0001f7e7" // orange square
- YellowSquare Emoji = "\U0001f7e8" // yellow square
- GreenSquare Emoji = "\U0001f7e9" // green square
- BlueSquare Emoji = "\U0001f7e6" // blue square
- PurpleSquare Emoji = "\U0001f7ea" // purple square
- BrownSquare Emoji = "\U0001f7eb" // brown square
- BlackLargeSquare Emoji = "\u2b1b" // black large square
- WhiteLargeSquare Emoji = "\u2b1c" // white large square
- BlackMediumSquare Emoji = "\u25fc\ufe0f" // black medium square
- WhiteMediumSquare Emoji = "\u25fb\ufe0f" // white medium square
- BlackMediumSmallSquare Emoji = "\u25fe" // black medium-small square
- WhiteMediumSmallSquare Emoji = "\u25fd" // white medium-small square
- BlackSmallSquare Emoji = "\u25aa\ufe0f" // black small square
- WhiteSmallSquare Emoji = "\u25ab\ufe0f" // white small square
- LargeOrangeDiamond Emoji = "\U0001f536" // large orange diamond
- LargeBlueDiamond Emoji = "\U0001f537" // large blue diamond
- SmallOrangeDiamond Emoji = "\U0001f538" // small orange diamond
- SmallBlueDiamond Emoji = "\U0001f539" // small blue diamond
- RedTrianglePointedUp Emoji = "\U0001f53a" // red triangle pointed up
- RedTrianglePointedDown Emoji = "\U0001f53b" // red triangle pointed down
- DiamondWithADot Emoji = "\U0001f4a0" // diamond with a dot
- RadioButton Emoji = "\U0001f518" // radio button
- WhiteSquareButton Emoji = "\U0001f533" // white square button
- BlackSquareButton Emoji = "\U0001f532" // black square button
-
- // GROUP: Flags
- // SUBGROUP: flag
- ChequeredFlag Emoji = "\U0001f3c1" // chequered flag
- TriangularFlag Emoji = "\U0001f6a9" // triangular flag
- CrossedFlags Emoji = "\U0001f38c" // crossed flags
- BlackFlag Emoji = "\U0001f3f4" // black flag
- WhiteFlag Emoji = "\U0001f3f3\ufe0f" // white flag
- RainbowFlag Emoji = "\U0001f3f3\ufe0f\u200d\U0001f308" // rainbow flag
- TransgenderFlag Emoji = "\U0001f3f3\ufe0f\u200d\u26a7\ufe0f" // transgender flag
- PirateFlag Emoji = "\U0001f3f4\u200d\u2620\ufe0f" // pirate flag
- // SUBGROUP: country-flag
- FlagForAscensionIsland Emoji = "\U0001f1e6\U0001f1e8" // flag: Ascension Island
- FlagForAndorra Emoji = "\U0001f1e6\U0001f1e9" // flag: Andorra
- FlagForUnitedArabEmirates Emoji = "\U0001f1e6\U0001f1ea" // flag: United Arab Emirates
- FlagForAfghanistan Emoji = "\U0001f1e6\U0001f1eb" // flag: Afghanistan
- FlagForAntiguaAndBarbuda Emoji = "\U0001f1e6\U0001f1ec" // flag: Antigua & Barbuda
- FlagForAnguilla Emoji = "\U0001f1e6\U0001f1ee" // flag: Anguilla
- FlagForAlbania Emoji = "\U0001f1e6\U0001f1f1" // flag: Albania
- FlagForArmenia Emoji = "\U0001f1e6\U0001f1f2" // flag: Armenia
- FlagForAngola Emoji = "\U0001f1e6\U0001f1f4" // flag: Angola
- FlagForAntarctica Emoji = "\U0001f1e6\U0001f1f6" // flag: Antarctica
- FlagForArgentina Emoji = "\U0001f1e6\U0001f1f7" // flag: Argentina
- FlagForAmericanSamoa Emoji = "\U0001f1e6\U0001f1f8" // flag: American Samoa
- FlagForAustria Emoji = "\U0001f1e6\U0001f1f9" // flag: Austria
- FlagForAustralia Emoji = "\U0001f1e6\U0001f1fa" // flag: Australia
- FlagForAruba Emoji = "\U0001f1e6\U0001f1fc" // flag: Aruba
- FlagForAlandIslands Emoji = "\U0001f1e6\U0001f1fd" // flag: Åland Islands
- FlagForAzerbaijan Emoji = "\U0001f1e6\U0001f1ff" // flag: Azerbaijan
- FlagForBosniaAndHerzegovina Emoji = "\U0001f1e7\U0001f1e6" // flag: Bosnia & Herzegovina
- FlagForBarbados Emoji = "\U0001f1e7\U0001f1e7" // flag: Barbados
- FlagForBangladesh Emoji = "\U0001f1e7\U0001f1e9" // flag: Bangladesh
- FlagForBelgium Emoji = "\U0001f1e7\U0001f1ea" // flag: Belgium
- FlagForBurkinaFaso Emoji = "\U0001f1e7\U0001f1eb" // flag: Burkina Faso
- FlagForBulgaria Emoji = "\U0001f1e7\U0001f1ec" // flag: Bulgaria
- FlagForBahrain Emoji = "\U0001f1e7\U0001f1ed" // flag: Bahrain
- FlagForBurundi Emoji = "\U0001f1e7\U0001f1ee" // flag: Burundi
- FlagForBenin Emoji = "\U0001f1e7\U0001f1ef" // flag: Benin
- FlagForStBarthelemy Emoji = "\U0001f1e7\U0001f1f1" // flag: St. Barthélemy
- FlagForBermuda Emoji = "\U0001f1e7\U0001f1f2" // flag: Bermuda
- FlagForBrunei Emoji = "\U0001f1e7\U0001f1f3" // flag: Brunei
- FlagForBolivia Emoji = "\U0001f1e7\U0001f1f4" // flag: Bolivia
- FlagForCaribbeanNetherlands Emoji = "\U0001f1e7\U0001f1f6" // flag: Caribbean Netherlands
- FlagForBrazil Emoji = "\U0001f1e7\U0001f1f7" // flag: Brazil
- FlagForBahamas Emoji = "\U0001f1e7\U0001f1f8" // flag: Bahamas
- FlagForBhutan Emoji = "\U0001f1e7\U0001f1f9" // flag: Bhutan
- FlagForBouvetIsland Emoji = "\U0001f1e7\U0001f1fb" // flag: Bouvet Island
- FlagForBotswana Emoji = "\U0001f1e7\U0001f1fc" // flag: Botswana
- FlagForBelarus Emoji = "\U0001f1e7\U0001f1fe" // flag: Belarus
- FlagForBelize Emoji = "\U0001f1e7\U0001f1ff" // flag: Belize
- FlagForCanada Emoji = "\U0001f1e8\U0001f1e6" // flag: Canada
- FlagForCocosKeelingIslands Emoji = "\U0001f1e8\U0001f1e8" // flag: Cocos (Keeling) Islands
- FlagForCongoKinshasa Emoji = "\U0001f1e8\U0001f1e9" // flag: Congo - Kinshasa
- FlagForCentralAfricanRepublic Emoji = "\U0001f1e8\U0001f1eb" // flag: Central African Republic
- FlagForCongoBrazzaville Emoji = "\U0001f1e8\U0001f1ec" // flag: Congo - Brazzaville
- FlagForSwitzerland Emoji = "\U0001f1e8\U0001f1ed" // flag: Switzerland
- FlagForCoteDIvoire Emoji = "\U0001f1e8\U0001f1ee" // flag: Côte d’Ivoire
- FlagForCookIslands Emoji = "\U0001f1e8\U0001f1f0" // flag: Cook Islands
- FlagForChile Emoji = "\U0001f1e8\U0001f1f1" // flag: Chile
- FlagForCameroon Emoji = "\U0001f1e8\U0001f1f2" // flag: Cameroon
- FlagForChina Emoji = "\U0001f1e8\U0001f1f3" // flag: China
- FlagForColombia Emoji = "\U0001f1e8\U0001f1f4" // flag: Colombia
- FlagForClippertonIsland Emoji = "\U0001f1e8\U0001f1f5" // flag: Clipperton Island
- FlagForCostaRica Emoji = "\U0001f1e8\U0001f1f7" // flag: Costa Rica
- FlagForCuba Emoji = "\U0001f1e8\U0001f1fa" // flag: Cuba
- FlagForCapeVerde Emoji = "\U0001f1e8\U0001f1fb" // flag: Cape Verde
- FlagForCuracao Emoji = "\U0001f1e8\U0001f1fc" // flag: Curaçao
- FlagForChristmasIsland Emoji = "\U0001f1e8\U0001f1fd" // flag: Christmas Island
- FlagForCyprus Emoji = "\U0001f1e8\U0001f1fe" // flag: Cyprus
- FlagForCzechia Emoji = "\U0001f1e8\U0001f1ff" // flag: Czechia
- FlagForGermany Emoji = "\U0001f1e9\U0001f1ea" // flag: Germany
- FlagForDiegoGarcia Emoji = "\U0001f1e9\U0001f1ec" // flag: Diego Garcia
- FlagForDjibouti Emoji = "\U0001f1e9\U0001f1ef" // flag: Djibouti
- FlagForDenmark Emoji = "\U0001f1e9\U0001f1f0" // flag: Denmark
- FlagForDominica Emoji = "\U0001f1e9\U0001f1f2" // flag: Dominica
- FlagForDominicanRepublic Emoji = "\U0001f1e9\U0001f1f4" // flag: Dominican Republic
- FlagForAlgeria Emoji = "\U0001f1e9\U0001f1ff" // flag: Algeria
- FlagForCeutaAndMelilla Emoji = "\U0001f1ea\U0001f1e6" // flag: Ceuta & Melilla
- FlagForEcuador Emoji = "\U0001f1ea\U0001f1e8" // flag: Ecuador
- FlagForEstonia Emoji = "\U0001f1ea\U0001f1ea" // flag: Estonia
- FlagForEgypt Emoji = "\U0001f1ea\U0001f1ec" // flag: Egypt
- FlagForWesternSahara Emoji = "\U0001f1ea\U0001f1ed" // flag: Western Sahara
- FlagForEritrea Emoji = "\U0001f1ea\U0001f1f7" // flag: Eritrea
- FlagForSpain Emoji = "\U0001f1ea\U0001f1f8" // flag: Spain
- FlagForEthiopia Emoji = "\U0001f1ea\U0001f1f9" // flag: Ethiopia
- FlagForEuropeanUnion Emoji = "\U0001f1ea\U0001f1fa" // flag: European Union
- FlagForFinland Emoji = "\U0001f1eb\U0001f1ee" // flag: Finland
- FlagForFiji Emoji = "\U0001f1eb\U0001f1ef" // flag: Fiji
- FlagForFalklandIslands Emoji = "\U0001f1eb\U0001f1f0" // flag: Falkland Islands
- FlagForMicronesia Emoji = "\U0001f1eb\U0001f1f2" // flag: Micronesia
- FlagForFaroeIslands Emoji = "\U0001f1eb\U0001f1f4" // flag: Faroe Islands
- FlagForFrance Emoji = "\U0001f1eb\U0001f1f7" // flag: France
- FlagForGabon Emoji = "\U0001f1ec\U0001f1e6" // flag: Gabon
- FlagForUnitedKingdom Emoji = "\U0001f1ec\U0001f1e7" // flag: United Kingdom
- FlagForGrenada Emoji = "\U0001f1ec\U0001f1e9" // flag: Grenada
- FlagForGeorgia Emoji = "\U0001f1ec\U0001f1ea" // flag: Georgia
- FlagForFrenchGuiana Emoji = "\U0001f1ec\U0001f1eb" // flag: French Guiana
- FlagForGuernsey Emoji = "\U0001f1ec\U0001f1ec" // flag: Guernsey
- FlagForGhana Emoji = "\U0001f1ec\U0001f1ed" // flag: Ghana
- FlagForGibraltar Emoji = "\U0001f1ec\U0001f1ee" // flag: Gibraltar
- FlagForGreenland Emoji = "\U0001f1ec\U0001f1f1" // flag: Greenland
- FlagForGambia Emoji = "\U0001f1ec\U0001f1f2" // flag: Gambia
- FlagForGuinea Emoji = "\U0001f1ec\U0001f1f3" // flag: Guinea
- FlagForGuadeloupe Emoji = "\U0001f1ec\U0001f1f5" // flag: Guadeloupe
- FlagForEquatorialGuinea Emoji = "\U0001f1ec\U0001f1f6" // flag: Equatorial Guinea
- FlagForGreece Emoji = "\U0001f1ec\U0001f1f7" // flag: Greece
- FlagForSouthGeorgiaAndSouthSandwichIslands Emoji = "\U0001f1ec\U0001f1f8" // flag: South Georgia & South Sandwich Islands
- FlagForGuatemala Emoji = "\U0001f1ec\U0001f1f9" // flag: Guatemala
- FlagForGuam Emoji = "\U0001f1ec\U0001f1fa" // flag: Guam
- FlagForGuineaBissau Emoji = "\U0001f1ec\U0001f1fc" // flag: Guinea-Bissau
- FlagForGuyana Emoji = "\U0001f1ec\U0001f1fe" // flag: Guyana
- FlagForHongKongSarChina Emoji = "\U0001f1ed\U0001f1f0" // flag: Hong Kong SAR China
- FlagForHeardAndMcdonaldIslands Emoji = "\U0001f1ed\U0001f1f2" // flag: Heard & McDonald Islands
- FlagForHonduras Emoji = "\U0001f1ed\U0001f1f3" // flag: Honduras
- FlagForCroatia Emoji = "\U0001f1ed\U0001f1f7" // flag: Croatia
- FlagForHaiti Emoji = "\U0001f1ed\U0001f1f9" // flag: Haiti
- FlagForHungary Emoji = "\U0001f1ed\U0001f1fa" // flag: Hungary
- FlagForCanaryIslands Emoji = "\U0001f1ee\U0001f1e8" // flag: Canary Islands
- FlagForIndonesia Emoji = "\U0001f1ee\U0001f1e9" // flag: Indonesia
- FlagForIreland Emoji = "\U0001f1ee\U0001f1ea" // flag: Ireland
- FlagForIsrael Emoji = "\U0001f1ee\U0001f1f1" // flag: Israel
- FlagForIsleOfMan Emoji = "\U0001f1ee\U0001f1f2" // flag: Isle of Man
- FlagForIndia Emoji = "\U0001f1ee\U0001f1f3" // flag: India
- FlagForBritishIndianOceanTerritory Emoji = "\U0001f1ee\U0001f1f4" // flag: British Indian Ocean Territory
- FlagForIraq Emoji = "\U0001f1ee\U0001f1f6" // flag: Iraq
- FlagForIran Emoji = "\U0001f1ee\U0001f1f7" // flag: Iran
- FlagForIceland Emoji = "\U0001f1ee\U0001f1f8" // flag: Iceland
- FlagForItaly Emoji = "\U0001f1ee\U0001f1f9" // flag: Italy
- FlagForJersey Emoji = "\U0001f1ef\U0001f1ea" // flag: Jersey
- FlagForJamaica Emoji = "\U0001f1ef\U0001f1f2" // flag: Jamaica
- FlagForJordan Emoji = "\U0001f1ef\U0001f1f4" // flag: Jordan
- FlagForJapan Emoji = "\U0001f1ef\U0001f1f5" // flag: Japan
- FlagForKenya Emoji = "\U0001f1f0\U0001f1ea" // flag: Kenya
- FlagForKyrgyzstan Emoji = "\U0001f1f0\U0001f1ec" // flag: Kyrgyzstan
- FlagForCambodia Emoji = "\U0001f1f0\U0001f1ed" // flag: Cambodia
- FlagForKiribati Emoji = "\U0001f1f0\U0001f1ee" // flag: Kiribati
- FlagForComoros Emoji = "\U0001f1f0\U0001f1f2" // flag: Comoros
- FlagForStKittsAndNevis Emoji = "\U0001f1f0\U0001f1f3" // flag: St. Kitts & Nevis
- FlagForNorthKorea Emoji = "\U0001f1f0\U0001f1f5" // flag: North Korea
- FlagForSouthKorea Emoji = "\U0001f1f0\U0001f1f7" // flag: South Korea
- FlagForKuwait Emoji = "\U0001f1f0\U0001f1fc" // flag: Kuwait
- FlagForCaymanIslands Emoji = "\U0001f1f0\U0001f1fe" // flag: Cayman Islands
- FlagForKazakhstan Emoji = "\U0001f1f0\U0001f1ff" // flag: Kazakhstan
- FlagForLaos Emoji = "\U0001f1f1\U0001f1e6" // flag: Laos
- FlagForLebanon Emoji = "\U0001f1f1\U0001f1e7" // flag: Lebanon
- FlagForStLucia Emoji = "\U0001f1f1\U0001f1e8" // flag: St. Lucia
- FlagForLiechtenstein Emoji = "\U0001f1f1\U0001f1ee" // flag: Liechtenstein
- FlagForSriLanka Emoji = "\U0001f1f1\U0001f1f0" // flag: Sri Lanka
- FlagForLiberia Emoji = "\U0001f1f1\U0001f1f7" // flag: Liberia
- FlagForLesotho Emoji = "\U0001f1f1\U0001f1f8" // flag: Lesotho
- FlagForLithuania Emoji = "\U0001f1f1\U0001f1f9" // flag: Lithuania
- FlagForLuxembourg Emoji = "\U0001f1f1\U0001f1fa" // flag: Luxembourg
- FlagForLatvia Emoji = "\U0001f1f1\U0001f1fb" // flag: Latvia
- FlagForLibya Emoji = "\U0001f1f1\U0001f1fe" // flag: Libya
- FlagForMorocco Emoji = "\U0001f1f2\U0001f1e6" // flag: Morocco
- FlagForMonaco Emoji = "\U0001f1f2\U0001f1e8" // flag: Monaco
- FlagForMoldova Emoji = "\U0001f1f2\U0001f1e9" // flag: Moldova
- FlagForMontenegro Emoji = "\U0001f1f2\U0001f1ea" // flag: Montenegro
- FlagForStMartin Emoji = "\U0001f1f2\U0001f1eb" // flag: St. Martin
- FlagForMadagascar Emoji = "\U0001f1f2\U0001f1ec" // flag: Madagascar
- FlagForMarshallIslands Emoji = "\U0001f1f2\U0001f1ed" // flag: Marshall Islands
- FlagForNorthMacedonia Emoji = "\U0001f1f2\U0001f1f0" // flag: North Macedonia
- FlagForMali Emoji = "\U0001f1f2\U0001f1f1" // flag: Mali
- FlagForMyanmarBurma Emoji = "\U0001f1f2\U0001f1f2" // flag: Myanmar (Burma)
- FlagForMongolia Emoji = "\U0001f1f2\U0001f1f3" // flag: Mongolia
- FlagForMacaoSarChina Emoji = "\U0001f1f2\U0001f1f4" // flag: Macao SAR China
- FlagForNorthernMarianaIslands Emoji = "\U0001f1f2\U0001f1f5" // flag: Northern Mariana Islands
- FlagForMartinique Emoji = "\U0001f1f2\U0001f1f6" // flag: Martinique
- FlagForMauritania Emoji = "\U0001f1f2\U0001f1f7" // flag: Mauritania
- FlagForMontserrat Emoji = "\U0001f1f2\U0001f1f8" // flag: Montserrat
- FlagForMalta Emoji = "\U0001f1f2\U0001f1f9" // flag: Malta
- FlagForMauritius Emoji = "\U0001f1f2\U0001f1fa" // flag: Mauritius
- FlagForMaldives Emoji = "\U0001f1f2\U0001f1fb" // flag: Maldives
- FlagForMalawi Emoji = "\U0001f1f2\U0001f1fc" // flag: Malawi
- FlagForMexico Emoji = "\U0001f1f2\U0001f1fd" // flag: Mexico
- FlagForMalaysia Emoji = "\U0001f1f2\U0001f1fe" // flag: Malaysia
- FlagForMozambique Emoji = "\U0001f1f2\U0001f1ff" // flag: Mozambique
- FlagForNamibia Emoji = "\U0001f1f3\U0001f1e6" // flag: Namibia
- FlagForNewCaledonia Emoji = "\U0001f1f3\U0001f1e8" // flag: New Caledonia
- FlagForNiger Emoji = "\U0001f1f3\U0001f1ea" // flag: Niger
- FlagForNorfolkIsland Emoji = "\U0001f1f3\U0001f1eb" // flag: Norfolk Island
- FlagForNigeria Emoji = "\U0001f1f3\U0001f1ec" // flag: Nigeria
- FlagForNicaragua Emoji = "\U0001f1f3\U0001f1ee" // flag: Nicaragua
- FlagForNetherlands Emoji = "\U0001f1f3\U0001f1f1" // flag: Netherlands
- FlagForNorway Emoji = "\U0001f1f3\U0001f1f4" // flag: Norway
- FlagForNepal Emoji = "\U0001f1f3\U0001f1f5" // flag: Nepal
- FlagForNauru Emoji = "\U0001f1f3\U0001f1f7" // flag: Nauru
- FlagForNiue Emoji = "\U0001f1f3\U0001f1fa" // flag: Niue
- FlagForNewZealand Emoji = "\U0001f1f3\U0001f1ff" // flag: New Zealand
- FlagForOman Emoji = "\U0001f1f4\U0001f1f2" // flag: Oman
- FlagForPanama Emoji = "\U0001f1f5\U0001f1e6" // flag: Panama
- FlagForPeru Emoji = "\U0001f1f5\U0001f1ea" // flag: Peru
- FlagForFrenchPolynesia Emoji = "\U0001f1f5\U0001f1eb" // flag: French Polynesia
- FlagForPapuaNewGuinea Emoji = "\U0001f1f5\U0001f1ec" // flag: Papua New Guinea
- FlagForPhilippines Emoji = "\U0001f1f5\U0001f1ed" // flag: Philippines
- FlagForPakistan Emoji = "\U0001f1f5\U0001f1f0" // flag: Pakistan
- FlagForPoland Emoji = "\U0001f1f5\U0001f1f1" // flag: Poland
- FlagForStPierreAndMiquelon Emoji = "\U0001f1f5\U0001f1f2" // flag: St. Pierre & Miquelon
- FlagForPitcairnIslands Emoji = "\U0001f1f5\U0001f1f3" // flag: Pitcairn Islands
- FlagForPuertoRico Emoji = "\U0001f1f5\U0001f1f7" // flag: Puerto Rico
- FlagForPalestinianTerritories Emoji = "\U0001f1f5\U0001f1f8" // flag: Palestinian Territories
- FlagForPortugal Emoji = "\U0001f1f5\U0001f1f9" // flag: Portugal
- FlagForPalau Emoji = "\U0001f1f5\U0001f1fc" // flag: Palau
- FlagForParaguay Emoji = "\U0001f1f5\U0001f1fe" // flag: Paraguay
- FlagForQatar Emoji = "\U0001f1f6\U0001f1e6" // flag: Qatar
- FlagForReunion Emoji = "\U0001f1f7\U0001f1ea" // flag: Réunion
- FlagForRomania Emoji = "\U0001f1f7\U0001f1f4" // flag: Romania
- FlagForSerbia Emoji = "\U0001f1f7\U0001f1f8" // flag: Serbia
- FlagForRussia Emoji = "\U0001f1f7\U0001f1fa" // flag: Russia
- FlagForRwanda Emoji = "\U0001f1f7\U0001f1fc" // flag: Rwanda
- FlagForSaudiArabia Emoji = "\U0001f1f8\U0001f1e6" // flag: Saudi Arabia
- FlagForSolomonIslands Emoji = "\U0001f1f8\U0001f1e7" // flag: Solomon Islands
- FlagForSeychelles Emoji = "\U0001f1f8\U0001f1e8" // flag: Seychelles
- FlagForSudan Emoji = "\U0001f1f8\U0001f1e9" // flag: Sudan
- FlagForSweden Emoji = "\U0001f1f8\U0001f1ea" // flag: Sweden
- FlagForSingapore Emoji = "\U0001f1f8\U0001f1ec" // flag: Singapore
- FlagForStHelena Emoji = "\U0001f1f8\U0001f1ed" // flag: St. Helena
- FlagForSlovenia Emoji = "\U0001f1f8\U0001f1ee" // flag: Slovenia
- FlagForSvalbardAndJanMayen Emoji = "\U0001f1f8\U0001f1ef" // flag: Svalbard & Jan Mayen
- FlagForSlovakia Emoji = "\U0001f1f8\U0001f1f0" // flag: Slovakia
- FlagForSierraLeone Emoji = "\U0001f1f8\U0001f1f1" // flag: Sierra Leone
- FlagForSanMarino Emoji = "\U0001f1f8\U0001f1f2" // flag: San Marino
- FlagForSenegal Emoji = "\U0001f1f8\U0001f1f3" // flag: Senegal
- FlagForSomalia Emoji = "\U0001f1f8\U0001f1f4" // flag: Somalia
- FlagForSuriname Emoji = "\U0001f1f8\U0001f1f7" // flag: Suriname
- FlagForSouthSudan Emoji = "\U0001f1f8\U0001f1f8" // flag: South Sudan
- FlagForSaoTomeAndPrincipe Emoji = "\U0001f1f8\U0001f1f9" // flag: São Tomé & Príncipe
- FlagForElSalvador Emoji = "\U0001f1f8\U0001f1fb" // flag: El Salvador
- FlagForSintMaarten Emoji = "\U0001f1f8\U0001f1fd" // flag: Sint Maarten
- FlagForSyria Emoji = "\U0001f1f8\U0001f1fe" // flag: Syria
- FlagForEswatini Emoji = "\U0001f1f8\U0001f1ff" // flag: Eswatini
- FlagForTristanDaCunha Emoji = "\U0001f1f9\U0001f1e6" // flag: Tristan da Cunha
- FlagForTurksAndCaicosIslands Emoji = "\U0001f1f9\U0001f1e8" // flag: Turks & Caicos Islands
- FlagForChad Emoji = "\U0001f1f9\U0001f1e9" // flag: Chad
- FlagForFrenchSouthernTerritories Emoji = "\U0001f1f9\U0001f1eb" // flag: French Southern Territories
- FlagForTogo Emoji = "\U0001f1f9\U0001f1ec" // flag: Togo
- FlagForThailand Emoji = "\U0001f1f9\U0001f1ed" // flag: Thailand
- FlagForTajikistan Emoji = "\U0001f1f9\U0001f1ef" // flag: Tajikistan
- FlagForTokelau Emoji = "\U0001f1f9\U0001f1f0" // flag: Tokelau
- FlagForTimorLeste Emoji = "\U0001f1f9\U0001f1f1" // flag: Timor-Leste
- FlagForTurkmenistan Emoji = "\U0001f1f9\U0001f1f2" // flag: Turkmenistan
- FlagForTunisia Emoji = "\U0001f1f9\U0001f1f3" // flag: Tunisia
- FlagForTonga Emoji = "\U0001f1f9\U0001f1f4" // flag: Tonga
- FlagForTurkey Emoji = "\U0001f1f9\U0001f1f7" // flag: Turkey
- FlagForTrinidadAndTobago Emoji = "\U0001f1f9\U0001f1f9" // flag: Trinidad & Tobago
- FlagForTuvalu Emoji = "\U0001f1f9\U0001f1fb" // flag: Tuvalu
- FlagForTaiwan Emoji = "\U0001f1f9\U0001f1fc" // flag: Taiwan
- FlagForTanzania Emoji = "\U0001f1f9\U0001f1ff" // flag: Tanzania
- FlagForUkraine Emoji = "\U0001f1fa\U0001f1e6" // flag: Ukraine
- FlagForUganda Emoji = "\U0001f1fa\U0001f1ec" // flag: Uganda
- FlagForUsOutlyingIslands Emoji = "\U0001f1fa\U0001f1f2" // flag: U.S. Outlying Islands
- FlagForUnitedNations Emoji = "\U0001f1fa\U0001f1f3" // flag: United Nations
- FlagForUnitedStates Emoji = "\U0001f1fa\U0001f1f8" // flag: United States
- FlagForUruguay Emoji = "\U0001f1fa\U0001f1fe" // flag: Uruguay
- FlagForUzbekistan Emoji = "\U0001f1fa\U0001f1ff" // flag: Uzbekistan
- FlagForVaticanCity Emoji = "\U0001f1fb\U0001f1e6" // flag: Vatican City
- FlagForStVincentAndGrenadines Emoji = "\U0001f1fb\U0001f1e8" // flag: St. Vincent & Grenadines
- FlagForVenezuela Emoji = "\U0001f1fb\U0001f1ea" // flag: Venezuela
- FlagForBritishVirginIslands Emoji = "\U0001f1fb\U0001f1ec" // flag: British Virgin Islands
- FlagForUsVirginIslands Emoji = "\U0001f1fb\U0001f1ee" // flag: U.S. Virgin Islands
- FlagForVietnam Emoji = "\U0001f1fb\U0001f1f3" // flag: Vietnam
- FlagForVanuatu Emoji = "\U0001f1fb\U0001f1fa" // flag: Vanuatu
- FlagForWallisAndFutuna Emoji = "\U0001f1fc\U0001f1eb" // flag: Wallis & Futuna
- FlagForSamoa Emoji = "\U0001f1fc\U0001f1f8" // flag: Samoa
- FlagForKosovo Emoji = "\U0001f1fd\U0001f1f0" // flag: Kosovo
- FlagForYemen Emoji = "\U0001f1fe\U0001f1ea" // flag: Yemen
- FlagForMayotte Emoji = "\U0001f1fe\U0001f1f9" // flag: Mayotte
- FlagForSouthAfrica Emoji = "\U0001f1ff\U0001f1e6" // flag: South Africa
- FlagForZambia Emoji = "\U0001f1ff\U0001f1f2" // flag: Zambia
- FlagForZimbabwe Emoji = "\U0001f1ff\U0001f1fc" // flag: Zimbabwe
- // SUBGROUP: subdivision-flag
- FlagForEngland Emoji = "\U0001f3f4\U000e0067\U000e0062\U000e0065\U000e006e\U000e0067\U000e007f" // flag: England
- FlagForScotland Emoji = "\U0001f3f4\U000e0067\U000e0062\U000e0073\U000e0063\U000e0074\U000e007f" // flag: Scotland
- FlagForWales Emoji = "\U0001f3f4\U000e0067\U000e0062\U000e0077\U000e006c\U000e0073\U000e007f" // flag: Wales
-
-)
diff --git a/vendor/github.com/enescakir/emoji/doc.go b/vendor/github.com/enescakir/emoji/doc.go
deleted file mode 100644
index 61d831e..0000000
--- a/vendor/github.com/enescakir/emoji/doc.go
+++ /dev/null
@@ -1,4 +0,0 @@
-/*
-Package emoji makes working with emojis easier.
-*/
-package emoji
diff --git a/vendor/github.com/enescakir/emoji/emoji.go b/vendor/github.com/enescakir/emoji/emoji.go
deleted file mode 100644
index 2bcc3f3..0000000
--- a/vendor/github.com/enescakir/emoji/emoji.go
+++ /dev/null
@@ -1,124 +0,0 @@
-package emoji
-
-import (
- "fmt"
- "strings"
-)
-
-// Base attributes
-const (
- TonePlaceholder = "@"
- flagBaseIndex = '\U0001F1E6' - 'a'
-)
-
-// Skin tone colors
-const (
- Default Tone = ""
- Light Tone = "\U0001F3FB"
- MediumLight Tone = "\U0001F3FC"
- Medium Tone = "\U0001F3FD"
- MediumDark Tone = "\U0001F3FE"
- Dark Tone = "\U0001F3FF"
-)
-
-// Emoji defines an emoji object with no skin variations.
-type Emoji string
-
-// String returns string representation of the simple emoji.
-func (e Emoji) String() string {
- return string(e)
-}
-
-// EmojiWithTone defines an emoji object that has skin tone options.
-type EmojiWithTone struct {
- oneTonedCode string
- twoTonedCode string
- defaultTone Tone
-}
-
-// newEmojiWithTone constructs a new emoji object that has skin tone options.
-func newEmojiWithTone(codes ...string) EmojiWithTone {
- if len(codes) == 0 {
- return EmojiWithTone{}
- }
-
- one := codes[0]
- two := codes[0]
-
- if len(codes) > 1 {
- two = codes[1]
- }
-
- return EmojiWithTone{
- oneTonedCode: one,
- twoTonedCode: two,
- }
-}
-
-// withDefaultTone sets default tone for an emoji and returns it.
-func (e EmojiWithTone) withDefaultTone(tone string) EmojiWithTone {
- e.defaultTone = Tone(tone)
-
- return e
-}
-
-// String returns string representation of the emoji with default skin tone.
-func (e EmojiWithTone) String() string {
- return strings.ReplaceAll(e.oneTonedCode, TonePlaceholder, e.defaultTone.String())
-}
-
-// Tone returns string representation of the emoji with given skin tone.
-func (e EmojiWithTone) Tone(tones ...Tone) string {
- // if no tone given, return with default skin tone
- if len(tones) == 0 {
- return e.String()
- }
-
- str := e.twoTonedCode
- replaceCount := 1
-
- // if one tone given or emoji doesn't have twoTonedCode, use oneTonedCode
- // Also, replace all with one tone
- if len(tones) == 1 {
- str = e.oneTonedCode
- replaceCount = -1
- }
-
- // replace tone one by one
- for _, t := range tones {
- // use emoji's default tone
- if t == Default {
- t = e.defaultTone
- }
-
- str = strings.Replace(str, TonePlaceholder, t.String(), replaceCount)
- }
-
- return str
-}
-
-// Tone defines skin tone options for emojis.
-type Tone string
-
-// String returns string representation of the skin tone.
-func (t Tone) String() string {
- return string(t)
-}
-
-// CountryFlag returns a country flag emoji from given country code.
-// Full list of country codes: https://en.wikipedia.org/wiki/ISO_3166-1_alpha-2
-func CountryFlag(code string) (Emoji, error) {
- if len(code) != 2 {
- return "", fmt.Errorf("not valid country code: %q", code)
- }
-
- code = strings.ToLower(code)
- flag := countryCodeLetter(code[0]) + countryCodeLetter(code[1])
-
- return Emoji(flag), nil
-}
-
-// countryCodeLetter shifts given letter byte as flagBaseIndex.
-func countryCodeLetter(l byte) string {
- return string(rune(l) + flagBaseIndex)
-}
diff --git a/vendor/github.com/enescakir/emoji/fmt.go b/vendor/github.com/enescakir/emoji/fmt.go
deleted file mode 100644
index a63829e..0000000
--- a/vendor/github.com/enescakir/emoji/fmt.go
+++ /dev/null
@@ -1,56 +0,0 @@
-package emoji
-
-import (
- "fmt"
- "io"
-)
-
-// Sprint wraps fmt.Sprint with emoji support
-func Sprint(a ...interface{}) string {
- return Parse(fmt.Sprint(a...))
-}
-
-// Sprintf wraps fmt.Sprintf with emoji support
-func Sprintf(format string, a ...interface{}) string {
- return Parse(fmt.Sprintf(format, a...))
-}
-
-// Sprintln wraps fmt.Sprintln with emoji support
-func Sprintln(a ...interface{}) string {
- return Parse(fmt.Sprintln(a...))
-}
-
-// Print wraps fmt.Print with emoji support
-func Print(a ...interface{}) (n int, err error) {
- return fmt.Print(Sprint(a...))
-}
-
-// Println wraps fmt.Println with emoji support
-func Println(a ...interface{}) (n int, err error) {
- return fmt.Println(Sprint(a...))
-}
-
-// Printf wraps fmt.Printf with emoji support
-func Printf(format string, a ...interface{}) (n int, err error) {
- return fmt.Print(Sprintf(format, a...))
-}
-
-// Fprint wraps fmt.Fprint with emoji support
-func Fprint(w io.Writer, a ...interface{}) (n int, err error) {
- return fmt.Fprint(w, Sprint(a...))
-}
-
-// Fprintf wraps fmt.Fprintf with emoji support
-func Fprintf(w io.Writer, format string, a ...interface{}) (n int, err error) {
- return fmt.Fprint(w, Sprintf(format, a...))
-}
-
-// Fprintln wraps fmt.Fprintln with emoji support
-func Fprintln(w io.Writer, a ...interface{}) (n int, err error) {
- return fmt.Fprintln(w, Sprint(a...))
-}
-
-// Errorf wraps fmt.Errorf with emoji support
-func Errorf(format string, a ...interface{}) error {
- return fmt.Errorf(Sprintf(format, a...))
-}
diff --git a/vendor/github.com/enescakir/emoji/go.mod b/vendor/github.com/enescakir/emoji/go.mod
deleted file mode 100644
index 4fe23cb..0000000
--- a/vendor/github.com/enescakir/emoji/go.mod
+++ /dev/null
@@ -1,3 +0,0 @@
-module github.com/enescakir/emoji
-
-go 1.13
diff --git a/vendor/github.com/enescakir/emoji/go.sum b/vendor/github.com/enescakir/emoji/go.sum
deleted file mode 100644
index e69de29..0000000
diff --git a/vendor/github.com/enescakir/emoji/map.go b/vendor/github.com/enescakir/emoji/map.go
deleted file mode 100644
index a5b93e9..0000000
--- a/vendor/github.com/enescakir/emoji/map.go
+++ /dev/null
@@ -1,2715 +0,0 @@
-package emoji
-
-// Code generated by github.com/enescakir/emoji/internal/generator DO NOT EDIT.
