mirror of
https://github.com/drwetter/testssl.sh.git
synced 2025-01-01 06:19:44 +01:00
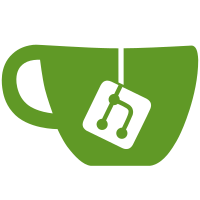
Travis updated the container images so that the perl reference to 5.18 was outdated. We use now 5.26 which works, however we should consider to be more flexible. JSON::Validator didn't compile in the container. Thus we switched to just use 'JSON'. That also supports JSON pretty. For the future we should just test for valid JSON in all unit test files as it is more effective.
84 lines
2.2 KiB
Perl
Executable File
84 lines
2.2 KiB
Perl
Executable File
#!/usr/bin/env perl
|
|
|
|
# This is more a PoC. Improvements welcome!
|
|
#
|
|
|
|
use strict;
|
|
use Test::More;
|
|
use JSON;
|
|
|
|
my $tests = 0;
|
|
my $prg="./testssl.sh";
|
|
my $check2run ="--ip=one -q -p --color 0";
|
|
my $uri="";
|
|
my $json="";
|
|
my $out="";
|
|
# Blacklists we use to trigger an error:
|
|
my $socket_regex_bl='(e|E)rror|\.\/testssl\.sh: line |(f|F)atal';
|
|
my $openssl_regex_bl='(e|E)rror|(f|F)atal|\.\/testssl\.sh: line |Oops|s_client connect problem';
|
|
|
|
die "Unable to open $prg" unless -f $prg;
|
|
|
|
my $uri="cloudflare.com";
|
|
|
|
#1
|
|
printf "\n%s\n", "Unit testing plain JSON output --> $uri ...";
|
|
$out = `./testssl.sh $check2run --jsonfile tmp.json $uri`;
|
|
$json = json('tmp.json');
|
|
unlink 'tmp.json';
|
|
my @errors=eval { decode_json($json) };
|
|
is(@errors,0,"no errors");
|
|
$tests++;
|
|
|
|
#2
|
|
printf "\n%s\n", "Unit testing pretty JSON output --> $uri ...";
|
|
$out = `./testssl.sh $check2run --jsonfile-pretty tmp.json $uri`;
|
|
$json = json('tmp.json');
|
|
unlink 'tmp.json';
|
|
@errors=eval { decode_json($json) };
|
|
is(@errors,0,"no errors");
|
|
$tests++;
|
|
|
|
|
|
#3
|
|
# This testss.sh run deliberately does NOT work as travis-ci.org blocks port 25 egress.
|
|
# but the output should be fine. The idea is to have a unit test for a failed connection.
|
|
printf "\n%s\n", "Checking plain JSON output for a failed run '--mx $uri' ...";
|
|
$out = `./testssl.sh --ssl-native --openssl-timeout=10 $check2run --jsonfile tmp.json --mx $uri`;
|
|
$json = json('tmp.json');
|
|
unlink 'tmp.json';
|
|
@errors=eval { decode_json($json) };
|
|
is(@errors,0,"no errors");
|
|
$tests++;
|
|
|
|
#4
|
|
# Same as above but with pretty JSON
|
|
printf "\n%s\n", "Checking pretty JSON output for a failed run '--mx $uri' ...";
|
|
$out = `./testssl.sh --ssl-native --openssl-timeout=10 $check2run --jsonfile-pretty tmp.json --mx $uri`;
|
|
$json = json('tmp.json');
|
|
unlink 'tmp.json';
|
|
@errors=eval { decode_json($json) };
|
|
is(@errors,0,"no errors");
|
|
$tests++;
|
|
|
|
#5
|
|
my $uri = "smtp-relay.gmail.com:587";
|
|
printf "\n%s\n", " Unit testing plain JSON output --> $uri ...";
|
|
$out = `./testssl.sh --jsonfile tmp.json $check2run -t smtp $uri`;
|
|
$json = json('tmp.json');
|
|
unlink 'tmp.json';
|
|
@errors=eval { decode_json($json) };
|
|
is(@errors,0,"no errors");
|
|
$tests++;
|
|
|
|
|
|
done_testing($tests);
|
|
|
|
sub json($) {
|
|
my $file = shift;
|
|
$file = `cat $file`;
|
|
return from_json($file);
|
|
}
|
|
|
|
|