-
-// Source: https://raw.githubusercontent.com/github/gemoji/master/db/emoji.json
-// Create at: 2020-03-08T15:58:37+03:00
-
-var emojiMap = map[string]string{
- ":+1:": "\U0001f44d",
- ":-1:": "\U0001f44e",
- ":100:": "\U0001f4af",
- ":1234:": "\U0001f522",
- ":1st_place_medal:": "\U0001f947",
- ":2nd_place_medal:": "\U0001f948",
- ":3rd_place_medal:": "\U0001f949",
- ":8ball:": "\U0001f3b1",
- ":a:": "\U0001f170\ufe0f",
- ":a_button_blood_type:": "\U0001f170\ufe0f",
- ":ab:": "\U0001f18e",
- ":ab_button_blood_type:": "\U0001f18e",
- ":abacus:": "\U0001f9ee",
- ":abc:": "\U0001f524",
- ":abcd:": "\U0001f521",
- ":accept:": "\U0001f251",
- ":accordion:": "\U0001fa97",
- ":adhesive_bandage:": "\U0001fa79",
- ":admission_tickets:": "\U0001f39f\ufe0f",
- ":adult:": "\U0001f9d1",
- ":aerial_tramway:": "\U0001f6a1",
- ":afghanistan:": "\U0001f1e6\U0001f1eb",
- ":airplane:": "\u2708\ufe0f",
- ":airplane_arrival:": "\U0001f6ec",
- ":airplane_departure:": "\U0001f6eb",
- ":aland_islands:": "\U0001f1e6\U0001f1fd",
- ":alarm_clock:": "\u23f0",
- ":albania:": "\U0001f1e6\U0001f1f1",
- ":alembic:": "\u2697\ufe0f",
- ":algeria:": "\U0001f1e9\U0001f1ff",
- ":alien:": "\U0001f47d",
- ":alien_monster:": "\U0001f47e",
- ":ambulance:": "\U0001f691",
- ":american_football:": "\U0001f3c8",
- ":american_samoa:": "\U0001f1e6\U0001f1f8",
- ":amphora:": "\U0001f3fa",
- ":anatomical_heart:": "\U0001fac0",
- ":anchor:": "\u2693",
- ":andorra:": "\U0001f1e6\U0001f1e9",
- ":angel:": "\U0001f47c",
- ":anger:": "\U0001f4a2",
- ":anger_symbol:": "\U0001f4a2",
- ":angola:": "\U0001f1e6\U0001f1f4",
- ":angry:": "\U0001f620",
- ":angry_face:": "\U0001f620",
- ":angry_face_with_horns:": "\U0001f47f",
- ":anguilla:": "\U0001f1e6\U0001f1ee",
- ":anguished:": "\U0001f627",
- ":anguished_face:": "\U0001f627",
- ":ant:": "\U0001f41c",
- ":antarctica:": "\U0001f1e6\U0001f1f6",
- ":antenna_bars:": "\U0001f4f6",
- ":antigua_barbuda:": "\U0001f1e6\U0001f1ec",
- ":anxious_face_with_sweat:": "\U0001f630",
- ":apple:": "\U0001f34e",
- ":aquarius:": "\u2652",
- ":argentina:": "\U0001f1e6\U0001f1f7",
- ":aries:": "\u2648",
- ":armenia:": "\U0001f1e6\U0001f1f2",
- ":arrow_backward:": "\u25c0\ufe0f",
- ":arrow_double_down:": "\u23ec",
- ":arrow_double_up:": "\u23eb",
- ":arrow_down:": "\u2b07\ufe0f",
- ":arrow_down_small:": "\U0001f53d",
- ":arrow_forward:": "\u25b6\ufe0f",
- ":arrow_heading_down:": "\u2935\ufe0f",
- ":arrow_heading_up:": "\u2934\ufe0f",
- ":arrow_left:": "\u2b05\ufe0f",
- ":arrow_lower_left:": "\u2199\ufe0f",
- ":arrow_lower_right:": "\u2198\ufe0f",
- ":arrow_right:": "\u27a1\ufe0f",
- ":arrow_right_hook:": "\u21aa\ufe0f",
- ":arrow_up:": "\u2b06\ufe0f",
- ":arrow_up_down:": "\u2195\ufe0f",
- ":arrow_up_small:": "\U0001f53c",
- ":arrow_upper_left:": "\u2196\ufe0f",
- ":arrow_upper_right:": "\u2197\ufe0f",
- ":arrows_clockwise:": "\U0001f503",
- ":arrows_counterclockwise:": "\U0001f504",
- ":art:": "\U0001f3a8",
- ":articulated_lorry:": "\U0001f69b",
- ":artificial_satellite:": "\U0001f6f0\ufe0f",
- ":artist:": "\U0001f9d1\u200d\U0001f3a8",
- ":artist_palette:": "\U0001f3a8",
- ":aruba:": "\U0001f1e6\U0001f1fc",
- ":ascension_island:": "\U0001f1e6\U0001f1e8",
- ":asterisk:": "*\ufe0f\u20e3",
- ":astonished:": "\U0001f632",
- ":astonished_face:": "\U0001f632",
- ":astronaut:": "\U0001f9d1\u200d\U0001f680",
- ":athletic_shoe:": "\U0001f45f",
- ":atm:": "\U0001f3e7",
- ":atm_sign:": "\U0001f3e7",
- ":atom_symbol:": "\u269b\ufe0f",
- ":australia:": "\U0001f1e6\U0001f1fa",
- ":austria:": "\U0001f1e6\U0001f1f9",
- ":auto_rickshaw:": "\U0001f6fa",
- ":automobile:": "\U0001f697",
- ":avocado:": "\U0001f951",
- ":axe:": "\U0001fa93",
- ":azerbaijan:": "\U0001f1e6\U0001f1ff",
- ":b:": "\U0001f171\ufe0f",
- ":b_button_blood_type:": "\U0001f171\ufe0f",
- ":baby:": "\U0001f476",
- ":baby_angel:": "\U0001f47c",
- ":baby_bottle:": "\U0001f37c",
- ":baby_chick:": "\U0001f424",
- ":baby_symbol:": "\U0001f6bc",
- ":back:": "\U0001f519",
- ":back_arrow:": "\U0001f519",
- ":backhand_index_pointing_down:": "\U0001f447",
- ":backhand_index_pointing_left:": "\U0001f448",
- ":backhand_index_pointing_right:": "\U0001f449",
- ":backhand_index_pointing_up:": "\U0001f446",
- ":backpack:": "\U0001f392",
- ":bacon:": "\U0001f953",
- ":badger:": "\U0001f9a1",
- ":badminton:": "\U0001f3f8",
- ":bagel:": "\U0001f96f",
- ":baggage_claim:": "\U0001f6c4",
- ":baguette_bread:": "\U0001f956",
- ":bahamas:": "\U0001f1e7\U0001f1f8",
- ":bahrain:": "\U0001f1e7\U0001f1ed",
- ":balance_scale:": "\u2696\ufe0f",
- ":bald:": "\U0001f9b2",
- ":bald_man:": "\U0001f468\u200d\U0001f9b2",
- ":bald_woman:": "\U0001f469\u200d\U0001f9b2",
- ":ballet_shoes:": "\U0001fa70",
- ":balloon:": "\U0001f388",
- ":ballot_box:": "\U0001f5f3\ufe0f",
- ":ballot_box_with_ballot:": "\U0001f5f3\ufe0f",
- ":ballot_box_with_check:": "\u2611\ufe0f",
- ":bamboo:": "\U0001f38d",
- ":banana:": "\U0001f34c",
- ":bangbang:": "\u203c\ufe0f",
- ":bangladesh:": "\U0001f1e7\U0001f1e9",
- ":banjo:": "\U0001fa95",
- ":bank:": "\U0001f3e6",
- ":bar_chart:": "\U0001f4ca",
- ":barbados:": "\U0001f1e7\U0001f1e7",
- ":barber:": "\U0001f488",
- ":barber_pole:": "\U0001f488",
- ":baseball:": "\u26be",
- ":basket:": "\U0001f9fa",
- ":basketball:": "\U0001f3c0",
- ":basketball_man:": "\u26f9\ufe0f\u200d\u2642\ufe0f",
- ":basketball_woman:": "\u26f9\ufe0f\u200d\u2640\ufe0f",
- ":bat:": "\U0001f987",
- ":bath:": "\U0001f6c0",
- ":bathtub:": "\U0001f6c1",
- ":battery:": "\U0001f50b",
- ":beach_umbrella:": "\U0001f3d6\ufe0f",
- ":beach_with_umbrella:": "\U0001f3d6\ufe0f",
- ":beaming_face_with_smiling_eyes:": "\U0001f601",
- ":bear:": "\U0001f43b",
- ":bearded_person:": "\U0001f9d4",
- ":beating_heart:": "\U0001f493",
- ":beaver:": "\U0001f9ab",
- ":bed:": "\U0001f6cf\ufe0f",
- ":bee:": "\U0001f41d",
- ":beer:": "\U0001f37a",
- ":beer_mug:": "\U0001f37a",
- ":beers:": "\U0001f37b",
- ":beetle:": "\U0001fab2",
- ":beginner:": "\U0001f530",
- ":belarus:": "\U0001f1e7\U0001f1fe",
- ":belgium:": "\U0001f1e7\U0001f1ea",
- ":belize:": "\U0001f1e7\U0001f1ff",
- ":bell:": "\U0001f514",
- ":bell_pepper:": "\U0001fad1",
- ":bell_with_slash:": "\U0001f515",
- ":bellhop_bell:": "\U0001f6ce\ufe0f",
- ":benin:": "\U0001f1e7\U0001f1ef",
- ":bento:": "\U0001f371",
- ":bento_box:": "\U0001f371",
- ":bermuda:": "\U0001f1e7\U0001f1f2",
- ":beverage_box:": "\U0001f9c3",
- ":bhutan:": "\U0001f1e7\U0001f1f9",
- ":bicycle:": "\U0001f6b2",
- ":bicyclist:": "\U0001f6b4",
- ":bike:": "\U0001f6b2",
- ":biking_man:": "\U0001f6b4\u200d\u2642\ufe0f",
- ":biking_woman:": "\U0001f6b4\u200d\u2640\ufe0f",
- ":bikini:": "\U0001f459",
- ":billed_cap:": "\U0001f9e2",
- ":biohazard:": "\u2623\ufe0f",
- ":bird:": "\U0001f426",
- ":birthday:": "\U0001f382",
- ":birthday_cake:": "\U0001f382",
- ":bison:": "\U0001f9ac",
- ":black_cat:": "\U0001f408\u200d\u2b1b",
- ":black_circle:": "\u26ab",
- ":black_flag:": "\U0001f3f4",
- ":black_heart:": "\U0001f5a4",
- ":black_joker:": "\U0001f0cf",
- ":black_large_square:": "\u2b1b",
- ":black_medium_small_square:": "\u25fe",
- ":black_medium_square:": "\u25fc\ufe0f",
- ":black_nib:": "\u2712\ufe0f",
- ":black_small_square:": "\u25aa\ufe0f",
- ":black_square_button:": "\U0001f532",
- ":blond_haired_man:": "\U0001f471\u200d\u2642\ufe0f",
- ":blond_haired_person:": "\U0001f471",
- ":blond_haired_woman:": "\U0001f471\u200d\u2640\ufe0f",
- ":blonde_woman:": "\U0001f471\u200d\u2640\ufe0f",
- ":blossom:": "\U0001f33c",
- ":blowfish:": "\U0001f421",
- ":blue_book:": "\U0001f4d8",
- ":blue_car:": "\U0001f699",
- ":blue_circle:": "\U0001f535",
- ":blue_heart:": "\U0001f499",
- ":blue_square:": "\U0001f7e6",
- ":blueberries:": "\U0001fad0",
- ":blush:": "\U0001f60a",
- ":boar:": "\U0001f417",
- ":boat:": "\u26f5",
- ":bolivia:": "\U0001f1e7\U0001f1f4",
- ":bomb:": "\U0001f4a3",
- ":bone:": "\U0001f9b4",
- ":book:": "\U0001f4d6",
- ":bookmark:": "\U0001f516",
- ":bookmark_tabs:": "\U0001f4d1",
- ":books:": "\U0001f4da",
- ":boom:": "\U0001f4a5",
- ":boomerang:": "\U0001fa83",
- ":boot:": "\U0001f462",
- ":bosnia_herzegovina:": "\U0001f1e7\U0001f1e6",
- ":botswana:": "\U0001f1e7\U0001f1fc",
- ":bottle_with_popping_cork:": "\U0001f37e",
- ":bouncing_ball_man:": "\u26f9\ufe0f\u200d\u2642\ufe0f",
- ":bouncing_ball_person:": "\u26f9\ufe0f",
- ":bouncing_ball_woman:": "\u26f9\ufe0f\u200d\u2640\ufe0f",
- ":bouquet:": "\U0001f490",
- ":bouvet_island:": "\U0001f1e7\U0001f1fb",
- ":bow:": "\U0001f647",
- ":bow_and_arrow:": "\U0001f3f9",
- ":bowing_man:": "\U0001f647\u200d\u2642\ufe0f",
- ":bowing_woman:": "\U0001f647\u200d\u2640\ufe0f",
- ":bowl_with_spoon:": "\U0001f963",
- ":bowling:": "\U0001f3b3",
- ":boxing_glove:": "\U0001f94a",
- ":boy:": "\U0001f466",
- ":brain:": "\U0001f9e0",
- ":brazil:": "\U0001f1e7\U0001f1f7",
- ":bread:": "\U0001f35e",
- ":breast_feeding:": "\U0001f931",
- ":brick:": "\U0001f9f1",
- ":bricks:": "\U0001f9f1",
- ":bride_with_veil:": "\U0001f470\u200d\u2640\ufe0f",
- ":bridge_at_night:": "\U0001f309",
- ":briefcase:": "\U0001f4bc",
- ":briefs:": "\U0001fa72",
- ":bright_button:": "\U0001f506",
- ":british_indian_ocean_territory:": "\U0001f1ee\U0001f1f4",
- ":british_virgin_islands:": "\U0001f1fb\U0001f1ec",
- ":broccoli:": "\U0001f966",
- ":broken_heart:": "\U0001f494",
- ":broom:": "\U0001f9f9",
- ":brown_circle:": "\U0001f7e4",
- ":brown_heart:": "\U0001f90e",
- ":brown_square:": "\U0001f7eb",
- ":brunei:": "\U0001f1e7\U0001f1f3",
- ":bubble_tea:": "\U0001f9cb",
- ":bucket:": "\U0001faa3",
- ":bug:": "\U0001f41b",
- ":building_construction:": "\U0001f3d7\ufe0f",
- ":bulb:": "\U0001f4a1",
- ":bulgaria:": "\U0001f1e7\U0001f1ec",
- ":bullet_train:": "\U0001f685",
- ":bullettrain_front:": "\U0001f685",
- ":bullettrain_side:": "\U0001f684",
- ":burkina_faso:": "\U0001f1e7\U0001f1eb",
- ":burrito:": "\U0001f32f",
- ":burundi:": "\U0001f1e7\U0001f1ee",
- ":bus:": "\U0001f68c",
- ":bus_stop:": "\U0001f68f",
- ":business_suit_levitating:": "\U0001f574\ufe0f",
- ":busstop:": "\U0001f68f",
- ":bust_in_silhouette:": "\U0001f464",
- ":busts_in_silhouette:": "\U0001f465",
- ":butter:": "\U0001f9c8",
- ":butterfly:": "\U0001f98b",
- ":cactus:": "\U0001f335",
- ":cake:": "\U0001f370",
- ":calendar:": "\U0001f4c6",
- ":call_me_hand:": "\U0001f919",
- ":calling:": "\U0001f4f2",
- ":cambodia:": "\U0001f1f0\U0001f1ed",
- ":camel:": "\U0001f42b",
- ":camera:": "\U0001f4f7",
- ":camera_flash:": "\U0001f4f8",
- ":camera_with_flash:": "\U0001f4f8",
- ":cameroon:": "\U0001f1e8\U0001f1f2",
- ":camping:": "\U0001f3d5\ufe0f",
- ":canada:": "\U0001f1e8\U0001f1e6",
- ":canary_islands:": "\U0001f1ee\U0001f1e8",
- ":cancer:": "\u264b",
- ":candle:": "\U0001f56f\ufe0f",
- ":candy:": "\U0001f36c",
- ":canned_food:": "\U0001f96b",
- ":canoe:": "\U0001f6f6",
- ":cape_verde:": "\U0001f1e8\U0001f1fb",
- ":capital_abcd:": "\U0001f520",
- ":capricorn:": "\u2651",
- ":car:": "\U0001f697",
- ":card_file_box:": "\U0001f5c3\ufe0f",
- ":card_index:": "\U0001f4c7",
- ":card_index_dividers:": "\U0001f5c2\ufe0f",
- ":caribbean_netherlands:": "\U0001f1e7\U0001f1f6",
- ":carousel_horse:": "\U0001f3a0",
- ":carp_streamer:": "\U0001f38f",
- ":carpentry_saw:": "\U0001fa9a",
- ":carrot:": "\U0001f955",
- ":cartwheeling:": "\U0001f938",
- ":castle:": "\U0001f3f0",
- ":cat2:": "\U0001f408",
- ":cat:": "\U0001f431",
- ":cat_face:": "\U0001f431",
- ":cat_with_tears_of_joy:": "\U0001f639",
- ":cat_with_wry_smile:": "\U0001f63c",
- ":cayman_islands:": "\U0001f1f0\U0001f1fe",
- ":cd:": "\U0001f4bf",
- ":central_african_republic:": "\U0001f1e8\U0001f1eb",
- ":ceuta_melilla:": "\U0001f1ea\U0001f1e6",
- ":chad:": "\U0001f1f9\U0001f1e9",
- ":chains:": "\u26d3\ufe0f",
- ":chair:": "\U0001fa91",
- ":champagne:": "\U0001f37e",
- ":chart:": "\U0001f4b9",
- ":chart_decreasing:": "\U0001f4c9",
- ":chart_increasing:": "\U0001f4c8",
- ":chart_increasing_with_yen:": "\U0001f4b9",
- ":chart_with_downwards_trend:": "\U0001f4c9",
- ":chart_with_upwards_trend:": "\U0001f4c8",
- ":check_box_with_check:": "\u2611\ufe0f",
- ":check_mark:": "\u2714\ufe0f",
- ":check_mark_button:": "\u2705",
- ":checkered_flag:": "\U0001f3c1",
- ":cheese:": "\U0001f9c0",
- ":cheese_wedge:": "\U0001f9c0",
- ":chequered_flag:": "\U0001f3c1",
- ":cherries:": "\U0001f352",
- ":cherry_blossom:": "\U0001f338",
- ":chess_pawn:": "\u265f\ufe0f",
- ":chestnut:": "\U0001f330",
- ":chicken:": "\U0001f414",
- ":child:": "\U0001f9d2",
- ":children_crossing:": "\U0001f6b8",
- ":chile:": "\U0001f1e8\U0001f1f1",
- ":chipmunk:": "\U0001f43f\ufe0f",
- ":chocolate_bar:": "\U0001f36b",
- ":chopsticks:": "\U0001f962",
- ":christmas_island:": "\U0001f1e8\U0001f1fd",
- ":christmas_tree:": "\U0001f384",
- ":church:": "\u26ea",
- ":cigarette:": "\U0001f6ac",
- ":cinema:": "\U0001f3a6",
- ":circled_m:": "\u24c2\ufe0f",
- ":circus_tent:": "\U0001f3aa",
- ":city_sunrise:": "\U0001f307",
- ":city_sunset:": "\U0001f306",
- ":cityscape:": "\U0001f3d9\ufe0f",
- ":cityscape_at_dusk:": "\U0001f306",
- ":cl:": "\U0001f191",
- ":cl_button:": "\U0001f191",
- ":clamp:": "\U0001f5dc\ufe0f",
- ":clap:": "\U0001f44f",
- ":clapper:": "\U0001f3ac",
- ":clapper_board:": "\U0001f3ac",
- ":clapping_hands:": "\U0001f44f",
- ":classical_building:": "\U0001f3db\ufe0f",
- ":climbing:": "\U0001f9d7",
- ":climbing_man:": "\U0001f9d7\u200d\u2642\ufe0f",
- ":climbing_woman:": "\U0001f9d7\u200d\u2640\ufe0f",
- ":clinking_beer_mugs:": "\U0001f37b",
- ":clinking_glasses:": "\U0001f942",
- ":clipboard:": "\U0001f4cb",
- ":clipperton_island:": "\U0001f1e8\U0001f1f5",
- ":clock1030:": "\U0001f565",
- ":clock10:": "\U0001f559",
- ":clock1130:": "\U0001f566",
- ":clock11:": "\U0001f55a",
- ":clock1230:": "\U0001f567",
- ":clock12:": "\U0001f55b",
- ":clock130:": "\U0001f55c",
- ":clock1:": "\U0001f550",
- ":clock230:": "\U0001f55d",
- ":clock2:": "\U0001f551",
- ":clock330:": "\U0001f55e",
- ":clock3:": "\U0001f552",
- ":clock430:": "\U0001f55f",
- ":clock4:": "\U0001f553",
- ":clock530:": "\U0001f560",
- ":clock5:": "\U0001f554",
- ":clock630:": "\U0001f561",
- ":clock6:": "\U0001f555",
- ":clock730:": "\U0001f562",
- ":clock7:": "\U0001f556",
- ":clock830:": "\U0001f563",
- ":clock8:": "\U0001f557",
- ":clock930:": "\U0001f564",
- ":clock9:": "\U0001f558",
- ":clockwise_vertical_arrows:": "\U0001f503",
- ":closed_book:": "\U0001f4d5",
- ":closed_lock_with_key:": "\U0001f510",
- ":closed_mailbox_with_lowered_flag:": "\U0001f4ea",
- ":closed_mailbox_with_raised_flag:": "\U0001f4eb",
- ":closed_umbrella:": "\U0001f302",
- ":cloud:": "\u2601\ufe0f",
- ":cloud_with_lightning:": "\U0001f329\ufe0f",
- ":cloud_with_lightning_and_rain:": "\u26c8\ufe0f",
- ":cloud_with_rain:": "\U0001f327\ufe0f",
- ":cloud_with_snow:": "\U0001f328\ufe0f",
- ":clown_face:": "\U0001f921",
- ":club_suit:": "\u2663\ufe0f",
- ":clubs:": "\u2663\ufe0f",
- ":clutch_bag:": "\U0001f45d",
- ":cn:": "\U0001f1e8\U0001f1f3",
- ":coat:": "\U0001f9e5",
- ":cockroach:": "\U0001fab3",
- ":cocktail:": "\U0001f378",
- ":cocktail_glass:": "\U0001f378",
- ":coconut:": "\U0001f965",
- ":cocos_islands:": "\U0001f1e8\U0001f1e8",
- ":coffee:": "\u2615",
- ":coffin:": "\u26b0\ufe0f",
- ":coin:": "\U0001fa99",
- ":cold_face:": "\U0001f976",
- ":cold_sweat:": "\U0001f630",
- ":collision:": "\U0001f4a5",
- ":colombia:": "\U0001f1e8\U0001f1f4",
- ":comet:": "\u2604\ufe0f",
- ":comoros:": "\U0001f1f0\U0001f1f2",
- ":compass:": "\U0001f9ed",
- ":computer:": "\U0001f4bb",
- ":computer_disk:": "\U0001f4bd",
- ":computer_mouse:": "\U0001f5b1\ufe0f",
- ":confetti_ball:": "\U0001f38a",
- ":confounded:": "\U0001f616",
- ":confounded_face:": "\U0001f616",
- ":confused:": "\U0001f615",
- ":confused_face:": "\U0001f615",
- ":congo_brazzaville:": "\U0001f1e8\U0001f1ec",
- ":congo_kinshasa:": "\U0001f1e8\U0001f1e9",
- ":congratulations:": "\u3297\ufe0f",
- ":construction:": "\U0001f6a7",
- ":construction_worker:": "\U0001f477",
- ":construction_worker_man:": "\U0001f477\u200d\u2642\ufe0f",
- ":construction_worker_woman:": "\U0001f477\u200d\u2640\ufe0f",
- ":control_knobs:": "\U0001f39b\ufe0f",
- ":convenience_store:": "\U0001f3ea",
- ":cook:": "\U0001f9d1\u200d\U0001f373",
- ":cook_islands:": "\U0001f1e8\U0001f1f0",
- ":cooked_rice:": "\U0001f35a",
- ":cookie:": "\U0001f36a",
- ":cooking:": "\U0001f373",
- ":cool:": "\U0001f192",
- ":cool_button:": "\U0001f192",
- ":cop:": "\U0001f46e",
- ":copyright:": "\u00a9\ufe0f",
- ":corn:": "\U0001f33d",
- ":costa_rica:": "\U0001f1e8\U0001f1f7",
- ":cote_divoire:": "\U0001f1e8\U0001f1ee",
- ":couch_and_lamp:": "\U0001f6cb\ufe0f",
- ":counterclockwise_arrows_button:": "\U0001f504",
- ":couple:": "\U0001f46b",
- ":couple_with_heart:": "\U0001f491",
- ":couple_with_heart_man_man:": "\U0001f468\u200d\u2764\ufe0f\u200d\U0001f468",
- ":couple_with_heart_woman_man:": "\U0001f469\u200d\u2764\ufe0f\u200d\U0001f468",
- ":couple_with_heart_woman_woman:": "\U0001f469\u200d\u2764\ufe0f\u200d\U0001f469",
- ":couplekiss:": "\U0001f48f",
- ":couplekiss_man_man:": "\U0001f468\u200d\u2764\ufe0f\u200d\U0001f48b\u200d\U0001f468",
- ":couplekiss_man_woman:": "\U0001f469\u200d\u2764\ufe0f\u200d\U0001f48b\u200d\U0001f468",
- ":couplekiss_woman_woman:": "\U0001f469\u200d\u2764\ufe0f\u200d\U0001f48b\u200d\U0001f469",
- ":cow2:": "\U0001f404",
- ":cow:": "\U0001f42e",
- ":cow_face:": "\U0001f42e",
- ":cowboy_hat_face:": "\U0001f920",
- ":crab:": "\U0001f980",
- ":crayon:": "\U0001f58d\ufe0f",
- ":credit_card:": "\U0001f4b3",
- ":crescent_moon:": "\U0001f319",
- ":cricket:": "\U0001f997",
- ":cricket_game:": "\U0001f3cf",
- ":croatia:": "\U0001f1ed\U0001f1f7",
- ":crocodile:": "\U0001f40a",
- ":croissant:": "\U0001f950",
- ":cross_mark:": "\u274c",
- ":cross_mark_button:": "\u274e",
- ":crossed_fingers:": "\U0001f91e",
- ":crossed_flags:": "\U0001f38c",
- ":crossed_swords:": "\u2694\ufe0f",
- ":crown:": "\U0001f451",
- ":cry:": "\U0001f622",
- ":crying_cat:": "\U0001f63f",
- ":crying_cat_face:": "\U0001f63f",
- ":crying_face:": "\U0001f622",
- ":crystal_ball:": "\U0001f52e",
- ":cuba:": "\U0001f1e8\U0001f1fa",
- ":cucumber:": "\U0001f952",
- ":cup_with_straw:": "\U0001f964",
- ":cupcake:": "\U0001f9c1",
- ":cupid:": "\U0001f498",
- ":curacao:": "\U0001f1e8\U0001f1fc",
- ":curling_stone:": "\U0001f94c",
- ":curly_hair:": "\U0001f9b1",
- ":curly_haired_man:": "\U0001f468\u200d\U0001f9b1",
- ":curly_haired_woman:": "\U0001f469\u200d\U0001f9b1",
- ":curly_loop:": "\u27b0",
- ":currency_exchange:": "\U0001f4b1",
- ":curry:": "\U0001f35b",
- ":curry_rice:": "\U0001f35b",
- ":cursing_face:": "\U0001f92c",
- ":custard:": "\U0001f36e",
- ":customs:": "\U0001f6c3",
- ":cut_of_meat:": "\U0001f969",
- ":cyclone:": "\U0001f300",
- ":cyprus:": "\U0001f1e8\U0001f1fe",
- ":czech_republic:": "\U0001f1e8\U0001f1ff",
- ":dagger:": "\U0001f5e1\ufe0f",
- ":dancer:": "\U0001f483",
- ":dancers:": "\U0001f46f",
- ":dancing_men:": "\U0001f46f\u200d\u2642\ufe0f",
- ":dancing_women:": "\U0001f46f\u200d\u2640\ufe0f",
- ":dango:": "\U0001f361",
- ":dark_skin_tone:": "\U0001f3ff",
- ":dark_sunglasses:": "\U0001f576\ufe0f",
- ":dart:": "\U0001f3af",
- ":dash:": "\U0001f4a8",
- ":dashing_away:": "\U0001f4a8",
- ":date:": "\U0001f4c5",
- ":de:": "\U0001f1e9\U0001f1ea",
- ":deaf_man:": "\U0001f9cf\u200d\u2642\ufe0f",
- ":deaf_person:": "\U0001f9cf",
- ":deaf_woman:": "\U0001f9cf\u200d\u2640\ufe0f",
- ":deciduous_tree:": "\U0001f333",
- ":deer:": "\U0001f98c",
- ":delivery_truck:": "\U0001f69a",
- ":denmark:": "\U0001f1e9\U0001f1f0",
- ":department_store:": "\U0001f3ec",
- ":derelict_house:": "\U0001f3da\ufe0f",
- ":desert:": "\U0001f3dc\ufe0f",
- ":desert_island:": "\U0001f3dd\ufe0f",
- ":desktop_computer:": "\U0001f5a5\ufe0f",
- ":detective:": "\U0001f575\ufe0f",
- ":diamond_shape_with_a_dot_inside:": "\U0001f4a0",
- ":diamond_suit:": "\u2666\ufe0f",
- ":diamond_with_a_dot:": "\U0001f4a0",
- ":diamonds:": "\u2666\ufe0f",
- ":diego_garcia:": "\U0001f1e9\U0001f1ec",
- ":dim_button:": "\U0001f505",
- ":direct_hit:": "\U0001f3af",
- ":disappointed:": "\U0001f61e",
- ":disappointed_face:": "\U0001f61e",
- ":disappointed_relieved:": "\U0001f625",
- ":disguised_face:": "\U0001f978",
- ":divide:": "\u2797",
- ":diving_mask:": "\U0001f93f",
- ":diya_lamp:": "\U0001fa94",
- ":dizzy:": "\U0001f4ab",
- ":dizzy_face:": "\U0001f635",
- ":djibouti:": "\U0001f1e9\U0001f1ef",
- ":dna:": "\U0001f9ec",
- ":do_not_litter:": "\U0001f6af",
- ":dodo:": "\U0001f9a4",
- ":dog2:": "\U0001f415",
- ":dog:": "\U0001f436",
- ":dog_face:": "\U0001f436",
- ":dollar:": "\U0001f4b5",
- ":dollar_banknote:": "\U0001f4b5",
- ":dolls:": "\U0001f38e",
- ":dolphin:": "\U0001f42c",
- ":dominica:": "\U0001f1e9\U0001f1f2",
- ":dominican_republic:": "\U0001f1e9\U0001f1f4",
- ":door:": "\U0001f6aa",
- ":dotted_six_pointed_star:": "\U0001f52f",
- ":double_curly_loop:": "\u27bf",
- ":double_exclamation_mark:": "\u203c\ufe0f",
- ":doughnut:": "\U0001f369",
- ":dove:": "\U0001f54a\ufe0f",
- ":down_arrow:": "\u2b07\ufe0f",
- ":down_left_arrow:": "\u2199\ufe0f",
- ":down_right_arrow:": "\u2198\ufe0f",
- ":downcast_face_with_sweat:": "\U0001f613",
- ":downwards_button:": "\U0001f53d",
- ":dragon:": "\U0001f409",
- ":dragon_face:": "\U0001f432",
- ":dress:": "\U0001f457",
- ":dromedary_camel:": "\U0001f42a",
- ":drooling_face:": "\U0001f924",
- ":drop_of_blood:": "\U0001fa78",
- ":droplet:": "\U0001f4a7",
- ":drum:": "\U0001f941",
- ":duck:": "\U0001f986",
- ":dumpling:": "\U0001f95f",
- ":dvd:": "\U0001f4c0",
- ":e-mail:": "\U0001f4e7",
- ":e_mail:": "\U0001f4e7",
- ":eagle:": "\U0001f985",
- ":ear:": "\U0001f442",
- ":ear_of_corn:": "\U0001f33d",
- ":ear_of_rice:": "\U0001f33e",
- ":ear_with_hearing_aid:": "\U0001f9bb",
- ":earth_africa:": "\U0001f30d",
- ":earth_americas:": "\U0001f30e",
- ":earth_asia:": "\U0001f30f",
- ":ecuador:": "\U0001f1ea\U0001f1e8",
- ":egg:": "\U0001f95a",
- ":eggplant:": "\U0001f346",
- ":egypt:": "\U0001f1ea\U0001f1ec",
- ":eight:": "8\ufe0f\u20e3",
- ":eight_o_clock:": "\U0001f557",
- ":eight_pointed_black_star:": "\u2734\ufe0f",
- ":eight_pointed_star:": "\u2734\ufe0f",
- ":eight_spoked_asterisk:": "\u2733\ufe0f",
- ":eight_thirty:": "\U0001f563",
- ":eject_button:": "\u23cf\ufe0f",
- ":el_salvador:": "\U0001f1f8\U0001f1fb",
- ":electric_plug:": "\U0001f50c",
- ":elephant:": "\U0001f418",
- ":elevator:": "\U0001f6d7",
- ":eleven_o_clock:": "\U0001f55a",
- ":eleven_thirty:": "\U0001f566",
- ":elf:": "\U0001f9dd",
- ":elf_man:": "\U0001f9dd\u200d\u2642\ufe0f",
- ":elf_woman:": "\U0001f9dd\u200d\u2640\ufe0f",
- ":email:": "\u2709\ufe0f",
- ":end:": "\U0001f51a",
- ":end_arrow:": "\U0001f51a",
- ":england:": "\U0001f3f4\U000e0067\U000e0062\U000e0065\U000e006e\U000e0067\U000e007f",
- ":envelope:": "\u2709\ufe0f",
- ":envelope_with_arrow:": "\U0001f4e9",
- ":equatorial_guinea:": "\U0001f1ec\U0001f1f6",
- ":eritrea:": "\U0001f1ea\U0001f1f7",
- ":es:": "\U0001f1ea\U0001f1f8",
- ":estonia:": "\U0001f1ea\U0001f1ea",
- ":ethiopia:": "\U0001f1ea\U0001f1f9",
- ":eu:": "\U0001f1ea\U0001f1fa",
- ":euro:": "\U0001f4b6",
- ":euro_banknote:": "\U0001f4b6",
- ":european_castle:": "\U0001f3f0",
- ":european_post_office:": "\U0001f3e4",
- ":european_union:": "\U0001f1ea\U0001f1fa",
- ":evergreen_tree:": "\U0001f332",
- ":ewe:": "\U0001f411",
- ":exclamation:": "\u2757",
- ":exclamation_mark:": "\u2757",
- ":exclamation_question_mark:": "\u2049\ufe0f",
- ":exploding_head:": "\U0001f92f",
- ":expressionless:": "\U0001f611",
- ":expressionless_face:": "\U0001f611",
- ":eye:": "\U0001f441\ufe0f",
- ":eye_in_speech_bubble:": "\U0001f441\ufe0f\u200d\U0001f5e8\ufe0f",
- ":eye_speech_bubble:": "\U0001f441\ufe0f\u200d\U0001f5e8\ufe0f",
- ":eyeglasses:": "\U0001f453",
- ":eyes:": "\U0001f440",
- ":face_blowing_a_kiss:": "\U0001f618",
- ":face_savoring_food:": "\U0001f60b",
- ":face_screaming_in_fear:": "\U0001f631",
- ":face_vomiting:": "\U0001f92e",
- ":face_with_hand_over_mouth:": "\U0001f92d",
- ":face_with_head_bandage:": "\U0001f915",
- ":face_with_medical_mask:": "\U0001f637",
- ":face_with_monocle:": "\U0001f9d0",
- ":face_with_open_mouth:": "\U0001f62e",
- ":face_with_raised_eyebrow:": "\U0001f928",
- ":face_with_rolling_eyes:": "\U0001f644",
- ":face_with_steam_from_nose:": "\U0001f624",
- ":face_with_symbols_on_mouth:": "\U0001f92c",
- ":face_with_tears_of_joy:": "\U0001f602",
- ":face_with_thermometer:": "\U0001f912",
- ":face_with_tongue:": "\U0001f61b",
- ":face_without_mouth:": "\U0001f636",
- ":facepalm:": "\U0001f926",
- ":facepunch:": "\U0001f44a",
- ":factory:": "\U0001f3ed",
- ":factory_worker:": "\U0001f9d1\u200d\U0001f3ed",
- ":fairy:": "\U0001f9da",
- ":fairy_man:": "\U0001f9da\u200d\u2642\ufe0f",
- ":fairy_woman:": "\U0001f9da\u200d\u2640\ufe0f",
- ":falafel:": "\U0001f9c6",
- ":falkland_islands:": "\U0001f1eb\U0001f1f0",
- ":fallen_leaf:": "\U0001f342",
- ":family:": "\U0001f46a",
- ":family_man_boy:": "\U0001f468\u200d\U0001f466",
- ":family_man_boy_boy:": "\U0001f468\u200d\U0001f466\u200d\U0001f466",
- ":family_man_girl:": "\U0001f468\u200d\U0001f467",
- ":family_man_girl_boy:": "\U0001f468\u200d\U0001f467\u200d\U0001f466",
- ":family_man_girl_girl:": "\U0001f468\u200d\U0001f467\u200d\U0001f467",
- ":family_man_man_boy:": "\U0001f468\u200d\U0001f468\u200d\U0001f466",
- ":family_man_man_boy_boy:": "\U0001f468\u200d\U0001f468\u200d\U0001f466\u200d\U0001f466",
- ":family_man_man_girl:": "\U0001f468\u200d\U0001f468\u200d\U0001f467",
- ":family_man_man_girl_boy:": "\U0001f468\u200d\U0001f468\u200d\U0001f467\u200d\U0001f466",
- ":family_man_man_girl_girl:": "\U0001f468\u200d\U0001f468\u200d\U0001f467\u200d\U0001f467",
- ":family_man_woman_boy:": "\U0001f468\u200d\U0001f469\u200d\U0001f466",
- ":family_man_woman_boy_boy:": "\U0001f468\u200d\U0001f469\u200d\U0001f466\u200d\U0001f466",
- ":family_man_woman_girl:": "\U0001f468\u200d\U0001f469\u200d\U0001f467",
- ":family_man_woman_girl_boy:": "\U0001f468\u200d\U0001f469\u200d\U0001f467\u200d\U0001f466",
- ":family_man_woman_girl_girl:": "\U0001f468\u200d\U0001f469\u200d\U0001f467\u200d\U0001f467",
- ":family_woman_boy:": "\U0001f469\u200d\U0001f466",
- ":family_woman_boy_boy:": "\U0001f469\u200d\U0001f466\u200d\U0001f466",
- ":family_woman_girl:": "\U0001f469\u200d\U0001f467",
- ":family_woman_girl_boy:": "\U0001f469\u200d\U0001f467\u200d\U0001f466",
- ":family_woman_girl_girl:": "\U0001f469\u200d\U0001f467\u200d\U0001f467",
- ":family_woman_woman_boy:": "\U0001f469\u200d\U0001f469\u200d\U0001f466",
- ":family_woman_woman_boy_boy:": "\U0001f469\u200d\U0001f469\u200d\U0001f466\u200d\U0001f466",
- ":family_woman_woman_girl:": "\U0001f469\u200d\U0001f469\u200d\U0001f467",
- ":family_woman_woman_girl_boy:": "\U0001f469\u200d\U0001f469\u200d\U0001f467\u200d\U0001f466",
- ":family_woman_woman_girl_girl:": "\U0001f469\u200d\U0001f469\u200d\U0001f467\u200d\U0001f467",
- ":farmer:": "\U0001f9d1\u200d\U0001f33e",
- ":faroe_islands:": "\U0001f1eb\U0001f1f4",
- ":fast_down_button:": "\u23ec",
- ":fast_forward:": "\u23e9",
- ":fast_forward_button:": "\u23e9",
- ":fast_reverse_button:": "\u23ea",
- ":fast_up_button:": "\u23eb",
- ":fax:": "\U0001f4e0",
- ":fax_machine:": "\U0001f4e0",
- ":fearful:": "\U0001f628",
- ":fearful_face:": "\U0001f628",
- ":feather:": "\U0001fab6",
- ":feet:": "\U0001f43e",
- ":female_detective:": "\U0001f575\ufe0f\u200d\u2640\ufe0f",
- ":female_sign:": "\u2640\ufe0f",
- ":ferris_wheel:": "\U0001f3a1",
- ":ferry:": "\u26f4\ufe0f",
- ":field_hockey:": "\U0001f3d1",
- ":fiji:": "\U0001f1eb\U0001f1ef",
- ":file_cabinet:": "\U0001f5c4\ufe0f",
- ":file_folder:": "\U0001f4c1",
- ":film_frames:": "\U0001f39e\ufe0f",
- ":film_projector:": "\U0001f4fd\ufe0f",
- ":film_strip:": "\U0001f39e\ufe0f",
- ":finland:": "\U0001f1eb\U0001f1ee",
- ":fire:": "\U0001f525",
- ":fire_engine:": "\U0001f692",
- ":fire_extinguisher:": "\U0001f9ef",
- ":firecracker:": "\U0001f9e8",
- ":firefighter:": "\U0001f9d1\u200d\U0001f692",
- ":fireworks:": "\U0001f386",
- ":first_place_medal:": "\U0001f947",
- ":first_quarter_moon:": "\U0001f313",
- ":first_quarter_moon_face:": "\U0001f31b",
- ":first_quarter_moon_with_face:": "\U0001f31b",
- ":fish:": "\U0001f41f",
- ":fish_cake:": "\U0001f365",
- ":fish_cake_with_swirl:": "\U0001f365",
- ":fishing_pole:": "\U0001f3a3",
- ":fishing_pole_and_fish:": "\U0001f3a3",
- ":fist:": "\u270a",
- ":fist_left:": "\U0001f91b",
- ":fist_oncoming:": "\U0001f44a",
- ":fist_raised:": "\u270a",
- ":fist_right:": "\U0001f91c",
- ":five:": "5\ufe0f\u20e3",
- ":five_o_clock:": "\U0001f554",
- ":five_thirty:": "\U0001f560",
- ":flag_for_afghanistan:": "\U0001f1e6\U0001f1eb",
- ":flag_for_aland_islands:": "\U0001f1e6\U0001f1fd",
- ":flag_for_albania:": "\U0001f1e6\U0001f1f1",
- ":flag_for_algeria:": "\U0001f1e9\U0001f1ff",
- ":flag_for_american_samoa:": "\U0001f1e6\U0001f1f8",
- ":flag_for_andorra:": "\U0001f1e6\U0001f1e9",
- ":flag_for_angola:": "\U0001f1e6\U0001f1f4",
- ":flag_for_anguilla:": "\U0001f1e6\U0001f1ee",
- ":flag_for_antarctica:": "\U0001f1e6\U0001f1f6",
- ":flag_for_antigua_and_barbuda:": "\U0001f1e6\U0001f1ec",
- ":flag_for_argentina:": "\U0001f1e6\U0001f1f7",
- ":flag_for_armenia:": "\U0001f1e6\U0001f1f2",
- ":flag_for_aruba:": "\U0001f1e6\U0001f1fc",
- ":flag_for_ascension_island:": "\U0001f1e6\U0001f1e8",
- ":flag_for_australia:": "\U0001f1e6\U0001f1fa",
- ":flag_for_austria:": "\U0001f1e6\U0001f1f9",
- ":flag_for_azerbaijan:": "\U0001f1e6\U0001f1ff",
- ":flag_for_bahamas:": "\U0001f1e7\U0001f1f8",
- ":flag_for_bahrain:": "\U0001f1e7\U0001f1ed",
- ":flag_for_bangladesh:": "\U0001f1e7\U0001f1e9",
- ":flag_for_barbados:": "\U0001f1e7\U0001f1e7",
- ":flag_for_belarus:": "\U0001f1e7\U0001f1fe",
- ":flag_for_belgium:": "\U0001f1e7\U0001f1ea",
- ":flag_for_belize:": "\U0001f1e7\U0001f1ff",
- ":flag_for_benin:": "\U0001f1e7\U0001f1ef",
- ":flag_for_bermuda:": "\U0001f1e7\U0001f1f2",
- ":flag_for_bhutan:": "\U0001f1e7\U0001f1f9",
- ":flag_for_bolivia:": "\U0001f1e7\U0001f1f4",
- ":flag_for_bosnia_and_herzegovina:": "\U0001f1e7\U0001f1e6",
- ":flag_for_botswana:": "\U0001f1e7\U0001f1fc",
- ":flag_for_bouvet_island:": "\U0001f1e7\U0001f1fb",
- ":flag_for_brazil:": "\U0001f1e7\U0001f1f7",
- ":flag_for_british_indian_ocean_territory:": "\U0001f1ee\U0001f1f4",
- ":flag_for_british_virgin_islands:": "\U0001f1fb\U0001f1ec",
- ":flag_for_brunei:": "\U0001f1e7\U0001f1f3",
- ":flag_for_bulgaria:": "\U0001f1e7\U0001f1ec",
- ":flag_for_burkina_faso:": "\U0001f1e7\U0001f1eb",
- ":flag_for_burundi:": "\U0001f1e7\U0001f1ee",
- ":flag_for_cambodia:": "\U0001f1f0\U0001f1ed",
- ":flag_for_cameroon:": "\U0001f1e8\U0001f1f2",
- ":flag_for_canada:": "\U0001f1e8\U0001f1e6",
- ":flag_for_canary_islands:": "\U0001f1ee\U0001f1e8",
- ":flag_for_cape_verde:": "\U0001f1e8\U0001f1fb",
- ":flag_for_caribbean_netherlands:": "\U0001f1e7\U0001f1f6",
- ":flag_for_cayman_islands:": "\U0001f1f0\U0001f1fe",
- ":flag_for_central_african_republic:": "\U0001f1e8\U0001f1eb",
- ":flag_for_ceuta_and_melilla:": "\U0001f1ea\U0001f1e6",
- ":flag_for_chad:": "\U0001f1f9\U0001f1e9",
- ":flag_for_chile:": "\U0001f1e8\U0001f1f1",
- ":flag_for_china:": "\U0001f1e8\U0001f1f3",
- ":flag_for_christmas_island:": "\U0001f1e8\U0001f1fd",
- ":flag_for_clipperton_island:": "\U0001f1e8\U0001f1f5",
- ":flag_for_cocos_keeling_islands:": "\U0001f1e8\U0001f1e8",
- ":flag_for_colombia:": "\U0001f1e8\U0001f1f4",
- ":flag_for_comoros:": "\U0001f1f0\U0001f1f2",
- ":flag_for_congo_brazzaville:": "\U0001f1e8\U0001f1ec",
- ":flag_for_congo_kinshasa:": "\U0001f1e8\U0001f1e9",
- ":flag_for_cook_islands:": "\U0001f1e8\U0001f1f0",
- ":flag_for_costa_rica:": "\U0001f1e8\U0001f1f7",
- ":flag_for_cote_d_ivoire:": "\U0001f1e8\U0001f1ee",
- ":flag_for_croatia:": "\U0001f1ed\U0001f1f7",
- ":flag_for_cuba:": "\U0001f1e8\U0001f1fa",
- ":flag_for_curacao:": "\U0001f1e8\U0001f1fc",
- ":flag_for_cyprus:": "\U0001f1e8\U0001f1fe",
- ":flag_for_czechia:": "\U0001f1e8\U0001f1ff",
- ":flag_for_denmark:": "\U0001f1e9\U0001f1f0",
- ":flag_for_diego_garcia:": "\U0001f1e9\U0001f1ec",
- ":flag_for_djibouti:": "\U0001f1e9\U0001f1ef",
- ":flag_for_dominica:": "\U0001f1e9\U0001f1f2",
- ":flag_for_dominican_republic:": "\U0001f1e9\U0001f1f4",
- ":flag_for_ecuador:": "\U0001f1ea\U0001f1e8",
- ":flag_for_egypt:": "\U0001f1ea\U0001f1ec",
- ":flag_for_el_salvador:": "\U0001f1f8\U0001f1fb",
- ":flag_for_england:": "\U0001f3f4\U000e0067\U000e0062\U000e0065\U000e006e\U000e0067\U000e007f",
- ":flag_for_equatorial_guinea:": "\U0001f1ec\U0001f1f6",
- ":flag_for_eritrea:": "\U0001f1ea\U0001f1f7",
- ":flag_for_estonia:": "\U0001f1ea\U0001f1ea",
- ":flag_for_eswatini:": "\U0001f1f8\U0001f1ff",
- ":flag_for_ethiopia:": "\U0001f1ea\U0001f1f9",
- ":flag_for_european_union:": "\U0001f1ea\U0001f1fa",
- ":flag_for_falkland_islands:": "\U0001f1eb\U0001f1f0",
- ":flag_for_faroe_islands:": "\U0001f1eb\U0001f1f4",
- ":flag_for_fiji:": "\U0001f1eb\U0001f1ef",
- ":flag_for_finland:": "\U0001f1eb\U0001f1ee",
- ":flag_for_france:": "\U0001f1eb\U0001f1f7",
- ":flag_for_french_guiana:": "\U0001f1ec\U0001f1eb",
- ":flag_for_french_polynesia:": "\U0001f1f5\U0001f1eb",
- ":flag_for_french_southern_territories:": "\U0001f1f9\U0001f1eb",
- ":flag_for_gabon:": "\U0001f1ec\U0001f1e6",
- ":flag_for_gambia:": "\U0001f1ec\U0001f1f2",
- ":flag_for_georgia:": "\U0001f1ec\U0001f1ea",
- ":flag_for_germany:": "\U0001f1e9\U0001f1ea",
- ":flag_for_ghana:": "\U0001f1ec\U0001f1ed",
- ":flag_for_gibraltar:": "\U0001f1ec\U0001f1ee",
- ":flag_for_greece:": "\U0001f1ec\U0001f1f7",
- ":flag_for_greenland:": "\U0001f1ec\U0001f1f1",
- ":flag_for_grenada:": "\U0001f1ec\U0001f1e9",
- ":flag_for_guadeloupe:": "\U0001f1ec\U0001f1f5",
- ":flag_for_guam:": "\U0001f1ec\U0001f1fa",
- ":flag_for_guatemala:": "\U0001f1ec\U0001f1f9",
- ":flag_for_guernsey:": "\U0001f1ec\U0001f1ec",
- ":flag_for_guinea:": "\U0001f1ec\U0001f1f3",
- ":flag_for_guinea_bissau:": "\U0001f1ec\U0001f1fc",
- ":flag_for_guyana:": "\U0001f1ec\U0001f1fe",
- ":flag_for_haiti:": "\U0001f1ed\U0001f1f9",
- ":flag_for_heard_and_mcdonald_islands:": "\U0001f1ed\U0001f1f2",
- ":flag_for_honduras:": "\U0001f1ed\U0001f1f3",
- ":flag_for_hong_kong_sar_china:": "\U0001f1ed\U0001f1f0",
- ":flag_for_hungary:": "\U0001f1ed\U0001f1fa",
- ":flag_for_iceland:": "\U0001f1ee\U0001f1f8",
- ":flag_for_india:": "\U0001f1ee\U0001f1f3",
- ":flag_for_indonesia:": "\U0001f1ee\U0001f1e9",
- ":flag_for_iran:": "\U0001f1ee\U0001f1f7",
- ":flag_for_iraq:": "\U0001f1ee\U0001f1f6",
- ":flag_for_ireland:": "\U0001f1ee\U0001f1ea",
- ":flag_for_isle_of_man:": "\U0001f1ee\U0001f1f2",
- ":flag_for_israel:": "\U0001f1ee\U0001f1f1",
- ":flag_for_italy:": "\U0001f1ee\U0001f1f9",
- ":flag_for_jamaica:": "\U0001f1ef\U0001f1f2",
- ":flag_for_japan:": "\U0001f1ef\U0001f1f5",
- ":flag_for_jersey:": "\U0001f1ef\U0001f1ea",
- ":flag_for_jordan:": "\U0001f1ef\U0001f1f4",
- ":flag_for_kazakhstan:": "\U0001f1f0\U0001f1ff",
- ":flag_for_kenya:": "\U0001f1f0\U0001f1ea",
- ":flag_for_kiribati:": "\U0001f1f0\U0001f1ee",
- ":flag_for_kosovo:": "\U0001f1fd\U0001f1f0",
- ":flag_for_kuwait:": "\U0001f1f0\U0001f1fc",
- ":flag_for_kyrgyzstan:": "\U0001f1f0\U0001f1ec",
- ":flag_for_laos:": "\U0001f1f1\U0001f1e6",
- ":flag_for_latvia:": "\U0001f1f1\U0001f1fb",
- ":flag_for_lebanon:": "\U0001f1f1\U0001f1e7",
- ":flag_for_lesotho:": "\U0001f1f1\U0001f1f8",
- ":flag_for_liberia:": "\U0001f1f1\U0001f1f7",
- ":flag_for_libya:": "\U0001f1f1\U0001f1fe",
- ":flag_for_liechtenstein:": "\U0001f1f1\U0001f1ee",
- ":flag_for_lithuania:": "\U0001f1f1\U0001f1f9",
- ":flag_for_luxembourg:": "\U0001f1f1\U0001f1fa",
- ":flag_for_macao_sar_china:": "\U0001f1f2\U0001f1f4",
- ":flag_for_madagascar:": "\U0001f1f2\U0001f1ec",
- ":flag_for_malawi:": "\U0001f1f2\U0001f1fc",
- ":flag_for_malaysia:": "\U0001f1f2\U0001f1fe",
- ":flag_for_maldives:": "\U0001f1f2\U0001f1fb",
- ":flag_for_mali:": "\U0001f1f2\U0001f1f1",
- ":flag_for_malta:": "\U0001f1f2\U0001f1f9",
- ":flag_for_marshall_islands:": "\U0001f1f2\U0001f1ed",
- ":flag_for_martinique:": "\U0001f1f2\U0001f1f6",
- ":flag_for_mauritania:": "\U0001f1f2\U0001f1f7",
- ":flag_for_mauritius:": "\U0001f1f2\U0001f1fa",
- ":flag_for_mayotte:": "\U0001f1fe\U0001f1f9",
- ":flag_for_mexico:": "\U0001f1f2\U0001f1fd",
- ":flag_for_micronesia:": "\U0001f1eb\U0001f1f2",
- ":flag_for_moldova:": "\U0001f1f2\U0001f1e9",
- ":flag_for_monaco:": "\U0001f1f2\U0001f1e8",
- ":flag_for_mongolia:": "\U0001f1f2\U0001f1f3",
- ":flag_for_montenegro:": "\U0001f1f2\U0001f1ea",
- ":flag_for_montserrat:": "\U0001f1f2\U0001f1f8",
- ":flag_for_morocco:": "\U0001f1f2\U0001f1e6",
- ":flag_for_mozambique:": "\U0001f1f2\U0001f1ff",
- ":flag_for_myanmar_burma:": "\U0001f1f2\U0001f1f2",
- ":flag_for_namibia:": "\U0001f1f3\U0001f1e6",
- ":flag_for_nauru:": "\U0001f1f3\U0001f1f7",
- ":flag_for_nepal:": "\U0001f1f3\U0001f1f5",
- ":flag_for_netherlands:": "\U0001f1f3\U0001f1f1",
- ":flag_for_new_caledonia:": "\U0001f1f3\U0001f1e8",
- ":flag_for_new_zealand:": "\U0001f1f3\U0001f1ff",
- ":flag_for_nicaragua:": "\U0001f1f3\U0001f1ee",
- ":flag_for_niger:": "\U0001f1f3\U0001f1ea",
- ":flag_for_nigeria:": "\U0001f1f3\U0001f1ec",
- ":flag_for_niue:": "\U0001f1f3\U0001f1fa",
- ":flag_for_norfolk_island:": "\U0001f1f3\U0001f1eb",
- ":flag_for_north_korea:": "\U0001f1f0\U0001f1f5",
- ":flag_for_north_macedonia:": "\U0001f1f2\U0001f1f0",
- ":flag_for_northern_mariana_islands:": "\U0001f1f2\U0001f1f5",
- ":flag_for_norway:": "\U0001f1f3\U0001f1f4",
- ":flag_for_oman:": "\U0001f1f4\U0001f1f2",
- ":flag_for_pakistan:": "\U0001f1f5\U0001f1f0",
- ":flag_for_palau:": "\U0001f1f5\U0001f1fc",
- ":flag_for_palestinian_territories:": "\U0001f1f5\U0001f1f8",
- ":flag_for_panama:": "\U0001f1f5\U0001f1e6",
- ":flag_for_papua_new_guinea:": "\U0001f1f5\U0001f1ec",
- ":flag_for_paraguay:": "\U0001f1f5\U0001f1fe",
- ":flag_for_peru:": "\U0001f1f5\U0001f1ea",
- ":flag_for_philippines:": "\U0001f1f5\U0001f1ed",
- ":flag_for_pitcairn_islands:": "\U0001f1f5\U0001f1f3",
- ":flag_for_poland:": "\U0001f1f5\U0001f1f1",
- ":flag_for_portugal:": "\U0001f1f5\U0001f1f9",
- ":flag_for_puerto_rico:": "\U0001f1f5\U0001f1f7",
- ":flag_for_qatar:": "\U0001f1f6\U0001f1e6",
- ":flag_for_reunion:": "\U0001f1f7\U0001f1ea",
- ":flag_for_romania:": "\U0001f1f7\U0001f1f4",
- ":flag_for_russia:": "\U0001f1f7\U0001f1fa",
- ":flag_for_rwanda:": "\U0001f1f7\U0001f1fc",
- ":flag_for_samoa:": "\U0001f1fc\U0001f1f8",
- ":flag_for_san_marino:": "\U0001f1f8\U0001f1f2",
- ":flag_for_sao_tome_and_principe:": "\U0001f1f8\U0001f1f9",
- ":flag_for_saudi_arabia:": "\U0001f1f8\U0001f1e6",
- ":flag_for_scotland:": "\U0001f3f4\U000e0067\U000e0062\U000e0073\U000e0063\U000e0074\U000e007f",
- ":flag_for_senegal:": "\U0001f1f8\U0001f1f3",
- ":flag_for_serbia:": "\U0001f1f7\U0001f1f8",
- ":flag_for_seychelles:": "\U0001f1f8\U0001f1e8",
- ":flag_for_sierra_leone:": "\U0001f1f8\U0001f1f1",
- ":flag_for_singapore:": "\U0001f1f8\U0001f1ec",
- ":flag_for_sint_maarten:": "\U0001f1f8\U0001f1fd",
- ":flag_for_slovakia:": "\U0001f1f8\U0001f1f0",
- ":flag_for_slovenia:": "\U0001f1f8\U0001f1ee",
- ":flag_for_solomon_islands:": "\U0001f1f8\U0001f1e7",
- ":flag_for_somalia:": "\U0001f1f8\U0001f1f4",
- ":flag_for_south_africa:": "\U0001f1ff\U0001f1e6",
- ":flag_for_south_georgia_and_south_sandwich_islands:": "\U0001f1ec\U0001f1f8",
- ":flag_for_south_korea:": "\U0001f1f0\U0001f1f7",
- ":flag_for_south_sudan:": "\U0001f1f8\U0001f1f8",
- ":flag_for_spain:": "\U0001f1ea\U0001f1f8",
- ":flag_for_sri_lanka:": "\U0001f1f1\U0001f1f0",
- ":flag_for_st_barthelemy:": "\U0001f1e7\U0001f1f1",
- ":flag_for_st_helena:": "\U0001f1f8\U0001f1ed",
- ":flag_for_st_kitts_and_nevis:": "\U0001f1f0\U0001f1f3",
- ":flag_for_st_lucia:": "\U0001f1f1\U0001f1e8",
- ":flag_for_st_martin:": "\U0001f1f2\U0001f1eb",
- ":flag_for_st_pierre_and_miquelon:": "\U0001f1f5\U0001f1f2",
- ":flag_for_st_vincent_and_grenadines:": "\U0001f1fb\U0001f1e8",
- ":flag_for_sudan:": "\U0001f1f8\U0001f1e9",
- ":flag_for_suriname:": "\U0001f1f8\U0001f1f7",
- ":flag_for_svalbard_and_jan_mayen:": "\U0001f1f8\U0001f1ef",
- ":flag_for_sweden:": "\U0001f1f8\U0001f1ea",
- ":flag_for_switzerland:": "\U0001f1e8\U0001f1ed",
- ":flag_for_syria:": "\U0001f1f8\U0001f1fe",
- ":flag_for_taiwan:": "\U0001f1f9\U0001f1fc",
- ":flag_for_tajikistan:": "\U0001f1f9\U0001f1ef",
- ":flag_for_tanzania:": "\U0001f1f9\U0001f1ff",
- ":flag_for_thailand:": "\U0001f1f9\U0001f1ed",
- ":flag_for_timor_leste:": "\U0001f1f9\U0001f1f1",
- ":flag_for_togo:": "\U0001f1f9\U0001f1ec",
- ":flag_for_tokelau:": "\U0001f1f9\U0001f1f0",
- ":flag_for_tonga:": "\U0001f1f9\U0001f1f4",
- ":flag_for_trinidad_and_tobago:": "\U0001f1f9\U0001f1f9",
- ":flag_for_tristan_da_cunha:": "\U0001f1f9\U0001f1e6",
- ":flag_for_tunisia:": "\U0001f1f9\U0001f1f3",
- ":flag_for_turkey:": "\U0001f1f9\U0001f1f7",
- ":flag_for_turkmenistan:": "\U0001f1f9\U0001f1f2",
- ":flag_for_turks_and_caicos_islands:": "\U0001f1f9\U0001f1e8",
- ":flag_for_tuvalu:": "\U0001f1f9\U0001f1fb",
- ":flag_for_uganda:": "\U0001f1fa\U0001f1ec",
- ":flag_for_ukraine:": "\U0001f1fa\U0001f1e6",
- ":flag_for_united_arab_emirates:": "\U0001f1e6\U0001f1ea",
- ":flag_for_united_kingdom:": "\U0001f1ec\U0001f1e7",
- ":flag_for_united_nations:": "\U0001f1fa\U0001f1f3",
- ":flag_for_united_states:": "\U0001f1fa\U0001f1f8",
- ":flag_for_uruguay:": "\U0001f1fa\U0001f1fe",
- ":flag_for_us_outlying_islands:": "\U0001f1fa\U0001f1f2",
- ":flag_for_us_virgin_islands:": "\U0001f1fb\U0001f1ee",
- ":flag_for_uzbekistan:": "\U0001f1fa\U0001f1ff",
- ":flag_for_vanuatu:": "\U0001f1fb\U0001f1fa",
- ":flag_for_vatican_city:": "\U0001f1fb\U0001f1e6",
- ":flag_for_venezuela:": "\U0001f1fb\U0001f1ea",
- ":flag_for_vietnam:": "\U0001f1fb\U0001f1f3",
- ":flag_for_wales:": "\U0001f3f4\U000e0067\U000e0062\U000e0077\U000e006c\U000e0073\U000e007f",
- ":flag_for_wallis_and_futuna:": "\U0001f1fc\U0001f1eb",
- ":flag_for_western_sahara:": "\U0001f1ea\U0001f1ed",
- ":flag_for_yemen:": "\U0001f1fe\U0001f1ea",
- ":flag_for_zambia:": "\U0001f1ff\U0001f1f2",
- ":flag_for_zimbabwe:": "\U0001f1ff\U0001f1fc",
- ":flag_in_hole:": "\u26f3",
- ":flags:": "\U0001f38f",
- ":flamingo:": "\U0001f9a9",
- ":flashlight:": "\U0001f526",
- ":flat_shoe:": "\U0001f97f",
- ":flatbread:": "\U0001fad3",
- ":fleur_de_lis:": "\u269c\ufe0f",
- ":flexed_biceps:": "\U0001f4aa",
- ":flight_arrival:": "\U0001f6ec",
- ":flight_departure:": "\U0001f6eb",
- ":flipper:": "\U0001f42c",
- ":floppy_disk:": "\U0001f4be",
- ":flower_playing_cards:": "\U0001f3b4",
- ":flushed:": "\U0001f633",
- ":flushed_face:": "\U0001f633",
- ":fly:": "\U0001fab0",
- ":flying_disc:": "\U0001f94f",
- ":flying_saucer:": "\U0001f6f8",
- ":fog:": "\U0001f32b\ufe0f",
- ":foggy:": "\U0001f301",
- ":folded_hands:": "\U0001f64f",
- ":fondue:": "\U0001fad5",
- ":foot:": "\U0001f9b6",
- ":football:": "\U0001f3c8",
- ":footprints:": "\U0001f463",
- ":fork_and_knife:": "\U0001f374",
- ":fork_and_knife_with_plate:": "\U0001f37d\ufe0f",
- ":fortune_cookie:": "\U0001f960",
- ":fountain:": "\u26f2",
- ":fountain_pen:": "\U0001f58b\ufe0f",
- ":four:": "4\ufe0f\u20e3",
- ":four_leaf_clover:": "\U0001f340",
- ":four_o_clock:": "\U0001f553",
- ":four_thirty:": "\U0001f55f",
- ":fox:": "\U0001f98a",
- ":fox_face:": "\U0001f98a",
- ":fr:": "\U0001f1eb\U0001f1f7",
- ":framed_picture:": "\U0001f5bc\ufe0f",
- ":free:": "\U0001f193",
- ":free_button:": "\U0001f193",
- ":french_fries:": "\U0001f35f",
- ":french_guiana:": "\U0001f1ec\U0001f1eb",
- ":french_polynesia:": "\U0001f1f5\U0001f1eb",
- ":french_southern_territories:": "\U0001f1f9\U0001f1eb",
- ":fried_egg:": "\U0001f373",
- ":fried_shrimp:": "\U0001f364",
- ":fries:": "\U0001f35f",
- ":frog:": "\U0001f438",
- ":front_facing_baby_chick:": "\U0001f425",
- ":frowning:": "\U0001f626",
- ":frowning_face:": "\u2639\ufe0f",
- ":frowning_face_with_open_mouth:": "\U0001f626",
- ":frowning_man:": "\U0001f64d\u200d\u2642\ufe0f",
- ":frowning_person:": "\U0001f64d",
- ":frowning_woman:": "\U0001f64d\u200d\u2640\ufe0f",
- ":fu:": "\U0001f595",
- ":fuel_pump:": "\u26fd",
- ":fuelpump:": "\u26fd",
- ":full_moon:": "\U0001f315",
- ":full_moon_face:": "\U0001f31d",
- ":full_moon_with_face:": "\U0001f31d",
- ":funeral_urn:": "\u26b1\ufe0f",
- ":gabon:": "\U0001f1ec\U0001f1e6",
- ":gambia:": "\U0001f1ec\U0001f1f2",
- ":game_die:": "\U0001f3b2",
- ":garlic:": "\U0001f9c4",
- ":gb:": "\U0001f1ec\U0001f1e7",
- ":gear:": "\u2699\ufe0f",
- ":gem:": "\U0001f48e",
- ":gem_stone:": "\U0001f48e",
- ":gemini:": "\u264a",
- ":genie:": "\U0001f9de",
- ":genie_man:": "\U0001f9de\u200d\u2642\ufe0f",
- ":genie_woman:": "\U0001f9de\u200d\u2640\ufe0f",
- ":georgia:": "\U0001f1ec\U0001f1ea",
- ":ghana:": "\U0001f1ec\U0001f1ed",
- ":ghost:": "\U0001f47b",
- ":gibraltar:": "\U0001f1ec\U0001f1ee",
- ":gift:": "\U0001f381",
- ":gift_heart:": "\U0001f49d",
- ":giraffe:": "\U0001f992",
- ":girl:": "\U0001f467",
- ":glass_of_milk:": "\U0001f95b",
- ":glasses:": "\U0001f453",
- ":globe_showing_americas:": "\U0001f30e",
- ":globe_showing_asia_australia:": "\U0001f30f",
- ":globe_showing_europe_africa:": "\U0001f30d",
- ":globe_with_meridians:": "\U0001f310",
- ":gloves:": "\U0001f9e4",
- ":glowing_star:": "\U0001f31f",
- ":goal_net:": "\U0001f945",
- ":goat:": "\U0001f410",
- ":goblin:": "\U0001f47a",
- ":goggles:": "\U0001f97d",
- ":golf:": "\u26f3",
- ":golfing:": "\U0001f3cc\ufe0f",
- ":golfing_man:": "\U0001f3cc\ufe0f\u200d\u2642\ufe0f",
- ":golfing_woman:": "\U0001f3cc\ufe0f\u200d\u2640\ufe0f",
- ":gorilla:": "\U0001f98d",
- ":graduation_cap:": "\U0001f393",
- ":grapes:": "\U0001f347",
- ":greece:": "\U0001f1ec\U0001f1f7",
- ":green_apple:": "\U0001f34f",
- ":green_book:": "\U0001f4d7",
- ":green_circle:": "\U0001f7e2",
- ":green_heart:": "\U0001f49a",
- ":green_salad:": "\U0001f957",
- ":green_square:": "\U0001f7e9",
- ":greenland:": "\U0001f1ec\U0001f1f1",
- ":grenada:": "\U0001f1ec\U0001f1e9",
- ":grey_exclamation:": "\u2755",
- ":grey_question:": "\u2754",
- ":grimacing:": "\U0001f62c",
- ":grimacing_face:": "\U0001f62c",
- ":grin:": "\U0001f601",
- ":grinning:": "\U0001f600",
- ":grinning_cat:": "\U0001f63a",
- ":grinning_cat_with_smiling_eyes:": "\U0001f638",
- ":grinning_face:": "\U0001f600",
- ":grinning_face_with_big_eyes:": "\U0001f603",
- ":grinning_face_with_smiling_eyes:": "\U0001f604",
- ":grinning_face_with_sweat:": "\U0001f605",
- ":grinning_squinting_face:": "\U0001f606",
- ":growing_heart:": "\U0001f497",
- ":guadeloupe:": "\U0001f1ec\U0001f1f5",
- ":guam:": "\U0001f1ec\U0001f1fa",
- ":guard:": "\U0001f482",
- ":guardsman:": "\U0001f482\u200d\u2642\ufe0f",
- ":guardswoman:": "\U0001f482\u200d\u2640\ufe0f",
- ":guatemala:": "\U0001f1ec\U0001f1f9",
- ":guernsey:": "\U0001f1ec\U0001f1ec",
- ":guide_dog:": "\U0001f9ae",
- ":guinea:": "\U0001f1ec\U0001f1f3",
- ":guinea_bissau:": "\U0001f1ec\U0001f1fc",
- ":guitar:": "\U0001f3b8",
- ":gun:": "\U0001f52b",
- ":guyana:": "\U0001f1ec\U0001f1fe",
- ":haircut:": "\U0001f487",
- ":haircut_man:": "\U0001f487\u200d\u2642\ufe0f",
- ":haircut_woman:": "\U0001f487\u200d\u2640\ufe0f",
- ":haiti:": "\U0001f1ed\U0001f1f9",
- ":hamburger:": "\U0001f354",
- ":hammer:": "\U0001f528",
- ":hammer_and_pick:": "\u2692\ufe0f",
- ":hammer_and_wrench:": "\U0001f6e0\ufe0f",
- ":hamster:": "\U0001f439",
- ":hand:": "\u270b",
- ":hand_over_mouth:": "\U0001f92d",
- ":hand_with_fingers_splayed:": "\U0001f590\ufe0f",
- ":handbag:": "\U0001f45c",
- ":handball_person:": "\U0001f93e",
- ":handshake:": "\U0001f91d",
- ":hankey:": "\U0001f4a9",
- ":hash:": "#\ufe0f\u20e3",
- ":hatched_chick:": "\U0001f425",
- ":hatching_chick:": "\U0001f423",
- ":headphone:": "\U0001f3a7",
- ":headphones:": "\U0001f3a7",
- ":headstone:": "\U0001faa6",
- ":health_worker:": "\U0001f9d1\u200d\u2695\ufe0f",
- ":hear_no_evil:": "\U0001f649",
- ":hear_no_evil_monkey:": "\U0001f649",
- ":heard_mcdonald_islands:": "\U0001f1ed\U0001f1f2",
- ":heart:": "\u2764\ufe0f",
- ":heart_decoration:": "\U0001f49f",
- ":heart_exclamation:": "\u2763\ufe0f",
- ":heart_eyes:": "\U0001f60d",
- ":heart_eyes_cat:": "\U0001f63b",
- ":heart_suit:": "\u2665\ufe0f",
- ":heart_with_arrow:": "\U0001f498",
- ":heart_with_ribbon:": "\U0001f49d",
- ":heartbeat:": "\U0001f493",
- ":heartpulse:": "\U0001f497",
- ":hearts:": "\u2665\ufe0f",
- ":heavy_check_mark:": "\u2714\ufe0f",
- ":heavy_division_sign:": "\u2797",
- ":heavy_dollar_sign:": "\U0001f4b2",
- ":heavy_exclamation_mark:": "\u2757",
- ":heavy_heart_exclamation:": "\u2763\ufe0f",
- ":heavy_minus_sign:": "\u2796",
- ":heavy_multiplication_x:": "\u2716\ufe0f",
- ":heavy_plus_sign:": "\u2795",
- ":hedgehog:": "\U0001f994",
- ":helicopter:": "\U0001f681",
- ":herb:": "\U0001f33f",
- ":hibiscus:": "\U0001f33a",
- ":high_brightness:": "\U0001f506",
- ":high_heel:": "\U0001f460",
- ":high_heeled_shoe:": "\U0001f460",
- ":high_speed_train:": "\U0001f684",
- ":high_voltage:": "\u26a1",
- ":hiking_boot:": "\U0001f97e",
- ":hindu_temple:": "\U0001f6d5",
- ":hippopotamus:": "\U0001f99b",
- ":hocho:": "\U0001f52a",
- ":hole:": "\U0001f573\ufe0f",
- ":hollow_red_circle:": "\u2b55",
- ":honduras:": "\U0001f1ed\U0001f1f3",
- ":honey_pot:": "\U0001f36f",
- ":honeybee:": "\U0001f41d",
- ":hong_kong:": "\U0001f1ed\U0001f1f0",
- ":hook:": "\U0001fa9d",
- ":horizontal_traffic_light:": "\U0001f6a5",
- ":horse:": "\U0001f434",
- ":horse_face:": "\U0001f434",
- ":horse_racing:": "\U0001f3c7",
- ":hospital:": "\U0001f3e5",
- ":hot_beverage:": "\u2615",
- ":hot_dog:": "\U0001f32d",
- ":hot_face:": "\U0001f975",
- ":hot_pepper:": "\U0001f336\ufe0f",
- ":hot_springs:": "\u2668\ufe0f",
- ":hotdog:": "\U0001f32d",
- ":hotel:": "\U0001f3e8",
- ":hotsprings:": "\u2668\ufe0f",
- ":hourglass:": "\u231b",
- ":hourglass_done:": "\u231b",
- ":hourglass_flowing_sand:": "\u23f3",
- ":hourglass_not_done:": "\u23f3",
- ":house:": "\U0001f3e0",
- ":house_with_garden:": "\U0001f3e1",
- ":houses:": "\U0001f3d8\ufe0f",
- ":hugging_face:": "\U0001f917",
- ":hugs:": "\U0001f917",
- ":hundred_points:": "\U0001f4af",
- ":hungary:": "\U0001f1ed\U0001f1fa",
- ":hushed:": "\U0001f62f",
- ":hushed_face:": "\U0001f62f",
- ":hut:": "\U0001f6d6",
- ":ice:": "\U0001f9ca",
- ":ice_cream:": "\U0001f368",
- ":ice_cube:": "\U0001f9ca",
- ":ice_hockey:": "\U0001f3d2",
- ":ice_skate:": "\u26f8\ufe0f",
- ":icecream:": "\U0001f366",
- ":iceland:": "\U0001f1ee\U0001f1f8",
- ":id:": "\U0001f194",
- ":id_button:": "\U0001f194",
- ":ideograph_advantage:": "\U0001f250",
- ":imp:": "\U0001f47f",
- ":inbox_tray:": "\U0001f4e5",
- ":incoming_envelope:": "\U0001f4e8",
- ":index_pointing_up:": "\u261d\ufe0f",
- ":india:": "\U0001f1ee\U0001f1f3",
- ":indonesia:": "\U0001f1ee\U0001f1e9",
- ":infinity:": "\u267e\ufe0f",
- ":information:": "\u2139\ufe0f",
- ":information_desk_person:": "\U0001f481",
- ":information_source:": "\u2139\ufe0f",
- ":innocent:": "\U0001f607",
- ":input_latin_letters:": "\U0001f524",
- ":input_latin_lowercase:": "\U0001f521",
- ":input_latin_uppercase:": "\U0001f520",
- ":input_numbers:": "\U0001f522",
- ":input_symbols:": "\U0001f523",
- ":interrobang:": "\u2049\ufe0f",
- ":iphone:": "\U0001f4f1",
- ":iran:": "\U0001f1ee\U0001f1f7",
- ":iraq:": "\U0001f1ee\U0001f1f6",
- ":ireland:": "\U0001f1ee\U0001f1ea",
- ":isle_of_man:": "\U0001f1ee\U0001f1f2",
- ":israel:": "\U0001f1ee\U0001f1f1",
- ":it:": "\U0001f1ee\U0001f1f9",
- ":izakaya_lantern:": "\U0001f3ee",
- ":jack_o_lantern:": "\U0001f383",
- ":jamaica:": "\U0001f1ef\U0001f1f2",
- ":japan:": "\U0001f5fe",
- ":japanese_acceptable_button:": "\U0001f251",
- ":japanese_application_button:": "\U0001f238",
- ":japanese_bargain_button:": "\U0001f250",
- ":japanese_castle:": "\U0001f3ef",
- ":japanese_congratulations_button:": "\u3297\ufe0f",
- ":japanese_discount_button:": "\U0001f239",
- ":japanese_dolls:": "\U0001f38e",
- ":japanese_free_of_charge_button:": "\U0001f21a",
- ":japanese_goblin:": "\U0001f47a",
- ":japanese_here_button:": "\U0001f201",
- ":japanese_monthly_amount_button:": "\U0001f237\ufe0f",
- ":japanese_no_vacancy_button:": "\U0001f235",
- ":japanese_not_free_of_charge_button:": "\U0001f236",
- ":japanese_ogre:": "\U0001f479",
- ":japanese_open_for_business_button:": "\U0001f23a",
- ":japanese_passing_grade_button:": "\U0001f234",
- ":japanese_post_office:": "\U0001f3e3",
- ":japanese_prohibited_button:": "\U0001f232",
- ":japanese_reserved_button:": "\U0001f22f",
- ":japanese_secret_button:": "\u3299\ufe0f",
- ":japanese_service_charge_button:": "\U0001f202\ufe0f",
- ":japanese_symbol_for_beginner:": "\U0001f530",
- ":japanese_vacancy_button:": "\U0001f233",
- ":jeans:": "\U0001f456",
- ":jersey:": "\U0001f1ef\U0001f1ea",
- ":jigsaw:": "\U0001f9e9",
- ":joker:": "\U0001f0cf",
- ":jordan:": "\U0001f1ef\U0001f1f4",
- ":joy:": "\U0001f602",
- ":joy_cat:": "\U0001f639",
- ":joystick:": "\U0001f579\ufe0f",
- ":jp:": "\U0001f1ef\U0001f1f5",
- ":judge:": "\U0001f9d1\u200d\u2696\ufe0f",
- ":juggling_person:": "\U0001f939",
- ":kaaba:": "\U0001f54b",
- ":kangaroo:": "\U0001f998",
- ":kazakhstan:": "\U0001f1f0\U0001f1ff",
- ":kenya:": "\U0001f1f0\U0001f1ea",
- ":key:": "\U0001f511",
- ":keyboard:": "\u2328\ufe0f",
- ":keycap_0:": "0\ufe0f\u20e3",
- ":keycap_10:": "\U0001f51f",
- ":keycap_1:": "1\ufe0f\u20e3",
- ":keycap_2:": "2\ufe0f\u20e3",
- ":keycap_3:": "3\ufe0f\u20e3",
- ":keycap_4:": "4\ufe0f\u20e3",
- ":keycap_5:": "5\ufe0f\u20e3",
- ":keycap_6:": "6\ufe0f\u20e3",
- ":keycap_7:": "7\ufe0f\u20e3",
- ":keycap_8:": "8\ufe0f\u20e3",
- ":keycap_9:": "9\ufe0f\u20e3",
- ":keycap_asterisk:": "*\ufe0f\u20e3",
- ":keycap_hash:": "#\ufe0f\u20e3",
- ":keycap_ten:": "\U0001f51f",
- ":kick_scooter:": "\U0001f6f4",
- ":kimono:": "\U0001f458",
- ":kiribati:": "\U0001f1f0\U0001f1ee",
- ":kiss:": "\U0001f48b",
- ":kiss_man_man:": "\U0001f468\u200d\u2764\ufe0f\u200d\U0001f48b\u200d\U0001f468",
- ":kiss_mark:": "\U0001f48b",
- ":kiss_woman_man:": "\U0001f469\u200d\u2764\ufe0f\u200d\U0001f48b\u200d\U0001f468",
- ":kiss_woman_woman:": "\U0001f469\u200d\u2764\ufe0f\u200d\U0001f48b\u200d\U0001f469",
- ":kissing:": "\U0001f617",
- ":kissing_cat:": "\U0001f63d",
- ":kissing_closed_eyes:": "\U0001f61a",
- ":kissing_face:": "\U0001f617",
- ":kissing_face_with_closed_eyes:": "\U0001f61a",
- ":kissing_face_with_smiling_eyes:": "\U0001f619",
- ":kissing_heart:": "\U0001f618",
- ":kissing_smiling_eyes:": "\U0001f619",
- ":kitchen_knife:": "\U0001f52a",
- ":kite:": "\U0001fa81",
- ":kiwi_fruit:": "\U0001f95d",
- ":kneeling_man:": "\U0001f9ce\u200d\u2642\ufe0f",
- ":kneeling_person:": "\U0001f9ce",
- ":kneeling_woman:": "\U0001f9ce\u200d\u2640\ufe0f",
- ":knife:": "\U0001f52a",
- ":knot:": "\U0001faa2",
- ":koala:": "\U0001f428",
- ":koko:": "\U0001f201",
- ":kosovo:": "\U0001f1fd\U0001f1f0",
- ":kr:": "\U0001f1f0\U0001f1f7",
- ":kuwait:": "\U0001f1f0\U0001f1fc",
- ":kyrgyzstan:": "\U0001f1f0\U0001f1ec",
- ":lab_coat:": "\U0001f97c",
- ":label:": "\U0001f3f7\ufe0f",
- ":lacrosse:": "\U0001f94d",
- ":ladder:": "\U0001fa9c",
- ":lady_beetle:": "\U0001f41e",
- ":lantern:": "\U0001f3ee",
- ":laos:": "\U0001f1f1\U0001f1e6",
- ":laptop:": "\U0001f4bb",
- ":large_blue_circle:": "\U0001f535",
- ":large_blue_diamond:": "\U0001f537",
- ":large_orange_diamond:": "\U0001f536",
- ":last_quarter_moon:": "\U0001f317",
- ":last_quarter_moon_face:": "\U0001f31c",
- ":last_quarter_moon_with_face:": "\U0001f31c",
- ":last_track_button:": "\u23ee\ufe0f",
- ":latin_cross:": "\u271d\ufe0f",
- ":latvia:": "\U0001f1f1\U0001f1fb",
- ":laughing:": "\U0001f606",
- ":leaf_fluttering_in_wind:": "\U0001f343",
- ":leafy_green:": "\U0001f96c",
- ":leaves:": "\U0001f343",
- ":lebanon:": "\U0001f1f1\U0001f1e7",
- ":ledger:": "\U0001f4d2",
- ":left_arrow:": "\u2b05\ufe0f",
- ":left_arrow_curving_right:": "\u21aa\ufe0f",
- ":left_facing_fist:": "\U0001f91b",
- ":left_luggage:": "\U0001f6c5",
- ":left_right_arrow:": "\u2194\ufe0f",
- ":left_speech_bubble:": "\U0001f5e8\ufe0f",
- ":leftwards_arrow_with_hook:": "\u21a9\ufe0f",
- ":leg:": "\U0001f9b5",
- ":lemon:": "\U0001f34b",
- ":leo:": "\u264c",
- ":leopard:": "\U0001f406",
- ":lesotho:": "\U0001f1f1\U0001f1f8",
- ":level_slider:": "\U0001f39a\ufe0f",
- ":liberia:": "\U0001f1f1\U0001f1f7",
- ":libra:": "\u264e",
- ":libya:": "\U0001f1f1\U0001f1fe",
- ":liechtenstein:": "\U0001f1f1\U0001f1ee",
- ":light_bulb:": "\U0001f4a1",
- ":light_rail:": "\U0001f688",
- ":light_skin_tone:": "\U0001f3fb",
- ":link:": "\U0001f517",
- ":linked_paperclips:": "\U0001f587\ufe0f",
- ":lion:": "\U0001f981",
- ":lips:": "\U0001f444",
- ":lipstick:": "\U0001f484",
- ":lithuania:": "\U0001f1f1\U0001f1f9",
- ":litter_in_bin_sign:": "\U0001f6ae",
- ":lizard:": "\U0001f98e",
- ":llama:": "\U0001f999",
- ":lobster:": "\U0001f99e",
- ":lock:": "\U0001f512",
- ":lock_with_ink_pen:": "\U0001f50f",
- ":locked:": "\U0001f512",
- ":locked_with_key:": "\U0001f510",
- ":locked_with_pen:": "\U0001f50f",
- ":locomotive:": "\U0001f682",
- ":lollipop:": "\U0001f36d",
- ":long_drum:": "\U0001fa98",
- ":loop:": "\u27bf",
- ":lotion_bottle:": "\U0001f9f4",
- ":lotus_position:": "\U0001f9d8",
- ":lotus_position_man:": "\U0001f9d8\u200d\u2642\ufe0f",
- ":lotus_position_woman:": "\U0001f9d8\u200d\u2640\ufe0f",
- ":loud_sound:": "\U0001f50a",
- ":loudly_crying_face:": "\U0001f62d",
- ":loudspeaker:": "\U0001f4e2",
- ":love_hotel:": "\U0001f3e9",
- ":love_letter:": "\U0001f48c",
- ":love_you_gesture:": "\U0001f91f",
- ":low_brightness:": "\U0001f505",
- ":luggage:": "\U0001f9f3",
- ":lungs:": "\U0001fac1",
- ":luxembourg:": "\U0001f1f1\U0001f1fa",
- ":lying_face:": "\U0001f925",
- ":m:": "\u24c2\ufe0f",
- ":macau:": "\U0001f1f2\U0001f1f4",
- ":macedonia:": "\U0001f1f2\U0001f1f0",
- ":madagascar:": "\U0001f1f2\U0001f1ec",
- ":mag:": "\U0001f50d",
- ":mag_right:": "\U0001f50e",
- ":mage:": "\U0001f9d9",
- ":mage_man:": "\U0001f9d9\u200d\u2642\ufe0f",
- ":mage_woman:": "\U0001f9d9\u200d\u2640\ufe0f",
- ":magic_wand:": "\U0001fa84",
- ":magnet:": "\U0001f9f2",
- ":magnifying_glass_tilted_left:": "\U0001f50d",
- ":magnifying_glass_tilted_right:": "\U0001f50e",
- ":mahjong:": "\U0001f004",
- ":mahjong_red_dragon:": "\U0001f004",
- ":mailbox:": "\U0001f4eb",
- ":mailbox_closed:": "\U0001f4ea",
- ":mailbox_with_mail:": "\U0001f4ec",
- ":mailbox_with_no_mail:": "\U0001f4ed",
- ":malawi:": "\U0001f1f2\U0001f1fc",
- ":malaysia:": "\U0001f1f2\U0001f1fe",
- ":maldives:": "\U0001f1f2\U0001f1fb",
- ":male_detective:": "\U0001f575\ufe0f\u200d\u2642\ufe0f",
- ":male_sign:": "\u2642\ufe0f",
- ":mali:": "\U0001f1f2\U0001f1f1",
- ":malta:": "\U0001f1f2\U0001f1f9",
- ":mammoth:": "\U0001f9a3",
- ":man:": "\U0001f468",
- ":man_artist:": "\U0001f468\u200d\U0001f3a8",
- ":man_astronaut:": "\U0001f468\u200d\U0001f680",
- ":man_bald:": "\U0001f468\u200d\U0001f9b2",
- ":man_biking:": "\U0001f6b4\u200d\u2642\ufe0f",
- ":man_bouncing_ball:": "\u26f9\ufe0f\u200d\u2642\ufe0f",
- ":man_bowing:": "\U0001f647\u200d\u2642\ufe0f",
- ":man_cartwheeling:": "\U0001f938\u200d\u2642\ufe0f",
- ":man_climbing:": "\U0001f9d7\u200d\u2642\ufe0f",
- ":man_construction_worker:": "\U0001f477\u200d\u2642\ufe0f",
- ":man_cook:": "\U0001f468\u200d\U0001f373",
- ":man_dancing:": "\U0001f57a",
- ":man_detective:": "\U0001f575\ufe0f\u200d\u2642\ufe0f",
- ":man_elf:": "\U0001f9dd\u200d\u2642\ufe0f",
- ":man_facepalming:": "\U0001f926\u200d\u2642\ufe0f",
- ":man_factory_worker:": "\U0001f468\u200d\U0001f3ed",
- ":man_fairy:": "\U0001f9da\u200d\u2642\ufe0f",
- ":man_farmer:": "\U0001f468\u200d\U0001f33e",
- ":man_feeding_baby:": "\U0001f468\u200d\U0001f37c",
- ":man_firefighter:": "\U0001f468\u200d\U0001f692",
- ":man_frowning:": "\U0001f64d\u200d\u2642\ufe0f",
- ":man_genie:": "\U0001f9de\u200d\u2642\ufe0f",
- ":man_gesturing_no:": "\U0001f645\u200d\u2642\ufe0f",
- ":man_gesturing_ok:": "\U0001f646\u200d\u2642\ufe0f",
- ":man_getting_haircut:": "\U0001f487\u200d\u2642\ufe0f",
- ":man_getting_massage:": "\U0001f486\u200d\u2642\ufe0f",
- ":man_golfing:": "\U0001f3cc\ufe0f\u200d\u2642\ufe0f",
- ":man_guard:": "\U0001f482\u200d\u2642\ufe0f",
- ":man_health_worker:": "\U0001f468\u200d\u2695\ufe0f",
- ":man_in_lotus_position:": "\U0001f9d8\u200d\u2642\ufe0f",
- ":man_in_manual_wheelchair:": "\U0001f468\u200d\U0001f9bd",
- ":man_in_motorized_wheelchair:": "\U0001f468\u200d\U0001f9bc",
- ":man_in_steamy_room:": "\U0001f9d6\u200d\u2642\ufe0f",
- ":man_in_tuxedo:": "\U0001f935\u200d\u2642\ufe0f",
- ":man_judge:": "\U0001f468\u200d\u2696\ufe0f",
- ":man_juggling:": "\U0001f939\u200d\u2642\ufe0f",
- ":man_kneeling:": "\U0001f9ce\u200d\u2642\ufe0f",
- ":man_lifting_weights:": "\U0001f3cb\ufe0f\u200d\u2642\ufe0f",
- ":man_mage:": "\U0001f9d9\u200d\u2642\ufe0f",
- ":man_mechanic:": "\U0001f468\u200d\U0001f527",
- ":man_mountain_biking:": "\U0001f6b5\u200d\u2642\ufe0f",
- ":man_office_worker:": "\U0001f468\u200d\U0001f4bc",
- ":man_pilot:": "\U0001f468\u200d\u2708\ufe0f",
- ":man_playing_handball:": "\U0001f93e\u200d\u2642\ufe0f",
- ":man_playing_water_polo:": "\U0001f93d\u200d\u2642\ufe0f",
- ":man_police_officer:": "\U0001f46e\u200d\u2642\ufe0f",
- ":man_pouting:": "\U0001f64e\u200d\u2642\ufe0f",
- ":man_raising_hand:": "\U0001f64b\u200d\u2642\ufe0f",
- ":man_rowing_boat:": "\U0001f6a3\u200d\u2642\ufe0f",
- ":man_running:": "\U0001f3c3\u200d\u2642\ufe0f",
- ":man_s_shoe:": "\U0001f45e",
- ":man_scientist:": "\U0001f468\u200d\U0001f52c",
- ":man_shrugging:": "\U0001f937\u200d\u2642\ufe0f",
- ":man_singer:": "\U0001f468\u200d\U0001f3a4",
- ":man_standing:": "\U0001f9cd\u200d\u2642\ufe0f",
- ":man_student:": "\U0001f468\u200d\U0001f393",
- ":man_superhero:": "\U0001f9b8\u200d\u2642\ufe0f",
- ":man_supervillain:": "\U0001f9b9\u200d\u2642\ufe0f",
- ":man_surfing:": "\U0001f3c4\u200d\u2642\ufe0f",
- ":man_swimming:": "\U0001f3ca\u200d\u2642\ufe0f",
- ":man_teacher:": "\U0001f468\u200d\U0001f3eb",
- ":man_technologist:": "\U0001f468\u200d\U0001f4bb",
- ":man_tipping_hand:": "\U0001f481\u200d\u2642\ufe0f",
- ":man_vampire:": "\U0001f9db\u200d\u2642\ufe0f",
- ":man_walking:": "\U0001f6b6\u200d\u2642\ufe0f",
- ":man_wearing_turban:": "\U0001f473\u200d\u2642\ufe0f",
- ":man_with_beard:": "\U0001f9d4",
- ":man_with_blond_hair:": "\U0001f471\u200d\u2642\ufe0f",
- ":man_with_curly_hair:": "\U0001f468\u200d\U0001f9b1",
- ":man_with_gua_pi_mao:": "\U0001f472",
- ":man_with_probing_cane:": "\U0001f468\u200d\U0001f9af",
- ":man_with_red_hair:": "\U0001f468\u200d\U0001f9b0",
- ":man_with_turban:": "\U0001f473\u200d\u2642\ufe0f",
- ":man_with_veil:": "\U0001f470\u200d\u2642\ufe0f",
- ":man_with_white_cane:": "\U0001f468\u200d\U0001f9af",
- ":man_with_white_hair:": "\U0001f468\u200d\U0001f9b3",
- ":man_zombie:": "\U0001f9df\u200d\u2642\ufe0f",
- ":mandarin:": "\U0001f34a",
- ":mango:": "\U0001f96d",
- ":mans_shoe:": "\U0001f45e",
- ":mantelpiece_clock:": "\U0001f570\ufe0f",
- ":manual_wheelchair:": "\U0001f9bd",
- ":map_of_japan:": "\U0001f5fe",
- ":maple_leaf:": "\U0001f341",
- ":marshall_islands:": "\U0001f1f2\U0001f1ed",
- ":martial_arts_uniform:": "\U0001f94b",
- ":martinique:": "\U0001f1f2\U0001f1f6",
- ":mask:": "\U0001f637",
- ":massage:": "\U0001f486",
- ":massage_man:": "\U0001f486\u200d\u2642\ufe0f",
- ":massage_woman:": "\U0001f486\u200d\u2640\ufe0f",
- ":mate:": "\U0001f9c9",
- ":mauritania:": "\U0001f1f2\U0001f1f7",
- ":mauritius:": "\U0001f1f2\U0001f1fa",
- ":mayotte:": "\U0001f1fe\U0001f1f9",
- ":meat_on_bone:": "\U0001f356",
- ":mechanic:": "\U0001f9d1\u200d\U0001f527",
- ":mechanical_arm:": "\U0001f9be",
- ":mechanical_leg:": "\U0001f9bf",
- ":medal_military:": "\U0001f396\ufe0f",
- ":medal_sports:": "\U0001f3c5",
- ":medical_symbol:": "\u2695\ufe0f",
- ":medium_dark_skin_tone:": "\U0001f3fe",
- ":medium_light_skin_tone:": "\U0001f3fc",
- ":medium_skin_tone:": "\U0001f3fd",
- ":mega:": "\U0001f4e3",
- ":megaphone:": "\U0001f4e3",
- ":melon:": "\U0001f348",
- ":memo:": "\U0001f4dd",
- ":men_holding_hands:": "\U0001f46c",
- ":men_s_room:": "\U0001f6b9",
- ":men_with_bunny_ears:": "\U0001f46f\u200d\u2642\ufe0f",
- ":men_wrestling:": "\U0001f93c\u200d\u2642\ufe0f",
- ":menorah:": "\U0001f54e",
- ":mens:": "\U0001f6b9",
- ":mermaid:": "\U0001f9dc\u200d\u2640\ufe0f",
- ":merman:": "\U0001f9dc\u200d\u2642\ufe0f",
- ":merperson:": "\U0001f9dc",
- ":metal:": "\U0001f918",
- ":metro:": "\U0001f687",
- ":mexico:": "\U0001f1f2\U0001f1fd",
- ":microbe:": "\U0001f9a0",
- ":micronesia:": "\U0001f1eb\U0001f1f2",
- ":microphone:": "\U0001f3a4",
- ":microscope:": "\U0001f52c",
- ":middle_finger:": "\U0001f595",
- ":military_helmet:": "\U0001fa96",
- ":military_medal:": "\U0001f396\ufe0f",
- ":milk_glass:": "\U0001f95b",
- ":milky_way:": "\U0001f30c",
- ":minibus:": "\U0001f690",
- ":minidisc:": "\U0001f4bd",
- ":minus:": "\u2796",
- ":mirror:": "\U0001fa9e",
- ":moai:": "\U0001f5ff",
- ":mobile_phone:": "\U0001f4f1",
- ":mobile_phone_off:": "\U0001f4f4",
- ":mobile_phone_with_arrow:": "\U0001f4f2",
- ":moldova:": "\U0001f1f2\U0001f1e9",
- ":monaco:": "\U0001f1f2\U0001f1e8",
- ":money_bag:": "\U0001f4b0",
- ":money_mouth_face:": "\U0001f911",
- ":money_with_wings:": "\U0001f4b8",
- ":moneybag:": "\U0001f4b0",
- ":mongolia:": "\U0001f1f2\U0001f1f3",
- ":monkey:": "\U0001f412",
- ":monkey_face:": "\U0001f435",
- ":monocle_face:": "\U0001f9d0",
- ":monorail:": "\U0001f69d",
- ":montenegro:": "\U0001f1f2\U0001f1ea",
- ":montserrat:": "\U0001f1f2\U0001f1f8",
- ":moon:": "\U0001f314",
- ":moon_cake:": "\U0001f96e",
- ":moon_viewing_ceremony:": "\U0001f391",
- ":morocco:": "\U0001f1f2\U0001f1e6",
- ":mortar_board:": "\U0001f393",
- ":mosque:": "\U0001f54c",
- ":mosquito:": "\U0001f99f",
- ":motor_boat:": "\U0001f6e5\ufe0f",
- ":motor_scooter:": "\U0001f6f5",
- ":motorcycle:": "\U0001f3cd\ufe0f",
- ":motorized_wheelchair:": "\U0001f9bc",
- ":motorway:": "\U0001f6e3\ufe0f",
- ":mount_fuji:": "\U0001f5fb",
- ":mountain:": "\u26f0\ufe0f",
- ":mountain_bicyclist:": "\U0001f6b5",
- ":mountain_biking_man:": "\U0001f6b5\u200d\u2642\ufe0f",
- ":mountain_biking_woman:": "\U0001f6b5\u200d\u2640\ufe0f",
- ":mountain_cableway:": "\U0001f6a0",
- ":mountain_railway:": "\U0001f69e",
- ":mountain_snow:": "\U0001f3d4\ufe0f",
- ":mouse2:": "\U0001f401",
- ":mouse:": "\U0001f42d",
- ":mouse_face:": "\U0001f42d",
- ":mouse_trap:": "\U0001faa4",
- ":mouth:": "\U0001f444",
- ":movie_camera:": "\U0001f3a5",
- ":moyai:": "\U0001f5ff",
- ":mozambique:": "\U0001f1f2\U0001f1ff",
- ":mrs_claus:": "\U0001f936",
- ":multiply:": "\u2716\ufe0f",
- ":muscle:": "\U0001f4aa",
- ":mushroom:": "\U0001f344",
- ":musical_keyboard:": "\U0001f3b9",
- ":musical_note:": "\U0001f3b5",
- ":musical_notes:": "\U0001f3b6",
- ":musical_score:": "\U0001f3bc",
- ":mute:": "\U0001f507",
- ":muted_speaker:": "\U0001f507",
- ":mx_claus:": "\U0001f9d1\u200d\U0001f384",
- ":myanmar:": "\U0001f1f2\U0001f1f2",
- ":nail_care:": "\U0001f485",
- ":nail_polish:": "\U0001f485",
- ":name_badge:": "\U0001f4db",
- ":namibia:": "\U0001f1f3\U0001f1e6",
- ":national_park:": "\U0001f3de\ufe0f",
- ":nauru:": "\U0001f1f3\U0001f1f7",
- ":nauseated_face:": "\U0001f922",
- ":nazar_amulet:": "\U0001f9ff",
- ":necktie:": "\U0001f454",
- ":negative_squared_cross_mark:": "\u274e",
- ":nepal:": "\U0001f1f3\U0001f1f5",
- ":nerd_face:": "\U0001f913",
- ":nesting_dolls:": "\U0001fa86",
- ":netherlands:": "\U0001f1f3\U0001f1f1",
- ":neutral_face:": "\U0001f610",
- ":new:": "\U0001f195",
- ":new_button:": "\U0001f195",
- ":new_caledonia:": "\U0001f1f3\U0001f1e8",
- ":new_moon:": "\U0001f311",
- ":new_moon_face:": "\U0001f31a",
- ":new_moon_with_face:": "\U0001f31a",
- ":new_zealand:": "\U0001f1f3\U0001f1ff",
- ":newspaper:": "\U0001f4f0",
- ":newspaper_roll:": "\U0001f5de\ufe0f",
- ":next_track_button:": "\u23ed\ufe0f",
- ":ng:": "\U0001f196",
- ":ng_button:": "\U0001f196",
- ":ng_man:": "\U0001f645\u200d\u2642\ufe0f",
- ":ng_woman:": "\U0001f645\u200d\u2640\ufe0f",
- ":nicaragua:": "\U0001f1f3\U0001f1ee",
- ":niger:": "\U0001f1f3\U0001f1ea",
- ":nigeria:": "\U0001f1f3\U0001f1ec",
- ":night_with_stars:": "\U0001f303",
- ":nine:": "9\ufe0f\u20e3",
- ":nine_o_clock:": "\U0001f558",
- ":nine_thirty:": "\U0001f564",
- ":ninja:": "\U0001f977",
- ":niue:": "\U0001f1f3\U0001f1fa",
- ":no_bell:": "\U0001f515",
- ":no_bicycles:": "\U0001f6b3",
- ":no_entry:": "\u26d4",
- ":no_entry_sign:": "\U0001f6ab",
- ":no_good:": "\U0001f645",
- ":no_good_man:": "\U0001f645\u200d\u2642\ufe0f",
- ":no_good_woman:": "\U0001f645\u200d\u2640\ufe0f",
- ":no_littering:": "\U0001f6af",
- ":no_mobile_phones:": "\U0001f4f5",
- ":no_mouth:": "\U0001f636",
- ":no_one_under_eighteen:": "\U0001f51e",
- ":no_pedestrians:": "\U0001f6b7",
- ":no_smoking:": "\U0001f6ad",
- ":non-potable_water:": "\U0001f6b1",
- ":non_potable_water:": "\U0001f6b1",
- ":norfolk_island:": "\U0001f1f3\U0001f1eb",
- ":north_korea:": "\U0001f1f0\U0001f1f5",
- ":northern_mariana_islands:": "\U0001f1f2\U0001f1f5",
- ":norway:": "\U0001f1f3\U0001f1f4",
- ":nose:": "\U0001f443",
- ":notebook:": "\U0001f4d3",
- ":notebook_with_decorative_cover:": "\U0001f4d4",
- ":notes:": "\U0001f3b6",
- ":nut_and_bolt:": "\U0001f529",
- ":o2:": "\U0001f17e\ufe0f",
- ":o:": "\u2b55",
- ":o_button_blood_type:": "\U0001f17e\ufe0f",
- ":ocean:": "\U0001f30a",
- ":octopus:": "\U0001f419",
- ":oden:": "\U0001f362",
- ":office:": "\U0001f3e2",
- ":office_building:": "\U0001f3e2",
- ":office_worker:": "\U0001f9d1\u200d\U0001f4bc",
- ":ogre:": "\U0001f479",
- ":oil_drum:": "\U0001f6e2\ufe0f",
- ":ok:": "\U0001f197",
- ":ok_button:": "\U0001f197",
- ":ok_hand:": "\U0001f44c",
- ":ok_man:": "\U0001f646\u200d\u2642\ufe0f",
- ":ok_person:": "\U0001f646",
- ":ok_woman:": "\U0001f646\u200d\u2640\ufe0f",
- ":old_key:": "\U0001f5dd\ufe0f",
- ":old_man:": "\U0001f474",
- ":old_woman:": "\U0001f475",
- ":older_adult:": "\U0001f9d3",
- ":older_man:": "\U0001f474",
- ":older_person:": "\U0001f9d3",
- ":older_woman:": "\U0001f475",
- ":olive:": "\U0001fad2",
- ":om:": "\U0001f549\ufe0f",
- ":oman:": "\U0001f1f4\U0001f1f2",
- ":on:": "\U0001f51b",
- ":on_arrow:": "\U0001f51b",
- ":oncoming_automobile:": "\U0001f698",
- ":oncoming_bus:": "\U0001f68d",
- ":oncoming_fist:": "\U0001f44a",
- ":oncoming_police_car:": "\U0001f694",
- ":oncoming_taxi:": "\U0001f696",
- ":one:": "1\ufe0f\u20e3",
- ":one_o_clock:": "\U0001f550",
- ":one_piece_swimsuit:": "\U0001fa71",
- ":one_thirty:": "\U0001f55c",
- ":onion:": "\U0001f9c5",
- ":open_book:": "\U0001f4d6",
- ":open_file_folder:": "\U0001f4c2",
- ":open_hands:": "\U0001f450",
- ":open_mailbox_with_lowered_flag:": "\U0001f4ed",
- ":open_mailbox_with_raised_flag:": "\U0001f4ec",
- ":open_mouth:": "\U0001f62e",
- ":open_umbrella:": "\u2602\ufe0f",
- ":ophiuchus:": "\u26ce",
- ":optical_disk:": "\U0001f4bf",
- ":orange:": "\U0001f34a",
- ":orange_book:": "\U0001f4d9",
- ":orange_circle:": "\U0001f7e0",
- ":orange_heart:": "\U0001f9e1",
- ":orange_square:": "\U0001f7e7",
- ":orangutan:": "\U0001f9a7",
- ":orthodox_cross:": "\u2626\ufe0f",
- ":otter:": "\U0001f9a6",
- ":outbox_tray:": "\U0001f4e4",
- ":owl:": "\U0001f989",
- ":ox:": "\U0001f402",
- ":oyster:": "\U0001f9aa",
- ":p_button:": "\U0001f17f\ufe0f",
- ":package:": "\U0001f4e6",
- ":page_facing_up:": "\U0001f4c4",
- ":page_with_curl:": "\U0001f4c3",
- ":pager:": "\U0001f4df",
- ":paintbrush:": "\U0001f58c\ufe0f",
- ":pakistan:": "\U0001f1f5\U0001f1f0",
- ":palau:": "\U0001f1f5\U0001f1fc",
- ":palestinian_territories:": "\U0001f1f5\U0001f1f8",
- ":palm_tree:": "\U0001f334",
- ":palms_up_together:": "\U0001f932",
- ":panama:": "\U0001f1f5\U0001f1e6",
- ":pancakes:": "\U0001f95e",
- ":panda:": "\U0001f43c",
- ":panda_face:": "\U0001f43c",
- ":paperclip:": "\U0001f4ce",
- ":paperclips:": "\U0001f587\ufe0f",
- ":papua_new_guinea:": "\U0001f1f5\U0001f1ec",
- ":parachute:": "\U0001fa82",
- ":paraguay:": "\U0001f1f5\U0001f1fe",
- ":parasol_on_ground:": "\u26f1\ufe0f",
- ":parking:": "\U0001f17f\ufe0f",
- ":parrot:": "\U0001f99c",
- ":part_alternation_mark:": "\u303d\ufe0f",
- ":partly_sunny:": "\u26c5",
- ":party_popper:": "\U0001f389",
- ":partying_face:": "\U0001f973",
- ":passenger_ship:": "\U0001f6f3\ufe0f",
- ":passport_control:": "\U0001f6c2",
- ":pause_button:": "\u23f8\ufe0f",
- ":paw_prints:": "\U0001f43e",
- ":peace_symbol:": "\u262e\ufe0f",
- ":peach:": "\U0001f351",
- ":peacock:": "\U0001f99a",
- ":peanuts:": "\U0001f95c",
- ":pear:": "\U0001f350",
- ":pen:": "\U0001f58a\ufe0f",
- ":pencil2:": "\u270f\ufe0f",
- ":pencil:": "\U0001f4dd",
- ":penguin:": "\U0001f427",
- ":pensive:": "\U0001f614",
- ":pensive_face:": "\U0001f614",
- ":people_holding_hands:": "\U0001f9d1\u200d\U0001f91d\u200d\U0001f9d1",
- ":people_hugging:": "\U0001fac2",
- ":people_with_bunny_ears:": "\U0001f46f",
- ":people_wrestling:": "\U0001f93c",
- ":performing_arts:": "\U0001f3ad",
- ":persevere:": "\U0001f623",
- ":persevering_face:": "\U0001f623",
- ":person:": "\U0001f9d1",
- ":person_bald:": "\U0001f9d1\u200d\U0001f9b2",
- ":person_biking:": "\U0001f6b4",
- ":person_bouncing_ball:": "\u26f9\ufe0f",
- ":person_bowing:": "\U0001f647",
- ":person_cartwheeling:": "\U0001f938",
- ":person_climbing:": "\U0001f9d7",
- ":person_curly_hair:": "\U0001f9d1\u200d\U0001f9b1",
- ":person_facepalming:": "\U0001f926",
- ":person_feeding_baby:": "\U0001f9d1\u200d\U0001f37c",
- ":person_fencing:": "\U0001f93a",
- ":person_frowning:": "\U0001f64d",
- ":person_gesturing_no:": "\U0001f645",
- ":person_gesturing_ok:": "\U0001f646",
- ":person_getting_haircut:": "\U0001f487",
- ":person_getting_massage:": "\U0001f486",
- ":person_golfing:": "\U0001f3cc\ufe0f",
- ":person_in_bed:": "\U0001f6cc",
- ":person_in_lotus_position:": "\U0001f9d8",
- ":person_in_manual_wheelchair:": "\U0001f9d1\u200d\U0001f9bd",
- ":person_in_motorized_wheelchair:": "\U0001f9d1\u200d\U0001f9bc",
- ":person_in_steamy_room:": "\U0001f9d6",
- ":person_in_suit_levitating:": "\U0001f574\ufe0f",
- ":person_in_tuxedo:": "\U0001f935",
- ":person_juggling:": "\U0001f939",
- ":person_kneeling:": "\U0001f9ce",
- ":person_lifting_weights:": "\U0001f3cb\ufe0f",
- ":person_mountain_biking:": "\U0001f6b5",
- ":person_playing_handball:": "\U0001f93e",
- ":person_playing_water_polo:": "\U0001f93d",
- ":person_pouting:": "\U0001f64e",
- ":person_raising_hand:": "\U0001f64b",
- ":person_red_hair:": "\U0001f9d1\u200d\U0001f9b0",
- ":person_rowing_boat:": "\U0001f6a3",
- ":person_running:": "\U0001f3c3",
- ":person_shrugging:": "\U0001f937",
- ":person_standing:": "\U0001f9cd",
- ":person_surfing:": "\U0001f3c4",
- ":person_swimming:": "\U0001f3ca",
- ":person_taking_bath:": "\U0001f6c0",
- ":person_tipping_hand:": "\U0001f481",
- ":person_walking:": "\U0001f6b6",
- ":person_wearing_turban:": "\U0001f473",
- ":person_white_hair:": "\U0001f9d1\u200d\U0001f9b3",
- ":person_with_blond_hair:": "\U0001f471",
- ":person_with_curly_hair:": "\U0001f9d1\u200d\U0001f9b1",
- ":person_with_probing_cane:": "\U0001f9d1\u200d\U0001f9af",
- ":person_with_red_hair:": "\U0001f9d1\u200d\U0001f9b0",
- ":person_with_skullcap:": "\U0001f472",
- ":person_with_turban:": "\U0001f473",
- ":person_with_veil:": "\U0001f470",
- ":person_with_white_cane:": "\U0001f9d1\u200d\U0001f9af",
- ":person_with_white_hair:": "\U0001f9d1\u200d\U0001f9b3",
- ":peru:": "\U0001f1f5\U0001f1ea",
- ":petri_dish:": "\U0001f9eb",
- ":philippines:": "\U0001f1f5\U0001f1ed",
- ":phone:": "\u260e\ufe0f",
- ":pi_ata:": "\U0001fa85",
- ":pick:": "\u26cf\ufe0f",
- ":pickup_truck:": "\U0001f6fb",
- ":pie:": "\U0001f967",
- ":pig2:": "\U0001f416",
- ":pig:": "\U0001f437",
- ":pig_face:": "\U0001f437",
- ":pig_nose:": "\U0001f43d",
- ":pile_of_poo:": "\U0001f4a9",
- ":pill:": "\U0001f48a",
- ":pilot:": "\U0001f9d1\u200d\u2708\ufe0f",
- ":pinata:": "\U0001fa85",
- ":pinched_fingers:": "\U0001f90c",
- ":pinching_hand:": "\U0001f90f",
- ":pine_decoration:": "\U0001f38d",
- ":pineapple:": "\U0001f34d",
- ":ping_pong:": "\U0001f3d3",
- ":pirate_flag:": "\U0001f3f4\u200d\u2620\ufe0f",
- ":pisces:": "\u2653",
- ":pistol:": "\U0001f52b",
- ":pitcairn_islands:": "\U0001f1f5\U0001f1f3",
- ":pizza:": "\U0001f355",
- ":placard:": "\U0001faa7",
- ":place_of_worship:": "\U0001f6d0",
- ":plate_with_cutlery:": "\U0001f37d\ufe0f",
- ":play_button:": "\u25b6\ufe0f",
- ":play_or_pause_button:": "\u23ef\ufe0f",
- ":pleading_face:": "\U0001f97a",
- ":plunger:": "\U0001faa0",
- ":plus:": "\u2795",
- ":point_down:": "\U0001f447",
- ":point_left:": "\U0001f448",
- ":point_right:": "\U0001f449",
- ":point_up:": "\u261d\ufe0f",
- ":point_up_2:": "\U0001f446",
- ":poland:": "\U0001f1f5\U0001f1f1",
- ":polar_bear:": "\U0001f43b\u200d\u2744\ufe0f",
- ":police_car:": "\U0001f693",
- ":police_car_light:": "\U0001f6a8",
- ":police_officer:": "\U0001f46e",
- ":policeman:": "\U0001f46e\u200d\u2642\ufe0f",
- ":policewoman:": "\U0001f46e\u200d\u2640\ufe0f",
- ":poodle:": "\U0001f429",
- ":pool_8_ball:": "\U0001f3b1",
- ":poop:": "\U0001f4a9",
- ":popcorn:": "\U0001f37f",
- ":portugal:": "\U0001f1f5\U0001f1f9",
- ":post_office:": "\U0001f3e3",
- ":postal_horn:": "\U0001f4ef",
- ":postbox:": "\U0001f4ee",
- ":pot_of_food:": "\U0001f372",
- ":potable_water:": "\U0001f6b0",
- ":potato:": "\U0001f954",
- ":potted_plant:": "\U0001fab4",
- ":pouch:": "\U0001f45d",
- ":poultry_leg:": "\U0001f357",
- ":pound:": "\U0001f4b7",
- ":pound_banknote:": "\U0001f4b7",
- ":pout:": "\U0001f621",
- ":pouting_cat:": "\U0001f63e",
- ":pouting_face:": "\U0001f64e",
- ":pouting_man:": "\U0001f64e\u200d\u2642\ufe0f",
- ":pouting_woman:": "\U0001f64e\u200d\u2640\ufe0f",
- ":pray:": "\U0001f64f",
- ":prayer_beads:": "\U0001f4ff",
- ":pregnant_woman:": "\U0001f930",
- ":pretzel:": "\U0001f968",
- ":previous_track_button:": "\u23ee\ufe0f",
- ":prince:": "\U0001f934",
- ":princess:": "\U0001f478",
- ":printer:": "\U0001f5a8\ufe0f",
- ":probing_cane:": "\U0001f9af",
- ":prohibited:": "\U0001f6ab",
- ":puerto_rico:": "\U0001f1f5\U0001f1f7",
- ":punch:": "\U0001f44a",
- ":purple_circle:": "\U0001f7e3",
- ":purple_heart:": "\U0001f49c",
- ":purple_square:": "\U0001f7ea",
- ":purse:": "\U0001f45b",
- ":pushpin:": "\U0001f4cc",
- ":put_litter_in_its_place:": "\U0001f6ae",
- ":puzzle_piece:": "\U0001f9e9",
- ":qatar:": "\U0001f1f6\U0001f1e6",
- ":question:": "\u2753",
- ":question_mark:": "\u2753",
- ":rabbit2:": "\U0001f407",
- ":rabbit:": "\U0001f430",
- ":rabbit_face:": "\U0001f430",
- ":raccoon:": "\U0001f99d",
- ":racehorse:": "\U0001f40e",
- ":racing_car:": "\U0001f3ce\ufe0f",
- ":radio:": "\U0001f4fb",
- ":radio_button:": "\U0001f518",
- ":radioactive:": "\u2622\ufe0f",
- ":rage:": "\U0001f621",
- ":railway_car:": "\U0001f683",
- ":railway_track:": "\U0001f6e4\ufe0f",
- ":rainbow:": "\U0001f308",
- ":rainbow_flag:": "\U0001f3f3\ufe0f\u200d\U0001f308",
- ":raised_back_of_hand:": "\U0001f91a",
- ":raised_eyebrow:": "\U0001f928",
- ":raised_fist:": "\u270a",
- ":raised_hand:": "\u270b",
- ":raised_hand_with_fingers_splayed:": "\U0001f590\ufe0f",
- ":raised_hands:": "\U0001f64c",
- ":raising_hand:": "\U0001f64b",
- ":raising_hand_man:": "\U0001f64b\u200d\u2642\ufe0f",
- ":raising_hand_woman:": "\U0001f64b\u200d\u2640\ufe0f",
- ":raising_hands:": "\U0001f64c",
- ":ram:": "\U0001f40f",
- ":ramen:": "\U0001f35c",
- ":rat:": "\U0001f400",
- ":razor:": "\U0001fa92",
- ":receipt:": "\U0001f9fe",
- ":record_button:": "\u23fa\ufe0f",
- ":recycle:": "\u267b\ufe0f",
- ":recycling_symbol:": "\u267b\ufe0f",
- ":red_apple:": "\U0001f34e",
- ":red_car:": "\U0001f697",
- ":red_circle:": "\U0001f534",
- ":red_envelope:": "\U0001f9e7",
- ":red_hair:": "\U0001f9b0",
- ":red_haired_man:": "\U0001f468\u200d\U0001f9b0",
- ":red_haired_woman:": "\U0001f469\u200d\U0001f9b0",
- ":red_heart:": "\u2764\ufe0f",
- ":red_paper_lantern:": "\U0001f3ee",
- ":red_square:": "\U0001f7e5",
- ":red_triangle_pointed_down:": "\U0001f53b",
- ":red_triangle_pointed_up:": "\U0001f53a",
- ":registered:": "\u00ae\ufe0f",
- ":relaxed:": "\u263a\ufe0f",
- ":relieved:": "\U0001f60c",
- ":relieved_face:": "\U0001f60c",
- ":reminder_ribbon:": "\U0001f397\ufe0f",
- ":repeat:": "\U0001f501",
- ":repeat_button:": "\U0001f501",
- ":repeat_one:": "\U0001f502",
- ":repeat_single_button:": "\U0001f502",
- ":rescue_worker_helmet:": "\u26d1\ufe0f",
- ":rescue_worker_s_helmet:": "\u26d1\ufe0f",
- ":restroom:": "\U0001f6bb",
- ":reunion:": "\U0001f1f7\U0001f1ea",
- ":reverse_button:": "\u25c0\ufe0f",
- ":revolving_hearts:": "\U0001f49e",
- ":rewind:": "\u23ea",
- ":rhinoceros:": "\U0001f98f",
- ":ribbon:": "\U0001f380",
- ":rice:": "\U0001f35a",
- ":rice_ball:": "\U0001f359",
- ":rice_cracker:": "\U0001f358",
- ":rice_scene:": "\U0001f391",
- ":right_anger_bubble:": "\U0001f5ef\ufe0f",
- ":right_arrow:": "\u27a1\ufe0f",
- ":right_arrow_curving_down:": "\u2935\ufe0f",
- ":right_arrow_curving_left:": "\u21a9\ufe0f",
- ":right_arrow_curving_up:": "\u2934\ufe0f",
- ":right_facing_fist:": "\U0001f91c",
- ":ring:": "\U0001f48d",
- ":ringed_planet:": "\U0001fa90",
- ":roasted_sweet_potato:": "\U0001f360",
- ":robot:": "\U0001f916",
- ":robot_face:": "\U0001f916",
- ":rock:": "\U0001faa8",
- ":rocket:": "\U0001f680",
- ":rofl:": "\U0001f923",
- ":roll_eyes:": "\U0001f644",
- ":roll_of_paper:": "\U0001f9fb",
- ":rolled_up_newspaper:": "\U0001f5de\ufe0f",
- ":roller_coaster:": "\U0001f3a2",
- ":roller_skate:": "\U0001f6fc",
- ":rolling_on_the_floor_laughing:": "\U0001f923",
- ":romania:": "\U0001f1f7\U0001f1f4",
- ":rooster:": "\U0001f413",
- ":rose:": "\U0001f339",
- ":rosette:": "\U0001f3f5\ufe0f",
- ":rotating_light:": "\U0001f6a8",
- ":round_pushpin:": "\U0001f4cd",
- ":rowboat:": "\U0001f6a3",
- ":rowing_man:": "\U0001f6a3\u200d\u2642\ufe0f",
- ":rowing_woman:": "\U0001f6a3\u200d\u2640\ufe0f",
- ":ru:": "\U0001f1f7\U0001f1fa",
- ":rugby_football:": "\U0001f3c9",
- ":runner:": "\U0001f3c3",
- ":running:": "\U0001f3c3",
- ":running_man:": "\U0001f3c3\u200d\u2642\ufe0f",
- ":running_shirt:": "\U0001f3bd",
- ":running_shirt_with_sash:": "\U0001f3bd",
- ":running_shoe:": "\U0001f45f",
- ":running_woman:": "\U0001f3c3\u200d\u2640\ufe0f",
- ":rwanda:": "\U0001f1f7\U0001f1fc",
- ":sa:": "\U0001f202\ufe0f",
- ":sad_but_relieved_face:": "\U0001f625",
- ":safety_pin:": "\U0001f9f7",
- ":safety_vest:": "\U0001f9ba",
- ":sagittarius:": "\u2650",
- ":sailboat:": "\u26f5",
- ":sake:": "\U0001f376",
- ":salt:": "\U0001f9c2",
- ":samoa:": "\U0001f1fc\U0001f1f8",
- ":san_marino:": "\U0001f1f8\U0001f1f2",
- ":sandal:": "\U0001f461",
- ":sandwich:": "\U0001f96a",
- ":santa:": "\U0001f385",
- ":santa_claus:": "\U0001f385",
- ":sao_tome_principe:": "\U0001f1f8\U0001f1f9",
- ":sari:": "\U0001f97b",
- ":sassy_man:": "\U0001f481\u200d\u2642\ufe0f",
- ":sassy_woman:": "\U0001f481\u200d\u2640\ufe0f",
- ":satellite:": "\U0001f4e1",
- ":satellite_antenna:": "\U0001f4e1",
- ":satisfied:": "\U0001f606",
- ":saudi_arabia:": "\U0001f1f8\U0001f1e6",
- ":sauna_man:": "\U0001f9d6\u200d\u2642\ufe0f",
- ":sauna_person:": "\U0001f9d6",
- ":sauna_woman:": "\U0001f9d6\u200d\u2640\ufe0f",
- ":sauropod:": "\U0001f995",
- ":saxophone:": "\U0001f3b7",
- ":scarf:": "\U0001f9e3",
- ":school:": "\U0001f3eb",
- ":school_satchel:": "\U0001f392",
- ":scientist:": "\U0001f9d1\u200d\U0001f52c",
- ":scissors:": "\u2702\ufe0f",
- ":scorpio:": "\u264f",
- ":scorpion:": "\U0001f982",
- ":scorpius:": "\u264f",
- ":scotland:": "\U0001f3f4\U000e0067\U000e0062\U000e0073\U000e0063\U000e0074\U000e007f",
- ":scream:": "\U0001f631",
- ":scream_cat:": "\U0001f640",
- ":screwdriver:": "\U0001fa9b",
- ":scroll:": "\U0001f4dc",
- ":seal:": "\U0001f9ad",
- ":seat:": "\U0001f4ba",
- ":second_place_medal:": "\U0001f948",
- ":secret:": "\u3299\ufe0f",
- ":see_no_evil:": "\U0001f648",
- ":see_no_evil_monkey:": "\U0001f648",
- ":seedling:": "\U0001f331",
- ":selfie:": "\U0001f933",
- ":senegal:": "\U0001f1f8\U0001f1f3",
- ":serbia:": "\U0001f1f7\U0001f1f8",
- ":service_dog:": "\U0001f415\u200d\U0001f9ba",
- ":seven:": "7\ufe0f\u20e3",
- ":seven_o_clock:": "\U0001f556",
- ":seven_thirty:": "\U0001f562",
- ":sewing_needle:": "\U0001faa1",
- ":seychelles:": "\U0001f1f8\U0001f1e8",
- ":shallow_pan_of_food:": "\U0001f958",
- ":shamrock:": "\u2618\ufe0f",
- ":shark:": "\U0001f988",
- ":shaved_ice:": "\U0001f367",
- ":sheaf_of_rice:": "\U0001f33e",
- ":sheep:": "\U0001f411",
- ":shell:": "\U0001f41a",
- ":shield:": "\U0001f6e1\ufe0f",
- ":shinto_shrine:": "\u26e9\ufe0f",
- ":ship:": "\U0001f6a2",
- ":shirt:": "\U0001f455",
- ":shit:": "\U0001f4a9",
- ":shoe:": "\U0001f45e",
- ":shooting_star:": "\U0001f320",
- ":shopping:": "\U0001f6cd\ufe0f",
- ":shopping_bags:": "\U0001f6cd\ufe0f",
- ":shopping_cart:": "\U0001f6d2",
- ":shortcake:": "\U0001f370",
- ":shorts:": "\U0001fa73",
- ":shower:": "\U0001f6bf",
- ":shrimp:": "\U0001f990",
- ":shrug:": "\U0001f937",
- ":shuffle_tracks_button:": "\U0001f500",
- ":shushing_face:": "\U0001f92b",
- ":sierra_leone:": "\U0001f1f8\U0001f1f1",
- ":sign_of_the_horns:": "\U0001f918",
- ":signal_strength:": "\U0001f4f6",
- ":singapore:": "\U0001f1f8\U0001f1ec",
- ":singer:": "\U0001f9d1\u200d\U0001f3a4",
- ":sint_maarten:": "\U0001f1f8\U0001f1fd",
- ":six:": "6\ufe0f\u20e3",
- ":six_o_clock:": "\U0001f555",
- ":six_pointed_star:": "\U0001f52f",
- ":six_thirty:": "\U0001f561",
- ":skateboard:": "\U0001f6f9",
- ":ski:": "\U0001f3bf",
- ":skier:": "\u26f7\ufe0f",
- ":skis:": "\U0001f3bf",
- ":skull:": "\U0001f480",
- ":skull_and_crossbones:": "\u2620\ufe0f",
- ":skunk:": "\U0001f9a8",
- ":sled:": "\U0001f6f7",
- ":sleeping:": "\U0001f634",
- ":sleeping_bed:": "\U0001f6cc",
- ":sleeping_face:": "\U0001f634",
- ":sleepy:": "\U0001f62a",
- ":sleepy_face:": "\U0001f62a",
- ":slightly_frowning_face:": "\U0001f641",
- ":slightly_smiling_face:": "\U0001f642",
- ":slot_machine:": "\U0001f3b0",
- ":sloth:": "\U0001f9a5",
- ":slovakia:": "\U0001f1f8\U0001f1f0",
- ":slovenia:": "\U0001f1f8\U0001f1ee",
- ":small_airplane:": "\U0001f6e9\ufe0f",
- ":small_blue_diamond:": "\U0001f539",
- ":small_orange_diamond:": "\U0001f538",
- ":small_red_triangle:": "\U0001f53a",
- ":small_red_triangle_down:": "\U0001f53b",
- ":smile:": "\U0001f604",
- ":smile_cat:": "\U0001f638",
- ":smiley:": "\U0001f603",
- ":smiley_cat:": "\U0001f63a",
- ":smiling_cat_with_heart_eyes:": "\U0001f63b",
- ":smiling_face:": "\u263a\ufe0f",
- ":smiling_face_with_halo:": "\U0001f607",
- ":smiling_face_with_heart_eyes:": "\U0001f60d",
- ":smiling_face_with_hearts:": "\U0001f970",
- ":smiling_face_with_horns:": "\U0001f608",
- ":smiling_face_with_smiling_eyes:": "\U0001f60a",
- ":smiling_face_with_sunglasses:": "\U0001f60e",
- ":smiling_face_with_tear:": "\U0001f972",
- ":smiling_face_with_three_hearts:": "\U0001f970",
- ":smiling_imp:": "\U0001f608",
- ":smirk:": "\U0001f60f",
- ":smirk_cat:": "\U0001f63c",
- ":smirking_face:": "\U0001f60f",
- ":smoking:": "\U0001f6ac",
- ":snail:": "\U0001f40c",
- ":snake:": "\U0001f40d",
- ":sneezing_face:": "\U0001f927",
- ":snow_capped_mountain:": "\U0001f3d4\ufe0f",
- ":snowboarder:": "\U0001f3c2",
- ":snowflake:": "\u2744\ufe0f",
- ":snowman:": "\u26c4",
- ":snowman_with_snow:": "\u2603\ufe0f",
- ":snowman_without_snow:": "\u26c4",
- ":soap:": "\U0001f9fc",
- ":sob:": "\U0001f62d",
- ":soccer:": "\u26bd",
- ":soccer_ball:": "\u26bd",
- ":socks:": "\U0001f9e6",
- ":soft_ice_cream:": "\U0001f366",
- ":softball:": "\U0001f94e",
- ":solomon_islands:": "\U0001f1f8\U0001f1e7",
- ":somalia:": "\U0001f1f8\U0001f1f4",
- ":soon:": "\U0001f51c",
- ":soon_arrow:": "\U0001f51c",
- ":sos:": "\U0001f198",
- ":sos_button:": "\U0001f198",
- ":sound:": "\U0001f509",
- ":south_africa:": "\U0001f1ff\U0001f1e6",
- ":south_georgia_south_sandwich_islands:": "\U0001f1ec\U0001f1f8",
- ":south_sudan:": "\U0001f1f8\U0001f1f8",
- ":space_invader:": "\U0001f47e",
- ":spade_suit:": "\u2660\ufe0f",
- ":spades:": "\u2660\ufe0f",
- ":spaghetti:": "\U0001f35d",
- ":sparkle:": "\u2747\ufe0f",
- ":sparkler:": "\U0001f387",
- ":sparkles:": "\u2728",
- ":sparkling_heart:": "\U0001f496",
- ":speak_no_evil:": "\U0001f64a",
- ":speak_no_evil_monkey:": "\U0001f64a",
- ":speaker:": "\U0001f508",
- ":speaker_high_volume:": "\U0001f50a",
- ":speaker_low_volume:": "\U0001f508",
- ":speaker_medium_volume:": "\U0001f509",
- ":speaking_head:": "\U0001f5e3\ufe0f",
- ":speech_balloon:": "\U0001f4ac",
- ":speedboat:": "\U0001f6a4",
- ":spider:": "\U0001f577\ufe0f",
- ":spider_web:": "\U0001f578\ufe0f",
- ":spiral_calendar:": "\U0001f5d3\ufe0f",
- ":spiral_notepad:": "\U0001f5d2\ufe0f",
- ":spiral_shell:": "\U0001f41a",
- ":sponge:": "\U0001f9fd",
- ":spoon:": "\U0001f944",
- ":sport_utility_vehicle:": "\U0001f699",
- ":sports_medal:": "\U0001f3c5",
- ":spouting_whale:": "\U0001f433",
- ":squid:": "\U0001f991",
- ":squinting_face_with_tongue:": "\U0001f61d",
- ":sri_lanka:": "\U0001f1f1\U0001f1f0",
- ":st_barthelemy:": "\U0001f1e7\U0001f1f1",
- ":st_helena:": "\U0001f1f8\U0001f1ed",
- ":st_kitts_nevis:": "\U0001f1f0\U0001f1f3",
- ":st_lucia:": "\U0001f1f1\U0001f1e8",
- ":st_martin:": "\U0001f1f2\U0001f1eb",
- ":st_pierre_miquelon:": "\U0001f1f5\U0001f1f2",
- ":st_vincent_grenadines:": "\U0001f1fb\U0001f1e8",
- ":stadium:": "\U0001f3df\ufe0f",
- ":standing_man:": "\U0001f9cd\u200d\u2642\ufe0f",
- ":standing_person:": "\U0001f9cd",
- ":standing_woman:": "\U0001f9cd\u200d\u2640\ufe0f",
- ":star2:": "\U0001f31f",
- ":star:": "\u2b50",
- ":star_and_crescent:": "\u262a\ufe0f",
- ":star_of_david:": "\u2721\ufe0f",
- ":star_struck:": "\U0001f929",
- ":stars:": "\U0001f320",
- ":station:": "\U0001f689",
- ":statue_of_liberty:": "\U0001f5fd",
- ":steam_locomotive:": "\U0001f682",
- ":steaming_bowl:": "\U0001f35c",
- ":stethoscope:": "\U0001fa7a",
- ":stew:": "\U0001f372",
- ":stop_button:": "\u23f9\ufe0f",
- ":stop_sign:": "\U0001f6d1",
- ":stopwatch:": "\u23f1\ufe0f",
- ":straight_ruler:": "\U0001f4cf",
- ":strawberry:": "\U0001f353",
- ":stuck_out_tongue:": "\U0001f61b",
- ":stuck_out_tongue_closed_eyes:": "\U0001f61d",
- ":stuck_out_tongue_winking_eye:": "\U0001f61c",
- ":student:": "\U0001f9d1\u200d\U0001f393",
- ":studio_microphone:": "\U0001f399\ufe0f",
- ":stuffed_flatbread:": "\U0001f959",
- ":sudan:": "\U0001f1f8\U0001f1e9",
- ":sun:": "\u2600\ufe0f",
- ":sun_behind_cloud:": "\u26c5",
- ":sun_behind_large_cloud:": "\U0001f325\ufe0f",
- ":sun_behind_rain_cloud:": "\U0001f326\ufe0f",
- ":sun_behind_small_cloud:": "\U0001f324\ufe0f",
- ":sun_with_face:": "\U0001f31e",
- ":sunflower:": "\U0001f33b",
- ":sunglasses:": "\U0001f60e",
- ":sunny:": "\u2600\ufe0f",
- ":sunrise:": "\U0001f305",
- ":sunrise_over_mountains:": "\U0001f304",
- ":sunset:": "\U0001f307",
- ":superhero:": "\U0001f9b8",
- ":superhero_man:": "\U0001f9b8\u200d\u2642\ufe0f",
- ":superhero_woman:": "\U0001f9b8\u200d\u2640\ufe0f",
- ":supervillain:": "\U0001f9b9",
- ":supervillain_man:": "\U0001f9b9\u200d\u2642\ufe0f",
- ":supervillain_woman:": "\U0001f9b9\u200d\u2640\ufe0f",
- ":surfer:": "\U0001f3c4",
- ":surfing_man:": "\U0001f3c4\u200d\u2642\ufe0f",
- ":surfing_woman:": "\U0001f3c4\u200d\u2640\ufe0f",
- ":suriname:": "\U0001f1f8\U0001f1f7",
- ":sushi:": "\U0001f363",
- ":suspension_railway:": "\U0001f69f",
- ":svalbard_jan_mayen:": "\U0001f1f8\U0001f1ef",
- ":swan:": "\U0001f9a2",
- ":swaziland:": "\U0001f1f8\U0001f1ff",
- ":sweat:": "\U0001f613",
- ":sweat_droplets:": "\U0001f4a6",
- ":sweat_drops:": "\U0001f4a6",
- ":sweat_smile:": "\U0001f605",
- ":sweden:": "\U0001f1f8\U0001f1ea",
- ":sweet_potato:": "\U0001f360",
- ":swim_brief:": "\U0001fa72",
- ":swimmer:": "\U0001f3ca",
- ":swimming_man:": "\U0001f3ca\u200d\u2642\ufe0f",
- ":swimming_woman:": "\U0001f3ca\u200d\u2640\ufe0f",
- ":switzerland:": "\U0001f1e8\U0001f1ed",
- ":symbols:": "\U0001f523",
- ":synagogue:": "\U0001f54d",
- ":syria:": "\U0001f1f8\U0001f1fe",
- ":syringe:": "\U0001f489",
- ":t-rex:": "\U0001f996",
- ":t_rex:": "\U0001f996",
- ":t_shirt:": "\U0001f455",
- ":taco:": "\U0001f32e",
- ":tada:": "\U0001f389",
- ":taiwan:": "\U0001f1f9\U0001f1fc",
- ":tajikistan:": "\U0001f1f9\U0001f1ef",
- ":takeout_box:": "\U0001f961",
- ":tamale:": "\U0001fad4",
- ":tanabata_tree:": "\U0001f38b",
- ":tangerine:": "\U0001f34a",
- ":tanzania:": "\U0001f1f9\U0001f1ff",
- ":taurus:": "\u2649",
- ":taxi:": "\U0001f695",
- ":tea:": "\U0001f375",
- ":teacher:": "\U0001f9d1\u200d\U0001f3eb",
- ":teacup_without_handle:": "\U0001f375",
- ":teapot:": "\U0001fad6",
- ":tear_off_calendar:": "\U0001f4c6",
- ":technologist:": "\U0001f9d1\u200d\U0001f4bb",
- ":teddy_bear:": "\U0001f9f8",
- ":telephone:": "\u260e\ufe0f",
- ":telephone_receiver:": "\U0001f4de",
- ":telescope:": "\U0001f52d",
- ":television:": "\U0001f4fa",
- ":ten_o_clock:": "\U0001f559",
- ":ten_thirty:": "\U0001f565",
- ":tennis:": "\U0001f3be",
- ":tent:": "\u26fa",
- ":test_tube:": "\U0001f9ea",
- ":thailand:": "\U0001f1f9\U0001f1ed",
- ":thermometer:": "\U0001f321\ufe0f",
- ":thinking:": "\U0001f914",
- ":thinking_face:": "\U0001f914",
- ":third_place_medal:": "\U0001f949",
- ":thong_sandal:": "\U0001fa74",
- ":thought_balloon:": "\U0001f4ad",
- ":thread:": "\U0001f9f5",
- ":three:": "3\ufe0f\u20e3",
- ":three_o_clock:": "\U0001f552",
- ":three_thirty:": "\U0001f55e",
- ":thumbs_down:": "\U0001f44e",
- ":thumbs_up:": "\U0001f44d",
- ":thumbsdown:": "\U0001f44e",
- ":thumbsup:": "\U0001f44d",
- ":ticket:": "\U0001f3ab",
- ":tickets:": "\U0001f39f\ufe0f",
- ":tiger2:": "\U0001f405",
- ":tiger:": "\U0001f42f",
- ":tiger_face:": "\U0001f42f",
- ":timer_clock:": "\u23f2\ufe0f",
- ":timor_leste:": "\U0001f1f9\U0001f1f1",
- ":tipping_hand_man:": "\U0001f481\u200d\u2642\ufe0f",
- ":tipping_hand_person:": "\U0001f481",
- ":tipping_hand_woman:": "\U0001f481\u200d\u2640\ufe0f",
- ":tired_face:": "\U0001f62b",
- ":tm:": "\u2122\ufe0f",
- ":togo:": "\U0001f1f9\U0001f1ec",
- ":toilet:": "\U0001f6bd",
- ":tokelau:": "\U0001f1f9\U0001f1f0",
- ":tokyo_tower:": "\U0001f5fc",
- ":tomato:": "\U0001f345",
- ":tonga:": "\U0001f1f9\U0001f1f4",
- ":tongue:": "\U0001f445",
- ":toolbox:": "\U0001f9f0",
- ":tooth:": "\U0001f9b7",
- ":toothbrush:": "\U0001faa5",
- ":top:": "\U0001f51d",
- ":top_arrow:": "\U0001f51d",
- ":top_hat:": "\U0001f3a9",
- ":tophat:": "\U0001f3a9",
- ":tornado:": "\U0001f32a\ufe0f",
- ":tr:": "\U0001f1f9\U0001f1f7",
- ":trackball:": "\U0001f5b2\ufe0f",
- ":tractor:": "\U0001f69c",
- ":trade_mark:": "\u2122\ufe0f",
- ":traffic_light:": "\U0001f6a5",
- ":train2:": "\U0001f686",
- ":train:": "\U0001f68b",
- ":tram:": "\U0001f68a",
- ":tram_car:": "\U0001f68b",
- ":transgender_flag:": "\U0001f3f3\ufe0f\u200d\u26a7\ufe0f",
- ":transgender_symbol:": "\u26a7\ufe0f",
- ":triangular_flag:": "\U0001f6a9",
- ":triangular_flag_on_post:": "\U0001f6a9",
- ":triangular_ruler:": "\U0001f4d0",
- ":trident:": "\U0001f531",
- ":trident_emblem:": "\U0001f531",
- ":trinidad_tobago:": "\U0001f1f9\U0001f1f9",
- ":tristan_da_cunha:": "\U0001f1f9\U0001f1e6",
- ":triumph:": "\U0001f624",
- ":trolleybus:": "\U0001f68e",
- ":trophy:": "\U0001f3c6",
- ":tropical_drink:": "\U0001f379",
- ":tropical_fish:": "\U0001f420",
- ":truck:": "\U0001f69a",
- ":trumpet:": "\U0001f3ba",
- ":tshirt:": "\U0001f455",
- ":tulip:": "\U0001f337",
- ":tumbler_glass:": "\U0001f943",
- ":tunisia:": "\U0001f1f9\U0001f1f3",
- ":turkey:": "\U0001f983",
- ":turkmenistan:": "\U0001f1f9\U0001f1f2",
- ":turks_caicos_islands:": "\U0001f1f9\U0001f1e8",
- ":turtle:": "\U0001f422",
- ":tuvalu:": "\U0001f1f9\U0001f1fb",
- ":tv:": "\U0001f4fa",
- ":twelve_o_clock:": "\U0001f55b",
- ":twelve_thirty:": "\U0001f567",
- ":twisted_rightwards_arrows:": "\U0001f500",
- ":two:": "2\ufe0f\u20e3",
- ":two_hearts:": "\U0001f495",
- ":two_hump_camel:": "\U0001f42b",
- ":two_men_holding_hands:": "\U0001f46c",
- ":two_o_clock:": "\U0001f551",
- ":two_thirty:": "\U0001f55d",
- ":two_women_holding_hands:": "\U0001f46d",
- ":u5272:": "\U0001f239",
- ":u5408:": "\U0001f234",
- ":u55b6:": "\U0001f23a",
- ":u6307:": "\U0001f22f",
- ":u6708:": "\U0001f237\ufe0f",
- ":u6709:": "\U0001f236",
- ":u6e80:": "\U0001f235",
- ":u7121:": "\U0001f21a",
- ":u7533:": "\U0001f238",
- ":u7981:": "\U0001f232",
- ":u7a7a:": "\U0001f233",
- ":uganda:": "\U0001f1fa\U0001f1ec",
- ":uk:": "\U0001f1ec\U0001f1e7",
- ":ukraine:": "\U0001f1fa\U0001f1e6",
- ":umbrella:": "\u2614",
- ":umbrella_on_ground:": "\u26f1\ufe0f",
- ":umbrella_with_rain_drops:": "\u2614",
- ":unamused:": "\U0001f612",
- ":unamused_face:": "\U0001f612",
- ":underage:": "\U0001f51e",
- ":unicorn:": "\U0001f984",
- ":united_arab_emirates:": "\U0001f1e6\U0001f1ea",
- ":united_nations:": "\U0001f1fa\U0001f1f3",
- ":unlock:": "\U0001f513",
- ":unlocked:": "\U0001f513",
- ":up:": "\U0001f199",
- ":up_arrow:": "\u2b06\ufe0f",
- ":up_button:": "\U0001f199",
- ":up_down_arrow:": "\u2195\ufe0f",
- ":up_left_arrow:": "\u2196\ufe0f",
- ":up_right_arrow:": "\u2197\ufe0f",
- ":upside_down_face:": "\U0001f643",
- ":upwards_button:": "\U0001f53c",
- ":uruguay:": "\U0001f1fa\U0001f1fe",
- ":us:": "\U0001f1fa\U0001f1f8",
- ":us_outlying_islands:": "\U0001f1fa\U0001f1f2",
- ":us_virgin_islands:": "\U0001f1fb\U0001f1ee",
- ":uzbekistan:": "\U0001f1fa\U0001f1ff",
- ":v:": "\u270c\ufe0f",
- ":vampire:": "\U0001f9db",
- ":vampire_man:": "\U0001f9db\u200d\u2642\ufe0f",
- ":vampire_woman:": "\U0001f9db\u200d\u2640\ufe0f",
- ":vanuatu:": "\U0001f1fb\U0001f1fa",
- ":vatican_city:": "\U0001f1fb\U0001f1e6",
- ":venezuela:": "\U0001f1fb\U0001f1ea",
- ":vertical_traffic_light:": "\U0001f6a6",
- ":vhs:": "\U0001f4fc",
- ":vibration_mode:": "\U0001f4f3",
- ":victory_hand:": "\u270c\ufe0f",
- ":video_camera:": "\U0001f4f9",
- ":video_game:": "\U0001f3ae",
- ":videocassette:": "\U0001f4fc",
- ":vietnam:": "\U0001f1fb\U0001f1f3",
- ":violin:": "\U0001f3bb",
- ":virgo:": "\u264d",
- ":volcano:": "\U0001f30b",
- ":volleyball:": "\U0001f3d0",
- ":vomiting_face:": "\U0001f92e",
- ":vs:": "\U0001f19a",
- ":vs_button:": "\U0001f19a",
- ":vulcan_salute:": "\U0001f596",
- ":waffle:": "\U0001f9c7",
- ":wales:": "\U0001f3f4\U000e0067\U000e0062\U000e0077\U000e006c\U000e0073\U000e007f",
- ":walking:": "\U0001f6b6",
- ":walking_man:": "\U0001f6b6\u200d\u2642\ufe0f",
- ":walking_woman:": "\U0001f6b6\u200d\u2640\ufe0f",
- ":wallis_futuna:": "\U0001f1fc\U0001f1eb",
- ":waning_crescent_moon:": "\U0001f318",
- ":waning_gibbous_moon:": "\U0001f316",
- ":warning:": "\u26a0\ufe0f",
- ":wastebasket:": "\U0001f5d1\ufe0f",
- ":watch:": "\u231a",
- ":water_buffalo:": "\U0001f403",
- ":water_closet:": "\U0001f6be",
- ":water_polo:": "\U0001f93d",
- ":water_wave:": "\U0001f30a",
- ":watermelon:": "\U0001f349",
- ":wave:": "\U0001f44b",
- ":waving_hand:": "\U0001f44b",
- ":wavy_dash:": "\u3030\ufe0f",
- ":waxing_crescent_moon:": "\U0001f312",
- ":waxing_gibbous_moon:": "\U0001f314",
- ":wc:": "\U0001f6be",
- ":weary:": "\U0001f629",
- ":weary_cat:": "\U0001f640",
- ":weary_face:": "\U0001f629",
- ":wedding:": "\U0001f492",
- ":weight_lifting:": "\U0001f3cb\ufe0f",
- ":weight_lifting_man:": "\U0001f3cb\ufe0f\u200d\u2642\ufe0f",
- ":weight_lifting_woman:": "\U0001f3cb\ufe0f\u200d\u2640\ufe0f",
- ":western_sahara:": "\U0001f1ea\U0001f1ed",
- ":whale2:": "\U0001f40b",
- ":whale:": "\U0001f433",
- ":wheel_of_dharma:": "\u2638\ufe0f",
- ":wheelchair:": "\u267f",
- ":wheelchair_symbol:": "\u267f",
- ":white_cane:": "\U0001f9af",
- ":white_check_mark:": "\u2705",
- ":white_circle:": "\u26aa",
- ":white_exclamation_mark:": "\u2755",
- ":white_flag:": "\U0001f3f3\ufe0f",
- ":white_flower:": "\U0001f4ae",
- ":white_hair:": "\U0001f9b3",
- ":white_haired_man:": "\U0001f468\u200d\U0001f9b3",
- ":white_haired_woman:": "\U0001f469\u200d\U0001f9b3",
- ":white_heart:": "\U0001f90d",
- ":white_large_square:": "\u2b1c",
- ":white_medium_small_square:": "\u25fd",
- ":white_medium_square:": "\u25fb\ufe0f",
- ":white_question_mark:": "\u2754",
- ":white_small_square:": "\u25ab\ufe0f",
- ":white_square_button:": "\U0001f533",
- ":wilted_flower:": "\U0001f940",
- ":wind_chime:": "\U0001f390",
- ":wind_face:": "\U0001f32c\ufe0f",
- ":window:": "\U0001fa9f",
- ":wine_glass:": "\U0001f377",
- ":wink:": "\U0001f609",
- ":winking_face:": "\U0001f609",
- ":winking_face_with_tongue:": "\U0001f61c",
- ":wolf:": "\U0001f43a",
- ":woman:": "\U0001f469",
- ":woman_and_man_holding_hands:": "\U0001f46b",
- ":woman_artist:": "\U0001f469\u200d\U0001f3a8",
- ":woman_astronaut:": "\U0001f469\u200d\U0001f680",
- ":woman_bald:": "\U0001f469\u200d\U0001f9b2",
- ":woman_biking:": "\U0001f6b4\u200d\u2640\ufe0f",
- ":woman_bouncing_ball:": "\u26f9\ufe0f\u200d\u2640\ufe0f",
- ":woman_bowing:": "\U0001f647\u200d\u2640\ufe0f",
- ":woman_cartwheeling:": "\U0001f938\u200d\u2640\ufe0f",
- ":woman_climbing:": "\U0001f9d7\u200d\u2640\ufe0f",
- ":woman_construction_worker:": "\U0001f477\u200d\u2640\ufe0f",
- ":woman_cook:": "\U0001f469\u200d\U0001f373",
- ":woman_dancing:": "\U0001f483",
- ":woman_detective:": "\U0001f575\ufe0f\u200d\u2640\ufe0f",
- ":woman_elf:": "\U0001f9dd\u200d\u2640\ufe0f",
- ":woman_facepalming:": "\U0001f926\u200d\u2640\ufe0f",
- ":woman_factory_worker:": "\U0001f469\u200d\U0001f3ed",
- ":woman_fairy:": "\U0001f9da\u200d\u2640\ufe0f",
- ":woman_farmer:": "\U0001f469\u200d\U0001f33e",
- ":woman_feeding_baby:": "\U0001f469\u200d\U0001f37c",
- ":woman_firefighter:": "\U0001f469\u200d\U0001f692",
- ":woman_frowning:": "\U0001f64d\u200d\u2640\ufe0f",
- ":woman_genie:": "\U0001f9de\u200d\u2640\ufe0f",
- ":woman_gesturing_no:": "\U0001f645\u200d\u2640\ufe0f",
- ":woman_gesturing_ok:": "\U0001f646\u200d\u2640\ufe0f",
- ":woman_getting_haircut:": "\U0001f487\u200d\u2640\ufe0f",
- ":woman_getting_massage:": "\U0001f486\u200d\u2640\ufe0f",
- ":woman_golfing:": "\U0001f3cc\ufe0f\u200d\u2640\ufe0f",
- ":woman_guard:": "\U0001f482\u200d\u2640\ufe0f",
- ":woman_health_worker:": "\U0001f469\u200d\u2695\ufe0f",
- ":woman_in_lotus_position:": "\U0001f9d8\u200d\u2640\ufe0f",
- ":woman_in_manual_wheelchair:": "\U0001f469\u200d\U0001f9bd",
- ":woman_in_motorized_wheelchair:": "\U0001f469\u200d\U0001f9bc",
- ":woman_in_steamy_room:": "\U0001f9d6\u200d\u2640\ufe0f",
- ":woman_in_tuxedo:": "\U0001f935\u200d\u2640\ufe0f",
- ":woman_judge:": "\U0001f469\u200d\u2696\ufe0f",
- ":woman_juggling:": "\U0001f939\u200d\u2640\ufe0f",
- ":woman_kneeling:": "\U0001f9ce\u200d\u2640\ufe0f",
- ":woman_lifting_weights:": "\U0001f3cb\ufe0f\u200d\u2640\ufe0f",
- ":woman_mage:": "\U0001f9d9\u200d\u2640\ufe0f",
- ":woman_mechanic:": "\U0001f469\u200d\U0001f527",
- ":woman_mountain_biking:": "\U0001f6b5\u200d\u2640\ufe0f",
- ":woman_office_worker:": "\U0001f469\u200d\U0001f4bc",
- ":woman_pilot:": "\U0001f469\u200d\u2708\ufe0f",
- ":woman_playing_handball:": "\U0001f93e\u200d\u2640\ufe0f",
- ":woman_playing_water_polo:": "\U0001f93d\u200d\u2640\ufe0f",
- ":woman_police_officer:": "\U0001f46e\u200d\u2640\ufe0f",
- ":woman_pouting:": "\U0001f64e\u200d\u2640\ufe0f",
- ":woman_raising_hand:": "\U0001f64b\u200d\u2640\ufe0f",
- ":woman_rowing_boat:": "\U0001f6a3\u200d\u2640\ufe0f",
- ":woman_running:": "\U0001f3c3\u200d\u2640\ufe0f",
- ":woman_s_boot:": "\U0001f462",
- ":woman_s_clothes:": "\U0001f45a",
- ":woman_s_hat:": "\U0001f452",
- ":woman_s_sandal:": "\U0001f461",
- ":woman_scientist:": "\U0001f469\u200d\U0001f52c",
- ":woman_shrugging:": "\U0001f937\u200d\u2640\ufe0f",
- ":woman_singer:": "\U0001f469\u200d\U0001f3a4",
- ":woman_standing:": "\U0001f9cd\u200d\u2640\ufe0f",
- ":woman_student:": "\U0001f469\u200d\U0001f393",
- ":woman_superhero:": "\U0001f9b8\u200d\u2640\ufe0f",
- ":woman_supervillain:": "\U0001f9b9\u200d\u2640\ufe0f",
- ":woman_surfing:": "\U0001f3c4\u200d\u2640\ufe0f",
- ":woman_swimming:": "\U0001f3ca\u200d\u2640\ufe0f",
- ":woman_teacher:": "\U0001f469\u200d\U0001f3eb",
- ":woman_technologist:": "\U0001f469\u200d\U0001f4bb",
- ":woman_tipping_hand:": "\U0001f481\u200d\u2640\ufe0f",
- ":woman_vampire:": "\U0001f9db\u200d\u2640\ufe0f",
- ":woman_walking:": "\U0001f6b6\u200d\u2640\ufe0f",
- ":woman_wearing_turban:": "\U0001f473\u200d\u2640\ufe0f",
- ":woman_with_blond_hair:": "\U0001f471\u200d\u2640\ufe0f",
- ":woman_with_curly_hair:": "\U0001f469\u200d\U0001f9b1",
- ":woman_with_headscarf:": "\U0001f9d5",
- ":woman_with_probing_cane:": "\U0001f469\u200d\U0001f9af",
- ":woman_with_red_hair:": "\U0001f469\u200d\U0001f9b0",
- ":woman_with_turban:": "\U0001f473\u200d\u2640\ufe0f",
- ":woman_with_veil:": "\U0001f470\u200d\u2640\ufe0f",
- ":woman_with_white_cane:": "\U0001f469\u200d\U0001f9af",
- ":woman_with_white_hair:": "\U0001f469\u200d\U0001f9b3",
- ":woman_zombie:": "\U0001f9df\u200d\u2640\ufe0f",
- ":womans_clothes:": "\U0001f45a",
- ":womans_hat:": "\U0001f452",
- ":women_holding_hands:": "\U0001f46d",
- ":women_s_room:": "\U0001f6ba",
- ":women_with_bunny_ears:": "\U0001f46f\u200d\u2640\ufe0f",
- ":women_wrestling:": "\U0001f93c\u200d\u2640\ufe0f",
- ":womens:": "\U0001f6ba",
- ":wood:": "\U0001fab5",
- ":woozy_face:": "\U0001f974",
- ":world_map:": "\U0001f5fa\ufe0f",
- ":worm:": "\U0001fab1",
- ":worried:": "\U0001f61f",
- ":worried_face:": "\U0001f61f",
- ":wrapped_gift:": "\U0001f381",
- ":wrench:": "\U0001f527",
- ":wrestling:": "\U0001f93c",
- ":writing_hand:": "\u270d\ufe0f",
- ":x:": "\u274c",
- ":yarn:": "\U0001f9f6",
- ":yawning_face:": "\U0001f971",
- ":yellow_circle:": "\U0001f7e1",
- ":yellow_heart:": "\U0001f49b",
- ":yellow_square:": "\U0001f7e8",
- ":yemen:": "\U0001f1fe\U0001f1ea",
- ":yen:": "\U0001f4b4",
- ":yen_banknote:": "\U0001f4b4",
- ":yin_yang:": "\u262f\ufe0f",
- ":yo_yo:": "\U0001fa80",
- ":yum:": "\U0001f60b",
- ":zambia:": "\U0001f1ff\U0001f1f2",
- ":zany_face:": "\U0001f92a",
- ":zap:": "\u26a1",
- ":zebra:": "\U0001f993",
- ":zero:": "0\ufe0f\u20e3",
- ":zimbabwe:": "\U0001f1ff\U0001f1fc",
- ":zipper_mouth_face:": "\U0001f910",
- ":zombie:": "\U0001f9df",
- ":zombie_man:": "\U0001f9df\u200d\u2642\ufe0f",
- ":zombie_woman:": "\U0001f9df\u200d\u2640\ufe0f",
- ":zzz:": "\U0001f4a4",
-}
diff --git a/vendor/github.com/enescakir/emoji/parser.go b/vendor/github.com/enescakir/emoji/parser.go
deleted file mode 100644
index 7d37e99..0000000
--- a/vendor/github.com/enescakir/emoji/parser.go
+++ /dev/null
@@ -1,127 +0,0 @@
-package emoji
-
-import (
- "fmt"
- "regexp"
- "strings"
- "unicode"
-)
-
-var (
- flagRegex = regexp.MustCompile(`^:flag-([a-zA-Z]{2}):$`)
-)
-
-// Parse replaces emoji aliases (:pizza:) with unicode representation.
-func Parse(input string) string {
- var matched strings.Builder
- var output strings.Builder
-
- for _, r := range input {
- // when it's not `:`, it might be inner or outer of the emoji alias
- if r != ':' {
- // if matched is empty, it's the outer of the emoji alias
- if matched.Len() == 0 {
- output.WriteRune(r)
- continue
- }
-
- matched.WriteRune(r)
-
- // if it's space, the alias's not valid.
- // reset matched for breaking the emoji alias
- if unicode.IsSpace(r) {
- output.WriteString(matched.String())
- matched.Reset()
- }
- continue
- }
-
- // r is `:` now
- // if matched is empty, it's the beginning of the emoji alias
- if matched.Len() == 0 {
- matched.WriteRune(r)
- continue
- }
-
- // it's the end of the emoji alias
- match := matched.String()
- alias := match + ":"
-
- // check for emoji alias
- if code, ok := Find(alias); ok {
- output.WriteString(code)
- matched.Reset()
- continue
- }
-
- // not found any emoji
- output.WriteString(match)
- // it might be the beginning of the another emoji alias
- matched.Reset()
- matched.WriteRune(r)
-
- }
-
- // if matched not empty, add it to output
- if matched.Len() != 0 {
- output.WriteString(matched.String())
- matched.Reset()
- }
-
- return output.String()
-}
-
-// Map returns the emojis map.
-// Key is the alias of the emoji.
-// Value is the code of the emoji.
-func Map() map[string]string {
- return emojiMap
-}
-
-// AppendAlias adds new emoji pair to the emojis map.
-func AppendAlias(alias, code string) error {
- if c, ok := emojiMap[alias]; ok {
- return fmt.Errorf("emoji already exist: %q => %+q", alias, c)
- }
-
- for _, r := range alias {
- if unicode.IsSpace(r) {
- return fmt.Errorf("emoji alias is not valid: %q", alias)
- }
- }
-
- emojiMap[alias] = code
-
- return nil
-}
-
-// Exist checks existence of the emoji by alias.
-func Exist(alias string) bool {
- _, ok := Find(alias)
-
- return ok
-}
-
-// Find returns the emoji code by alias.
-func Find(alias string) (string, bool) {
- if code, ok := emojiMap[alias]; ok {
- return code, true
- }
-
- if flag := checkFlag(alias); len(flag) > 0 {
- return flag, true
- }
-
- return "", false
-}
-
-// checkFlag finds flag emoji for `flag-[CODE]` pattern
-func checkFlag(alias string) string {
- if matches := flagRegex.FindStringSubmatch(alias); len(matches) == 2 {
- flag, _ := CountryFlag(matches[1])
-
- return flag.String()
- }
-
- return ""
-}
diff --git a/vendor/github.com/go-git/gcfg/LICENSE b/vendor/github.com/go-git/gcfg/LICENSE
deleted file mode 100644
index 87a5ced..0000000
--- a/vendor/github.com/go-git/gcfg/LICENSE
+++ /dev/null
@@ -1,28 +0,0 @@
-Copyright (c) 2012 Péter Surányi. Portions Copyright (c) 2009 The Go
-Authors. All rights reserved.
-
-Redistribution and use in source and binary forms, with or without
-modification, are permitted provided that the following conditions are
-met:
-
- * Redistributions of source code must retain the above copyright
-notice, this list of conditions and the following disclaimer.
- * Redistributions in binary form must reproduce the above
-copyright notice, this list of conditions and the following disclaimer
-in the documentation and/or other materials provided with the
-distribution.
- * Neither the name of Google Inc. nor the names of its
-contributors may be used to endorse or promote products derived from
-this software without specific prior written permission.
-
-THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
-"AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
-LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR
-A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT
-OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
-SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT
-LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
-DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
-THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
-(INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
-OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
diff --git a/vendor/github.com/go-git/gcfg/README b/vendor/github.com/go-git/gcfg/README
deleted file mode 100644
index 1ff233a..0000000
--- a/vendor/github.com/go-git/gcfg/README
+++ /dev/null
@@ -1,4 +0,0 @@
-Gcfg reads INI-style configuration files into Go structs;
-supports user-defined types and subsections.
-
-Package docs: https://godoc.org/gopkg.in/gcfg.v1
diff --git a/vendor/github.com/go-git/gcfg/doc.go b/vendor/github.com/go-git/gcfg/doc.go
deleted file mode 100644
index 7bdefbf..0000000
--- a/vendor/github.com/go-git/gcfg/doc.go
+++ /dev/null
@@ -1,145 +0,0 @@
-// Package gcfg reads "INI-style" text-based configuration files with
-// "name=value" pairs grouped into sections (gcfg files).
-//
-// This package is still a work in progress; see the sections below for planned
-// changes.
-//
-// Syntax
-//
-// The syntax is based on that used by git config:
-// http://git-scm.com/docs/git-config#_syntax .
-// There are some (planned) differences compared to the git config format:
-// - improve data portability:
-// - must be encoded in UTF-8 (for now) and must not contain the 0 byte
-// - include and "path" type is not supported
-// (path type may be implementable as a user-defined type)
-// - internationalization
-// - section and variable names can contain unicode letters, unicode digits
-// (as defined in http://golang.org/ref/spec#Characters ) and hyphens
-// (U+002D), starting with a unicode letter
-// - disallow potentially ambiguous or misleading definitions:
-// - `[sec.sub]` format is not allowed (deprecated in gitconfig)
-// - `[sec ""]` is not allowed
-// - use `[sec]` for section name "sec" and empty subsection name
-// - (planned) within a single file, definitions must be contiguous for each:
-// - section: '[secA]' -> '[secB]' -> '[secA]' is an error
-// - subsection: '[sec "A"]' -> '[sec "B"]' -> '[sec "A"]' is an error
-// - multivalued variable: 'multi=a' -> 'other=x' -> 'multi=b' is an error
-//
-// Data structure
-//
-// The functions in this package read values into a user-defined struct.
-// Each section corresponds to a struct field in the config struct, and each
-// variable in a section corresponds to a data field in the section struct.
-// The mapping of each section or variable name to fields is done either based
-// on the "gcfg" struct tag or by matching the name of the section or variable,
-// ignoring case. In the latter case, hyphens '-' in section and variable names
-// correspond to underscores '_' in field names.
-// Fields must be exported; to use a section or variable name starting with a
-// letter that is neither upper- or lower-case, prefix the field name with 'X'.
-// (See https://code.google.com/p/go/issues/detail?id=5763#c4 .)
-//
-// For sections with subsections, the corresponding field in config must be a
-// map, rather than a struct, with string keys and pointer-to-struct values.
-// Values for subsection variables are stored in the map with the subsection
-// name used as the map key.
-// (Note that unlike section and variable names, subsection names are case
-// sensitive.)
-// When using a map, and there is a section with the same section name but
-// without a subsection name, its values are stored with the empty string used
-// as the key.
-// It is possible to provide default values for subsections in the section
-// "default-" (or by setting values in the corresponding struct
-// field "Default_").
-//
-// The functions in this package panic if config is not a pointer to a struct,
-// or when a field is not of a suitable type (either a struct or a map with
-// string keys and pointer-to-struct values).
-//
-// Parsing of values
-//
-// The section structs in the config struct may contain single-valued or
-// multi-valued variables. Variables of unnamed slice type (that is, a type
-// starting with `[]`) are treated as multi-value; all others (including named
-// slice types) are treated as single-valued variables.
-//
-// Single-valued variables are handled based on the type as follows.
-// Unnamed pointer types (that is, types starting with `*`) are dereferenced,
-// and if necessary, a new instance is allocated.
-//
-// For types implementing the encoding.TextUnmarshaler interface, the
-// UnmarshalText method is used to set the value. Implementing this method is
-// the recommended way for parsing user-defined types.
-//
-// For fields of string kind, the value string is assigned to the field, after
-// unquoting and unescaping as needed.
-// For fields of bool kind, the field is set to true if the value is "true",
-// "yes", "on" or "1", and set to false if the value is "false", "no", "off" or
-// "0", ignoring case. In addition, single-valued bool fields can be specified
-// with a "blank" value (variable name without equals sign and value); in such
-// case the value is set to true.
-//
-// Predefined integer types [u]int(|8|16|32|64) and big.Int are parsed as
-// decimal or hexadecimal (if having '0x' prefix). (This is to prevent
-// unintuitively handling zero-padded numbers as octal.) Other types having
-// [u]int* as the underlying type, such as os.FileMode and uintptr allow
-// decimal, hexadecimal, or octal values.
-// Parsing mode for integer types can be overridden using the struct tag option
-// ",int=mode" where mode is a combination of the 'd', 'h', and 'o' characters
-// (each standing for decimal, hexadecimal, and octal, respectively.)
-//
-// All other types are parsed using fmt.Sscanf with the "%v" verb.
-//
-// For multi-valued variables, each individual value is parsed as above and
-// appended to the slice. If the first value is specified as a "blank" value
-// (variable name without equals sign and value), a new slice is allocated;
-// that is any values previously set in the slice will be ignored.
-//
-// The types subpackage for provides helpers for parsing "enum-like" and integer
-// types.
-//
-// Error handling
-//
-// There are 3 types of errors:
-//
-// - programmer errors / panics:
-// - invalid configuration structure
-// - data errors:
-// - fatal errors:
-// - invalid configuration syntax
-// - warnings:
-// - data that doesn't belong to any part of the config structure
-//
-// Programmer errors trigger panics. These are should be fixed by the programmer
-// before releasing code that uses gcfg.
-//
-// Data errors cause gcfg to return a non-nil error value. This includes the
-// case when there are extra unknown key-value definitions in the configuration
-// data (extra data).
-// However, in some occasions it is desirable to be able to proceed in
-// situations when the only data error is that of extra data.
-// These errors are handled at a different (warning) priority and can be
-// filtered out programmatically. To ignore extra data warnings, wrap the
-// gcfg.Read*Into invocation into a call to gcfg.FatalOnly.
-//
-// TODO
-//
-// The following is a list of changes under consideration:
-// - documentation
-// - self-contained syntax documentation
-// - more practical examples
-// - move TODOs to issue tracker (eventually)
-// - syntax
-// - reconsider valid escape sequences
-// (gitconfig doesn't support \r in value, \t in subsection name, etc.)
-// - reading / parsing gcfg files
-// - define internal representation structure
-// - support multiple inputs (readers, strings, files)
-// - support declaring encoding (?)
-// - support varying fields sets for subsections (?)
-// - writing gcfg files
-// - error handling
-// - make error context accessible programmatically?
-// - limit input size?
-//
-package gcfg // import "github.com/go-git/gcfg"
diff --git a/vendor/github.com/go-git/gcfg/errors.go b/vendor/github.com/go-git/gcfg/errors.go
deleted file mode 100644
index 853c760..0000000
--- a/vendor/github.com/go-git/gcfg/errors.go
+++ /dev/null
@@ -1,41 +0,0 @@
-package gcfg
-
-import (
- "gopkg.in/warnings.v0"
-)
-
-// FatalOnly filters the results of a Read*Into invocation and returns only
-// fatal errors. That is, errors (warnings) indicating data for unknown
-// sections / variables is ignored. Example invocation:
-//
-// err := gcfg.FatalOnly(gcfg.ReadFileInto(&cfg, configFile))
-// if err != nil {
-// ...
-//
-func FatalOnly(err error) error {
- return warnings.FatalOnly(err)
-}
-
-func isFatal(err error) bool {
- _, ok := err.(extraData)
- return !ok
-}
-
-type extraData struct {
- section string
- subsection *string
- variable *string
-}
-
-func (e extraData) Error() string {
- s := "can't store data at section \"" + e.section + "\""
- if e.subsection != nil {
- s += ", subsection \"" + *e.subsection + "\""
- }
- if e.variable != nil {
- s += ", variable \"" + *e.variable + "\""
- }
- return s
-}
-
-var _ error = extraData{}
diff --git a/vendor/github.com/go-git/gcfg/go1_0.go b/vendor/github.com/go-git/gcfg/go1_0.go
deleted file mode 100644
index 6670210..0000000
--- a/vendor/github.com/go-git/gcfg/go1_0.go
+++ /dev/null
@@ -1,7 +0,0 @@
-// +build !go1.2
-
-package gcfg
-
-type textUnmarshaler interface {
- UnmarshalText(text []byte) error
-}
diff --git a/vendor/github.com/go-git/gcfg/go1_2.go b/vendor/github.com/go-git/gcfg/go1_2.go
deleted file mode 100644
index 6f5843b..0000000
--- a/vendor/github.com/go-git/gcfg/go1_2.go
+++ /dev/null
@@ -1,9 +0,0 @@
-// +build go1.2
-
-package gcfg
-
-import (
- "encoding"
-)
-
-type textUnmarshaler encoding.TextUnmarshaler
diff --git a/vendor/github.com/go-git/gcfg/read.go b/vendor/github.com/go-git/gcfg/read.go
deleted file mode 100644
index 4dfdc5c..0000000
--- a/vendor/github.com/go-git/gcfg/read.go
+++ /dev/null
@@ -1,273 +0,0 @@
-package gcfg
-
-import (
- "fmt"
- "io"
- "io/ioutil"
- "os"
- "strings"
-
- "github.com/go-git/gcfg/scanner"
- "github.com/go-git/gcfg/token"
- "gopkg.in/warnings.v0"
-)
-
-var unescape = map[rune]rune{'\\': '\\', '"': '"', 'n': '\n', 't': '\t', 'b': '\b'}
-
-// no error: invalid literals should be caught by scanner
-func unquote(s string) string {
- u, q, esc := make([]rune, 0, len(s)), false, false
- for _, c := range s {
- if esc {
- uc, ok := unescape[c]
- switch {
- case ok:
- u = append(u, uc)
- fallthrough
- case !q && c == '\n':
- esc = false
- continue
- }
- panic("invalid escape sequence")
- }
- switch c {
- case '"':
- q = !q
- case '\\':
- esc = true
- default:
- u = append(u, c)
- }
- }
- if q {
- panic("missing end quote")
- }
- if esc {
- panic("invalid escape sequence")
- }
- return string(u)
-}
-
-func read(c *warnings.Collector, callback func(string, string, string, string, bool) error,
- fset *token.FileSet, file *token.File, src []byte) error {
- //
- var s scanner.Scanner
- var errs scanner.ErrorList
- s.Init(file, src, func(p token.Position, m string) { errs.Add(p, m) }, 0)
- sect, sectsub := "", ""
- pos, tok, lit := s.Scan()
- errfn := func(msg string) error {
- return fmt.Errorf("%s: %s", fset.Position(pos), msg)
- }
- for {
- if errs.Len() > 0 {
- if err := c.Collect(errs.Err()); err != nil {
- return err
- }
- }
- switch tok {
- case token.EOF:
- return nil
- case token.EOL, token.COMMENT:
- pos, tok, lit = s.Scan()
- case token.LBRACK:
- pos, tok, lit = s.Scan()
- if errs.Len() > 0 {
- if err := c.Collect(errs.Err()); err != nil {
- return err
- }
- }
- if tok != token.IDENT {
- if err := c.Collect(errfn("expected section name")); err != nil {
- return err
- }
- }
- sect, sectsub = lit, ""
- pos, tok, lit = s.Scan()
- if errs.Len() > 0 {
- if err := c.Collect(errs.Err()); err != nil {
- return err
- }
- }
- if tok == token.STRING {
- sectsub = unquote(lit)
- if sectsub == "" {
- if err := c.Collect(errfn("empty subsection name")); err != nil {
- return err
- }
- }
- pos, tok, lit = s.Scan()
- if errs.Len() > 0 {
- if err := c.Collect(errs.Err()); err != nil {
- return err
- }
- }
- }
- if tok != token.RBRACK {
- if sectsub == "" {
- if err := c.Collect(errfn("expected subsection name or right bracket")); err != nil {
- return err
- }
- }
- if err := c.Collect(errfn("expected right bracket")); err != nil {
- return err
- }
- }
- pos, tok, lit = s.Scan()
- if tok != token.EOL && tok != token.EOF && tok != token.COMMENT {
- if err := c.Collect(errfn("expected EOL, EOF, or comment")); err != nil {
- return err
- }
- }
- // If a section/subsection header was found, ensure a
- // container object is created, even if there are no
- // variables further down.
- err := c.Collect(callback(sect, sectsub, "", "", true))
- if err != nil {
- return err
- }
- case token.IDENT:
- if sect == "" {
- if err := c.Collect(errfn("expected section header")); err != nil {
- return err
- }
- }
- n := lit
- pos, tok, lit = s.Scan()
- if errs.Len() > 0 {
- return errs.Err()
- }
- blank, v := tok == token.EOF || tok == token.EOL || tok == token.COMMENT, ""
- if !blank {
- if tok != token.ASSIGN {
- if err := c.Collect(errfn("expected '='")); err != nil {
- return err
- }
- }
- pos, tok, lit = s.Scan()
- if errs.Len() > 0 {
- if err := c.Collect(errs.Err()); err != nil {
- return err
- }
- }
- if tok != token.STRING {
- if err := c.Collect(errfn("expected value")); err != nil {
- return err
- }
- }
- v = unquote(lit)
- pos, tok, lit = s.Scan()
- if errs.Len() > 0 {
- if err := c.Collect(errs.Err()); err != nil {
- return err
- }
- }
- if tok != token.EOL && tok != token.EOF && tok != token.COMMENT {
- if err := c.Collect(errfn("expected EOL, EOF, or comment")); err != nil {
- return err
- }
- }
- }
- err := c.Collect(callback(sect, sectsub, n, v, blank))
- if err != nil {
- return err
- }
- default:
- if sect == "" {
- if err := c.Collect(errfn("expected section header")); err != nil {
- return err
- }
- }
- if err := c.Collect(errfn("expected section header or variable declaration")); err != nil {
- return err
- }
- }
- }
- panic("never reached")
-}
-
-func readInto(config interface{}, fset *token.FileSet, file *token.File,
- src []byte) error {
- //
- c := warnings.NewCollector(isFatal)
- firstPassCallback := func(s string, ss string, k string, v string, bv bool) error {
- return set(c, config, s, ss, k, v, bv, false)
- }
- err := read(c, firstPassCallback, fset, file, src)
- if err != nil {
- return err
- }
- secondPassCallback := func(s string, ss string, k string, v string, bv bool) error {
- return set(c, config, s, ss, k, v, bv, true)
- }
- err = read(c, secondPassCallback, fset, file, src)
- if err != nil {
- return err
- }
- return c.Done()
-}
-
-// ReadWithCallback reads gcfg formatted data from reader and calls
-// callback with each section and option found.
-//
-// Callback is called with section, subsection, option key, option value
-// and blank value flag as arguments.
-//
-// When a section is found, callback is called with nil subsection, option key
-// and option value.
-//
-// When a subsection is found, callback is called with nil option key and
-// option value.
-//
-// If blank value flag is true, it means that the value was not set for an option
-// (as opposed to set to empty string).
-//
-// If callback returns an error, ReadWithCallback terminates with an error too.
-func ReadWithCallback(reader io.Reader, callback func(string, string, string, string, bool) error) error {
- src, err := ioutil.ReadAll(reader)
- if err != nil {
- return err
- }
-
- fset := token.NewFileSet()
- file := fset.AddFile("", fset.Base(), len(src))
- c := warnings.NewCollector(isFatal)
-
- return read(c, callback, fset, file, src)
-}
-
-// ReadInto reads gcfg formatted data from reader and sets the values into the
-// corresponding fields in config.
-func ReadInto(config interface{}, reader io.Reader) error {
- src, err := ioutil.ReadAll(reader)
- if err != nil {
- return err
- }
- fset := token.NewFileSet()
- file := fset.AddFile("", fset.Base(), len(src))
- return readInto(config, fset, file, src)
-}
-
-// ReadStringInto reads gcfg formatted data from str and sets the values into
-// the corresponding fields in config.
-func ReadStringInto(config interface{}, str string) error {
- r := strings.NewReader(str)
- return ReadInto(config, r)
-}
-
-// ReadFileInto reads gcfg formatted data from the file filename and sets the
-// values into the corresponding fields in config.
-func ReadFileInto(config interface{}, filename string) error {
- f, err := os.Open(filename)
- if err != nil {
- return err
- }
- defer f.Close()
- src, err := ioutil.ReadAll(f)
- if err != nil {
- return err
- }
- fset := token.NewFileSet()
- file := fset.AddFile(filename, fset.Base(), len(src))
- return readInto(config, fset, file, src)
-}
diff --git a/vendor/github.com/go-git/gcfg/scanner/errors.go b/vendor/github.com/go-git/gcfg/scanner/errors.go
deleted file mode 100644
index a6e00f5..0000000
--- a/vendor/github.com/go-git/gcfg/scanner/errors.go
+++ /dev/null
@@ -1,121 +0,0 @@
-// Copyright 2009 The Go Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package scanner
-
-import (
- "fmt"
- "io"
- "sort"
-)
-
-import (
- "github.com/go-git/gcfg/token"
-)
-
-// In an ErrorList, an error is represented by an *Error.
-// The position Pos, if valid, points to the beginning of
-// the offending token, and the error condition is described
-// by Msg.
-//
-type Error struct {
- Pos token.Position
- Msg string
-}
-
-// Error implements the error interface.
-func (e Error) Error() string {
- if e.Pos.Filename != "" || e.Pos.IsValid() {
- // don't print ""
- // TODO(gri) reconsider the semantics of Position.IsValid
- return e.Pos.String() + ": " + e.Msg
- }
- return e.Msg
-}
-
-// ErrorList is a list of *Errors.
-// The zero value for an ErrorList is an empty ErrorList ready to use.
-//
-type ErrorList []*Error
-
-// Add adds an Error with given position and error message to an ErrorList.
-func (p *ErrorList) Add(pos token.Position, msg string) {
- *p = append(*p, &Error{pos, msg})
-}
-
-// Reset resets an ErrorList to no errors.
-func (p *ErrorList) Reset() { *p = (*p)[0:0] }
-
-// ErrorList implements the sort Interface.
-func (p ErrorList) Len() int { return len(p) }
-func (p ErrorList) Swap(i, j int) { p[i], p[j] = p[j], p[i] }
-
-func (p ErrorList) Less(i, j int) bool {
- e := &p[i].Pos
- f := &p[j].Pos
- if e.Filename < f.Filename {
- return true
- }
- if e.Filename == f.Filename {
- return e.Offset < f.Offset
- }
- return false
-}
-
-// Sort sorts an ErrorList. *Error entries are sorted by position,
-// other errors are sorted by error message, and before any *Error
-// entry.
-//
-func (p ErrorList) Sort() {
- sort.Sort(p)
-}
-
-// RemoveMultiples sorts an ErrorList and removes all but the first error per line.
-func (p *ErrorList) RemoveMultiples() {
- sort.Sort(p)
- var last token.Position // initial last.Line is != any legal error line
- i := 0
- for _, e := range *p {
- if e.Pos.Filename != last.Filename || e.Pos.Line != last.Line {
- last = e.Pos
- (*p)[i] = e
- i++
- }
- }
- (*p) = (*p)[0:i]
-}
-
-// An ErrorList implements the error interface.
-func (p ErrorList) Error() string {
- switch len(p) {
- case 0:
- return "no errors"
- case 1:
- return p[0].Error()
- }
- return fmt.Sprintf("%s (and %d more errors)", p[0], len(p)-1)
-}
-
-// Err returns an error equivalent to this error list.
-// If the list is empty, Err returns nil.
-func (p ErrorList) Err() error {
- if len(p) == 0 {
- return nil
- }
- return p
-}
-
-// PrintError is a utility function that prints a list of errors to w,
-// one error per line, if the err parameter is an ErrorList. Otherwise
-// it prints the err string.
-//
-func PrintError(w io.Writer, err error) {
- if list, ok := err.(ErrorList); ok {
- for _, e := range list {
- fmt.Fprintf(w, "%s\n", e)
- }
- } else if err != nil {
- fmt.Fprintf(w, "%s\n", err)
- }
-}
diff --git a/vendor/github.com/go-git/gcfg/scanner/scanner.go b/vendor/github.com/go-git/gcfg/scanner/scanner.go
deleted file mode 100644
index 41aafec..0000000
--- a/vendor/github.com/go-git/gcfg/scanner/scanner.go
+++ /dev/null
@@ -1,342 +0,0 @@
-// Copyright 2009 The Go Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-// Package scanner implements a scanner for gcfg configuration text.
-// It takes a []byte as source which can then be tokenized
-// through repeated calls to the Scan method.
-//
-// Note that the API for the scanner package may change to accommodate new
-// features or implementation changes in gcfg.
-//
-package scanner
-
-import (
- "fmt"
- "path/filepath"
- "unicode"
- "unicode/utf8"
-)
-
-import (
- "github.com/go-git/gcfg/token"
-)
-
-// An ErrorHandler may be provided to Scanner.Init. If a syntax error is
-// encountered and a handler was installed, the handler is called with a
-// position and an error message. The position points to the beginning of
-// the offending token.
-//
-type ErrorHandler func(pos token.Position, msg string)
-
-// A Scanner holds the scanner's internal state while processing
-// a given text. It can be allocated as part of another data
-// structure but must be initialized via Init before use.
-//
-type Scanner struct {
- // immutable state
- file *token.File // source file handle
- dir string // directory portion of file.Name()
- src []byte // source
- err ErrorHandler // error reporting; or nil
- mode Mode // scanning mode
-
- // scanning state
- ch rune // current character
- offset int // character offset
- rdOffset int // reading offset (position after current character)
- lineOffset int // current line offset
- nextVal bool // next token is expected to be a value
-
- // public state - ok to modify
- ErrorCount int // number of errors encountered
-}
-
-// Read the next Unicode char into s.ch.
-// s.ch < 0 means end-of-file.
-//
-func (s *Scanner) next() {
- if s.rdOffset < len(s.src) {
- s.offset = s.rdOffset
- if s.ch == '\n' {
- s.lineOffset = s.offset
- s.file.AddLine(s.offset)
- }
- r, w := rune(s.src[s.rdOffset]), 1
- switch {
- case r == 0:
- s.error(s.offset, "illegal character NUL")
- case r >= 0x80:
- // not ASCII
- r, w = utf8.DecodeRune(s.src[s.rdOffset:])
- if r == utf8.RuneError && w == 1 {
- s.error(s.offset, "illegal UTF-8 encoding")
- }
- }
- s.rdOffset += w
- s.ch = r
- } else {
- s.offset = len(s.src)
- if s.ch == '\n' {
- s.lineOffset = s.offset
- s.file.AddLine(s.offset)
- }
- s.ch = -1 // eof
- }
-}
-
-// A mode value is a set of flags (or 0).
-// They control scanner behavior.
-//
-type Mode uint
-
-const (
- ScanComments Mode = 1 << iota // return comments as COMMENT tokens
-)
-
-// Init prepares the scanner s to tokenize the text src by setting the
-// scanner at the beginning of src. The scanner uses the file set file
-// for position information and it adds line information for each line.
-// It is ok to re-use the same file when re-scanning the same file as
-// line information which is already present is ignored. Init causes a
-// panic if the file size does not match the src size.
-//
-// Calls to Scan will invoke the error handler err if they encounter a
-// syntax error and err is not nil. Also, for each error encountered,
-// the Scanner field ErrorCount is incremented by one. The mode parameter
-// determines how comments are handled.
-//
-// Note that Init may call err if there is an error in the first character
-// of the file.
-//
-func (s *Scanner) Init(file *token.File, src []byte, err ErrorHandler, mode Mode) {
- // Explicitly initialize all fields since a scanner may be reused.
- if file.Size() != len(src) {
- panic(fmt.Sprintf("file size (%d) does not match src len (%d)", file.Size(), len(src)))
- }
- s.file = file
- s.dir, _ = filepath.Split(file.Name())
- s.src = src
- s.err = err
- s.mode = mode
-
- s.ch = ' '
- s.offset = 0
- s.rdOffset = 0
- s.lineOffset = 0
- s.ErrorCount = 0
- s.nextVal = false
-
- s.next()
-}
-
-func (s *Scanner) error(offs int, msg string) {
- if s.err != nil {
- s.err(s.file.Position(s.file.Pos(offs)), msg)
- }
- s.ErrorCount++
-}
-
-func (s *Scanner) scanComment() string {
- // initial [;#] already consumed
- offs := s.offset - 1 // position of initial [;#]
-
- for s.ch != '\n' && s.ch >= 0 {
- s.next()
- }
- return string(s.src[offs:s.offset])
-}
-
-func isLetter(ch rune) bool {
- return 'a' <= ch && ch <= 'z' || 'A' <= ch && ch <= 'Z' || ch >= 0x80 && unicode.IsLetter(ch)
-}
-
-func isDigit(ch rune) bool {
- return '0' <= ch && ch <= '9' || ch >= 0x80 && unicode.IsDigit(ch)
-}
-
-func (s *Scanner) scanIdentifier() string {
- offs := s.offset
- for isLetter(s.ch) || isDigit(s.ch) || s.ch == '-' {
- s.next()
- }
- return string(s.src[offs:s.offset])
-}
-
-func (s *Scanner) scanEscape(val bool) {
- offs := s.offset
- ch := s.ch
- s.next() // always make progress
- switch ch {
- case '\\', '"':
- // ok
- case 'n', 't', 'b':
- if val {
- break // ok
- }
- fallthrough
- default:
- s.error(offs, "unknown escape sequence")
- }
-}
-
-func (s *Scanner) scanString() string {
- // '"' opening already consumed
- offs := s.offset - 1
-
- for s.ch != '"' {
- ch := s.ch
- s.next()
- if ch == '\n' || ch < 0 {
- s.error(offs, "string not terminated")
- break
- }
- if ch == '\\' {
- s.scanEscape(false)
- }
- }
-
- s.next()
-
- return string(s.src[offs:s.offset])
-}
-
-func stripCR(b []byte) []byte {
- c := make([]byte, len(b))
- i := 0
- for _, ch := range b {
- if ch != '\r' {
- c[i] = ch
- i++
- }
- }
- return c[:i]
-}
-
-func (s *Scanner) scanValString() string {
- offs := s.offset
-
- hasCR := false
- end := offs
- inQuote := false
-loop:
- for inQuote || s.ch >= 0 && s.ch != '\n' && s.ch != ';' && s.ch != '#' {
- ch := s.ch
- s.next()
- switch {
- case inQuote && ch == '\\':
- s.scanEscape(true)
- case !inQuote && ch == '\\':
- if s.ch == '\r' {
- hasCR = true
- s.next()
- }
- if s.ch != '\n' {
- s.scanEscape(true)
- } else {
- s.next()
- }
- case ch == '"':
- inQuote = !inQuote
- case ch == '\r':
- hasCR = true
- case ch < 0 || inQuote && ch == '\n':
- s.error(offs, "string not terminated")
- break loop
- }
- if inQuote || !isWhiteSpace(ch) {
- end = s.offset
- }
- }
-
- lit := s.src[offs:end]
- if hasCR {
- lit = stripCR(lit)
- }
-
- return string(lit)
-}
-
-func isWhiteSpace(ch rune) bool {
- return ch == ' ' || ch == '\t' || ch == '\r'
-}
-
-func (s *Scanner) skipWhitespace() {
- for isWhiteSpace(s.ch) {
- s.next()
- }
-}
-
-// Scan scans the next token and returns the token position, the token,
-// and its literal string if applicable. The source end is indicated by
-// token.EOF.
-//
-// If the returned token is a literal (token.IDENT, token.STRING) or
-// token.COMMENT, the literal string has the corresponding value.
-//
-// If the returned token is token.ILLEGAL, the literal string is the
-// offending character.
-//
-// In all other cases, Scan returns an empty literal string.
-//
-// For more tolerant parsing, Scan will return a valid token if
-// possible even if a syntax error was encountered. Thus, even
-// if the resulting token sequence contains no illegal tokens,
-// a client may not assume that no error occurred. Instead it
-// must check the scanner's ErrorCount or the number of calls
-// of the error handler, if there was one installed.
-//
-// Scan adds line information to the file added to the file
-// set with Init. Token positions are relative to that file
-// and thus relative to the file set.
-//
-func (s *Scanner) Scan() (pos token.Pos, tok token.Token, lit string) {
-scanAgain:
- s.skipWhitespace()
-
- // current token start
- pos = s.file.Pos(s.offset)
-
- // determine token value
- switch ch := s.ch; {
- case s.nextVal:
- lit = s.scanValString()
- tok = token.STRING
- s.nextVal = false
- case isLetter(ch):
- lit = s.scanIdentifier()
- tok = token.IDENT
- default:
- s.next() // always make progress
- switch ch {
- case -1:
- tok = token.EOF
- case '\n':
- tok = token.EOL
- case '"':
- tok = token.STRING
- lit = s.scanString()
- case '[':
- tok = token.LBRACK
- case ']':
- tok = token.RBRACK
- case ';', '#':
- // comment
- lit = s.scanComment()
- if s.mode&ScanComments == 0 {
- // skip comment
- goto scanAgain
- }
- tok = token.COMMENT
- case '=':
- tok = token.ASSIGN
- s.nextVal = true
- default:
- s.error(s.file.Offset(pos), fmt.Sprintf("illegal character %#U", ch))
- tok = token.ILLEGAL
- lit = string(ch)
- }
- }
-
- return
-}
diff --git a/vendor/github.com/go-git/gcfg/set.go b/vendor/github.com/go-git/gcfg/set.go
deleted file mode 100644
index e2d9278..0000000
--- a/vendor/github.com/go-git/gcfg/set.go
+++ /dev/null
@@ -1,332 +0,0 @@
-package gcfg
-
-import (
- "bytes"
- "encoding/gob"
- "fmt"
- "math/big"
- "reflect"
- "strings"
- "unicode"
- "unicode/utf8"
-
- "github.com/go-git/gcfg/types"
- "gopkg.in/warnings.v0"
-)
-
-type tag struct {
- ident string
- intMode string
-}
-
-func newTag(ts string) tag {
- t := tag{}
- s := strings.Split(ts, ",")
- t.ident = s[0]
- for _, tse := range s[1:] {
- if strings.HasPrefix(tse, "int=") {
- t.intMode = tse[len("int="):]
- }
- }
- return t
-}
-
-func fieldFold(v reflect.Value, name string) (reflect.Value, tag) {
- var n string
- r0, _ := utf8.DecodeRuneInString(name)
- if unicode.IsLetter(r0) && !unicode.IsLower(r0) && !unicode.IsUpper(r0) {
- n = "X"
- }
- n += strings.Replace(name, "-", "_", -1)
- f, ok := v.Type().FieldByNameFunc(func(fieldName string) bool {
- if !v.FieldByName(fieldName).CanSet() {
- return false
- }
- f, _ := v.Type().FieldByName(fieldName)
- t := newTag(f.Tag.Get("gcfg"))
- if t.ident != "" {
- return strings.EqualFold(t.ident, name)
- }
- return strings.EqualFold(n, fieldName)
- })
- if !ok {
- return reflect.Value{}, tag{}
- }
- return v.FieldByName(f.Name), newTag(f.Tag.Get("gcfg"))
-}
-
-type setter func(destp interface{}, blank bool, val string, t tag) error
-
-var errUnsupportedType = fmt.Errorf("unsupported type")
-var errBlankUnsupported = fmt.Errorf("blank value not supported for type")
-
-var setters = []setter{
- typeSetter, textUnmarshalerSetter, kindSetter, scanSetter,
-}
-
-func textUnmarshalerSetter(d interface{}, blank bool, val string, t tag) error {
- dtu, ok := d.(textUnmarshaler)
- if !ok {
- return errUnsupportedType
- }
- if blank {
- return errBlankUnsupported
- }
- return dtu.UnmarshalText([]byte(val))
-}
-
-func boolSetter(d interface{}, blank bool, val string, t tag) error {
- if blank {
- reflect.ValueOf(d).Elem().Set(reflect.ValueOf(true))
- return nil
- }
- b, err := types.ParseBool(val)
- if err == nil {
- reflect.ValueOf(d).Elem().Set(reflect.ValueOf(b))
- }
- return err
-}
-
-func intMode(mode string) types.IntMode {
- var m types.IntMode
- if strings.ContainsAny(mode, "dD") {
- m |= types.Dec
- }
- if strings.ContainsAny(mode, "hH") {
- m |= types.Hex
- }
- if strings.ContainsAny(mode, "oO") {
- m |= types.Oct
- }
- return m
-}
-
-var typeModes = map[reflect.Type]types.IntMode{
- reflect.TypeOf(int(0)): types.Dec | types.Hex,
- reflect.TypeOf(int8(0)): types.Dec | types.Hex,
- reflect.TypeOf(int16(0)): types.Dec | types.Hex,
- reflect.TypeOf(int32(0)): types.Dec | types.Hex,
- reflect.TypeOf(int64(0)): types.Dec | types.Hex,
- reflect.TypeOf(uint(0)): types.Dec | types.Hex,
- reflect.TypeOf(uint8(0)): types.Dec | types.Hex,
- reflect.TypeOf(uint16(0)): types.Dec | types.Hex,
- reflect.TypeOf(uint32(0)): types.Dec | types.Hex,
- reflect.TypeOf(uint64(0)): types.Dec | types.Hex,
- // use default mode (allow dec/hex/oct) for uintptr type
- reflect.TypeOf(big.Int{}): types.Dec | types.Hex,
-}
-
-func intModeDefault(t reflect.Type) types.IntMode {
- m, ok := typeModes[t]
- if !ok {
- m = types.Dec | types.Hex | types.Oct
- }
- return m
-}
-
-func intSetter(d interface{}, blank bool, val string, t tag) error {
- if blank {
- return errBlankUnsupported
- }
- mode := intMode(t.intMode)
- if mode == 0 {
- mode = intModeDefault(reflect.TypeOf(d).Elem())
- }
- return types.ParseInt(d, val, mode)
-}
-
-func stringSetter(d interface{}, blank bool, val string, t tag) error {
- if blank {
- return errBlankUnsupported
- }
- dsp, ok := d.(*string)
- if !ok {
- return errUnsupportedType
- }
- *dsp = val
- return nil
-}
-
-var kindSetters = map[reflect.Kind]setter{
- reflect.String: stringSetter,
- reflect.Bool: boolSetter,
- reflect.Int: intSetter,
- reflect.Int8: intSetter,
- reflect.Int16: intSetter,
- reflect.Int32: intSetter,
- reflect.Int64: intSetter,
- reflect.Uint: intSetter,
- reflect.Uint8: intSetter,
- reflect.Uint16: intSetter,
- reflect.Uint32: intSetter,
- reflect.Uint64: intSetter,
- reflect.Uintptr: intSetter,
-}
-
-var typeSetters = map[reflect.Type]setter{
- reflect.TypeOf(big.Int{}): intSetter,
-}
-
-func typeSetter(d interface{}, blank bool, val string, tt tag) error {
- t := reflect.ValueOf(d).Type().Elem()
- setter, ok := typeSetters[t]
- if !ok {
- return errUnsupportedType
- }
- return setter(d, blank, val, tt)
-}
-
-func kindSetter(d interface{}, blank bool, val string, tt tag) error {
- k := reflect.ValueOf(d).Type().Elem().Kind()
- setter, ok := kindSetters[k]
- if !ok {
- return errUnsupportedType
- }
- return setter(d, blank, val, tt)
-}
-
-func scanSetter(d interface{}, blank bool, val string, tt tag) error {
- if blank {
- return errBlankUnsupported
- }
- return types.ScanFully(d, val, 'v')
-}
-
-func newValue(c *warnings.Collector, sect string, vCfg reflect.Value,
- vType reflect.Type) (reflect.Value, error) {
- //
- pv := reflect.New(vType)
- dfltName := "default-" + sect
- dfltField, _ := fieldFold(vCfg, dfltName)
- var err error
- if dfltField.IsValid() {
- b := bytes.NewBuffer(nil)
- ge := gob.NewEncoder(b)
- if err = c.Collect(ge.EncodeValue(dfltField)); err != nil {
- return pv, err
- }
- gd := gob.NewDecoder(bytes.NewReader(b.Bytes()))
- if err = c.Collect(gd.DecodeValue(pv.Elem())); err != nil {
- return pv, err
- }
- }
- return pv, nil
-}
-
-func set(c *warnings.Collector, cfg interface{}, sect, sub, name string,
- value string, blankValue bool, subsectPass bool) error {
- //
- vPCfg := reflect.ValueOf(cfg)
- if vPCfg.Kind() != reflect.Ptr || vPCfg.Elem().Kind() != reflect.Struct {
- panic(fmt.Errorf("config must be a pointer to a struct"))
- }
- vCfg := vPCfg.Elem()
- vSect, _ := fieldFold(vCfg, sect)
- if !vSect.IsValid() {
- err := extraData{section: sect}
- return c.Collect(err)
- }
- isSubsect := vSect.Kind() == reflect.Map
- if subsectPass != isSubsect {
- return nil
- }
- if isSubsect {
- vst := vSect.Type()
- if vst.Key().Kind() != reflect.String ||
- vst.Elem().Kind() != reflect.Ptr ||
- vst.Elem().Elem().Kind() != reflect.Struct {
- panic(fmt.Errorf("map field for section must have string keys and "+
- " pointer-to-struct values: section %q", sect))
- }
- if vSect.IsNil() {
- vSect.Set(reflect.MakeMap(vst))
- }
- k := reflect.ValueOf(sub)
- pv := vSect.MapIndex(k)
- if !pv.IsValid() {
- vType := vSect.Type().Elem().Elem()
- var err error
- if pv, err = newValue(c, sect, vCfg, vType); err != nil {
- return err
- }
- vSect.SetMapIndex(k, pv)
- }
- vSect = pv.Elem()
- } else if vSect.Kind() != reflect.Struct {
- panic(fmt.Errorf("field for section must be a map or a struct: "+
- "section %q", sect))
- } else if sub != "" {
- err := extraData{section: sect, subsection: &sub}
- return c.Collect(err)
- }
- // Empty name is a special value, meaning that only the
- // section/subsection object is to be created, with no values set.
- if name == "" {
- return nil
- }
- vVar, t := fieldFold(vSect, name)
- if !vVar.IsValid() {
- var err error
- if isSubsect {
- err = extraData{section: sect, subsection: &sub, variable: &name}
- } else {
- err = extraData{section: sect, variable: &name}
- }
- return c.Collect(err)
- }
- // vVal is either single-valued var, or newly allocated value within multi-valued var
- var vVal reflect.Value
- // multi-value if unnamed slice type
- isMulti := vVar.Type().Name() == "" && vVar.Kind() == reflect.Slice ||
- vVar.Type().Name() == "" && vVar.Kind() == reflect.Ptr && vVar.Type().Elem().Name() == "" && vVar.Type().Elem().Kind() == reflect.Slice
- if isMulti && vVar.Kind() == reflect.Ptr {
- if vVar.IsNil() {
- vVar.Set(reflect.New(vVar.Type().Elem()))
- }
- vVar = vVar.Elem()
- }
- if isMulti && blankValue {
- vVar.Set(reflect.Zero(vVar.Type()))
- return nil
- }
- if isMulti {
- vVal = reflect.New(vVar.Type().Elem()).Elem()
- } else {
- vVal = vVar
- }
- isDeref := vVal.Type().Name() == "" && vVal.Type().Kind() == reflect.Ptr
- isNew := isDeref && vVal.IsNil()
- // vAddr is address of value to set (dereferenced & allocated as needed)
- var vAddr reflect.Value
- switch {
- case isNew:
- vAddr = reflect.New(vVal.Type().Elem())
- case isDeref && !isNew:
- vAddr = vVal
- default:
- vAddr = vVal.Addr()
- }
- vAddrI := vAddr.Interface()
- err, ok := error(nil), false
- for _, s := range setters {
- err = s(vAddrI, blankValue, value, t)
- if err == nil {
- ok = true
- break
- }
- if err != errUnsupportedType {
- return err
- }
- }
- if !ok {
- // in case all setters returned errUnsupportedType
- return err
- }
- if isNew { // set reference if it was dereferenced and newly allocated
- vVal.Set(vAddr)
- }
- if isMulti { // append if multi-valued
- vVar.Set(reflect.Append(vVar, vVal))
- }
- return nil
-}
diff --git a/vendor/github.com/go-git/gcfg/token/position.go b/vendor/github.com/go-git/gcfg/token/position.go
deleted file mode 100644
index fc45c1e..0000000
--- a/vendor/github.com/go-git/gcfg/token/position.go
+++ /dev/null
@@ -1,435 +0,0 @@
-// Copyright 2010 The Go Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-// TODO(gri) consider making this a separate package outside the go directory.
-
-package token
-
-import (
- "fmt"
- "sort"
- "sync"
-)
-
-// -----------------------------------------------------------------------------
-// Positions
-
-// Position describes an arbitrary source position
-// including the file, line, and column location.
-// A Position is valid if the line number is > 0.
-//
-type Position struct {
- Filename string // filename, if any
- Offset int // offset, starting at 0
- Line int // line number, starting at 1
- Column int // column number, starting at 1 (character count)
-}
-
-// IsValid returns true if the position is valid.
-func (pos *Position) IsValid() bool { return pos.Line > 0 }
-
-// String returns a string in one of several forms:
-//
-// file:line:column valid position with file name
-// line:column valid position without file name
-// file invalid position with file name
-// - invalid position without file name
-//
-func (pos Position) String() string {
- s := pos.Filename
- if pos.IsValid() {
- if s != "" {
- s += ":"
- }
- s += fmt.Sprintf("%d:%d", pos.Line, pos.Column)
- }
- if s == "" {
- s = "-"
- }
- return s
-}
-
-// Pos is a compact encoding of a source position within a file set.
-// It can be converted into a Position for a more convenient, but much
-// larger, representation.
-//
-// The Pos value for a given file is a number in the range [base, base+size],
-// where base and size are specified when adding the file to the file set via
-// AddFile.
-//
-// To create the Pos value for a specific source offset, first add
-// the respective file to the current file set (via FileSet.AddFile)
-// and then call File.Pos(offset) for that file. Given a Pos value p
-// for a specific file set fset, the corresponding Position value is
-// obtained by calling fset.Position(p).
-//
-// Pos values can be compared directly with the usual comparison operators:
-// If two Pos values p and q are in the same file, comparing p and q is
-// equivalent to comparing the respective source file offsets. If p and q
-// are in different files, p < q is true if the file implied by p was added
-// to the respective file set before the file implied by q.
-//
-type Pos int
-
-// The zero value for Pos is NoPos; there is no file and line information
-// associated with it, and NoPos().IsValid() is false. NoPos is always
-// smaller than any other Pos value. The corresponding Position value
-// for NoPos is the zero value for Position.
-//
-const NoPos Pos = 0
-
-// IsValid returns true if the position is valid.
-func (p Pos) IsValid() bool {
- return p != NoPos
-}
-
-// -----------------------------------------------------------------------------
-// File
-
-// A File is a handle for a file belonging to a FileSet.
-// A File has a name, size, and line offset table.
-//
-type File struct {
- set *FileSet
- name string // file name as provided to AddFile
- base int // Pos value range for this file is [base...base+size]
- size int // file size as provided to AddFile
-
- // lines and infos are protected by set.mutex
- lines []int
- infos []lineInfo
-}
-
-// Name returns the file name of file f as registered with AddFile.
-func (f *File) Name() string {
- return f.name
-}
-
-// Base returns the base offset of file f as registered with AddFile.
-func (f *File) Base() int {
- return f.base
-}
-
-// Size returns the size of file f as registered with AddFile.
-func (f *File) Size() int {
- return f.size
-}
-
-// LineCount returns the number of lines in file f.
-func (f *File) LineCount() int {
- f.set.mutex.RLock()
- n := len(f.lines)
- f.set.mutex.RUnlock()
- return n
-}
-
-// AddLine adds the line offset for a new line.
-// The line offset must be larger than the offset for the previous line
-// and smaller than the file size; otherwise the line offset is ignored.
-//
-func (f *File) AddLine(offset int) {
- f.set.mutex.Lock()
- if i := len(f.lines); (i == 0 || f.lines[i-1] < offset) && offset < f.size {
- f.lines = append(f.lines, offset)
- }
- f.set.mutex.Unlock()
-}
-
-// SetLines sets the line offsets for a file and returns true if successful.
-// The line offsets are the offsets of the first character of each line;
-// for instance for the content "ab\nc\n" the line offsets are {0, 3}.
-// An empty file has an empty line offset table.
-// Each line offset must be larger than the offset for the previous line
-// and smaller than the file size; otherwise SetLines fails and returns
-// false.
-//
-func (f *File) SetLines(lines []int) bool {
- // verify validity of lines table
- size := f.size
- for i, offset := range lines {
- if i > 0 && offset <= lines[i-1] || size <= offset {
- return false
- }
- }
-
- // set lines table
- f.set.mutex.Lock()
- f.lines = lines
- f.set.mutex.Unlock()
- return true
-}
-
-// SetLinesForContent sets the line offsets for the given file content.
-func (f *File) SetLinesForContent(content []byte) {
- var lines []int
- line := 0
- for offset, b := range content {
- if line >= 0 {
- lines = append(lines, line)
- }
- line = -1
- if b == '\n' {
- line = offset + 1
- }
- }
-
- // set lines table
- f.set.mutex.Lock()
- f.lines = lines
- f.set.mutex.Unlock()
-}
-
-// A lineInfo object describes alternative file and line number
-// information (such as provided via a //line comment in a .go
-// file) for a given file offset.
-type lineInfo struct {
- // fields are exported to make them accessible to gob
- Offset int
- Filename string
- Line int
-}
-
-// AddLineInfo adds alternative file and line number information for
-// a given file offset. The offset must be larger than the offset for
-// the previously added alternative line info and smaller than the
-// file size; otherwise the information is ignored.
-//
-// AddLineInfo is typically used to register alternative position
-// information for //line filename:line comments in source files.
-//
-func (f *File) AddLineInfo(offset int, filename string, line int) {
- f.set.mutex.Lock()
- if i := len(f.infos); i == 0 || f.infos[i-1].Offset < offset && offset < f.size {
- f.infos = append(f.infos, lineInfo{offset, filename, line})
- }
- f.set.mutex.Unlock()
-}
-
-// Pos returns the Pos value for the given file offset;
-// the offset must be <= f.Size().
-// f.Pos(f.Offset(p)) == p.
-//
-func (f *File) Pos(offset int) Pos {
- if offset > f.size {
- panic("illegal file offset")
- }
- return Pos(f.base + offset)
-}
-
-// Offset returns the offset for the given file position p;
-// p must be a valid Pos value in that file.
-// f.Offset(f.Pos(offset)) == offset.
-//
-func (f *File) Offset(p Pos) int {
- if int(p) < f.base || int(p) > f.base+f.size {
- panic("illegal Pos value")
- }
- return int(p) - f.base
-}
-
-// Line returns the line number for the given file position p;
-// p must be a Pos value in that file or NoPos.
-//
-func (f *File) Line(p Pos) int {
- // TODO(gri) this can be implemented much more efficiently
- return f.Position(p).Line
-}
-
-func searchLineInfos(a []lineInfo, x int) int {
- return sort.Search(len(a), func(i int) bool { return a[i].Offset > x }) - 1
-}
-
-// info returns the file name, line, and column number for a file offset.
-func (f *File) info(offset int) (filename string, line, column int) {
- filename = f.name
- if i := searchInts(f.lines, offset); i >= 0 {
- line, column = i+1, offset-f.lines[i]+1
- }
- if len(f.infos) > 0 {
- // almost no files have extra line infos
- if i := searchLineInfos(f.infos, offset); i >= 0 {
- alt := &f.infos[i]
- filename = alt.Filename
- if i := searchInts(f.lines, alt.Offset); i >= 0 {
- line += alt.Line - i - 1
- }
- }
- }
- return
-}
-
-func (f *File) position(p Pos) (pos Position) {
- offset := int(p) - f.base
- pos.Offset = offset
- pos.Filename, pos.Line, pos.Column = f.info(offset)
- return
-}
-
-// Position returns the Position value for the given file position p;
-// p must be a Pos value in that file or NoPos.
-//
-func (f *File) Position(p Pos) (pos Position) {
- if p != NoPos {
- if int(p) < f.base || int(p) > f.base+f.size {
- panic("illegal Pos value")
- }
- pos = f.position(p)
- }
- return
-}
-
-// -----------------------------------------------------------------------------
-// FileSet
-
-// A FileSet represents a set of source files.
-// Methods of file sets are synchronized; multiple goroutines
-// may invoke them concurrently.
-//
-type FileSet struct {
- mutex sync.RWMutex // protects the file set
- base int // base offset for the next file
- files []*File // list of files in the order added to the set
- last *File // cache of last file looked up
-}
-
-// NewFileSet creates a new file set.
-func NewFileSet() *FileSet {
- s := new(FileSet)
- s.base = 1 // 0 == NoPos
- return s
-}
-
-// Base returns the minimum base offset that must be provided to
-// AddFile when adding the next file.
-//
-func (s *FileSet) Base() int {
- s.mutex.RLock()
- b := s.base
- s.mutex.RUnlock()
- return b
-
-}
-
-// AddFile adds a new file with a given filename, base offset, and file size
-// to the file set s and returns the file. Multiple files may have the same
-// name. The base offset must not be smaller than the FileSet's Base(), and
-// size must not be negative.
-//
-// Adding the file will set the file set's Base() value to base + size + 1
-// as the minimum base value for the next file. The following relationship
-// exists between a Pos value p for a given file offset offs:
-//
-// int(p) = base + offs
-//
-// with offs in the range [0, size] and thus p in the range [base, base+size].
-// For convenience, File.Pos may be used to create file-specific position
-// values from a file offset.
-//
-func (s *FileSet) AddFile(filename string, base, size int) *File {
- s.mutex.Lock()
- defer s.mutex.Unlock()
- if base < s.base || size < 0 {
- panic("illegal base or size")
- }
- // base >= s.base && size >= 0
- f := &File{s, filename, base, size, []int{0}, nil}
- base += size + 1 // +1 because EOF also has a position
- if base < 0 {
- panic("token.Pos offset overflow (> 2G of source code in file set)")
- }
- // add the file to the file set
- s.base = base
- s.files = append(s.files, f)
- s.last = f
- return f
-}
-
-// Iterate calls f for the files in the file set in the order they were added
-// until f returns false.
-//
-func (s *FileSet) Iterate(f func(*File) bool) {
- for i := 0; ; i++ {
- var file *File
- s.mutex.RLock()
- if i < len(s.files) {
- file = s.files[i]
- }
- s.mutex.RUnlock()
- if file == nil || !f(file) {
- break
- }
- }
-}
-
-func searchFiles(a []*File, x int) int {
- return sort.Search(len(a), func(i int) bool { return a[i].base > x }) - 1
-}
-
-func (s *FileSet) file(p Pos) *File {
- // common case: p is in last file
- if f := s.last; f != nil && f.base <= int(p) && int(p) <= f.base+f.size {
- return f
- }
- // p is not in last file - search all files
- if i := searchFiles(s.files, int(p)); i >= 0 {
- f := s.files[i]
- // f.base <= int(p) by definition of searchFiles
- if int(p) <= f.base+f.size {
- s.last = f
- return f
- }
- }
- return nil
-}
-
-// File returns the file that contains the position p.
-// If no such file is found (for instance for p == NoPos),
-// the result is nil.
-//
-func (s *FileSet) File(p Pos) (f *File) {
- if p != NoPos {
- s.mutex.RLock()
- f = s.file(p)
- s.mutex.RUnlock()
- }
- return
-}
-
-// Position converts a Pos in the fileset into a general Position.
-func (s *FileSet) Position(p Pos) (pos Position) {
- if p != NoPos {
- s.mutex.RLock()
- if f := s.file(p); f != nil {
- pos = f.position(p)
- }
- s.mutex.RUnlock()
- }
- return
-}
-
-// -----------------------------------------------------------------------------
-// Helper functions
-
-func searchInts(a []int, x int) int {
- // This function body is a manually inlined version of:
- //
- // return sort.Search(len(a), func(i int) bool { return a[i] > x }) - 1
- //
- // With better compiler optimizations, this may not be needed in the
- // future, but at the moment this change improves the go/printer
- // benchmark performance by ~30%. This has a direct impact on the
- // speed of gofmt and thus seems worthwhile (2011-04-29).
- // TODO(gri): Remove this when compilers have caught up.
- i, j := 0, len(a)
- for i < j {
- h := i + (j-i)/2 // avoid overflow when computing h
- // i ≤ h < j
- if a[h] <= x {
- i = h + 1
- } else {
- j = h
- }
- }
- return i - 1
-}
diff --git a/vendor/github.com/go-git/gcfg/token/serialize.go b/vendor/github.com/go-git/gcfg/token/serialize.go
deleted file mode 100644
index 4adc8f9..0000000
--- a/vendor/github.com/go-git/gcfg/token/serialize.go
+++ /dev/null
@@ -1,56 +0,0 @@
-// Copyright 2011 The Go Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package token
-
-type serializedFile struct {
- // fields correspond 1:1 to fields with same (lower-case) name in File
- Name string
- Base int
- Size int
- Lines []int
- Infos []lineInfo
-}
-
-type serializedFileSet struct {
- Base int
- Files []serializedFile
-}
-
-// Read calls decode to deserialize a file set into s; s must not be nil.
-func (s *FileSet) Read(decode func(interface{}) error) error {
- var ss serializedFileSet
- if err := decode(&ss); err != nil {
- return err
- }
-
- s.mutex.Lock()
- s.base = ss.Base
- files := make([]*File, len(ss.Files))
- for i := 0; i < len(ss.Files); i++ {
- f := &ss.Files[i]
- files[i] = &File{s, f.Name, f.Base, f.Size, f.Lines, f.Infos}
- }
- s.files = files
- s.last = nil
- s.mutex.Unlock()
-
- return nil
-}
-
-// Write calls encode to serialize the file set s.
-func (s *FileSet) Write(encode func(interface{}) error) error {
- var ss serializedFileSet
-
- s.mutex.Lock()
- ss.Base = s.base
- files := make([]serializedFile, len(s.files))
- for i, f := range s.files {
- files[i] = serializedFile{f.name, f.base, f.size, f.lines, f.infos}
- }
- ss.Files = files
- s.mutex.Unlock()
-
- return encode(ss)
-}
diff --git a/vendor/github.com/go-git/gcfg/token/token.go b/vendor/github.com/go-git/gcfg/token/token.go
deleted file mode 100644
index b3c7c83..0000000
--- a/vendor/github.com/go-git/gcfg/token/token.go
+++ /dev/null
@@ -1,83 +0,0 @@
-// Copyright 2009 The Go Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-// Package token defines constants representing the lexical tokens of the gcfg
-// configuration syntax and basic operations on tokens (printing, predicates).
-//
-// Note that the API for the token package may change to accommodate new
-// features or implementation changes in gcfg.
-//
-package token
-
-import "strconv"
-
-// Token is the set of lexical tokens of the gcfg configuration syntax.
-type Token int
-
-// The list of tokens.
-const (
- // Special tokens
- ILLEGAL Token = iota
- EOF
- COMMENT
-
- literal_beg
- // Identifiers and basic type literals
- // (these tokens stand for classes of literals)
- IDENT // section-name, variable-name
- STRING // "subsection-name", variable value
- literal_end
-
- operator_beg
- // Operators and delimiters
- ASSIGN // =
- LBRACK // [
- RBRACK // ]
- EOL // \n
- operator_end
-)
-
-var tokens = [...]string{
- ILLEGAL: "ILLEGAL",
-
- EOF: "EOF",
- COMMENT: "COMMENT",
-
- IDENT: "IDENT",
- STRING: "STRING",
-
- ASSIGN: "=",
- LBRACK: "[",
- RBRACK: "]",
- EOL: "\n",
-}
-
-// String returns the string corresponding to the token tok.
-// For operators and delimiters, the string is the actual token character
-// sequence (e.g., for the token ASSIGN, the string is "="). For all other
-// tokens the string corresponds to the token constant name (e.g. for the
-// token IDENT, the string is "IDENT").
-//
-func (tok Token) String() string {
- s := ""
- if 0 <= tok && tok < Token(len(tokens)) {
- s = tokens[tok]
- }
- if s == "" {
- s = "token(" + strconv.Itoa(int(tok)) + ")"
- }
- return s
-}
-
-// Predicates
-
-// IsLiteral returns true for tokens corresponding to identifiers
-// and basic type literals; it returns false otherwise.
-//
-func (tok Token) IsLiteral() bool { return literal_beg < tok && tok < literal_end }
-
-// IsOperator returns true for tokens corresponding to operators and
-// delimiters; it returns false otherwise.
-//
-func (tok Token) IsOperator() bool { return operator_beg < tok && tok < operator_end }
diff --git a/vendor/github.com/go-git/gcfg/types/bool.go b/vendor/github.com/go-git/gcfg/types/bool.go
deleted file mode 100644
index 8dcae0d..0000000
--- a/vendor/github.com/go-git/gcfg/types/bool.go
+++ /dev/null
@@ -1,23 +0,0 @@
-package types
-
-// BoolValues defines the name and value mappings for ParseBool.
-var BoolValues = map[string]interface{}{
- "true": true, "yes": true, "on": true, "1": true,
- "false": false, "no": false, "off": false, "0": false,
-}
-
-var boolParser = func() *EnumParser {
- ep := &EnumParser{}
- ep.AddVals(BoolValues)
- return ep
-}()
-
-// ParseBool parses bool values according to the definitions in BoolValues.
-// Parsing is case-insensitive.
-func ParseBool(s string) (bool, error) {
- v, err := boolParser.Parse(s)
- if err != nil {
- return false, err
- }
- return v.(bool), nil
-}
diff --git a/vendor/github.com/go-git/gcfg/types/doc.go b/vendor/github.com/go-git/gcfg/types/doc.go
deleted file mode 100644
index 9f9c345..0000000
--- a/vendor/github.com/go-git/gcfg/types/doc.go
+++ /dev/null
@@ -1,4 +0,0 @@
-// Package types defines helpers for type conversions.
-//
-// The API for this package is not finalized yet.
-package types
diff --git a/vendor/github.com/go-git/gcfg/types/enum.go b/vendor/github.com/go-git/gcfg/types/enum.go
deleted file mode 100644
index 1a0c7ef..0000000
--- a/vendor/github.com/go-git/gcfg/types/enum.go
+++ /dev/null
@@ -1,44 +0,0 @@
-package types
-
-import (
- "fmt"
- "reflect"
- "strings"
-)
-
-// EnumParser parses "enum" values; i.e. a predefined set of strings to
-// predefined values.
-type EnumParser struct {
- Type string // type name; if not set, use type of first value added
- CaseMatch bool // if true, matching of strings is case-sensitive
- // PrefixMatch bool
- vals map[string]interface{}
-}
-
-// AddVals adds strings and values to an EnumParser.
-func (ep *EnumParser) AddVals(vals map[string]interface{}) {
- if ep.vals == nil {
- ep.vals = make(map[string]interface{})
- }
- for k, v := range vals {
- if ep.Type == "" {
- ep.Type = reflect.TypeOf(v).Name()
- }
- if !ep.CaseMatch {
- k = strings.ToLower(k)
- }
- ep.vals[k] = v
- }
-}
-
-// Parse parses the string and returns the value or an error.
-func (ep EnumParser) Parse(s string) (interface{}, error) {
- if !ep.CaseMatch {
- s = strings.ToLower(s)
- }
- v, ok := ep.vals[s]
- if !ok {
- return false, fmt.Errorf("failed to parse %s %#q", ep.Type, s)
- }
- return v, nil
-}
diff --git a/vendor/github.com/go-git/gcfg/types/int.go b/vendor/github.com/go-git/gcfg/types/int.go
deleted file mode 100644
index af7e75c..0000000
--- a/vendor/github.com/go-git/gcfg/types/int.go
+++ /dev/null
@@ -1,86 +0,0 @@
-package types
-
-import (
- "fmt"
- "strings"
-)
-
-// An IntMode is a mode for parsing integer values, representing a set of
-// accepted bases.
-type IntMode uint8
-
-// IntMode values for ParseInt; can be combined using binary or.
-const (
- Dec IntMode = 1 << iota
- Hex
- Oct
-)
-
-// String returns a string representation of IntMode; e.g. `IntMode(Dec|Hex)`.
-func (m IntMode) String() string {
- var modes []string
- if m&Dec != 0 {
- modes = append(modes, "Dec")
- }
- if m&Hex != 0 {
- modes = append(modes, "Hex")
- }
- if m&Oct != 0 {
- modes = append(modes, "Oct")
- }
- return "IntMode(" + strings.Join(modes, "|") + ")"
-}
-
-var errIntAmbig = fmt.Errorf("ambiguous integer value; must include '0' prefix")
-
-func prefix0(val string) bool {
- return strings.HasPrefix(val, "0") || strings.HasPrefix(val, "-0")
-}
-
-func prefix0x(val string) bool {
- return strings.HasPrefix(val, "0x") || strings.HasPrefix(val, "-0x")
-}
-
-// ParseInt parses val using mode into intptr, which must be a pointer to an
-// integer kind type. Non-decimal value require prefix `0` or `0x` in the cases
-// when mode permits ambiguity of base; otherwise the prefix can be omitted.
-func ParseInt(intptr interface{}, val string, mode IntMode) error {
- val = strings.TrimSpace(val)
- verb := byte(0)
- switch mode {
- case Dec:
- verb = 'd'
- case Dec + Hex:
- if prefix0x(val) {
- verb = 'v'
- } else {
- verb = 'd'
- }
- case Dec + Oct:
- if prefix0(val) && !prefix0x(val) {
- verb = 'v'
- } else {
- verb = 'd'
- }
- case Dec + Hex + Oct:
- verb = 'v'
- case Hex:
- if prefix0x(val) {
- verb = 'v'
- } else {
- verb = 'x'
- }
- case Oct:
- verb = 'o'
- case Hex + Oct:
- if prefix0(val) {
- verb = 'v'
- } else {
- return errIntAmbig
- }
- }
- if verb == 0 {
- panic("unsupported mode")
- }
- return ScanFully(intptr, val, verb)
-}
diff --git a/vendor/github.com/go-git/gcfg/types/scan.go b/vendor/github.com/go-git/gcfg/types/scan.go
deleted file mode 100644
index db2f6ed..0000000
--- a/vendor/github.com/go-git/gcfg/types/scan.go
+++ /dev/null
@@ -1,23 +0,0 @@
-package types
-
-import (
- "fmt"
- "io"
- "reflect"
-)
-
-// ScanFully uses fmt.Sscanf with verb to fully scan val into ptr.
-func ScanFully(ptr interface{}, val string, verb byte) error {
- t := reflect.ValueOf(ptr).Elem().Type()
- // attempt to read extra bytes to make sure the value is consumed
- var b []byte
- n, err := fmt.Sscanf(val, "%"+string(verb)+"%s", ptr, &b)
- switch {
- case n < 1 || n == 1 && err != io.EOF:
- return fmt.Errorf("failed to parse %q as %v: %v", val, t, err)
- case n > 1:
- return fmt.Errorf("failed to parse %q as %v: extra characters %q", val, t, string(b))
- }
- // n == 1 && err == io.EOF
- return nil
-}
diff --git a/vendor/github.com/go-git/go-billy/v5/.gitignore b/vendor/github.com/go-git/go-billy/v5/.gitignore
deleted file mode 100644
index 7aeb466..0000000
--- a/vendor/github.com/go-git/go-billy/v5/.gitignore
+++ /dev/null
@@ -1,4 +0,0 @@
-/coverage.txt
-/vendor
-Gopkg.lock
-Gopkg.toml
diff --git a/vendor/github.com/go-git/go-billy/v5/LICENSE b/vendor/github.com/go-git/go-billy/v5/LICENSE
deleted file mode 100644
index 9d60756..0000000
--- a/vendor/github.com/go-git/go-billy/v5/LICENSE
+++ /dev/null
@@ -1,201 +0,0 @@
- Apache License
- Version 2.0, January 2004
- http://www.apache.org/licenses/
-
- TERMS AND CONDITIONS FOR USE, REPRODUCTION, AND DISTRIBUTION
-
- 1. Definitions.
-
- "License" shall mean the terms and conditions for use, reproduction,
- and distribution as defined by Sections 1 through 9 of this document.
-
- "Licensor" shall mean the copyright owner or entity authorized by
- the copyright owner that is granting the License.
-
- "Legal Entity" shall mean the union of the acting entity and all
- other entities that control, are controlled by, or are under common
- control with that entity. For the purposes of this definition,
- "control" means (i) the power, direct or indirect, to cause the
- direction or management of such entity, whether by contract or
- otherwise, or (ii) ownership of fifty percent (50%) or more of the
- outstanding shares, or (iii) beneficial ownership of such entity.
-
- "You" (or "Your") shall mean an individual or Legal Entity
- exercising permissions granted by this License.
-
- "Source" form shall mean the preferred form for making modifications,
- including but not limited to software source code, documentation
- source, and configuration files.
-
- "Object" form shall mean any form resulting from mechanical
- transformation or translation of a Source form, including but
- not limited to compiled object code, generated documentation,
- and conversions to other media types.
-
- "Work" shall mean the work of authorship, whether in Source or
- Object form, made available under the License, as indicated by a
- copyright notice that is included in or attached to the work
- (an example is provided in the Appendix below).
-
- "Derivative Works" shall mean any work, whether in Source or Object
- form, that is based on (or derived from) the Work and for which the
- editorial revisions, annotations, elaborations, or other modifications
- represent, as a whole, an original work of authorship. For the purposes
- of this License, Derivative Works shall not include works that remain
- separable from, or merely link (or bind by name) to the interfaces of,
- the Work and Derivative Works thereof.
-
- "Contribution" shall mean any work of authorship, including
- the original version of the Work and any modifications or additions
- to that Work or Derivative Works thereof, that is intentionally
- submitted to Licensor for inclusion in the Work by the copyright owner
- or by an individual or Legal Entity authorized to submit on behalf of
- the copyright owner. For the purposes of this definition, "submitted"
- means any form of electronic, verbal, or written communication sent
- to the Licensor or its representatives, including but not limited to
- communication on electronic mailing lists, source code control systems,
- and issue tracking systems that are managed by, or on behalf of, the
- Licensor for the purpose of discussing and improving the Work, but
- excluding communication that is conspicuously marked or otherwise
- designated in writing by the copyright owner as "Not a Contribution."
-
- "Contributor" shall mean Licensor and any individual or Legal Entity
- on behalf of whom a Contribution has been received by Licensor and
- subsequently incorporated within the Work.
-
- 2. Grant of Copyright License. Subject to the terms and conditions of
- this License, each Contributor hereby grants to You a perpetual,
- worldwide, non-exclusive, no-charge, royalty-free, irrevocable
- copyright license to reproduce, prepare Derivative Works of,
- publicly display, publicly perform, sublicense, and distribute the
- Work and such Derivative Works in Source or Object form.
-
- 3. Grant of Patent License. Subject to the terms and conditions of
- this License, each Contributor hereby grants to You a perpetual,
- worldwide, non-exclusive, no-charge, royalty-free, irrevocable
- (except as stated in this section) patent license to make, have made,
- use, offer to sell, sell, import, and otherwise transfer the Work,
- where such license applies only to those patent claims licensable
- by such Contributor that are necessarily infringed by their
- Contribution(s) alone or by combination of their Contribution(s)
- with the Work to which such Contribution(s) was submitted. If You
- institute patent litigation against any entity (including a
- cross-claim or counterclaim in a lawsuit) alleging that the Work
- or a Contribution incorporated within the Work constitutes direct
- or contributory patent infringement, then any patent licenses
- granted to You under this License for that Work shall terminate
- as of the date such litigation is filed.
-
- 4. Redistribution. You may reproduce and distribute copies of the
- Work or Derivative Works thereof in any medium, with or without
- modifications, and in Source or Object form, provided that You
- meet the following conditions:
-
- (a) You must give any other recipients of the Work or
- Derivative Works a copy of this License; and
-
- (b) You must cause any modified files to carry prominent notices
- stating that You changed the files; and
-
- (c) You must retain, in the Source form of any Derivative Works
- that You distribute, all copyright, patent, trademark, and
- attribution notices from the Source form of the Work,
- excluding those notices that do not pertain to any part of
- the Derivative Works; and
-
- (d) If the Work includes a "NOTICE" text file as part of its
- distribution, then any Derivative Works that You distribute must
- include a readable copy of the attribution notices contained
- within such NOTICE file, excluding those notices that do not
- pertain to any part of the Derivative Works, in at least one
- of the following places: within a NOTICE text file distributed
- as part of the Derivative Works; within the Source form or
- documentation, if provided along with the Derivative Works; or,
- within a display generated by the Derivative Works, if and
- wherever such third-party notices normally appear. The contents
- of the NOTICE file are for informational purposes only and
- do not modify the License. You may add Your own attribution
- notices within Derivative Works that You distribute, alongside
- or as an addendum to the NOTICE text from the Work, provided
- that such additional attribution notices cannot be construed
- as modifying the License.
-
- You may add Your own copyright statement to Your modifications and
- may provide additional or different license terms and conditions
- for use, reproduction, or distribution of Your modifications, or
- for any such Derivative Works as a whole, provided Your use,
- reproduction, and distribution of the Work otherwise complies with
- the conditions stated in this License.
-
- 5. Submission of Contributions. Unless You explicitly state otherwise,
- any Contribution intentionally submitted for inclusion in the Work
- by You to the Licensor shall be under the terms and conditions of
- this License, without any additional terms or conditions.
- Notwithstanding the above, nothing herein shall supersede or modify
- the terms of any separate license agreement you may have executed
- with Licensor regarding such Contributions.
-
- 6. Trademarks. This License does not grant permission to use the trade
- names, trademarks, service marks, or product names of the Licensor,
- except as required for reasonable and customary use in describing the
- origin of the Work and reproducing the content of the NOTICE file.
-
- 7. Disclaimer of Warranty. Unless required by applicable law or
- agreed to in writing, Licensor provides the Work (and each
- Contributor provides its Contributions) on an "AS IS" BASIS,
- WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
- implied, including, without limitation, any warranties or conditions
- of TITLE, NON-INFRINGEMENT, MERCHANTABILITY, or FITNESS FOR A
- PARTICULAR PURPOSE. You are solely responsible for determining the
- appropriateness of using or redistributing the Work and assume any
- risks associated with Your exercise of permissions under this License.
-
- 8. Limitation of Liability. In no event and under no legal theory,
- whether in tort (including negligence), contract, or otherwise,
- unless required by applicable law (such as deliberate and grossly
- negligent acts) or agreed to in writing, shall any Contributor be
- liable to You for damages, including any direct, indirect, special,
- incidental, or consequential damages of any character arising as a
- result of this License or out of the use or inability to use the
- Work (including but not limited to damages for loss of goodwill,
- work stoppage, computer failure or malfunction, or any and all
- other commercial damages or losses), even if such Contributor
- has been advised of the possibility of such damages.
-
- 9. Accepting Warranty or Additional Liability. While redistributing
- the Work or Derivative Works thereof, You may choose to offer,
- and charge a fee for, acceptance of support, warranty, indemnity,
- or other liability obligations and/or rights consistent with this
- License. However, in accepting such obligations, You may act only
- on Your own behalf and on Your sole responsibility, not on behalf
- of any other Contributor, and only if You agree to indemnify,
- defend, and hold each Contributor harmless for any liability
- incurred by, or claims asserted against, such Contributor by reason
- of your accepting any such warranty or additional liability.
-
- END OF TERMS AND CONDITIONS
-
- APPENDIX: How to apply the Apache License to your work.
-
- To apply the Apache License to your work, attach the following
- boilerplate notice, with the fields enclosed by brackets "{}"
- replaced with your own identifying information. (Don't include
- the brackets!) The text should be enclosed in the appropriate
- comment syntax for the file format. We also recommend that a
- file or class name and description of purpose be included on the
- same "printed page" as the copyright notice for easier
- identification within third-party archives.
-
- Copyright 2017 Sourced Technologies S.L.
-
- Licensed under the Apache License, Version 2.0 (the "License");
- you may not use this file except in compliance with the License.
- You may obtain a copy of the License at
-
- http://www.apache.org/licenses/LICENSE-2.0
-
- Unless required by applicable law or agreed to in writing, software
- distributed under the License is distributed on an "AS IS" BASIS,
- WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
- See the License for the specific language governing permissions and
- limitations under the License.
diff --git a/vendor/github.com/go-git/go-billy/v5/README.md b/vendor/github.com/go-git/go-billy/v5/README.md
deleted file mode 100644
index da5c074..0000000
--- a/vendor/github.com/go-git/go-billy/v5/README.md
+++ /dev/null
@@ -1,73 +0,0 @@
-# go-billy [](https://pkg.go.dev/github.com/go-git/go-billy/v5) [](https://github.com/go-git/go-billy/actions?query=workflow%3ATest)
-
-The missing interface filesystem abstraction for Go.
-Billy implements an interface based on the `os` standard library, allowing to develop applications without dependency on the underlying storage. Makes it virtually free to implement mocks and testing over filesystem operations.
-
-Billy was born as part of [go-git/go-git](https://github.com/go-git/go-git) project.
-
-## Installation
-
-```go
-import "github.com/go-git/go-billy/v5" // with go modules enabled (GO111MODULE=on or outside GOPATH)
-import "github.com/go-git/go-billy" // with go modules disabled
-```
-
-## Usage
-
-Billy exposes filesystems using the
-[`Filesystem` interface](https://pkg.go.dev/github.com/go-git/go-billy/v5?tab=doc#Filesystem).
-Each filesystem implementation gives you a `New` method, whose arguments depend on
-the implementation itself, that returns a new `Filesystem`.
-
-The following example caches in memory all readable files in a directory from any
-billy's filesystem implementation.
-
-```go
-func LoadToMemory(origin billy.Filesystem, path string) (*memory.Memory, error) {
- memory := memory.New()
-
- files, err := origin.ReadDir("/")
- if err != nil {
- return nil, err
- }
-
- for _, file := range files {
- if file.IsDir() {
- continue
- }
-
- src, err := origin.Open(file.Name())
- if err != nil {
- return nil, err
- }
-
- dst, err := memory.Create(file.Name())
- if err != nil {
- return nil, err
- }
-
- if _, err = io.Copy(dst, src); err != nil {
- return nil, err
- }
-
- if err := dst.Close(); err != nil {
- return nil, err
- }
-
- if err := src.Close(); err != nil {
- return nil, err
- }
- }
-
- return memory, nil
-}
-```
-
-## Why billy?
-
-The library billy deals with storage systems and Billy is the name of a well-known, IKEA
-bookcase. That's it.
-
-## License
-
-Apache License Version 2.0, see [LICENSE](LICENSE)
diff --git a/vendor/github.com/go-git/go-billy/v5/fs.go b/vendor/github.com/go-git/go-billy/v5/fs.go
deleted file mode 100644
index a9efccd..0000000
--- a/vendor/github.com/go-git/go-billy/v5/fs.go
+++ /dev/null
@@ -1,202 +0,0 @@
-package billy
-
-import (
- "errors"
- "io"
- "os"
- "time"
-)
-
-var (
- ErrReadOnly = errors.New("read-only filesystem")
- ErrNotSupported = errors.New("feature not supported")
- ErrCrossedBoundary = errors.New("chroot boundary crossed")
-)
-
-// Capability holds the supported features of a billy filesystem. This does
-// not mean that the capability has to be supported by the underlying storage.
-// For example, a billy filesystem may support WriteCapability but the
-// storage be mounted in read only mode.
-type Capability uint64
-
-const (
- // WriteCapability means that the fs is writable.
- WriteCapability Capability = 1 << iota
- // ReadCapability means that the fs is readable.
- ReadCapability
- // ReadAndWriteCapability is the ability to open a file in read and write mode.
- ReadAndWriteCapability
- // SeekCapability means it is able to move position inside the file.
- SeekCapability
- // TruncateCapability means that a file can be truncated.
- TruncateCapability
- // LockCapability is the ability to lock a file.
- LockCapability
-
- // DefaultCapabilities lists all capable features supported by filesystems
- // without Capability interface. This list should not be changed until a
- // major version is released.
- DefaultCapabilities Capability = WriteCapability | ReadCapability |
- ReadAndWriteCapability | SeekCapability | TruncateCapability |
- LockCapability
-
- // AllCapabilities lists all capable features.
- AllCapabilities Capability = WriteCapability | ReadCapability |
- ReadAndWriteCapability | SeekCapability | TruncateCapability |
- LockCapability
-)
-
-// Filesystem abstract the operations in a storage-agnostic interface.
-// Each method implementation mimics the behavior of the equivalent functions
-// at the os package from the standard library.
-type Filesystem interface {
- Basic
- TempFile
- Dir
- Symlink
- Chroot
-}
-
-// Basic abstract the basic operations in a storage-agnostic interface as
-// an extension to the Basic interface.
-type Basic interface {
- // Create creates the named file with mode 0666 (before umask), truncating
- // it if it already exists. If successful, methods on the returned File can
- // be used for I/O; the associated file descriptor has mode O_RDWR.
- Create(filename string) (File, error)
- // Open opens the named file for reading. If successful, methods on the
- // returned file can be used for reading; the associated file descriptor has
- // mode O_RDONLY.
- Open(filename string) (File, error)
- // OpenFile is the generalized open call; most users will use Open or Create
- // instead. It opens the named file with specified flag (O_RDONLY etc.) and
- // perm, (0666 etc.) if applicable. If successful, methods on the returned
- // File can be used for I/O.
- OpenFile(filename string, flag int, perm os.FileMode) (File, error)
- // Stat returns a FileInfo describing the named file.
- Stat(filename string) (os.FileInfo, error)
- // Rename renames (moves) oldpath to newpath. If newpath already exists and
- // is not a directory, Rename replaces it. OS-specific restrictions may
- // apply when oldpath and newpath are in different directories.
- Rename(oldpath, newpath string) error
- // Remove removes the named file or directory.
- Remove(filename string) error
- // Join joins any number of path elements into a single path, adding a
- // Separator if necessary. Join calls filepath.Clean on the result; in
- // particular, all empty strings are ignored. On Windows, the result is a
- // UNC path if and only if the first path element is a UNC path.
- Join(elem ...string) string
-}
-
-type TempFile interface {
- // TempFile creates a new temporary file in the directory dir with a name
- // beginning with prefix, opens the file for reading and writing, and
- // returns the resulting *os.File. If dir is the empty string, TempFile
- // uses the default directory for temporary files (see os.TempDir).
- // Multiple programs calling TempFile simultaneously will not choose the
- // same file. The caller can use f.Name() to find the pathname of the file.
- // It is the caller's responsibility to remove the file when no longer
- // needed.
- TempFile(dir, prefix string) (File, error)
-}
-
-// Dir abstract the dir related operations in a storage-agnostic interface as
-// an extension to the Basic interface.
-type Dir interface {
- // ReadDir reads the directory named by dirname and returns a list of
- // directory entries sorted by filename.
- ReadDir(path string) ([]os.FileInfo, error)
- // MkdirAll creates a directory named path, along with any necessary
- // parents, and returns nil, or else returns an error. The permission bits
- // perm are used for all directories that MkdirAll creates. If path is/
- // already a directory, MkdirAll does nothing and returns nil.
- MkdirAll(filename string, perm os.FileMode) error
-}
-
-// Symlink abstract the symlink related operations in a storage-agnostic
-// interface as an extension to the Basic interface.
-type Symlink interface {
- // Lstat returns a FileInfo describing the named file. If the file is a
- // symbolic link, the returned FileInfo describes the symbolic link. Lstat
- // makes no attempt to follow the link.
- Lstat(filename string) (os.FileInfo, error)
- // Symlink creates a symbolic-link from link to target. target may be an
- // absolute or relative path, and need not refer to an existing node.
- // Parent directories of link are created as necessary.
- Symlink(target, link string) error
- // Readlink returns the target path of link.
- Readlink(link string) (string, error)
-}
-
-// Change abstract the FileInfo change related operations in a storage-agnostic
-// interface as an extension to the Basic interface
-type Change interface {
- // Chmod changes the mode of the named file to mode. If the file is a
- // symbolic link, it changes the mode of the link's target.
- Chmod(name string, mode os.FileMode) error
- // Lchown changes the numeric uid and gid of the named file. If the file is
- // a symbolic link, it changes the uid and gid of the link itself.
- Lchown(name string, uid, gid int) error
- // Chown changes the numeric uid and gid of the named file. If the file is a
- // symbolic link, it changes the uid and gid of the link's target.
- Chown(name string, uid, gid int) error
- // Chtimes changes the access and modification times of the named file,
- // similar to the Unix utime() or utimes() functions.
- //
- // The underlying filesystem may truncate or round the values to a less
- // precise time unit.
- Chtimes(name string, atime time.Time, mtime time.Time) error
-}
-
-// Chroot abstract the chroot related operations in a storage-agnostic interface
-// as an extension to the Basic interface.
-type Chroot interface {
- // Chroot returns a new filesystem from the same type where the new root is
- // the given path. Files outside of the designated directory tree cannot be
- // accessed.
- Chroot(path string) (Filesystem, error)
- // Root returns the root path of the filesystem.
- Root() string
-}
-
-// File represent a file, being a subset of the os.File
-type File interface {
- // Name returns the name of the file as presented to Open.
- Name() string
- io.Writer
- io.Reader
- io.ReaderAt
- io.Seeker
- io.Closer
- // Lock locks the file like e.g. flock. It protects against access from
- // other processes.
- Lock() error
- // Unlock unlocks the file.
- Unlock() error
- // Truncate the file.
- Truncate(size int64) error
-}
-
-// Capable interface can return the available features of a filesystem.
-type Capable interface {
- // Capabilities returns the capabilities of a filesystem in bit flags.
- Capabilities() Capability
-}
-
-// Capabilities returns the features supported by a filesystem. If the FS
-// does not implement Capable interface it returns all features.
-func Capabilities(fs Basic) Capability {
- capable, ok := fs.(Capable)
- if !ok {
- return DefaultCapabilities
- }
-
- return capable.Capabilities()
-}
-
-// CapabilityCheck tests the filesystem for the provided capabilities and
-// returns true in case it supports all of them.
-func CapabilityCheck(fs Basic, capabilities Capability) bool {
- fsCaps := Capabilities(fs)
- return fsCaps&capabilities == capabilities
-}
diff --git a/vendor/github.com/go-git/go-billy/v5/go.mod b/vendor/github.com/go-git/go-billy/v5/go.mod
deleted file mode 100644
index 78ce0af..0000000
--- a/vendor/github.com/go-git/go-billy/v5/go.mod
+++ /dev/null
@@ -1,10 +0,0 @@
-module github.com/go-git/go-billy/v5
-
-require (
- github.com/kr/text v0.2.0 // indirect
- github.com/niemeyer/pretty v0.0.0-20200227124842-a10e7caefd8e // indirect
- golang.org/x/sys v0.0.0-20200302150141-5c8b2ff67527
- gopkg.in/check.v1 v1.0.0-20200227125254-8fa46927fb4f
-)
-
-go 1.13
diff --git a/vendor/github.com/go-git/go-billy/v5/go.sum b/vendor/github.com/go-git/go-billy/v5/go.sum
deleted file mode 100644
index cdc052b..0000000
--- a/vendor/github.com/go-git/go-billy/v5/go.sum
+++ /dev/null
@@ -1,14 +0,0 @@
-github.com/creack/pty v1.1.9/go.mod h1:oKZEueFk5CKHvIhNR5MUki03XCEU+Q6VDXinZuGJ33E=
-github.com/go-git/go-billy v1.0.0 h1:bXR6Zu3opPSg0R4dDxqaLglY4rxw7ja7wS16qSpOKL4=
-github.com/go-git/go-billy v3.1.0+incompatible h1:dwrJ8G2Jt1srYgIJs+lRjA36qBY68O2Lg5idKG8ef5M=
-github.com/kr/pty v1.1.1/go.mod h1:pFQYn66WHrOpPYNljwOMqo10TkYh1fy3cYio2l3bCsQ=
-github.com/kr/text v0.1.0 h1:45sCR5RtlFHMR4UwH9sdQ5TC8v0qDQCHnXt+kaKSTVE=
-github.com/kr/text v0.1.0/go.mod h1:4Jbv+DJW3UT/LiOwJeYQe1efqtUx/iVham/4vfdArNI=
-github.com/kr/text v0.2.0 h1:5Nx0Ya0ZqY2ygV366QzturHI13Jq95ApcVaJBhpS+AY=
-github.com/kr/text v0.2.0/go.mod h1:eLer722TekiGuMkidMxC/pM04lWEeraHUUmBw8l2grE=
-github.com/niemeyer/pretty v0.0.0-20200227124842-a10e7caefd8e h1:fD57ERR4JtEqsWbfPhv4DMiApHyliiK5xCTNVSPiaAs=
-github.com/niemeyer/pretty v0.0.0-20200227124842-a10e7caefd8e/go.mod h1:zD1mROLANZcx1PVRCS0qkT7pwLkGfwJo4zjcN/Tysno=
-golang.org/x/sys v0.0.0-20200302150141-5c8b2ff67527 h1:uYVVQ9WP/Ds2ROhcaGPeIdVq0RIXVLwsHlnvJ+cT1So=
-golang.org/x/sys v0.0.0-20200302150141-5c8b2ff67527/go.mod h1:h1NjWce9XRLGQEsW7wpKNCjG9DtNlClVuFLEZdDNbEs=
-gopkg.in/check.v1 v1.0.0-20200227125254-8fa46927fb4f h1:BLraFXnmrev5lT+xlilqcH8XK9/i0At2xKjWk4p6zsU=
-gopkg.in/check.v1 v1.0.0-20200227125254-8fa46927fb4f/go.mod h1:Co6ibVJAznAaIkqp8huTwlJQCZ016jof/cbN4VW5Yz0=
diff --git a/vendor/github.com/go-git/go-billy/v5/helper/chroot/chroot.go b/vendor/github.com/go-git/go-billy/v5/helper/chroot/chroot.go
deleted file mode 100644
index 8b44e78..0000000
--- a/vendor/github.com/go-git/go-billy/v5/helper/chroot/chroot.go
+++ /dev/null
@@ -1,242 +0,0 @@
-package chroot
-
-import (
- "os"
- "path/filepath"
- "strings"
-
- "github.com/go-git/go-billy/v5"
- "github.com/go-git/go-billy/v5/helper/polyfill"
-)
-
-// ChrootHelper is a helper to implement billy.Chroot.
-type ChrootHelper struct {
- underlying billy.Filesystem
- base string
-}
-
-// New creates a new filesystem wrapping up the given 'fs'.
-// The created filesystem has its base in the given ChrootHelperectory of the
-// underlying filesystem.
-func New(fs billy.Basic, base string) billy.Filesystem {
- return &ChrootHelper{
- underlying: polyfill.New(fs),
- base: base,
- }
-}
-
-func (fs *ChrootHelper) underlyingPath(filename string) (string, error) {
- if isCrossBoundaries(filename) {
- return "", billy.ErrCrossedBoundary
- }
-
- return fs.Join(fs.Root(), filename), nil
-}
-
-func isCrossBoundaries(path string) bool {
- path = filepath.ToSlash(path)
- path = filepath.Clean(path)
-
- return strings.HasPrefix(path, ".."+string(filepath.Separator))
-}
-
-func (fs *ChrootHelper) Create(filename string) (billy.File, error) {
- fullpath, err := fs.underlyingPath(filename)
- if err != nil {
- return nil, err
- }
-
- f, err := fs.underlying.Create(fullpath)
- if err != nil {
- return nil, err
- }
-
- return newFile(fs, f, filename), nil
-}
-
-func (fs *ChrootHelper) Open(filename string) (billy.File, error) {
- fullpath, err := fs.underlyingPath(filename)
- if err != nil {
- return nil, err
- }
-
- f, err := fs.underlying.Open(fullpath)
- if err != nil {
- return nil, err
- }
-
- return newFile(fs, f, filename), nil
-}
-
-func (fs *ChrootHelper) OpenFile(filename string, flag int, mode os.FileMode) (billy.File, error) {
- fullpath, err := fs.underlyingPath(filename)
- if err != nil {
- return nil, err
- }
-
- f, err := fs.underlying.OpenFile(fullpath, flag, mode)
- if err != nil {
- return nil, err
- }
-
- return newFile(fs, f, filename), nil
-}
-
-func (fs *ChrootHelper) Stat(filename string) (os.FileInfo, error) {
- fullpath, err := fs.underlyingPath(filename)
- if err != nil {
- return nil, err
- }
-
- return fs.underlying.Stat(fullpath)
-}
-
-func (fs *ChrootHelper) Rename(from, to string) error {
- var err error
- from, err = fs.underlyingPath(from)
- if err != nil {
- return err
- }
-
- to, err = fs.underlyingPath(to)
- if err != nil {
- return err
- }
-
- return fs.underlying.Rename(from, to)
-}
-
-func (fs *ChrootHelper) Remove(path string) error {
- fullpath, err := fs.underlyingPath(path)
- if err != nil {
- return err
- }
-
- return fs.underlying.Remove(fullpath)
-}
-
-func (fs *ChrootHelper) Join(elem ...string) string {
- return fs.underlying.Join(elem...)
-}
-
-func (fs *ChrootHelper) TempFile(dir, prefix string) (billy.File, error) {
- fullpath, err := fs.underlyingPath(dir)
- if err != nil {
- return nil, err
- }
-
- f, err := fs.underlying.(billy.TempFile).TempFile(fullpath, prefix)
- if err != nil {
- return nil, err
- }
-
- return newFile(fs, f, fs.Join(dir, filepath.Base(f.Name()))), nil
-}
-
-func (fs *ChrootHelper) ReadDir(path string) ([]os.FileInfo, error) {
- fullpath, err := fs.underlyingPath(path)
- if err != nil {
- return nil, err
- }
-
- return fs.underlying.(billy.Dir).ReadDir(fullpath)
-}
-
-func (fs *ChrootHelper) MkdirAll(filename string, perm os.FileMode) error {
- fullpath, err := fs.underlyingPath(filename)
- if err != nil {
- return err
- }
-
- return fs.underlying.(billy.Dir).MkdirAll(fullpath, perm)
-}
-
-func (fs *ChrootHelper) Lstat(filename string) (os.FileInfo, error) {
- fullpath, err := fs.underlyingPath(filename)
- if err != nil {
- return nil, err
- }
-
- return fs.underlying.(billy.Symlink).Lstat(fullpath)
-}
-
-func (fs *ChrootHelper) Symlink(target, link string) error {
- target = filepath.FromSlash(target)
-
- // only rewrite target if it's already absolute
- if filepath.IsAbs(target) || strings.HasPrefix(target, string(filepath.Separator)) {
- target = fs.Join(fs.Root(), target)
- target = filepath.Clean(filepath.FromSlash(target))
- }
-
- link, err := fs.underlyingPath(link)
- if err != nil {
- return err
- }
-
- return fs.underlying.(billy.Symlink).Symlink(target, link)
-}
-
-func (fs *ChrootHelper) Readlink(link string) (string, error) {
- fullpath, err := fs.underlyingPath(link)
- if err != nil {
- return "", err
- }
-
- target, err := fs.underlying.(billy.Symlink).Readlink(fullpath)
- if err != nil {
- return "", err
- }
-
- if !filepath.IsAbs(target) && !strings.HasPrefix(target, string(filepath.Separator)) {
- return target, nil
- }
-
- target, err = filepath.Rel(fs.base, target)
- if err != nil {
- return "", err
- }
-
- return string(os.PathSeparator) + target, nil
-}
-
-func (fs *ChrootHelper) Chroot(path string) (billy.Filesystem, error) {
- fullpath, err := fs.underlyingPath(path)
- if err != nil {
- return nil, err
- }
-
- return New(fs.underlying, fullpath), nil
-}
-
-func (fs *ChrootHelper) Root() string {
- return fs.base
-}
-
-func (fs *ChrootHelper) Underlying() billy.Basic {
- return fs.underlying
-}
-
-// Capabilities implements the Capable interface.
-func (fs *ChrootHelper) Capabilities() billy.Capability {
- return billy.Capabilities(fs.underlying)
-}
-
-type file struct {
- billy.File
- name string
-}
-
-func newFile(fs billy.Filesystem, f billy.File, filename string) billy.File {
- filename = fs.Join(fs.Root(), filename)
- filename, _ = filepath.Rel(fs.Root(), filename)
-
- return &file{
- File: f,
- name: filename,
- }
-}
-
-func (f *file) Name() string {
- return f.name
-}
diff --git a/vendor/github.com/go-git/go-billy/v5/helper/polyfill/polyfill.go b/vendor/github.com/go-git/go-billy/v5/helper/polyfill/polyfill.go
deleted file mode 100644
index 1efce0e..0000000
--- a/vendor/github.com/go-git/go-billy/v5/helper/polyfill/polyfill.go
+++ /dev/null
@@ -1,105 +0,0 @@
-package polyfill
-
-import (
- "os"
- "path/filepath"
-
- "github.com/go-git/go-billy/v5"
-)
-
-// Polyfill is a helper that implements all missing method from billy.Filesystem.
-type Polyfill struct {
- billy.Basic
- c capabilities
-}
-
-type capabilities struct{ tempfile, dir, symlink, chroot bool }
-
-// New creates a new filesystem wrapping up 'fs' the intercepts all the calls
-// made and errors if fs doesn't implement any of the billy interfaces.
-func New(fs billy.Basic) billy.Filesystem {
- if original, ok := fs.(billy.Filesystem); ok {
- return original
- }
-
- h := &Polyfill{Basic: fs}
-
- _, h.c.tempfile = h.Basic.(billy.TempFile)
- _, h.c.dir = h.Basic.(billy.Dir)
- _, h.c.symlink = h.Basic.(billy.Symlink)
- _, h.c.chroot = h.Basic.(billy.Chroot)
- return h
-}
-
-func (h *Polyfill) TempFile(dir, prefix string) (billy.File, error) {
- if !h.c.tempfile {
- return nil, billy.ErrNotSupported
- }
-
- return h.Basic.(billy.TempFile).TempFile(dir, prefix)
-}
-
-func (h *Polyfill) ReadDir(path string) ([]os.FileInfo, error) {
- if !h.c.dir {
- return nil, billy.ErrNotSupported
- }
-
- return h.Basic.(billy.Dir).ReadDir(path)
-}
-
-func (h *Polyfill) MkdirAll(filename string, perm os.FileMode) error {
- if !h.c.dir {
- return billy.ErrNotSupported
- }
-
- return h.Basic.(billy.Dir).MkdirAll(filename, perm)
-}
-
-func (h *Polyfill) Symlink(target, link string) error {
- if !h.c.symlink {
- return billy.ErrNotSupported
- }
-
- return h.Basic.(billy.Symlink).Symlink(target, link)
-}
-
-func (h *Polyfill) Readlink(link string) (string, error) {
- if !h.c.symlink {
- return "", billy.ErrNotSupported
- }
-
- return h.Basic.(billy.Symlink).Readlink(link)
-}
-
-func (h *Polyfill) Lstat(path string) (os.FileInfo, error) {
- if !h.c.symlink {
- return nil, billy.ErrNotSupported
- }
-
- return h.Basic.(billy.Symlink).Lstat(path)
-}
-
-func (h *Polyfill) Chroot(path string) (billy.Filesystem, error) {
- if !h.c.chroot {
- return nil, billy.ErrNotSupported
- }
-
- return h.Basic.(billy.Chroot).Chroot(path)
-}
-
-func (h *Polyfill) Root() string {
- if !h.c.chroot {
- return string(filepath.Separator)
- }
-
- return h.Basic.(billy.Chroot).Root()
-}
-
-func (h *Polyfill) Underlying() billy.Basic {
- return h.Basic
-}
-
-// Capabilities implements the Capable interface.
-func (h *Polyfill) Capabilities() billy.Capability {
- return billy.Capabilities(h.Basic)
-}
diff --git a/vendor/github.com/go-git/go-billy/v5/memfs/memory.go b/vendor/github.com/go-git/go-billy/v5/memfs/memory.go
deleted file mode 100644
index f217693..0000000
--- a/vendor/github.com/go-git/go-billy/v5/memfs/memory.go
+++ /dev/null
@@ -1,410 +0,0 @@
-// Package memfs provides a billy filesystem base on memory.
-package memfs // import "github.com/go-git/go-billy/v5/memfs"
-
-import (
- "errors"
- "fmt"
- "io"
- "os"
- "path/filepath"
- "sort"
- "strings"
- "time"
-
- "github.com/go-git/go-billy/v5"
- "github.com/go-git/go-billy/v5/helper/chroot"
- "github.com/go-git/go-billy/v5/util"
-)
-
-const separator = filepath.Separator
-
-// Memory a very convenient filesystem based on memory files
-type Memory struct {
- s *storage
-
- tempCount int
-}
-
-//New returns a new Memory filesystem.
-func New() billy.Filesystem {
- fs := &Memory{s: newStorage()}
- return chroot.New(fs, string(separator))
-}
-
-func (fs *Memory) Create(filename string) (billy.File, error) {
- return fs.OpenFile(filename, os.O_RDWR|os.O_CREATE|os.O_TRUNC, 0666)
-}
-
-func (fs *Memory) Open(filename string) (billy.File, error) {
- return fs.OpenFile(filename, os.O_RDONLY, 0)
-}
-
-func (fs *Memory) OpenFile(filename string, flag int, perm os.FileMode) (billy.File, error) {
- f, has := fs.s.Get(filename)
- if !has {
- if !isCreate(flag) {
- return nil, os.ErrNotExist
- }
-
- var err error
- f, err = fs.s.New(filename, perm, flag)
- if err != nil {
- return nil, err
- }
- } else {
- if isExclusive(flag) {
- return nil, os.ErrExist
- }
-
- if target, isLink := fs.resolveLink(filename, f); isLink {
- return fs.OpenFile(target, flag, perm)
- }
- }
-
- if f.mode.IsDir() {
- return nil, fmt.Errorf("cannot open directory: %s", filename)
- }
-
- return f.Duplicate(filename, perm, flag), nil
-}
-
-var errNotLink = errors.New("not a link")
-
-func (fs *Memory) resolveLink(fullpath string, f *file) (target string, isLink bool) {
- if !isSymlink(f.mode) {
- return fullpath, false
- }
-
- target = string(f.content.bytes)
- if !isAbs(target) {
- target = fs.Join(filepath.Dir(fullpath), target)
- }
-
- return target, true
-}
-
-// On Windows OS, IsAbs validates if a path is valid based on if stars with a
-// unit (eg.: `C:\`) to assert that is absolute, but in this mem implementation
-// any path starting by `separator` is also considered absolute.
-func isAbs(path string) bool {
- return filepath.IsAbs(path) || strings.HasPrefix(path, string(separator))
-}
-
-func (fs *Memory) Stat(filename string) (os.FileInfo, error) {
- f, has := fs.s.Get(filename)
- if !has {
- return nil, os.ErrNotExist
- }
-
- fi, _ := f.Stat()
-
- var err error
- if target, isLink := fs.resolveLink(filename, f); isLink {
- fi, err = fs.Stat(target)
- if err != nil {
- return nil, err
- }
- }
-
- // the name of the file should always the name of the stated file, so we
- // overwrite the Stat returned from the storage with it, since the
- // filename may belong to a link.
- fi.(*fileInfo).name = filepath.Base(filename)
- return fi, nil
-}
-
-func (fs *Memory) Lstat(filename string) (os.FileInfo, error) {
- f, has := fs.s.Get(filename)
- if !has {
- return nil, os.ErrNotExist
- }
-
- return f.Stat()
-}
-
-type ByName []os.FileInfo
-
-func (a ByName) Len() int { return len(a) }
-func (a ByName) Less(i, j int) bool { return a[i].Name() < a[j].Name() }
-func (a ByName) Swap(i, j int) { a[i], a[j] = a[j], a[i] }
-
-func (fs *Memory) ReadDir(path string) ([]os.FileInfo, error) {
- if f, has := fs.s.Get(path); has {
- if target, isLink := fs.resolveLink(path, f); isLink {
- return fs.ReadDir(target)
- }
- }
-
- var entries []os.FileInfo
- for _, f := range fs.s.Children(path) {
- fi, _ := f.Stat()
- entries = append(entries, fi)
- }
-
- sort.Sort(ByName(entries))
-
- return entries, nil
-}
-
-func (fs *Memory) MkdirAll(path string, perm os.FileMode) error {
- _, err := fs.s.New(path, perm|os.ModeDir, 0)
- return err
-}
-
-func (fs *Memory) TempFile(dir, prefix string) (billy.File, error) {
- return util.TempFile(fs, dir, prefix)
-}
-
-func (fs *Memory) getTempFilename(dir, prefix string) string {
- fs.tempCount++
- filename := fmt.Sprintf("%s_%d_%d", prefix, fs.tempCount, time.Now().UnixNano())
- return fs.Join(dir, filename)
-}
-
-func (fs *Memory) Rename(from, to string) error {
- return fs.s.Rename(from, to)
-}
-
-func (fs *Memory) Remove(filename string) error {
- return fs.s.Remove(filename)
-}
-
-func (fs *Memory) Join(elem ...string) string {
- return filepath.Join(elem...)
-}
-
-func (fs *Memory) Symlink(target, link string) error {
- _, err := fs.Stat(link)
- if err == nil {
- return os.ErrExist
- }
-
- if !os.IsNotExist(err) {
- return err
- }
-
- return util.WriteFile(fs, link, []byte(target), 0777|os.ModeSymlink)
-}
-
-func (fs *Memory) Readlink(link string) (string, error) {
- f, has := fs.s.Get(link)
- if !has {
- return "", os.ErrNotExist
- }
-
- if !isSymlink(f.mode) {
- return "", &os.PathError{
- Op: "readlink",
- Path: link,
- Err: fmt.Errorf("not a symlink"),
- }
- }
-
- return string(f.content.bytes), nil
-}
-
-// Capabilities implements the Capable interface.
-func (fs *Memory) Capabilities() billy.Capability {
- return billy.WriteCapability |
- billy.ReadCapability |
- billy.ReadAndWriteCapability |
- billy.SeekCapability |
- billy.TruncateCapability
-}
-
-type file struct {
- name string
- content *content
- position int64
- flag int
- mode os.FileMode
-
- isClosed bool
-}
-
-func (f *file) Name() string {
- return f.name
-}
-
-func (f *file) Read(b []byte) (int, error) {
- n, err := f.ReadAt(b, f.position)
- f.position += int64(n)
-
- if err == io.EOF && n != 0 {
- err = nil
- }
-
- return n, err
-}
-
-func (f *file) ReadAt(b []byte, off int64) (int, error) {
- if f.isClosed {
- return 0, os.ErrClosed
- }
-
- if !isReadAndWrite(f.flag) && !isReadOnly(f.flag) {
- return 0, errors.New("read not supported")
- }
-
- n, err := f.content.ReadAt(b, off)
-
- return n, err
-}
-
-func (f *file) Seek(offset int64, whence int) (int64, error) {
- if f.isClosed {
- return 0, os.ErrClosed
- }
-
- switch whence {
- case io.SeekCurrent:
- f.position += offset
- case io.SeekStart:
- f.position = offset
- case io.SeekEnd:
- f.position = int64(f.content.Len()) + offset
- }
-
- return f.position, nil
-}
-
-func (f *file) Write(p []byte) (int, error) {
- if f.isClosed {
- return 0, os.ErrClosed
- }
-
- if !isReadAndWrite(f.flag) && !isWriteOnly(f.flag) {
- return 0, errors.New("write not supported")
- }
-
- n, err := f.content.WriteAt(p, f.position)
- f.position += int64(n)
-
- return n, err
-}
-
-func (f *file) Close() error {
- if f.isClosed {
- return os.ErrClosed
- }
-
- f.isClosed = true
- return nil
-}
-
-func (f *file) Truncate(size int64) error {
- if size < int64(len(f.content.bytes)) {
- f.content.bytes = f.content.bytes[:size]
- } else if more := int(size) - len(f.content.bytes); more > 0 {
- f.content.bytes = append(f.content.bytes, make([]byte, more)...)
- }
-
- return nil
-}
-
-func (f *file) Duplicate(filename string, mode os.FileMode, flag int) billy.File {
- new := &file{
- name: filename,
- content: f.content,
- mode: mode,
- flag: flag,
- }
-
- if isAppend(flag) {
- new.position = int64(new.content.Len())
- }
-
- if isTruncate(flag) {
- new.content.Truncate()
- }
-
- return new
-}
-
-func (f *file) Stat() (os.FileInfo, error) {
- return &fileInfo{
- name: f.Name(),
- mode: f.mode,
- size: f.content.Len(),
- }, nil
-}
-
-// Lock is a no-op in memfs.
-func (f *file) Lock() error {
- return nil
-}
-
-// Unlock is a no-op in memfs.
-func (f *file) Unlock() error {
- return nil
-}
-
-type fileInfo struct {
- name string
- size int
- mode os.FileMode
-}
-
-func (fi *fileInfo) Name() string {
- return fi.name
-}
-
-func (fi *fileInfo) Size() int64 {
- return int64(fi.size)
-}
-
-func (fi *fileInfo) Mode() os.FileMode {
- return fi.mode
-}
-
-func (*fileInfo) ModTime() time.Time {
- return time.Now()
-}
-
-func (fi *fileInfo) IsDir() bool {
- return fi.mode.IsDir()
-}
-
-func (*fileInfo) Sys() interface{} {
- return nil
-}
-
-func (c *content) Truncate() {
- c.bytes = make([]byte, 0)
-}
-
-func (c *content) Len() int {
- return len(c.bytes)
-}
-
-func isCreate(flag int) bool {
- return flag&os.O_CREATE != 0
-}
-
-func isExclusive(flag int) bool {
- return flag&os.O_EXCL != 0
-}
-
-func isAppend(flag int) bool {
- return flag&os.O_APPEND != 0
-}
-
-func isTruncate(flag int) bool {
- return flag&os.O_TRUNC != 0
-}
-
-func isReadAndWrite(flag int) bool {
- return flag&os.O_RDWR != 0
-}
-
-func isReadOnly(flag int) bool {
- return flag == os.O_RDONLY
-}
-
-func isWriteOnly(flag int) bool {
- return flag&os.O_WRONLY != 0
-}
-
-func isSymlink(m os.FileMode) bool {
- return m&os.ModeSymlink != 0
-}
diff --git a/vendor/github.com/go-git/go-billy/v5/memfs/storage.go b/vendor/github.com/go-git/go-billy/v5/memfs/storage.go
deleted file mode 100644
index d3ff5a2..0000000
--- a/vendor/github.com/go-git/go-billy/v5/memfs/storage.go
+++ /dev/null
@@ -1,229 +0,0 @@
-package memfs
-
-import (
- "errors"
- "fmt"
- "io"
- "os"
- "path/filepath"
-)
-
-type storage struct {
- files map[string]*file
- children map[string]map[string]*file
-}
-
-func newStorage() *storage {
- return &storage{
- files: make(map[string]*file, 0),
- children: make(map[string]map[string]*file, 0),
- }
-}
-
-func (s *storage) Has(path string) bool {
- path = clean(path)
-
- _, ok := s.files[path]
- return ok
-}
-
-func (s *storage) New(path string, mode os.FileMode, flag int) (*file, error) {
- path = clean(path)
- if s.Has(path) {
- if !s.MustGet(path).mode.IsDir() {
- return nil, fmt.Errorf("file already exists %q", path)
- }
-
- return nil, nil
- }
-
- name := filepath.Base(path)
-
- f := &file{
- name: name,
- content: &content{name: name},
- mode: mode,
- flag: flag,
- }
-
- s.files[path] = f
- s.createParent(path, mode, f)
- return f, nil
-}
-
-func (s *storage) createParent(path string, mode os.FileMode, f *file) error {
- base := filepath.Dir(path)
- base = clean(base)
- if f.Name() == string(separator) {
- return nil
- }
-
- if _, err := s.New(base, mode.Perm()|os.ModeDir, 0); err != nil {
- return err
- }
-
- if _, ok := s.children[base]; !ok {
- s.children[base] = make(map[string]*file, 0)
- }
-
- s.children[base][f.Name()] = f
- return nil
-}
-
-func (s *storage) Children(path string) []*file {
- path = clean(path)
-
- l := make([]*file, 0)
- for _, f := range s.children[path] {
- l = append(l, f)
- }
-
- return l
-}
-
-func (s *storage) MustGet(path string) *file {
- f, ok := s.Get(path)
- if !ok {
- panic(fmt.Errorf("couldn't find %q", path))
- }
-
- return f
-}
-
-func (s *storage) Get(path string) (*file, bool) {
- path = clean(path)
- if !s.Has(path) {
- return nil, false
- }
-
- file, ok := s.files[path]
- return file, ok
-}
-
-func (s *storage) Rename(from, to string) error {
- from = clean(from)
- to = clean(to)
-
- if !s.Has(from) {
- return os.ErrNotExist
- }
-
- move := [][2]string{{from, to}}
-
- for pathFrom := range s.files {
- if pathFrom == from || !filepath.HasPrefix(pathFrom, from) {
- continue
- }
-
- rel, _ := filepath.Rel(from, pathFrom)
- pathTo := filepath.Join(to, rel)
-
- move = append(move, [2]string{pathFrom, pathTo})
- }
-
- for _, ops := range move {
- from := ops[0]
- to := ops[1]
-
- if err := s.move(from, to); err != nil {
- return err
- }
- }
-
- return nil
-}
-
-func (s *storage) move(from, to string) error {
- s.files[to] = s.files[from]
- s.files[to].name = filepath.Base(to)
- s.children[to] = s.children[from]
-
- defer func() {
- delete(s.children, from)
- delete(s.files, from)
- delete(s.children[filepath.Dir(from)], filepath.Base(from))
- }()
-
- return s.createParent(to, 0644, s.files[to])
-}
-
-func (s *storage) Remove(path string) error {
- path = clean(path)
-
- f, has := s.Get(path)
- if !has {
- return os.ErrNotExist
- }
-
- if f.mode.IsDir() && len(s.children[path]) != 0 {
- return fmt.Errorf("dir: %s contains files", path)
- }
-
- base, file := filepath.Split(path)
- base = filepath.Clean(base)
-
- delete(s.children[base], file)
- delete(s.files, path)
- return nil
-}
-
-func clean(path string) string {
- return filepath.Clean(filepath.FromSlash(path))
-}
-
-type content struct {
- name string
- bytes []byte
-}
-
-func (c *content) WriteAt(p []byte, off int64) (int, error) {
- if off < 0 {
- return 0, &os.PathError{
- Op: "writeat",
- Path: c.name,
- Err: errors.New("negative offset"),
- }
- }
-
- prev := len(c.bytes)
-
- diff := int(off) - prev
- if diff > 0 {
- c.bytes = append(c.bytes, make([]byte, diff)...)
- }
-
- c.bytes = append(c.bytes[:off], p...)
- if len(c.bytes) < prev {
- c.bytes = c.bytes[:prev]
- }
-
- return len(p), nil
-}
-
-func (c *content) ReadAt(b []byte, off int64) (n int, err error) {
- if off < 0 {
- return 0, &os.PathError{
- Op: "readat",
- Path: c.name,
- Err: errors.New("negative offset"),
- }
- }
-
- size := int64(len(c.bytes))
- if off >= size {
- return 0, io.EOF
- }
-
- l := int64(len(b))
- if off+l > size {
- l = size - off
- }
-
- btr := c.bytes[off : off+l]
- if len(btr) < len(b) {
- err = io.EOF
- }
- n = copy(b, btr)
-
- return
-}
diff --git a/vendor/github.com/go-git/go-billy/v5/osfs/os.go b/vendor/github.com/go-git/go-billy/v5/osfs/os.go
deleted file mode 100644
index 9665d27..0000000
--- a/vendor/github.com/go-git/go-billy/v5/osfs/os.go
+++ /dev/null
@@ -1,144 +0,0 @@
-// +build !js
-
-// Package osfs provides a billy filesystem for the OS.
-package osfs // import "github.com/go-git/go-billy/v5/osfs"
-
-import (
- "io/ioutil"
- "os"
- "path/filepath"
- "sync"
-
- "github.com/go-git/go-billy/v5"
- "github.com/go-git/go-billy/v5/helper/chroot"
-)
-
-const (
- defaultDirectoryMode = 0755
- defaultCreateMode = 0666
-)
-
-// Default Filesystem representing the root of the os filesystem.
-var Default = &OS{}
-
-// OS is a filesystem based on the os filesystem.
-type OS struct{}
-
-// New returns a new OS filesystem.
-func New(baseDir string) billy.Filesystem {
- return chroot.New(Default, baseDir)
-}
-
-func (fs *OS) Create(filename string) (billy.File, error) {
- return fs.OpenFile(filename, os.O_RDWR|os.O_CREATE|os.O_TRUNC, defaultCreateMode)
-}
-
-func (fs *OS) OpenFile(filename string, flag int, perm os.FileMode) (billy.File, error) {
- if flag&os.O_CREATE != 0 {
- if err := fs.createDir(filename); err != nil {
- return nil, err
- }
- }
-
- f, err := os.OpenFile(filename, flag, perm)
- if err != nil {
- return nil, err
- }
- return &file{File: f}, err
-}
-
-func (fs *OS) createDir(fullpath string) error {
- dir := filepath.Dir(fullpath)
- if dir != "." {
- if err := os.MkdirAll(dir, defaultDirectoryMode); err != nil {
- return err
- }
- }
-
- return nil
-}
-
-func (fs *OS) ReadDir(path string) ([]os.FileInfo, error) {
- l, err := ioutil.ReadDir(path)
- if err != nil {
- return nil, err
- }
-
- var s = make([]os.FileInfo, len(l))
- for i, f := range l {
- s[i] = f
- }
-
- return s, nil
-}
-
-func (fs *OS) Rename(from, to string) error {
- if err := fs.createDir(to); err != nil {
- return err
- }
-
- return rename(from, to)
-}
-
-func (fs *OS) MkdirAll(path string, perm os.FileMode) error {
- return os.MkdirAll(path, defaultDirectoryMode)
-}
-
-func (fs *OS) Open(filename string) (billy.File, error) {
- return fs.OpenFile(filename, os.O_RDONLY, 0)
-}
-
-func (fs *OS) Stat(filename string) (os.FileInfo, error) {
- return os.Stat(filename)
-}
-
-func (fs *OS) Remove(filename string) error {
- return os.Remove(filename)
-}
-
-func (fs *OS) TempFile(dir, prefix string) (billy.File, error) {
- if err := fs.createDir(dir + string(os.PathSeparator)); err != nil {
- return nil, err
- }
-
- f, err := ioutil.TempFile(dir, prefix)
- if err != nil {
- return nil, err
- }
- return &file{File: f}, nil
-}
-
-func (fs *OS) Join(elem ...string) string {
- return filepath.Join(elem...)
-}
-
-func (fs *OS) RemoveAll(path string) error {
- return os.RemoveAll(filepath.Clean(path))
-}
-
-func (fs *OS) Lstat(filename string) (os.FileInfo, error) {
- return os.Lstat(filepath.Clean(filename))
-}
-
-func (fs *OS) Symlink(target, link string) error {
- if err := fs.createDir(link); err != nil {
- return err
- }
-
- return os.Symlink(target, link)
-}
-
-func (fs *OS) Readlink(link string) (string, error) {
- return os.Readlink(link)
-}
-
-// Capabilities implements the Capable interface.
-func (fs *OS) Capabilities() billy.Capability {
- return billy.DefaultCapabilities
-}
-
-// file is a wrapper for an os.File which adds support for file locking.
-type file struct {
- *os.File
- m sync.Mutex
-}
diff --git a/vendor/github.com/go-git/go-billy/v5/osfs/os_js.go b/vendor/github.com/go-git/go-billy/v5/osfs/os_js.go
deleted file mode 100644
index 8ae68fe..0000000
--- a/vendor/github.com/go-git/go-billy/v5/osfs/os_js.go
+++ /dev/null
@@ -1,21 +0,0 @@
-// +build js
-
-package osfs
-
-import (
- "github.com/go-git/go-billy/v5"
- "github.com/go-git/go-billy/v5/helper/chroot"
- "github.com/go-git/go-billy/v5/memfs"
-)
-
-// globalMemFs is the global memory fs
-var globalMemFs = memfs.New()
-
-// Default Filesystem representing the root of in-memory filesystem for a
-// js/wasm environment.
-var Default = memfs.New()
-
-// New returns a new OS filesystem.
-func New(baseDir string) billy.Filesystem {
- return chroot.New(Default, Default.Join("/", baseDir))
-}
diff --git a/vendor/github.com/go-git/go-billy/v5/osfs/os_plan9.go b/vendor/github.com/go-git/go-billy/v5/osfs/os_plan9.go
deleted file mode 100644
index e8f519f..0000000
--- a/vendor/github.com/go-git/go-billy/v5/osfs/os_plan9.go
+++ /dev/null
@@ -1,85 +0,0 @@
-// +build plan9
-
-package osfs
-
-import (
- "io"
- "os"
- "path/filepath"
- "syscall"
-)
-
-func (f *file) Lock() error {
- // Plan 9 uses a mode bit instead of explicit lock/unlock syscalls.
- //
- // Per http://man.cat-v.org/plan_9/5/stat: “Exclusive use files may be open
- // for I/O by only one fid at a time across all clients of the server. If a
- // second open is attempted, it draws an error.”
- //
- // There is no obvious way to implement this function using the exclusive use bit.
- // See https://golang.org/src/cmd/go/internal/lockedfile/lockedfile_plan9.go
- // for how file locking is done by the go tool on Plan 9.
- return nil
-}
-
-func (f *file) Unlock() error {
- return nil
-}
-
-func rename(from, to string) error {
- // If from and to are in different directories, copy the file
- // since Plan 9 does not support cross-directory rename.
- if filepath.Dir(from) != filepath.Dir(to) {
- fi, err := os.Stat(from)
- if err != nil {
- return &os.LinkError{"rename", from, to, err}
- }
- if fi.Mode().IsDir() {
- return &os.LinkError{"rename", from, to, syscall.EISDIR}
- }
- fromFile, err := os.Open(from)
- if err != nil {
- return &os.LinkError{"rename", from, to, err}
- }
- toFile, err := os.OpenFile(to, os.O_WRONLY|os.O_CREATE|os.O_TRUNC, fi.Mode())
- if err != nil {
- return &os.LinkError{"rename", from, to, err}
- }
- _, err = io.Copy(toFile, fromFile)
- if err != nil {
- return &os.LinkError{"rename", from, to, err}
- }
-
- // Copy mtime and mode from original file.
- // We need only one syscall if we avoid os.Chmod and os.Chtimes.
- dir := fi.Sys().(*syscall.Dir)
- var d syscall.Dir
- d.Null()
- d.Mtime = dir.Mtime
- d.Mode = dir.Mode
- if err = dirwstat(to, &d); err != nil {
- return &os.LinkError{"rename", from, to, err}
- }
-
- // Remove original file.
- err = os.Remove(from)
- if err != nil {
- return &os.LinkError{"rename", from, to, err}
- }
- return nil
- }
- return os.Rename(from, to)
-}
-
-func dirwstat(name string, d *syscall.Dir) error {
- var buf [syscall.STATFIXLEN]byte
-
- n, err := d.Marshal(buf[:])
- if err != nil {
- return &os.PathError{"dirwstat", name, err}
- }
- if err = syscall.Wstat(name, buf[:n]); err != nil {
- return &os.PathError{"dirwstat", name, err}
- }
- return nil
-}
diff --git a/vendor/github.com/go-git/go-billy/v5/osfs/os_posix.go b/vendor/github.com/go-git/go-billy/v5/osfs/os_posix.go
deleted file mode 100644
index c74d60e..0000000
--- a/vendor/github.com/go-git/go-billy/v5/osfs/os_posix.go
+++ /dev/null
@@ -1,27 +0,0 @@
-// +build !plan9,!windows,!js
-
-package osfs
-
-import (
- "os"
-
- "golang.org/x/sys/unix"
-)
-
-func (f *file) Lock() error {
- f.m.Lock()
- defer f.m.Unlock()
-
- return unix.Flock(int(f.File.Fd()), unix.LOCK_EX)
-}
-
-func (f *file) Unlock() error {
- f.m.Lock()
- defer f.m.Unlock()
-
- return unix.Flock(int(f.File.Fd()), unix.LOCK_UN)
-}
-
-func rename(from, to string) error {
- return os.Rename(from, to)
-}
diff --git a/vendor/github.com/go-git/go-billy/v5/osfs/os_windows.go b/vendor/github.com/go-git/go-billy/v5/osfs/os_windows.go
deleted file mode 100644
index 8f5caeb..0000000
--- a/vendor/github.com/go-git/go-billy/v5/osfs/os_windows.go
+++ /dev/null
@@ -1,61 +0,0 @@
-// +build windows
-
-package osfs
-
-import (
- "os"
- "runtime"
- "unsafe"
-
- "golang.org/x/sys/windows"
-)
-
-type fileInfo struct {
- os.FileInfo
- name string
-}
-
-func (fi *fileInfo) Name() string {
- return fi.name
-}
-
-var (
- kernel32DLL = windows.NewLazySystemDLL("kernel32.dll")
- lockFileExProc = kernel32DLL.NewProc("LockFileEx")
- unlockFileProc = kernel32DLL.NewProc("UnlockFile")
-)
-
-const (
- lockfileExclusiveLock = 0x2
-)
-
-func (f *file) Lock() error {
- f.m.Lock()
- defer f.m.Unlock()
-
- var overlapped windows.Overlapped
- // err is always non-nil as per sys/windows semantics.
- ret, _, err := lockFileExProc.Call(f.File.Fd(), lockfileExclusiveLock, 0, 0xFFFFFFFF, 0,
- uintptr(unsafe.Pointer(&overlapped)))
- runtime.KeepAlive(&overlapped)
- if ret == 0 {
- return err
- }
- return nil
-}
-
-func (f *file) Unlock() error {
- f.m.Lock()
- defer f.m.Unlock()
-
- // err is always non-nil as per sys/windows semantics.
- ret, _, err := unlockFileProc.Call(f.File.Fd(), 0, 0, 0xFFFFFFFF, 0)
- if ret == 0 {
- return err
- }
- return nil
-}
-
-func rename(from, to string) error {
- return os.Rename(from, to)
-}
diff --git a/vendor/github.com/go-git/go-billy/v5/util/glob.go b/vendor/github.com/go-git/go-billy/v5/util/glob.go
deleted file mode 100644
index f7cb1de..0000000
--- a/vendor/github.com/go-git/go-billy/v5/util/glob.go
+++ /dev/null
@@ -1,111 +0,0 @@
-package util
-
-import (
- "path/filepath"
- "sort"
- "strings"
-
- "github.com/go-git/go-billy/v5"
-)
-
-// Glob returns the names of all files matching pattern or nil
-// if there is no matching file. The syntax of patterns is the same
-// as in Match. The pattern may describe hierarchical names such as
-// /usr/*/bin/ed (assuming the Separator is '/').
-//
-// Glob ignores file system errors such as I/O errors reading directories.
-// The only possible returned error is ErrBadPattern, when pattern
-// is malformed.
-//
-// Function originally from https://golang.org/src/path/filepath/match_test.go
-func Glob(fs billy.Filesystem, pattern string) (matches []string, err error) {
- if !hasMeta(pattern) {
- if _, err = fs.Lstat(pattern); err != nil {
- return nil, nil
- }
- return []string{pattern}, nil
- }
-
- dir, file := filepath.Split(pattern)
- // Prevent infinite recursion. See issue 15879.
- if dir == pattern {
- return nil, filepath.ErrBadPattern
- }
-
- var m []string
- m, err = Glob(fs, cleanGlobPath(dir))
- if err != nil {
- return
- }
- for _, d := range m {
- matches, err = glob(fs, d, file, matches)
- if err != nil {
- return
- }
- }
- return
-}
-
-// cleanGlobPath prepares path for glob matching.
-func cleanGlobPath(path string) string {
- switch path {
- case "":
- return "."
- case string(filepath.Separator):
- // do nothing to the path
- return path
- default:
- return path[0 : len(path)-1] // chop off trailing separator
- }
-}
-
-// glob searches for files matching pattern in the directory dir
-// and appends them to matches. If the directory cannot be
-// opened, it returns the existing matches. New matches are
-// added in lexicographical order.
-func glob(fs billy.Filesystem, dir, pattern string, matches []string) (m []string, e error) {
- m = matches
- fi, err := fs.Stat(dir)
- if err != nil {
- return
- }
-
- if !fi.IsDir() {
- return
- }
-
- names, _ := readdirnames(fs, dir)
- sort.Strings(names)
-
- for _, n := range names {
- matched, err := filepath.Match(pattern, n)
- if err != nil {
- return m, err
- }
- if matched {
- m = append(m, filepath.Join(dir, n))
- }
- }
- return
-}
-
-// hasMeta reports whether path contains any of the magic characters
-// recognized by Match.
-func hasMeta(path string) bool {
- // TODO(niemeyer): Should other magic characters be added here?
- return strings.ContainsAny(path, "*?[")
-}
-
-func readdirnames(fs billy.Filesystem, dir string) ([]string, error) {
- files, err := fs.ReadDir(dir)
- if err != nil {
- return nil, err
- }
-
- var names []string
- for _, file := range files {
- names = append(names, file.Name())
- }
-
- return names, nil
-}
diff --git a/vendor/github.com/go-git/go-billy/v5/util/util.go b/vendor/github.com/go-git/go-billy/v5/util/util.go
deleted file mode 100644
index 5c77128..0000000
--- a/vendor/github.com/go-git/go-billy/v5/util/util.go
+++ /dev/null
@@ -1,282 +0,0 @@
-package util
-
-import (
- "io"
- "os"
- "path/filepath"
- "strconv"
- "sync"
- "time"
-
- "github.com/go-git/go-billy/v5"
-)
-
-// RemoveAll removes path and any children it contains. It removes everything it
-// can but returns the first error it encounters. If the path does not exist,
-// RemoveAll returns nil (no error).
-func RemoveAll(fs billy.Basic, path string) error {
- fs, path = getUnderlyingAndPath(fs, path)
-
- if r, ok := fs.(removerAll); ok {
- return r.RemoveAll(path)
- }
-
- return removeAll(fs, path)
-}
-
-type removerAll interface {
- RemoveAll(string) error
-}
-
-func removeAll(fs billy.Basic, path string) error {
- // This implementation is adapted from os.RemoveAll.
-
- // Simple case: if Remove works, we're done.
- err := fs.Remove(path)
- if err == nil || os.IsNotExist(err) {
- return nil
- }
-
- // Otherwise, is this a directory we need to recurse into?
- dir, serr := fs.Stat(path)
- if serr != nil {
- if os.IsNotExist(serr) {
- return nil
- }
-
- return serr
- }
-
- if !dir.IsDir() {
- // Not a directory; return the error from Remove.
- return err
- }
-
- dirfs, ok := fs.(billy.Dir)
- if !ok {
- return billy.ErrNotSupported
- }
-
- // Directory.
- fis, err := dirfs.ReadDir(path)
- if err != nil {
- if os.IsNotExist(err) {
- // Race. It was deleted between the Lstat and Open.
- // Return nil per RemoveAll's docs.
- return nil
- }
-
- return err
- }
-
- // Remove contents & return first error.
- err = nil
- for _, fi := range fis {
- cpath := fs.Join(path, fi.Name())
- err1 := removeAll(fs, cpath)
- if err == nil {
- err = err1
- }
- }
-
- // Remove directory.
- err1 := fs.Remove(path)
- if err1 == nil || os.IsNotExist(err1) {
- return nil
- }
-
- if err == nil {
- err = err1
- }
-
- return err
-
-}
-
-// WriteFile writes data to a file named by filename in the given filesystem.
-// If the file does not exist, WriteFile creates it with permissions perm;
-// otherwise WriteFile truncates it before writing.
-func WriteFile(fs billy.Basic, filename string, data []byte, perm os.FileMode) error {
- f, err := fs.OpenFile(filename, os.O_WRONLY|os.O_CREATE|os.O_TRUNC, perm)
- if err != nil {
- return err
- }
-
- n, err := f.Write(data)
- if err == nil && n < len(data) {
- err = io.ErrShortWrite
- }
-
- if err1 := f.Close(); err == nil {
- err = err1
- }
-
- return err
-}
-
-// Random number state.
-// We generate random temporary file names so that there's a good
-// chance the file doesn't exist yet - keeps the number of tries in
-// TempFile to a minimum.
-var rand uint32
-var randmu sync.Mutex
-
-func reseed() uint32 {
- return uint32(time.Now().UnixNano() + int64(os.Getpid()))
-}
-
-func nextSuffix() string {
- randmu.Lock()
- r := rand
- if r == 0 {
- r = reseed()
- }
- r = r*1664525 + 1013904223 // constants from Numerical Recipes
- rand = r
- randmu.Unlock()
- return strconv.Itoa(int(1e9 + r%1e9))[1:]
-}
-
-// TempFile creates a new temporary file in the directory dir with a name
-// beginning with prefix, opens the file for reading and writing, and returns
-// the resulting *os.File. If dir is the empty string, TempFile uses the default
-// directory for temporary files (see os.TempDir). Multiple programs calling
-// TempFile simultaneously will not choose the same file. The caller can use
-// f.Name() to find the pathname of the file. It is the caller's responsibility
-// to remove the file when no longer needed.
-func TempFile(fs billy.Basic, dir, prefix string) (f billy.File, err error) {
- // This implementation is based on stdlib ioutil.TempFile.
- if dir == "" {
- dir = getTempDir(fs)
- }
-
- nconflict := 0
- for i := 0; i < 10000; i++ {
- name := filepath.Join(dir, prefix+nextSuffix())
- f, err = fs.OpenFile(name, os.O_RDWR|os.O_CREATE|os.O_EXCL, 0600)
- if os.IsExist(err) {
- if nconflict++; nconflict > 10 {
- randmu.Lock()
- rand = reseed()
- randmu.Unlock()
- }
- continue
- }
- break
- }
- return
-}
-
-// TempDir creates a new temporary directory in the directory dir
-// with a name beginning with prefix and returns the path of the
-// new directory. If dir is the empty string, TempDir uses the
-// default directory for temporary files (see os.TempDir).
-// Multiple programs calling TempDir simultaneously
-// will not choose the same directory. It is the caller's responsibility
-// to remove the directory when no longer needed.
-func TempDir(fs billy.Dir, dir, prefix string) (name string, err error) {
- // This implementation is based on stdlib ioutil.TempDir
-
- if dir == "" {
- dir = getTempDir(fs.(billy.Basic))
- }
-
- nconflict := 0
- for i := 0; i < 10000; i++ {
- try := filepath.Join(dir, prefix+nextSuffix())
- err = fs.MkdirAll(try, 0700)
- if os.IsExist(err) {
- if nconflict++; nconflict > 10 {
- randmu.Lock()
- rand = reseed()
- randmu.Unlock()
- }
- continue
- }
- if os.IsNotExist(err) {
- if _, err := os.Stat(dir); os.IsNotExist(err) {
- return "", err
- }
- }
- if err == nil {
- name = try
- }
- break
- }
- return
-}
-
-func getTempDir(fs billy.Basic) string {
- ch, ok := fs.(billy.Chroot)
- if !ok || ch.Root() == "" || ch.Root() == "/" || ch.Root() == string(filepath.Separator) {
- return os.TempDir()
- }
-
- return ".tmp"
-}
-
-type underlying interface {
- Underlying() billy.Basic
-}
-
-func getUnderlyingAndPath(fs billy.Basic, path string) (billy.Basic, string) {
- u, ok := fs.(underlying)
- if !ok {
- return fs, path
- }
- if ch, ok := fs.(billy.Chroot); ok {
- path = fs.Join(ch.Root(), path)
- }
-
- return u.Underlying(), path
-}
-
-// ReadFile reads the named file and returns the contents from the given filesystem.
-// A successful call returns err == nil, not err == EOF.
-// Because ReadFile reads the whole file, it does not treat an EOF from Read
-// as an error to be reported.
-func ReadFile(fs billy.Basic, name string) ([]byte, error) {
- f, err := fs.Open(name)
- if err != nil {
- return nil, err
- }
-
- defer f.Close()
-
- var size int
- if info, err := fs.Stat(name); err == nil {
- size64 := info.Size()
- if int64(int(size64)) == size64 {
- size = int(size64)
- }
- }
-
- size++ // one byte for final read at EOF
- // If a file claims a small size, read at least 512 bytes.
- // In particular, files in Linux's /proc claim size 0 but
- // then do not work right if read in small pieces,
- // so an initial read of 1 byte would not work correctly.
-
- if size < 512 {
- size = 512
- }
-
- data := make([]byte, 0, size)
- for {
- if len(data) >= cap(data) {
- d := append(data[:cap(data)], 0)
- data = d[:len(data)]
- }
-
- n, err := f.Read(data[len(data):cap(data)])
- data = data[:len(data)+n]
-
- if err != nil {
- if err == io.EOF {
- err = nil
- }
-
- return data, err
- }
- }
-}
diff --git a/vendor/github.com/go-git/go-git/v5/.gitignore b/vendor/github.com/go-git/go-git/v5/.gitignore
deleted file mode 100644
index 038dd9f..0000000
--- a/vendor/github.com/go-git/go-git/v5/.gitignore
+++ /dev/null
@@ -1,4 +0,0 @@
-coverage.out
-*~
-coverage.txt
-profile.out
diff --git a/vendor/github.com/go-git/go-git/v5/CODE_OF_CONDUCT.md b/vendor/github.com/go-git/go-git/v5/CODE_OF_CONDUCT.md
deleted file mode 100644
index a689fa3..0000000
--- a/vendor/github.com/go-git/go-git/v5/CODE_OF_CONDUCT.md
+++ /dev/null
@@ -1,74 +0,0 @@
-# Contributor Covenant Code of Conduct
-
-## Our Pledge
-
-In the interest of fostering an open and welcoming environment, we as
-contributors and maintainers pledge to making participation in our project and
-our community a harassment-free experience for everyone, regardless of age, body
-size, disability, ethnicity, gender identity and expression, level of experience,
-education, socio-economic status, nationality, personal appearance, race,
-religion, or sexual identity and orientation.
-
-## Our Standards
-
-Examples of behavior that contributes to creating a positive environment
-include:
-
-* Using welcoming and inclusive language
-* Being respectful of differing viewpoints and experiences
-* Gracefully accepting constructive criticism
-* Focusing on what is best for the community
-* Showing empathy towards other community members
-
-Examples of unacceptable behavior by participants include:
-
-* The use of sexualized language or imagery and unwelcome sexual attention or
- advances
-* Trolling, insulting/derogatory comments, and personal or political attacks
-* Public or private harassment
-* Publishing others' private information, such as a physical or electronic
- address, without explicit permission
-* Other conduct which could reasonably be considered inappropriate in a
- professional setting
-
-## Our Responsibilities
-
-Project maintainers are responsible for clarifying the standards of acceptable
-behavior and are expected to take appropriate and fair corrective action in
-response to any instances of unacceptable behavior.
-
-Project maintainers have the right and responsibility to remove, edit, or
-reject comments, commits, code, wiki edits, issues, and other contributions
-that are not aligned to this Code of Conduct, or to ban temporarily or
-permanently any contributor for other behaviors that they deem inappropriate,
-threatening, offensive, or harmful.
-
-## Scope
-
-This Code of Conduct applies both within project spaces and in public spaces
-when an individual is representing the project or its community. Examples of
-representing a project or community include using an official project e-mail
-address, posting via an official social media account, or acting as an appointed
-representative at an online or offline event. Representation of a project may be
-further defined and clarified by project maintainers.
-
-## Enforcement
-
-Instances of abusive, harassing, or otherwise unacceptable behavior may be
-reported by contacting the project team at conduct@sourced.tech. All
-complaints will be reviewed and investigated and will result in a response that
-is deemed necessary and appropriate to the circumstances. The project team is
-obligated to maintain confidentiality with regard to the reporter of an incident.
-Further details of specific enforcement policies may be posted separately.
-
-Project maintainers who do not follow or enforce the Code of Conduct in good
-faith may face temporary or permanent repercussions as determined by other
-members of the project's leadership.
-
-## Attribution
-
-This Code of Conduct is adapted from the [Contributor Covenant][homepage], version 1.4,
-available at https://www.contributor-covenant.org/version/1/4/code-of-conduct.html
-
-[homepage]: https://www.contributor-covenant.org
-
diff --git a/vendor/github.com/go-git/go-git/v5/COMPATIBILITY.md b/vendor/github.com/go-git/go-git/v5/COMPATIBILITY.md
deleted file mode 100644
index 2a72b50..0000000
--- a/vendor/github.com/go-git/go-git/v5/COMPATIBILITY.md
+++ /dev/null
@@ -1,111 +0,0 @@
-Supported Capabilities
-======================
-
-Here is a non-comprehensive table of git commands and features whose equivalent
-is supported by go-git.
-
-| Feature | Status | Notes |
-|---------------------------------------|--------|-------|
-| **config** |
-| config | ✔ | Reading and modifying per-repository configuration (`.git/config`) is supported. Global configuration (`$HOME/.gitconfig`) is not. |
-| **getting and creating repositories** |
-| init | ✔ | Plain init and `--bare` are supported. Flags `--template`, `--separate-git-dir` and `--shared` are not. |
-| clone | ✔ | Plain clone and equivalents to `--progress`, `--single-branch`, `--depth`, `--origin`, `--recurse-submodules` are supported. Others are not. |
-| **basic snapshotting** |
-| add | ✔ | Plain add is supported. Any other flags aren't supported |
-| status | ✔ |
-| commit | ✔ |
-| reset | ✔ |
-| rm | ✔ |
-| mv | ✔ |
-| **branching and merging** |
-| branch | ✔ |
-| checkout | ✔ | Basic usages of checkout are supported. |
-| merge | ✖ |
-| mergetool | ✖ |
-| stash | ✖ |
-| tag | ✔ |
-| **sharing and updating projects** |
-| fetch | ✔ |
-| pull | ✔ | Only supports merges where the merge can be resolved as a fast-forward. |
-| push | ✔ |
-| remote | ✔ |
-| submodule | ✔ |
-| **inspection and comparison** |
-| show | ✔ |
-| log | ✔ |
-| shortlog | (see log) |
-| describe | |
-| **patching** |
-| apply | ✖ |
-| cherry-pick | ✖ |
-| diff | ✔ | Patch object with UnifiedDiff output representation |
-| rebase | ✖ |
-| revert | ✖ |
-| **debugging** |
-| bisect | ✖ |
-| blame | ✔ |
-| grep | ✔ |
-| **email** ||
-| am | ✖ |
-| apply | ✖ |
-| format-patch | ✖ |
-| send-email | ✖ |
-| request-pull | ✖ |
-| **external systems** |
-| svn | ✖ |
-| fast-import | ✖ |
-| **administration** |
-| clean | ✔ |
-| gc | ✖ |
-| fsck | ✖ |
-| reflog | ✖ |
-| filter-branch | ✖ |
-| instaweb | ✖ |
-| archive | ✖ |
-| bundle | ✖ |
-| prune | ✖ |
-| repack | ✖ |
-| **server admin** |
-| daemon | |
-| update-server-info | |
-| **advanced** |
-| notes | ✖ |
-| replace | ✖ |
-| worktree | ✖ |
-| annotate | (see blame) |
-| **gpg** |
-| git-verify-commit | ✔ |
-| git-verify-tag | ✔ |
-| **plumbing commands** |
-| cat-file | ✔ |
-| check-ignore | |
-| commit-tree | |
-| count-objects | |
-| diff-index | |
-| for-each-ref | ✔ |
-| hash-object | ✔ |
-| ls-files | ✔ |
-| merge-base | ✔ | Calculates the merge-base only between two commits, and supports `--independent` and `--is-ancestor` modifiers; Does not support `--fork-point` nor `--octopus` modifiers. |
-| read-tree | |
-| rev-list | ✔ |
-| rev-parse | |
-| show-ref | ✔ |
-| symbolic-ref | ✔ |
-| update-index | |
-| update-ref | |
-| verify-pack | |
-| write-tree | |
-| **protocols** |
-| http(s):// (dumb) | ✖ |
-| http(s):// (smart) | ✔ |
-| git:// | ✔ |
-| ssh:// | ✔ |
-| file:// | partial | Warning: this is not pure Golang. This shells out to the `git` binary. |
-| custom | ✔ |
-| **other features** |
-| gitignore | ✔ |
-| gitattributes | ✖ |
-| index version | |
-| packfile version | |
-| push-certs | ✖ |
diff --git a/vendor/github.com/go-git/go-git/v5/CONTRIBUTING.md b/vendor/github.com/go-git/go-git/v5/CONTRIBUTING.md
deleted file mode 100644
index fce2532..0000000
--- a/vendor/github.com/go-git/go-git/v5/CONTRIBUTING.md
+++ /dev/null
@@ -1,46 +0,0 @@
-# Contributing Guidelines
-
-source{d} go-git project is [Apache 2.0 licensed](LICENSE) and accepts
-contributions via GitHub pull requests. This document outlines some of the
-conventions on development workflow, commit message formatting, contact points,
-and other resources to make it easier to get your contribution accepted.
-
-## Support Channels
-
-The official support channels, for both users and contributors, are:
-
-- [StackOverflow go-git tag](https://stackoverflow.com/questions/tagged/go-git) for user questions.
-- GitHub [Issues](https://github.com/src-d/go-git/issues)* for bug reports and feature requests.
-
-*Before opening a new issue or submitting a new pull request, it's helpful to
-search the project - it's likely that another user has already reported the
-issue you're facing, or it's a known issue that we're already aware of.
-
-
-## How to Contribute
-
-Pull Requests (PRs) are the main and exclusive way to contribute to the official go-git project.
-In order for a PR to be accepted it needs to pass a list of requirements:
-
-- You should be able to run the same query using `git`. We don't accept features that are not implemented in the official git implementation.
-- The expected behavior must match the [official git implementation](https://github.com/git/git).
-- The actual behavior must be correctly explained with natural language and providing a minimum working example in Go that reproduces it.
-- All PRs must be written in idiomatic Go, formatted according to [gofmt](https://golang.org/cmd/gofmt/), and without any warnings from [go lint](https://github.com/golang/lint) nor [go vet](https://golang.org/cmd/vet/).
-- They should in general include tests, and those shall pass.
-- If the PR is a bug fix, it has to include a suite of unit tests for the new functionality.
-- If the PR is a new feature, it has to come with a suite of unit tests, that tests the new functionality.
-- In any case, all the PRs have to pass the personal evaluation of at least one of the maintainers of go-git.
-
-### Format of the commit message
-
-Every commit message should describe what was changed, under which context and, if applicable, the GitHub issue it relates to:
-
-```
-plumbing: packp, Skip argument validations for unknown capabilities. Fixes #623
-```
-
-The format can be described more formally as follows:
-
-```
-: , . [Fixes #]
-```
diff --git a/vendor/github.com/go-git/go-git/v5/LICENSE b/vendor/github.com/go-git/go-git/v5/LICENSE
deleted file mode 100644
index 8aa3d85..0000000
--- a/vendor/github.com/go-git/go-git/v5/LICENSE
+++ /dev/null
@@ -1,201 +0,0 @@
- Apache License
- Version 2.0, January 2004
- http://www.apache.org/licenses/
-
- TERMS AND CONDITIONS FOR USE, REPRODUCTION, AND DISTRIBUTION
-
- 1. Definitions.
-
- "License" shall mean the terms and conditions for use, reproduction,
- and distribution as defined by Sections 1 through 9 of this document.
-
- "Licensor" shall mean the copyright owner or entity authorized by
- the copyright owner that is granting the License.
-
- "Legal Entity" shall mean the union of the acting entity and all
- other entities that control, are controlled by, or are under common
- control with that entity. For the purposes of this definition,
- "control" means (i) the power, direct or indirect, to cause the
- direction or management of such entity, whether by contract or
- otherwise, or (ii) ownership of fifty percent (50%) or more of the
- outstanding shares, or (iii) beneficial ownership of such entity.
-
- "You" (or "Your") shall mean an individual or Legal Entity
- exercising permissions granted by this License.
-
- "Source" form shall mean the preferred form for making modifications,
- including but not limited to software source code, documentation
- source, and configuration files.
-
- "Object" form shall mean any form resulting from mechanical
- transformation or translation of a Source form, including but
- not limited to compiled object code, generated documentation,
- and conversions to other media types.
-
- "Work" shall mean the work of authorship, whether in Source or
- Object form, made available under the License, as indicated by a
- copyright notice that is included in or attached to the work
- (an example is provided in the Appendix below).
-
- "Derivative Works" shall mean any work, whether in Source or Object
- form, that is based on (or derived from) the Work and for which the
- editorial revisions, annotations, elaborations, or other modifications
- represent, as a whole, an original work of authorship. For the purposes
- of this License, Derivative Works shall not include works that remain
- separable from, or merely link (or bind by name) to the interfaces of,
- the Work and Derivative Works thereof.
-
- "Contribution" shall mean any work of authorship, including
- the original version of the Work and any modifications or additions
- to that Work or Derivative Works thereof, that is intentionally
- submitted to Licensor for inclusion in the Work by the copyright owner
- or by an individual or Legal Entity authorized to submit on behalf of
- the copyright owner. For the purposes of this definition, "submitted"
- means any form of electronic, verbal, or written communication sent
- to the Licensor or its representatives, including but not limited to
- communication on electronic mailing lists, source code control systems,
- and issue tracking systems that are managed by, or on behalf of, the
- Licensor for the purpose of discussing and improving the Work, but
- excluding communication that is conspicuously marked or otherwise
- designated in writing by the copyright owner as "Not a Contribution."
-
- "Contributor" shall mean Licensor and any individual or Legal Entity
- on behalf of whom a Contribution has been received by Licensor and
- subsequently incorporated within the Work.
-
- 2. Grant of Copyright License. Subject to the terms and conditions of
- this License, each Contributor hereby grants to You a perpetual,
- worldwide, non-exclusive, no-charge, royalty-free, irrevocable
- copyright license to reproduce, prepare Derivative Works of,
- publicly display, publicly perform, sublicense, and distribute the
- Work and such Derivative Works in Source or Object form.
-
- 3. Grant of Patent License. Subject to the terms and conditions of
- this License, each Contributor hereby grants to You a perpetual,
- worldwide, non-exclusive, no-charge, royalty-free, irrevocable
- (except as stated in this section) patent license to make, have made,
- use, offer to sell, sell, import, and otherwise transfer the Work,
- where such license applies only to those patent claims licensable
- by such Contributor that are necessarily infringed by their
- Contribution(s) alone or by combination of their Contribution(s)
- with the Work to which such Contribution(s) was submitted. If You
- institute patent litigation against any entity (including a
- cross-claim or counterclaim in a lawsuit) alleging that the Work
- or a Contribution incorporated within the Work constitutes direct
- or contributory patent infringement, then any patent licenses
- granted to You under this License for that Work shall terminate
- as of the date such litigation is filed.
-
- 4. Redistribution. You may reproduce and distribute copies of the
- Work or Derivative Works thereof in any medium, with or without
- modifications, and in Source or Object form, provided that You
- meet the following conditions:
-
- (a) You must give any other recipients of the Work or
- Derivative Works a copy of this License; and
-
- (b) You must cause any modified files to carry prominent notices
- stating that You changed the files; and
-
- (c) You must retain, in the Source form of any Derivative Works
- that You distribute, all copyright, patent, trademark, and
- attribution notices from the Source form of the Work,
- excluding those notices that do not pertain to any part of
- the Derivative Works; and
-
- (d) If the Work includes a "NOTICE" text file as part of its
- distribution, then any Derivative Works that You distribute must
- include a readable copy of the attribution notices contained
- within such NOTICE file, excluding those notices that do not
- pertain to any part of the Derivative Works, in at least one
- of the following places: within a NOTICE text file distributed
- as part of the Derivative Works; within the Source form or
- documentation, if provided along with the Derivative Works; or,
- within a display generated by the Derivative Works, if and
- wherever such third-party notices normally appear. The contents
- of the NOTICE file are for informational purposes only and
- do not modify the License. You may add Your own attribution
- notices within Derivative Works that You distribute, alongside
- or as an addendum to the NOTICE text from the Work, provided
- that such additional attribution notices cannot be construed
- as modifying the License.
-
- You may add Your own copyright statement to Your modifications and
- may provide additional or different license terms and conditions
- for use, reproduction, or distribution of Your modifications, or
- for any such Derivative Works as a whole, provided Your use,
- reproduction, and distribution of the Work otherwise complies with
- the conditions stated in this License.
-
- 5. Submission of Contributions. Unless You explicitly state otherwise,
- any Contribution intentionally submitted for inclusion in the Work
- by You to the Licensor shall be under the terms and conditions of
- this License, without any additional terms or conditions.
- Notwithstanding the above, nothing herein shall supersede or modify
- the terms of any separate license agreement you may have executed
- with Licensor regarding such Contributions.
-
- 6. Trademarks. This License does not grant permission to use the trade
- names, trademarks, service marks, or product names of the Licensor,
- except as required for reasonable and customary use in describing the
- origin of the Work and reproducing the content of the NOTICE file.
-
- 7. Disclaimer of Warranty. Unless required by applicable law or
- agreed to in writing, Licensor provides the Work (and each
- Contributor provides its Contributions) on an "AS IS" BASIS,
- WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
- implied, including, without limitation, any warranties or conditions
- of TITLE, NON-INFRINGEMENT, MERCHANTABILITY, or FITNESS FOR A
- PARTICULAR PURPOSE. You are solely responsible for determining the
- appropriateness of using or redistributing the Work and assume any
- risks associated with Your exercise of permissions under this License.
-
- 8. Limitation of Liability. In no event and under no legal theory,
- whether in tort (including negligence), contract, or otherwise,
- unless required by applicable law (such as deliberate and grossly
- negligent acts) or agreed to in writing, shall any Contributor be
- liable to You for damages, including any direct, indirect, special,
- incidental, or consequential damages of any character arising as a
- result of this License or out of the use or inability to use the
- Work (including but not limited to damages for loss of goodwill,
- work stoppage, computer failure or malfunction, or any and all
- other commercial damages or losses), even if such Contributor
- has been advised of the possibility of such damages.
-
- 9. Accepting Warranty or Additional Liability. While redistributing
- the Work or Derivative Works thereof, You may choose to offer,
- and charge a fee for, acceptance of support, warranty, indemnity,
- or other liability obligations and/or rights consistent with this
- License. However, in accepting such obligations, You may act only
- on Your own behalf and on Your sole responsibility, not on behalf
- of any other Contributor, and only if You agree to indemnify,
- defend, and hold each Contributor harmless for any liability
- incurred by, or claims asserted against, such Contributor by reason
- of your accepting any such warranty or additional liability.
-
- END OF TERMS AND CONDITIONS
-
- APPENDIX: How to apply the Apache License to your work.
-
- To apply the Apache License to your work, attach the following
- boilerplate notice, with the fields enclosed by brackets "{}"
- replaced with your own identifying information. (Don't include
- the brackets!) The text should be enclosed in the appropriate
- comment syntax for the file format. We also recommend that a
- file or class name and description of purpose be included on the
- same "printed page" as the copyright notice for easier
- identification within third-party archives.
-
- Copyright 2018 Sourced Technologies, S.L.
-
- Licensed under the Apache License, Version 2.0 (the "License");
- you may not use this file except in compliance with the License.
- You may obtain a copy of the License at
-
- http://www.apache.org/licenses/LICENSE-2.0
-
- Unless required by applicable law or agreed to in writing, software
- distributed under the License is distributed on an "AS IS" BASIS,
- WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
- See the License for the specific language governing permissions and
- limitations under the License.
diff --git a/vendor/github.com/go-git/go-git/v5/Makefile b/vendor/github.com/go-git/go-git/v5/Makefile
deleted file mode 100644
index d10922f..0000000
--- a/vendor/github.com/go-git/go-git/v5/Makefile
+++ /dev/null
@@ -1,38 +0,0 @@
-# General
-WORKDIR = $(PWD)
-
-# Go parameters
-GOCMD = go
-GOTEST = $(GOCMD) test
-
-# Git config
-GIT_VERSION ?=
-GIT_DIST_PATH ?= $(PWD)/.git-dist
-GIT_REPOSITORY = http://github.com/git/git.git
-
-# Coverage
-COVERAGE_REPORT = coverage.out
-COVERAGE_MODE = count
-
-build-git:
- @if [ -f $(GIT_DIST_PATH)/git ]; then \
- echo "nothing to do, using cache $(GIT_DIST_PATH)"; \
- else \
- git clone $(GIT_REPOSITORY) -b $(GIT_VERSION) --depth 1 --single-branch $(GIT_DIST_PATH); \
- cd $(GIT_DIST_PATH); \
- make configure; \
- ./configure; \
- make all; \
- fi
-
-test:
- @echo "running against `git version`"; \
- $(GOTEST) ./...
-
-test-coverage:
- @echo "running against `git version`"; \
- echo "" > $(COVERAGE_REPORT); \
- $(GOTEST) -coverprofile=$(COVERAGE_REPORT) -coverpkg=./... -covermode=$(COVERAGE_MODE) ./...
-
-clean:
- rm -rf $(GIT_DIST_PATH)
\ No newline at end of file
diff --git a/vendor/github.com/go-git/go-git/v5/README.md b/vendor/github.com/go-git/go-git/v5/README.md
deleted file mode 100644
index ff0c9b7..0000000
--- a/vendor/github.com/go-git/go-git/v5/README.md
+++ /dev/null
@@ -1,131 +0,0 @@
-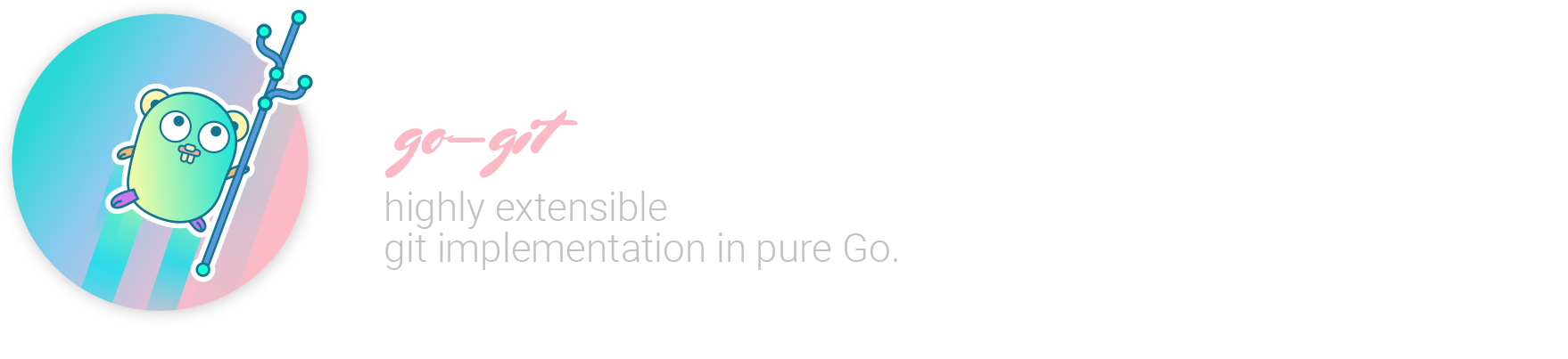
-[](https://pkg.go.dev/github.com/go-git/go-git/v5) [](https://github.com/go-git/go-git/actions) [](https://goreportcard.com/report/github.com/go-git/go-git)
-
-*go-git* is a highly extensible git implementation library written in **pure Go**.
-
-It can be used to manipulate git repositories at low level *(plumbing)* or high level *(porcelain)*, through an idiomatic Go API. It also supports several types of storage, such as in-memory filesystems, or custom implementations, thanks to the [`Storer`](https://pkg.go.dev/github.com/go-git/go-git/v5/plumbing/storer) interface.
-
-It's being actively developed since 2015 and is being used extensively by [Keybase](https://keybase.io/blog/encrypted-git-for-everyone), [Gitea](https://gitea.io/en-us/) or [Pulumi](https://github.com/search?q=org%3Apulumi+go-git&type=Code), and by many other libraries and tools.
-
-Project Status
---------------
-
-After the legal issues with the [`src-d`](https://github.com/src-d) organization, the lack of update for four months and the requirement to make a hard fork, the project is **now back to normality**.
-
-The project is currently actively maintained by individual contributors, including several of the original authors, but also backed by a new company, [gitsight](https://github.com/gitsight), where `go-git` is a critical component used at scale.
-
-
-Comparison with git
--------------------
-
-*go-git* aims to be fully compatible with [git](https://github.com/git/git), all the *porcelain* operations are implemented to work exactly as *git* does.
-
-*git* is a humongous project with years of development by thousands of contributors, making it challenging for *go-git* to implement all the features. You can find a comparison of *go-git* vs *git* in the [compatibility documentation](COMPATIBILITY.md).
-
-
-Installation
-------------
-
-The recommended way to install *go-git* is:
-
-```go
-import "github.com/go-git/go-git/v5" // with go modules enabled (GO111MODULE=on or outside GOPATH)
-import "github.com/go-git/go-git" // with go modules disabled
-```
-
-
-Examples
---------
-
-> Please note that the `CheckIfError` and `Info` functions used in the examples are from the [examples package](https://github.com/go-git/go-git/blob/master/_examples/common.go#L19) just to be used in the examples.
-
-
-### Basic example
-
-A basic example that mimics the standard `git clone` command
-
-```go
-// Clone the given repository to the given directory
-Info("git clone https://github.com/go-git/go-git")
-
-_, err := git.PlainClone("/tmp/foo", false, &git.CloneOptions{
- URL: "https://github.com/go-git/go-git",
- Progress: os.Stdout,
-})
-
-CheckIfError(err)
-```
-
-Outputs:
-```
-Counting objects: 4924, done.
-Compressing objects: 100% (1333/1333), done.
-Total 4924 (delta 530), reused 6 (delta 6), pack-reused 3533
-```
-
-### In-memory example
-
-Cloning a repository into memory and printing the history of HEAD, just like `git log` does
-
-
-```go
-// Clones the given repository in memory, creating the remote, the local
-// branches and fetching the objects, exactly as:
-Info("git clone https://github.com/go-git/go-billy")
-
-r, err := git.Clone(memory.NewStorage(), nil, &git.CloneOptions{
- URL: "https://github.com/go-git/go-billy",
-})
-
-CheckIfError(err)
-
-// Gets the HEAD history from HEAD, just like this command:
-Info("git log")
-
-// ... retrieves the branch pointed by HEAD
-ref, err := r.Head()
-CheckIfError(err)
-
-
-// ... retrieves the commit history
-cIter, err := r.Log(&git.LogOptions{From: ref.Hash()})
-CheckIfError(err)
-
-// ... just iterates over the commits, printing it
-err = cIter.ForEach(func(c *object.Commit) error {
- fmt.Println(c)
- return nil
-})
-CheckIfError(err)
-```
-
-Outputs:
-```
-commit ded8054fd0c3994453e9c8aacaf48d118d42991e
-Author: Santiago M. Mola
-Date: Sat Nov 12 21:18:41 2016 +0100
-
- index: ReadFrom/WriteTo returns IndexReadError/IndexWriteError. (#9)
-
-commit df707095626f384ce2dc1a83b30f9a21d69b9dfc
-Author: Santiago M. Mola
-Date: Fri Nov 11 13:23:22 2016 +0100
-
- readwriter: fix bug when writing index. (#10)
-
- When using ReadWriter on an existing siva file, absolute offset for
- index entries was not being calculated correctly.
-...
-```
-
-You can find this [example](_examples/log/main.go) and many others in the [examples](_examples) folder.
-
-Contribute
-----------
-
-[Contributions](https://github.com/go-git/go-git/issues?q=is%3Aissue+is%3Aopen+label%3A%22help+wanted%22) are more than welcome, if you are interested please take a look to
-our [Contributing Guidelines](CONTRIBUTING.md).
-
-License
--------
-Apache License Version 2.0, see [LICENSE](LICENSE)
diff --git a/vendor/github.com/go-git/go-git/v5/blame.go b/vendor/github.com/go-git/go-git/v5/blame.go
deleted file mode 100644
index 43634b3..0000000
--- a/vendor/github.com/go-git/go-git/v5/blame.go
+++ /dev/null
@@ -1,302 +0,0 @@
-package git
-
-import (
- "bytes"
- "errors"
- "fmt"
- "strconv"
- "strings"
- "time"
- "unicode/utf8"
-
- "github.com/go-git/go-git/v5/plumbing"
- "github.com/go-git/go-git/v5/plumbing/object"
- "github.com/go-git/go-git/v5/utils/diff"
-)
-
-// BlameResult represents the result of a Blame operation.
-type BlameResult struct {
- // Path is the path of the File that we're blaming.
- Path string
- // Rev (Revision) is the hash of the specified Commit used to generate this result.
- Rev plumbing.Hash
- // Lines contains every line with its authorship.
- Lines []*Line
-}
-
-// Blame returns a BlameResult with the information about the last author of
-// each line from file `path` at commit `c`.
-func Blame(c *object.Commit, path string) (*BlameResult, error) {
- // The file to blame is identified by the input arguments:
- // commit and path. commit is a Commit object obtained from a Repository. Path
- // represents a path to a specific file contained into the repository.
- //
- // Blaming a file is a two step process:
- //
- // 1. Create a linear history of the commits affecting a file. We use
- // revlist.New for that.
- //
- // 2. Then build a graph with a node for every line in every file in
- // the history of the file.
- //
- // Each node is assigned a commit: Start by the nodes in the first
- // commit. Assign that commit as the creator of all its lines.
- //
- // Then jump to the nodes in the next commit, and calculate the diff
- // between the two files. Newly created lines get
- // assigned the new commit as its origin. Modified lines also get
- // this new commit. Untouched lines retain the old commit.
- //
- // All this work is done in the assignOrigin function which holds all
- // the internal relevant data in a "blame" struct, that is not
- // exported.
- //
- // TODO: ways to improve the efficiency of this function:
- // 1. Improve revlist
- // 2. Improve how to traverse the history (example a backward traversal will
- // be much more efficient)
- //
- // TODO: ways to improve the function in general:
- // 1. Add memoization between revlist and assign.
- // 2. It is using much more memory than needed, see the TODOs below.
-
- b := new(blame)
- b.fRev = c
- b.path = path
-
- // get all the file revisions
- if err := b.fillRevs(); err != nil {
- return nil, err
- }
-
- // calculate the line tracking graph and fill in
- // file contents in data.
- if err := b.fillGraphAndData(); err != nil {
- return nil, err
- }
-
- file, err := b.fRev.File(b.path)
- if err != nil {
- return nil, err
- }
- finalLines, err := file.Lines()
- if err != nil {
- return nil, err
- }
-
- // Each node (line) holds the commit where it was introduced or
- // last modified. To achieve that we use the FORWARD algorithm
- // described in Zimmermann, et al. "Mining Version Archives for
- // Co-changed Lines", in proceedings of the Mining Software
- // Repositories workshop, Shanghai, May 22-23, 2006.
- lines, err := newLines(finalLines, b.sliceGraph(len(b.graph)-1))
- if err != nil {
- return nil, err
- }
-
- return &BlameResult{
- Path: path,
- Rev: c.Hash,
- Lines: lines,
- }, nil
-}
-
-// Line values represent the contents and author of a line in BlamedResult values.
-type Line struct {
- // Author is the email address of the last author that modified the line.
- Author string
- // Text is the original text of the line.
- Text string
- // Date is when the original text of the line was introduced
- Date time.Time
- // Hash is the commit hash that introduced the original line
- Hash plumbing.Hash
-}
-
-func newLine(author, text string, date time.Time, hash plumbing.Hash) *Line {
- return &Line{
- Author: author,
- Text: text,
- Hash: hash,
- Date: date,
- }
-}
-
-func newLines(contents []string, commits []*object.Commit) ([]*Line, error) {
- lcontents := len(contents)
- lcommits := len(commits)
-
- if lcontents != lcommits {
- if lcontents == lcommits-1 && contents[lcontents-1] != "\n" {
- contents = append(contents, "\n")
- } else {
- return nil, errors.New("contents and commits have different length")
- }
- }
-
- result := make([]*Line, 0, lcontents)
- for i := range contents {
- result = append(result, newLine(
- commits[i].Author.Email, contents[i],
- commits[i].Author.When, commits[i].Hash,
- ))
- }
-
- return result, nil
-}
-
-// this struct is internally used by the blame function to hold its
-// inputs, outputs and state.
-type blame struct {
- // the path of the file to blame
- path string
- // the commit of the final revision of the file to blame
- fRev *object.Commit
- // the chain of revisions affecting the the file to blame
- revs []*object.Commit
- // the contents of the file across all its revisions
- data []string
- // the graph of the lines in the file across all the revisions
- graph [][]*object.Commit
-}
-
-// calculate the history of a file "path", starting from commit "from", sorted by commit date.
-func (b *blame) fillRevs() error {
- var err error
-
- b.revs, err = references(b.fRev, b.path)
- return err
-}
-
-// build graph of a file from its revision history
-func (b *blame) fillGraphAndData() error {
- //TODO: not all commits are needed, only the current rev and the prev
- b.graph = make([][]*object.Commit, len(b.revs))
- b.data = make([]string, len(b.revs)) // file contents in all the revisions
- // for every revision of the file, starting with the first
- // one...
- for i, rev := range b.revs {
- // get the contents of the file
- file, err := rev.File(b.path)
- if err != nil {
- return nil
- }
- b.data[i], err = file.Contents()
- if err != nil {
- return err
- }
- nLines := countLines(b.data[i])
- // create a node for each line
- b.graph[i] = make([]*object.Commit, nLines)
- // assign a commit to each node
- // if this is the first revision, then the node is assigned to
- // this first commit.
- if i == 0 {
- for j := 0; j < nLines; j++ {
- b.graph[i][j] = b.revs[i]
- }
- } else {
- // if this is not the first commit, then assign to the old
- // commit or to the new one, depending on what the diff
- // says.
- b.assignOrigin(i, i-1)
- }
- }
- return nil
-}
-
-// sliceGraph returns a slice of commits (one per line) for a particular
-// revision of a file (0=first revision).
-func (b *blame) sliceGraph(i int) []*object.Commit {
- fVs := b.graph[i]
- result := make([]*object.Commit, 0, len(fVs))
- for _, v := range fVs {
- c := *v
- result = append(result, &c)
- }
- return result
-}
-
-// Assigns origin to vertexes in current (c) rev from data in its previous (p)
-// revision
-func (b *blame) assignOrigin(c, p int) {
- // assign origin based on diff info
- hunks := diff.Do(b.data[p], b.data[c])
- sl := -1 // source line
- dl := -1 // destination line
- for h := range hunks {
- hLines := countLines(hunks[h].Text)
- for hl := 0; hl < hLines; hl++ {
- switch {
- case hunks[h].Type == 0:
- sl++
- dl++
- b.graph[c][dl] = b.graph[p][sl]
- case hunks[h].Type == 1:
- dl++
- b.graph[c][dl] = b.revs[c]
- case hunks[h].Type == -1:
- sl++
- default:
- panic("unreachable")
- }
- }
- }
-}
-
-// GoString prints the results of a Blame using git-blame's style.
-func (b *blame) GoString() string {
- var buf bytes.Buffer
-
- file, err := b.fRev.File(b.path)
- if err != nil {
- panic("PrettyPrint: internal error in repo.Data")
- }
- contents, err := file.Contents()
- if err != nil {
- panic("PrettyPrint: internal error in repo.Data")
- }
-
- lines := strings.Split(contents, "\n")
- // max line number length
- mlnl := len(strconv.Itoa(len(lines)))
- // max author length
- mal := b.maxAuthorLength()
- format := fmt.Sprintf("%%s (%%-%ds %%%dd) %%s\n",
- mal, mlnl)
-
- fVs := b.graph[len(b.graph)-1]
- for ln, v := range fVs {
- fmt.Fprintf(&buf, format, v.Hash.String()[:8],
- prettyPrintAuthor(fVs[ln]), ln+1, lines[ln])
- }
- return buf.String()
-}
-
-// utility function to pretty print the author.
-func prettyPrintAuthor(c *object.Commit) string {
- return fmt.Sprintf("%s %s", c.Author.Name, c.Author.When.Format("2006-01-02"))
-}
-
-// utility function to calculate the number of runes needed
-// to print the longest author name in the blame of a file.
-func (b *blame) maxAuthorLength() int {
- memo := make(map[plumbing.Hash]struct{}, len(b.graph)-1)
- fVs := b.graph[len(b.graph)-1]
- m := 0
- for ln := range fVs {
- if _, ok := memo[fVs[ln].Hash]; ok {
- continue
- }
- memo[fVs[ln].Hash] = struct{}{}
- m = max(m, utf8.RuneCountInString(prettyPrintAuthor(fVs[ln])))
- }
- return m
-}
-
-func max(a, b int) int {
- if a > b {
- return a
- }
- return b
-}
diff --git a/vendor/github.com/go-git/go-git/v5/common.go b/vendor/github.com/go-git/go-git/v5/common.go
deleted file mode 100644
index 6174339..0000000
--- a/vendor/github.com/go-git/go-git/v5/common.go
+++ /dev/null
@@ -1,20 +0,0 @@
-package git
-
-import "strings"
-
-// countLines returns the number of lines in a string à la git, this is
-// The newline character is assumed to be '\n'. The empty string
-// contains 0 lines. If the last line of the string doesn't end with a
-// newline, it will still be considered a line.
-func countLines(s string) int {
- if s == "" {
- return 0
- }
-
- nEOL := strings.Count(s, "\n")
- if strings.HasSuffix(s, "\n") {
- return nEOL
- }
-
- return nEOL + 1
-}
diff --git a/vendor/github.com/go-git/go-git/v5/config/branch.go b/vendor/github.com/go-git/go-git/v5/config/branch.go
deleted file mode 100644
index fe86cf5..0000000
--- a/vendor/github.com/go-git/go-git/v5/config/branch.go
+++ /dev/null
@@ -1,90 +0,0 @@
-package config
-
-import (
- "errors"
-
- "github.com/go-git/go-git/v5/plumbing"
- format "github.com/go-git/go-git/v5/plumbing/format/config"
-)
-
-var (
- errBranchEmptyName = errors.New("branch config: empty name")
- errBranchInvalidMerge = errors.New("branch config: invalid merge")
- errBranchInvalidRebase = errors.New("branch config: rebase must be one of 'true' or 'interactive'")
-)
-
-// Branch contains information on the
-// local branches and which remote to track
-type Branch struct {
- // Name of branch
- Name string
- // Remote name of remote to track
- Remote string
- // Merge is the local refspec for the branch
- Merge plumbing.ReferenceName
- // Rebase instead of merge when pulling. Valid values are
- // "true" and "interactive". "false" is undocumented and
- // typically represented by the non-existence of this field
- Rebase string
-
- raw *format.Subsection
-}
-
-// Validate validates fields of branch
-func (b *Branch) Validate() error {
- if b.Name == "" {
- return errBranchEmptyName
- }
-
- if b.Merge != "" && !b.Merge.IsBranch() {
- return errBranchInvalidMerge
- }
-
- if b.Rebase != "" &&
- b.Rebase != "true" &&
- b.Rebase != "interactive" &&
- b.Rebase != "false" {
- return errBranchInvalidRebase
- }
-
- return nil
-}
-
-func (b *Branch) marshal() *format.Subsection {
- if b.raw == nil {
- b.raw = &format.Subsection{}
- }
-
- b.raw.Name = b.Name
-
- if b.Remote == "" {
- b.raw.RemoveOption(remoteSection)
- } else {
- b.raw.SetOption(remoteSection, b.Remote)
- }
-
- if b.Merge == "" {
- b.raw.RemoveOption(mergeKey)
- } else {
- b.raw.SetOption(mergeKey, string(b.Merge))
- }
-
- if b.Rebase == "" {
- b.raw.RemoveOption(rebaseKey)
- } else {
- b.raw.SetOption(rebaseKey, b.Rebase)
- }
-
- return b.raw
-}
-
-func (b *Branch) unmarshal(s *format.Subsection) error {
- b.raw = s
-
- b.Name = b.raw.Name
- b.Remote = b.raw.Options.Get(remoteSection)
- b.Merge = plumbing.ReferenceName(b.raw.Options.Get(mergeKey))
- b.Rebase = b.raw.Options.Get(rebaseKey)
-
- return b.Validate()
-}
diff --git a/vendor/github.com/go-git/go-git/v5/config/config.go b/vendor/github.com/go-git/go-git/v5/config/config.go
deleted file mode 100644
index 1aee25a..0000000
--- a/vendor/github.com/go-git/go-git/v5/config/config.go
+++ /dev/null
@@ -1,659 +0,0 @@
-// Package config contains the abstraction of multiple config files
-package config
-
-import (
- "bytes"
- "errors"
- "fmt"
- "io"
- "io/ioutil"
- "os"
- "path/filepath"
- "sort"
- "strconv"
-
- "github.com/go-git/go-billy/v5/osfs"
- "github.com/go-git/go-git/v5/internal/url"
- format "github.com/go-git/go-git/v5/plumbing/format/config"
- "github.com/mitchellh/go-homedir"
-)
-
-const (
- // DefaultFetchRefSpec is the default refspec used for fetch.
- DefaultFetchRefSpec = "+refs/heads/*:refs/remotes/%s/*"
- // DefaultPushRefSpec is the default refspec used for push.
- DefaultPushRefSpec = "refs/heads/*:refs/heads/*"
-)
-
-// ConfigStorer generic storage of Config object
-type ConfigStorer interface {
- Config() (*Config, error)
- SetConfig(*Config) error
-}
-
-var (
- ErrInvalid = errors.New("config invalid key in remote or branch")
- ErrRemoteConfigNotFound = errors.New("remote config not found")
- ErrRemoteConfigEmptyURL = errors.New("remote config: empty URL")
- ErrRemoteConfigEmptyName = errors.New("remote config: empty name")
-)
-
-// Scope defines the scope of a config file, such as local, global or system.
-type Scope int
-
-// Available ConfigScope's
-const (
- LocalScope Scope = iota
- GlobalScope
- SystemScope
-)
-
-// Config contains the repository configuration
-// https://www.kernel.org/pub/software/scm/git/docs/git-config.html#FILES
-type Config struct {
- Core struct {
- // IsBare if true this repository is assumed to be bare and has no
- // working directory associated with it.
- IsBare bool
- // Worktree is the path to the root of the working tree.
- Worktree string
- // CommentChar is the character indicating the start of a
- // comment for commands like commit and tag
- CommentChar string
- }
-
- User struct {
- // Name is the personal name of the author and the commiter of a commit.
- Name string
- // Email is the email of the author and the commiter of a commit.
- Email string
- }
-
- Author struct {
- // Name is the personal name of the author of a commit.
- Name string
- // Email is the email of the author of a commit.
- Email string
- }
-
- Committer struct {
- // Name is the personal name of the commiter of a commit.
- Name string
- // Email is the email of the the commiter of a commit.
- Email string
- }
-
- Pack struct {
- // Window controls the size of the sliding window for delta
- // compression. The default is 10. A value of 0 turns off
- // delta compression entirely.
- Window uint
- }
-
- Init struct {
- // DefaultBranch Allows overriding the default branch name
- // e.g. when initializing a new repository or when cloning
- // an empty repository.
- DefaultBranch string
- }
-
- // Remotes list of repository remotes, the key of the map is the name
- // of the remote, should equal to RemoteConfig.Name.
- Remotes map[string]*RemoteConfig
- // Submodules list of repository submodules, the key of the map is the name
- // of the submodule, should equal to Submodule.Name.
- Submodules map[string]*Submodule
- // Branches list of branches, the key is the branch name and should
- // equal Branch.Name
- Branches map[string]*Branch
- // URLs list of url rewrite rules, if repo url starts with URL.InsteadOf value, it will be replaced with the
- // key instead.
- URLs map[string]*URL
- // Raw contains the raw information of a config file. The main goal is
- // preserve the parsed information from the original format, to avoid
- // dropping unsupported fields.
- Raw *format.Config
-}
-
-// NewConfig returns a new empty Config.
-func NewConfig() *Config {
- config := &Config{
- Remotes: make(map[string]*RemoteConfig),
- Submodules: make(map[string]*Submodule),
- Branches: make(map[string]*Branch),
- URLs: make(map[string]*URL),
- Raw: format.New(),
- }
-
- config.Pack.Window = DefaultPackWindow
-
- return config
-}
-
-// ReadConfig reads a config file from a io.Reader.
-func ReadConfig(r io.Reader) (*Config, error) {
- b, err := ioutil.ReadAll(r)
- if err != nil {
- return nil, err
- }
-
- cfg := NewConfig()
- if err = cfg.Unmarshal(b); err != nil {
- return nil, err
- }
-
- return cfg, nil
-}
-
-// LoadConfig loads a config file from a given scope. The returned Config,
-// contains exclusively information fom the given scope. If couldn't find a
-// config file to the given scope, a empty one is returned.
-func LoadConfig(scope Scope) (*Config, error) {
- if scope == LocalScope {
- return nil, fmt.Errorf("LocalScope should be read from the a ConfigStorer.")
- }
-
- files, err := Paths(scope)
- if err != nil {
- return nil, err
- }
-
- for _, file := range files {
- f, err := osfs.Default.Open(file)
- if err != nil {
- if os.IsNotExist(err) {
- continue
- }
-
- return nil, err
- }
-
- defer f.Close()
- return ReadConfig(f)
- }
-
- return NewConfig(), nil
-}
-
-// Paths returns the config file location for a given scope.
-func Paths(scope Scope) ([]string, error) {
- var files []string
- switch scope {
- case GlobalScope:
- xdg := os.Getenv("XDG_CONFIG_HOME")
- if xdg != "" {
- files = append(files, filepath.Join(xdg, "git/config"))
- }
-
- home, err := homedir.Dir()
- if err != nil {
- return nil, err
- }
-
- files = append(files,
- filepath.Join(home, ".gitconfig"),
- filepath.Join(home, ".config/git/config"),
- )
- case SystemScope:
- files = append(files, "/etc/gitconfig")
- }
-
- return files, nil
-}
-
-// Validate validates the fields and sets the default values.
-func (c *Config) Validate() error {
- for name, r := range c.Remotes {
- if r.Name != name {
- return ErrInvalid
- }
-
- if err := r.Validate(); err != nil {
- return err
- }
- }
-
- for name, b := range c.Branches {
- if b.Name != name {
- return ErrInvalid
- }
-
- if err := b.Validate(); err != nil {
- return err
- }
- }
-
- return nil
-}
-
-const (
- remoteSection = "remote"
- submoduleSection = "submodule"
- branchSection = "branch"
- coreSection = "core"
- packSection = "pack"
- userSection = "user"
- authorSection = "author"
- committerSection = "committer"
- initSection = "init"
- urlSection = "url"
- fetchKey = "fetch"
- urlKey = "url"
- bareKey = "bare"
- worktreeKey = "worktree"
- commentCharKey = "commentChar"
- windowKey = "window"
- mergeKey = "merge"
- rebaseKey = "rebase"
- nameKey = "name"
- emailKey = "email"
- defaultBranchKey = "defaultBranch"
-
- // DefaultPackWindow holds the number of previous objects used to
- // generate deltas. The value 10 is the same used by git command.
- DefaultPackWindow = uint(10)
-)
-
-// Unmarshal parses a git-config file and stores it.
-func (c *Config) Unmarshal(b []byte) error {
- r := bytes.NewBuffer(b)
- d := format.NewDecoder(r)
-
- c.Raw = format.New()
- if err := d.Decode(c.Raw); err != nil {
- return err
- }
-
- c.unmarshalCore()
- c.unmarshalUser()
- c.unmarshalInit()
- if err := c.unmarshalPack(); err != nil {
- return err
- }
- unmarshalSubmodules(c.Raw, c.Submodules)
-
- if err := c.unmarshalBranches(); err != nil {
- return err
- }
-
- if err := c.unmarshalURLs(); err != nil {
- return err
- }
-
- return c.unmarshalRemotes()
-}
-
-func (c *Config) unmarshalCore() {
- s := c.Raw.Section(coreSection)
- if s.Options.Get(bareKey) == "true" {
- c.Core.IsBare = true
- }
-
- c.Core.Worktree = s.Options.Get(worktreeKey)
- c.Core.CommentChar = s.Options.Get(commentCharKey)
-}
-
-func (c *Config) unmarshalUser() {
- s := c.Raw.Section(userSection)
- c.User.Name = s.Options.Get(nameKey)
- c.User.Email = s.Options.Get(emailKey)
-
- s = c.Raw.Section(authorSection)
- c.Author.Name = s.Options.Get(nameKey)
- c.Author.Email = s.Options.Get(emailKey)
-
- s = c.Raw.Section(committerSection)
- c.Committer.Name = s.Options.Get(nameKey)
- c.Committer.Email = s.Options.Get(emailKey)
-}
-
-func (c *Config) unmarshalPack() error {
- s := c.Raw.Section(packSection)
- window := s.Options.Get(windowKey)
- if window == "" {
- c.Pack.Window = DefaultPackWindow
- } else {
- winUint, err := strconv.ParseUint(window, 10, 32)
- if err != nil {
- return err
- }
- c.Pack.Window = uint(winUint)
- }
- return nil
-}
-
-func (c *Config) unmarshalRemotes() error {
- s := c.Raw.Section(remoteSection)
- for _, sub := range s.Subsections {
- r := &RemoteConfig{}
- if err := r.unmarshal(sub); err != nil {
- return err
- }
-
- c.Remotes[r.Name] = r
- }
-
- // Apply insteadOf url rules
- for _, r := range c.Remotes {
- r.applyURLRules(c.URLs)
- }
-
- return nil
-}
-
-func (c *Config) unmarshalURLs() error {
- s := c.Raw.Section(urlSection)
- for _, sub := range s.Subsections {
- r := &URL{}
- if err := r.unmarshal(sub); err != nil {
- return err
- }
-
- c.URLs[r.Name] = r
- }
-
- return nil
-}
-
-func unmarshalSubmodules(fc *format.Config, submodules map[string]*Submodule) {
- s := fc.Section(submoduleSection)
- for _, sub := range s.Subsections {
- m := &Submodule{}
- m.unmarshal(sub)
-
- if m.Validate() == ErrModuleBadPath {
- continue
- }
-
- submodules[m.Name] = m
- }
-}
-
-func (c *Config) unmarshalBranches() error {
- bs := c.Raw.Section(branchSection)
- for _, sub := range bs.Subsections {
- b := &Branch{}
-
- if err := b.unmarshal(sub); err != nil {
- return err
- }
-
- c.Branches[b.Name] = b
- }
- return nil
-}
-
-func (c *Config) unmarshalInit() {
- s := c.Raw.Section(initSection)
- c.Init.DefaultBranch = s.Options.Get(defaultBranchKey)
-}
-
-// Marshal returns Config encoded as a git-config file.
-func (c *Config) Marshal() ([]byte, error) {
- c.marshalCore()
- c.marshalUser()
- c.marshalPack()
- c.marshalRemotes()
- c.marshalSubmodules()
- c.marshalBranches()
- c.marshalURLs()
- c.marshalInit()
-
- buf := bytes.NewBuffer(nil)
- if err := format.NewEncoder(buf).Encode(c.Raw); err != nil {
- return nil, err
- }
-
- return buf.Bytes(), nil
-}
-
-func (c *Config) marshalCore() {
- s := c.Raw.Section(coreSection)
- s.SetOption(bareKey, fmt.Sprintf("%t", c.Core.IsBare))
-
- if c.Core.Worktree != "" {
- s.SetOption(worktreeKey, c.Core.Worktree)
- }
-}
-
-func (c *Config) marshalUser() {
- s := c.Raw.Section(userSection)
- if c.User.Name != "" {
- s.SetOption(nameKey, c.User.Name)
- }
-
- if c.User.Email != "" {
- s.SetOption(emailKey, c.User.Email)
- }
-
- s = c.Raw.Section(authorSection)
- if c.Author.Name != "" {
- s.SetOption(nameKey, c.Author.Name)
- }
-
- if c.Author.Email != "" {
- s.SetOption(emailKey, c.Author.Email)
- }
-
- s = c.Raw.Section(committerSection)
- if c.Committer.Name != "" {
- s.SetOption(nameKey, c.Committer.Name)
- }
-
- if c.Committer.Email != "" {
- s.SetOption(emailKey, c.Committer.Email)
- }
-}
-
-func (c *Config) marshalPack() {
- s := c.Raw.Section(packSection)
- if c.Pack.Window != DefaultPackWindow {
- s.SetOption(windowKey, fmt.Sprintf("%d", c.Pack.Window))
- }
-}
-
-func (c *Config) marshalRemotes() {
- s := c.Raw.Section(remoteSection)
- newSubsections := make(format.Subsections, 0, len(c.Remotes))
- added := make(map[string]bool)
- for _, subsection := range s.Subsections {
- if remote, ok := c.Remotes[subsection.Name]; ok {
- newSubsections = append(newSubsections, remote.marshal())
- added[subsection.Name] = true
- }
- }
-
- remoteNames := make([]string, 0, len(c.Remotes))
- for name := range c.Remotes {
- remoteNames = append(remoteNames, name)
- }
-
- sort.Strings(remoteNames)
-
- for _, name := range remoteNames {
- if !added[name] {
- newSubsections = append(newSubsections, c.Remotes[name].marshal())
- }
- }
-
- s.Subsections = newSubsections
-}
-
-func (c *Config) marshalSubmodules() {
- s := c.Raw.Section(submoduleSection)
- s.Subsections = make(format.Subsections, len(c.Submodules))
-
- var i int
- for _, r := range c.Submodules {
- section := r.marshal()
- // the submodule section at config is a subset of the .gitmodule file
- // we should remove the non-valid options for the config file.
- section.RemoveOption(pathKey)
- s.Subsections[i] = section
- i++
- }
-}
-
-func (c *Config) marshalBranches() {
- s := c.Raw.Section(branchSection)
- newSubsections := make(format.Subsections, 0, len(c.Branches))
- added := make(map[string]bool)
- for _, subsection := range s.Subsections {
- if branch, ok := c.Branches[subsection.Name]; ok {
- newSubsections = append(newSubsections, branch.marshal())
- added[subsection.Name] = true
- }
- }
-
- branchNames := make([]string, 0, len(c.Branches))
- for name := range c.Branches {
- branchNames = append(branchNames, name)
- }
-
- sort.Strings(branchNames)
-
- for _, name := range branchNames {
- if !added[name] {
- newSubsections = append(newSubsections, c.Branches[name].marshal())
- }
- }
-
- s.Subsections = newSubsections
-}
-
-func (c *Config) marshalURLs() {
- s := c.Raw.Section(urlSection)
- s.Subsections = make(format.Subsections, len(c.URLs))
-
- var i int
- for _, r := range c.URLs {
- section := r.marshal()
- // the submodule section at config is a subset of the .gitmodule file
- // we should remove the non-valid options for the config file.
- s.Subsections[i] = section
- i++
- }
-}
-
-func (c *Config) marshalInit() {
- s := c.Raw.Section(initSection)
- if c.Init.DefaultBranch != "" {
- s.SetOption(defaultBranchKey, c.Init.DefaultBranch)
- }
-}
-
-// RemoteConfig contains the configuration for a given remote repository.
-type RemoteConfig struct {
- // Name of the remote
- Name string
- // URLs the URLs of a remote repository. It must be non-empty. Fetch will
- // always use the first URL, while push will use all of them.
- URLs []string
-
- // insteadOfRulesApplied have urls been modified
- insteadOfRulesApplied bool
- // originalURLs are the urls before applying insteadOf rules
- originalURLs []string
-
- // Fetch the default set of "refspec" for fetch operation
- Fetch []RefSpec
-
- // raw representation of the subsection, filled by marshal or unmarshal are
- // called
- raw *format.Subsection
-}
-
-// Validate validates the fields and sets the default values.
-func (c *RemoteConfig) Validate() error {
- if c.Name == "" {
- return ErrRemoteConfigEmptyName
- }
-
- if len(c.URLs) == 0 {
- return ErrRemoteConfigEmptyURL
- }
-
- for _, r := range c.Fetch {
- if err := r.Validate(); err != nil {
- return err
- }
- }
-
- if len(c.Fetch) == 0 {
- c.Fetch = []RefSpec{RefSpec(fmt.Sprintf(DefaultFetchRefSpec, c.Name))}
- }
-
- return nil
-}
-
-func (c *RemoteConfig) unmarshal(s *format.Subsection) error {
- c.raw = s
-
- fetch := []RefSpec{}
- for _, f := range c.raw.Options.GetAll(fetchKey) {
- rs := RefSpec(f)
- if err := rs.Validate(); err != nil {
- return err
- }
-
- fetch = append(fetch, rs)
- }
-
- c.Name = c.raw.Name
- c.URLs = append([]string(nil), c.raw.Options.GetAll(urlKey)...)
- c.Fetch = fetch
-
- return nil
-}
-
-func (c *RemoteConfig) marshal() *format.Subsection {
- if c.raw == nil {
- c.raw = &format.Subsection{}
- }
-
- c.raw.Name = c.Name
- if len(c.URLs) == 0 {
- c.raw.RemoveOption(urlKey)
- } else {
- urls := c.URLs
- if c.insteadOfRulesApplied {
- urls = c.originalURLs
- }
-
- c.raw.SetOption(urlKey, urls...)
- }
-
- if len(c.Fetch) == 0 {
- c.raw.RemoveOption(fetchKey)
- } else {
- var values []string
- for _, rs := range c.Fetch {
- values = append(values, rs.String())
- }
-
- c.raw.SetOption(fetchKey, values...)
- }
-
- return c.raw
-}
-
-func (c *RemoteConfig) IsFirstURLLocal() bool {
- return url.IsLocalEndpoint(c.URLs[0])
-}
-
-func (c *RemoteConfig) applyURLRules(urlRules map[string]*URL) {
- // save original urls
- originalURLs := make([]string, len(c.URLs))
- copy(originalURLs, c.URLs)
-
- for i, url := range c.URLs {
- if matchingURLRule := findLongestInsteadOfMatch(url, urlRules); matchingURLRule != nil {
- c.URLs[i] = matchingURLRule.ApplyInsteadOf(c.URLs[i])
- c.insteadOfRulesApplied = true
- }
- }
-
- if c.insteadOfRulesApplied {
- c.originalURLs = originalURLs
- }
-}
diff --git a/vendor/github.com/go-git/go-git/v5/config/modules.go b/vendor/github.com/go-git/go-git/v5/config/modules.go
deleted file mode 100644
index 1c10aa3..0000000
--- a/vendor/github.com/go-git/go-git/v5/config/modules.go
+++ /dev/null
@@ -1,139 +0,0 @@
-package config
-
-import (
- "bytes"
- "errors"
- "regexp"
-
- format "github.com/go-git/go-git/v5/plumbing/format/config"
-)
-
-var (
- ErrModuleEmptyURL = errors.New("module config: empty URL")
- ErrModuleEmptyPath = errors.New("module config: empty path")
- ErrModuleBadPath = errors.New("submodule has an invalid path")
-)
-
-var (
- // Matches module paths with dotdot ".." components.
- dotdotPath = regexp.MustCompile(`(^|[/\\])\.\.([/\\]|$)`)
-)
-
-// Modules defines the submodules properties, represents a .gitmodules file
-// https://www.kernel.org/pub/software/scm/git/docs/gitmodules.html
-type Modules struct {
- // Submodules is a map of submodules being the key the name of the submodule.
- Submodules map[string]*Submodule
-
- raw *format.Config
-}
-
-// NewModules returns a new empty Modules
-func NewModules() *Modules {
- return &Modules{
- Submodules: make(map[string]*Submodule),
- raw: format.New(),
- }
-}
-
-const (
- pathKey = "path"
- branchKey = "branch"
-)
-
-// Unmarshal parses a git-config file and stores it.
-func (m *Modules) Unmarshal(b []byte) error {
- r := bytes.NewBuffer(b)
- d := format.NewDecoder(r)
-
- m.raw = format.New()
- if err := d.Decode(m.raw); err != nil {
- return err
- }
-
- unmarshalSubmodules(m.raw, m.Submodules)
- return nil
-}
-
-// Marshal returns Modules encoded as a git-config file.
-func (m *Modules) Marshal() ([]byte, error) {
- s := m.raw.Section(submoduleSection)
- s.Subsections = make(format.Subsections, len(m.Submodules))
-
- var i int
- for _, r := range m.Submodules {
- s.Subsections[i] = r.marshal()
- i++
- }
-
- buf := bytes.NewBuffer(nil)
- if err := format.NewEncoder(buf).Encode(m.raw); err != nil {
- return nil, err
- }
-
- return buf.Bytes(), nil
-}
-
-// Submodule defines a submodule.
-type Submodule struct {
- // Name module name
- Name string
- // Path defines the path, relative to the top-level directory of the Git
- // working tree.
- Path string
- // URL defines a URL from which the submodule repository can be cloned.
- URL string
- // Branch is a remote branch name for tracking updates in the upstream
- // submodule. Optional value.
- Branch string
-
- // raw representation of the subsection, filled by marshal or unmarshal are
- // called.
- raw *format.Subsection
-}
-
-// Validate validates the fields and sets the default values.
-func (m *Submodule) Validate() error {
- if m.Path == "" {
- return ErrModuleEmptyPath
- }
-
- if m.URL == "" {
- return ErrModuleEmptyURL
- }
-
- if dotdotPath.MatchString(m.Path) {
- return ErrModuleBadPath
- }
-
- return nil
-}
-
-func (m *Submodule) unmarshal(s *format.Subsection) {
- m.raw = s
-
- m.Name = m.raw.Name
- m.Path = m.raw.Option(pathKey)
- m.URL = m.raw.Option(urlKey)
- m.Branch = m.raw.Option(branchKey)
-}
-
-func (m *Submodule) marshal() *format.Subsection {
- if m.raw == nil {
- m.raw = &format.Subsection{}
- }
-
- m.raw.Name = m.Name
- if m.raw.Name == "" {
- m.raw.Name = m.Path
- }
-
- m.raw.SetOption(pathKey, m.Path)
- m.raw.SetOption(urlKey, m.URL)
-
- if m.Branch != "" {
- m.raw.SetOption(branchKey, m.Branch)
- }
-
- return m.raw
-}
diff --git a/vendor/github.com/go-git/go-git/v5/config/refspec.go b/vendor/github.com/go-git/go-git/v5/config/refspec.go
deleted file mode 100644
index 4bfaa37..0000000
--- a/vendor/github.com/go-git/go-git/v5/config/refspec.go
+++ /dev/null
@@ -1,155 +0,0 @@
-package config
-
-import (
- "errors"
- "strings"
-
- "github.com/go-git/go-git/v5/plumbing"
-)
-
-const (
- refSpecWildcard = "*"
- refSpecForce = "+"
- refSpecSeparator = ":"
-)
-
-var (
- ErrRefSpecMalformedSeparator = errors.New("malformed refspec, separators are wrong")
- ErrRefSpecMalformedWildcard = errors.New("malformed refspec, mismatched number of wildcards")
-)
-
-// RefSpec is a mapping from local branches to remote references.
-// The format of the refspec is an optional +, followed by